PasswordSaver.py: Caesar Cypher Password Storage Program for CSC101
VerifiedAdded on  2019/09/18
|5
|1446
|497
Homework Assignment
AI Summary
This assignment focuses on developing a password saver program in Python, incorporating the Caesar cypher for encrypting and decrypting passwords. The program is designed to store website usernames and passwords securely. It includes functionalities for loading passwords from a file, looking up passwords for specific websites, adding new passwords (with encryption), saving the password file, and optionally, printing the encrypted password list for testing purposes. Students are required to complete missing code segments to implement these features, including the lookup and add password functionalities. The assignment emphasizes practical application of programming concepts and encryption techniques, with a grading rubric assessing program correctness, readability, and adherence to assignment specifications. The program should be able to load passwords from a file, lookup passwords for websites, add new passwords for websites (encrypting them with the caesar cypher), and store these passwords to a file on the computer.
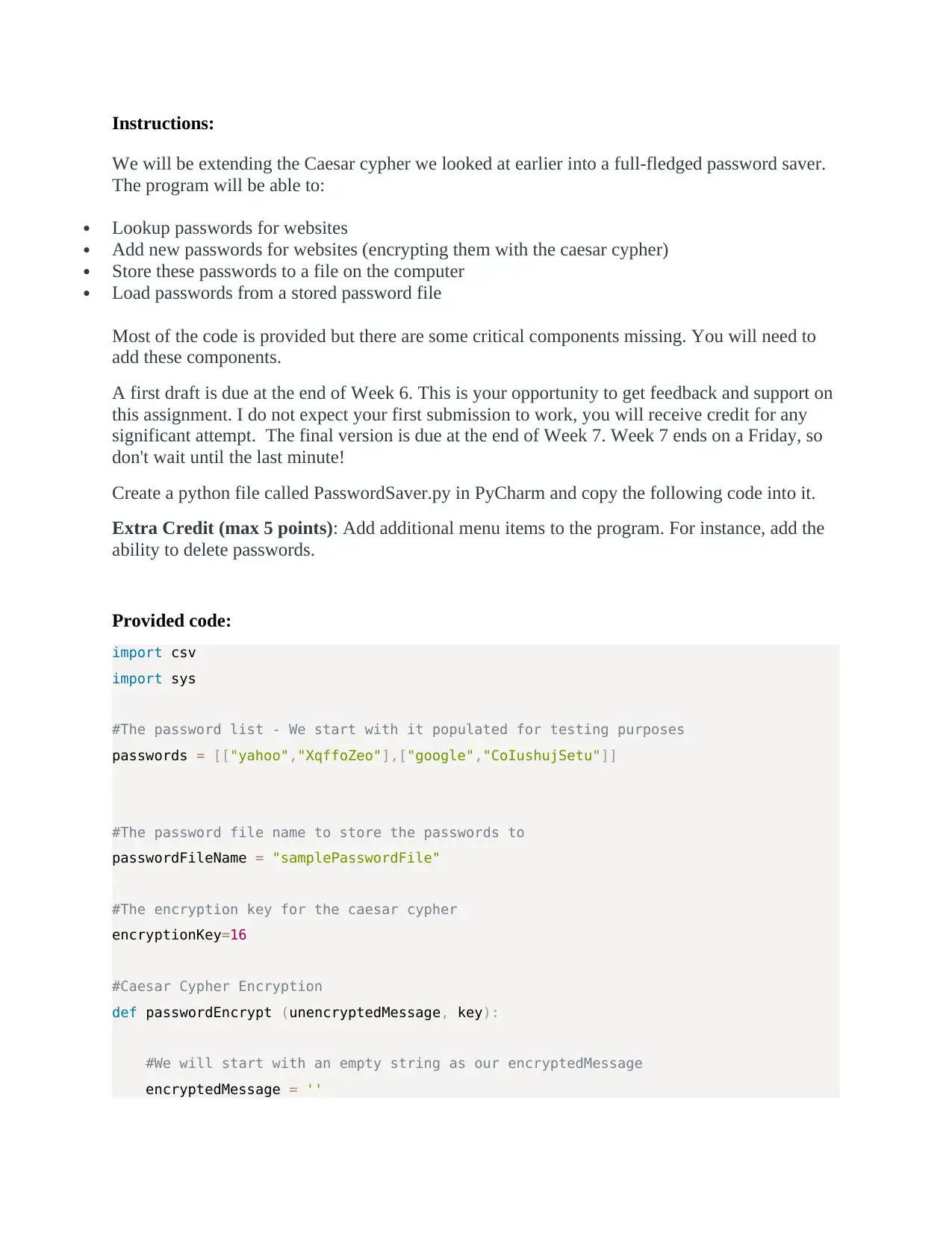
Instructions:
We will be extending the Caesar cypher we looked at earlier into a full-fledged password saver.
