Advanced Programming: OOP Concepts, Design Patterns, UML Diagrams
VerifiedAdded on 2021/05/07
|28
|9269
|140
Report
AI Summary
This report provides a comprehensive overview of advanced programming concepts, focusing on Object-Oriented Programming (OOP) principles. It explores key concepts such as encapsulation, abstraction, inheritance, and polymorphism, explaining their significance and implementation. The report a...
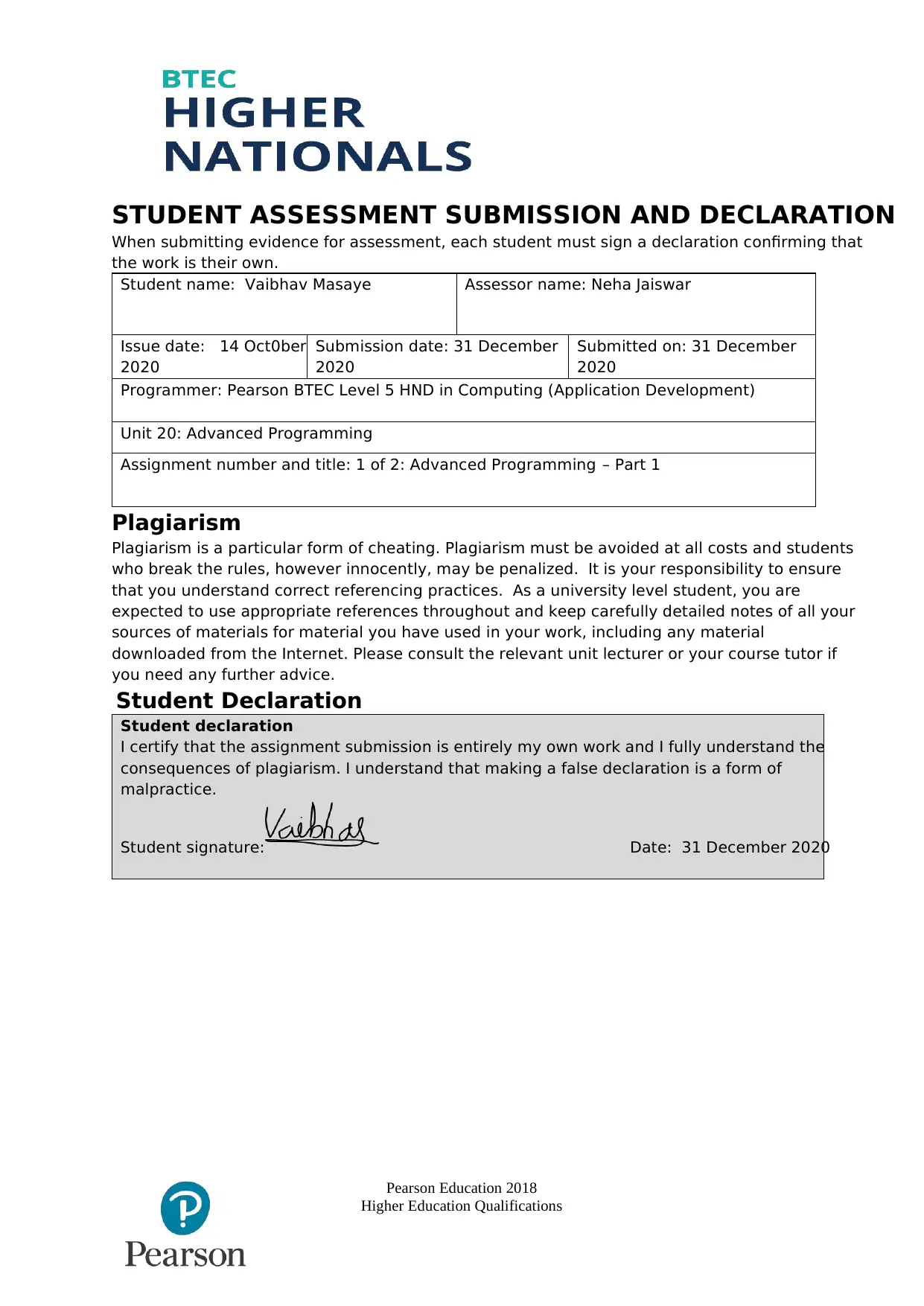
Pearson Education 2018
Higher Education Qualifications
STUDENT ASSESSMENT SUBMISSION AND DECLARATION
When submitting evidence for assessment, each student must sign a declaration confirming that
the work is their own.
Student name: Vaibhav Masaye Assessor name: Neha Jaiswar
Issue date: 14 Oct0ber
2020
Submission date: 31 December
2020
Submitted on: 31 December
2020
Programmer: Pearson BTEC Level 5 HND in Computing (Application Development)
Unit 20: Advanced Programming
Assignment number and title: 1 of 2: Advanced Programming – Part 1
Plagiarism
Plagiarism is a particular form of cheating. Plagiarism must be avoided at all costs and students
who break the rules, however innocently, may be penalized. It is your responsibility to ensure
that you understand correct referencing practices. As a university level student, you are
expected to use appropriate references throughout and keep carefully detailed notes of all your
sources of materials for material you have used in your work, including any material
downloaded from the Internet. Please consult the relevant unit lecturer or your course tutor if
you need any further advice.
Student Declaration
Student declaration
I certify that the assignment submission is entirely my own work and I fully understand the
consequences of plagiarism. I understand that making a false declaration is a form of
malpractice.
Student signature: Date: 31 December 2020
Higher Education Qualifications
STUDENT ASSESSMENT SUBMISSION AND DECLARATION
When submitting evidence for assessment, each student must sign a declaration confirming that
the work is their own.
Student name: Vaibhav Masaye Assessor name: Neha Jaiswar
Issue date: 14 Oct0ber
2020
Submission date: 31 December
2020
Submitted on: 31 December
2020
Programmer: Pearson BTEC Level 5 HND in Computing (Application Development)
Unit 20: Advanced Programming
Assignment number and title: 1 of 2: Advanced Programming – Part 1
Plagiarism
Plagiarism is a particular form of cheating. Plagiarism must be avoided at all costs and students
who break the rules, however innocently, may be penalized. It is your responsibility to ensure
that you understand correct referencing practices. As a university level student, you are
expected to use appropriate references throughout and keep carefully detailed notes of all your
sources of materials for material you have used in your work, including any material
downloaded from the Internet. Please consult the relevant unit lecturer or your course tutor if
you need any further advice.
Student Declaration
Student declaration
I certify that the assignment submission is entirely my own work and I fully understand the
consequences of plagiarism. I understand that making a false declaration is a form of
malpractice.
Student signature: Date: 31 December 2020
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
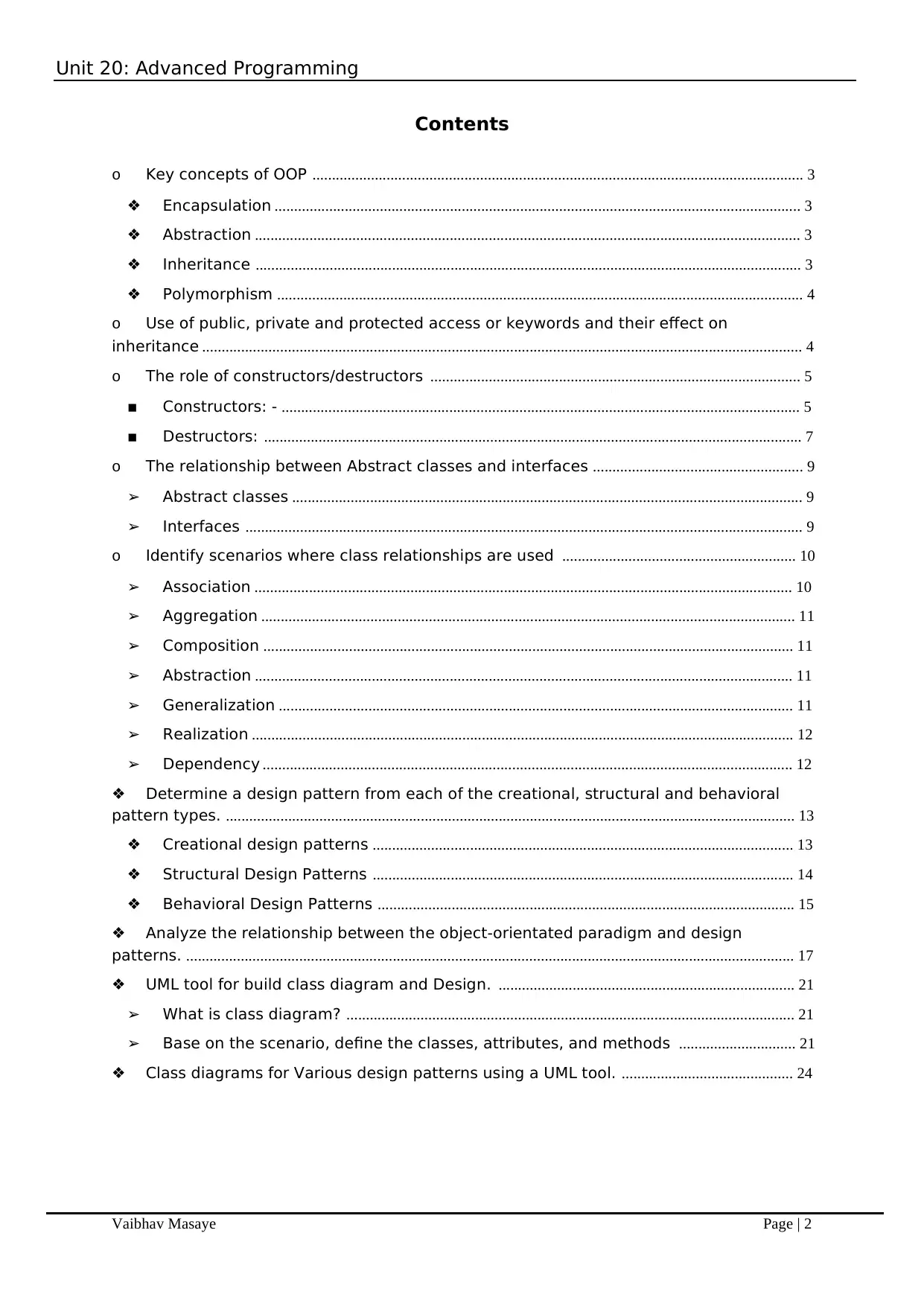
Unit 20: Advanced Programming
Vaibhav Masaye Page | 2
Contents
o Key concepts of OOP .............................................................................................................................. 3
❖ Encapsulation ....................................................................................................................................... 3
❖ Abstraction ............................................................................................................................................ 3
❖ Inheritance ............................................................................................................................................ 3
❖ Polymorphism ....................................................................................................................................... 4
o Use of public, private and protected access or keywords and their effect on
inheritance .......................................................................................................................................................... 4
o The role of constructors/destructors ............................................................................................... 5
▪ Constructors: - ..................................................................................................................................... 5
▪ Destructors: .......................................................................................................................................... 7
o The relationship between Abstract classes and interfaces ...................................................... 9
➢ Abstract classes ................................................................................................................................... 9
➢ Interfaces ............................................................................................................................................... 9
o Identify scenarios where class relationships are used ............................................................ 10
➢ Association .......................................................................................................................................... 10
➢ Aggregation ......................................................................................................................................... 11
➢ Composition ........................................................................................................................................ 11
➢ Abstraction .......................................................................................................................................... 11
➢ Generalization .................................................................................................................................... 11
➢ Realization ........................................................................................................................................... 12
➢ Dependency ........................................................................................................................................ 12
❖ Determine a design pattern from each of the creational, structural and behavioral
pattern types. .................................................................................................................................................. 13
❖ Creational design patterns ............................................................................................................ 13
❖ Structural Design Patterns ............................................................................................................ 14
❖ Behavioral Design Patterns ........................................................................................................... 15
❖ Analyze the relationship between the object-orientated paradigm and design
patterns. ............................................................................................................................................................ 17
❖ UML tool for build class diagram and Design. ............................................................................ 21
➢ What is class diagram? ................................................................................................................... 21
➢ Base on the scenario, define the classes, attributes, and methods .............................. 21
❖ Class diagrams for Various design patterns using a UML tool. ............................................ 24
Vaibhav Masaye Page | 2
Contents
o Key concepts of OOP .............................................................................................................................. 3
❖ Encapsulation ....................................................................................................................................... 3
❖ Abstraction ............................................................................................................................................ 3
❖ Inheritance ............................................................................................................................................ 3
❖ Polymorphism ....................................................................................................................................... 4
o Use of public, private and protected access or keywords and their effect on
inheritance .......................................................................................................................................................... 4
o The role of constructors/destructors ............................................................................................... 5
▪ Constructors: - ..................................................................................................................................... 5
▪ Destructors: .......................................................................................................................................... 7
o The relationship between Abstract classes and interfaces ...................................................... 9
➢ Abstract classes ................................................................................................................................... 9
➢ Interfaces ............................................................................................................................................... 9
o Identify scenarios where class relationships are used ............................................................ 10
➢ Association .......................................................................................................................................... 10
➢ Aggregation ......................................................................................................................................... 11
➢ Composition ........................................................................................................................................ 11
➢ Abstraction .......................................................................................................................................... 11
➢ Generalization .................................................................................................................................... 11
➢ Realization ........................................................................................................................................... 12
➢ Dependency ........................................................................................................................................ 12
❖ Determine a design pattern from each of the creational, structural and behavioral
pattern types. .................................................................................................................................................. 13
❖ Creational design patterns ............................................................................................................ 13
❖ Structural Design Patterns ............................................................................................................ 14
❖ Behavioral Design Patterns ........................................................................................................... 15
❖ Analyze the relationship between the object-orientated paradigm and design
patterns. ............................................................................................................................................................ 17
❖ UML tool for build class diagram and Design. ............................................................................ 21
➢ What is class diagram? ................................................................................................................... 21
➢ Base on the scenario, define the classes, attributes, and methods .............................. 21
❖ Class diagrams for Various design patterns using a UML tool. ............................................ 24
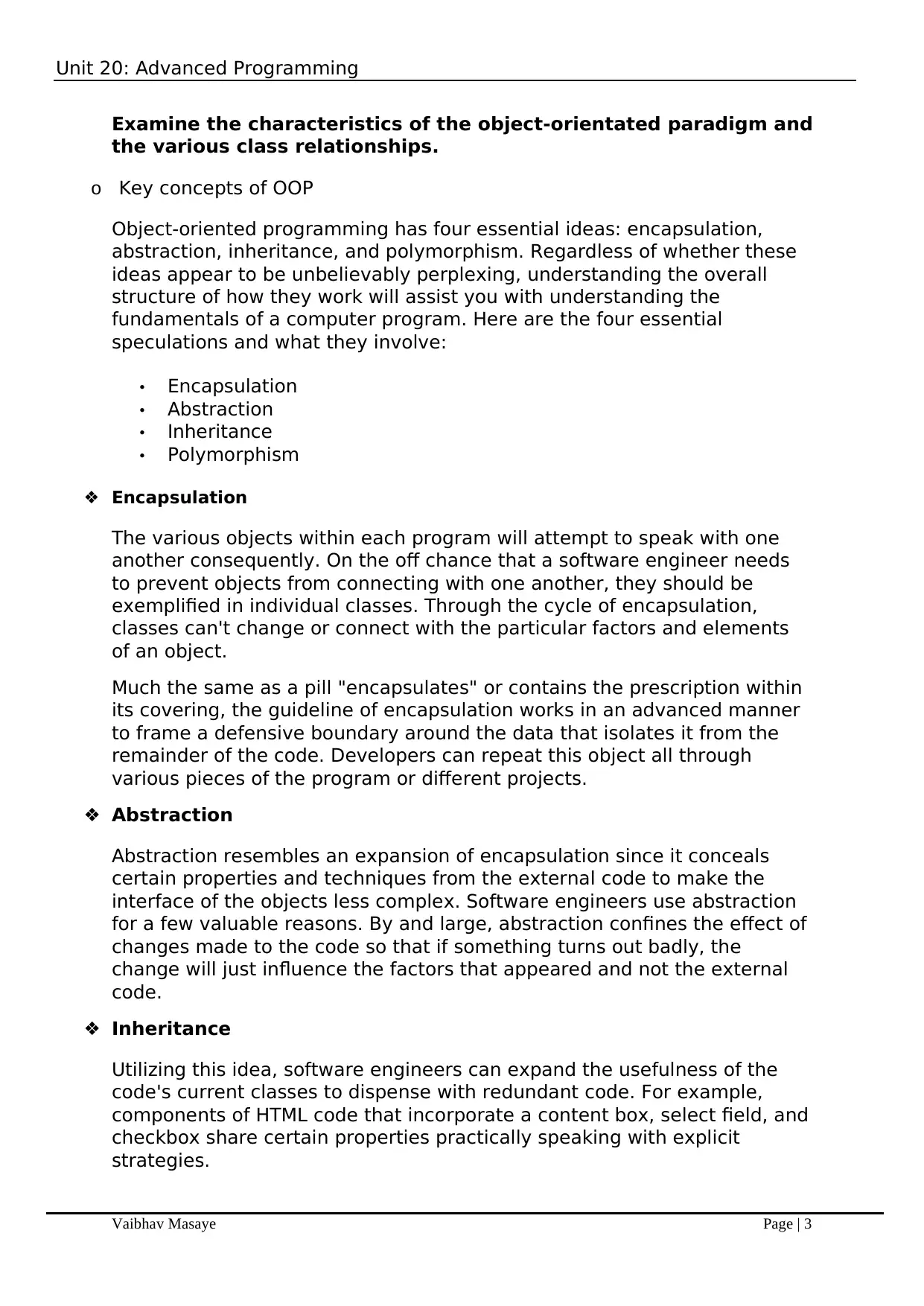
Unit 20: Advanced Programming
Vaibhav Masaye Page | 3
Examine the characteristics of the object-orientated paradigm and
the various class relationships.
o Key concepts of OOP
Object-oriented programming has four essential ideas: encapsulation,
abstraction, inheritance, and polymorphism. Regardless of whether these
ideas appear to be unbelievably perplexing, understanding the overall
structure of how they work will assist you with understanding the
fundamentals of a computer program. Here are the four essential
speculations and what they involve:
• Encapsulation
• Abstraction
• Inheritance
• Polymorphism
❖ Encapsulation
The various objects within each program will attempt to speak with one
another consequently. On the off chance that a software engineer needs
to prevent objects from connecting with one another, they should be
exemplified in individual classes. Through the cycle of encapsulation,
classes can't change or connect with the particular factors and elements
of an object.
Much the same as a pill "encapsulates" or contains the prescription within
its covering, the guideline of encapsulation works in an advanced manner
to frame a defensive boundary around the data that isolates it from the
remainder of the code. Developers can repeat this object all through
various pieces of the program or different projects.
❖ Abstraction
Abstraction resembles an expansion of encapsulation since it conceals
certain properties and techniques from the external code to make the
interface of the objects less complex. Software engineers use abstraction
for a few valuable reasons. By and large, abstraction confines the effect of
changes made to the code so that if something turns out badly, the
change will just influence the factors that appeared and not the external
code.
❖ Inheritance
Utilizing this idea, software engineers can expand the usefulness of the
code's current classes to dispense with redundant code. For example,
components of HTML code that incorporate a content box, select field, and
checkbox share certain properties practically speaking with explicit
strategies.
Vaibhav Masaye Page | 3
Examine the characteristics of the object-orientated paradigm and
the various class relationships.
o Key concepts of OOP
Object-oriented programming has four essential ideas: encapsulation,
abstraction, inheritance, and polymorphism. Regardless of whether these
ideas appear to be unbelievably perplexing, understanding the overall
structure of how they work will assist you with understanding the
fundamentals of a computer program. Here are the four essential
speculations and what they involve:
• Encapsulation
• Abstraction
• Inheritance
• Polymorphism
❖ Encapsulation
The various objects within each program will attempt to speak with one
another consequently. On the off chance that a software engineer needs
to prevent objects from connecting with one another, they should be
exemplified in individual classes. Through the cycle of encapsulation,
classes can't change or connect with the particular factors and elements
of an object.
Much the same as a pill "encapsulates" or contains the prescription within
its covering, the guideline of encapsulation works in an advanced manner
to frame a defensive boundary around the data that isolates it from the
remainder of the code. Developers can repeat this object all through
various pieces of the program or different projects.
❖ Abstraction
Abstraction resembles an expansion of encapsulation since it conceals
certain properties and techniques from the external code to make the
interface of the objects less complex. Software engineers use abstraction
for a few valuable reasons. By and large, abstraction confines the effect of
changes made to the code so that if something turns out badly, the
change will just influence the factors that appeared and not the external
code.
❖ Inheritance
Utilizing this idea, software engineers can expand the usefulness of the
code's current classes to dispense with redundant code. For example,
components of HTML code that incorporate a content box, select field, and
checkbox share certain properties practically speaking with explicit
strategies.
