Software Engineering Order Assignment: Singleton Design Pattern 12
VerifiedAdded on 2022/08/10
|12
|2215
|23
Homework Assignment
AI Summary
This assignment delves into the Singleton Design Pattern, a creational design pattern used in software engineering to restrict a class to a single instance. The assignment explores the pattern's implementation, including UML diagrams, eager and lazy initialization approaches, and thread safety considerations. It examines the use of mutexes for thread synchronization to address potential issues when multiple threads access the same instance. The assignment also covers the pattern's application in scenarios like logging, driver objects, and database connections. Furthermore, it highlights the pattern's advantages in encapsulating data and ensuring uniqueness, while also acknowledging criticisms that label it as an anti-pattern in certain contexts. The solution includes code examples and discusses the implications of creating multiple instances and the importance of adhering to the Singleton's core principles.
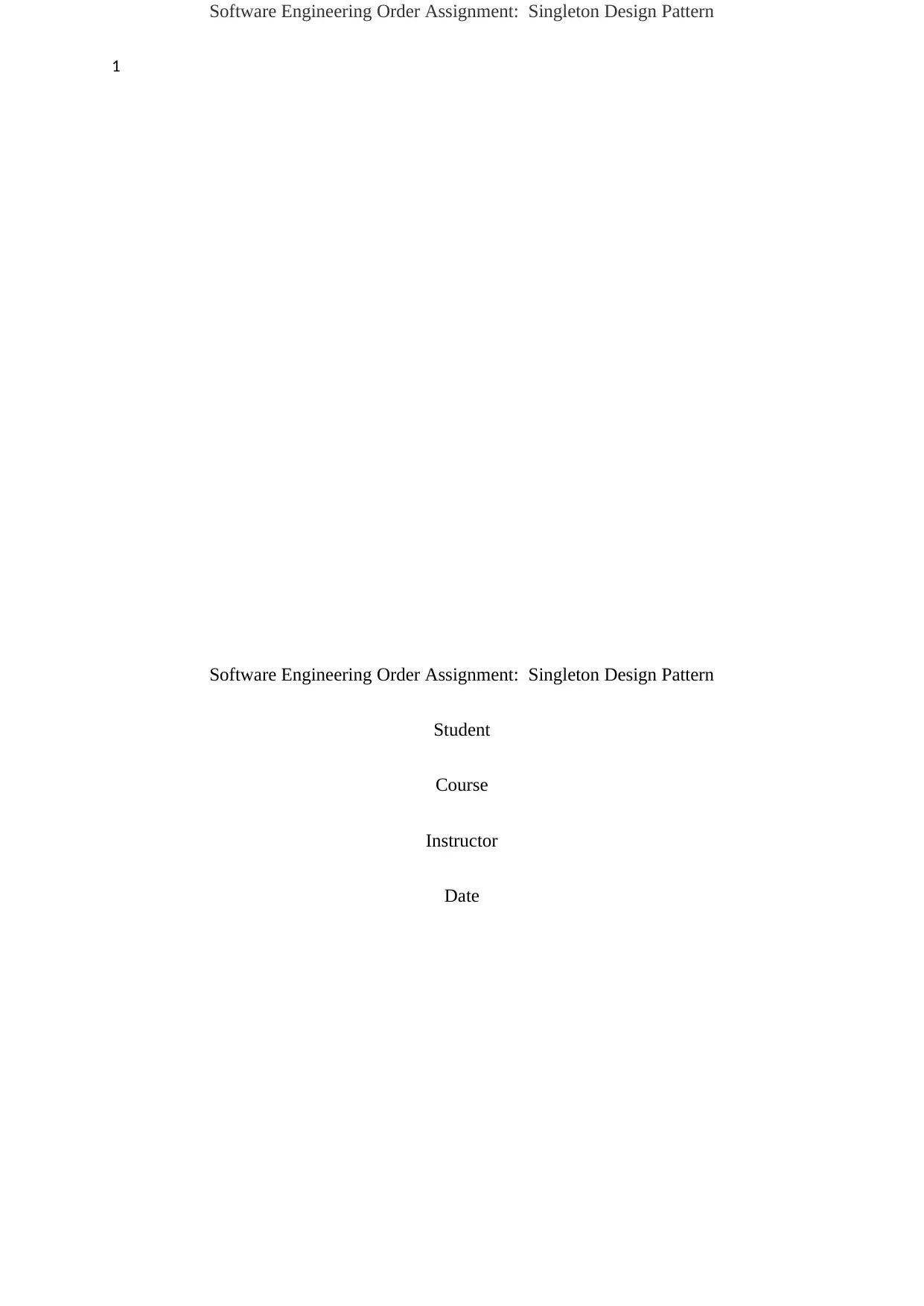
Software Engineering Order Assignment: Singleton Design Pattern
1
Software Engineering Order Assignment: Singleton Design Pattern
Student
Course
Instructor
Date
1
Software Engineering Order Assignment: Singleton Design Pattern
Student
Course
Instructor
Date
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
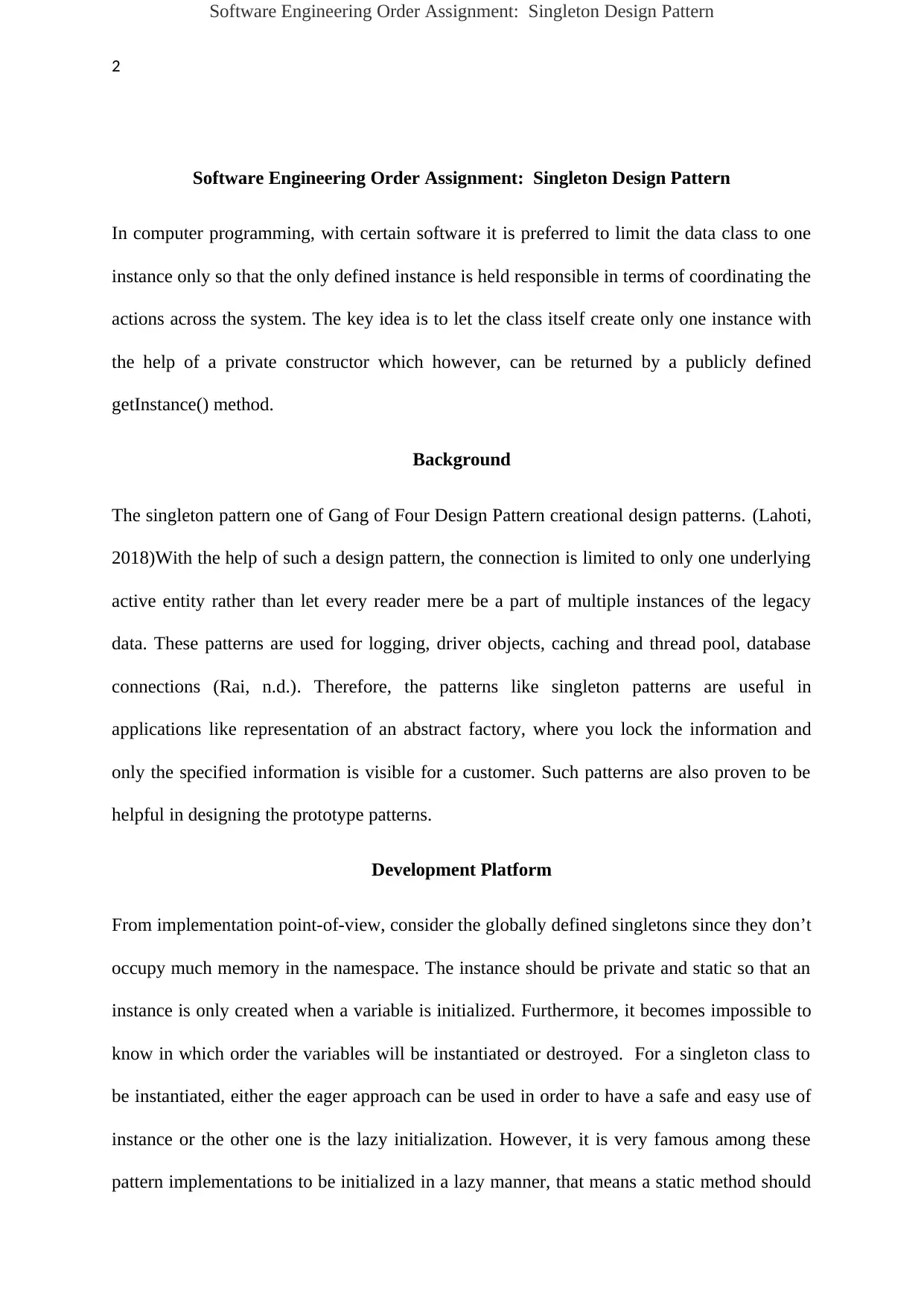
Software Engineering Order Assignment: Singleton Design Pattern
2
Software Engineering Order Assignment: Singleton Design Pattern
In computer programming, with certain software it is preferred to limit the data class to one
instance only so that the only defined instance is held responsible in terms of coordinating the
actions across the system. The key idea is to let the class itself create only one instance with
the help of a private constructor which however, can be returned by a publicly defined
getInstance() method.