The program will be able to:
ï‚· Lookup passwords for websites
ï‚· Add new passwords for websites (encrypting them with the caesar cypher)
ï‚· Store these passwords to a file on the computer
ï‚· Load passwords from a stored password file
Most of the code is provided but there are some critical components missing. You will need to
add these components.
A first draft is due at the end of Week 6. This is your opportunity to get feedback and support on
this assignment. I do not expect your first submission to work, you will receive credit for any
significant attempt. The final version is due at the end of Week 7. Week 7 ends on a Friday, so
don't wait until the last minute!
Create a python file called PasswordSaver.py in PyCharm and copy the following code into it.
Extra Credit (max 5 points): Add additional menu items to the program. For instance, add the
ability to delete passwords.
Provided code:
import csv
import sys
#The password list - We start with it populated for testing purposes
passwords = [["yahoo","XqffoZeo"],["google","CoIushujSetu"]]
#The password file name to store the passwords to
passwordFileName = "samplePasswordFile"
#The encryption key for the caesar cypher
encryptionKey=16
#Caesar Cypher Encryption
def passwordEncrypt (unencryptedMessage, key):
#We will start with an empty string as our encryptedMessage
encryptedMessage = ''
We will be extending the Caesar cypher we looked at earlier into a full-fledged password saver.
The program will be able to:
ï‚· Lookup passwords for websites
ï‚· Add new passwords for websites (encrypting them with the caesar cypher)
ï‚· Store these passwords to a file on the computer