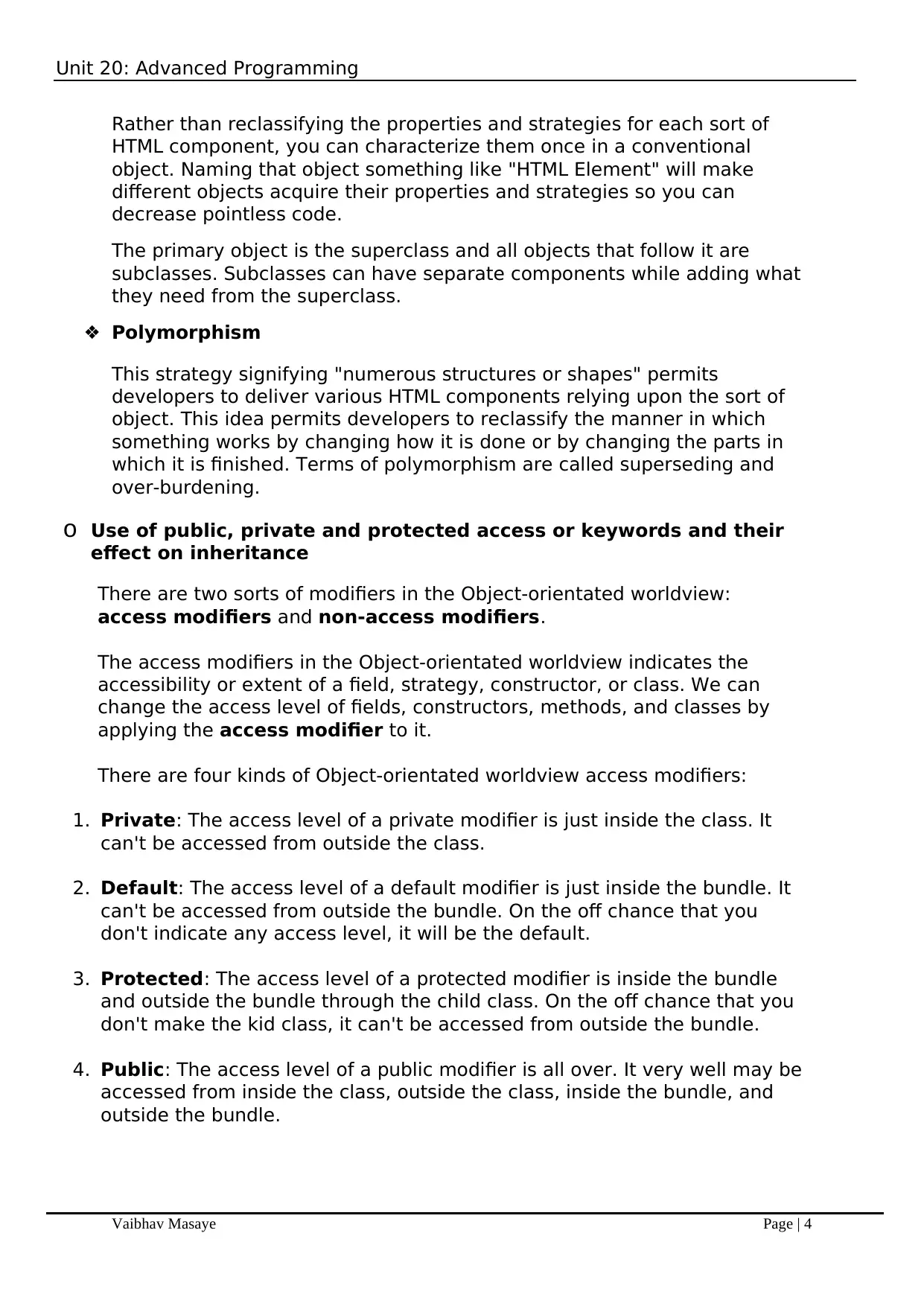
Unit 20: Advanced Programming
Vaibhav Masaye Page | 4
Rather than reclassifying the properties and strategies for each sort of
HTML component, you can characterize them once in a conventional
object. Naming that object something like "HTML Element" will make
different objects acquire their properties and strategies so you can
decrease pointless code.
The primary object is the superclass and all objects that follow it are
subclasses. Subclasses can have separate components while adding what
they need from the superclass.
❖ Polymorphism
This strategy signifying "numerous structures or shapes" permits
developers to deliver various HTML components relying upon the sort of
object. This idea permits developers to reclassify the manner in which
something works by changing how it is done or by changing the parts in
which it is finished. Terms of polymorphism are called superseding and
over-burdening.
o Use of public, private and protected access or keywords and their
effect on inheritance
There are two sorts of modifiers in the Object-orientated worldview:
access modifiers and non-access modifiers.
The access modifiers in the Object-orientated worldview indicates the
accessibility or extent of a field, strategy, constructor, or class. We can
change the access level of fields, constructors, methods, and classes by
applying the access modifier to it.
There are four kinds of Object-orientated worldview access modifiers:
1. Private: The access level of a private modifier is just inside the class. It
can't be accessed from outside the class.
2. Default: The access level of a default modifier is just inside the bundle. It
can't be accessed from outside the bundle. On the off chance that you
don't indicate any access level, it will be the default.
3. Protected: The access level of a protected modifier is inside the bundle
and outside the bundle through the child class. On the off chance that you
don't make the kid class, it can't be accessed from outside the bundle.
4. Public: The access level of a public modifier is all over. It very well may be
accessed from inside the class, outside the class, inside the bundle, and
outside the bundle.
Vaibhav Masaye Page | 4
Rather than reclassifying the properties and strategies for each sort of
HTML component, you can characterize them once in a conventional
object. Naming that object something like "HTML Element" will make
different objects acquire their properties and strategies so you can
decrease pointless code.
The primary object is the superclass and all objects that follow it are
subclasses. Subclasses can have separate components while adding what
they need from the superclass.
❖ Polymorphism
This strategy signifying "numerous structures or shapes" permits
developers to deliver various HTML components relying upon the sort of
object. This idea permits developers to reclassify the manner in which
something works by changing how it is done or by changing the parts in
which it is finished. Terms of polymorphism are called superseding and
over-burdening.
o Use of public, private and protected access or keywords and their
effect on inheritance
There are two sorts of modifiers in the Object-orientated worldview:
access modifiers and non-access modifiers.
The access modifiers in the Object-orientated worldview indicates the
accessibility or extent of a field, strategy, constructor, or class. We can
change the access level of fields, constructors, methods, and classes by
applying the access modifier to it.
There are four kinds of Object-orientated worldview access modifiers:
1. Private: The access level of a private modifier is just inside the class. It
can't be accessed from outside the class.
2. Default: The access level of a default modifier is just inside the bundle. It
can't be accessed from outside the bundle. On the off chance that you
don't indicate any access level, it will be the default.
3. Protected: The access level of a protected modifier is inside the bundle
and outside the bundle through the child class. On the off chance that you
don't make the kid class, it can't be accessed from outside the bundle.
4. Public: The access level of a public modifier is all over. It very well may be
accessed from inside the class, outside the class, inside the bundle, and
outside the bundle.
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
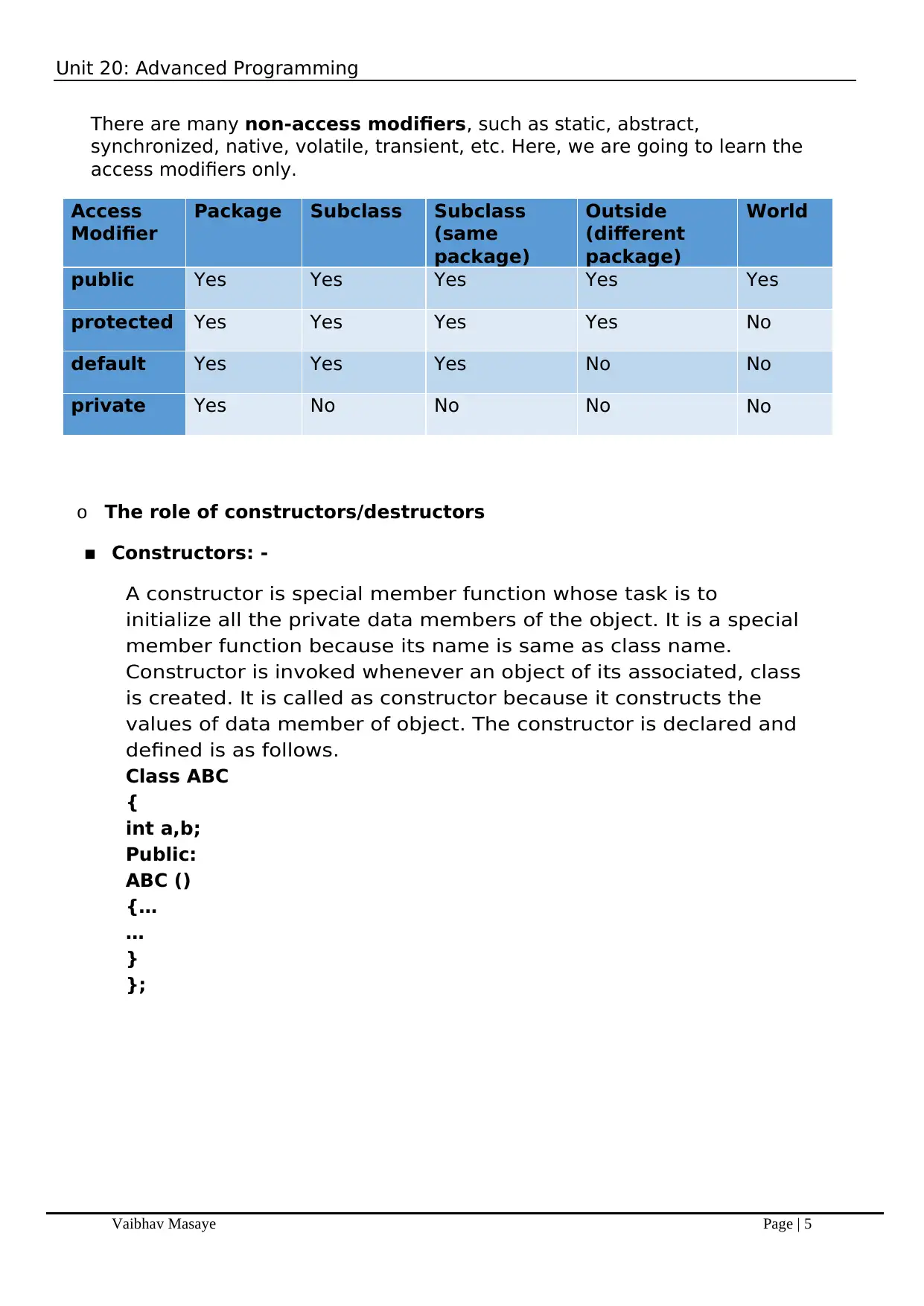
Unit 20: Advanced Programming
Vaibhav Masaye Page | 5
There are many non-access modifiers, such as static, abstract,
synchronized, native, volatile, transient, etc. Here, we are going to learn the
access modifiers only.
Access
Modifier
Package Subclass Subclass
(same
package)
Outside
(different
package)
World
public Yes Yes Yes Yes Yes
protected Yes Yes Yes Yes No
default Yes Yes Yes No No
private Yes No No No No
o The role of constructors/destructors
▪ Constructors: -
A constructor is special member function whose task is to
initialize all the private data members of the object. It is a special
member function because its name is same as class name.
Constructor is invoked whenever an object of its associated, class
is created. It is called as constructor because it constructs the
values of data member of object. The constructor is declared and
defined is as follows.
Class ABC
{
int a,b;
Public:
ABC ()
{…
…
}
};
Vaibhav Masaye Page | 5
There are many non-access modifiers, such as static, abstract,
synchronized, native, volatile, transient, etc. Here, we are going to learn the
access modifiers only.
Access
Modifier
Package Subclass Subclass
(same
package)
Outside
(different
package)
World
public Yes Yes Yes Yes Yes
protected Yes Yes Yes Yes No
default Yes Yes Yes No No
private Yes No No No No
o The role of constructors/destructors
▪ Constructors: -
A constructor is special member function whose task is to
initialize all the private data members of the object. It is a special
member function because its name is same as class name.
Constructor is invoked whenever an object of its associated, class
is created. It is called as constructor because it constructs the
values of data member of object. The constructor is declared and
defined is as follows.
Class ABC
{
int a,b;
Public:
ABC ()
{…
…
}
};
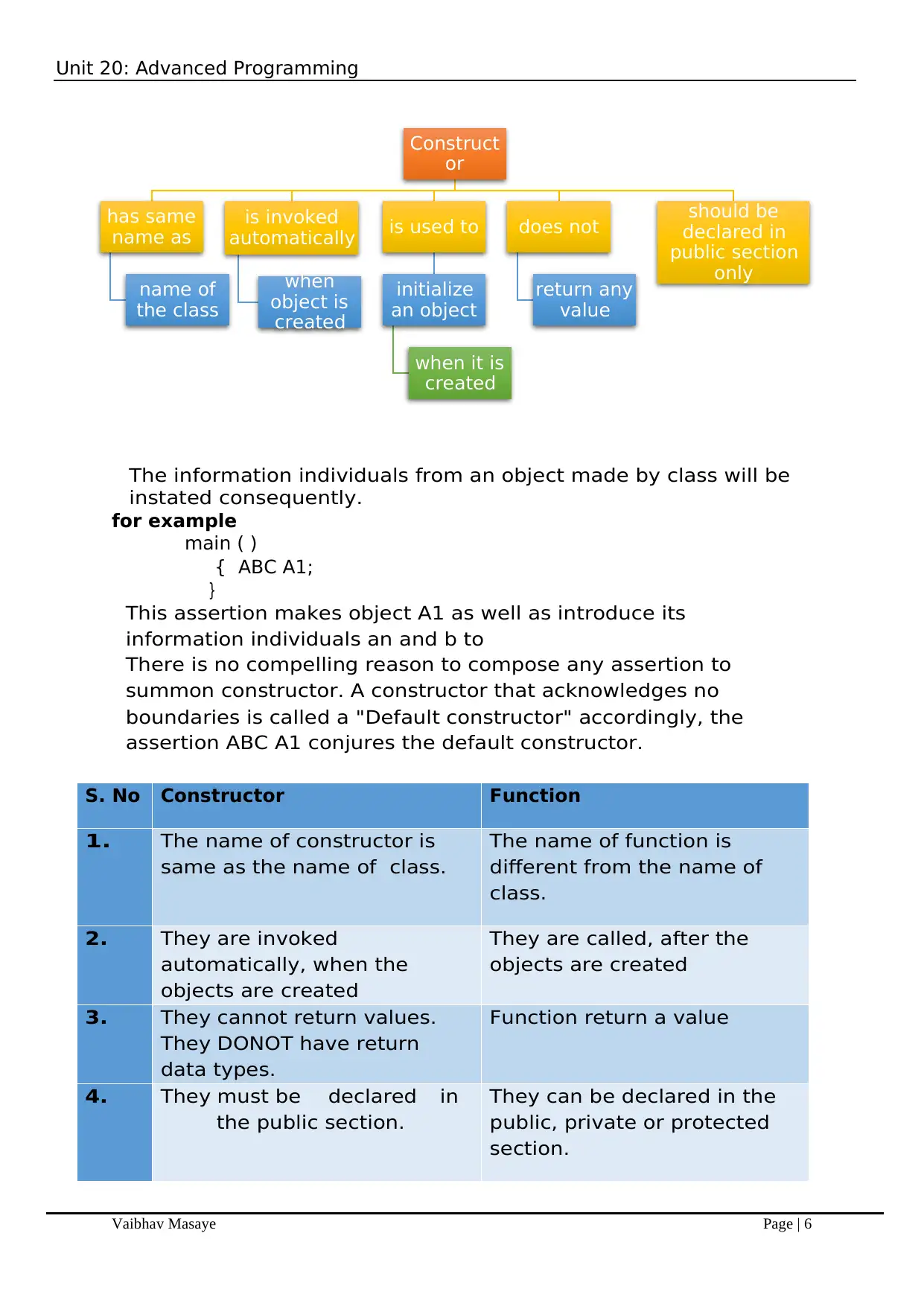
Unit 20: Advanced Programming
Vaibhav Masaye Page | 6
The information individuals from an object made by class will be
instated consequently.
for example
main ( )
{ ABC A1;
}
This assertion makes object A1 as well as introduce its
information individuals an and b to
There is no compelling reason to compose any assertion to
summon constructor. A constructor that acknowledges no
boundaries is called a "Default constructor" accordingly, the
assertion ABC A1 conjures the default constructor.
S. No Constructor Function
1. The name of constructor is
same as the name of class.
The name of function is
different from the name of
class.
2. They are invoked
automatically, when the
objects are created
They are called, after the
objects are created
3. They cannot return values.
They DONOT have return
data types.
Function return a value
4. They must be declared in
the public section.
They can be declared in the
public, private or protected
section.
Construct
or
has same
name as
name of
the class
is invoked
automatically
when
object is
created
is used to
initialize
an object
when it is
created
does not
return any
value
should be
declared in
public section
only
Vaibhav Masaye Page | 6
The information individuals from an object made by class will be
instated consequently.
for example
main ( )
{ ABC A1;
}
This assertion makes object A1 as well as introduce its
information individuals an and b to
There is no compelling reason to compose any assertion to
summon constructor. A constructor that acknowledges no
boundaries is called a "Default constructor" accordingly, the
assertion ABC A1 conjures the default constructor.
S. No Constructor Function
1. The name of constructor is
same as the name of class.
The name of function is
different from the name of
class.
2. They are invoked
automatically, when the
objects are created
They are called, after the
objects are created
3. They cannot return values.
They DONOT have return
data types.
Function return a value
4. They must be declared in
the public section.
They can be declared in the
public, private or protected
section.
Construct
or
has same
name as
name of
the class
is invoked
automatically
when
object is
created
is used to
initialize
an object
when it is
created
does not
return any
value
should be
declared in
public section
only
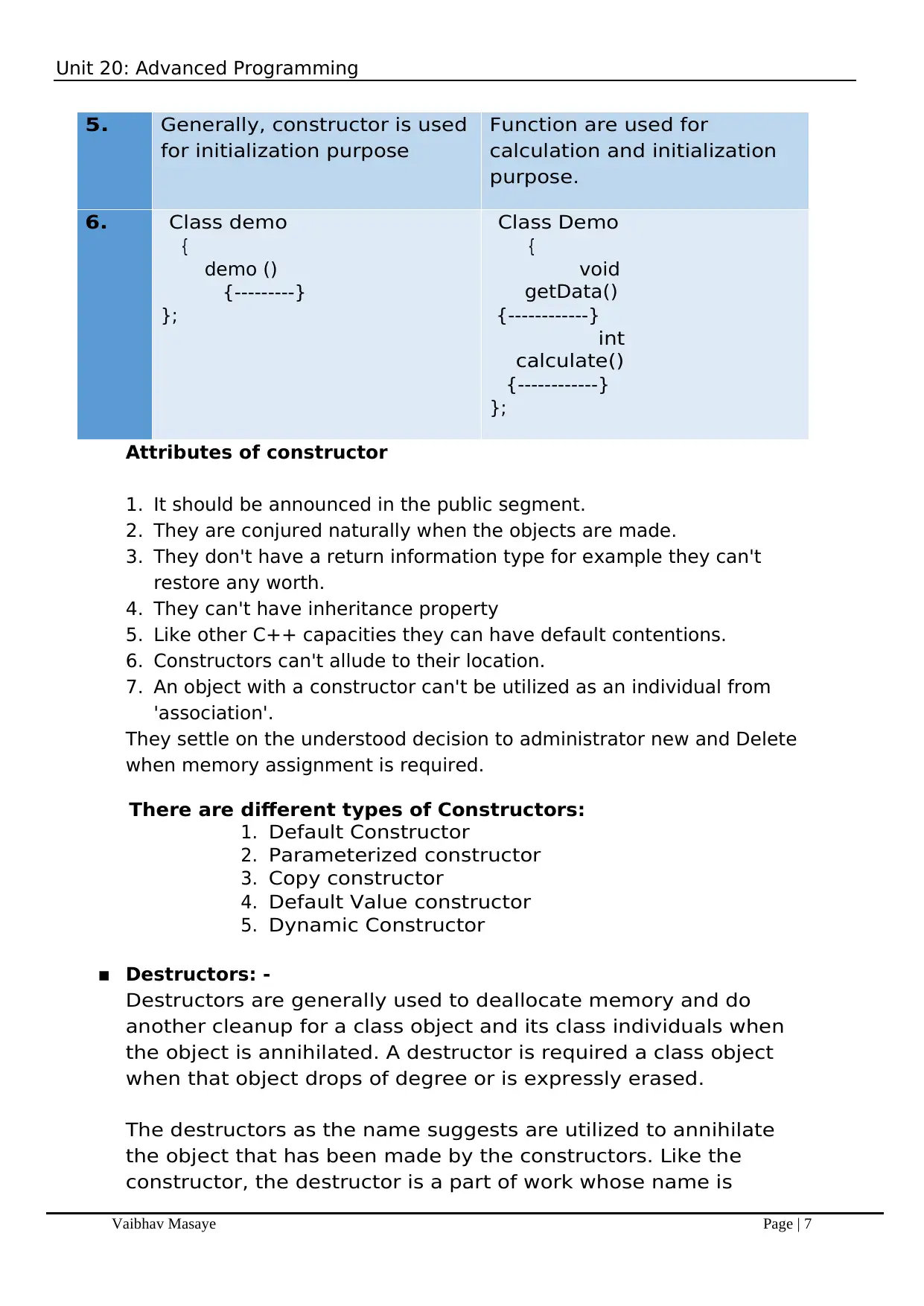
Unit 20: Advanced Programming
Vaibhav Masaye Page | 7
5. Generally, constructor is used
for initialization purpose
Function are used for
calculation and initialization
purpose.
6. Class demo
{
demo ()
{---------}
};
Class Demo
{
void
getData()
{------------}
int
calculate()
{------------}
};
Attributes of constructor
1. It should be announced in the public segment.
2. They are conjured naturally when the objects are made.
3. They don't have a return information type for example they can't
restore any worth.
4. They can't have inheritance property
5. Like other C++ capacities they can have default contentions.
6. Constructors can't allude to their location.
7. An object with a constructor can't be utilized as an individual from
'association'.
They settle on the understood decision to administrator new and Delete
when memory assignment is required.
There are different types of Constructors:
1. Default Constructor
2. Parameterized constructor
3. Copy constructor
4. Default Value constructor
5. Dynamic Constructor
▪ Destructors: -
Destructors are generally used to deallocate memory and do
another cleanup for a class object and its class individuals when
the object is annihilated. A destructor is required a class object
when that object drops of degree or is expressly erased.
The destructors as the name suggests are utilized to annihilate
the object that has been made by the constructors. Like the
constructor, the destructor is a part of work whose name is
Vaibhav Masaye Page | 7
5. Generally, constructor is used
for initialization purpose
Function are used for
calculation and initialization
purpose.
6. Class demo
{
demo ()
{---------}
};
Class Demo
{
void
getData()
{------------}
int
calculate()
{------------}
};
Attributes of constructor
1. It should be announced in the public segment.
2. They are conjured naturally when the objects are made.
3. They don't have a return information type for example they can't
restore any worth.
4. They can't have inheritance property
5. Like other C++ capacities they can have default contentions.
6. Constructors can't allude to their location.
7. An object with a constructor can't be utilized as an individual from
'association'.
They settle on the understood decision to administrator new and Delete
when memory assignment is required.
There are different types of Constructors:
1. Default Constructor
2. Parameterized constructor
3. Copy constructor
4. Default Value constructor
5. Dynamic Constructor
▪ Destructors: -
Destructors are generally used to deallocate memory and do
another cleanup for a class object and its class individuals when
the object is annihilated. A destructor is required a class object
when that object drops of degree or is expressly erased.
