Mr. Bill's Vending Machine System: Data Structures Report
VerifiedAdded on 2022/08/18
|20
|2990
|16
Report
AI Summary
This report examines the implementation of data structures and algorithms for Mr. Bill's vegetarian food store, focusing on a vending machine system. It analyzes the problem domain, including IoT system architecture and system coding platforms, and proposes solutions for managing data (add, edit, delete, view) using ArrayLists, accepting sales information via queues, and analyzing sales revenue using stacks. The report includes pseudocode, flowchart diagrams, and complexity analyses for each operation, demonstrating how these data structures can be effectively utilized to create a real-time information system that integrates with the business processes. The report concludes with a discussion on the compatibility of the system with different combinational data structures. The report also discusses the operations of the selected data structures, pseudo code, flow chart and the complexity analysis of the algorithms for the operations.
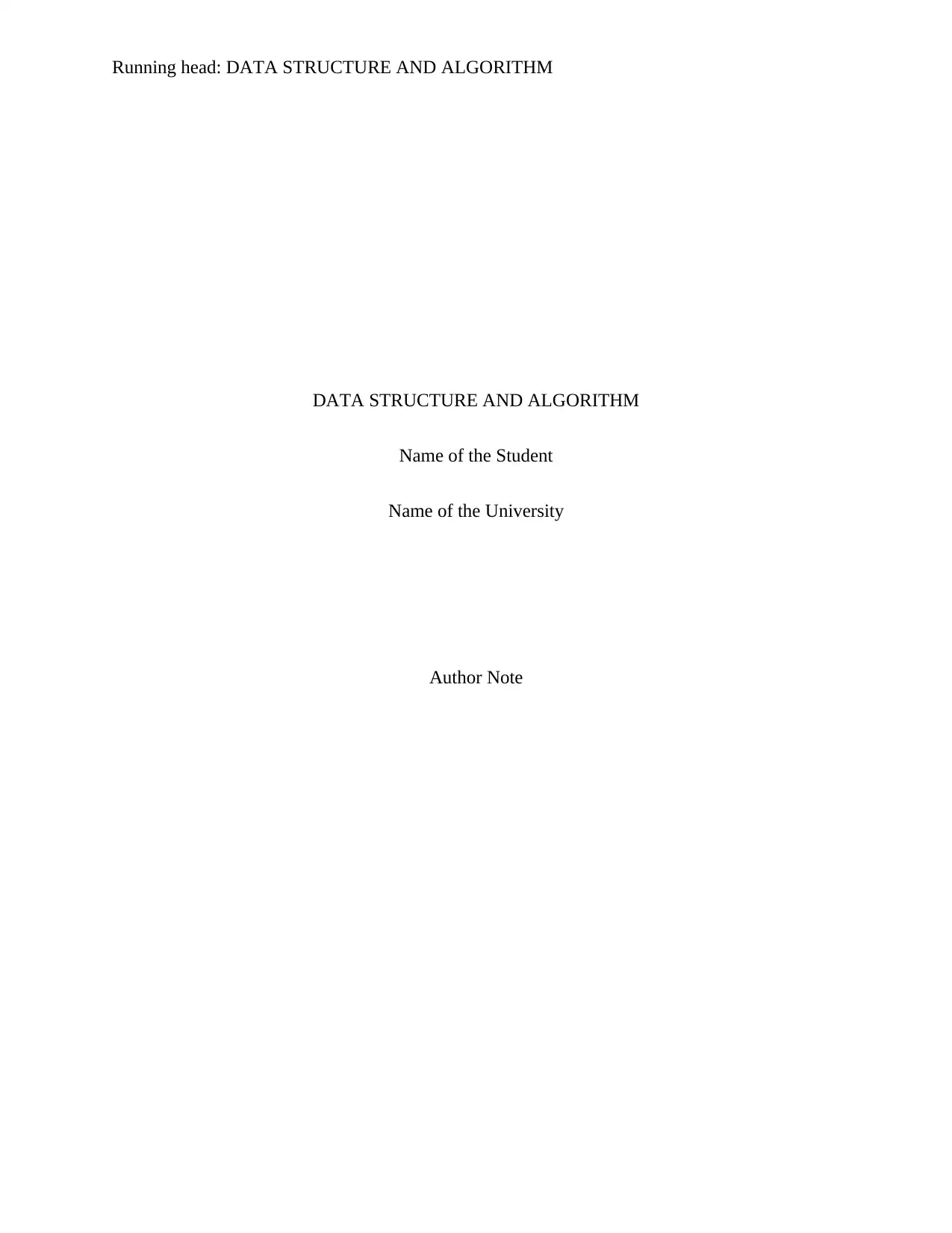
Running head: DATA STRUCTURE AND ALGORITHM
DATA STRUCTURE AND ALGORITHM
Name of the Student
Name of the University
Author Note
DATA STRUCTURE AND ALGORITHM
Name of the Student
Name of the University
Author Note
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser

1DATA STRUCTURE AND ALGORITHM
Table of Contents
Introduction......................................................................................................................................2
Problem Analysis.............................................................................................................................2
Operations........................................................................................................................................4
Manage (add, edit, delete, view)..................................................................................................4
Data Structure..........................................................................................................................4
Pseudo code.............................................................................................................................6
Complexity Analysis.............................................................................................................10
Accept sales information from the vending machines...........................................................10
Analysis the sales revenue for store and/or vending machine...............................................14
Conclusion.....................................................................................................................................17
References......................................................................................................................................18
Table of Contents
Introduction......................................................................................................................................2
Problem Analysis.............................................................................................................................2
Operations........................................................................................................................................4
Manage (add, edit, delete, view)..................................................................................................4
Data Structure..........................................................................................................................4
Pseudo code.............................................................................................................................6
Complexity Analysis.............................................................................................................10
Accept sales information from the vending machines...........................................................10
Analysis the sales revenue for store and/or vending machine...............................................14
Conclusion.....................................................................................................................................17
References......................................................................................................................................18
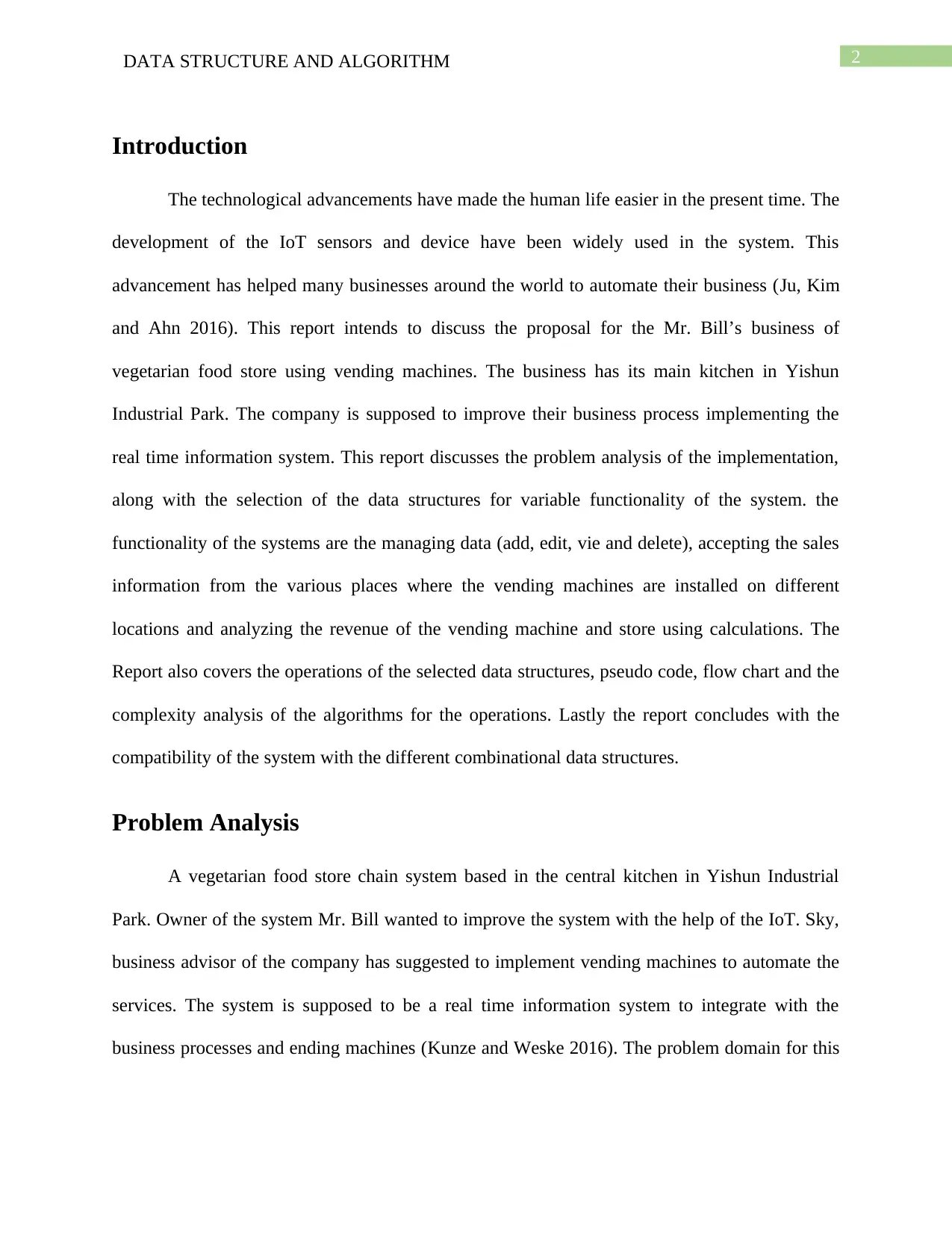
2DATA STRUCTURE AND ALGORITHM
Introduction
The technological advancements have made the human life easier in the present time. The
development of the IoT sensors and device have been widely used in the system. This
advancement has helped many businesses around the world to automate their business (Ju, Kim
and Ahn 2016). This report intends to discuss the proposal for the Mr. Bill’s business of
vegetarian food store using vending machines. The business has its main kitchen in Yishun
Industrial Park. The company is supposed to improve their business process implementing the
real time information system. This report discusses the problem analysis of the implementation,
along with the selection of the data structures for variable functionality of the system. the
functionality of the systems are the managing data (add, edit, vie and delete), accepting the sales
information from the various places where the vending machines are installed on different
locations and analyzing the revenue of the vending machine and store using calculations. The
Report also covers the operations of the selected data structures, pseudo code, flow chart and the
complexity analysis of the algorithms for the operations. Lastly the report concludes with the
compatibility of the system with the different combinational data structures.
