Object-Oriented Applications: Polymorphism, Inheritance, Encapsulation
VerifiedAdded on 2019/09/20
|11
|1647
|313
Homework Assignment
AI Summary
This document provides a comprehensive overview of object-oriented programming (OOP) concepts. It begins by defining object orientation and its core principles, including classes, objects, and the mapping of real-world entities to programming structures. The document then delves into the four key principles of OOP: encapsulation, polymorphism, inheritance, and abstraction. Polymorphism is explained in detail, differentiating between static (compile-time) and dynamic (runtime) polymorphism, with examples of method overloading and overriding. Encapsulation is discussed, emphasizing data binding and hiding, along with its advantages. Inheritance is presented as a powerful tool for code reuse and maintenance, with explanations of single, multilevel, hierarchical, and hybrid inheritance. Finally, the document references relevant literature supporting the discussed OOP concepts.
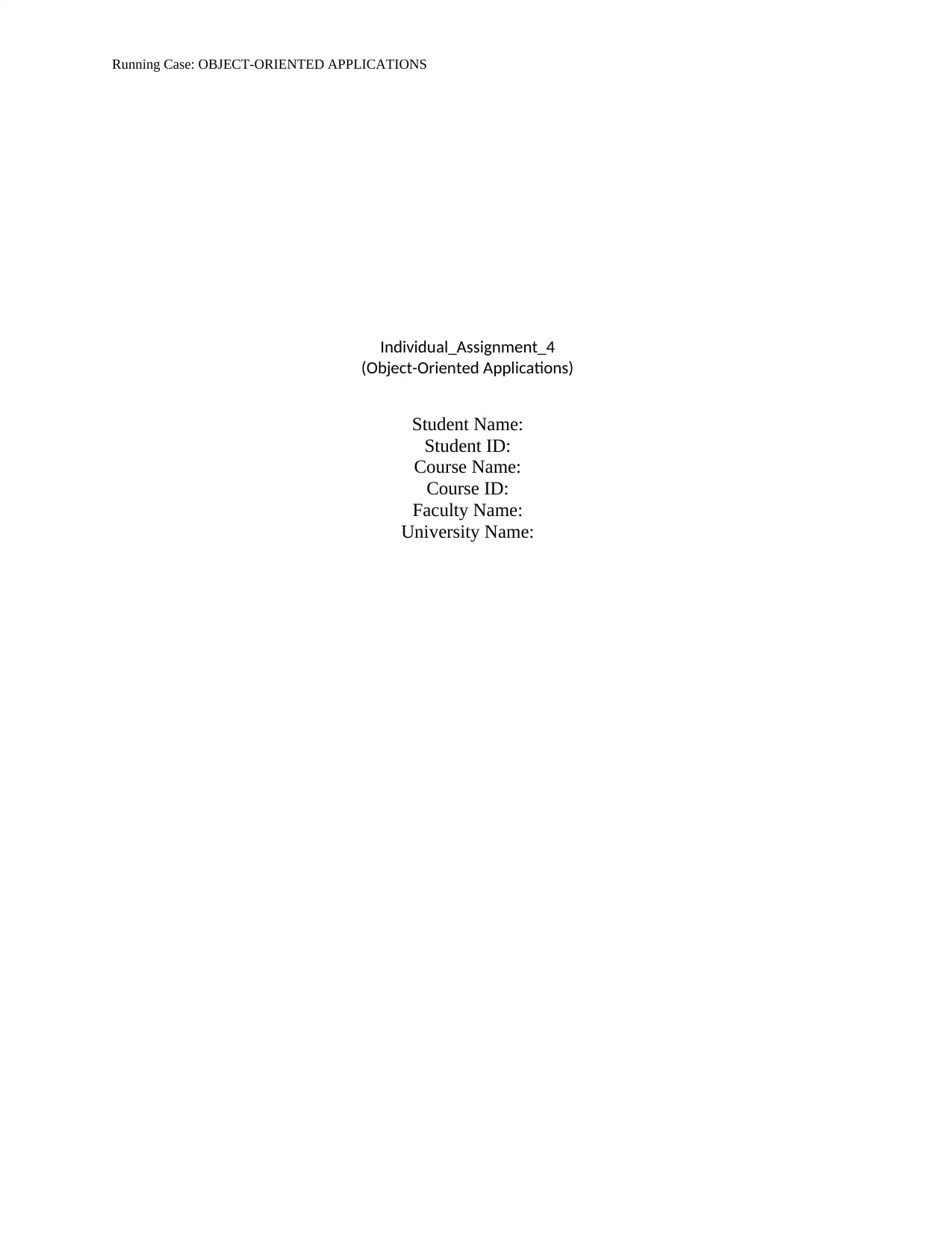
Running Case: OBJECT-ORIENTED APPLICATIONS
Individual_Assignment_4
(Object-Oriented Applications)
Student Name:
Student ID:
Course Name:
Course ID:
Faculty Name:
University Name:
Individual_Assignment_4
(Object-Oriented Applications)
Student Name:
Student ID:
Course Name:
Course ID:
Faculty Name:
University Name:
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
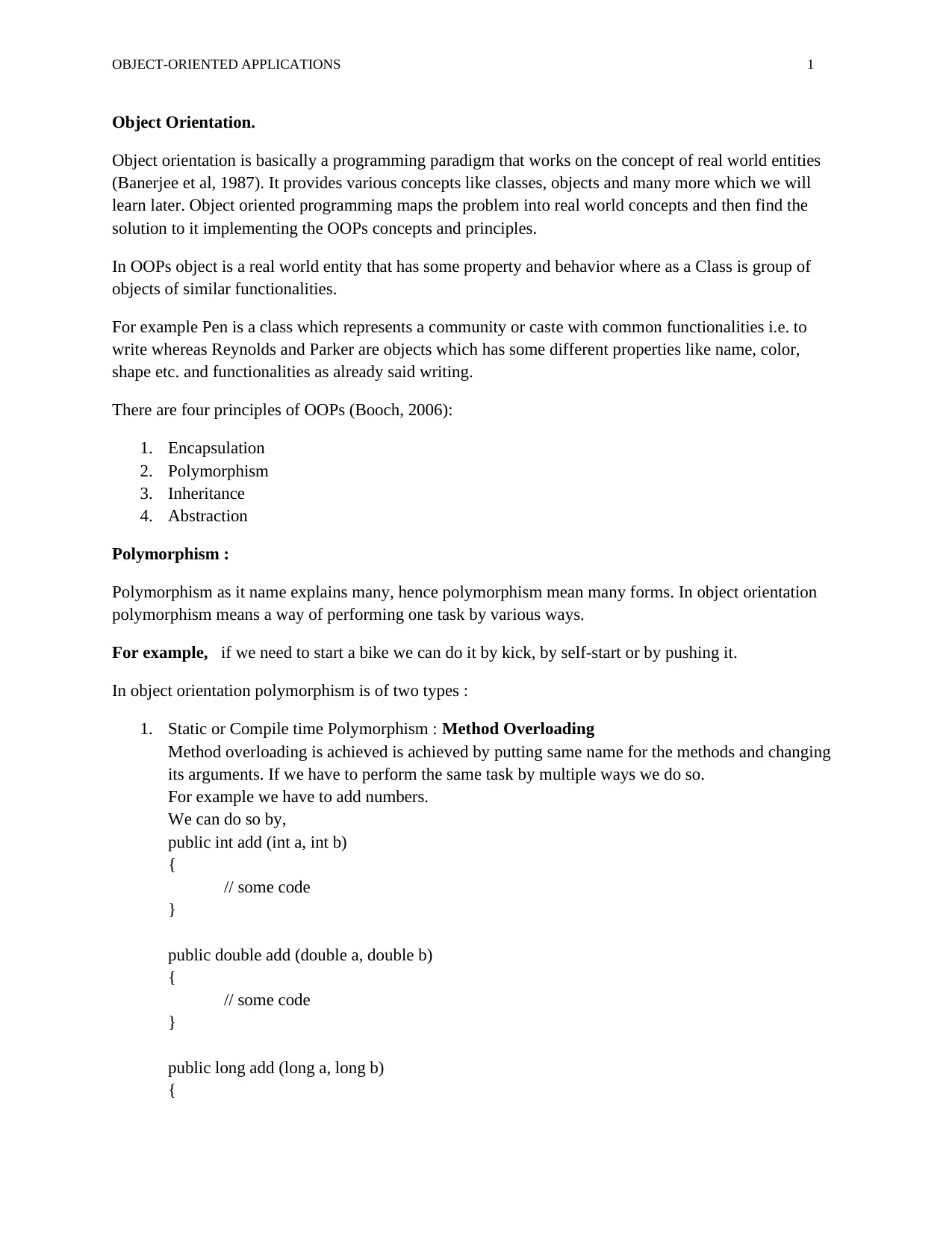
OBJECT-ORIENTED APPLICATIONS 1
Object Orientation.