Background
The singleton pattern one of Gang of Four Design Pattern creational design patterns. (Lahoti,
2018)With the help of such a design pattern, the connection is limited to only one underlying
active entity rather than let every reader mere be a part of multiple instances of the legacy
data. These patterns are used for logging, driver objects, caching and thread pool, database
connections (Rai, n.d.). Therefore, the patterns like singleton patterns are useful in
applications like representation of an abstract factory, where you lock the information and
only the specified information is visible for a customer. Such patterns are also proven to be
helpful in designing the prototype patterns.
Development Platform
From implementation point-of-view, consider the globally defined singletons since they don’t
occupy much memory in the namespace. The instance should be private and static so that an
instance is only created when a variable is initialized. Furthermore, it becomes impossible to
know in which order the variables will be instantiated or destroyed. For a singleton class to
be instantiated, either the eager approach can be used in order to have a safe and easy use of
instance or the other one is the lazy initialization. However, it is very famous among these
pattern implementations to be initialized in a lazy manner, that means a static method should
2
Software Engineering Order Assignment: Singleton Design Pattern
In computer programming, with certain software it is preferred to limit the data class to one
instance only so that the only defined instance is held responsible in terms of coordinating the
actions across the system. The key idea is to let the class itself create only one instance with
the help of a private constructor which however, can be returned by a publicly defined
getInstance() method.
Background
The singleton pattern one of Gang of Four Design Pattern creational design patterns. (Lahoti,
2018)With the help of such a design pattern, the connection is limited to only one underlying
active entity rather than let every reader mere be a part of multiple instances of the legacy
data. These patterns are used for logging, driver objects, caching and thread pool, database
connections (Rai, n.d.). Therefore, the patterns like singleton patterns are useful in
applications like representation of an abstract factory, where you lock the information and
only the specified information is visible for a customer. Such patterns are also proven to be
helpful in designing the prototype patterns.
Development Platform
From implementation point-of-view, consider the globally defined singletons since they don’t
occupy much memory in the namespace. The instance should be private and static so that an
instance is only created when a variable is initialized. Furthermore, it becomes impossible to
know in which order the variables will be instantiated or destroyed. For a singleton class to
be instantiated, either the eager approach can be used in order to have a safe and easy use of
instance or the other one is the lazy initialization. However, it is very famous among these
pattern implementations to be initialized in a lazy manner, that means a static method should
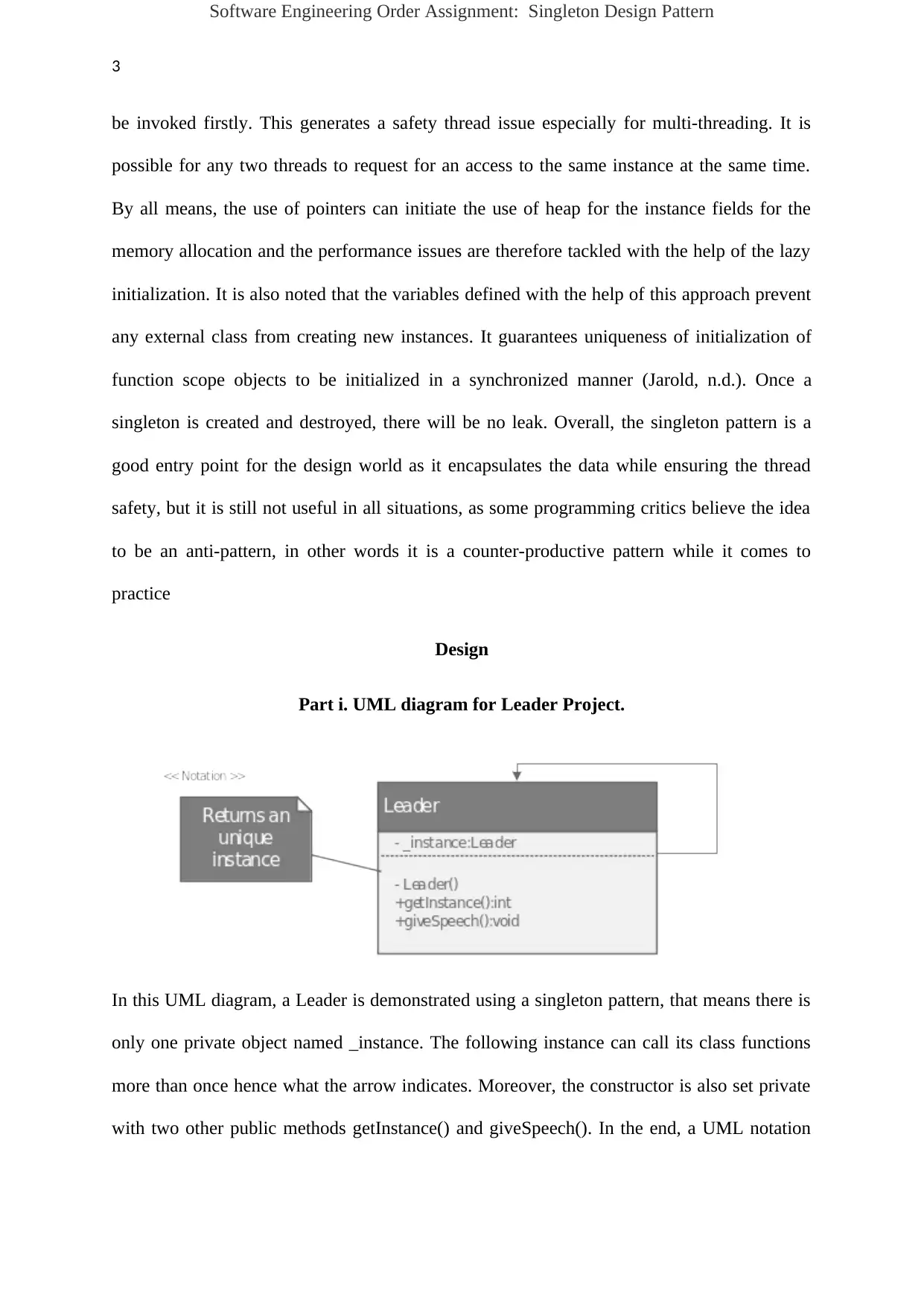
Software Engineering Order Assignment: Singleton Design Pattern
3
be invoked firstly. This generates a safety thread issue especially for multi-threading. It is
possible for any two threads to request for an access to the same instance at the same time.