Problem Analysis
A vegetarian food store chain system based in the central kitchen in Yishun Industrial
Park. Owner of the system Mr. Bill wanted to improve the system with the help of the IoT. Sky,
business advisor of the company has suggested to implement vending machines to automate the
services. The system is supposed to be a real time information system to integrate with the
business processes and ending machines (Kunze and Weske 2016). The problem domain for this
Introduction
The technological advancements have made the human life easier in the present time. The
development of the IoT sensors and device have been widely used in the system. This
advancement has helped many businesses around the world to automate their business (Ju, Kim
and Ahn 2016). This report intends to discuss the proposal for the Mr. Bill’s business of
vegetarian food store using vending machines. The business has its main kitchen in Yishun
Industrial Park. The company is supposed to improve their business process implementing the
real time information system. This report discusses the problem analysis of the implementation,
along with the selection of the data structures for variable functionality of the system. the
functionality of the systems are the managing data (add, edit, vie and delete), accepting the sales
information from the various places where the vending machines are installed on different
locations and analyzing the revenue of the vending machine and store using calculations. The
Report also covers the operations of the selected data structures, pseudo code, flow chart and the
complexity analysis of the algorithms for the operations. Lastly the report concludes with the
compatibility of the system with the different combinational data structures.
Problem Analysis
A vegetarian food store chain system based in the central kitchen in Yishun Industrial
Park. Owner of the system Mr. Bill wanted to improve the system with the help of the IoT. Sky,
business advisor of the company has suggested to implement vending machines to automate the
services. The system is supposed to be a real time information system to integrate with the
business processes and ending machines (Kunze and Weske 2016). The problem domain for this
You're viewing a preview
Unlock full access by subscribing today!
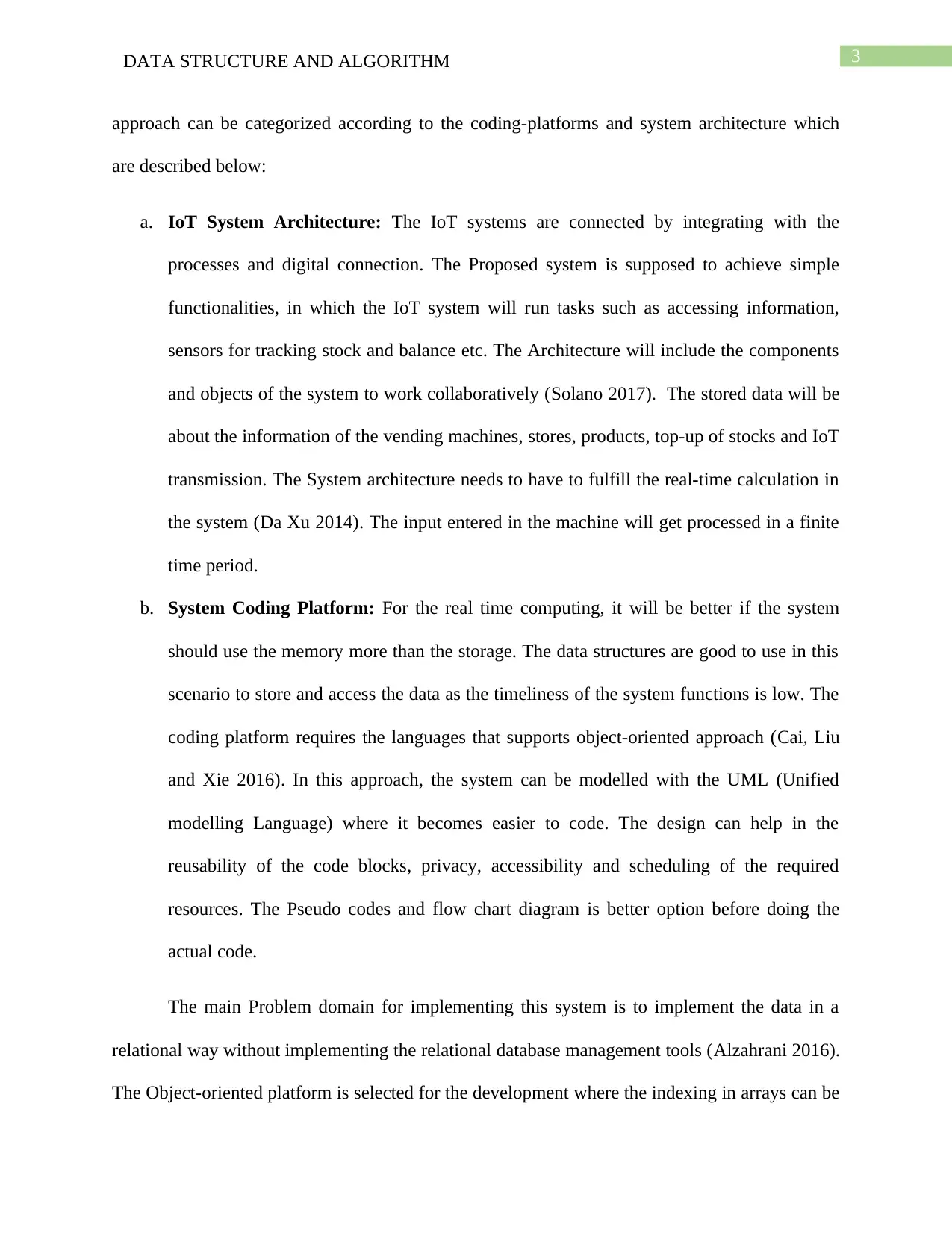
3DATA STRUCTURE AND ALGORITHM
approach can be categorized according to the coding-platforms and system architecture which
are described below:
a. IoT System Architecture: The IoT systems are connected by integrating with the
processes and digital connection. The Proposed system is supposed to achieve simple
functionalities, in which the IoT system will run tasks such as accessing information,
sensors for tracking stock and balance etc. The Architecture will include the components
and objects of the system to work collaboratively (Solano 2017). The stored data will be
about the information of the vending machines, stores, products, top-up of stocks and IoT
transmission. The System architecture needs to have to fulfill the real-time calculation in
the system (Da Xu 2014). The input entered in the machine will get processed in a finite
time period.
b. System Coding Platform: For the real time computing, it will be better if the system
should use the memory more than the storage. The data structures are good to use in this
scenario to store and access the data as the timeliness of the system functions is low. The
coding platform requires the languages that supports object-oriented approach (Cai, Liu
and Xie 2016). In this approach, the system can be modelled with the UML (Unified
modelling Language) where it becomes easier to code. The design can help in the
reusability of the code blocks, privacy, accessibility and scheduling of the required
resources. The Pseudo codes and flow chart diagram is better option before doing the
actual code.
The main Problem domain for implementing this system is to implement the data in a
relational way without implementing the relational database management tools (Alzahrani 2016).
The Object-oriented platform is selected for the development where the indexing in arrays can be
approach can be categorized according to the coding-platforms and system architecture which
are described below:
a. IoT System Architecture: The IoT systems are connected by integrating with the
processes and digital connection. The Proposed system is supposed to achieve simple
functionalities, in which the IoT system will run tasks such as accessing information,
sensors for tracking stock and balance etc. The Architecture will include the components
and objects of the system to work collaboratively (Solano 2017). The stored data will be
about the information of the vending machines, stores, products, top-up of stocks and IoT
transmission. The System architecture needs to have to fulfill the real-time calculation in
the system (Da Xu 2014). The input entered in the machine will get processed in a finite
time period.
b. System Coding Platform: For the real time computing, it will be better if the system
should use the memory more than the storage. The data structures are good to use in this
scenario to store and access the data as the timeliness of the system functions is low. The
coding platform requires the languages that supports object-oriented approach (Cai, Liu
and Xie 2016). In this approach, the system can be modelled with the UML (Unified
modelling Language) where it becomes easier to code. The design can help in the
reusability of the code blocks, privacy, accessibility and scheduling of the required
resources. The Pseudo codes and flow chart diagram is better option before doing the
actual code.
The main Problem domain for implementing this system is to implement the data in a
relational way without implementing the relational database management tools (Alzahrani 2016).
The Object-oriented platform is selected for the development where the indexing in arrays can be
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
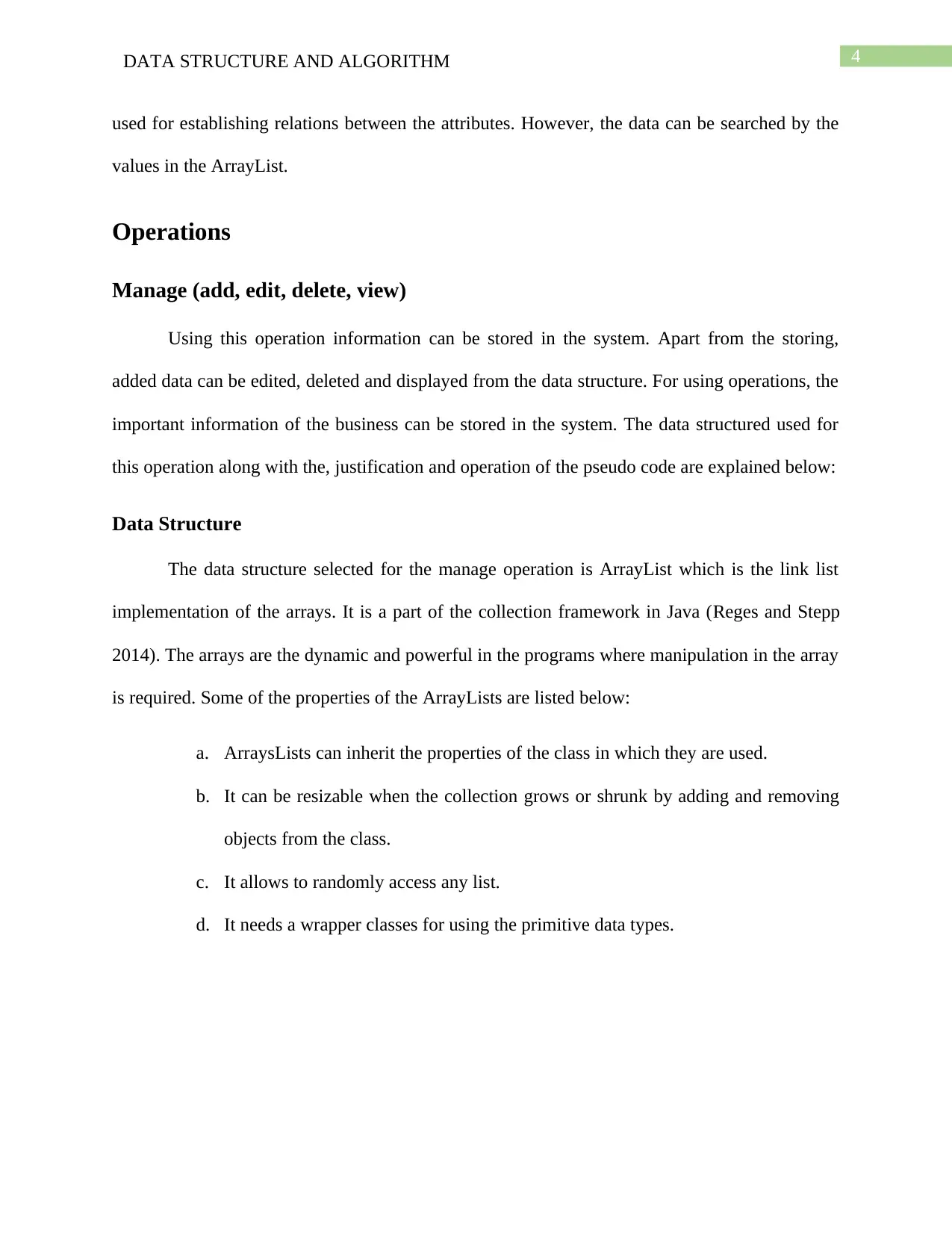
4DATA STRUCTURE AND ALGORITHM
used for establishing relations between the attributes. However, the data can be searched by the
values in the ArrayList.