The destructors as the name suggests are utilized to annihilate
the object that has been made by the constructors. Like the
constructor, the destructor is a part of work whose name is
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
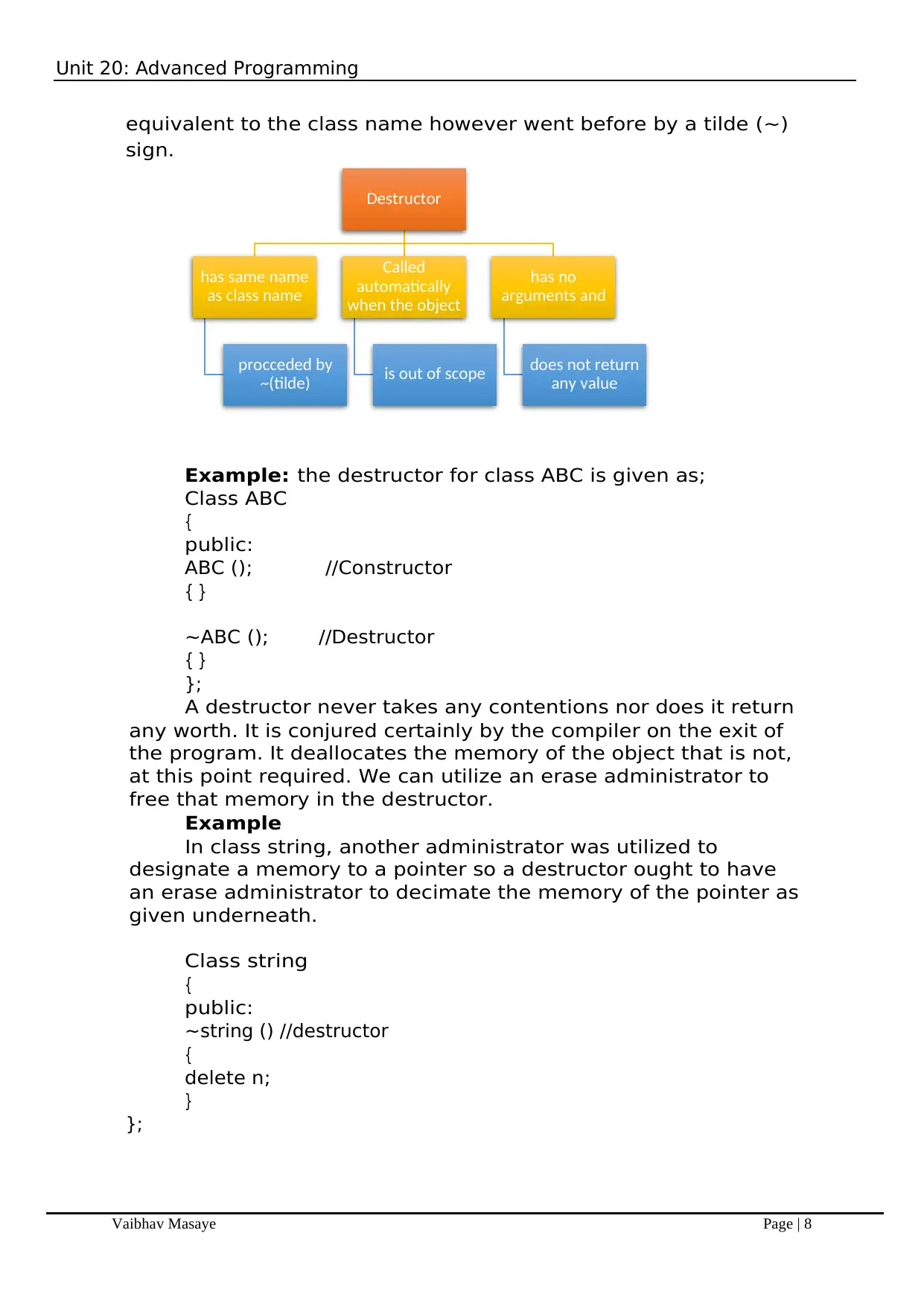
Unit 20: Advanced Programming
Vaibhav Masaye Page | 8
equivalent to the class name however went before by a tilde (~)
sign.
Example: the destructor for class ABC is given as;
Class ABC
{
public:
ABC (); //Constructor
{ }
~ABC (); //Destructor
{ }
};
A destructor never takes any contentions nor does it return
any worth. It is conjured certainly by the compiler on the exit of
the program. It deallocates the memory of the object that is not,
at this point required. We can utilize an erase administrator to
free that memory in the destructor.
Example
In class string, another administrator was utilized to
designate a memory to a pointer so a destructor ought to have
an erase administrator to decimate the memory of the pointer as
given underneath.
Class string
{
public:
~string () //destructor
{
delete n;
}
};
Destructor
has same name
as class name
procceded by
~(tilde)
Called
automatically
when the object
is out of scope
has no
arguments and
does not return
any value
Vaibhav Masaye Page | 8
equivalent to the class name however went before by a tilde (~)
sign.
Example: the destructor for class ABC is given as;
Class ABC
{
public:
ABC (); //Constructor
{ }
~ABC (); //Destructor
{ }
};
A destructor never takes any contentions nor does it return
any worth. It is conjured certainly by the compiler on the exit of
the program. It deallocates the memory of the object that is not,
at this point required. We can utilize an erase administrator to
free that memory in the destructor.
Example
In class string, another administrator was utilized to
designate a memory to a pointer so a destructor ought to have
an erase administrator to decimate the memory of the pointer as
given underneath.
Class string
{
public:
~string () //destructor
{
delete n;
}
};
Destructor
has same name
as class name
procceded by
~(tilde)
Called
automatically
when the object
is out of scope
has no
arguments and
does not return
any value
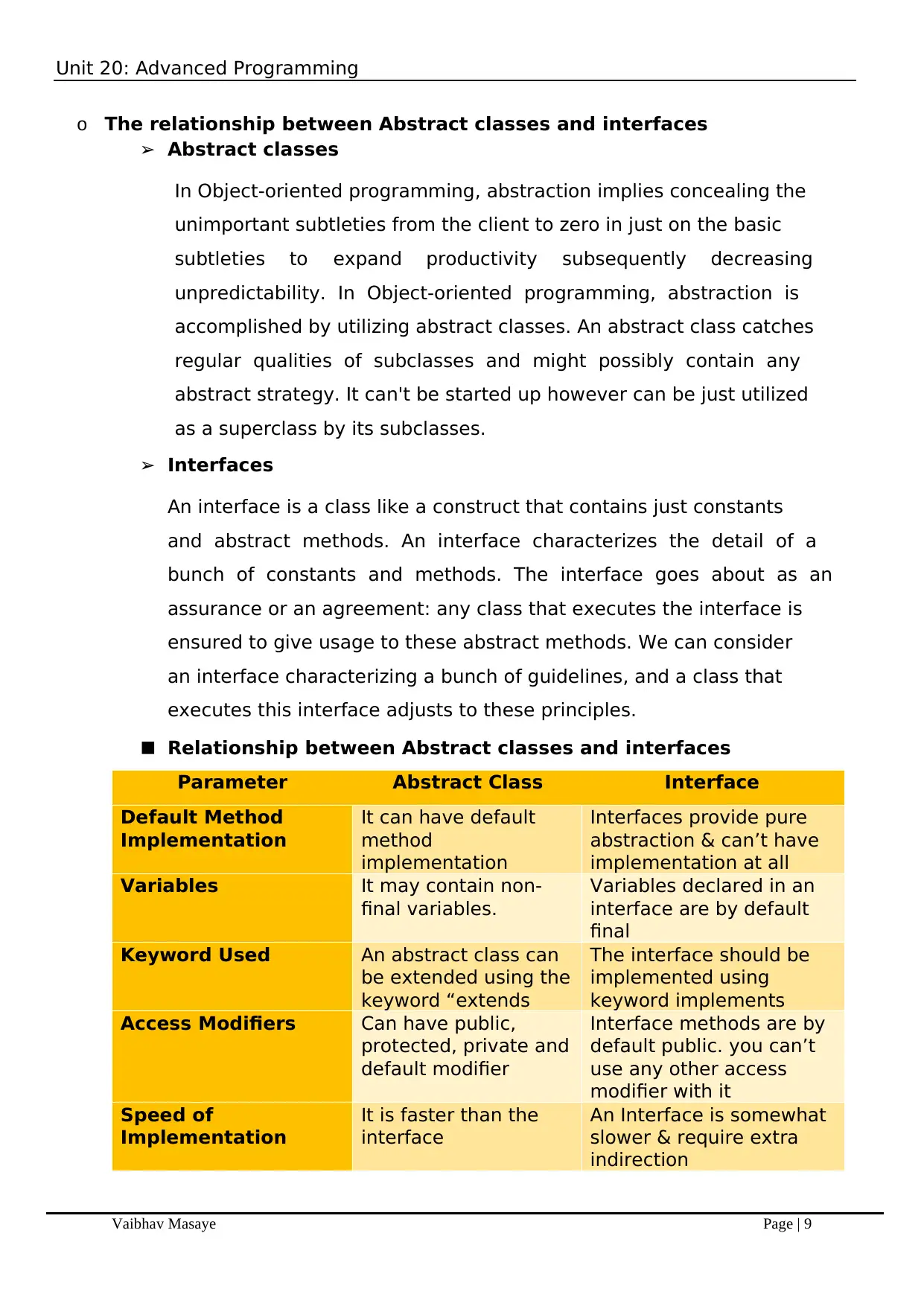
Unit 20: Advanced Programming
Vaibhav Masaye Page | 9
o The relationship between Abstract classes and interfaces
➢ Abstract classes
In Object-oriented programming, abstraction implies concealing the
unimportant subtleties from the client to zero in just on the basic
subtleties to expand productivity subsequently decreasing
unpredictability. In Object-oriented programming, abstraction is
accomplished by utilizing abstract classes. An abstract class catches
regular qualities of subclasses and might possibly contain any
abstract strategy. It can't be started up however can be just utilized
as a superclass by its subclasses.
➢ Interfaces
An interface is a class like a construct that contains just constants
and abstract methods. An interface characterizes the detail of a
bunch of constants and methods. The interface goes about as an
assurance or an agreement: any class that executes the interface is
ensured to give usage to these abstract methods. We can consider
an interface characterizing a bunch of guidelines, and a class that
executes this interface adjusts to these principles.
▪ Relationship between Abstract classes and interfaces
Parameter Abstract Class Interface
Default Method
Implementation
It can have default
method
implementation
Interfaces provide pure
abstraction & can’t have
implementation at all
Variables It may contain non-
final variables.
Variables declared in an
interface are by default
final
Keyword Used An abstract class can
be extended using the
keyword “extends
The interface should be
implemented using
keyword implements
Access Modifiers Can have public,
protected, private and
default modifier
Interface methods are by
default public. you can’t
use any other access
modifier with it
Speed of
Implementation
It is faster than the
interface
An Interface is somewhat
slower & require extra
indirection
Vaibhav Masaye Page | 9
o The relationship between Abstract classes and interfaces
➢ Abstract classes
In Object-oriented programming, abstraction implies concealing the
unimportant subtleties from the client to zero in just on the basic
subtleties to expand productivity subsequently decreasing
unpredictability. In Object-oriented programming, abstraction is
accomplished by utilizing abstract classes. An abstract class catches
regular qualities of subclasses and might possibly contain any
abstract strategy. It can't be started up however can be just utilized
as a superclass by its subclasses.
➢ Interfaces
An interface is a class like a construct that contains just constants
and abstract methods. An interface characterizes the detail of a
bunch of constants and methods. The interface goes about as an
assurance or an agreement: any class that executes the interface is
ensured to give usage to these abstract methods. We can consider
an interface characterizing a bunch of guidelines, and a class that
executes this interface adjusts to these principles.
▪ Relationship between Abstract classes and interfaces
Parameter Abstract Class Interface
Default Method
Implementation
It can have default
method
implementation
Interfaces provide pure
abstraction & can’t have
implementation at all
Variables It may contain non-
final variables.
Variables declared in an
interface are by default
final
Keyword Used An abstract class can
be extended using the
keyword “extends
The interface should be
implemented using
keyword implements
Access Modifiers Can have public,
protected, private and
default modifier
Interface methods are by
default public. you can’t
use any other access
modifier with it
Speed of
Implementation
It is faster than the
interface
An Interface is somewhat
slower & require extra
indirection
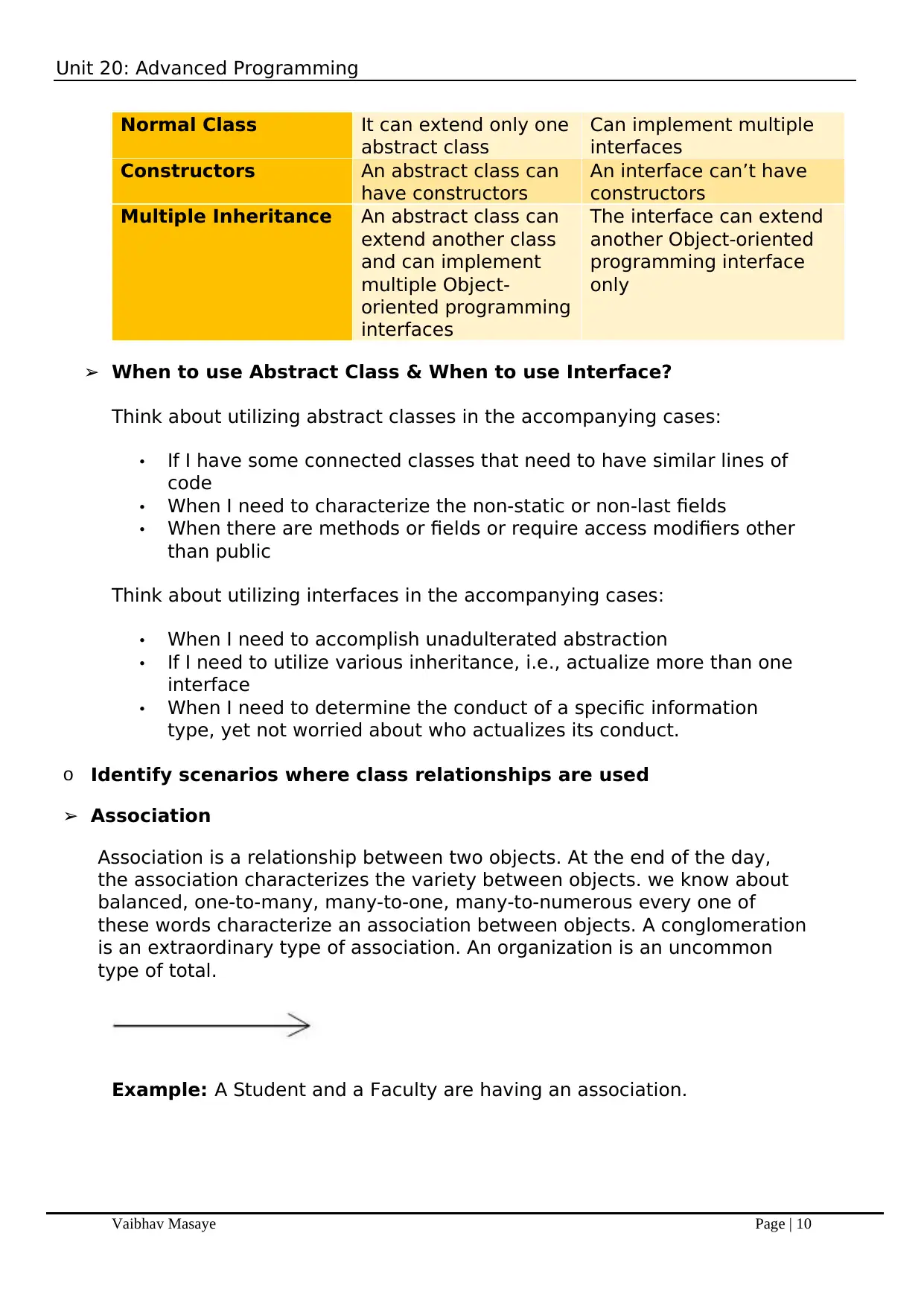
Unit 20: Advanced Programming
Vaibhav Masaye Page | 10
Normal Class It can extend only one
abstract class
Can implement multiple
interfaces
Constructors An abstract class can
have constructors
An interface can’t have
constructors
Multiple Inheritance An abstract class can
extend another class
and can implement
multiple Object-
oriented programming
interfaces
The interface can extend
another Object-oriented
programming interface
only
➢ When to use Abstract Class & When to use Interface?
Think about utilizing abstract classes in the accompanying cases:
• If I have some connected classes that need to have similar lines of
code
• When I need to characterize the non-static or non-last fields
• When there are methods or fields or require access modifiers other
than public
Think about utilizing interfaces in the accompanying cases:
• When I need to accomplish unadulterated abstraction
• If I need to utilize various inheritance, i.e., actualize more than one
interface
• When I need to determine the conduct of a specific information
type, yet not worried about who actualizes its conduct.
o Identify scenarios where class relationships are used
➢ Association
Association is a relationship between two objects. At the end of the day,
the association characterizes the variety between objects. we know about
balanced, one-to-many, many-to-one, many-to-numerous every one of
these words characterize an association between objects. A conglomeration
is an extraordinary type of association. An organization is an uncommon
type of total.
Example: A Student and a Faculty are having an association.
Vaibhav Masaye Page | 10
Normal Class It can extend only one
abstract class
Can implement multiple
interfaces
Constructors An abstract class can
have constructors
An interface can’t have
constructors
Multiple Inheritance An abstract class can
extend another class
and can implement
multiple Object-
oriented programming
interfaces
The interface can extend
another Object-oriented
programming interface
only
➢ When to use Abstract Class & When to use Interface?
Think about utilizing abstract classes in the accompanying cases:
• If I have some connected classes that need to have similar lines of
code
• When I need to characterize the non-static or non-last fields
• When there are methods or fields or require access modifiers other
than public
Think about utilizing interfaces in the accompanying cases:
• When I need to accomplish unadulterated abstraction
• If I need to utilize various inheritance, i.e., actualize more than one
interface
• When I need to determine the conduct of a specific information
type, yet not worried about who actualizes its conduct.
o Identify scenarios where class relationships are used
➢ Association
Association is a relationship between two objects. At the end of the day,
the association characterizes the variety between objects. we know about
balanced, one-to-many, many-to-one, many-to-numerous every one of
these words characterize an association between objects. A conglomeration
is an extraordinary type of association. An organization is an uncommon
type of total.
Example: A Student and a Faculty are having an association.
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
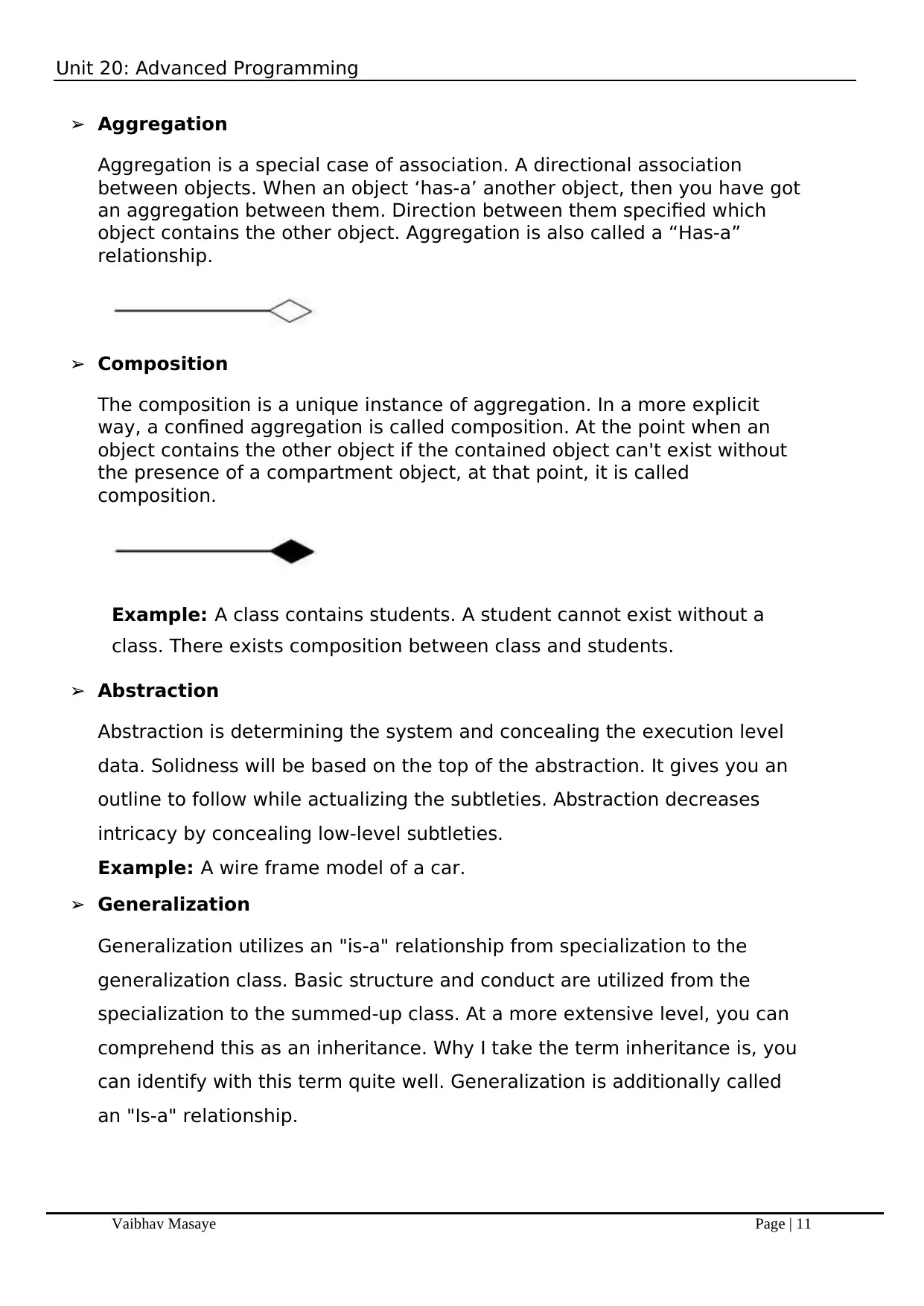
Unit 20: Advanced Programming
Vaibhav Masaye Page | 11
➢ Aggregation
Aggregation is a special case of association. A directional association
between objects. When an object ‘has-a’ another object, then you have got
an aggregation between them. Direction between them specified which
object contains the other object. Aggregation is also called a “Has-a”
relationship.
➢ Composition
The composition is a unique instance of aggregation. In a more explicit
way, a confined aggregation is called composition. At the point when an
object contains the other object if the contained object can't exist without
the presence of a compartment object, at that point, it is called
composition.
Example: A class contains students. A student cannot exist without a
class. There exists composition between class and students.