Object orientation is basically a programming paradigm that works on the concept of real world entities
(Banerjee et al, 1987). It provides various concepts like classes, objects and many more which we will
learn later. Object oriented programming maps the problem into real world concepts and then find the
solution to it implementing the OOPs concepts and principles.
In OOPs object is a real world entity that has some property and behavior where as a Class is group of
objects of similar functionalities.
For example Pen is a class which represents a community or caste with common functionalities i.e. to
write whereas Reynolds and Parker are objects which has some different properties like name, color,
shape etc. and functionalities as already said writing.
There are four principles of OOPs (Booch, 2006):
1. Encapsulation
2. Polymorphism
3. Inheritance
4. Abstraction
Polymorphism :
Polymorphism as it name explains many, hence polymorphism mean many forms. In object orientation
polymorphism means a way of performing one task by various ways.
For example, if we need to start a bike we can do it by kick, by self-start or by pushing it.
In object orientation polymorphism is of two types :
1. Static or Compile time Polymorphism : Method Overloading
Method overloading is achieved is achieved by putting same name for the methods and changing
its arguments. If we have to perform the same task by multiple ways we do so.
For example we have to add numbers.
We can do so by,
public int add (int a, int b)
{
// some code
}
public double add (double a, double b)
{
// some code
}
public long add (long a, long b)
{
Object Orientation.
Object orientation is basically a programming paradigm that works on the concept of real world entities
(Banerjee et al, 1987). It provides various concepts like classes, objects and many more which we will
learn later. Object oriented programming maps the problem into real world concepts and then find the
solution to it implementing the OOPs concepts and principles.
In OOPs object is a real world entity that has some property and behavior where as a Class is group of
objects of similar functionalities.
For example Pen is a class which represents a community or caste with common functionalities i.e. to
write whereas Reynolds and Parker are objects which has some different properties like name, color,
shape etc. and functionalities as already said writing.
There are four principles of OOPs (Booch, 2006):
1. Encapsulation
2. Polymorphism
3. Inheritance
4. Abstraction
Polymorphism :
Polymorphism as it name explains many, hence polymorphism mean many forms. In object orientation
polymorphism means a way of performing one task by various ways.
For example, if we need to start a bike we can do it by kick, by self-start or by pushing it.
In object orientation polymorphism is of two types :
1. Static or Compile time Polymorphism : Method Overloading
Method overloading is achieved is achieved by putting same name for the methods and changing
its arguments. If we have to perform the same task by multiple ways we do so.
For example we have to add numbers.
We can do so by,
public int add (int a, int b)
{
// some code
}
public double add (double a, double b)
{
// some code
}
public long add (long a, long b)
{
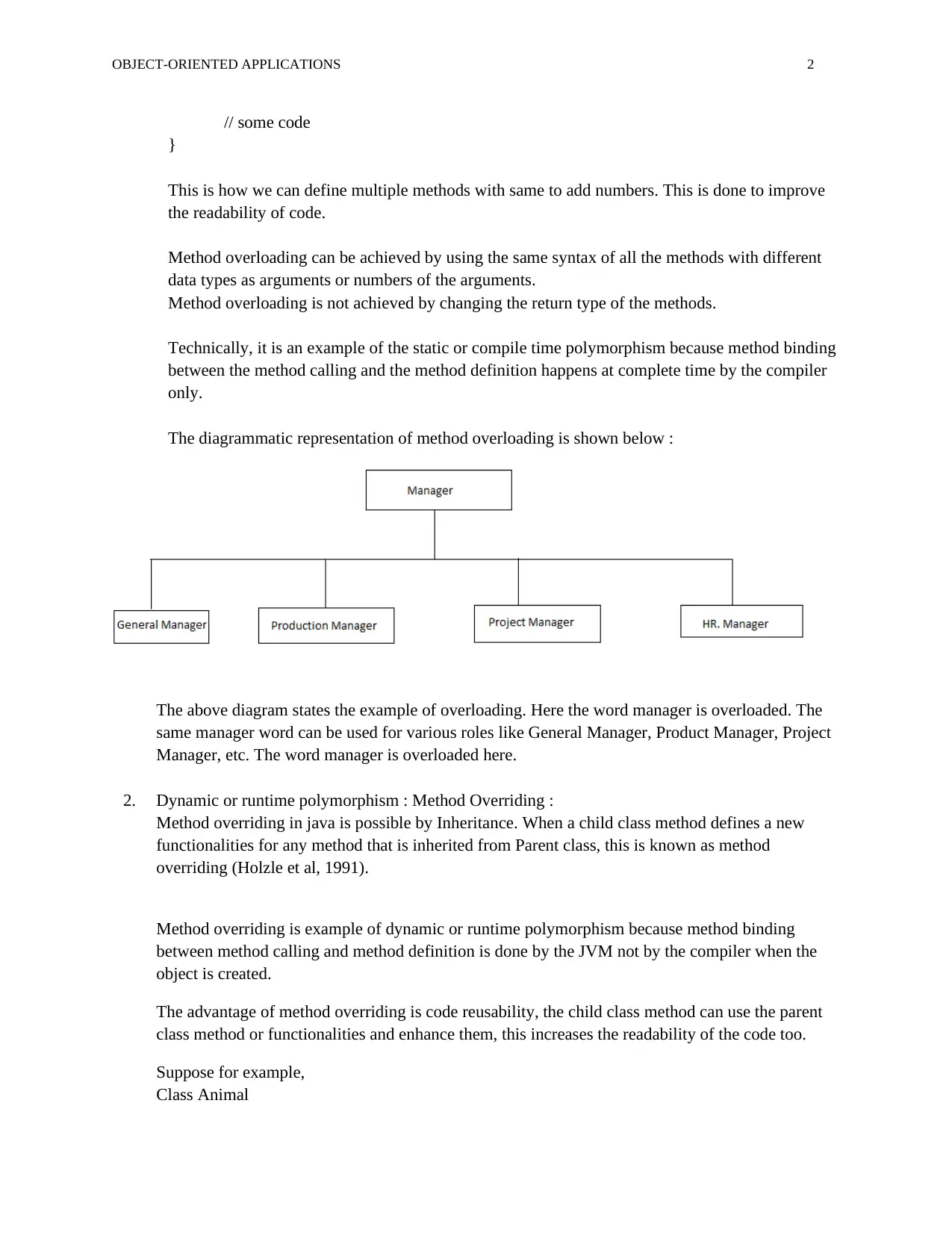
OBJECT-ORIENTED APPLICATIONS 2
// some code
}
This is how we can define multiple methods with same to add numbers. This is done to improve
the readability of code.