By all means, the use of pointers can initiate the use of heap for the instance fields for the
memory allocation and the performance issues are therefore tackled with the help of the lazy
initialization. It is also noted that the variables defined with the help of this approach prevent
any external class from creating new instances. It guarantees uniqueness of initialization of
function scope objects to be initialized in a synchronized manner (Jarold, n.d.). Once a
singleton is created and destroyed, there will be no leak. Overall, the singleton pattern is a
good entry point for the design world as it encapsulates the data while ensuring the thread
safety, but it is still not useful in all situations, as some programming critics believe the idea
to be an anti-pattern, in other words it is a counter-productive pattern while it comes to
practice
Design
Part i. UML diagram for Leader Project.
In this UML diagram, a Leader is demonstrated using a singleton pattern, that means there is
only one private object named _instance. The following instance can call its class functions
more than once hence what the arrow indicates. Moreover, the constructor is also set private
with two other public methods getInstance() and giveSpeech(). In the end, a UML notation
3
be invoked firstly. This generates a safety thread issue especially for multi-threading. It is
possible for any two threads to request for an access to the same instance at the same time.
By all means, the use of pointers can initiate the use of heap for the instance fields for the
memory allocation and the performance issues are therefore tackled with the help of the lazy
initialization. It is also noted that the variables defined with the help of this approach prevent
any external class from creating new instances. It guarantees uniqueness of initialization of
function scope objects to be initialized in a synchronized manner (Jarold, n.d.). Once a
singleton is created and destroyed, there will be no leak. Overall, the singleton pattern is a
good entry point for the design world as it encapsulates the data while ensuring the thread
safety, but it is still not useful in all situations, as some programming critics believe the idea
to be an anti-pattern, in other words it is a counter-productive pattern while it comes to
practice
Design
Part i. UML diagram for Leader Project.
In this UML diagram, a Leader is demonstrated using a singleton pattern, that means there is
only one private object named _instance. The following instance can call its class functions
more than once hence what the arrow indicates. Moreover, the constructor is also set private
with two other public methods getInstance() and giveSpeech(). In the end, a UML notation
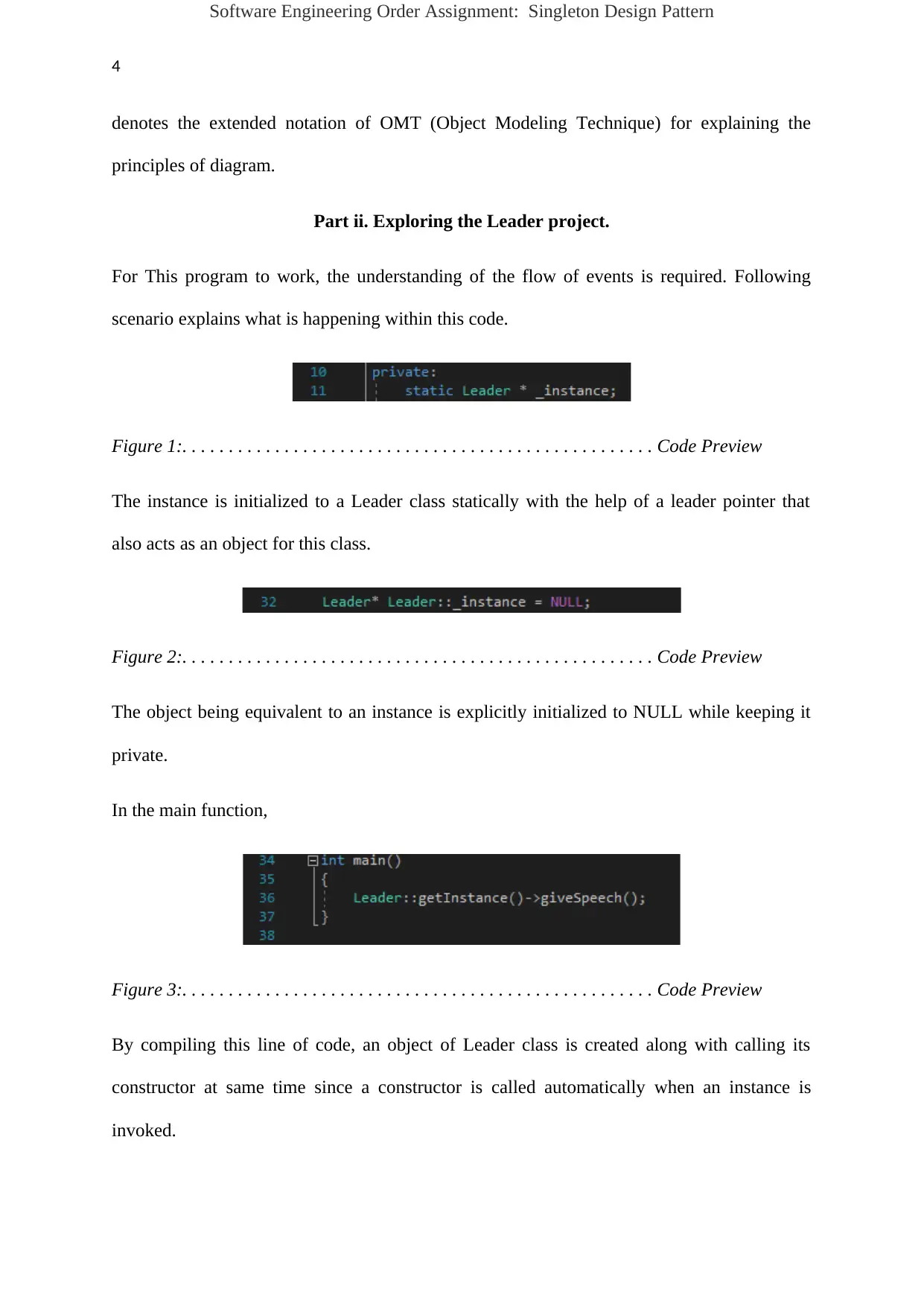
Software Engineering Order Assignment: Singleton Design Pattern
4
denotes the extended notation of OMT (Object Modeling Technique) for explaining the
principles of diagram.
Part ii. Exploring the Leader project.
For This program to work, the understanding of the flow of events is required. Following
scenario explains what is happening within this code.
Figure 1:. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . Code Preview
The instance is initialized to a Leader class statically with the help of a leader pointer that
also acts as an object for this class.
Figure 2:. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . Code Preview
The object being equivalent to an instance is explicitly initialized to NULL while keeping it
private.
In the main function,
Figure 3:. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . Code Preview
By compiling this line of code, an object of Leader class is created along with calling its
constructor at same time since a constructor is called automatically when an instance is
invoked.
4
denotes the extended notation of OMT (Object Modeling Technique) for explaining the
principles of diagram.
Part ii. Exploring the Leader project.
For This program to work, the understanding of the flow of events is required. Following
scenario explains what is happening within this code.
Figure 1:. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . Code Preview
The instance is initialized to a Leader class statically with the help of a leader pointer that
also acts as an object for this class.
Figure 2:. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . Code Preview
The object being equivalent to an instance is explicitly initialized to NULL while keeping it
private.
In the main function,
Figure 3:. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . Code Preview
By compiling this line of code, an object of Leader class is created along with calling its
constructor at same time since a constructor is called automatically when an instance is
invoked.