➢ Abstraction
Abstraction is determining the system and concealing the execution level
data. Solidness will be based on the top of the abstraction. It gives you an
outline to follow while actualizing the subtleties. Abstraction decreases
intricacy by concealing low-level subtleties.
Example: A wire frame model of a car.
➢ Generalization
Generalization utilizes an "is-a" relationship from specialization to the
generalization class. Basic structure and conduct are utilized from the
specialization to the summed-up class. At a more extensive level, you can
comprehend this as an inheritance. Why I take the term inheritance is, you
can identify with this term quite well. Generalization is additionally called
an "Is-a" relationship.
Vaibhav Masaye Page | 11
➢ Aggregation
Aggregation is a special case of association. A directional association
between objects. When an object ‘has-a’ another object, then you have got
an aggregation between them. Direction between them specified which
object contains the other object. Aggregation is also called a “Has-a”
relationship.
➢ Composition
The composition is a unique instance of aggregation. In a more explicit
way, a confined aggregation is called composition. At the point when an
object contains the other object if the contained object can't exist without
the presence of a compartment object, at that point, it is called
composition.
Example: A class contains students. A student cannot exist without a
class. There exists composition between class and students.
➢ Abstraction
Abstraction is determining the system and concealing the execution level
data. Solidness will be based on the top of the abstraction. It gives you an
outline to follow while actualizing the subtleties. Abstraction decreases
intricacy by concealing low-level subtleties.
Example: A wire frame model of a car.
➢ Generalization
Generalization utilizes an "is-a" relationship from specialization to the
generalization class. Basic structure and conduct are utilized from the
specialization to the summed-up class. At a more extensive level, you can
comprehend this as an inheritance. Why I take the term inheritance is, you
can identify with this term quite well. Generalization is additionally called
an "Is-a" relationship.
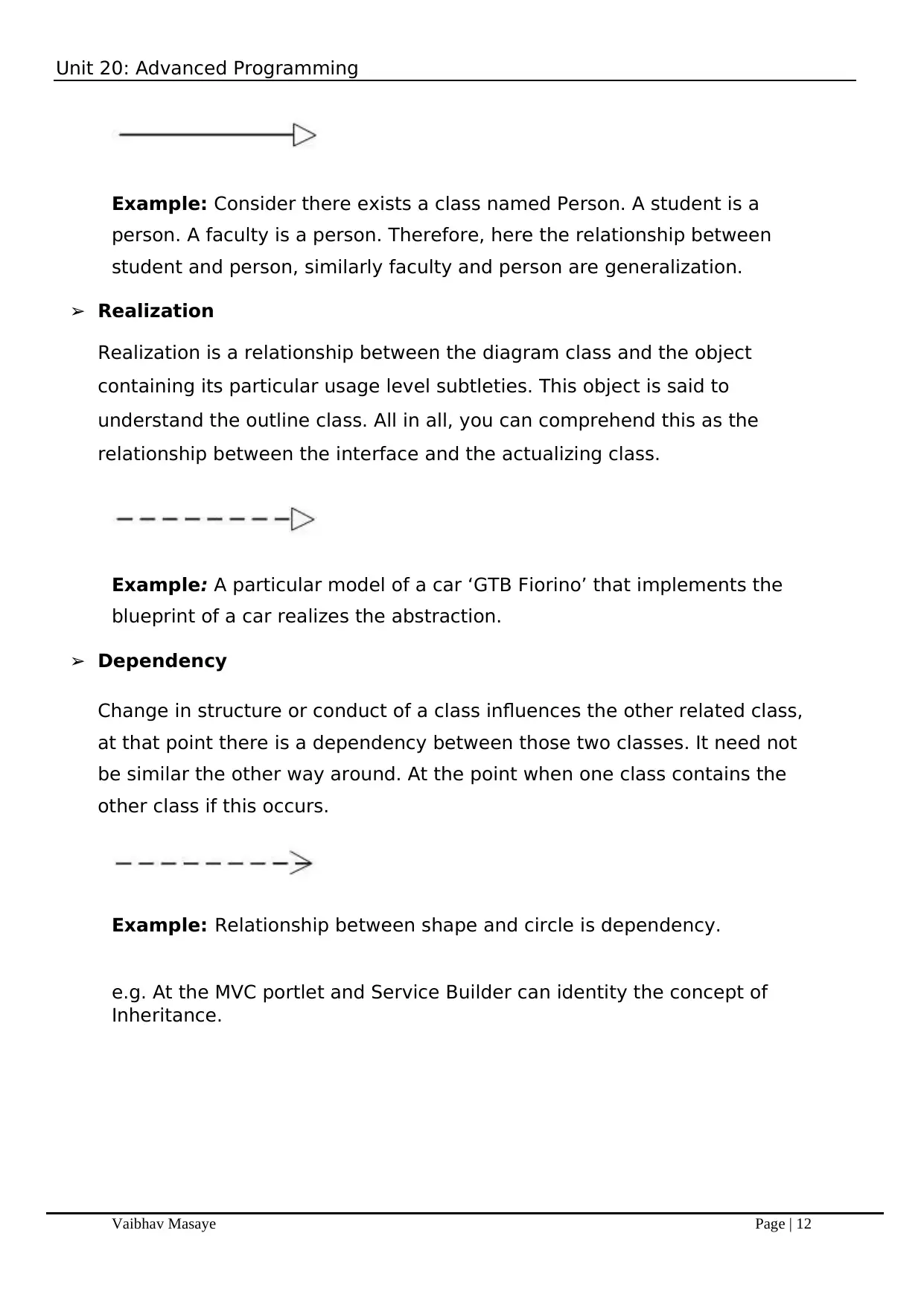
Unit 20: Advanced Programming
Vaibhav Masaye Page | 12
Example: Consider there exists a class named Person. A student is a
person. A faculty is a person. Therefore, here the relationship between
student and person, similarly faculty and person are generalization.
➢ Realization
Realization is a relationship between the diagram class and the object
containing its particular usage level subtleties. This object is said to
understand the outline class. All in all, you can comprehend this as the
relationship between the interface and the actualizing class.
Example: A particular model of a car ‘GTB Fiorino’ that implements the
blueprint of a car realizes the abstraction.
➢ Dependency
Change in structure or conduct of a class influences the other related class,
at that point there is a dependency between those two classes. It need not
be similar the other way around. At the point when one class contains the
other class if this occurs.
Example: Relationship between shape and circle is dependency.
e.g. At the MVC portlet and Service Builder can identity the concept of
Inheritance.
Vaibhav Masaye Page | 12
Example: Consider there exists a class named Person. A student is a
person. A faculty is a person. Therefore, here the relationship between
student and person, similarly faculty and person are generalization.
➢ Realization
Realization is a relationship between the diagram class and the object
containing its particular usage level subtleties. This object is said to
understand the outline class. All in all, you can comprehend this as the
relationship between the interface and the actualizing class.
Example: A particular model of a car ‘GTB Fiorino’ that implements the
blueprint of a car realizes the abstraction.
➢ Dependency
Change in structure or conduct of a class influences the other related class,
at that point there is a dependency between those two classes. It need not
be similar the other way around. At the point when one class contains the
other class if this occurs.
Example: Relationship between shape and circle is dependency.
e.g. At the MVC portlet and Service Builder can identity the concept of
Inheritance.
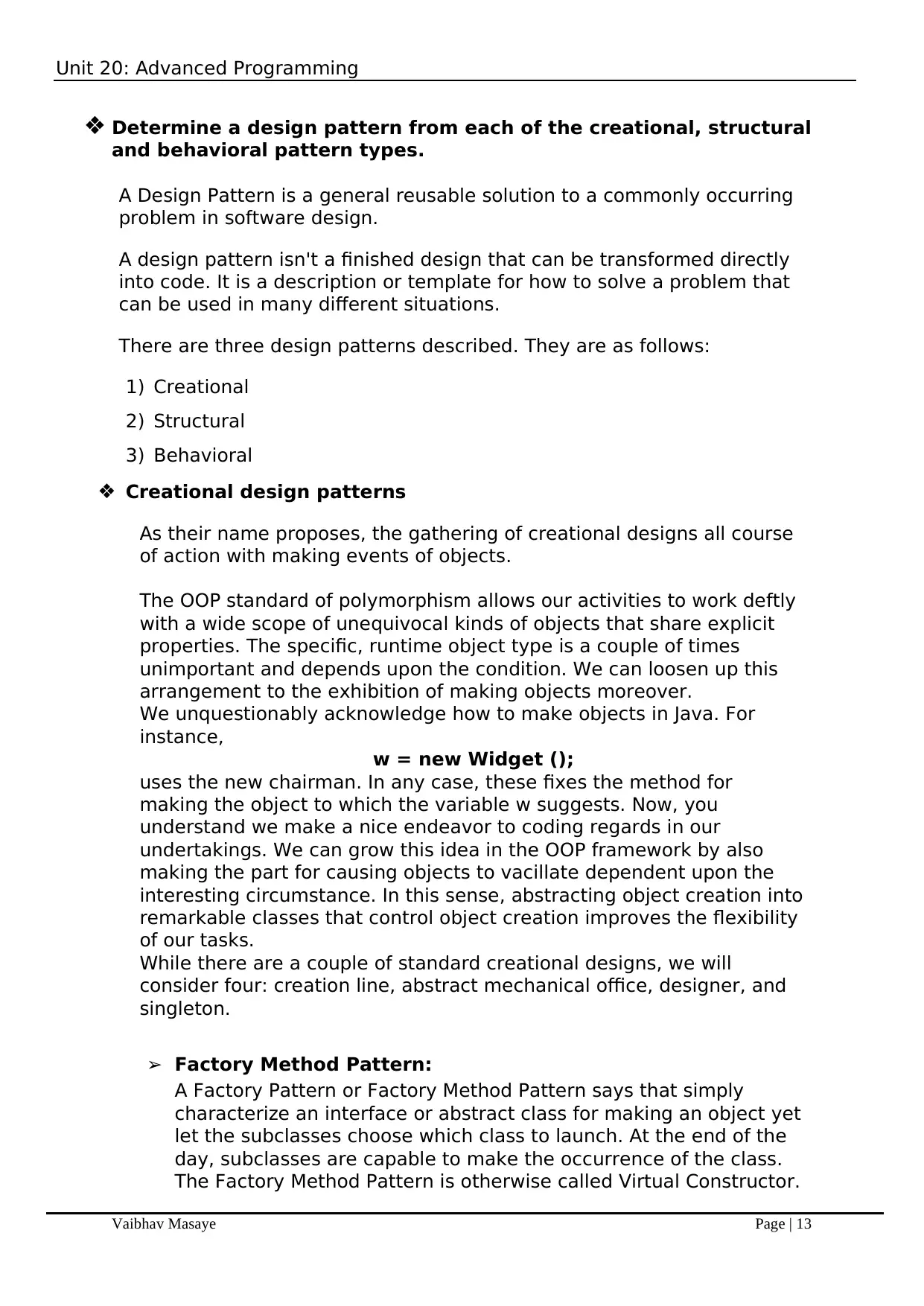
Unit 20: Advanced Programming
Vaibhav Masaye Page | 13
❖ Determine a design pattern from each of the creational, structural
and behavioral pattern types.
A Design Pattern is a general reusable solution to a commonly occurring
problem in software design.
A design pattern isn't a finished design that can be transformed directly
into code. It is a description or template for how to solve a problem that
can be used in many different situations.
There are three design patterns described. They are as follows:
1) Creational
2) Structural
3) Behavioral
❖ Creational design patterns
As their name proposes, the gathering of creational designs all course
of action with making events of objects.
The OOP standard of polymorphism allows our activities to work deftly
with a wide scope of unequivocal kinds of objects that share explicit
properties. The specific, runtime object type is a couple of times
unimportant and depends upon the condition. We can loosen up this
arrangement to the exhibition of making objects moreover.
We unquestionably acknowledge how to make objects in Java. For
instance,
w = new Widget ();
uses the new chairman. In any case, these fixes the method for
making the object to which the variable w suggests. Now, you
understand we make a nice endeavor to coding regards in our
undertakings. We can grow this idea in the OOP framework by also
making the part for causing objects to vacillate dependent upon the
interesting circumstance. In this sense, abstracting object creation into
remarkable classes that control object creation improves the flexibility
of our tasks.
While there are a couple of standard creational designs, we will
consider four: creation line, abstract mechanical office, designer, and
singleton.
➢ Factory Method Pattern:
A Factory Pattern or Factory Method Pattern says that simply
characterize an interface or abstract class for making an object yet
let the subclasses choose which class to launch. At the end of the
day, subclasses are capable to make the occurrence of the class.
The Factory Method Pattern is otherwise called Virtual Constructor.
Vaibhav Masaye Page | 13
❖ Determine a design pattern from each of the creational, structural
and behavioral pattern types.
A Design Pattern is a general reusable solution to a commonly occurring
problem in software design.
A design pattern isn't a finished design that can be transformed directly
into code. It is a description or template for how to solve a problem that
can be used in many different situations.
There are three design patterns described. They are as follows:
1) Creational
2) Structural
3) Behavioral
❖ Creational design patterns
As their name proposes, the gathering of creational designs all course
of action with making events of objects.
The OOP standard of polymorphism allows our activities to work deftly
with a wide scope of unequivocal kinds of objects that share explicit
properties. The specific, runtime object type is a couple of times
unimportant and depends upon the condition. We can loosen up this
arrangement to the exhibition of making objects moreover.
We unquestionably acknowledge how to make objects in Java. For
instance,
w = new Widget ();
uses the new chairman. In any case, these fixes the method for
making the object to which the variable w suggests. Now, you
understand we make a nice endeavor to coding regards in our
undertakings. We can grow this idea in the OOP framework by also
making the part for causing objects to vacillate dependent upon the
interesting circumstance. In this sense, abstracting object creation into
remarkable classes that control object creation improves the flexibility
of our tasks.
While there are a couple of standard creational designs, we will
consider four: creation line, abstract mechanical office, designer, and
singleton.
➢ Factory Method Pattern:
A Factory Pattern or Factory Method Pattern says that simply
characterize an interface or abstract class for making an object yet
let the subclasses choose which class to launch. At the end of the
day, subclasses are capable to make the occurrence of the class.
The Factory Method Pattern is otherwise called Virtual Constructor.
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
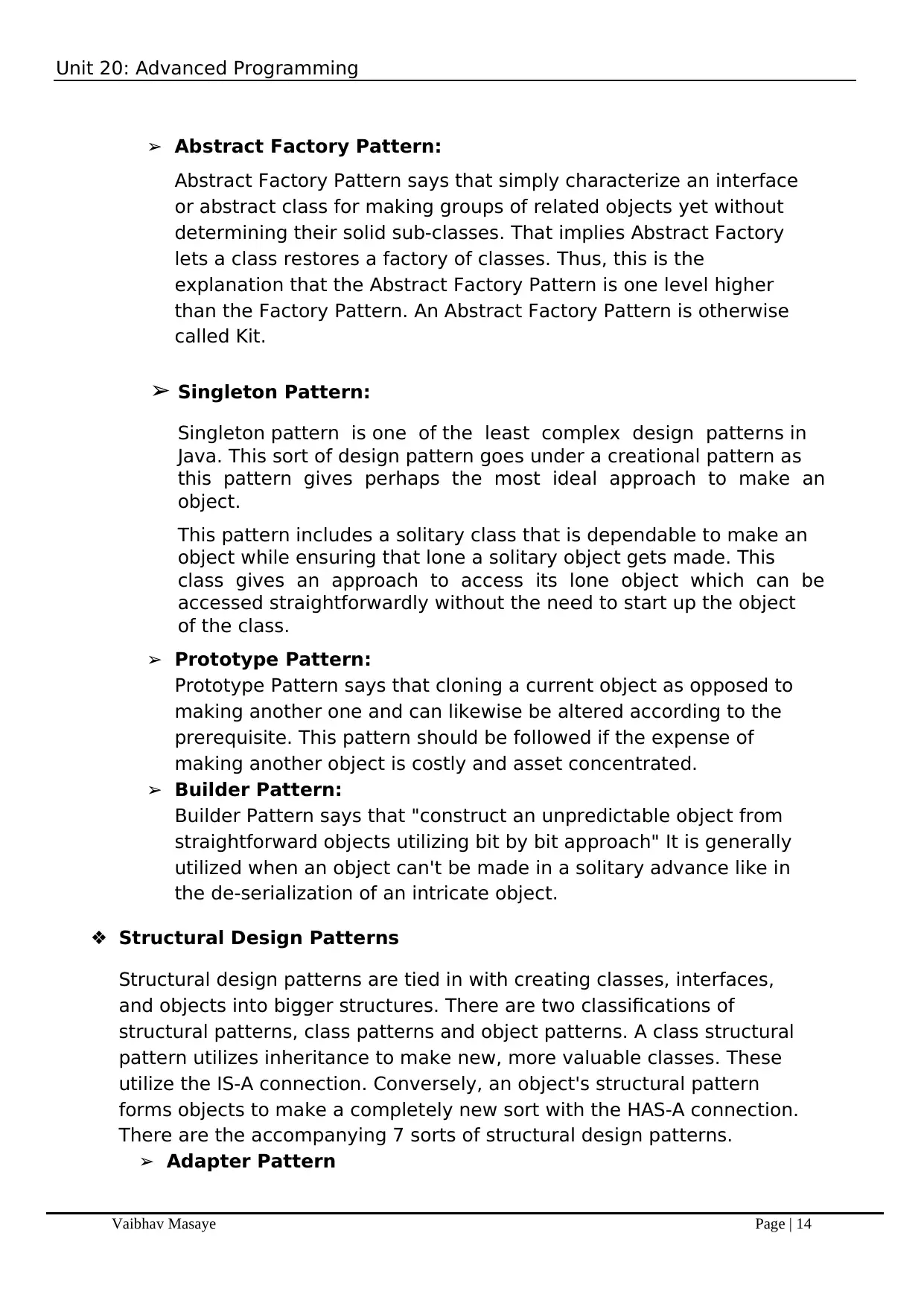
Unit 20: Advanced Programming
Vaibhav Masaye Page | 14
➢ Abstract Factory Pattern:
Abstract Factory Pattern says that simply characterize an interface
or abstract class for making groups of related objects yet without
determining their solid sub-classes. That implies Abstract Factory
lets a class restores a factory of classes. Thus, this is the
explanation that the Abstract Factory Pattern is one level higher
than the Factory Pattern. An Abstract Factory Pattern is otherwise
called Kit.
➢ Singleton Pattern:
Singleton pattern is one of the least complex design patterns in
Java. This sort of design pattern goes under a creational pattern as
this pattern gives perhaps the most ideal approach to make an
object.
This pattern includes a solitary class that is dependable to make an
object while ensuring that lone a solitary object gets made. This
class gives an approach to access its lone object which can be
accessed straightforwardly without the need to start up the object
of the class.
➢ Prototype Pattern:
Prototype Pattern says that cloning a current object as opposed to
making another one and can likewise be altered according to the
prerequisite. This pattern should be followed if the expense of
making another object is costly and asset concentrated.
➢ Builder Pattern:
Builder Pattern says that "construct an unpredictable object from
straightforward objects utilizing bit by bit approach" It is generally
utilized when an object can't be made in a solitary advance like in
the de-serialization of an intricate object.
❖ Structural Design Patterns
Structural design patterns are tied in with creating classes, interfaces,
and objects into bigger structures. There are two classifications of
structural patterns, class patterns and object patterns. A class structural
pattern utilizes inheritance to make new, more valuable classes. These
utilize the IS-A connection. Conversely, an object's structural pattern
forms objects to make a completely new sort with the HAS-A connection.
There are the accompanying 7 sorts of structural design patterns.
➢ Adapter Pattern
Vaibhav Masaye Page | 14
➢ Abstract Factory Pattern:
Abstract Factory Pattern says that simply characterize an interface
or abstract class for making groups of related objects yet without
determining their solid sub-classes. That implies Abstract Factory
lets a class restores a factory of classes. Thus, this is the
explanation that the Abstract Factory Pattern is one level higher
than the Factory Pattern. An Abstract Factory Pattern is otherwise
called Kit.
➢ Singleton Pattern:
Singleton pattern is one of the least complex design patterns in
Java. This sort of design pattern goes under a creational pattern as
this pattern gives perhaps the most ideal approach to make an
object.
This pattern includes a solitary class that is dependable to make an
object while ensuring that lone a solitary object gets made. This
class gives an approach to access its lone object which can be
accessed straightforwardly without the need to start up the object
of the class.
➢ Prototype Pattern:
Prototype Pattern says that cloning a current object as opposed to
making another one and can likewise be altered according to the
prerequisite. This pattern should be followed if the expense of
making another object is costly and asset concentrated.
➢ Builder Pattern:
Builder Pattern says that "construct an unpredictable object from
straightforward objects utilizing bit by bit approach" It is generally
utilized when an object can't be made in a solitary advance like in
the de-serialization of an intricate object.
❖ Structural Design Patterns
Structural design patterns are tied in with creating classes, interfaces,
and objects into bigger structures. There are two classifications of
structural patterns, class patterns and object patterns. A class structural
pattern utilizes inheritance to make new, more valuable classes. These
utilize the IS-A connection. Conversely, an object's structural pattern
forms objects to make a completely new sort with the HAS-A connection.
There are the accompanying 7 sorts of structural design patterns.