Method overloading can be achieved by using the same syntax of all the methods with different
data types as arguments or numbers of the arguments.
Method overloading is not achieved by changing the return type of the methods.
Technically, it is an example of the static or compile time polymorphism because method binding
between the method calling and the method definition happens at complete time by the compiler
only.
The diagrammatic representation of method overloading is shown below :
The above diagram states the example of overloading. Here the word manager is overloaded. The
same manager word can be used for various roles like General Manager, Product Manager, Project
Manager, etc. The word manager is overloaded here.
2. Dynamic or runtime polymorphism : Method Overriding :
Method overriding in java is possible by Inheritance. When a child class method defines a new
functionalities for any method that is inherited from Parent class, this is known as method
overriding (Holzle et al, 1991).
Method overriding is example of dynamic or runtime polymorphism because method binding
between method calling and method definition is done by the JVM not by the compiler when the
object is created.
The advantage of method overriding is code reusability, the child class method can use the parent
class method or functionalities and enhance them, this increases the readability of the code too.
Suppose for example,
Class Animal
// some code
}
This is how we can define multiple methods with same to add numbers. This is done to improve
the readability of code.
Method overloading can be achieved by using the same syntax of all the methods with different
data types as arguments or numbers of the arguments.
Method overloading is not achieved by changing the return type of the methods.
Technically, it is an example of the static or compile time polymorphism because method binding
between the method calling and the method definition happens at complete time by the compiler
only.
The diagrammatic representation of method overloading is shown below :
The above diagram states the example of overloading. Here the word manager is overloaded. The
same manager word can be used for various roles like General Manager, Product Manager, Project
Manager, etc. The word manager is overloaded here.
2. Dynamic or runtime polymorphism : Method Overriding :
Method overriding in java is possible by Inheritance. When a child class method defines a new
functionalities for any method that is inherited from Parent class, this is known as method
overriding (Holzle et al, 1991).
Method overriding is example of dynamic or runtime polymorphism because method binding
between method calling and method definition is done by the JVM not by the compiler when the
object is created.
The advantage of method overriding is code reusability, the child class method can use the parent
class method or functionalities and enhance them, this increases the readability of the code too.
Suppose for example,
Class Animal
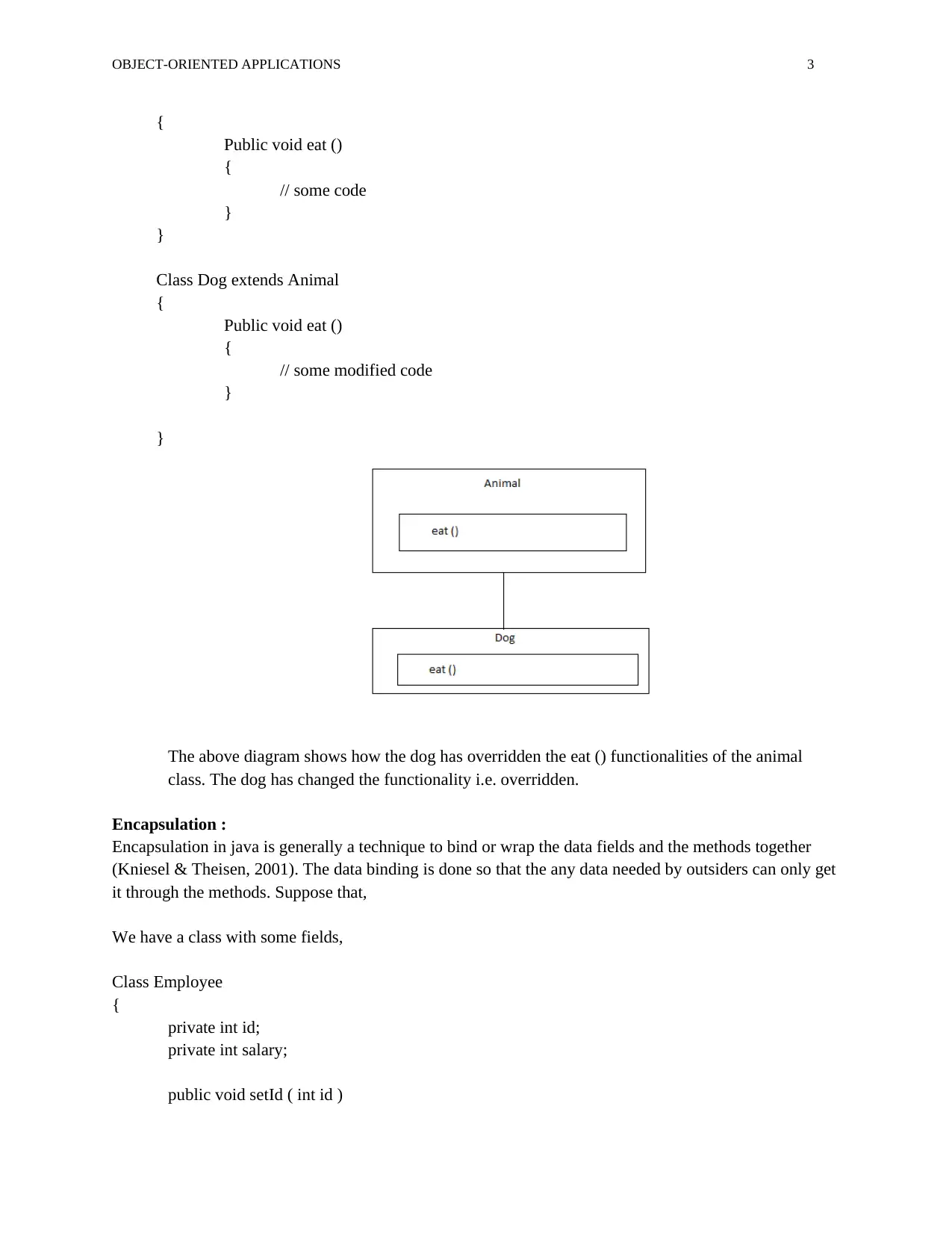
OBJECT-ORIENTED APPLICATIONS 3
{
Public void eat ()
{
// some code
}
}
Class Dog extends Animal
{
Public void eat ()
{
// some modified code
}
}
The above diagram shows how the dog has overridden the eat () functionalities of the animal
class. The dog has changed the functionality i.e. overridden.