ï‚· Load passwords from a stored password file
Most of the code is provided but there are some critical components missing. You will need to
add these components.
A first draft is due at the end of Week 6. This is your opportunity to get feedback and support on
this assignment. I do not expect your first submission to work, you will receive credit for any
significant attempt. The final version is due at the end of Week 7. Week 7 ends on a Friday, so
don't wait until the last minute!
Create a python file called PasswordSaver.py in PyCharm and copy the following code into it.
Extra Credit (max 5 points): Add additional menu items to the program. For instance, add the
ability to delete passwords.
Provided code:
import csv
import sys
#The password list - We start with it populated for testing purposes
passwords = [["yahoo","XqffoZeo"],["google","CoIushujSetu"]]
#The password file name to store the passwords to
passwordFileName = "samplePasswordFile"
#The encryption key for the caesar cypher
encryptionKey=16
#Caesar Cypher Encryption
def passwordEncrypt (unencryptedMessage, key):
#We will start with an empty string as our encryptedMessage
encryptedMessage = ''
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
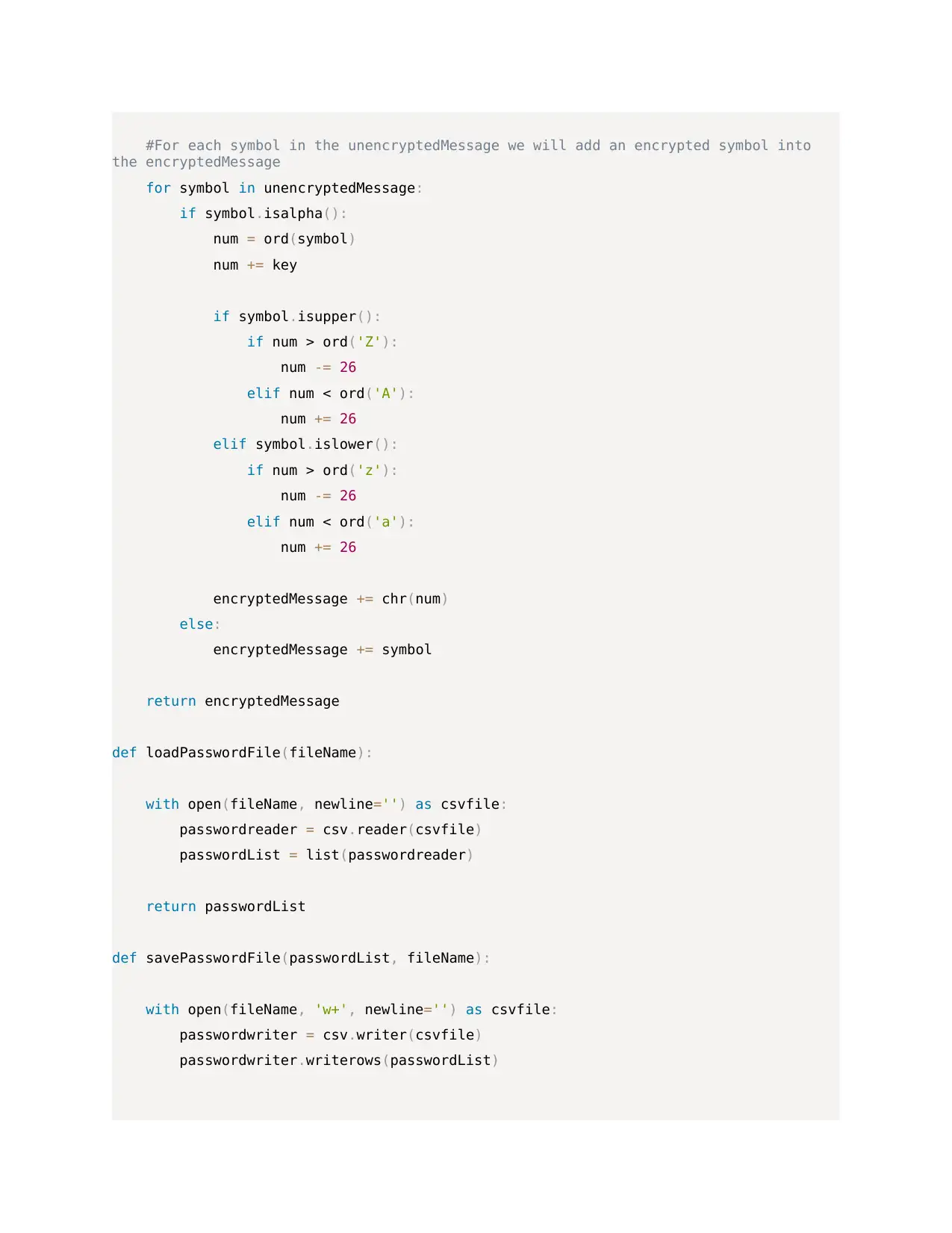
#For each symbol in the unencryptedMessage we will add an encrypted symbol into
the encryptedMessage
for symbol in unencryptedMessage:
if symbol.isalpha():
num = ord(symbol)
num += key
if symbol.isupper():
if num > ord('Z'):
num -= 26
elif num < ord('A'):
num += 26
elif symbol.islower():
if num > ord('z'):
num -= 26
elif num < ord('a'):
num += 26
encryptedMessage += chr(num)