Operations
Manage (add, edit, delete, view)
Using this operation information can be stored in the system. Apart from the storing,
added data can be edited, deleted and displayed from the data structure. For using operations, the
important information of the business can be stored in the system. The data structured used for
this operation along with the, justification and operation of the pseudo code are explained below:
Data Structure
The data structure selected for the manage operation is ArrayList which is the link list
implementation of the arrays. It is a part of the collection framework in Java (Reges and Stepp
2014). The arrays are the dynamic and powerful in the programs where manipulation in the array
is required. Some of the properties of the ArrayLists are listed below:
a. ArraysLists can inherit the properties of the class in which they are used.
b. It can be resizable when the collection grows or shrunk by adding and removing
objects from the class.
c. It allows to randomly access any list.
d. It needs a wrapper classes for using the primitive data types.
used for establishing relations between the attributes. However, the data can be searched by the
values in the ArrayList.
Operations
Manage (add, edit, delete, view)
Using this operation information can be stored in the system. Apart from the storing,
added data can be edited, deleted and displayed from the data structure. For using operations, the
important information of the business can be stored in the system. The data structured used for
this operation along with the, justification and operation of the pseudo code are explained below:
Data Structure
The data structure selected for the manage operation is ArrayList which is the link list
implementation of the arrays. It is a part of the collection framework in Java (Reges and Stepp
2014). The arrays are the dynamic and powerful in the programs where manipulation in the array
is required. Some of the properties of the ArrayLists are listed below:
a. ArraysLists can inherit the properties of the class in which they are used.
b. It can be resizable when the collection grows or shrunk by adding and removing
objects from the class.
c. It allows to randomly access any list.
d. It needs a wrapper classes for using the primitive data types.
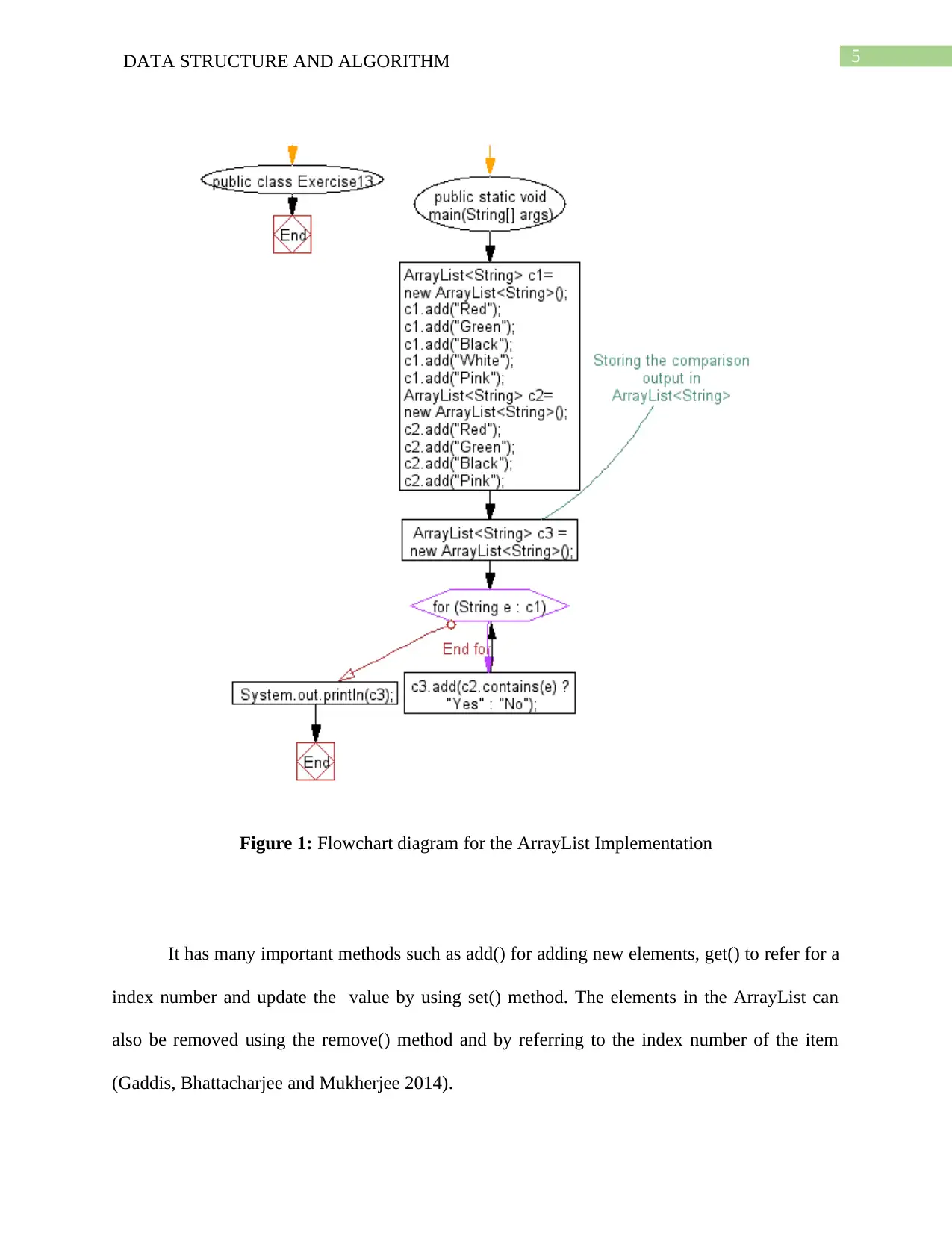
5DATA STRUCTURE AND ALGORITHM
Figure 1: Flowchart diagram for the ArrayList Implementation
It has many important methods such as add() for adding new elements, get() to refer for a
index number and update the value by using set() method. The elements in the ArrayList can
also be removed using the remove() method and by referring to the index number of the item
(Gaddis, Bhattacharjee and Mukherjee 2014).
Figure 1: Flowchart diagram for the ArrayList Implementation
It has many important methods such as add() for adding new elements, get() to refer for a
index number and update the value by using set() method. The elements in the ArrayList can
also be removed using the remove() method and by referring to the index number of the item
(Gaddis, Bhattacharjee and Mukherjee 2014).
You're viewing a preview
Unlock full access by subscribing today!