➢ Adapter Pattern
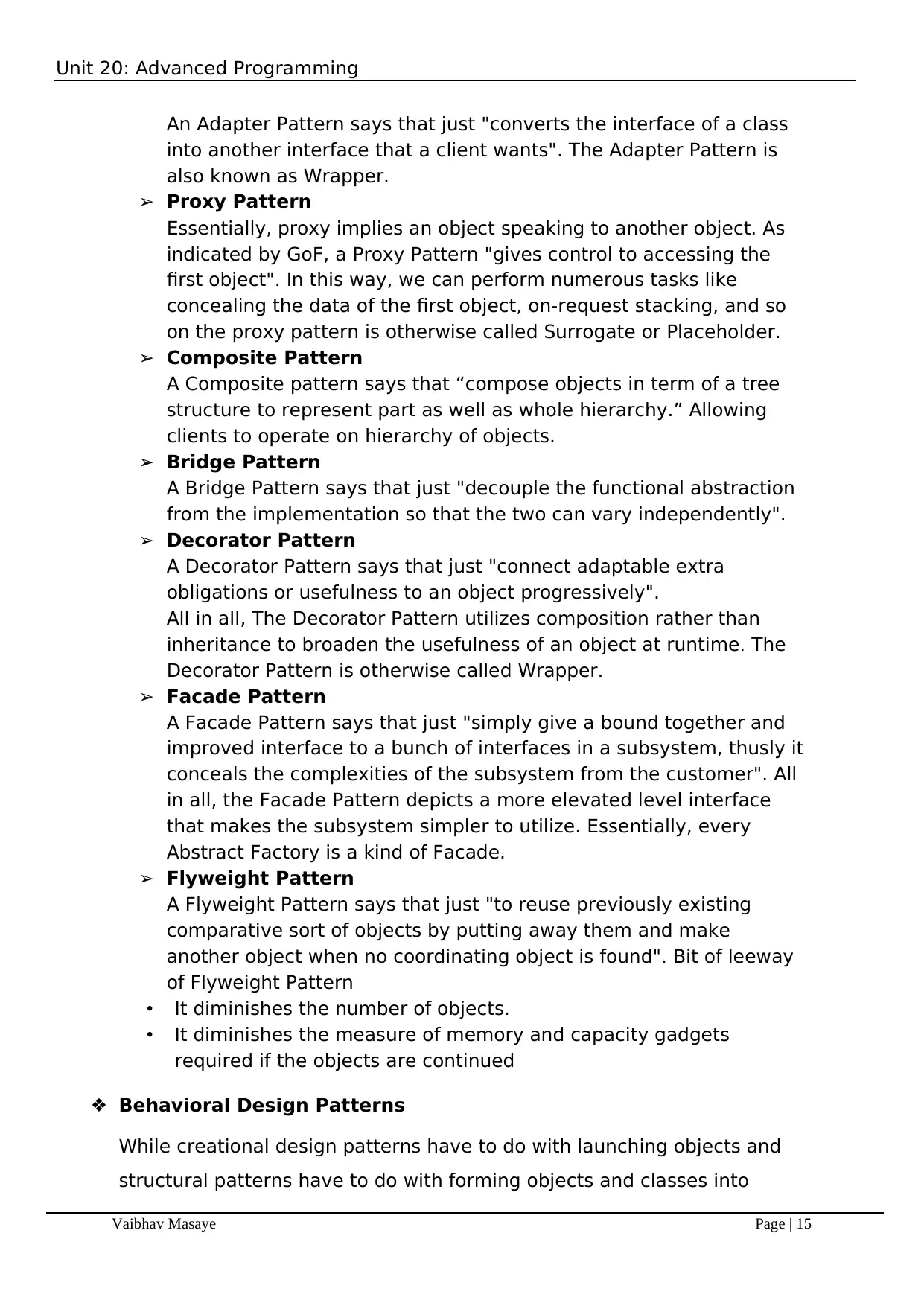
Unit 20: Advanced Programming
Vaibhav Masaye Page | 15
An Adapter Pattern says that just "converts the interface of a class
into another interface that a client wants". The Adapter Pattern is
also known as Wrapper.
➢ Proxy Pattern
Essentially, proxy implies an object speaking to another object. As
indicated by GoF, a Proxy Pattern "gives control to accessing the
first object". In this way, we can perform numerous tasks like
concealing the data of the first object, on-request stacking, and so
on the proxy pattern is otherwise called Surrogate or Placeholder.
➢ Composite Pattern
A Composite pattern says that “compose objects in term of a tree
structure to represent part as well as whole hierarchy.” Allowing
clients to operate on hierarchy of objects.
➢ Bridge Pattern
A Bridge Pattern says that just "decouple the functional abstraction
from the implementation so that the two can vary independently".
➢ Decorator Pattern
A Decorator Pattern says that just "connect adaptable extra
obligations or usefulness to an object progressively".
All in all, The Decorator Pattern utilizes composition rather than
inheritance to broaden the usefulness of an object at runtime. The
Decorator Pattern is otherwise called Wrapper.
➢ Facade Pattern
A Facade Pattern says that just "simply give a bound together and
improved interface to a bunch of interfaces in a subsystem, thusly it
conceals the complexities of the subsystem from the customer". All
in all, the Facade Pattern depicts a more elevated level interface
that makes the subsystem simpler to utilize. Essentially, every
Abstract Factory is a kind of Facade.
➢ Flyweight Pattern
A Flyweight Pattern says that just "to reuse previously existing
comparative sort of objects by putting away them and make
another object when no coordinating object is found". Bit of leeway
of Flyweight Pattern
• It diminishes the number of objects.
• It diminishes the measure of memory and capacity gadgets
required if the objects are continued
❖ Behavioral Design Patterns
While creational design patterns have to do with launching objects and
structural patterns have to do with forming objects and classes into
Vaibhav Masaye Page | 15
An Adapter Pattern says that just "converts the interface of a class
into another interface that a client wants". The Adapter Pattern is
also known as Wrapper.
➢ Proxy Pattern
Essentially, proxy implies an object speaking to another object. As
indicated by GoF, a Proxy Pattern "gives control to accessing the
first object". In this way, we can perform numerous tasks like
concealing the data of the first object, on-request stacking, and so
on the proxy pattern is otherwise called Surrogate or Placeholder.
➢ Composite Pattern
A Composite pattern says that “compose objects in term of a tree
structure to represent part as well as whole hierarchy.” Allowing
clients to operate on hierarchy of objects.
➢ Bridge Pattern
A Bridge Pattern says that just "decouple the functional abstraction
from the implementation so that the two can vary independently".
➢ Decorator Pattern
A Decorator Pattern says that just "connect adaptable extra
obligations or usefulness to an object progressively".
All in all, The Decorator Pattern utilizes composition rather than
inheritance to broaden the usefulness of an object at runtime. The
Decorator Pattern is otherwise called Wrapper.
➢ Facade Pattern
A Facade Pattern says that just "simply give a bound together and
improved interface to a bunch of interfaces in a subsystem, thusly it
conceals the complexities of the subsystem from the customer". All
in all, the Facade Pattern depicts a more elevated level interface
that makes the subsystem simpler to utilize. Essentially, every
Abstract Factory is a kind of Facade.
➢ Flyweight Pattern
A Flyweight Pattern says that just "to reuse previously existing
comparative sort of objects by putting away them and make
another object when no coordinating object is found". Bit of leeway
of Flyweight Pattern
• It diminishes the number of objects.
• It diminishes the measure of memory and capacity gadgets
required if the objects are continued
❖ Behavioral Design Patterns
While creational design patterns have to do with launching objects and
structural patterns have to do with forming objects and classes into
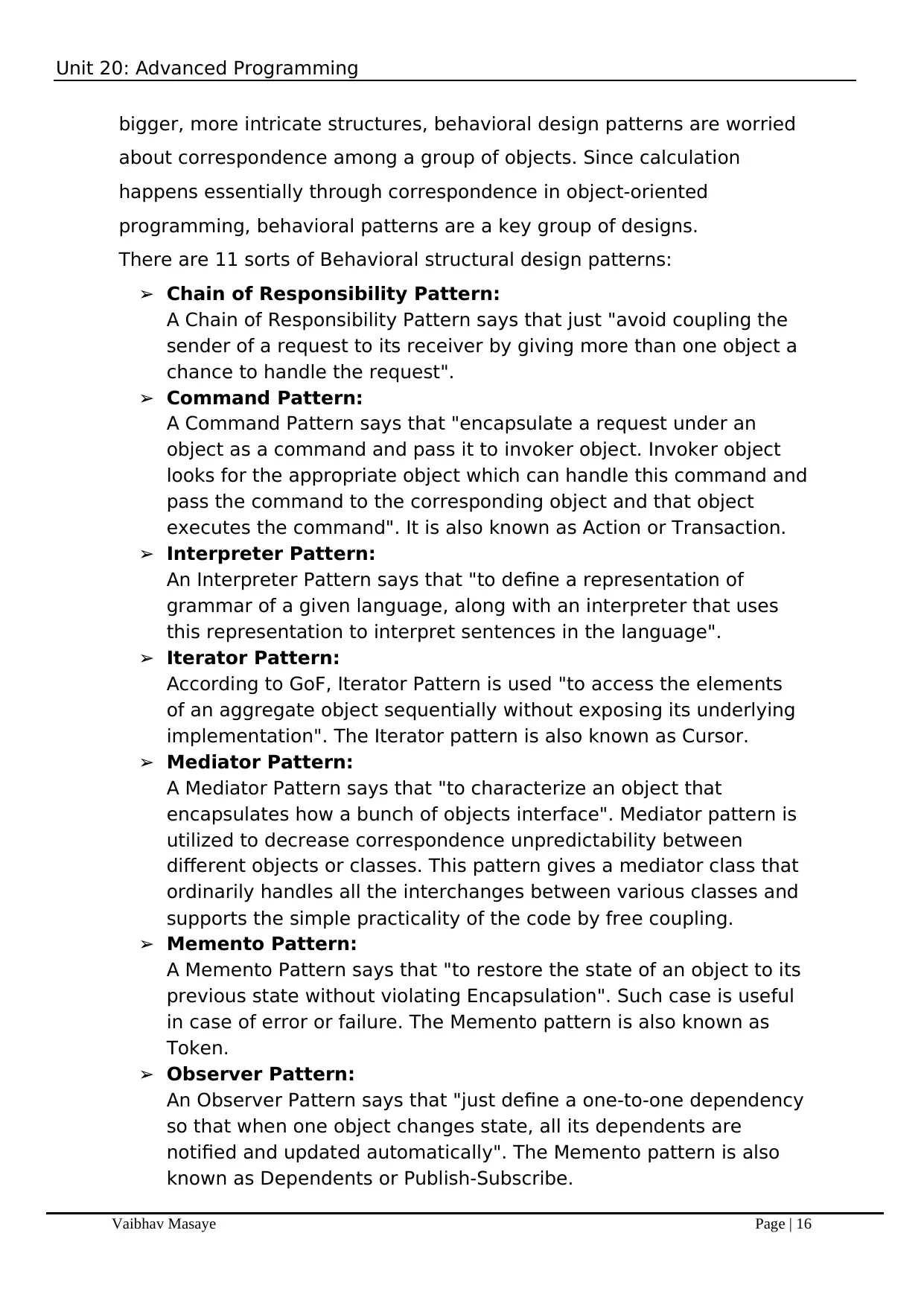
Unit 20: Advanced Programming
Vaibhav Masaye Page | 16
bigger, more intricate structures, behavioral design patterns are worried
about correspondence among a group of objects. Since calculation
happens essentially through correspondence in object-oriented
programming, behavioral patterns are a key group of designs.
There are 11 sorts of Behavioral structural design patterns:
➢ Chain of Responsibility Pattern:
A Chain of Responsibility Pattern says that just "avoid coupling the
sender of a request to its receiver by giving more than one object a
chance to handle the request".
➢ Command Pattern:
A Command Pattern says that "encapsulate a request under an
object as a command and pass it to invoker object. Invoker object
looks for the appropriate object which can handle this command and
pass the command to the corresponding object and that object
executes the command". It is also known as Action or Transaction.
➢ Interpreter Pattern:
An Interpreter Pattern says that "to define a representation of
grammar of a given language, along with an interpreter that uses
this representation to interpret sentences in the language".
➢ Iterator Pattern:
According to GoF, Iterator Pattern is used "to access the elements
of an aggregate object sequentially without exposing its underlying
implementation". The Iterator pattern is also known as Cursor.
➢ Mediator Pattern:
A Mediator Pattern says that "to characterize an object that
encapsulates how a bunch of objects interface". Mediator pattern is
utilized to decrease correspondence unpredictability between
different objects or classes. This pattern gives a mediator class that
ordinarily handles all the interchanges between various classes and
supports the simple practicality of the code by free coupling.
➢ Memento Pattern:
A Memento Pattern says that "to restore the state of an object to its
previous state without violating Encapsulation". Such case is useful
in case of error or failure. The Memento pattern is also known as
Token.
➢ Observer Pattern:
An Observer Pattern says that "just define a one-to-one dependency
so that when one object changes state, all its dependents are
notified and updated automatically". The Memento pattern is also
known as Dependents or Publish-Subscribe.
Vaibhav Masaye Page | 16
bigger, more intricate structures, behavioral design patterns are worried
about correspondence among a group of objects. Since calculation
happens essentially through correspondence in object-oriented
programming, behavioral patterns are a key group of designs.
There are 11 sorts of Behavioral structural design patterns:
➢ Chain of Responsibility Pattern:
A Chain of Responsibility Pattern says that just "avoid coupling the
sender of a request to its receiver by giving more than one object a
chance to handle the request".
➢ Command Pattern:
A Command Pattern says that "encapsulate a request under an
object as a command and pass it to invoker object. Invoker object
looks for the appropriate object which can handle this command and
pass the command to the corresponding object and that object
executes the command". It is also known as Action or Transaction.
➢ Interpreter Pattern:
An Interpreter Pattern says that "to define a representation of
grammar of a given language, along with an interpreter that uses
this representation to interpret sentences in the language".
➢ Iterator Pattern:
According to GoF, Iterator Pattern is used "to access the elements
of an aggregate object sequentially without exposing its underlying
implementation". The Iterator pattern is also known as Cursor.
➢ Mediator Pattern:
A Mediator Pattern says that "to characterize an object that
encapsulates how a bunch of objects interface". Mediator pattern is
utilized to decrease correspondence unpredictability between
different objects or classes. This pattern gives a mediator class that
ordinarily handles all the interchanges between various classes and
supports the simple practicality of the code by free coupling.
➢ Memento Pattern:
A Memento Pattern says that "to restore the state of an object to its
previous state without violating Encapsulation". Such case is useful
in case of error or failure. The Memento pattern is also known as
Token.
➢ Observer Pattern:
An Observer Pattern says that "just define a one-to-one dependency
so that when one object changes state, all its dependents are
notified and updated automatically". The Memento pattern is also
known as Dependents or Publish-Subscribe.
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
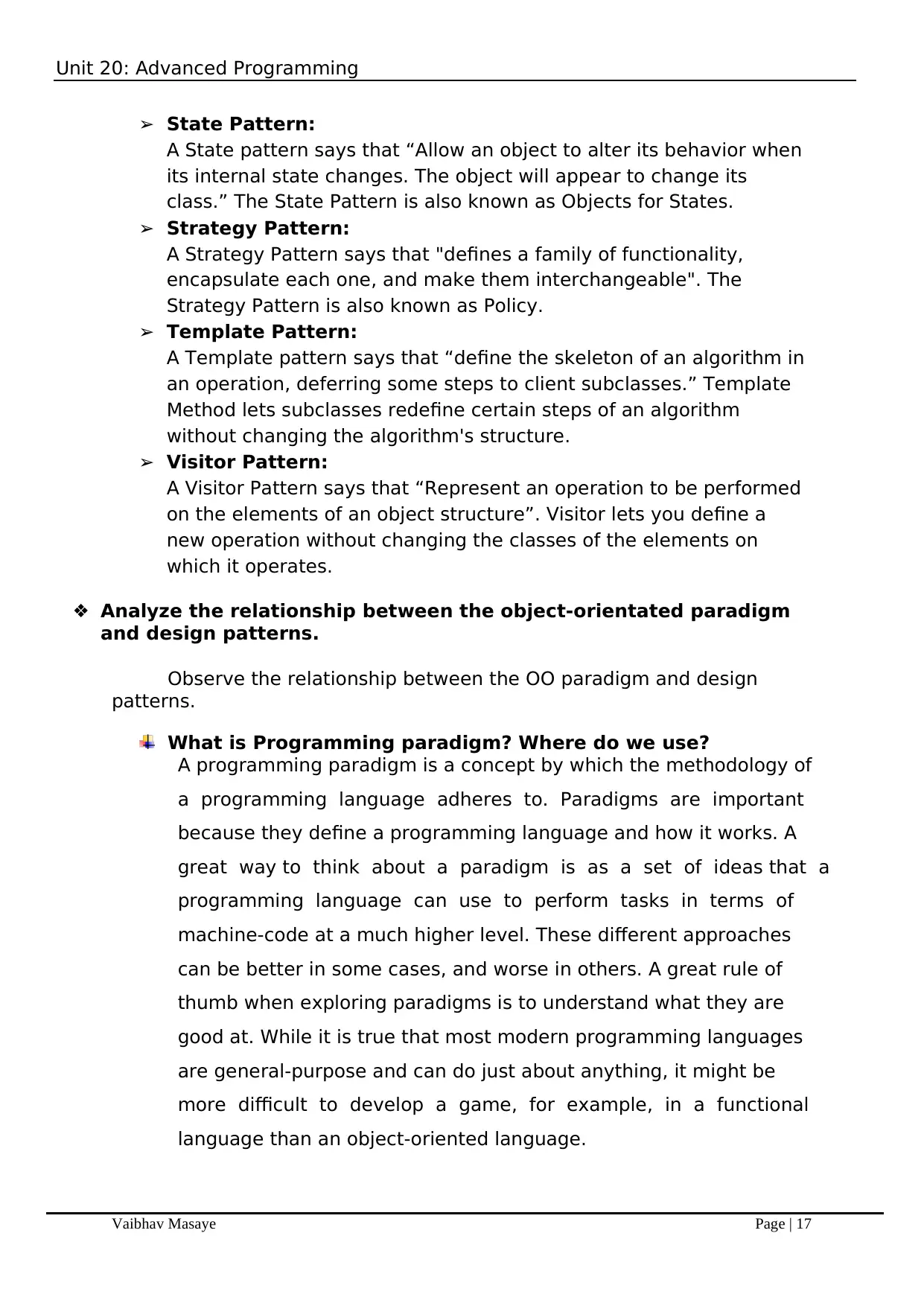
Unit 20: Advanced Programming
Vaibhav Masaye Page | 17
➢ State Pattern:
A State pattern says that “Allow an object to alter its behavior when
its internal state changes. The object will appear to change its
class.” The State Pattern is also known as Objects for States.
➢ Strategy Pattern:
A Strategy Pattern says that "defines a family of functionality,
encapsulate each one, and make them interchangeable". The
Strategy Pattern is also known as Policy.
➢ Template Pattern:
A Template pattern says that “define the skeleton of an algorithm in
an operation, deferring some steps to client subclasses.” Template
Method lets subclasses redefine certain steps of an algorithm
without changing the algorithm's structure.
➢ Visitor Pattern:
A Visitor Pattern says that “Represent an operation to be performed
on the elements of an object structure”. Visitor lets you define a
new operation without changing the classes of the elements on
which it operates.
❖ Analyze the relationship between the object-orientated paradigm
and design patterns.
Observe the relationship between the OO paradigm and design
patterns.
What is Programming paradigm? Where do we use?
A programming paradigm is a concept by which the methodology of
a programming language adheres to. Paradigms are important
because they define a programming language and how it works. A
great way to think about a paradigm is as a set of ideas that a
programming language can use to perform tasks in terms of
machine-code at a much higher level. These different approaches
can be better in some cases, and worse in others. A great rule of
thumb when exploring paradigms is to understand what they are
good at. While it is true that most modern programming languages
are general-purpose and can do just about anything, it might be
more difficult to develop a game, for example, in a functional
language than an object-oriented language.
Vaibhav Masaye Page | 17
➢ State Pattern:
A State pattern says that “Allow an object to alter its behavior when
its internal state changes. The object will appear to change its
class.” The State Pattern is also known as Objects for States.
➢ Strategy Pattern:
A Strategy Pattern says that "defines a family of functionality,
encapsulate each one, and make them interchangeable". The
Strategy Pattern is also known as Policy.
➢ Template Pattern:
A Template pattern says that “define the skeleton of an algorithm in
an operation, deferring some steps to client subclasses.” Template
Method lets subclasses redefine certain steps of an algorithm
without changing the algorithm's structure.
➢ Visitor Pattern:
A Visitor Pattern says that “Represent an operation to be performed
on the elements of an object structure”. Visitor lets you define a
new operation without changing the classes of the elements on
which it operates.
❖ Analyze the relationship between the object-orientated paradigm
and design patterns.
Observe the relationship between the OO paradigm and design
patterns.
What is Programming paradigm? Where do we use?
A programming paradigm is a concept by which the methodology of
a programming language adheres to. Paradigms are important
because they define a programming language and how it works. A
great way to think about a paradigm is as a set of ideas that a
programming language can use to perform tasks in terms of
machine-code at a much higher level. These different approaches
can be better in some cases, and worse in others. A great rule of
thumb when exploring paradigms is to understand what they are
good at. While it is true that most modern programming languages
are general-purpose and can do just about anything, it might be
more difficult to develop a game, for example, in a functional
language than an object-oriented language.
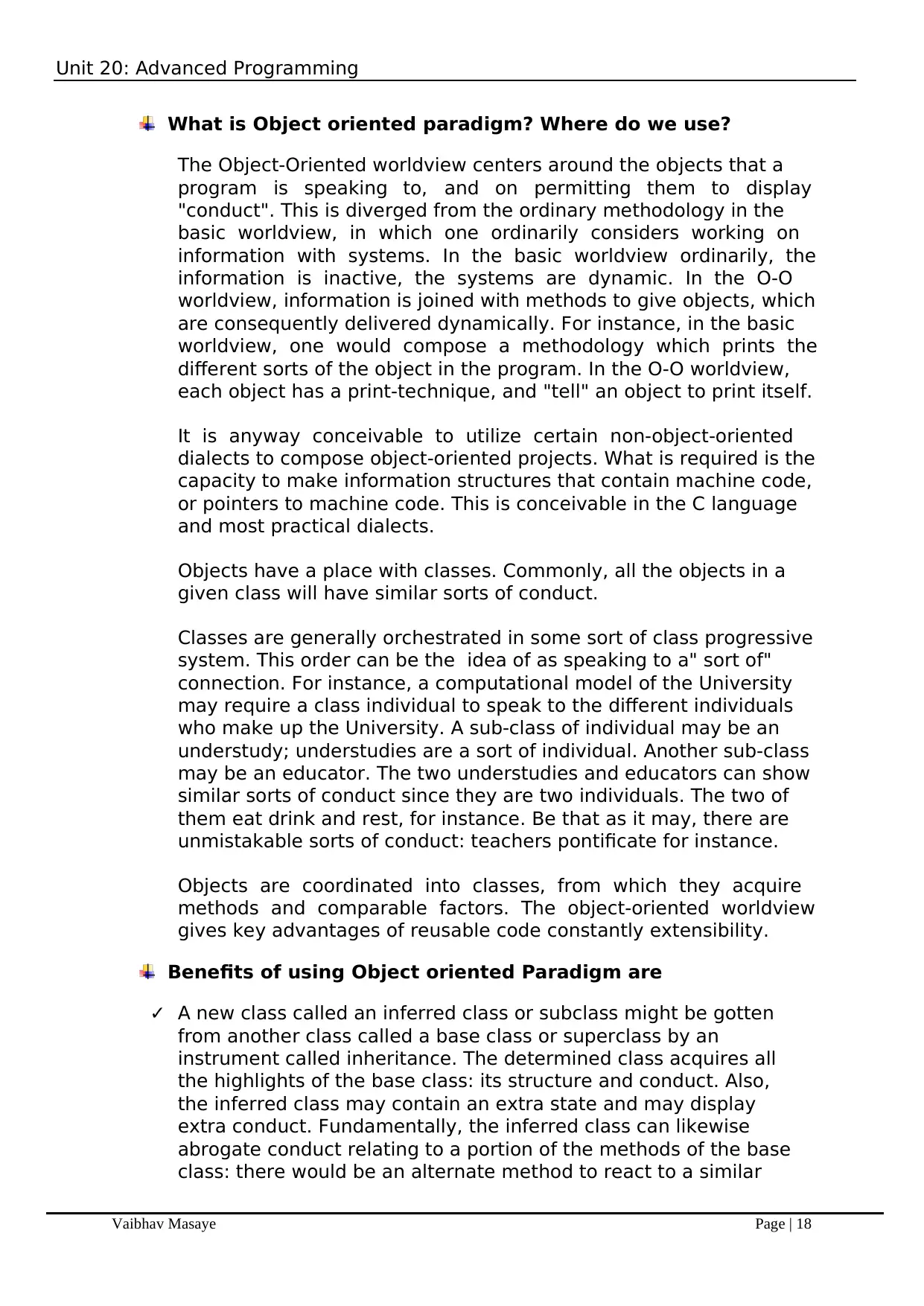
Unit 20: Advanced Programming
Vaibhav Masaye Page | 18
What is Object oriented paradigm? Where do we use?