else:
encryptedMessage += symbol
return encryptedMessage
def loadPasswordFile(fileName):
with open(fileName, newline='') as csvfile:
passwordreader = csv.reader(csvfile)
passwordList = list(passwordreader)
return passwordList
def savePasswordFile(passwordList, fileName):
with open(fileName, 'w+', newline='') as csvfile:
passwordwriter = csv.writer(csvfile)
passwordwriter.writerows(passwordList)
the encryptedMessage
for symbol in unencryptedMessage:
if symbol.isalpha():
num = ord(symbol)
num += key
if symbol.isupper():
if num > ord('Z'):
num -= 26
elif num < ord('A'):
num += 26
elif symbol.islower():
if num > ord('z'):
num -= 26
elif num < ord('a'):
num += 26
encryptedMessage += chr(num)
else:
encryptedMessage += symbol
return encryptedMessage
def loadPasswordFile(fileName):
with open(fileName, newline='') as csvfile:
passwordreader = csv.reader(csvfile)
passwordList = list(passwordreader)
return passwordList
def savePasswordFile(passwordList, fileName):
with open(fileName, 'w+', newline='') as csvfile:
passwordwriter = csv.writer(csvfile)
passwordwriter.writerows(passwordList)
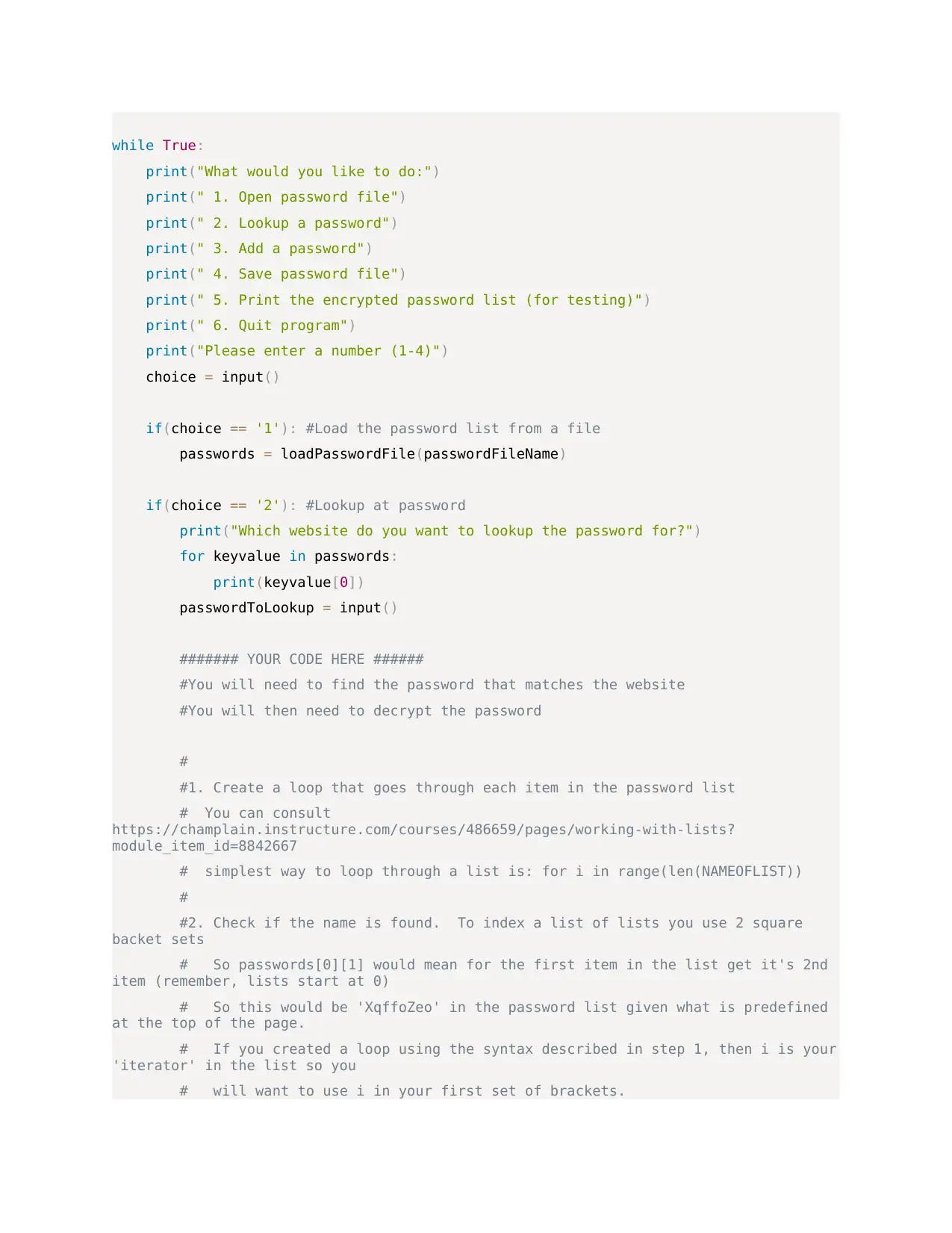
while True:
print("What would you like to do:")
print(" 1. Open password file")
print(" 2. Lookup a password")
print(" 3. Add a password")
print(" 4. Save password file")
print(" 5. Print the encrypted password list (for testing)")
print(" 6. Quit program")
print("Please enter a number (1-4)")
choice = input()
if(choice == '1'): #Load the password list from a file
passwords = loadPasswordFile(passwordFileName)
if(choice == '2'): #Lookup at password
print("Which website do you want to lookup the password for?")