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
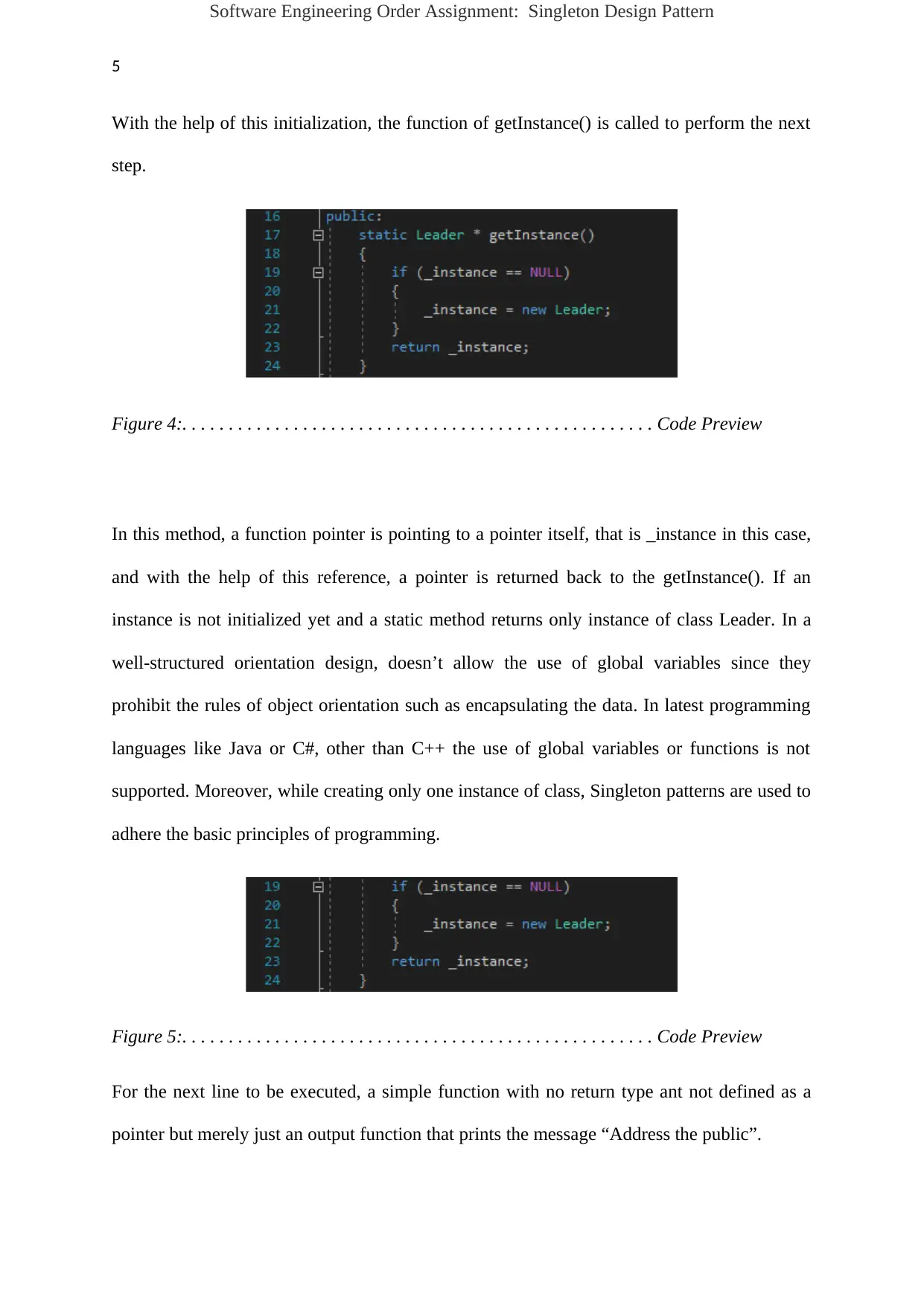
Software Engineering Order Assignment: Singleton Design Pattern
5
With the help of this initialization, the function of getInstance() is called to perform the next
step.
Figure 4:. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . Code Preview
In this method, a function pointer is pointing to a pointer itself, that is _instance in this case,
and with the help of this reference, a pointer is returned back to the getInstance(). If an
instance is not initialized yet and a static method returns only instance of class Leader. In a
well-structured orientation design, doesn’t allow the use of global variables since they
prohibit the rules of object orientation such as encapsulating the data. In latest programming
languages like Java or C#, other than C++ the use of global variables or functions is not
supported. Moreover, while creating only one instance of class, Singleton patterns are used to
adhere the basic principles of programming.
Figure 5:. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . Code Preview
For the next line to be executed, a simple function with no return type ant not defined as a
pointer but merely just an output function that prints the message “Address the public”.
5
With the help of this initialization, the function of getInstance() is called to perform the next
step.
Figure 4:. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . Code Preview
In this method, a function pointer is pointing to a pointer itself, that is _instance in this case,
and with the help of this reference, a pointer is returned back to the getInstance(). If an
instance is not initialized yet and a static method returns only instance of class Leader. In a
well-structured orientation design, doesn’t allow the use of global variables since they
prohibit the rules of object orientation such as encapsulating the data. In latest programming
languages like Java or C#, other than C++ the use of global variables or functions is not
supported. Moreover, while creating only one instance of class, Singleton patterns are used to
adhere the basic principles of programming.
Figure 5:. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . Code Preview
For the next line to be executed, a simple function with no return type ant not defined as a
pointer but merely just an output function that prints the message “Address the public”.
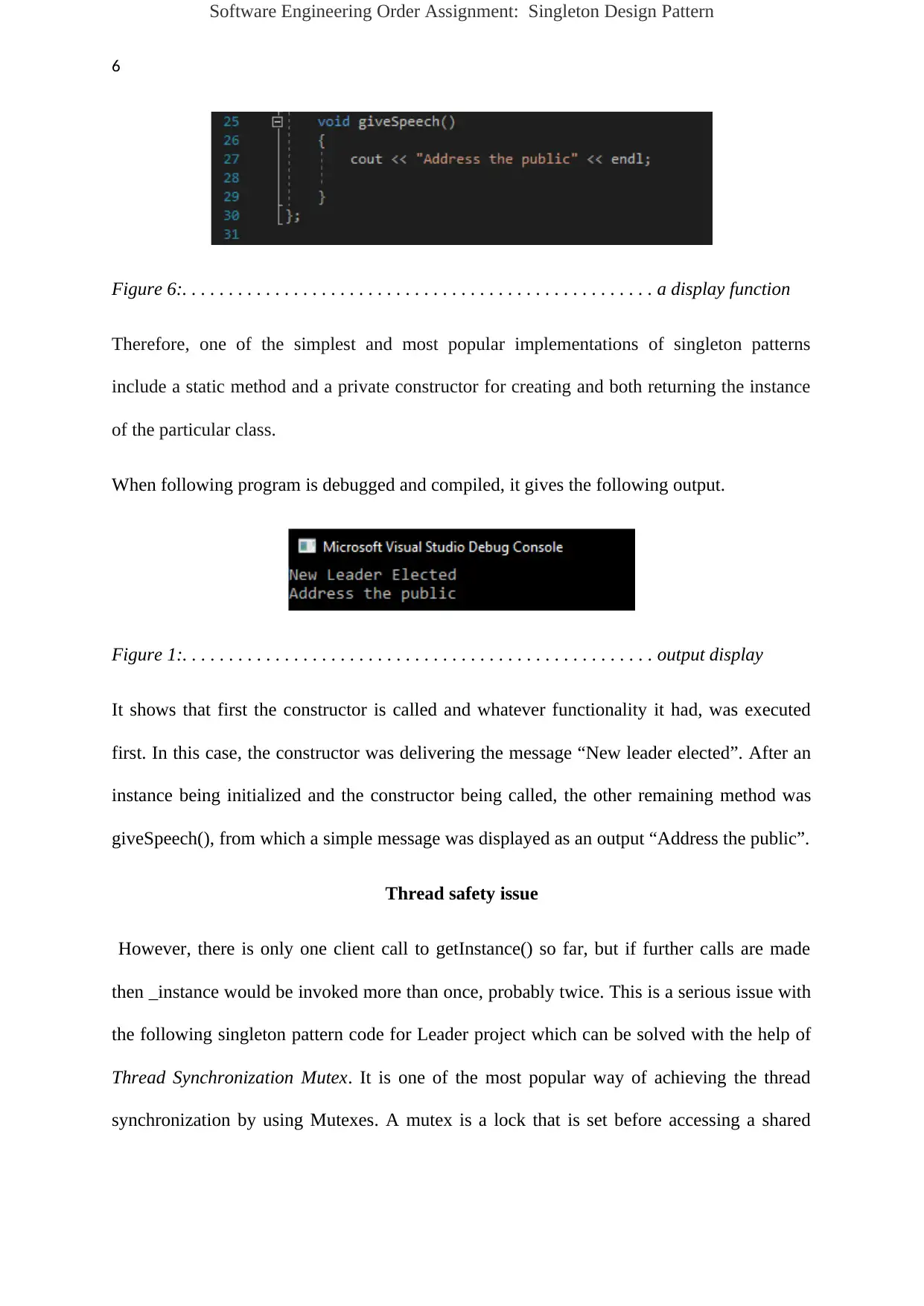
Software Engineering Order Assignment: Singleton Design Pattern
6
Figure 6:. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . a display function
Therefore, one of the simplest and most popular implementations of singleton patterns
include a static method and a private constructor for creating and both returning the instance
of the particular class.