Encapsulation :
Encapsulation in java is generally a technique to bind or wrap the data fields and the methods together
(Kniesel & Theisen, 2001). The data binding is done so that the any data needed by outsiders can only get
it through the methods. Suppose that,
We have a class with some fields,
Class Employee
{
private int id;
private int salary;
public void setId ( int id )
{
Public void eat ()
{
// some code
}
}
Class Dog extends Animal
{
Public void eat ()
{
// some modified code
}
}
The above diagram shows how the dog has overridden the eat () functionalities of the animal
class. The dog has changed the functionality i.e. overridden.
Encapsulation :
Encapsulation in java is generally a technique to bind or wrap the data fields and the methods together
(Kniesel & Theisen, 2001). The data binding is done so that the any data needed by outsiders can only get
it through the methods. Suppose that,
We have a class with some fields,
Class Employee
{
private int id;
private int salary;
public void setId ( int id )
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
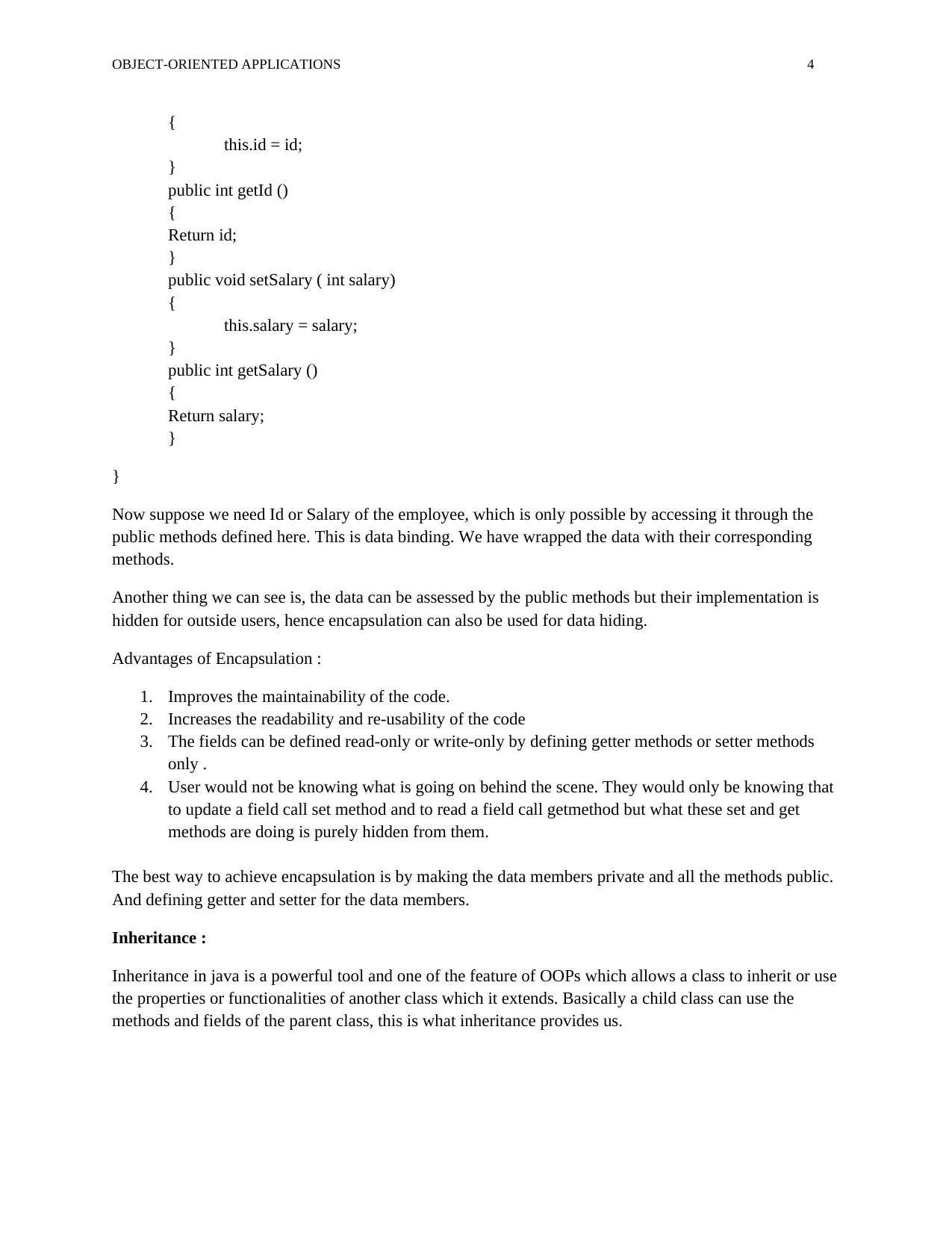
OBJECT-ORIENTED APPLICATIONS 4
{
this.id = id;
}
public int getId ()
{
Return id;
}
public void setSalary ( int salary)
{
this.salary = salary;
}
public int getSalary ()
{
Return salary;
}
}
Now suppose we need Id or Salary of the employee, which is only possible by accessing it through the
public methods defined here. This is data binding. We have wrapped the data with their corresponding
methods.
Another thing we can see is, the data can be assessed by the public methods but their implementation is
hidden for outside users, hence encapsulation can also be used for data hiding.
Advantages of Encapsulation :
1. Improves the maintainability of the code.
2. Increases the readability and re-usability of the code
3. The fields can be defined read-only or write-only by defining getter methods or setter methods
only .
4. User would not be knowing what is going on behind the scene. They would only be knowing that
to update a field call set method and to read a field call getmethod but what these set and get
methods are doing is purely hidden from them.
The best way to achieve encapsulation is by making the data members private and all the methods public.
And defining getter and setter for the data members.
Inheritance :
Inheritance in java is a powerful tool and one of the feature of OOPs which allows a class to inherit or use
the properties or functionalities of another class which it extends. Basically a child class can use the
methods and fields of the parent class, this is what inheritance provides us.
{
this.id = id;
}
public int getId ()
{
Return id;
}
public void setSalary ( int salary)
{
this.salary = salary;
}
public int getSalary ()
{
Return salary;
}
}
Now suppose we need Id or Salary of the employee, which is only possible by accessing it through the
public methods defined here. This is data binding. We have wrapped the data with their corresponding
methods.
Another thing we can see is, the data can be assessed by the public methods but their implementation is
hidden for outside users, hence encapsulation can also be used for data hiding.
Advantages of Encapsulation :
1. Improves the maintainability of the code.
2. Increases the readability and re-usability of the code
3. The fields can be defined read-only or write-only by defining getter methods or setter methods
only .
4. User would not be knowing what is going on behind the scene. They would only be knowing that
to update a field call set method and to read a field call getmethod but what these set and get
methods are doing is purely hidden from them.
The best way to achieve encapsulation is by making the data members private and all the methods public.
And defining getter and setter for the data members.
Inheritance :
Inheritance in java is a powerful tool and one of the feature of OOPs which allows a class to inherit or use
the properties or functionalities of another class which it extends. Basically a child class can use the
methods and fields of the parent class, this is what inheritance provides us.