The Object-Oriented worldview centers around the objects that a
program is speaking to, and on permitting them to display
"conduct". This is diverged from the ordinary methodology in the
basic worldview, in which one ordinarily considers working on
information with systems. In the basic worldview ordinarily, the
information is inactive, the systems are dynamic. In the O-O
worldview, information is joined with methods to give objects, which
are consequently delivered dynamically. For instance, in the basic
worldview, one would compose a methodology which prints the
different sorts of the object in the program. In the O-O worldview,
each object has a print-technique, and "tell" an object to print itself.
It is anyway conceivable to utilize certain non-object-oriented
dialects to compose object-oriented projects. What is required is the
capacity to make information structures that contain machine code,
or pointers to machine code. This is conceivable in the C language
and most practical dialects.
Objects have a place with classes. Commonly, all the objects in a
given class will have similar sorts of conduct.
Classes are generally orchestrated in some sort of class progressive
system. This order can be the idea of as speaking to a" sort of"
connection. For instance, a computational model of the University
may require a class individual to speak to the different individuals
who make up the University. A sub-class of individual may be an
understudy; understudies are a sort of individual. Another sub-class
may be an educator. The two understudies and educators can show
similar sorts of conduct since they are two individuals. The two of
them eat drink and rest, for instance. Be that as it may, there are
unmistakable sorts of conduct: teachers pontificate for instance.
Objects are coordinated into classes, from which they acquire
methods and comparable factors. The object-oriented worldview
gives key advantages of reusable code constantly extensibility.
Benefits of using Object oriented Paradigm are
✓ A new class called an inferred class or subclass might be gotten
from another class called a base class or superclass by an
instrument called inheritance. The determined class acquires all
the highlights of the base class: its structure and conduct. Also,
the inferred class may contain an extra state and may display
extra conduct. Fundamentally, the inferred class can likewise
abrogate conduct relating to a portion of the methods of the base
class: there would be an alternate method to react to a similar
Vaibhav Masaye Page | 18
What is Object oriented paradigm? Where do we use?
The Object-Oriented worldview centers around the objects that a
program is speaking to, and on permitting them to display
"conduct". This is diverged from the ordinary methodology in the
basic worldview, in which one ordinarily considers working on
information with systems. In the basic worldview ordinarily, the
information is inactive, the systems are dynamic. In the O-O
worldview, information is joined with methods to give objects, which
are consequently delivered dynamically. For instance, in the basic
worldview, one would compose a methodology which prints the
different sorts of the object in the program. In the O-O worldview,
each object has a print-technique, and "tell" an object to print itself.
It is anyway conceivable to utilize certain non-object-oriented
dialects to compose object-oriented projects. What is required is the
capacity to make information structures that contain machine code,
or pointers to machine code. This is conceivable in the C language
and most practical dialects.
Objects have a place with classes. Commonly, all the objects in a
given class will have similar sorts of conduct.
Classes are generally orchestrated in some sort of class progressive
system. This order can be the idea of as speaking to a" sort of"
connection. For instance, a computational model of the University
may require a class individual to speak to the different individuals
who make up the University. A sub-class of individual may be an
understudy; understudies are a sort of individual. Another sub-class
may be an educator. The two understudies and educators can show
similar sorts of conduct since they are two individuals. The two of
them eat drink and rest, for instance. Be that as it may, there are
unmistakable sorts of conduct: teachers pontificate for instance.
Objects are coordinated into classes, from which they acquire
methods and comparable factors. The object-oriented worldview
gives key advantages of reusable code constantly extensibility.
Benefits of using Object oriented Paradigm are
✓ A new class called an inferred class or subclass might be gotten
from another class called a base class or superclass by an
instrument called inheritance. The determined class acquires all
the highlights of the base class: its structure and conduct. Also,
the inferred class may contain an extra state and may display
extra conduct. Fundamentally, the inferred class can likewise
abrogate conduct relating to a portion of the methods of the base
class: there would be an alternate method to react to a similar
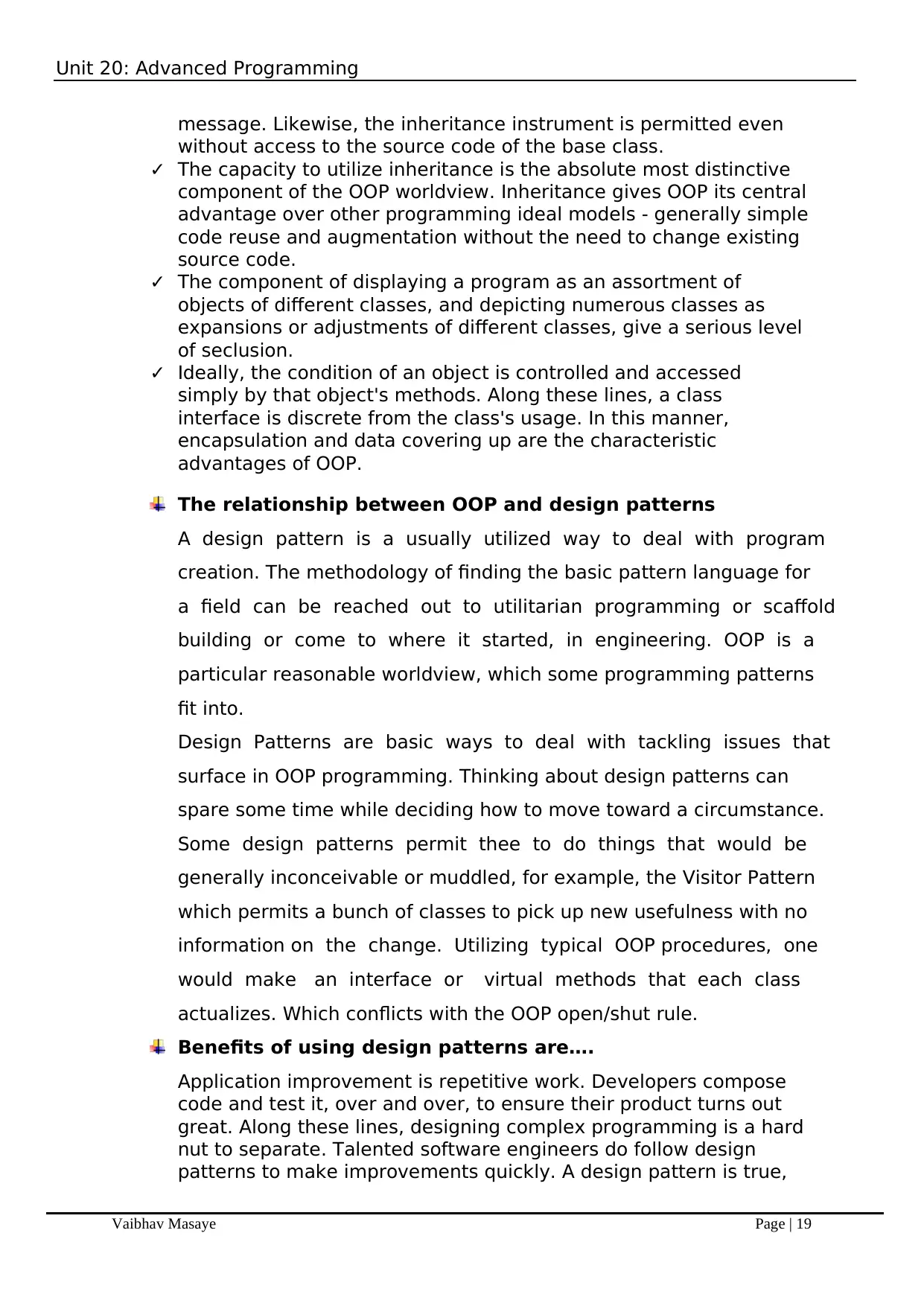
Unit 20: Advanced Programming
Vaibhav Masaye Page | 19
message. Likewise, the inheritance instrument is permitted even
without access to the source code of the base class.
✓ The capacity to utilize inheritance is the absolute most distinctive
component of the OOP worldview. Inheritance gives OOP its central
advantage over other programming ideal models - generally simple
code reuse and augmentation without the need to change existing
source code.
✓ The component of displaying a program as an assortment of
objects of different classes, and depicting numerous classes as
expansions or adjustments of different classes, give a serious level
of seclusion.
✓ Ideally, the condition of an object is controlled and accessed
simply by that object's methods. Along these lines, a class
interface is discrete from the class's usage. In this manner,
encapsulation and data covering up are the characteristic
advantages of OOP.
The relationship between OOP and design patterns
A design pattern is a usually utilized way to deal with program
creation. The methodology of finding the basic pattern language for
a field can be reached out to utilitarian programming or scaffold
building or come to where it started, in engineering. OOP is a
particular reasonable worldview, which some programming patterns
fit into.
Design Patterns are basic ways to deal with tackling issues that
surface in OOP programming. Thinking about design patterns can
spare some time while deciding how to move toward a circumstance.
Some design patterns permit thee to do things that would be
generally inconceivable or muddled, for example, the Visitor Pattern
which permits a bunch of classes to pick up new usefulness with no
information on the change. Utilizing typical OOP procedures, one
would make an interface or virtual methods that each class
actualizes. Which conflicts with the OOP open/shut rule.
Benefits of using design patterns are….
Application improvement is repetitive work. Developers compose
code and test it, over and over, to ensure their product turns out
great. Along these lines, designing complex programming is a hard
nut to separate. Talented software engineers do follow design
patterns to make improvements quickly. A design pattern is true,
Vaibhav Masaye Page | 19
message. Likewise, the inheritance instrument is permitted even
without access to the source code of the base class.
✓ The capacity to utilize inheritance is the absolute most distinctive
component of the OOP worldview. Inheritance gives OOP its central
advantage over other programming ideal models - generally simple
code reuse and augmentation without the need to change existing
source code.
✓ The component of displaying a program as an assortment of
objects of different classes, and depicting numerous classes as
expansions or adjustments of different classes, give a serious level
of seclusion.
✓ Ideally, the condition of an object is controlled and accessed
simply by that object's methods. Along these lines, a class
interface is discrete from the class's usage. In this manner,
encapsulation and data covering up are the characteristic
advantages of OOP.
The relationship between OOP and design patterns
A design pattern is a usually utilized way to deal with program
creation. The methodology of finding the basic pattern language for
a field can be reached out to utilitarian programming or scaffold
building or come to where it started, in engineering. OOP is a
particular reasonable worldview, which some programming patterns
fit into.
Design Patterns are basic ways to deal with tackling issues that
surface in OOP programming. Thinking about design patterns can
spare some time while deciding how to move toward a circumstance.
Some design patterns permit thee to do things that would be
generally inconceivable or muddled, for example, the Visitor Pattern
which permits a bunch of classes to pick up new usefulness with no
information on the change. Utilizing typical OOP procedures, one
would make an interface or virtual methods that each class
actualizes. Which conflicts with the OOP open/shut rule.
Benefits of using design patterns are….
Application improvement is repetitive work. Developers compose
code and test it, over and over, to ensure their product turns out
great. Along these lines, designing complex programming is a hard
nut to separate. Talented software engineers do follow design
patterns to make improvements quickly. A design pattern is true,
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
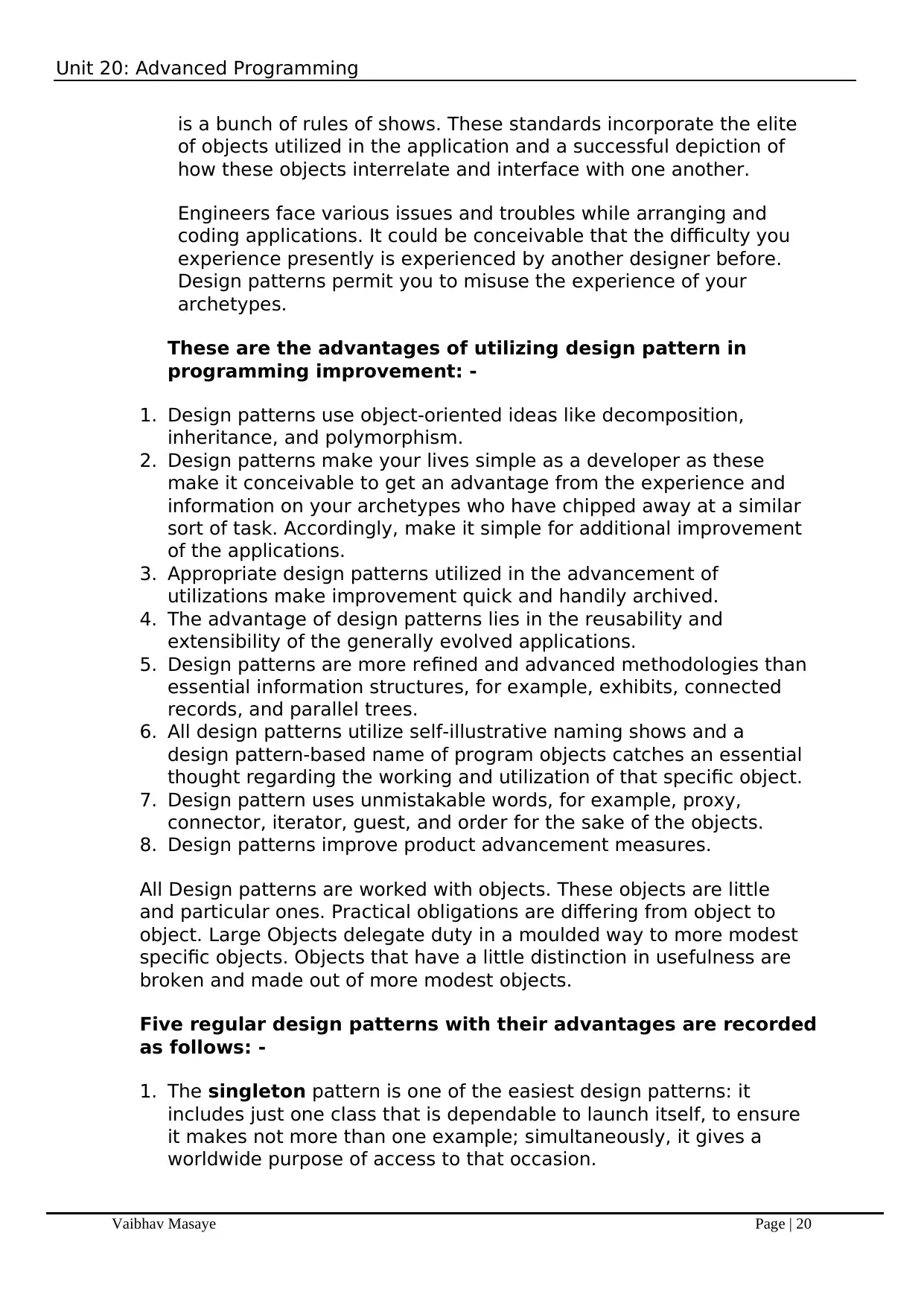
Unit 20: Advanced Programming
Vaibhav Masaye Page | 20
is a bunch of rules of shows. These standards incorporate the elite
of objects utilized in the application and a successful depiction of
how these objects interrelate and interface with one another.
Engineers face various issues and troubles while arranging and
coding applications. It could be conceivable that the difficulty you
experience presently is experienced by another designer before.
Design patterns permit you to misuse the experience of your
archetypes.
These are the advantages of utilizing design pattern in
programming improvement: -
1. Design patterns use object-oriented ideas like decomposition,
inheritance, and polymorphism.
2. Design patterns make your lives simple as a developer as these
make it conceivable to get an advantage from the experience and
information on your archetypes who have chipped away at a similar
sort of task. Accordingly, make it simple for additional improvement
of the applications.
3. Appropriate design patterns utilized in the advancement of
utilizations make improvement quick and handily archived.
4. The advantage of design patterns lies in the reusability and
extensibility of the generally evolved applications.
5. Design patterns are more refined and advanced methodologies than
essential information structures, for example, exhibits, connected
records, and parallel trees.
6. All design patterns utilize self-illustrative naming shows and a
design pattern-based name of program objects catches an essential
thought regarding the working and utilization of that specific object.
7. Design pattern uses unmistakable words, for example, proxy,
connector, iterator, guest, and order for the sake of the objects.
8. Design patterns improve product advancement measures.
All Design patterns are worked with objects. These objects are little
and particular ones. Practical obligations are differing from object to
object. Large Objects delegate duty in a moulded way to more modest
specific objects. Objects that have a little distinction in usefulness are
broken and made out of more modest objects.
Five regular design patterns with their advantages are recorded
as follows: -
1. The singleton pattern is one of the easiest design patterns: it
includes just one class that is dependable to launch itself, to ensure
it makes not more than one example; simultaneously, it gives a
worldwide purpose of access to that occasion.
Vaibhav Masaye Page | 20
is a bunch of rules of shows. These standards incorporate the elite
of objects utilized in the application and a successful depiction of
how these objects interrelate and interface with one another.
Engineers face various issues and troubles while arranging and
coding applications. It could be conceivable that the difficulty you
experience presently is experienced by another designer before.
Design patterns permit you to misuse the experience of your
archetypes.
These are the advantages of utilizing design pattern in
programming improvement: -
1. Design patterns use object-oriented ideas like decomposition,
inheritance, and polymorphism.
2. Design patterns make your lives simple as a developer as these
make it conceivable to get an advantage from the experience and
information on your archetypes who have chipped away at a similar
sort of task. Accordingly, make it simple for additional improvement
of the applications.
3. Appropriate design patterns utilized in the advancement of
utilizations make improvement quick and handily archived.
4. The advantage of design patterns lies in the reusability and
extensibility of the generally evolved applications.
5. Design patterns are more refined and advanced methodologies than
essential information structures, for example, exhibits, connected
records, and parallel trees.
6. All design patterns utilize self-illustrative naming shows and a
design pattern-based name of program objects catches an essential
thought regarding the working and utilization of that specific object.
7. Design pattern uses unmistakable words, for example, proxy,
connector, iterator, guest, and order for the sake of the objects.
8. Design patterns improve product advancement measures.
All Design patterns are worked with objects. These objects are little
and particular ones. Practical obligations are differing from object to
object. Large Objects delegate duty in a moulded way to more modest
specific objects. Objects that have a little distinction in usefulness are
broken and made out of more modest objects.
Five regular design patterns with their advantages are recorded
as follows: -
1. The singleton pattern is one of the easiest design patterns: it
includes just one class that is dependable to launch itself, to ensure
it makes not more than one example; simultaneously, it gives a
worldwide purpose of access to that occasion.
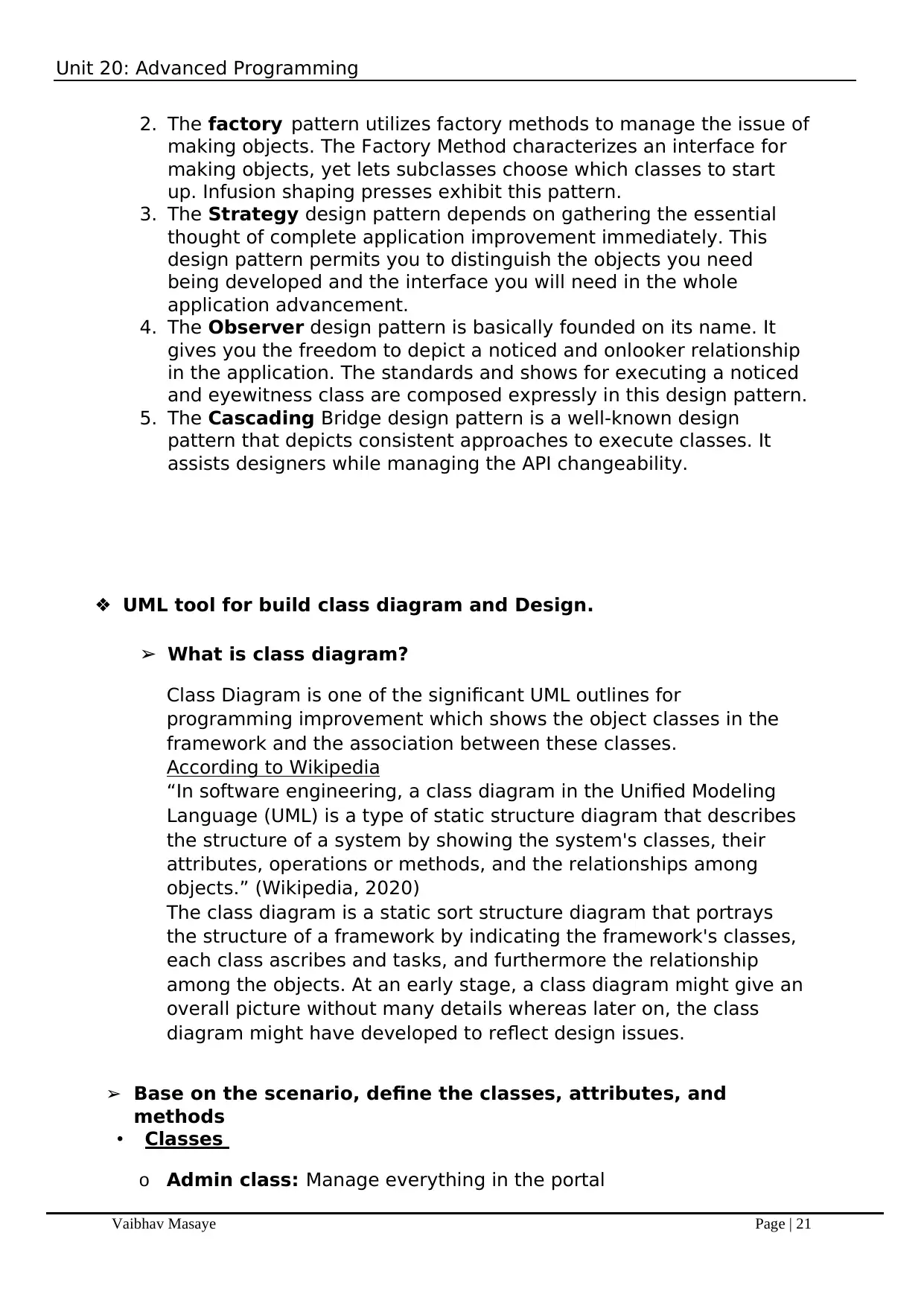
Unit 20: Advanced Programming
Vaibhav Masaye Page | 21
2. The factory pattern utilizes factory methods to manage the issue of
making objects. The Factory Method characterizes an interface for
making objects, yet lets subclasses choose which classes to start
up. Infusion shaping presses exhibit this pattern.