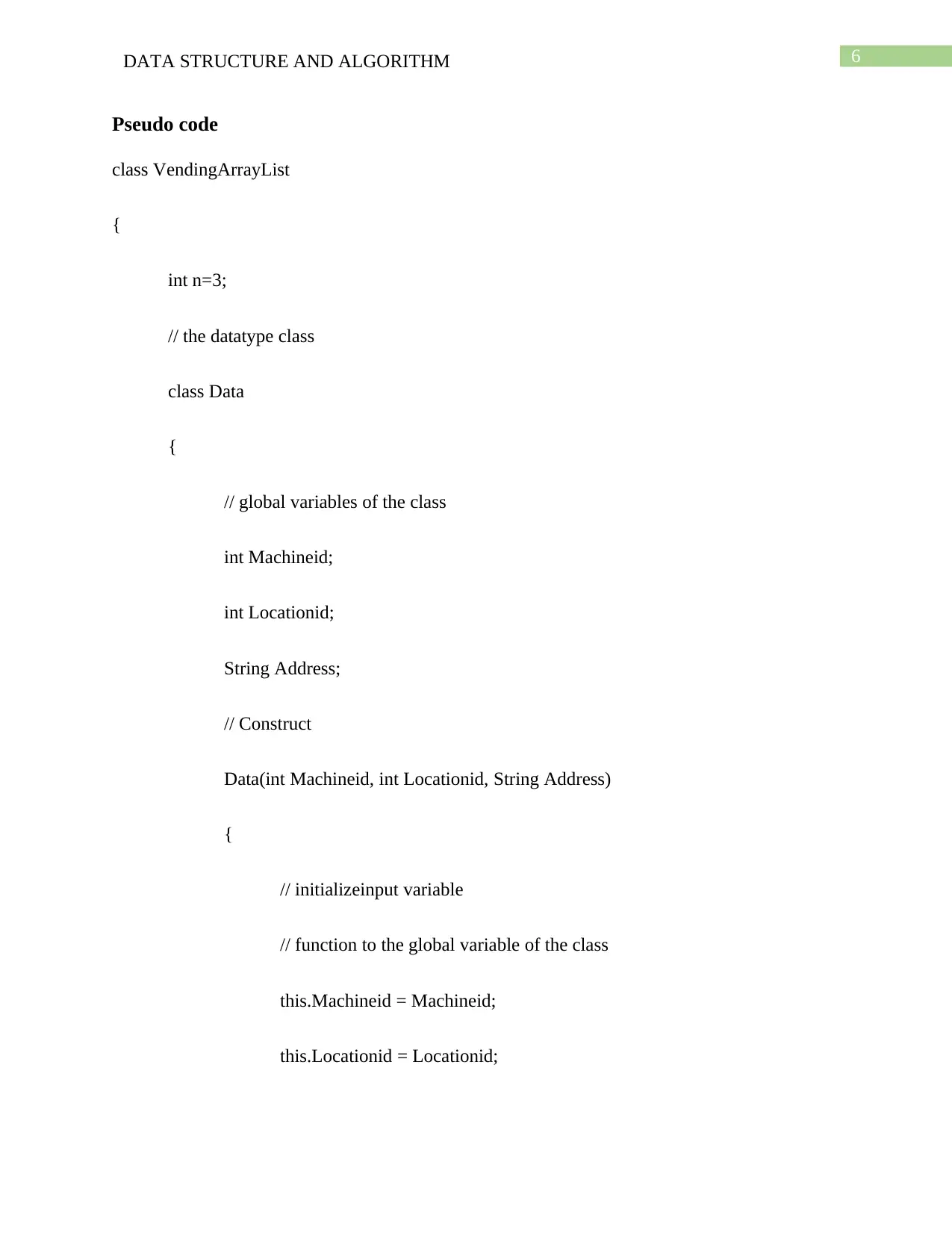
6DATA STRUCTURE AND ALGORITHM
Pseudo code
class VendingArrayList
{
int n=3;
// the datatype class
class Data
{
// global variables of the class
int Machineid;
int Locationid;
String Address;
// Construct
Data(int Machineid, int Locationid, String Address)
{
// initializeinput variable
// function to the global variable of the class
this.Machineid = Machineid;
this.Locationid = Locationid;
Pseudo code
class VendingArrayList
{
int n=3;
// the datatype class
class Data
{
// global variables of the class
int Machineid;
int Locationid;
String Address;
// Construct
Data(int Machineid, int Locationid, String Address)
{
// initializeinput variable
// function to the global variable of the class
this.Machineid = Machineid;
this.Locationid = Locationid;
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
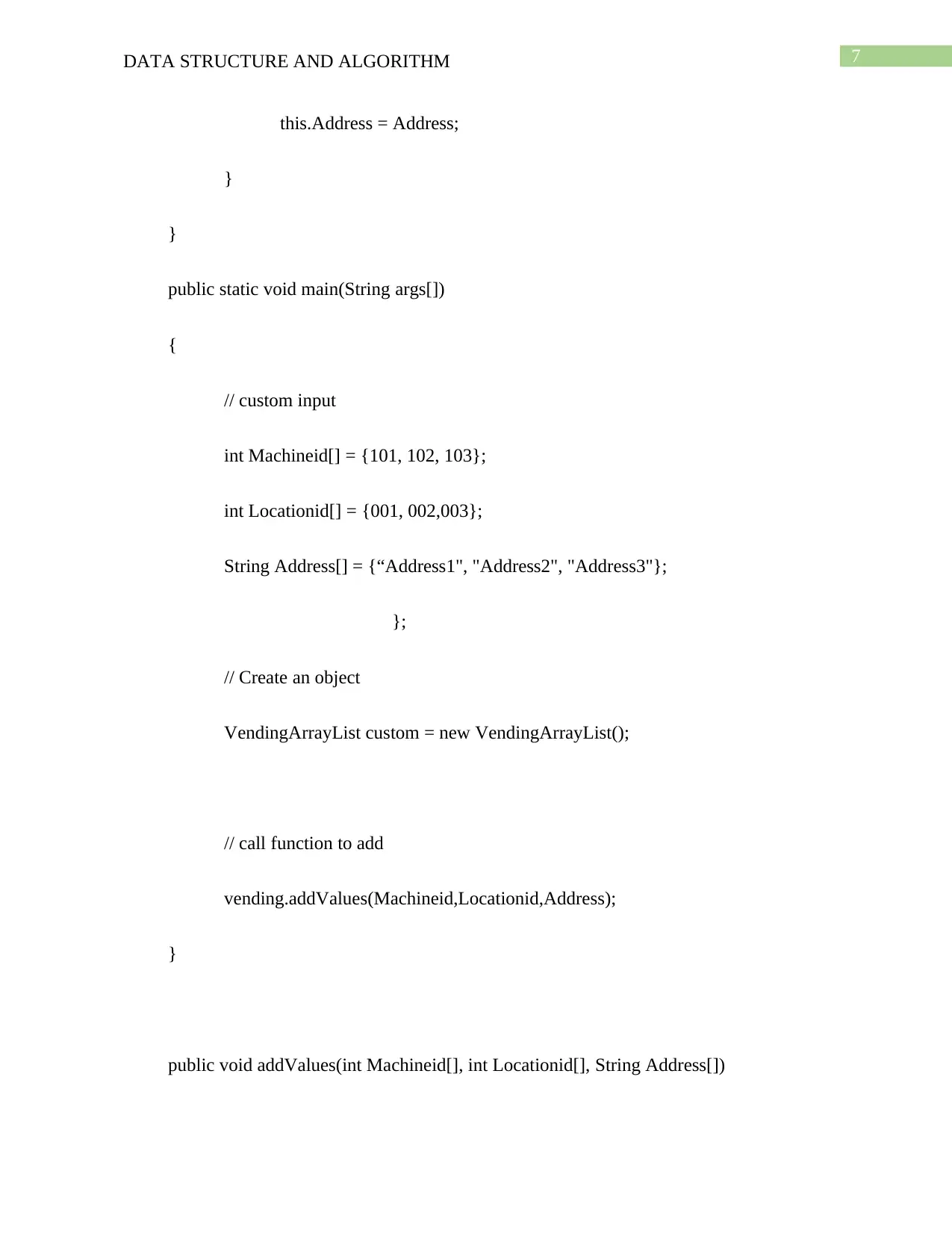
7DATA STRUCTURE AND ALGORITHM
this.Address = Address;
}
}
public static void main(String args[])
{
// custom input
int Machineid[] = {101, 102, 103};
int Locationid[] = {001, 002,003};
String Address[] = {“Address1", "Address2", "Address3"};
};
// Create an object
VendingArrayList custom = new VendingArrayList();
// call function to add
vending.addValues(Machineid,Locationid,Address);
}
public void addValues(int Machineid[], int Locationid[], String Address[])
this.Address = Address;
}
}
public static void main(String args[])
{
// custom input
int Machineid[] = {101, 102, 103};
int Locationid[] = {001, 002,003};
String Address[] = {“Address1", "Address2", "Address3"};
};
// Create an object
VendingArrayList custom = new VendingArrayList();
// call function to add
vending.addValues(Machineid,Locationid,Address);
}
public void addValues(int Machineid[], int Locationid[], String Address[])
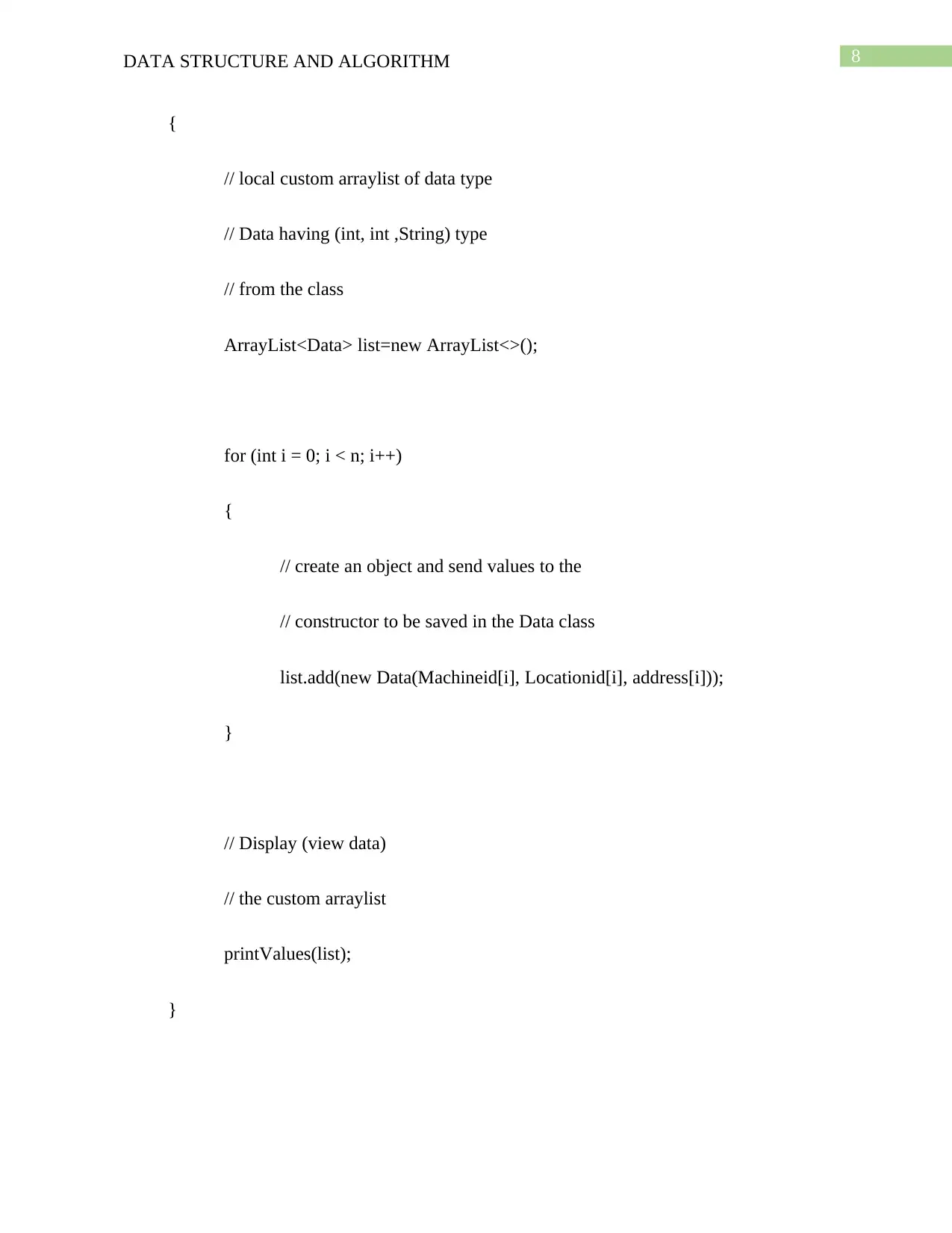
8DATA STRUCTURE AND ALGORITHM
{
// local custom arraylist of data type
// Data having (int, int ,String) type
// from the class
ArrayList<Data> list=new ArrayList<>();
for (int i = 0; i < n; i++)
{
// create an object and send values to the
// constructor to be saved in the Data class
list.add(new Data(Machineid[i], Locationid[i], address[i]));
}
// Display (view data)
// the custom arraylist
printValues(list);
}
{
// local custom arraylist of data type
// Data having (int, int ,String) type
// from the class
ArrayList<Data> list=new ArrayList<>();
for (int i = 0; i < n; i++)
{
// create an object and send values to the
// constructor to be saved in the Data class
list.add(new Data(Machineid[i], Locationid[i], address[i]));
}
// Display (view data)
// the custom arraylist
printValues(list);
}
You're viewing a preview
Unlock full access by subscribing today!