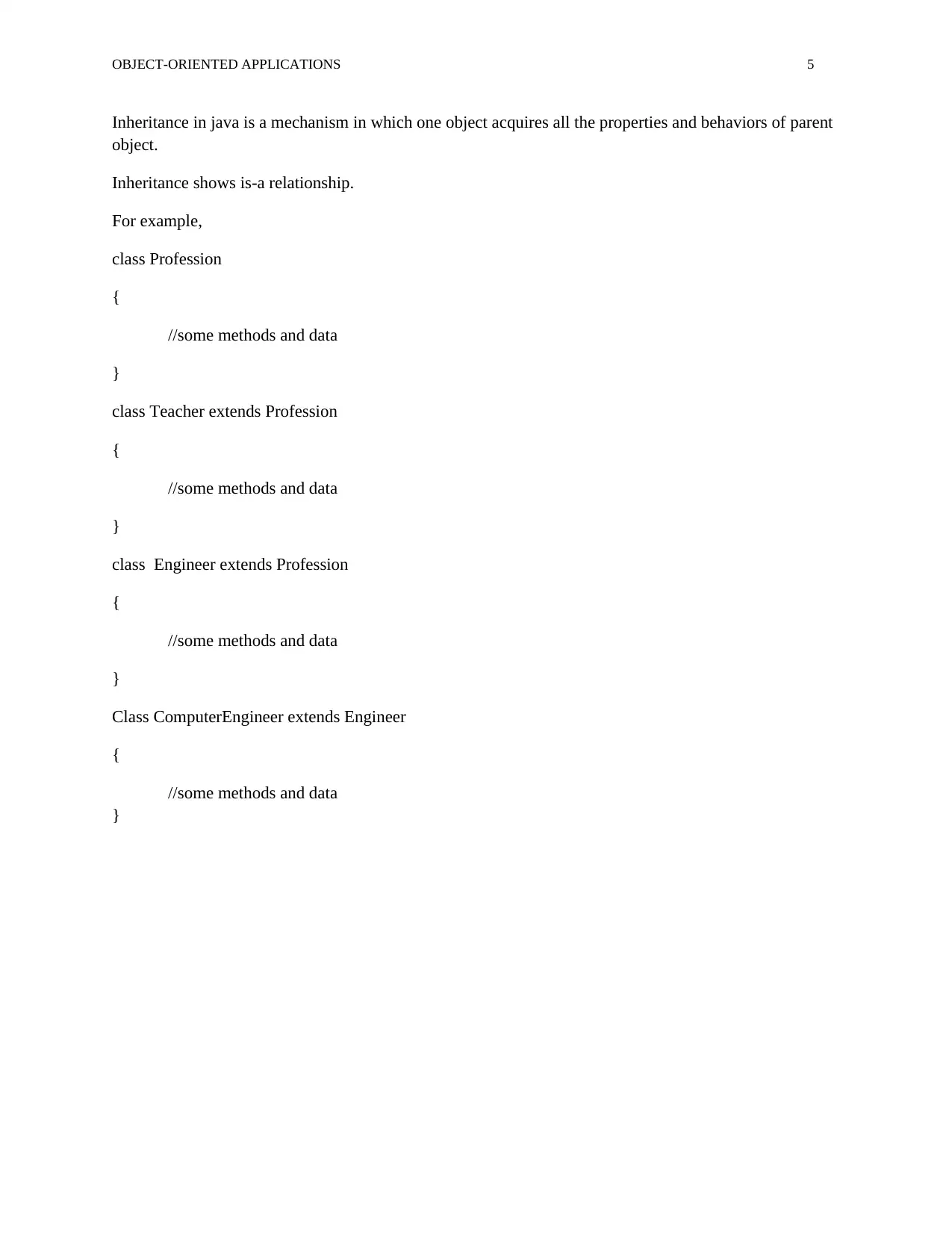
OBJECT-ORIENTED APPLICATIONS 5
Inheritance in java is a mechanism in which one object acquires all the properties and behaviors of parent
object.
Inheritance shows is-a relationship.
For example,
class Profession
{
//some methods and data
}
class Teacher extends Profession
{
//some methods and data
}
class Engineer extends Profession
{
//some methods and data
}
Class ComputerEngineer extends Engineer
{
//some methods and data
}
Inheritance in java is a mechanism in which one object acquires all the properties and behaviors of parent
object.
Inheritance shows is-a relationship.
For example,
class Profession
{
//some methods and data
}
class Teacher extends Profession
{
//some methods and data
}
class Engineer extends Profession
{
//some methods and data
}
Class ComputerEngineer extends Engineer
{
//some methods and data
}
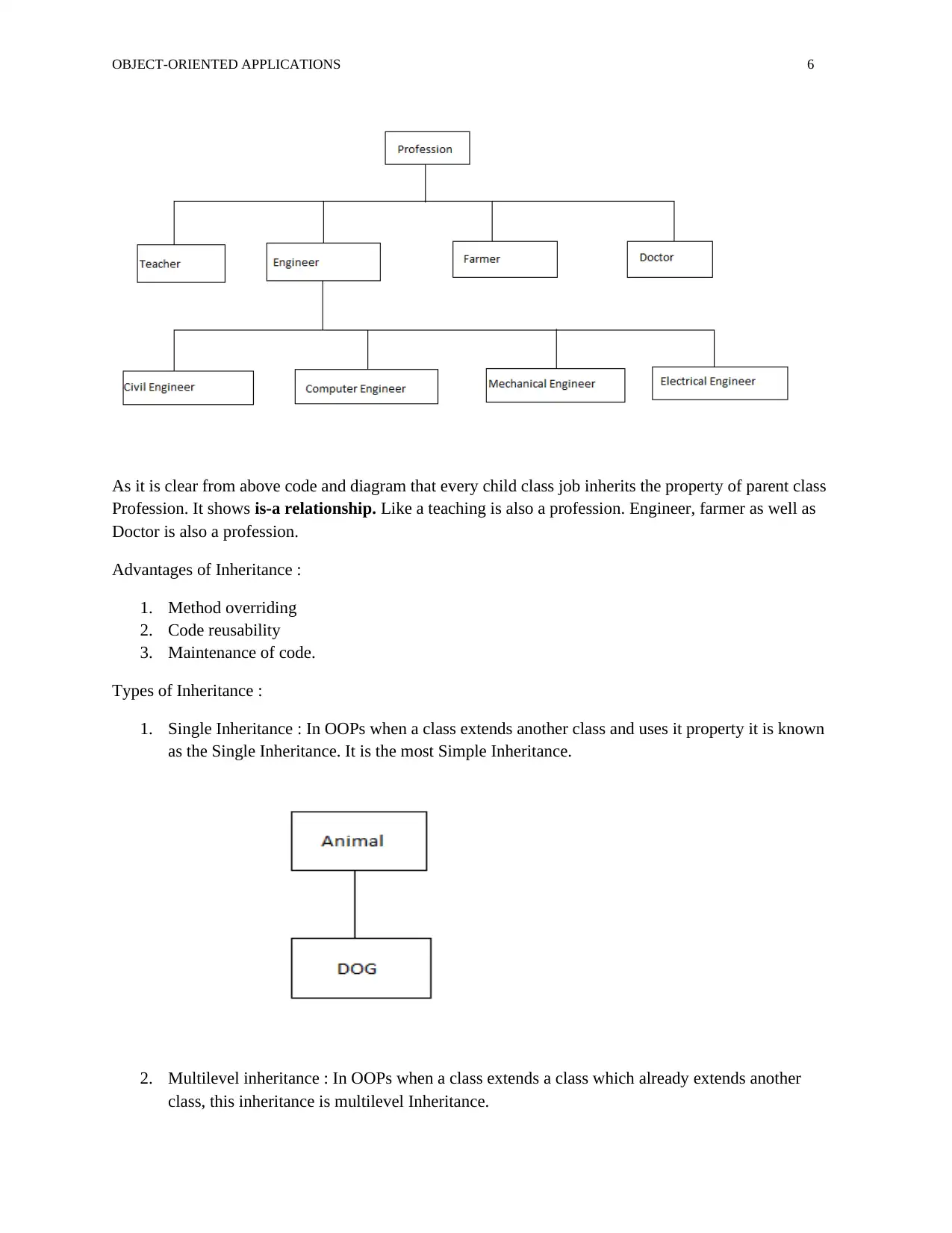
OBJECT-ORIENTED APPLICATIONS 6
As it is clear from above code and diagram that every child class job inherits the property of parent class
Profession. It shows is-a relationship. Like a teaching is also a profession. Engineer, farmer as well as
Doctor is also a profession.