3. The Strategy design pattern depends on gathering the essential
thought of complete application improvement immediately. This
design pattern permits you to distinguish the objects you need
being developed and the interface you will need in the whole
application advancement.
4. The Observer design pattern is basically founded on its name. It
gives you the freedom to depict a noticed and onlooker relationship
in the application. The standards and shows for executing a noticed
and eyewitness class are composed expressly in this design pattern.
5. The Cascading Bridge design pattern is a well-known design
pattern that depicts consistent approaches to execute classes. It
assists designers while managing the API changeability.
❖ UML tool for build class diagram and Design.
➢ What is class diagram?
Class Diagram is one of the significant UML outlines for
programming improvement which shows the object classes in the
framework and the association between these classes.
According to Wikipedia
“In software engineering, a class diagram in the Unified Modeling
Language (UML) is a type of static structure diagram that describes
the structure of a system by showing the system's classes, their
attributes, operations or methods, and the relationships among
objects.” (Wikipedia, 2020)
The class diagram is a static sort structure diagram that portrays
the structure of a framework by indicating the framework's classes,
each class ascribes and tasks, and furthermore the relationship
among the objects. At an early stage, a class diagram might give an
overall picture without many details whereas later on, the class
diagram might have developed to reflect design issues.
➢ Base on the scenario, define the classes, attributes, and
methods
• Classes
o Admin class: Manage everything in the portal
Vaibhav Masaye Page | 21
2. The factory pattern utilizes factory methods to manage the issue of
making objects. The Factory Method characterizes an interface for
making objects, yet lets subclasses choose which classes to start
up. Infusion shaping presses exhibit this pattern.
3. The Strategy design pattern depends on gathering the essential
thought of complete application improvement immediately. This
design pattern permits you to distinguish the objects you need
being developed and the interface you will need in the whole
application advancement.
4. The Observer design pattern is basically founded on its name. It
gives you the freedom to depict a noticed and onlooker relationship
in the application. The standards and shows for executing a noticed
and eyewitness class are composed expressly in this design pattern.
5. The Cascading Bridge design pattern is a well-known design
pattern that depicts consistent approaches to execute classes. It
assists designers while managing the API changeability.
❖ UML tool for build class diagram and Design.
➢ What is class diagram?
Class Diagram is one of the significant UML outlines for
programming improvement which shows the object classes in the
framework and the association between these classes.
According to Wikipedia
“In software engineering, a class diagram in the Unified Modeling
Language (UML) is a type of static structure diagram that describes
the structure of a system by showing the system's classes, their
attributes, operations or methods, and the relationships among
objects.” (Wikipedia, 2020)
The class diagram is a static sort structure diagram that portrays
the structure of a framework by indicating the framework's classes,
each class ascribes and tasks, and furthermore the relationship
among the objects. At an early stage, a class diagram might give an
overall picture without many details whereas later on, the class
diagram might have developed to reflect design issues.
➢ Base on the scenario, define the classes, attributes, and
methods
• Classes
o Admin class: Manage everything in the portal
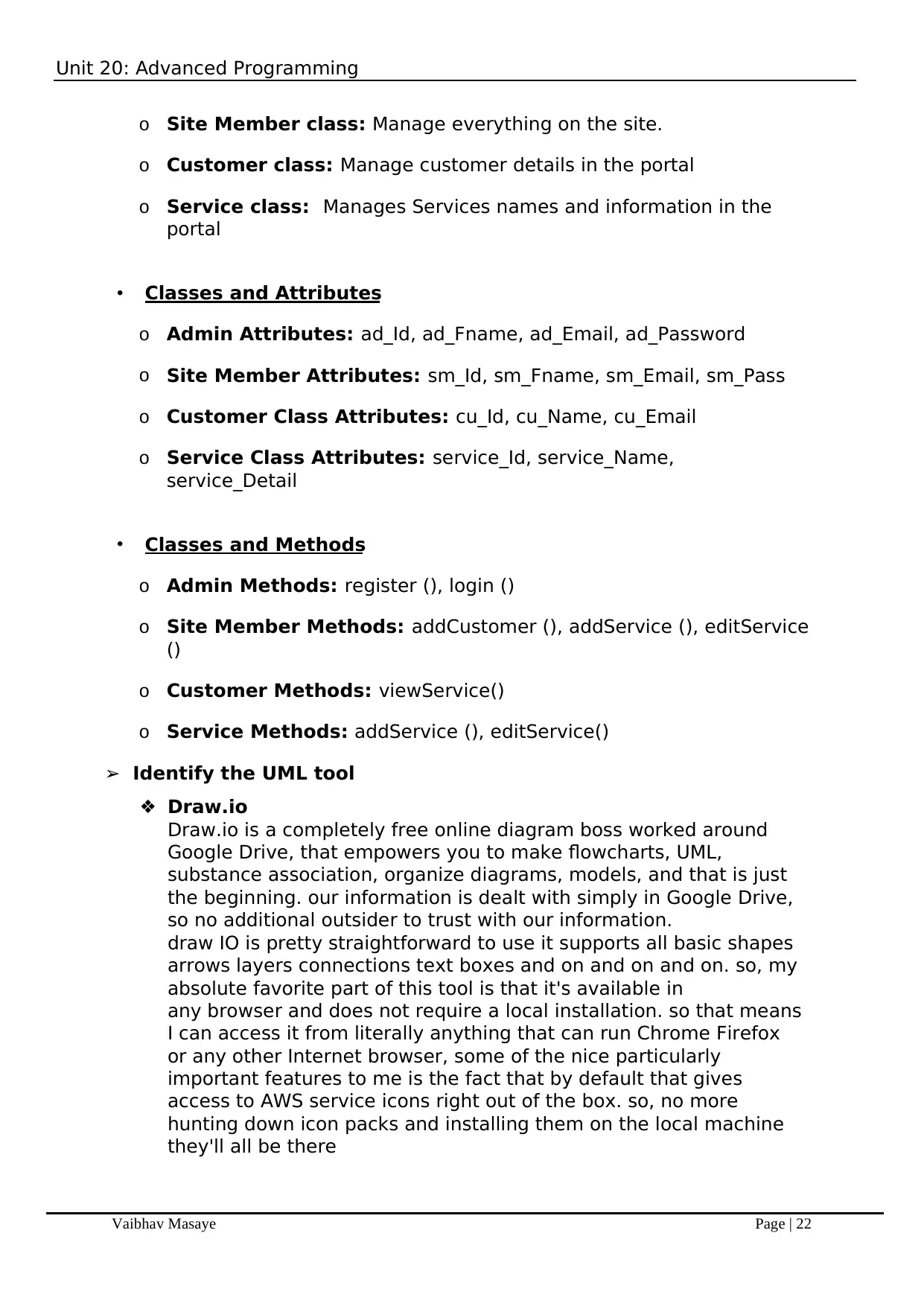
Unit 20: Advanced Programming
Vaibhav Masaye Page | 22
o Site Member class: Manage everything on the site.
o Customer class: Manage customer details in the portal
o Service class: Manages Services names and information in the
portal
• Classes and Attributes
o Admin Attributes: ad_Id, ad_Fname, ad_Email, ad_Password
o Site Member Attributes: sm_Id, sm_Fname, sm_Email, sm_Pass
o Customer Class Attributes: cu_Id, cu_Name, cu_Email
o Service Class Attributes: service_Id, service_Name,
service_Detail
• Classes and Methods
o Admin Methods: register (), login ()
o Site Member Methods: addCustomer (), addService (), editService
()
o Customer Methods: viewService()
o Service Methods: addService (), editService()
➢ Identify the UML tool
❖ Draw.io
Draw.io is a completely free online diagram boss worked around
Google Drive, that empowers you to make flowcharts, UML,
substance association, organize diagrams, models, and that is just
the beginning. our information is dealt with simply in Google Drive,
so no additional outsider to trust with our information.
draw IO is pretty straightforward to use it supports all basic shapes
arrows layers connections text boxes and on and on and on. so, my
absolute favorite part of this tool is that it's available in
any browser and does not require a local installation. so that means
I can access it from literally anything that can run Chrome Firefox
or any other Internet browser, some of the nice particularly
important features to me is the fact that by default that gives
access to AWS service icons right out of the box. so, no more
hunting down icon packs and installing them on the local machine
they'll all be there
Vaibhav Masaye Page | 22
o Site Member class: Manage everything on the site.
o Customer class: Manage customer details in the portal
o Service class: Manages Services names and information in the
portal
• Classes and Attributes
o Admin Attributes: ad_Id, ad_Fname, ad_Email, ad_Password
o Site Member Attributes: sm_Id, sm_Fname, sm_Email, sm_Pass
o Customer Class Attributes: cu_Id, cu_Name, cu_Email
o Service Class Attributes: service_Id, service_Name,
service_Detail
• Classes and Methods
o Admin Methods: register (), login ()
o Site Member Methods: addCustomer (), addService (), editService
()
o Customer Methods: viewService()
o Service Methods: addService (), editService()
➢ Identify the UML tool
❖ Draw.io
Draw.io is a completely free online diagram boss worked around
Google Drive, that empowers you to make flowcharts, UML,
substance association, organize diagrams, models, and that is just
the beginning. our information is dealt with simply in Google Drive,
so no additional outsider to trust with our information.
draw IO is pretty straightforward to use it supports all basic shapes
arrows layers connections text boxes and on and on and on. so, my
absolute favorite part of this tool is that it's available in
any browser and does not require a local installation. so that means
I can access it from literally anything that can run Chrome Firefox
or any other Internet browser, some of the nice particularly
important features to me is the fact that by default that gives
access to AWS service icons right out of the box. so, no more
hunting down icon packs and installing them on the local machine
they'll all be there
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
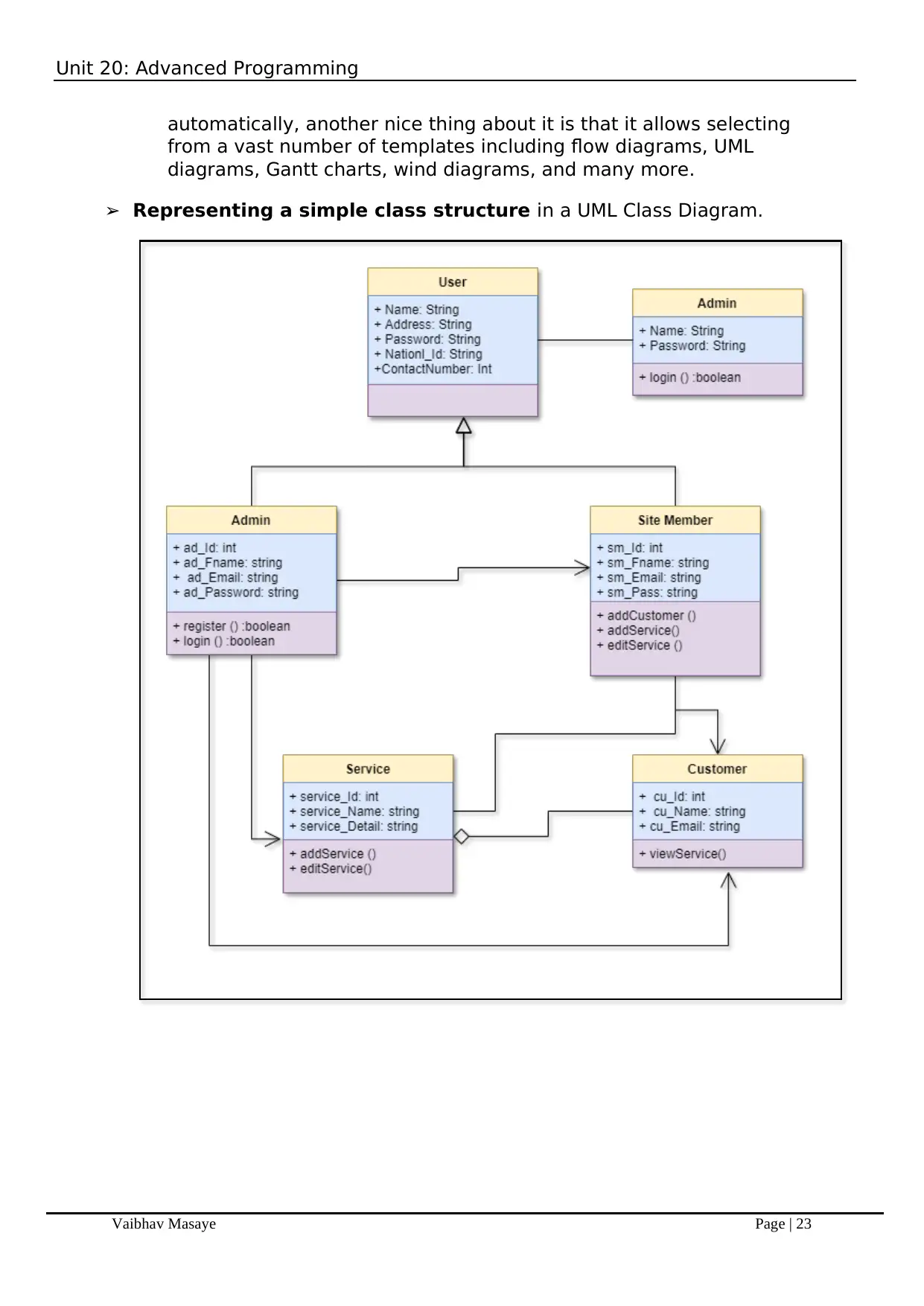
Unit 20: Advanced Programming
Vaibhav Masaye Page | 23
automatically, another nice thing about it is that it allows selecting
from a vast number of templates including flow diagrams, UML
diagrams, Gantt charts, wind diagrams, and many more.
➢ Representing a simple class structure in a UML Class Diagram.
Vaibhav Masaye Page | 23
automatically, another nice thing about it is that it allows selecting
from a vast number of templates including flow diagrams, UML
diagrams, Gantt charts, wind diagrams, and many more.
➢ Representing a simple class structure in a UML Class Diagram.
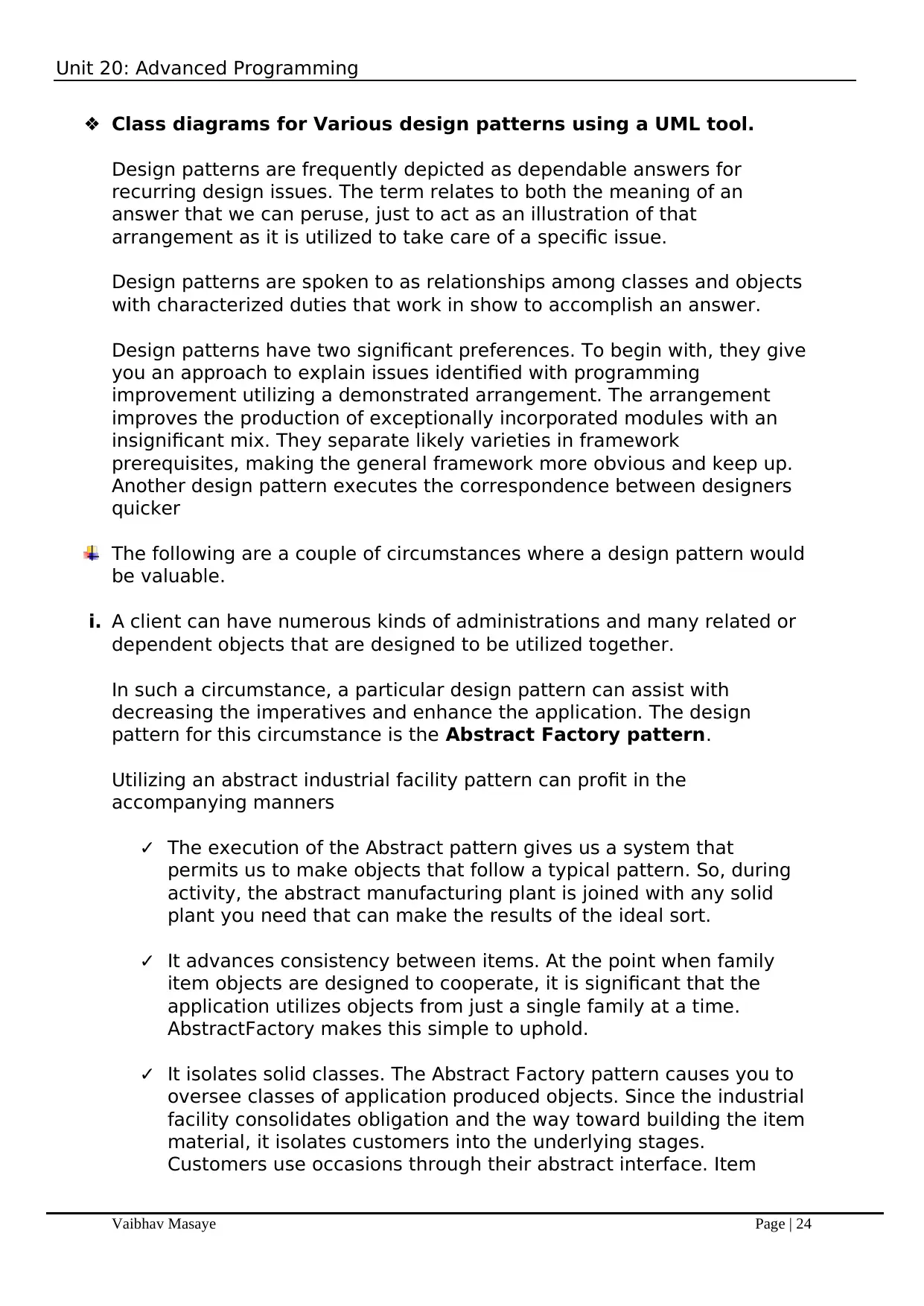
Unit 20: Advanced Programming
Vaibhav Masaye Page | 24
❖ Class diagrams for Various design patterns using a UML tool.
Design patterns are frequently depicted as dependable answers for
recurring design issues. The term relates to both the meaning of an
answer that we can peruse, just to act as an illustration of that
arrangement as it is utilized to take care of a specific issue.
Design patterns are spoken to as relationships among classes and objects
with characterized duties that work in show to accomplish an answer.
Design patterns have two significant preferences. To begin with, they give
you an approach to explain issues identified with programming
improvement utilizing a demonstrated arrangement. The arrangement
improves the production of exceptionally incorporated modules with an
insignificant mix. They separate likely varieties in framework
prerequisites, making the general framework more obvious and keep up.
Another design pattern executes the correspondence between designers
quicker
The following are a couple of circumstances where a design pattern would
be valuable.
i. A client can have numerous kinds of administrations and many related or
dependent objects that are designed to be utilized together.
In such a circumstance, a particular design pattern can assist with
decreasing the imperatives and enhance the application. The design
pattern for this circumstance is the Abstract Factory pattern.
Utilizing an abstract industrial facility pattern can profit in the
accompanying manners
✓ The execution of the Abstract pattern gives us a system that
permits us to make objects that follow a typical pattern. So, during
activity, the abstract manufacturing plant is joined with any solid
plant you need that can make the results of the ideal sort.
✓ It advances consistency between items. At the point when family
item objects are designed to cooperate, it is significant that the
application utilizes objects from just a single family at a time.
AbstractFactory makes this simple to uphold.
✓ It isolates solid classes. The Abstract Factory pattern causes you to
oversee classes of application produced objects. Since the industrial
facility consolidates obligation and the way toward building the item
material, it isolates customers into the underlying stages.
Customers use occasions through their abstract interface. Item
Vaibhav Masaye Page | 24
❖ Class diagrams for Various design patterns using a UML tool.
Design patterns are frequently depicted as dependable answers for
recurring design issues. The term relates to both the meaning of an
answer that we can peruse, just to act as an illustration of that
arrangement as it is utilized to take care of a specific issue.
Design patterns are spoken to as relationships among classes and objects
with characterized duties that work in show to accomplish an answer.
Design patterns have two significant preferences. To begin with, they give
you an approach to explain issues identified with programming
improvement utilizing a demonstrated arrangement. The arrangement
improves the production of exceptionally incorporated modules with an
insignificant mix. They separate likely varieties in framework
prerequisites, making the general framework more obvious and keep up.
Another design pattern executes the correspondence between designers
quicker
The following are a couple of circumstances where a design pattern would
be valuable.
i. A client can have numerous kinds of administrations and many related or
dependent objects that are designed to be utilized together.
In such a circumstance, a particular design pattern can assist with
decreasing the imperatives and enhance the application. The design
pattern for this circumstance is the Abstract Factory pattern.
Utilizing an abstract industrial facility pattern can profit in the
accompanying manners
✓ The execution of the Abstract pattern gives us a system that
permits us to make objects that follow a typical pattern. So, during
activity, the abstract manufacturing plant is joined with any solid
plant you need that can make the results of the ideal sort.
✓ It advances consistency between items. At the point when family
item objects are designed to cooperate, it is significant that the
application utilizes objects from just a single family at a time.
AbstractFactory makes this simple to uphold.
✓ It isolates solid classes. The Abstract Factory pattern causes you to
oversee classes of application produced objects. Since the industrial
facility consolidates obligation and the way toward building the item
material, it isolates customers into the underlying stages.