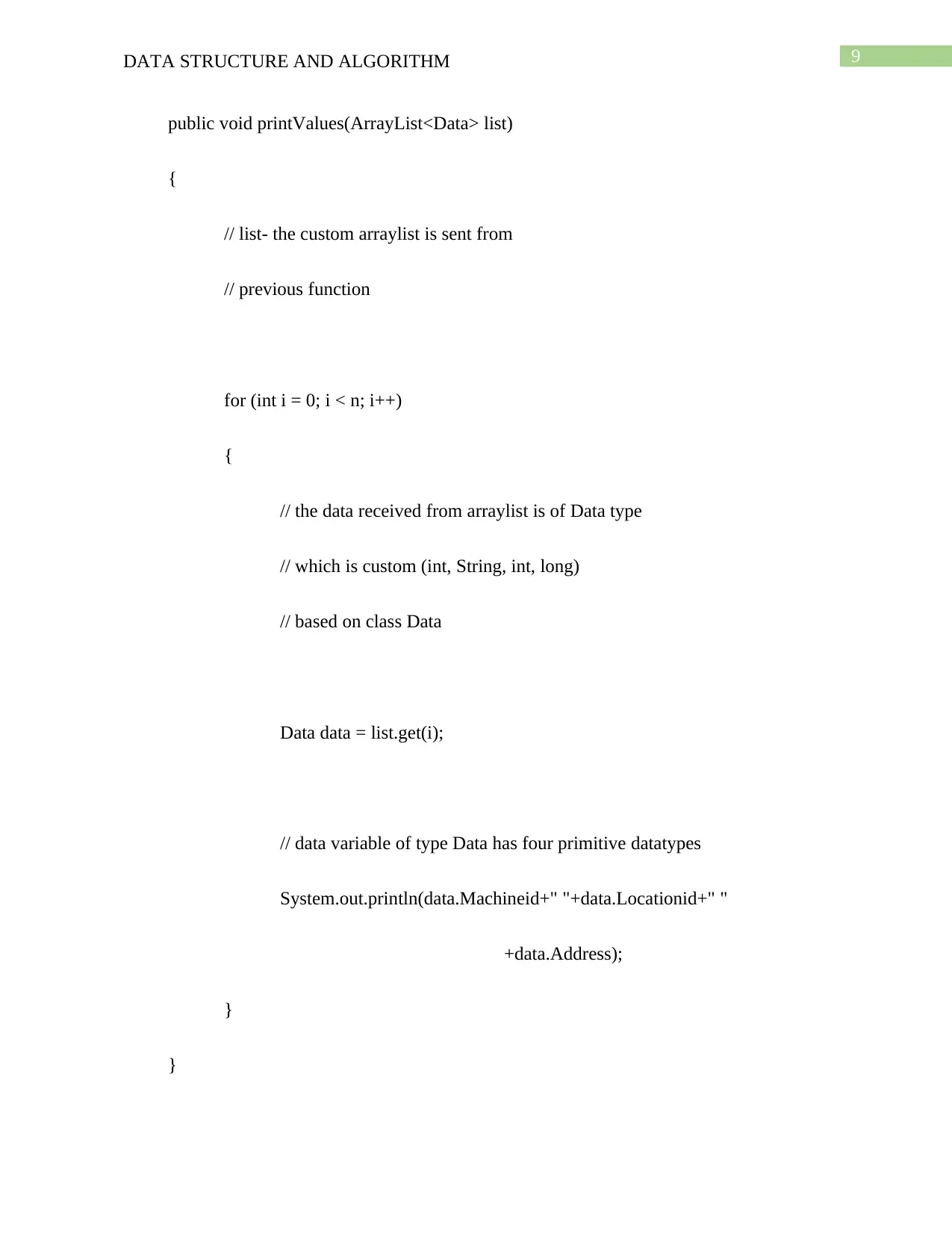
9DATA STRUCTURE AND ALGORITHM
public void printValues(ArrayList<Data> list)
{
// list- the custom arraylist is sent from
// previous function
for (int i = 0; i < n; i++)
{
// the data received from arraylist is of Data type
// which is custom (int, String, int, long)
// based on class Data
Data data = list.get(i);
// data variable of type Data has four primitive datatypes
System.out.println(data.Machineid+" "+data.Locationid+" "
+data.Address);
}
}
public void printValues(ArrayList<Data> list)
{
// list- the custom arraylist is sent from
// previous function
for (int i = 0; i < n; i++)
{
// the data received from arraylist is of Data type
// which is custom (int, String, int, long)
// based on class Data
Data data = list.get(i);
// data variable of type Data has four primitive datatypes
System.out.println(data.Machineid+" "+data.Locationid+" "
+data.Address);
}
}
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
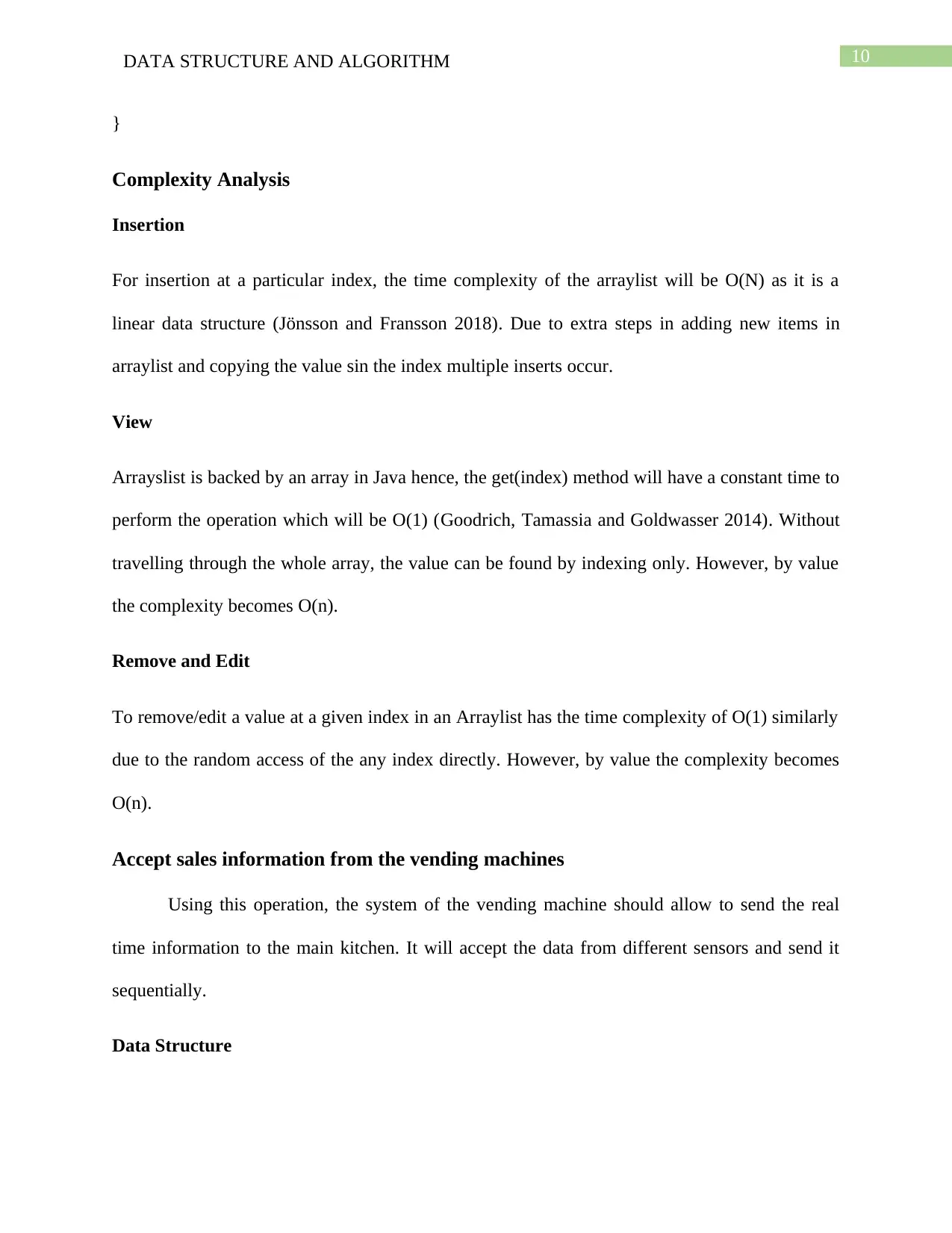
10DATA STRUCTURE AND ALGORITHM
}
Complexity Analysis
Insertion
For insertion at a particular index, the time complexity of the arraylist will be O(N) as it is a
linear data structure (Jönsson and Fransson 2018). Due to extra steps in adding new items in
arraylist and copying the value sin the index multiple inserts occur.
View
Arrayslist is backed by an array in Java hence, the get(index) method will have a constant time to
perform the operation which will be O(1) (Goodrich, Tamassia and Goldwasser 2014). Without
travelling through the whole array, the value can be found by indexing only. However, by value
the complexity becomes O(n).
Remove and Edit
To remove/edit a value at a given index in an Arraylist has the time complexity of O(1) similarly
due to the random access of the any index directly. However, by value the complexity becomes
O(n).
Accept sales information from the vending machines
Using this operation, the system of the vending machine should allow to send the real
time information to the main kitchen. It will accept the data from different sensors and send it
sequentially.
Data Structure
}
Complexity Analysis
Insertion
For insertion at a particular index, the time complexity of the arraylist will be O(N) as it is a
linear data structure (Jönsson and Fransson 2018). Due to extra steps in adding new items in
arraylist and copying the value sin the index multiple inserts occur.