When following program is debugged and compiled, it gives the following output.
Figure 1:. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . output display
It shows that first the constructor is called and whatever functionality it had, was executed
first. In this case, the constructor was delivering the message “New leader elected”. After an
instance being initialized and the constructor being called, the other remaining method was
giveSpeech(), from which a simple message was displayed as an output “Address the public”.
Thread safety issue
However, there is only one client call to getInstance() so far, but if further calls are made
then _instance would be invoked more than once, probably twice. This is a serious issue with
the following singleton pattern code for Leader project which can be solved with the help of
Thread Synchronization Mutex. It is one of the most popular way of achieving the thread
synchronization by using Mutexes. A mutex is a lock that is set before accessing a shared
6
Figure 6:. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . a display function
Therefore, one of the simplest and most popular implementations of singleton patterns
include a static method and a private constructor for creating and both returning the instance
of the particular class.
When following program is debugged and compiled, it gives the following output.
Figure 1:. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . output display
It shows that first the constructor is called and whatever functionality it had, was executed
first. In this case, the constructor was delivering the message “New leader elected”. After an
instance being initialized and the constructor being called, the other remaining method was
giveSpeech(), from which a simple message was displayed as an output “Address the public”.
Thread safety issue
However, there is only one client call to getInstance() so far, but if further calls are made
then _instance would be invoked more than once, probably twice. This is a serious issue with
the following singleton pattern code for Leader project which can be solved with the help of
Thread Synchronization Mutex. It is one of the most popular way of achieving the thread
synchronization by using Mutexes. A mutex is a lock that is set before accessing a shared
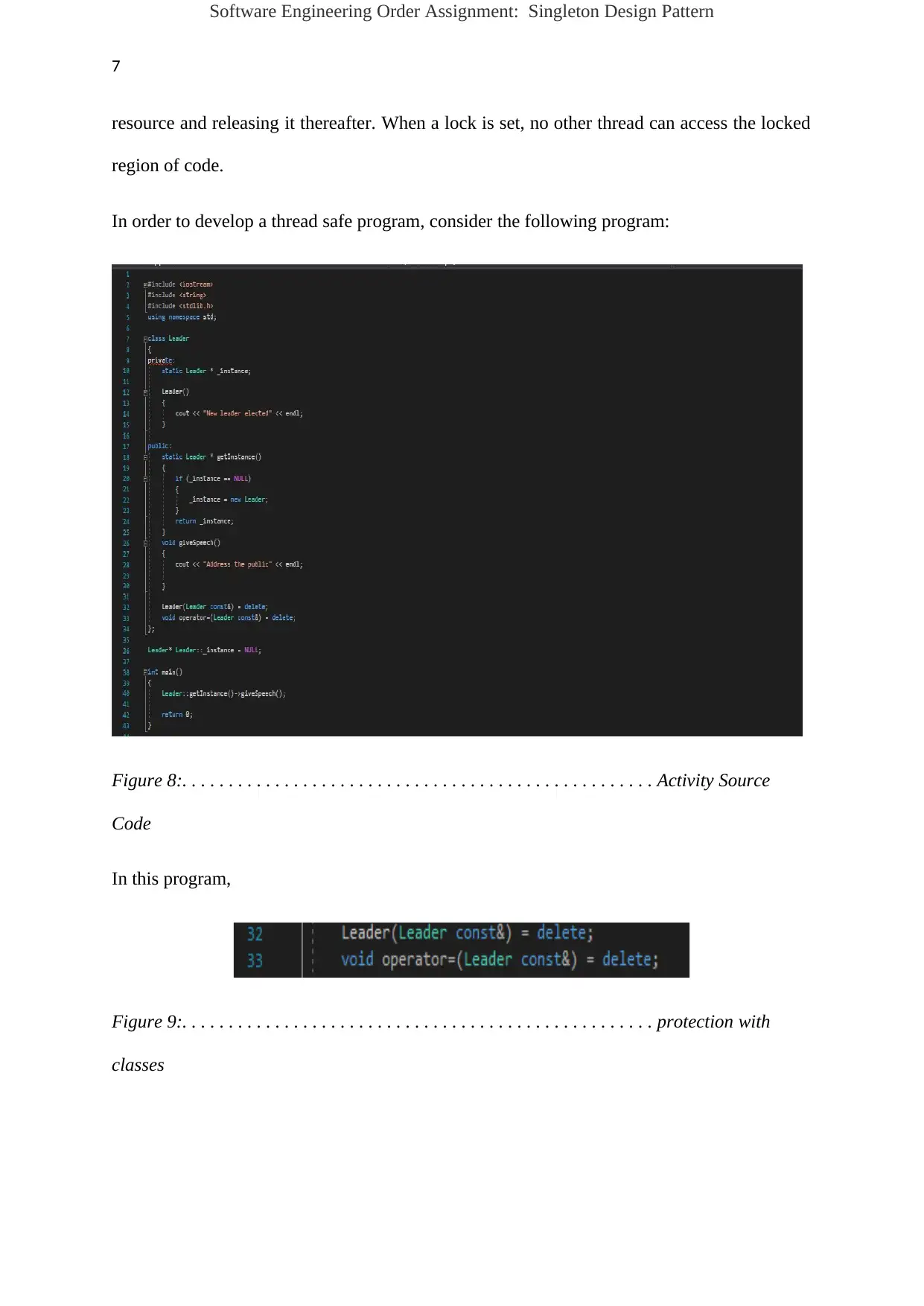
Software Engineering Order Assignment: Singleton Design Pattern
7
resource and releasing it thereafter. When a lock is set, no other thread can access the locked
region of code.
In order to develop a thread safe program, consider the following program:
Figure 8:. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . Activity Source
Code
In this program,
Figure 9:. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . protection with
classes
7
resource and releasing it thereafter. When a lock is set, no other thread can access the locked
region of code.