Advantages of Inheritance :
1. Method overriding
2. Code reusability
3. Maintenance of code.
Types of Inheritance :
1. Single Inheritance : In OOPs when a class extends another class and uses it property it is known
as the Single Inheritance. It is the most Simple Inheritance.
2. Multilevel inheritance : In OOPs when a class extends a class which already extends another
class, this inheritance is multilevel Inheritance.
As it is clear from above code and diagram that every child class job inherits the property of parent class
Profession. It shows is-a relationship. Like a teaching is also a profession. Engineer, farmer as well as
Doctor is also a profession.
Advantages of Inheritance :
1. Method overriding
2. Code reusability
3. Maintenance of code.
Types of Inheritance :
1. Single Inheritance : In OOPs when a class extends another class and uses it property it is known
as the Single Inheritance. It is the most Simple Inheritance.
2. Multilevel inheritance : In OOPs when a class extends a class which already extends another
class, this inheritance is multilevel Inheritance.
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
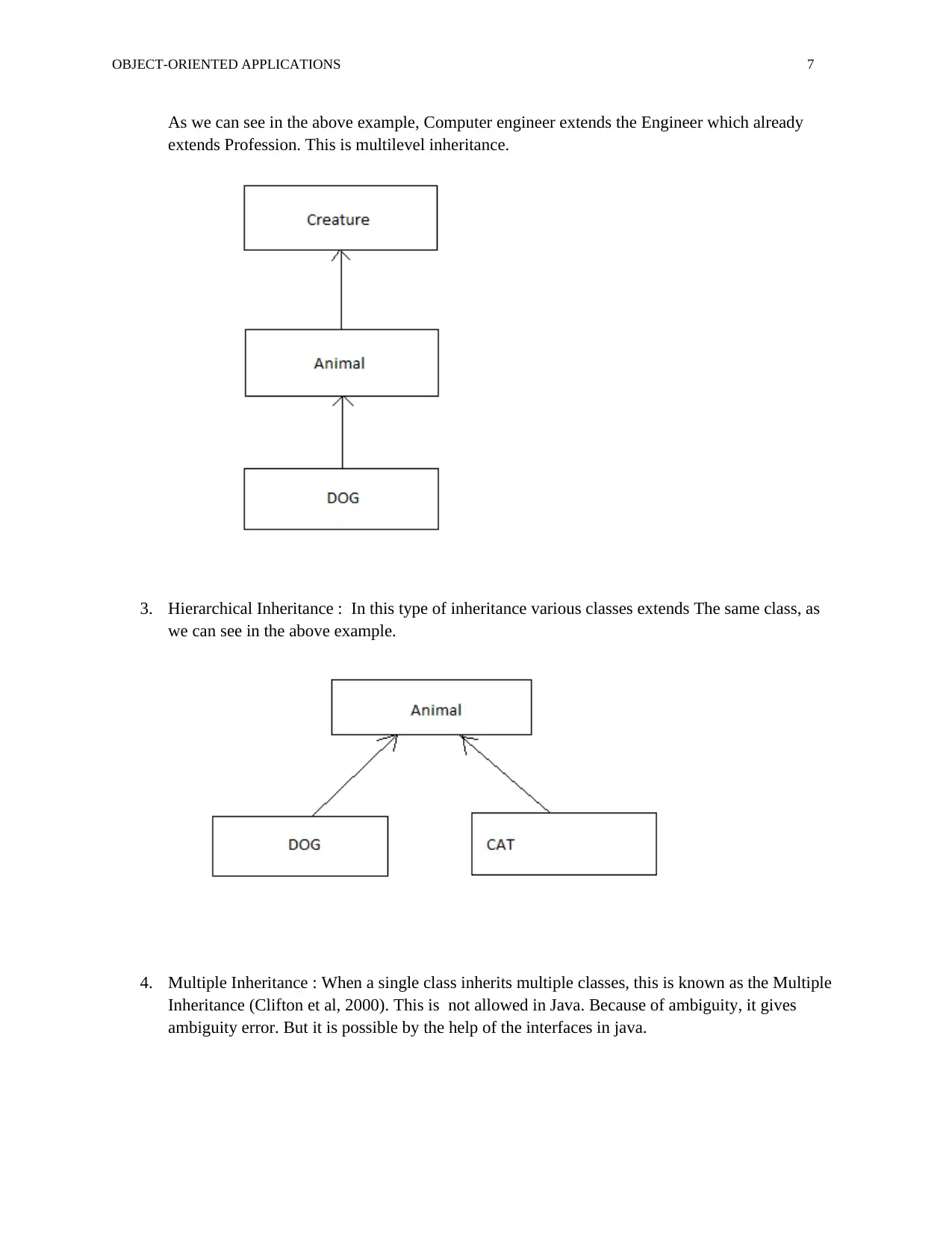
OBJECT-ORIENTED APPLICATIONS 7
As we can see in the above example, Computer engineer extends the Engineer which already
extends Profession. This is multilevel inheritance.
3. Hierarchical Inheritance : In this type of inheritance various classes extends The same class, as
we can see in the above example.
4. Multiple Inheritance : When a single class inherits multiple classes, this is known as the Multiple
Inheritance (Clifton et al, 2000). This is not allowed in Java. Because of ambiguity, it gives
ambiguity error. But it is possible by the help of the interfaces in java.
As we can see in the above example, Computer engineer extends the Engineer which already
extends Profession. This is multilevel inheritance.
3. Hierarchical Inheritance : In this type of inheritance various classes extends The same class, as
we can see in the above example.
4. Multiple Inheritance : When a single class inherits multiple classes, this is known as the Multiple
Inheritance (Clifton et al, 2000). This is not allowed in Java. Because of ambiguity, it gives
ambiguity error. But it is possible by the help of the interfaces in java.