View
Arrayslist is backed by an array in Java hence, the get(index) method will have a constant time to
perform the operation which will be O(1) (Goodrich, Tamassia and Goldwasser 2014). Without
travelling through the whole array, the value can be found by indexing only. However, by value
the complexity becomes O(n).
Remove and Edit
To remove/edit a value at a given index in an Arraylist has the time complexity of O(1) similarly
due to the random access of the any index directly. However, by value the complexity becomes
O(n).
Accept sales information from the vending machines
Using this operation, the system of the vending machine should allow to send the real
time information to the main kitchen. It will accept the data from different sensors and send it
sequentially.
Data Structure
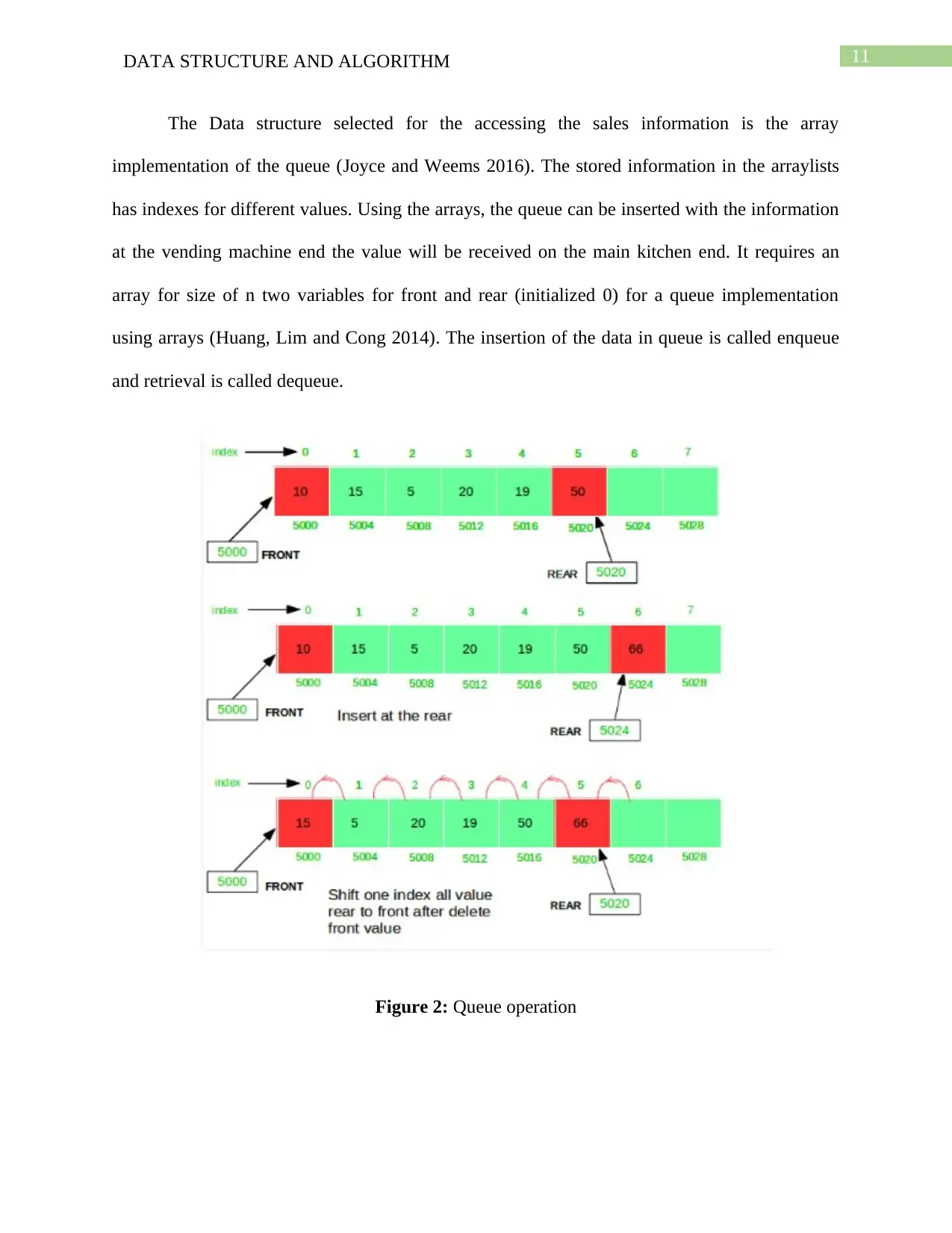
11DATA STRUCTURE AND ALGORITHM
The Data structure selected for the accessing the sales information is the array
implementation of the queue (Joyce and Weems 2016). The stored information in the arraylists
has indexes for different values. Using the arrays, the queue can be inserted with the information
at the vending machine end the value will be received on the main kitchen end. It requires an
array for size of n two variables for front and rear (initialized 0) for a queue implementation
using arrays (Huang, Lim and Cong 2014). The insertion of the data in queue is called enqueue
and retrieval is called dequeue.
Figure 2: Queue operation
The Data structure selected for the accessing the sales information is the array
implementation of the queue (Joyce and Weems 2016). The stored information in the arraylists
has indexes for different values. Using the arrays, the queue can be inserted with the information
at the vending machine end the value will be received on the main kitchen end. It requires an
array for size of n two variables for front and rear (initialized 0) for a queue implementation
using arrays (Huang, Lim and Cong 2014). The insertion of the data in queue is called enqueue
and retrieval is called dequeue.
Figure 2: Queue operation
You're viewing a preview
Unlock full access by subscribing today!
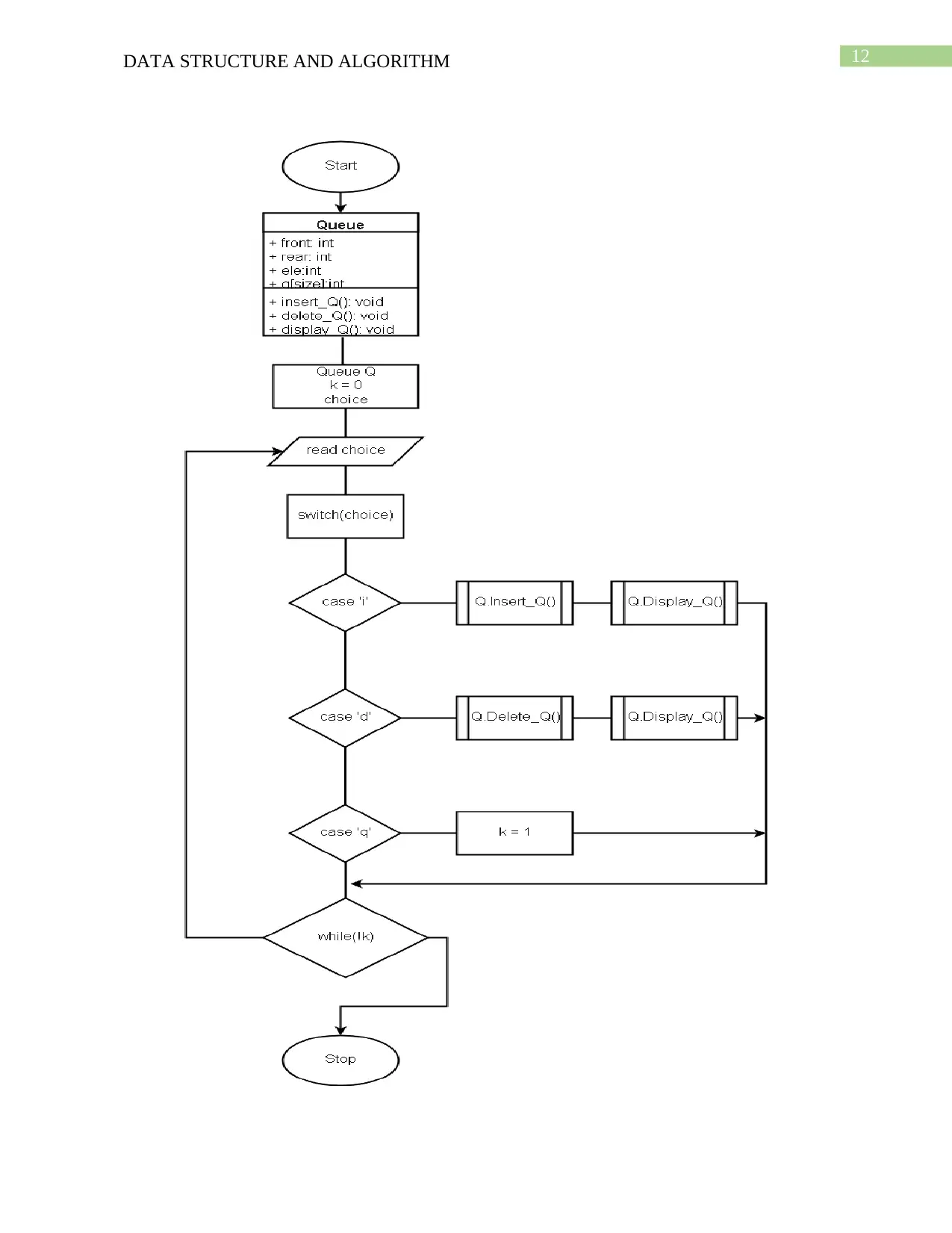
12DATA STRUCTURE AND ALGORITHM
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
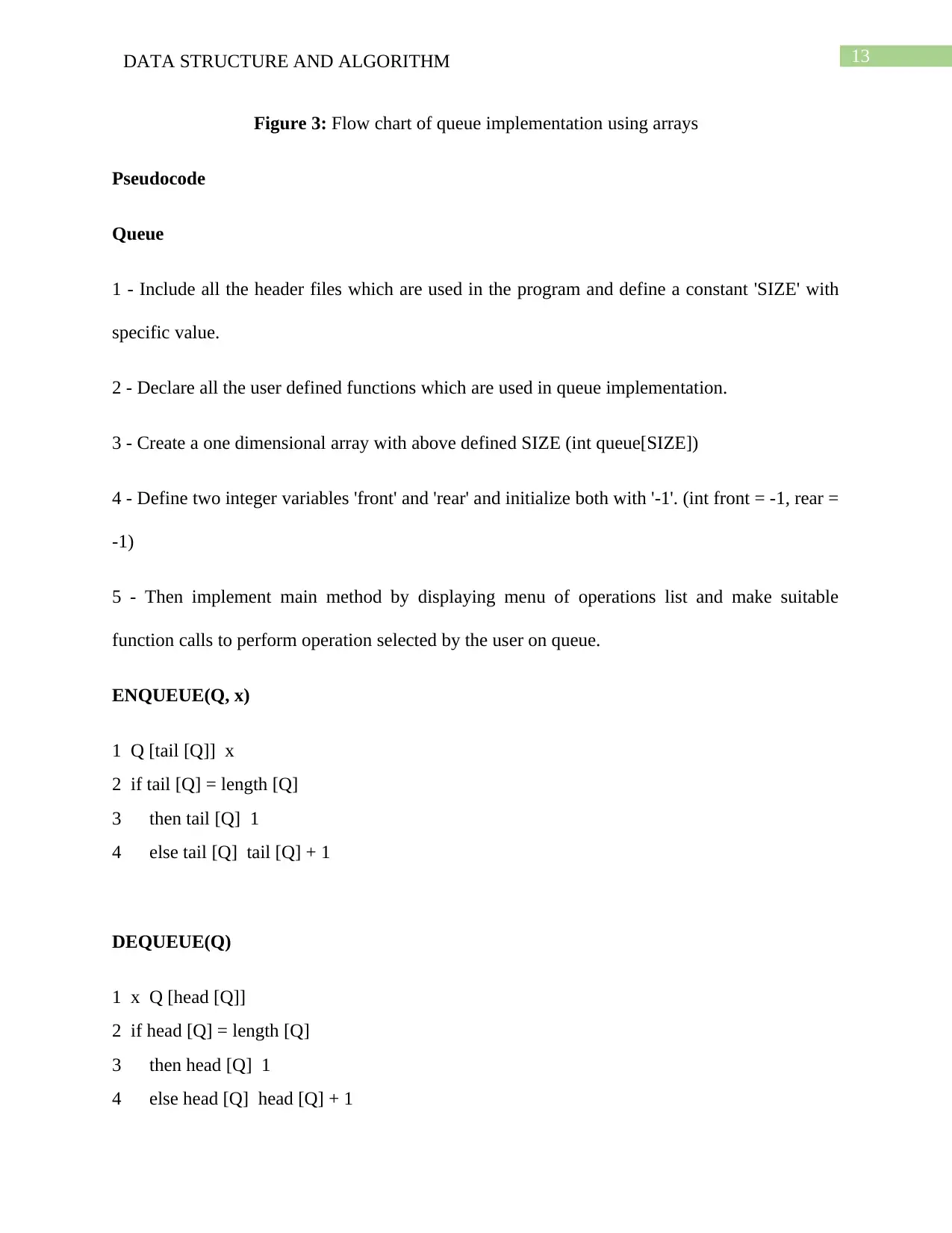
13DATA STRUCTURE AND ALGORITHM
Figure 3: Flow chart of queue implementation using arrays
Pseudocode
Queue
1 - Include all the header files which are used in the program and define a constant 'SIZE' with
specific value.