In order to develop a thread safe program, consider the following program:
Figure 8:. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . Activity Source
Code
In this program,
Figure 9:. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . protection with
classes
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
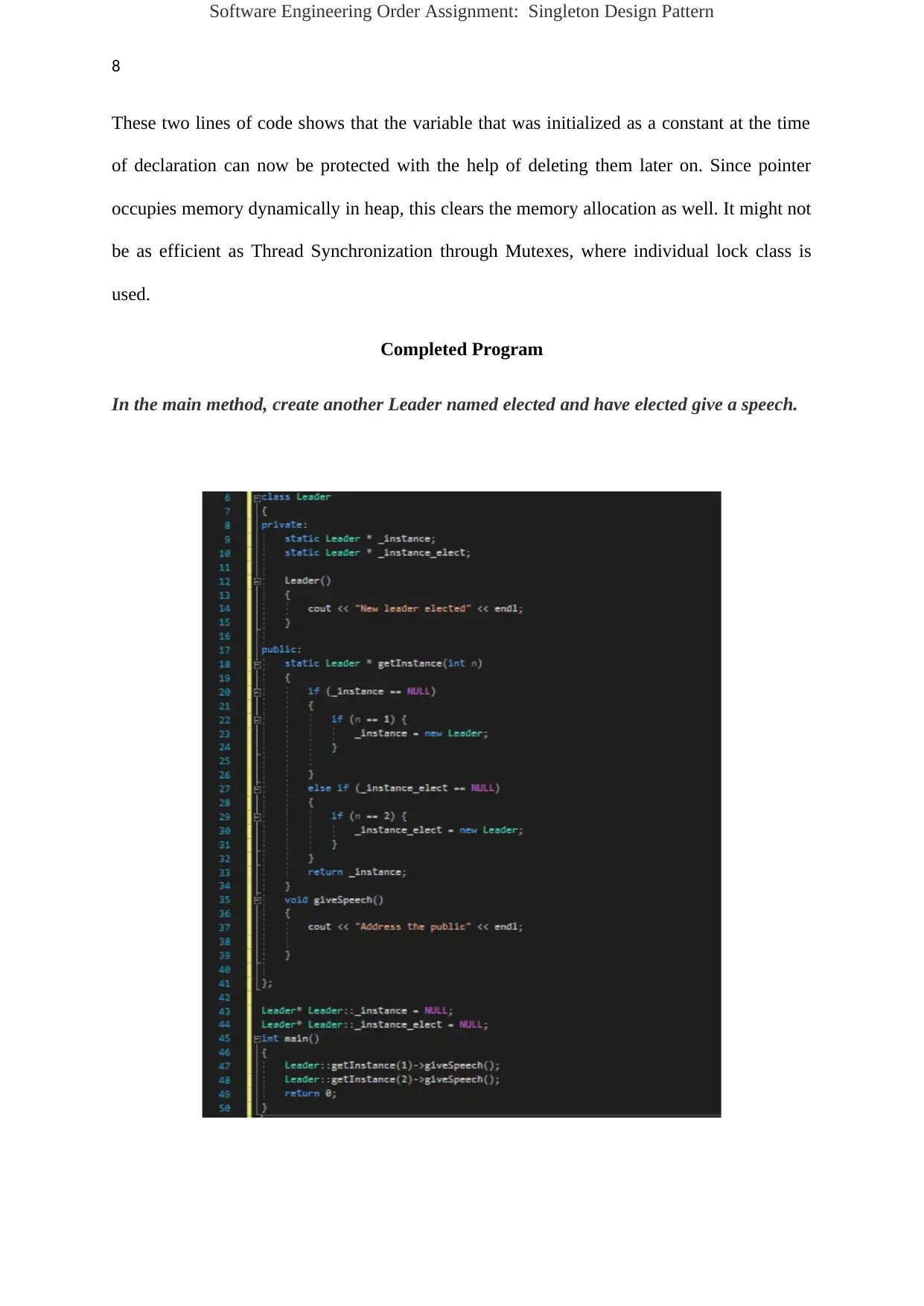
Software Engineering Order Assignment: Singleton Design Pattern
8
These two lines of code shows that the variable that was initialized as a constant at the time
of declaration can now be protected with the help of deleting them later on. Since pointer
occupies memory dynamically in heap, this clears the memory allocation as well. It might not
be as efficient as Thread Synchronization through Mutexes, where individual lock class is
used.
Completed Program
In the main method, create another Leader named elected and have elected give a speech.
8
These two lines of code shows that the variable that was initialized as a constant at the time
of declaration can now be protected with the help of deleting them later on. Since pointer
occupies memory dynamically in heap, this clears the memory allocation as well. It might not
be as efficient as Thread Synchronization through Mutexes, where individual lock class is
used.
Completed Program
In the main method, create another Leader named elected and have elected give a speech.
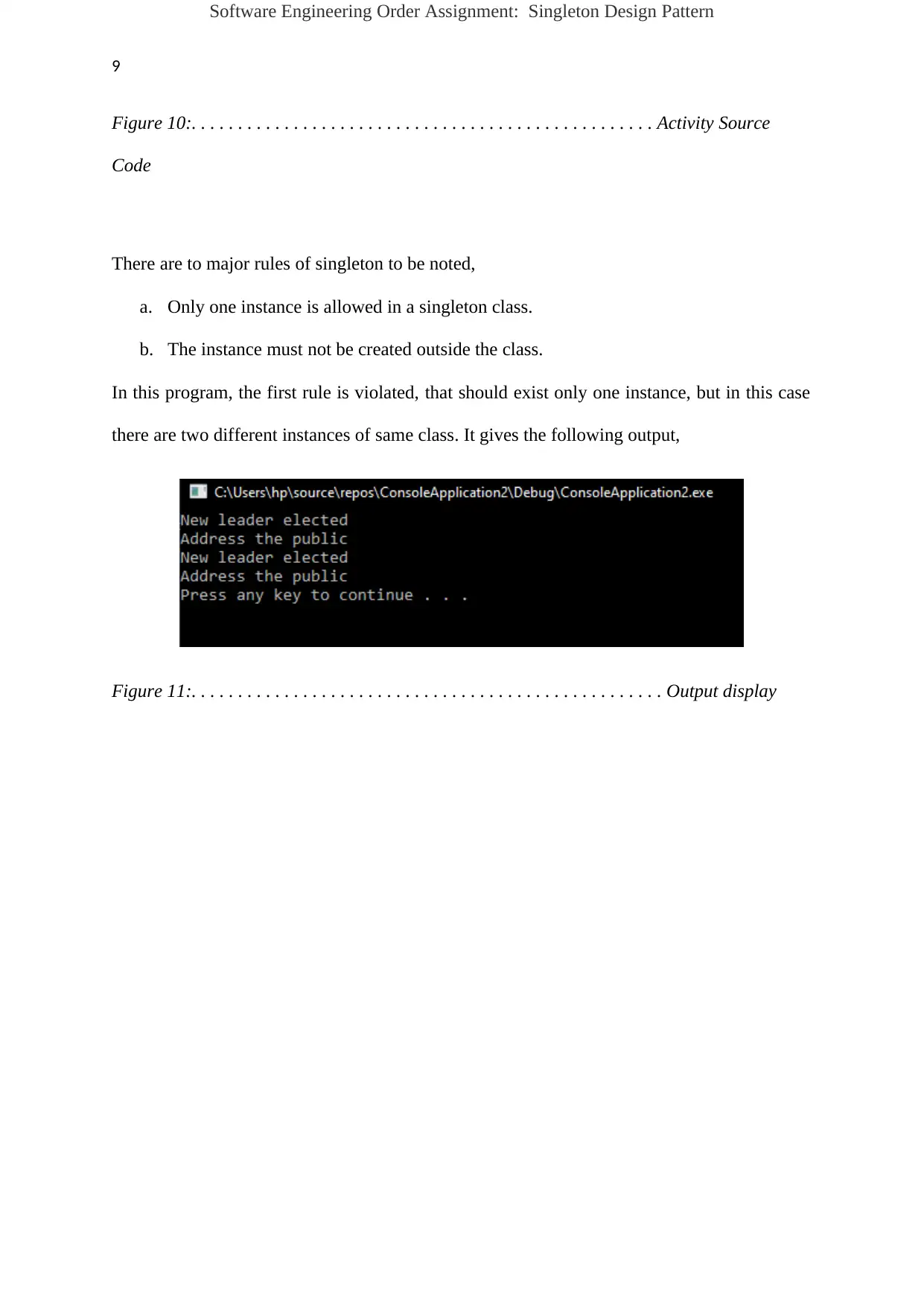
Software Engineering Order Assignment: Singleton Design Pattern
9
Figure 10:. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . Activity Source
Code
There are to major rules of singleton to be noted,
a. Only one instance is allowed in a singleton class.