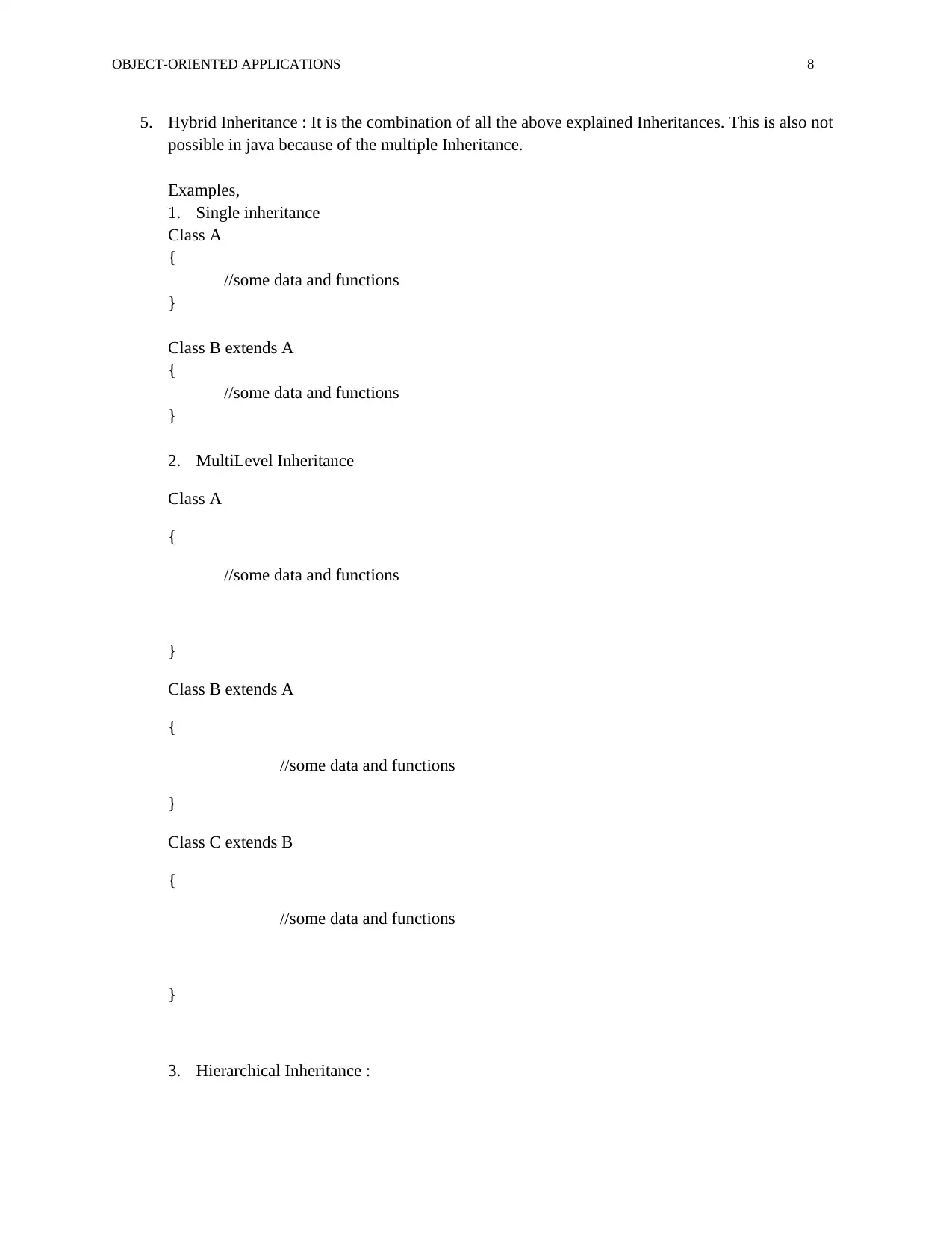
OBJECT-ORIENTED APPLICATIONS 8
5. Hybrid Inheritance : It is the combination of all the above explained Inheritances. This is also not
possible in java because of the multiple Inheritance.
Examples,
1. Single inheritance
Class A
{
//some data and functions
}
Class B extends A
{
//some data and functions
}
2. MultiLevel Inheritance
Class A
{
//some data and functions
}
Class B extends A
{
//some data and functions
}
Class C extends B
{
//some data and functions
}
3. Hierarchical Inheritance :
5. Hybrid Inheritance : It is the combination of all the above explained Inheritances. This is also not
possible in java because of the multiple Inheritance.
Examples,
1. Single inheritance
Class A
{
//some data and functions
}
Class B extends A
{
//some data and functions
}
2. MultiLevel Inheritance
Class A
{
//some data and functions
}
Class B extends A
{
//some data and functions
}
Class C extends B
{
//some data and functions
}
3. Hierarchical Inheritance :
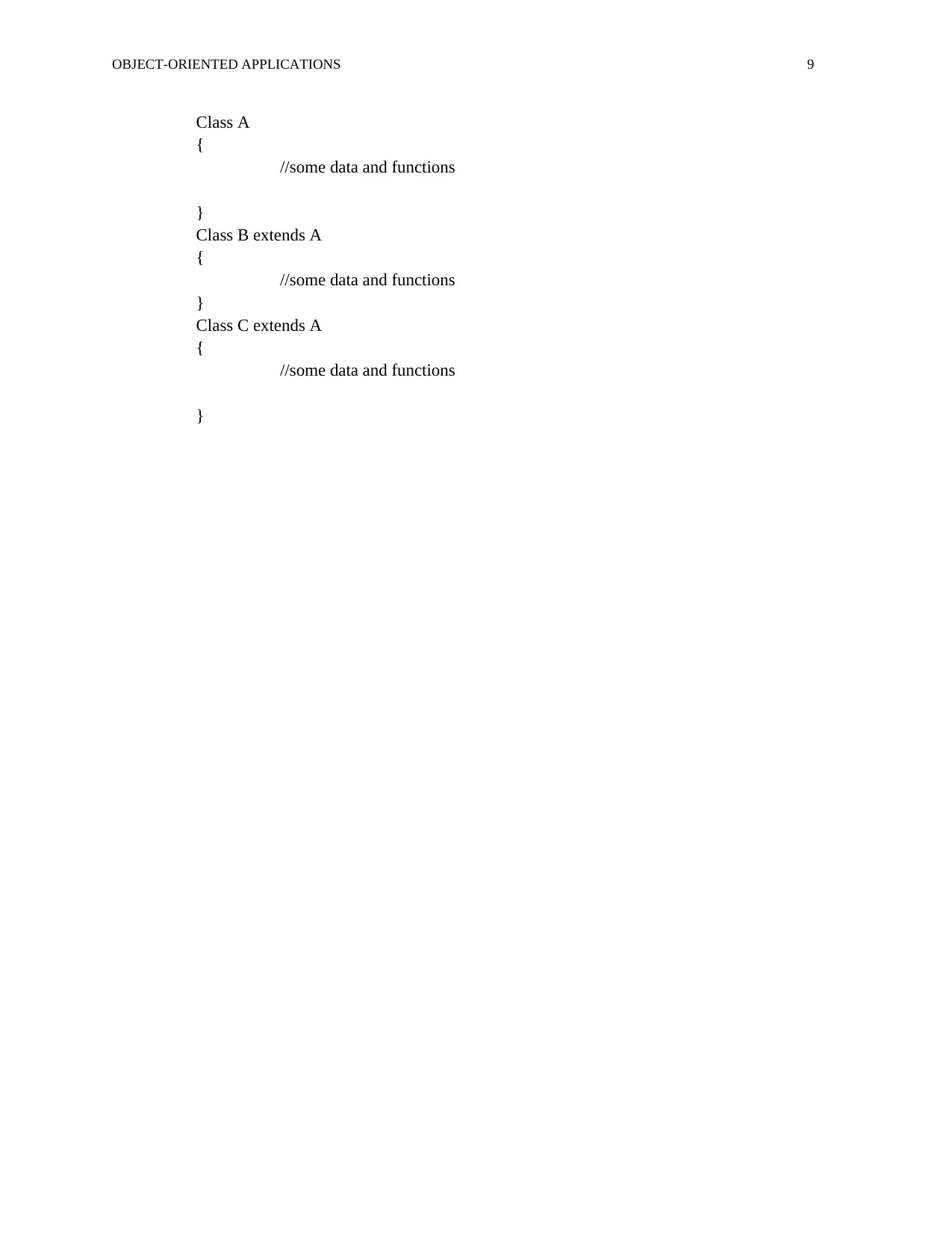
OBJECT-ORIENTED APPLICATIONS 9
Class A
{
//some data and functions
}
Class B extends A
{
//some data and functions
}
Class C extends A
{
//some data and functions
}
Class A
{
//some data and functions
}
Class B extends A
{
//some data and functions
}
Class C extends A
{
//some data and functions
}
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
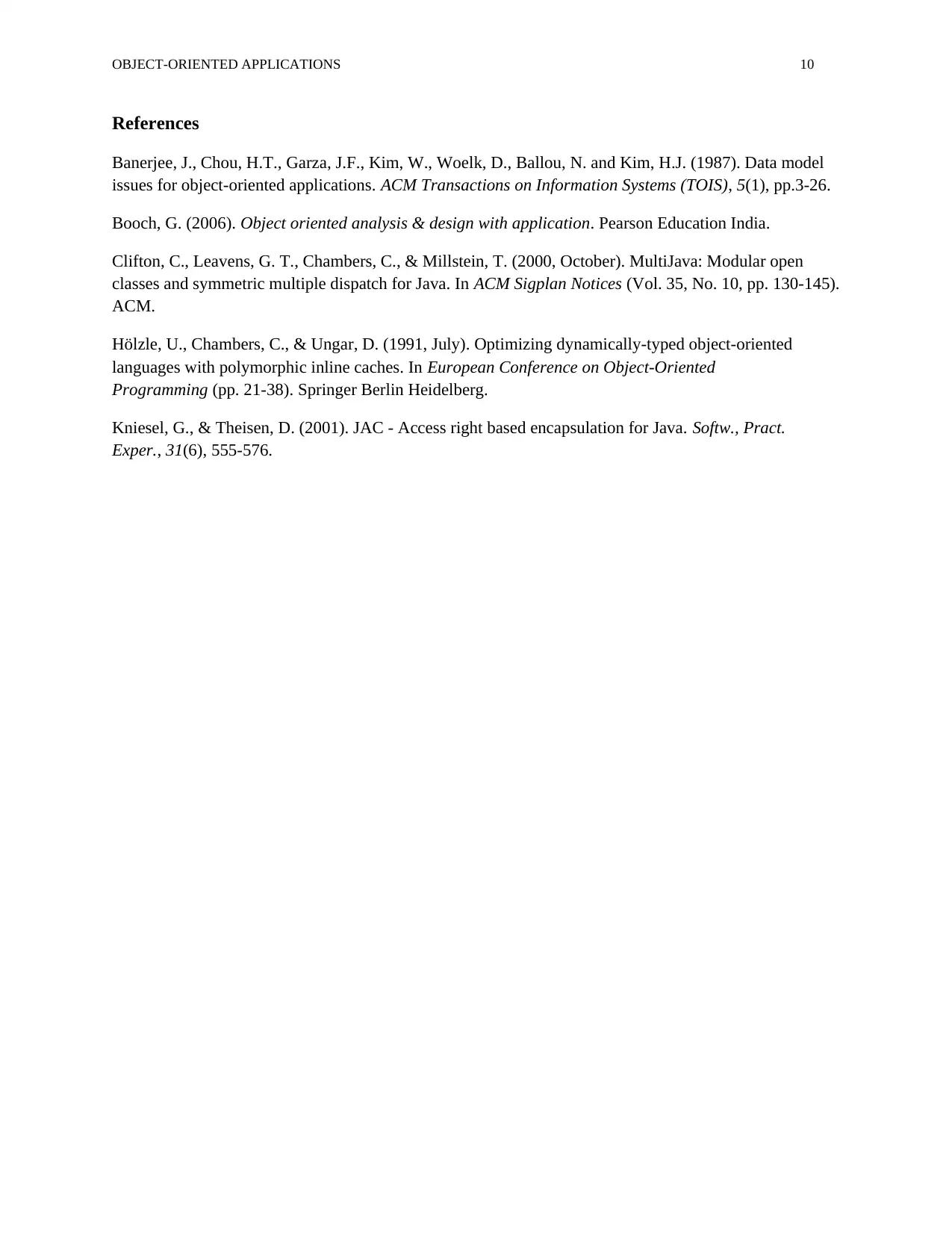
OBJECT-ORIENTED APPLICATIONS 10
References
Banerjee, J., Chou, H.T., Garza, J.F., Kim, W., Woelk, D., Ballou, N. and Kim, H.J. (1987). Data model
issues for object-oriented applications. ACM Transactions on Information Systems (TOIS), 5(1), pp.3-26.