for keyvalue in passwords:
print(keyvalue[0])
passwordToLookup = input()
####### YOUR CODE HERE ######
#You will need to find the password that matches the website
#You will then need to decrypt the password
#
#1. Create a loop that goes through each item in the password list
# You can consult
https://champlain.instructure.com/courses/486659/pages/working-with-lists?
module_item_id=8842667
# simplest way to loop through a list is: for i in range(len(NAMEOFLIST))
#
#2. Check if the name is found. To index a list of lists you use 2 square
backet sets
# So passwords[0][1] would mean for the first item in the list get it's 2nd
item (remember, lists start at 0)
# So this would be 'XqffoZeo' in the password list given what is predefined
at the top of the page.
# If you created a loop using the syntax described in step 1, then i is your
'iterator' in the list so you
# will want to use i in your first set of brackets.
print("What would you like to do:")
print(" 1. Open password file")
print(" 2. Lookup a password")
print(" 3. Add a password")
print(" 4. Save password file")
print(" 5. Print the encrypted password list (for testing)")
print(" 6. Quit program")
print("Please enter a number (1-4)")
choice = input()
if(choice == '1'): #Load the password list from a file
passwords = loadPasswordFile(passwordFileName)
if(choice == '2'): #Lookup at password
print("Which website do you want to lookup the password for?")
for keyvalue in passwords:
print(keyvalue[0])
passwordToLookup = input()
####### YOUR CODE HERE ######
#You will need to find the password that matches the website
#You will then need to decrypt the password
#
#1. Create a loop that goes through each item in the password list
# You can consult
https://champlain.instructure.com/courses/486659/pages/working-with-lists?
module_item_id=8842667
# simplest way to loop through a list is: for i in range(len(NAMEOFLIST))
#
#2. Check if the name is found. To index a list of lists you use 2 square
backet sets
# So passwords[0][1] would mean for the first item in the list get it's 2nd
item (remember, lists start at 0)
# So this would be 'XqffoZeo' in the password list given what is predefined
at the top of the page.