b. The instance must not be created outside the class.
In this program, the first rule is violated, that should exist only one instance, but in this case
there are two different instances of same class. It gives the following output,
Figure 11:. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . Output display
9
Figure 10:. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . Activity Source
Code
There are to major rules of singleton to be noted,
a. Only one instance is allowed in a singleton class.
b. The instance must not be created outside the class.
In this program, the first rule is violated, that should exist only one instance, but in this case
there are two different instances of same class. It gives the following output,
Figure 11:. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . Output display
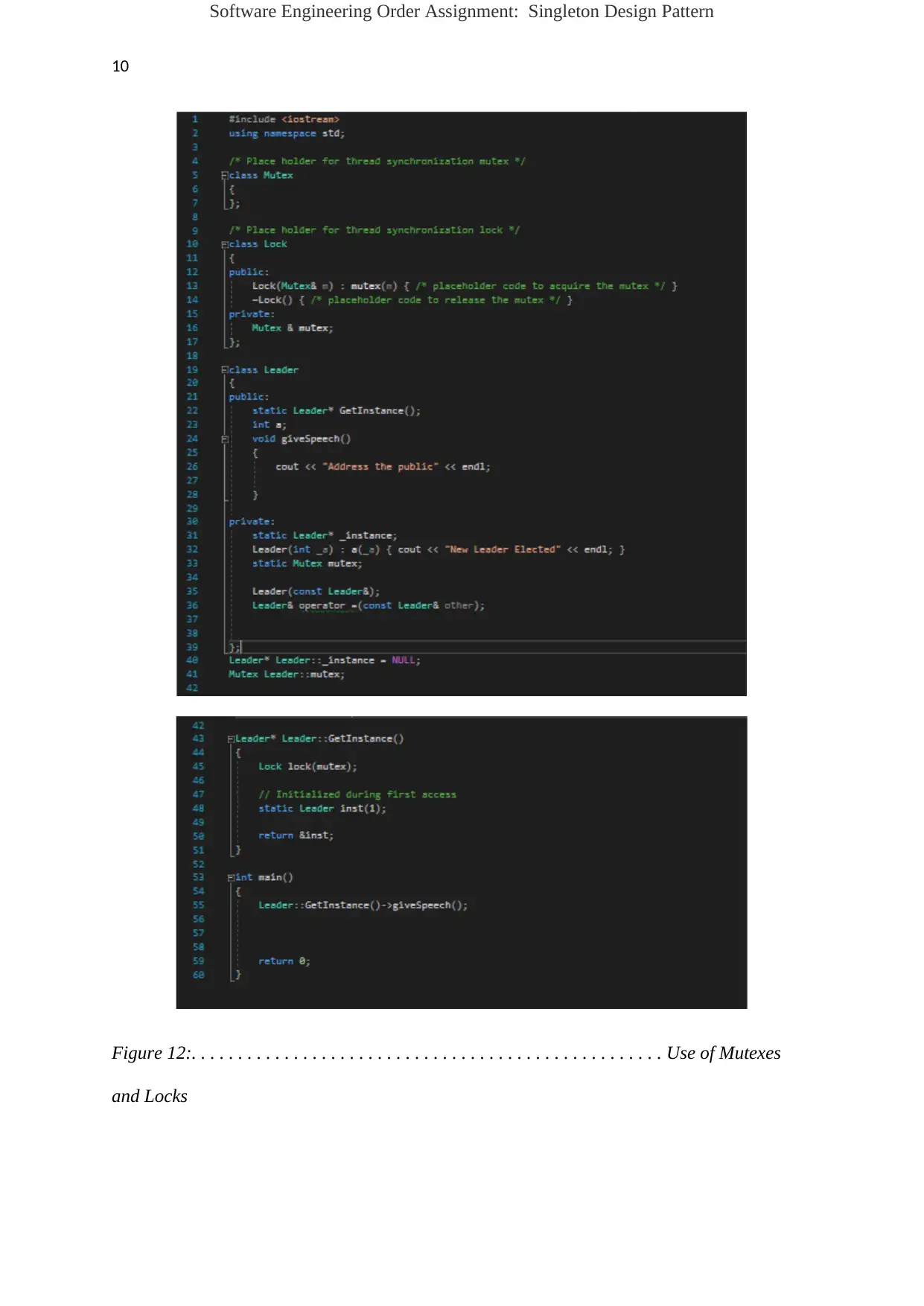
Software Engineering Order Assignment: Singleton Design Pattern
10
Figure 12:. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . Use of Mutexes
and Locks
10
Figure 12:. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . Use of Mutexes
and Locks
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
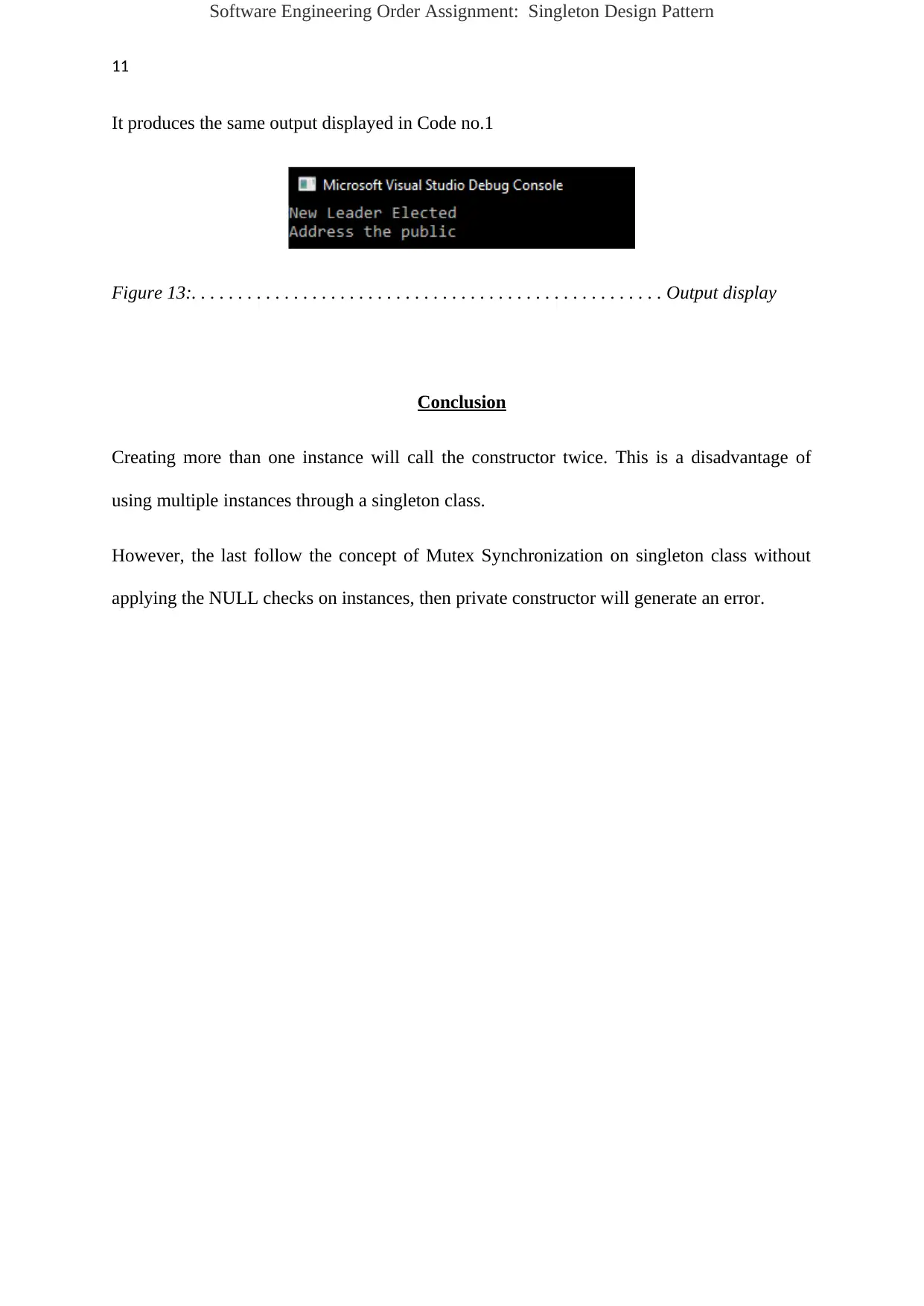
Software Engineering Order Assignment: Singleton Design Pattern
11
It produces the same output displayed in Code no.1
Figure 13:. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . Output display
Conclusion
Creating more than one instance will call the constructor twice. This is a disadvantage of
using multiple instances through a singleton class.