Booch, G. (2006). Object oriented analysis & design with application. Pearson Education India.
Clifton, C., Leavens, G. T., Chambers, C., & Millstein, T. (2000, October). MultiJava: Modular open
classes and symmetric multiple dispatch for Java. In ACM Sigplan Notices (Vol. 35, No. 10, pp. 130-145).
ACM.
Hölzle, U., Chambers, C., & Ungar, D. (1991, July). Optimizing dynamically-typed object-oriented
languages with polymorphic inline caches. In European Conference on Object-Oriented
Programming (pp. 21-38). Springer Berlin Heidelberg.
Kniesel, G., & Theisen, D. (2001). JAC - Access right based encapsulation for Java. Softw., Pract.
Exper., 31(6), 555-576.
References
Banerjee, J., Chou, H.T., Garza, J.F., Kim, W., Woelk, D., Ballou, N. and Kim, H.J. (1987). Data model
issues for object-oriented applications. ACM Transactions on Information Systems (TOIS), 5(1), pp.3-26.
Booch, G. (2006). Object oriented analysis & design with application. Pearson Education India.
Clifton, C., Leavens, G. T., Chambers, C., & Millstein, T. (2000, October). MultiJava: Modular open
classes and symmetric multiple dispatch for Java. In ACM Sigplan Notices (Vol. 35, No. 10, pp. 130-145).
ACM.
Hölzle, U., Chambers, C., & Ungar, D. (1991, July). Optimizing dynamically-typed object-oriented
languages with polymorphic inline caches. In European Conference on Object-Oriented
Programming (pp. 21-38). Springer Berlin Heidelberg.
Kniesel, G., & Theisen, D. (2001). JAC - Access right based encapsulation for Java. Softw., Pract.
Exper., 31(6), 555-576.
1 out of 11
Related Documents

Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.