# If you created a loop using the syntax described in step 1, then i is your
'iterator' in the list so you
# will want to use i in your first set of brackets.
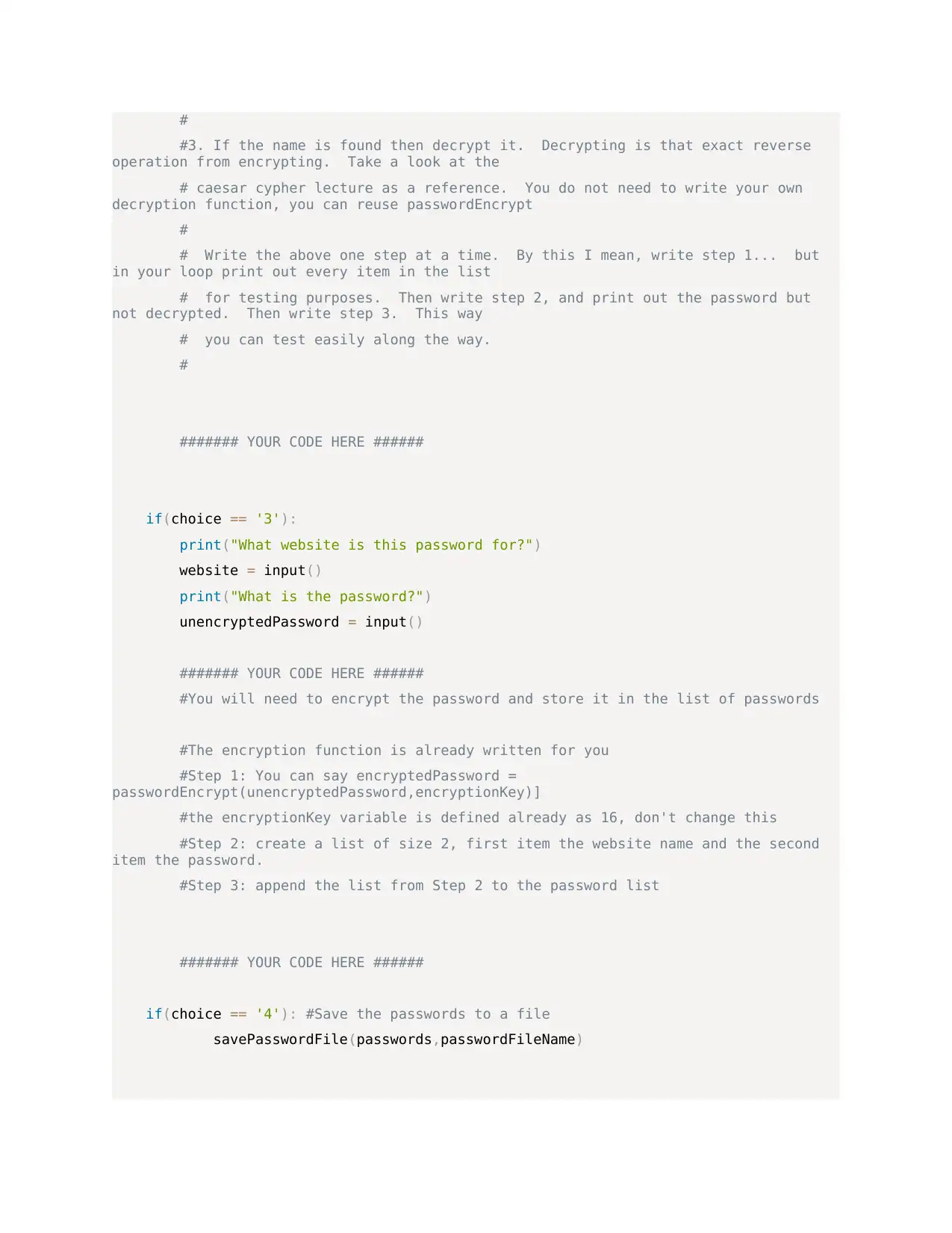
#
#3. If the name is found then decrypt it. Decrypting is that exact reverse
operation from encrypting. Take a look at the
# caesar cypher lecture as a reference. You do not need to write your own
decryption function, you can reuse passwordEncrypt
#
# Write the above one step at a time. By this I mean, write step 1... but
in your loop print out every item in the list
# for testing purposes. Then write step 2, and print out the password but
not decrypted. Then write step 3. This way
# you can test easily along the way.
#
####### YOUR CODE HERE ######
if(choice == '3'):
print("What website is this password for?")
website = input()
print("What is the password?")
unencryptedPassword = input()
####### YOUR CODE HERE ######
#You will need to encrypt the password and store it in the list of passwords
#The encryption function is already written for you
#Step 1: You can say encryptedPassword =
passwordEncrypt(unencryptedPassword,encryptionKey)]
#the encryptionKey variable is defined already as 16, don't change this
#Step 2: create a list of size 2, first item the website name and the second
item the password.