2 - Declare all the user defined functions which are used in queue implementation.
3 - Create a one dimensional array with above defined SIZE (int queue[SIZE])
4 - Define two integer variables 'front' and 'rear' and initialize both with '-1'. (int front = -1, rear =
-1)
5 - Then implement main method by displaying menu of operations list and make suitable
function calls to perform operation selected by the user on queue.
ENQUEUE(Q, x)
1 Q [tail [Q]] x
2 if tail [Q] = length [Q]
3 then tail [Q] 1
4 else tail [Q] tail [Q] + 1
DEQUEUE(Q)
1 x Q [head [Q]]
2 if head [Q] = length [Q]
3 then head [Q] 1
4 else head [Q] head [Q] + 1
Figure 3: Flow chart of queue implementation using arrays
Pseudocode
Queue
1 - Include all the header files which are used in the program and define a constant 'SIZE' with
specific value.
2 - Declare all the user defined functions which are used in queue implementation.
3 - Create a one dimensional array with above defined SIZE (int queue[SIZE])
4 - Define two integer variables 'front' and 'rear' and initialize both with '-1'. (int front = -1, rear =
-1)
5 - Then implement main method by displaying menu of operations list and make suitable
function calls to perform operation selected by the user on queue.
ENQUEUE(Q, x)
1 Q [tail [Q]] x
2 if tail [Q] = length [Q]
3 then tail [Q] 1
4 else tail [Q] tail [Q] + 1
DEQUEUE(Q)
1 x Q [head [Q]]
2 if head [Q] = length [Q]
3 then head [Q] 1
4 else head [Q] head [Q] + 1
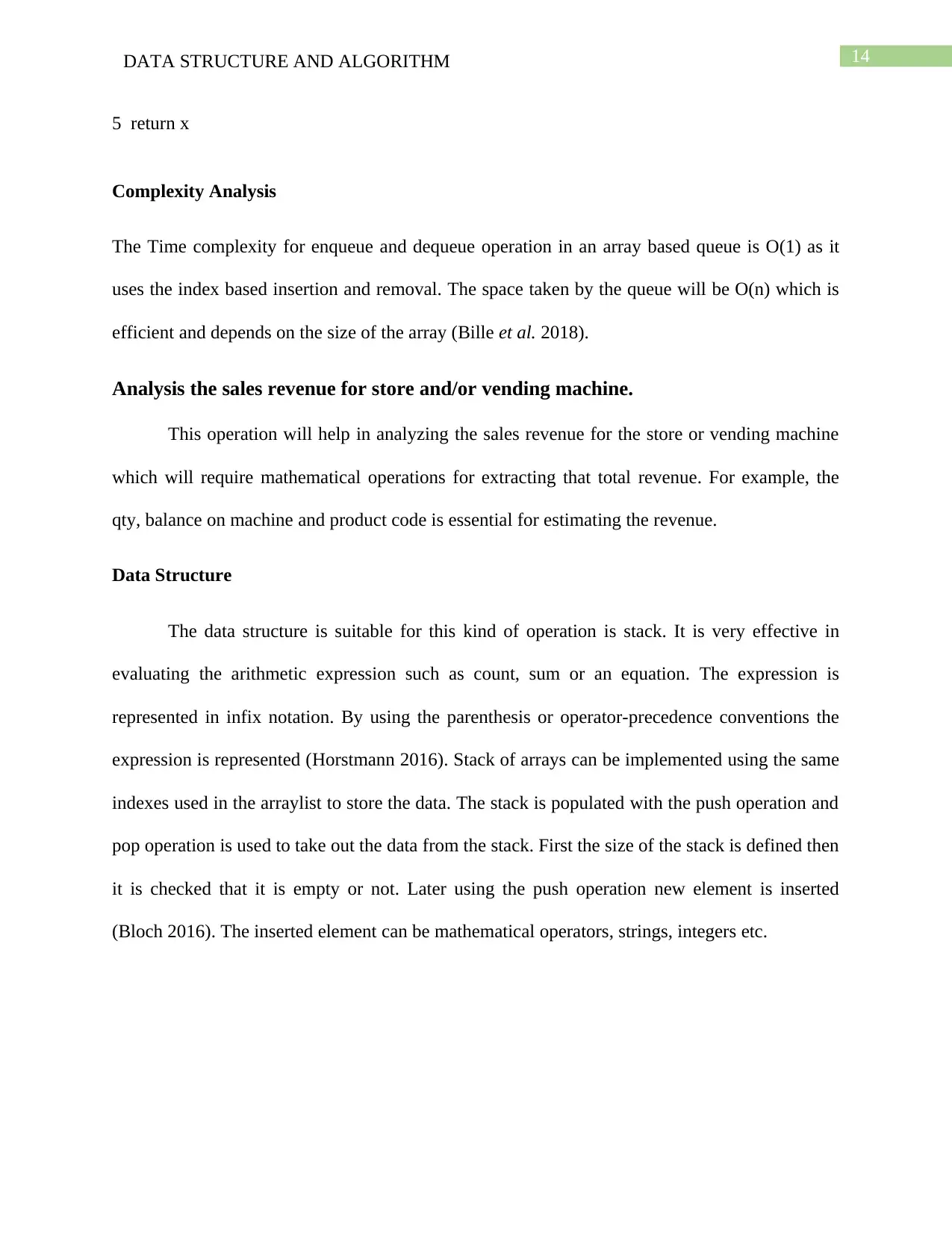
14DATA STRUCTURE AND ALGORITHM
5 return x
Complexity Analysis
The Time complexity for enqueue and dequeue operation in an array based queue is O(1) as it
uses the index based insertion and removal. The space taken by the queue will be O(n) which is
efficient and depends on the size of the array (Bille et al. 2018).
Analysis the sales revenue for store and/or vending machine.
This operation will help in analyzing the sales revenue for the store or vending machine
which will require mathematical operations for extracting that total revenue. For example, the
qty, balance on machine and product code is essential for estimating the revenue.
Data Structure
The data structure is suitable for this kind of operation is stack. It is very effective in
evaluating the arithmetic expression such as count, sum or an equation. The expression is
represented in infix notation. By using the parenthesis or operator-precedence conventions the
expression is represented (Horstmann 2016). Stack of arrays can be implemented using the same
indexes used in the arraylist to store the data. The stack is populated with the push operation and
pop operation is used to take out the data from the stack. First the size of the stack is defined then
it is checked that it is empty or not. Later using the push operation new element is inserted
(Bloch 2016). The inserted element can be mathematical operators, strings, integers etc.
5 return x
Complexity Analysis
The Time complexity for enqueue and dequeue operation in an array based queue is O(1) as it
uses the index based insertion and removal. The space taken by the queue will be O(n) which is
efficient and depends on the size of the array (Bille et al. 2018).
Analysis the sales revenue for store and/or vending machine.
This operation will help in analyzing the sales revenue for the store or vending machine
which will require mathematical operations for extracting that total revenue. For example, the
qty, balance on machine and product code is essential for estimating the revenue.
Data Structure
The data structure is suitable for this kind of operation is stack. It is very effective in
evaluating the arithmetic expression such as count, sum or an equation. The expression is
represented in infix notation. By using the parenthesis or operator-precedence conventions the
expression is represented (Horstmann 2016). Stack of arrays can be implemented using the same
indexes used in the arraylist to store the data. The stack is populated with the push operation and
pop operation is used to take out the data from the stack. First the size of the stack is defined then
it is checked that it is empty or not. Later using the push operation new element is inserted
(Bloch 2016). The inserted element can be mathematical operators, strings, integers etc.
You're viewing a preview
Unlock full access by subscribing today!