However, the last follow the concept of Mutex Synchronization on singleton class without
applying the NULL checks on instances, then private constructor will generate an error.
11
It produces the same output displayed in Code no.1
Figure 13:. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . Output display
Conclusion
Creating more than one instance will call the constructor twice. This is a disadvantage of
using multiple instances through a singleton class.
However, the last follow the concept of Mutex Synchronization on singleton class without
applying the NULL checks on instances, then private constructor will generate an error.
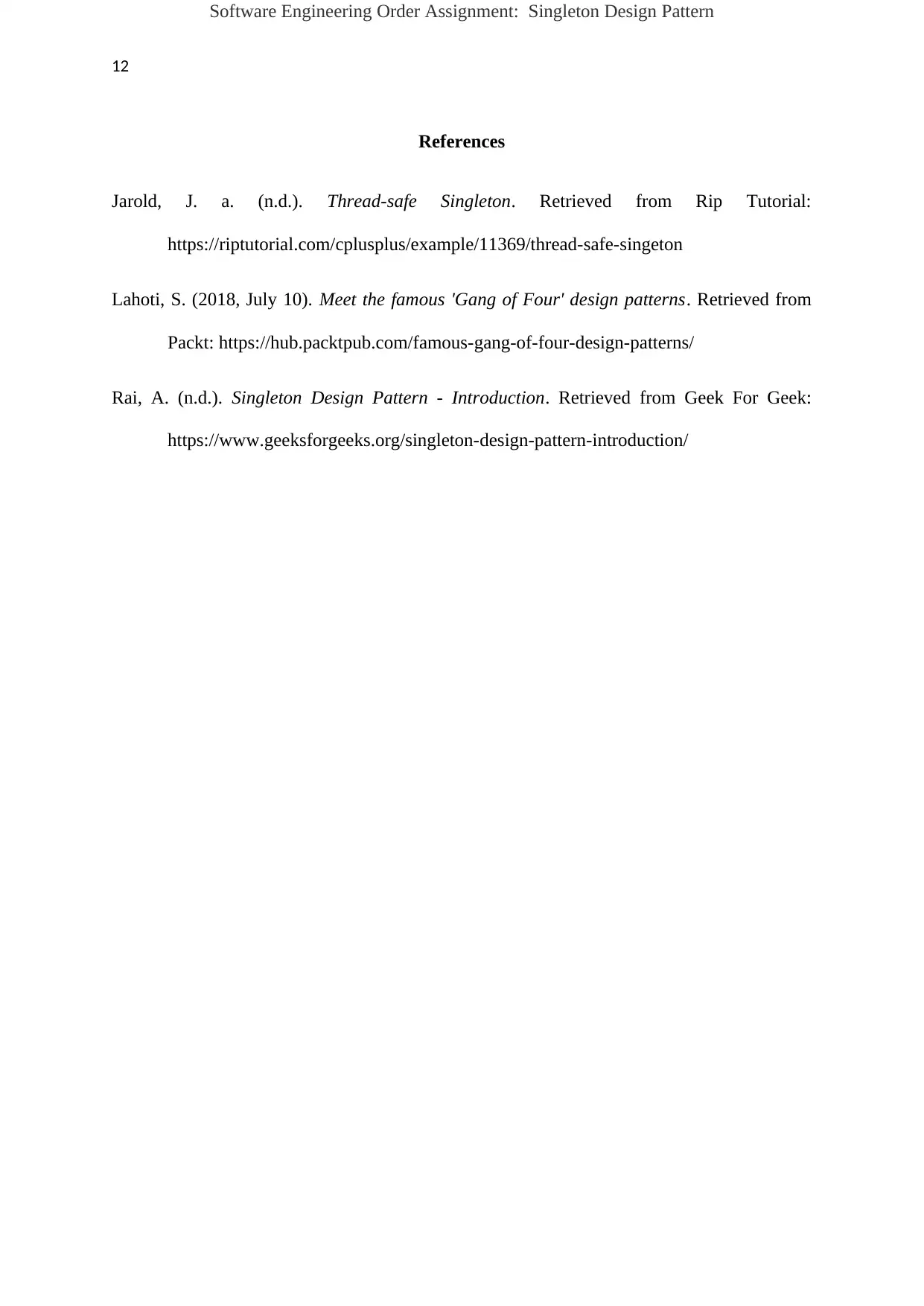
Software Engineering Order Assignment: Singleton Design Pattern
12
References
Jarold, J. a. (n.d.). Thread-safe Singleton. Retrieved from Rip Tutorial:
https://riptutorial.com/cplusplus/example/11369/thread-safe-singeton
Lahoti, S. (2018, July 10). Meet the famous 'Gang of Four' design patterns. Retrieved from
Packt: https://hub.packtpub.com/famous-gang-of-four-design-patterns/
Rai, A. (n.d.). Singleton Design Pattern - Introduction. Retrieved from Geek For Geek:
https://www.geeksforgeeks.org/singleton-design-pattern-introduction/
12
References
Jarold, J. a. (n.d.). Thread-safe Singleton. Retrieved from Rip Tutorial:
https://riptutorial.com/cplusplus/example/11369/thread-safe-singeton
Lahoti, S. (2018, July 10). Meet the famous 'Gang of Four' design patterns. Retrieved from
Packt: https://hub.packtpub.com/famous-gang-of-four-design-patterns/
Rai, A. (n.d.). Singleton Design Pattern - Introduction. Retrieved from Geek For Geek:
https://www.geeksforgeeks.org/singleton-design-pattern-introduction/
1 out of 12
Related Documents

Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.