#Step 3: append the list from Step 2 to the password list
####### YOUR CODE HERE ######
if(choice == '4'): #Save the passwords to a file
savePasswordFile(passwords,passwordFileName)
#3. If the name is found then decrypt it. Decrypting is that exact reverse
operation from encrypting. Take a look at the
# caesar cypher lecture as a reference. You do not need to write your own
decryption function, you can reuse passwordEncrypt
#
# Write the above one step at a time. By this I mean, write step 1... but
in your loop print out every item in the list
# for testing purposes. Then write step 2, and print out the password but
not decrypted. Then write step 3. This way
# you can test easily along the way.
#
####### YOUR CODE HERE ######
if(choice == '3'):
print("What website is this password for?")
website = input()
print("What is the password?")
unencryptedPassword = input()
####### YOUR CODE HERE ######
#You will need to encrypt the password and store it in the list of passwords
#The encryption function is already written for you
#Step 1: You can say encryptedPassword =
passwordEncrypt(unencryptedPassword,encryptionKey)]
#the encryptionKey variable is defined already as 16, don't change this
#Step 2: create a list of size 2, first item the website name and the second
item the password.
#Step 3: append the list from Step 2 to the password list
####### YOUR CODE HERE ######
if(choice == '4'): #Save the passwords to a file
savePasswordFile(passwords,passwordFileName)
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
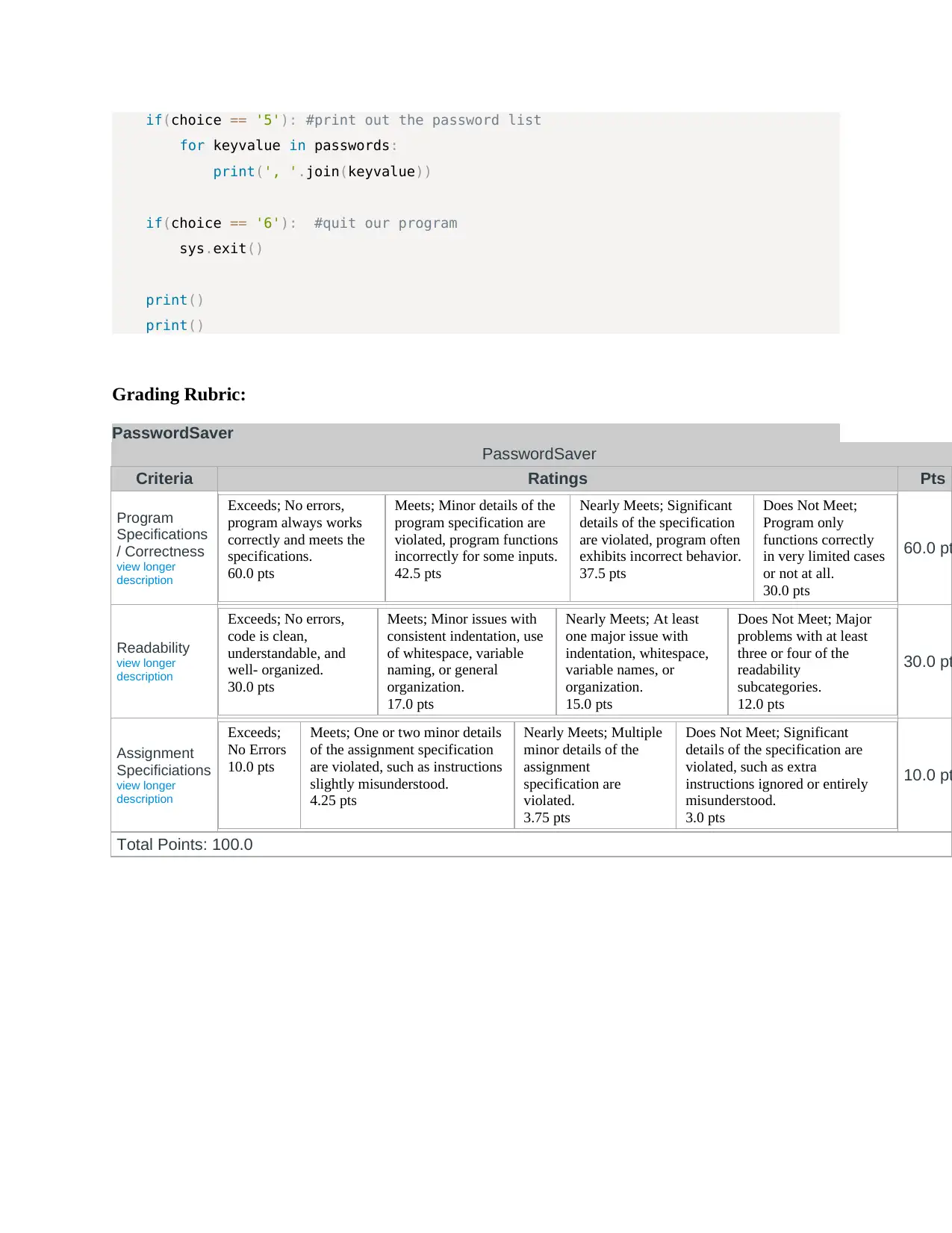
if(choice == '5'): #print out the password list
for keyvalue in passwords:
print(', '.join(keyvalue))
if(choice == '6'): #quit our program
sys.exit()
print()
print()
Grading Rubric:
PasswordSaver
PasswordSaver
Criteria Ratings Pts
Program
Specifications
/ Correctness
view longer
description
Exceeds; No errors,
program always works
correctly and meets the
specifications.