Customers use occasions through their abstract interface. Item
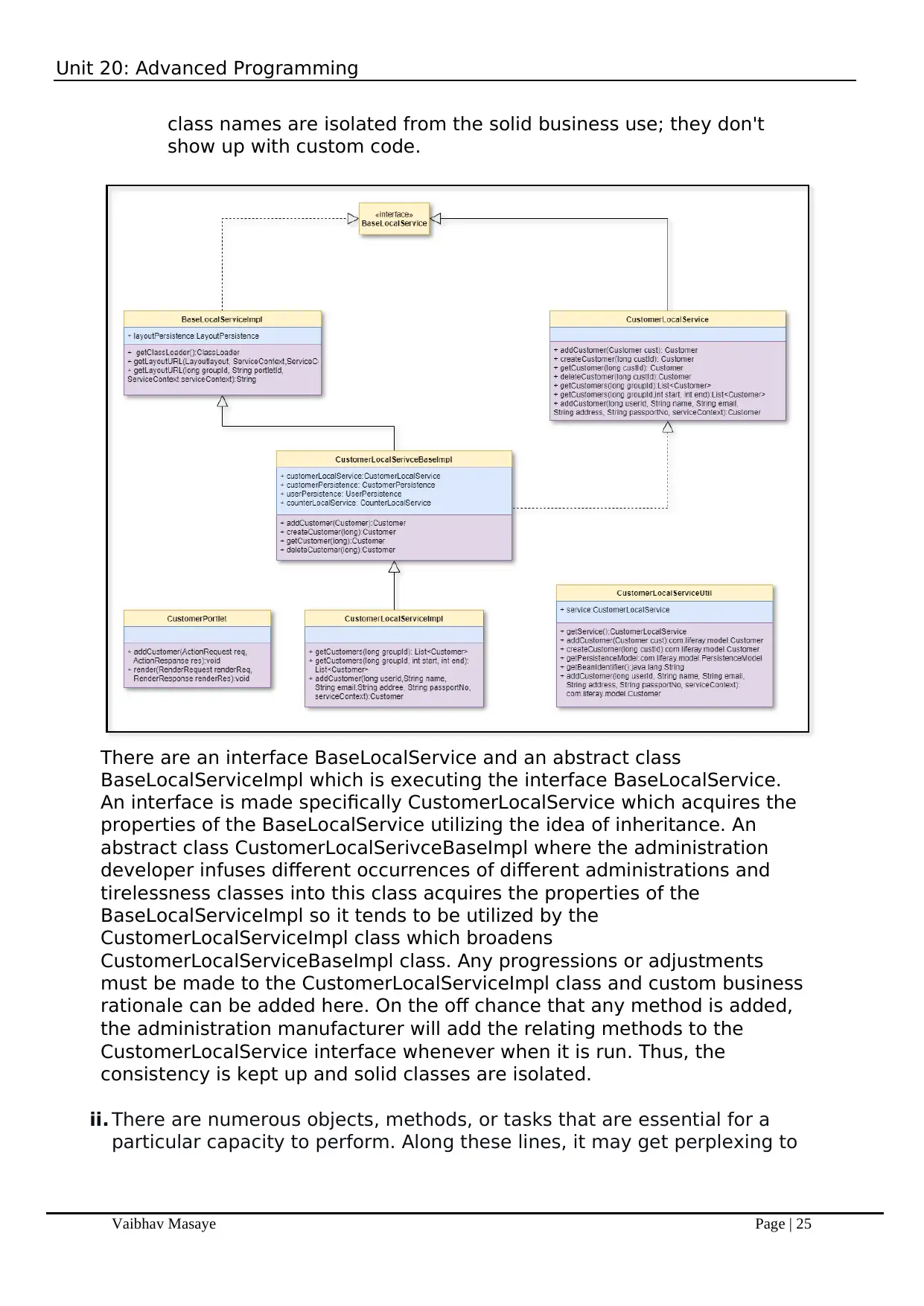
Unit 20: Advanced Programming
Vaibhav Masaye Page | 25
class names are isolated from the solid business use; they don't
show up with custom code.
There are an interface BaseLocalService and an abstract class
BaseLocalServiceImpl which is executing the interface BaseLocalService.
An interface is made specifically CustomerLocalService which acquires the
properties of the BaseLocalService utilizing the idea of inheritance. An
abstract class CustomerLocalSerivceBaseImpl where the administration
developer infuses different occurrences of different administrations and
tirelessness classes into this class acquires the properties of the
BaseLocalServiceImpl so it tends to be utilized by the
CustomerLocalServiceImpl class which broadens
CustomerLocalServiceBaseImpl class. Any progressions or adjustments
must be made to the CustomerLocalServiceImpl class and custom business
rationale can be added here. On the off chance that any method is added,
the administration manufacturer will add the relating methods to the
CustomerLocalService interface whenever when it is run. Thus, the
consistency is kept up and solid classes are isolated.
ii. There are numerous objects, methods, or tasks that are essential for a
particular capacity to perform. Along these lines, it may get perplexing to
Vaibhav Masaye Page | 25
class names are isolated from the solid business use; they don't
show up with custom code.
There are an interface BaseLocalService and an abstract class
BaseLocalServiceImpl which is executing the interface BaseLocalService.
An interface is made specifically CustomerLocalService which acquires the
properties of the BaseLocalService utilizing the idea of inheritance. An
abstract class CustomerLocalSerivceBaseImpl where the administration
developer infuses different occurrences of different administrations and
tirelessness classes into this class acquires the properties of the
BaseLocalServiceImpl so it tends to be utilized by the
CustomerLocalServiceImpl class which broadens
CustomerLocalServiceBaseImpl class. Any progressions or adjustments
must be made to the CustomerLocalServiceImpl class and custom business
rationale can be added here. On the off chance that any method is added,
the administration manufacturer will add the relating methods to the
CustomerLocalService interface whenever when it is run. Thus, the
consistency is kept up and solid classes are isolated.
ii. There are numerous objects, methods, or tasks that are essential for a
particular capacity to perform. Along these lines, it may get perplexing to
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
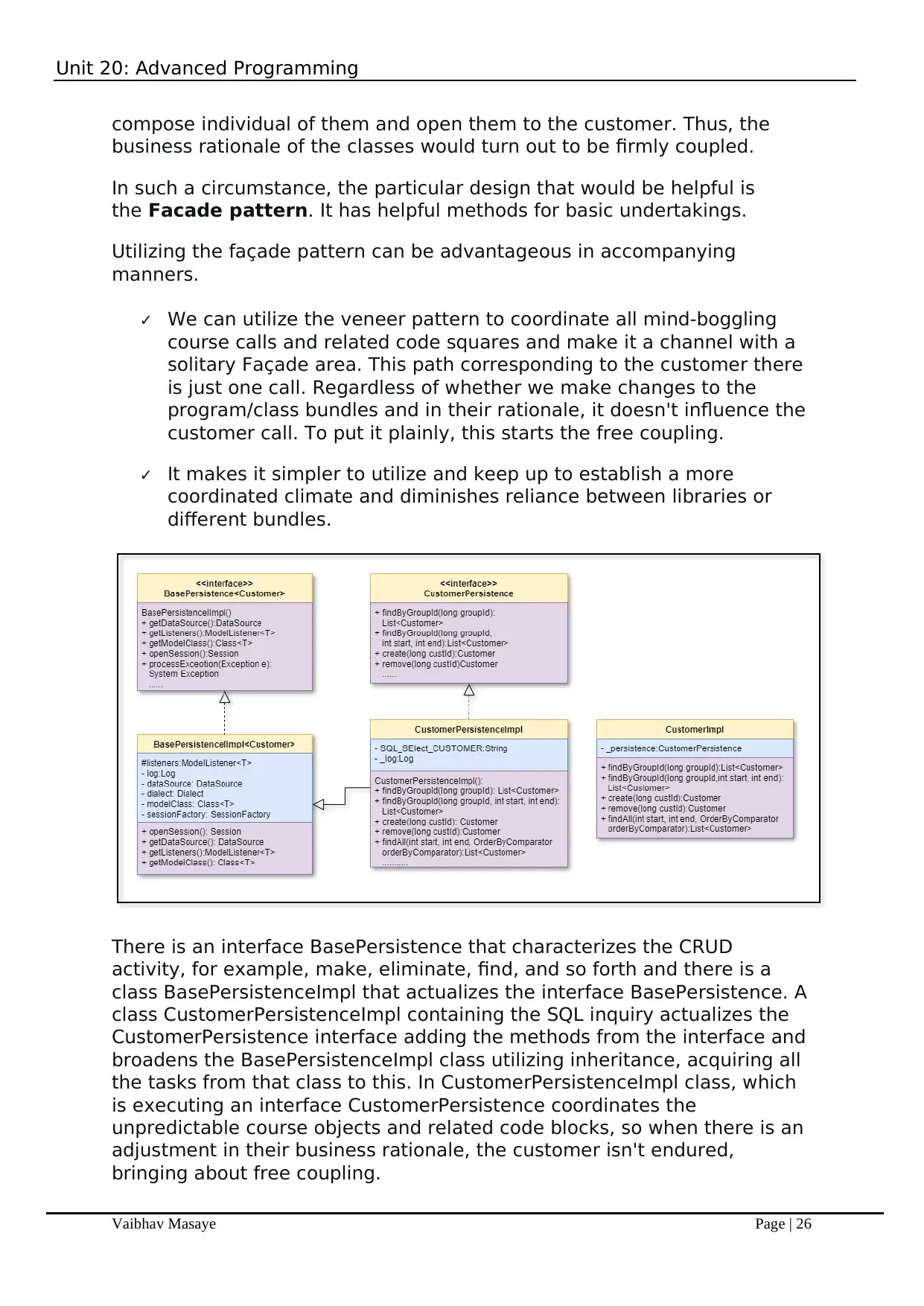
Unit 20: Advanced Programming
Vaibhav Masaye Page | 26
compose individual of them and open them to the customer. Thus, the
business rationale of the classes would turn out to be firmly coupled.
In such a circumstance, the particular design that would be helpful is
the Facade pattern. It has helpful methods for basic undertakings.
Utilizing the façade pattern can be advantageous in accompanying
manners.
✓ We can utilize the veneer pattern to coordinate all mind-boggling
course calls and related code squares and make it a channel with a
solitary Façade area. This path corresponding to the customer there
is just one call. Regardless of whether we make changes to the
program/class bundles and in their rationale, it doesn't influence the
customer call. To put it plainly, this starts the free coupling.
✓ It makes it simpler to utilize and keep up to establish a more
coordinated climate and diminishes reliance between libraries or
different bundles.
There is an interface BasePersistence that characterizes the CRUD
activity, for example, make, eliminate, find, and so forth and there is a
class BasePersistenceImpl that actualizes the interface BasePersistence. A
class CustomerPersistenceImpl containing the SQL inquiry actualizes the
CustomerPersistence interface adding the methods from the interface and
broadens the BasePersistenceImpl class utilizing inheritance, acquiring all
the tasks from that class to this. In CustomerPersistenceImpl class, which
is executing an interface CustomerPersistence coordinates the
unpredictable course objects and related code blocks, so when there is an
adjustment in their business rationale, the customer isn't endured,
bringing about free coupling.
Vaibhav Masaye Page | 26
compose individual of them and open them to the customer. Thus, the
business rationale of the classes would turn out to be firmly coupled.
In such a circumstance, the particular design that would be helpful is
the Facade pattern. It has helpful methods for basic undertakings.
Utilizing the façade pattern can be advantageous in accompanying
manners.
✓ We can utilize the veneer pattern to coordinate all mind-boggling
course calls and related code squares and make it a channel with a
solitary Façade area. This path corresponding to the customer there
is just one call. Regardless of whether we make changes to the
program/class bundles and in their rationale, it doesn't influence the
customer call. To put it plainly, this starts the free coupling.
✓ It makes it simpler to utilize and keep up to establish a more
coordinated climate and diminishes reliance between libraries or
different bundles.
There is an interface BasePersistence that characterizes the CRUD
activity, for example, make, eliminate, find, and so forth and there is a
class BasePersistenceImpl that actualizes the interface BasePersistence. A
class CustomerPersistenceImpl containing the SQL inquiry actualizes the
CustomerPersistence interface adding the methods from the interface and
broadens the BasePersistenceImpl class utilizing inheritance, acquiring all
the tasks from that class to this. In CustomerPersistenceImpl class, which
is executing an interface CustomerPersistence coordinates the
unpredictable course objects and related code blocks, so when there is an
adjustment in their business rationale, the customer isn't endured,
bringing about free coupling.
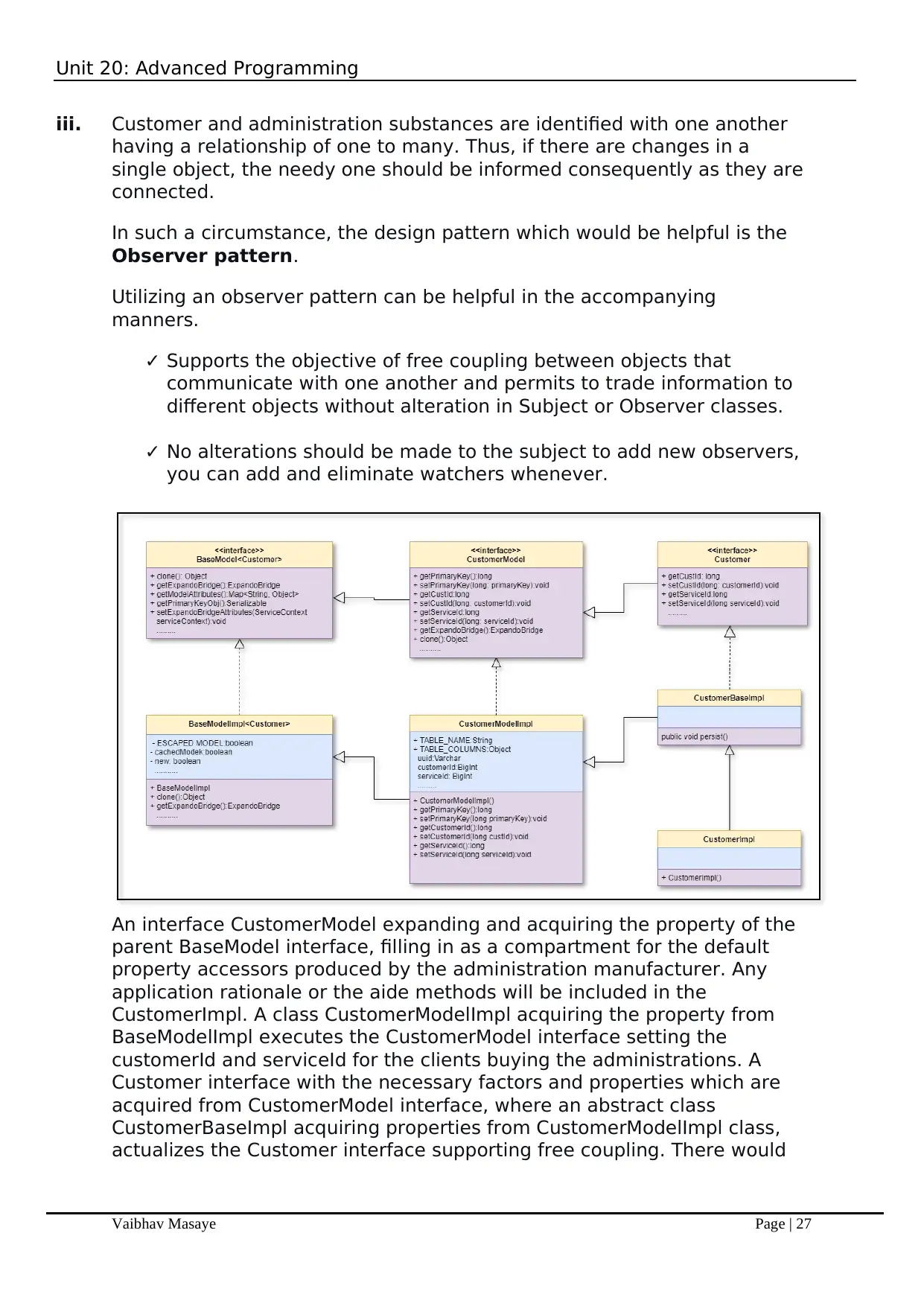
Unit 20: Advanced Programming
Vaibhav Masaye Page | 27
iii. Customer and administration substances are identified with one another
having a relationship of one to many. Thus, if there are changes in a
single object, the needy one should be informed consequently as they are
connected.
In such a circumstance, the design pattern which would be helpful is the
Observer pattern.
Utilizing an observer pattern can be helpful in the accompanying
manners.
✓ Supports the objective of free coupling between objects that
communicate with one another and permits to trade information to
different objects without alteration in Subject or Observer classes.
✓ No alterations should be made to the subject to add new observers,
you can add and eliminate watchers whenever.
An interface CustomerModel expanding and acquiring the property of the
parent BaseModel interface, filling in as a compartment for the default
property accessors produced by the administration manufacturer. Any
application rationale or the aide methods will be included in the
CustomerImpl. A class CustomerModelImpl acquiring the property from
BaseModelImpl executes the CustomerModel interface setting the
customerId and serviceId for the clients buying the administrations. A
Customer interface with the necessary factors and properties which are
acquired from CustomerModel interface, where an abstract class
CustomerBaseImpl acquiring properties from CustomerModelImpl class,
actualizes the Customer interface supporting free coupling. There would
Vaibhav Masaye Page | 27
iii. Customer and administration substances are identified with one another
having a relationship of one to many. Thus, if there are changes in a
single object, the needy one should be informed consequently as they are
connected.
In such a circumstance, the design pattern which would be helpful is the
Observer pattern.
Utilizing an observer pattern can be helpful in the accompanying
manners.
✓ Supports the objective of free coupling between objects that
communicate with one another and permits to trade information to
different objects without alteration in Subject or Observer classes.
✓ No alterations should be made to the subject to add new observers,
you can add and eliminate watchers whenever.
An interface CustomerModel expanding and acquiring the property of the
parent BaseModel interface, filling in as a compartment for the default
property accessors produced by the administration manufacturer. Any
application rationale or the aide methods will be included in the
CustomerImpl. A class CustomerModelImpl acquiring the property from
BaseModelImpl executes the CustomerModel interface setting the
customerId and serviceId for the clients buying the administrations. A
Customer interface with the necessary factors and properties which are
acquired from CustomerModel interface, where an abstract class
CustomerBaseImpl acquiring properties from CustomerModelImpl class,
actualizes the Customer interface supporting free coupling. There would
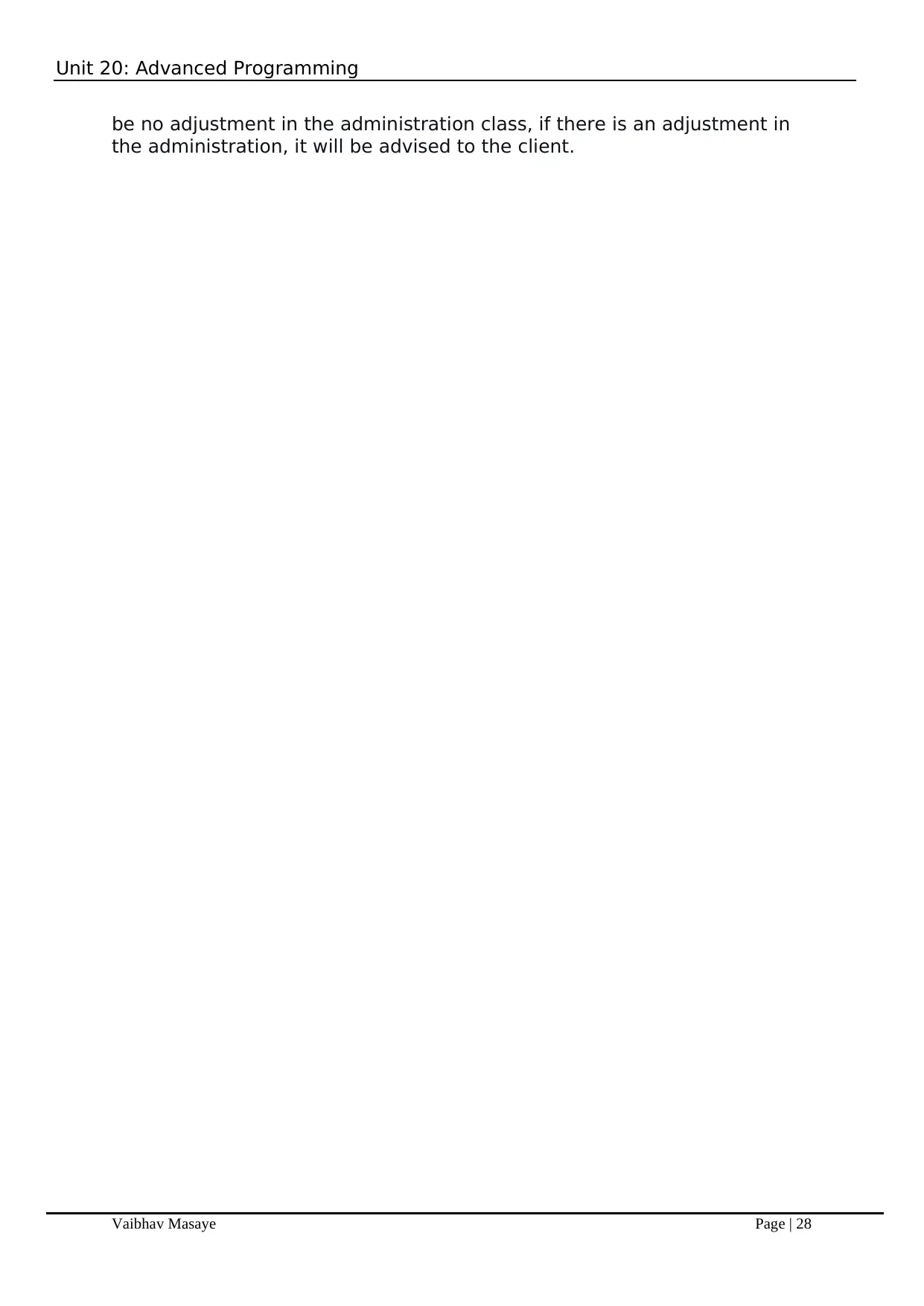
Unit 20: Advanced Programming
Vaibhav Masaye Page | 28
be no adjustment in the administration class, if there is an adjustment in
the administration, it will be advised to the client.
Vaibhav Masaye Page | 28
be no adjustment in the administration class, if there is an adjustment in
the administration, it will be advised to the client.
1 out of 28
Related Documents

Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.