60.0 pts
Meets; Minor details of the
program specification are
violated, program functions
incorrectly for some inputs.
42.5 pts
Nearly Meets; Significant
details of the specification
are violated, program often
exhibits incorrect behavior.
37.5 pts
Does Not Meet;
Program only
functions correctly
in very limited cases
or not at all.
30.0 pts
60.0 pt
Readability
view longer
description
Exceeds; No errors,
code is clean,
understandable, and
well- organized.
30.0 pts
Meets; Minor issues with
consistent indentation, use
of whitespace, variable
naming, or general
organization.
17.0 pts
Nearly Meets; At least
one major issue with
indentation, whitespace,
variable names, or
organization.
15.0 pts
Does Not Meet; Major
problems with at least
three or four of the
readability
subcategories.
12.0 pts
30.0 pt
Assignment
Specificiations
view longer
description
Exceeds;
No Errors
10.0 pts
Meets; One or two minor details
of the assignment specification
are violated, such as instructions
slightly misunderstood.
4.25 pts
Nearly Meets; Multiple
minor details of the
assignment
specification are
violated.
3.75 pts
Does Not Meet; Significant
details of the specification are
violated, such as extra
instructions ignored or entirely
misunderstood.
3.0 pts
10.0 pt
Total Points: 100.0
for keyvalue in passwords:
print(', '.join(keyvalue))
if(choice == '6'): #quit our program
sys.exit()
print()
print()
Grading Rubric:
PasswordSaver
PasswordSaver
Criteria Ratings Pts
Program
Specifications
/ Correctness
view longer
description
Exceeds; No errors,
program always works
correctly and meets the
specifications.
60.0 pts
Meets; Minor details of the
program specification are
violated, program functions
incorrectly for some inputs.
42.5 pts
Nearly Meets; Significant
details of the specification
are violated, program often
exhibits incorrect behavior.
37.5 pts
Does Not Meet;
Program only
functions correctly
in very limited cases
or not at all.
30.0 pts
60.0 pt
Readability
view longer
description
Exceeds; No errors,
code is clean,
understandable, and
well- organized.
30.0 pts
Meets; Minor issues with
consistent indentation, use
of whitespace, variable
naming, or general
organization.
17.0 pts
Nearly Meets; At least
one major issue with
indentation, whitespace,
variable names, or
organization.
15.0 pts
Does Not Meet; Major
problems with at least
three or four of the
readability
subcategories.
12.0 pts
30.0 pt
Assignment
Specificiations
view longer
description
Exceeds;
No Errors
10.0 pts
Meets; One or two minor details
of the assignment specification
are violated, such as instructions
slightly misunderstood.
4.25 pts
Nearly Meets; Multiple
minor details of the
assignment
specification are
violated.
3.75 pts
Does Not Meet; Significant
details of the specification are
violated, such as extra
instructions ignored or entirely
misunderstood.
3.0 pts
10.0 pt
Total Points: 100.0
1 out of 5

Your All-in-One AI-Powered Toolkit for Academic Success.
 +13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024  |  Zucol Services PVT LTD  |  All rights reserved.