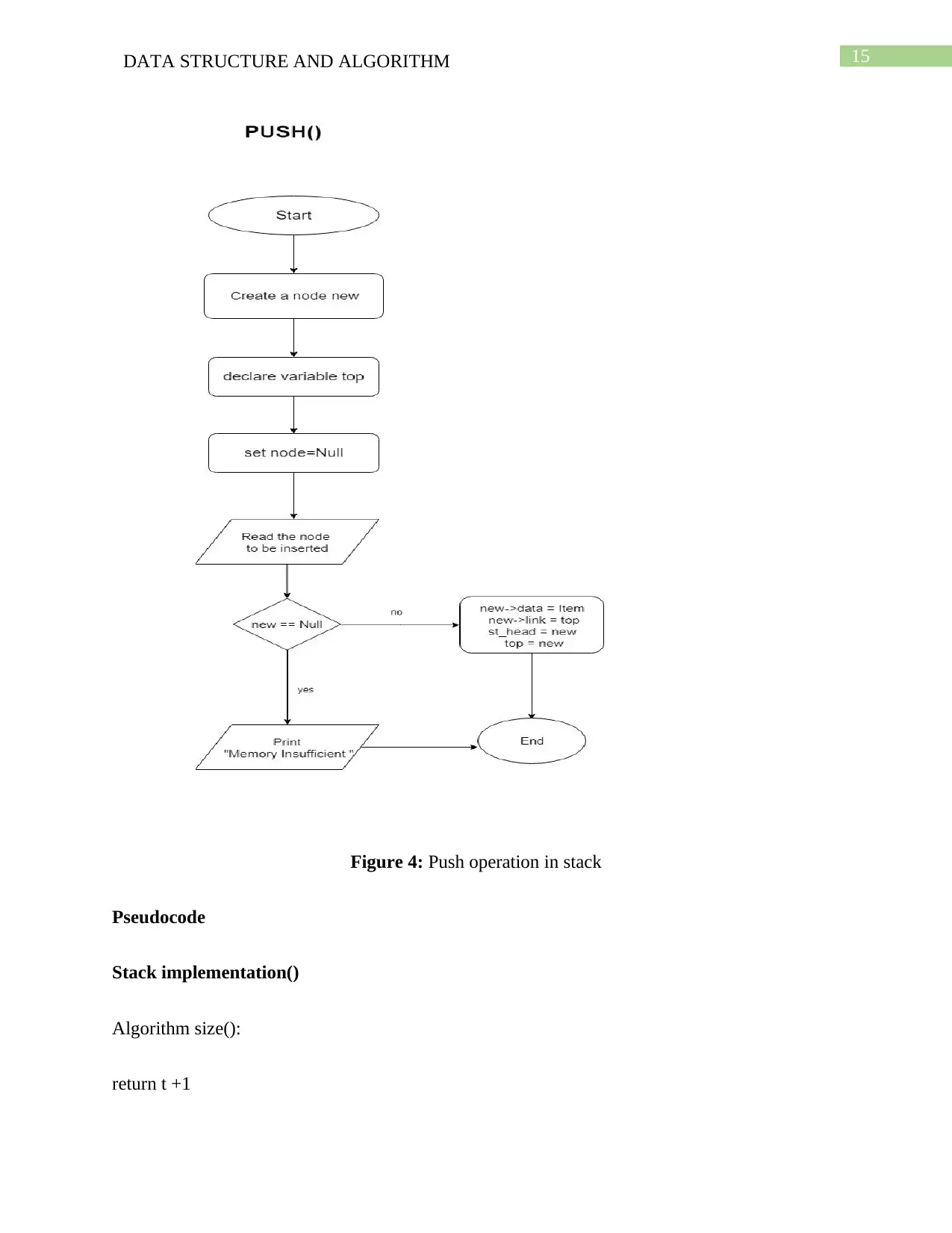
15DATA STRUCTURE AND ALGORITHM
Figure 4: Push operation in stack
Pseudocode
Stack implementation()
Algorithm size():
return t +1
Figure 4: Push operation in stack
Pseudocode
Stack implementation()
Algorithm size():
return t +1
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser

16DATA STRUCTURE AND ALGORITHM
Algorithm isEmpty():
return (t<0)
Algorithm top():
if isEmpty() then
throw a StackEmptyException
return S[t]
push operation ()
Algorithm push(o):
if size() = N then
throw a StackFullException
t ← t + 1
S[t] ← o
Complexity Analysis
For standard stack operations which are the push, pop, isEmpty, and size the time complexity is
O(1). However it is not always possible to implement stack with O(1) complexity. The size and
isEmpty are the constan operations along with the push and pop which only deal with the one
end of the stack. Hence the overall time complexity of the stack can be O(1). The indexing is
helpful in the time and space trade-off for entering new element in the stack and removing
elements (Ortega et al. 2018).
Algorithm isEmpty():
return (t<0)
Algorithm top():
if isEmpty() then
throw a StackEmptyException
return S[t]
push operation ()
Algorithm push(o):
if size() = N then
throw a StackFullException
t ← t + 1
S[t] ← o
Complexity Analysis
For standard stack operations which are the push, pop, isEmpty, and size the time complexity is
O(1). However it is not always possible to implement stack with O(1) complexity. The size and
isEmpty are the constan operations along with the push and pop which only deal with the one
end of the stack. Hence the overall time complexity of the stack can be O(1). The indexing is
helpful in the time and space trade-off for entering new element in the stack and removing
elements (Ortega et al. 2018).
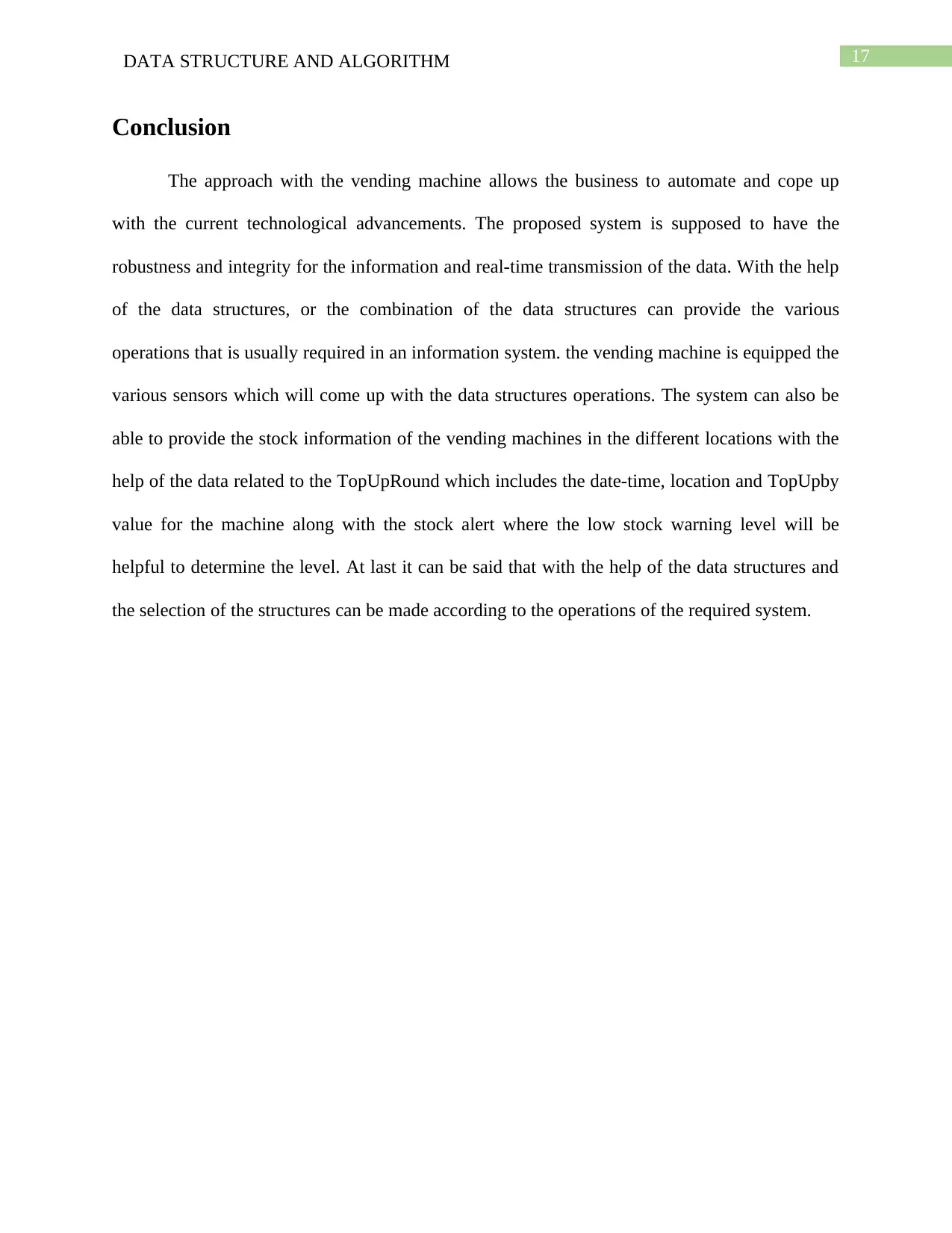
17DATA STRUCTURE AND ALGORITHM
Conclusion
The approach with the vending machine allows the business to automate and cope up
with the current technological advancements. The proposed system is supposed to have the
robustness and integrity for the information and real-time transmission of the data. With the help
of the data structures, or the combination of the data structures can provide the various
operations that is usually required in an information system. the vending machine is equipped the
various sensors which will come up with the data structures operations. The system can also be
able to provide the stock information of the vending machines in the different locations with the
help of the data related to the TopUpRound which includes the date-time, location and TopUpby
value for the machine along with the stock alert where the low stock warning level will be
helpful to determine the level. At last it can be said that with the help of the data structures and
the selection of the structures can be made according to the operations of the required system.
Conclusion
The approach with the vending machine allows the business to automate and cope up
with the current technological advancements. The proposed system is supposed to have the
robustness and integrity for the information and real-time transmission of the data. With the help
of the data structures, or the combination of the data structures can provide the various
operations that is usually required in an information system. the vending machine is equipped the
various sensors which will come up with the data structures operations. The system can also be
able to provide the stock information of the vending machines in the different locations with the
help of the data related to the TopUpRound which includes the date-time, location and TopUpby
value for the machine along with the stock alert where the low stock warning level will be
helpful to determine the level. At last it can be said that with the help of the data structures and
the selection of the structures can be made according to the operations of the required system.
You're viewing a preview
Unlock full access by subscribing today!
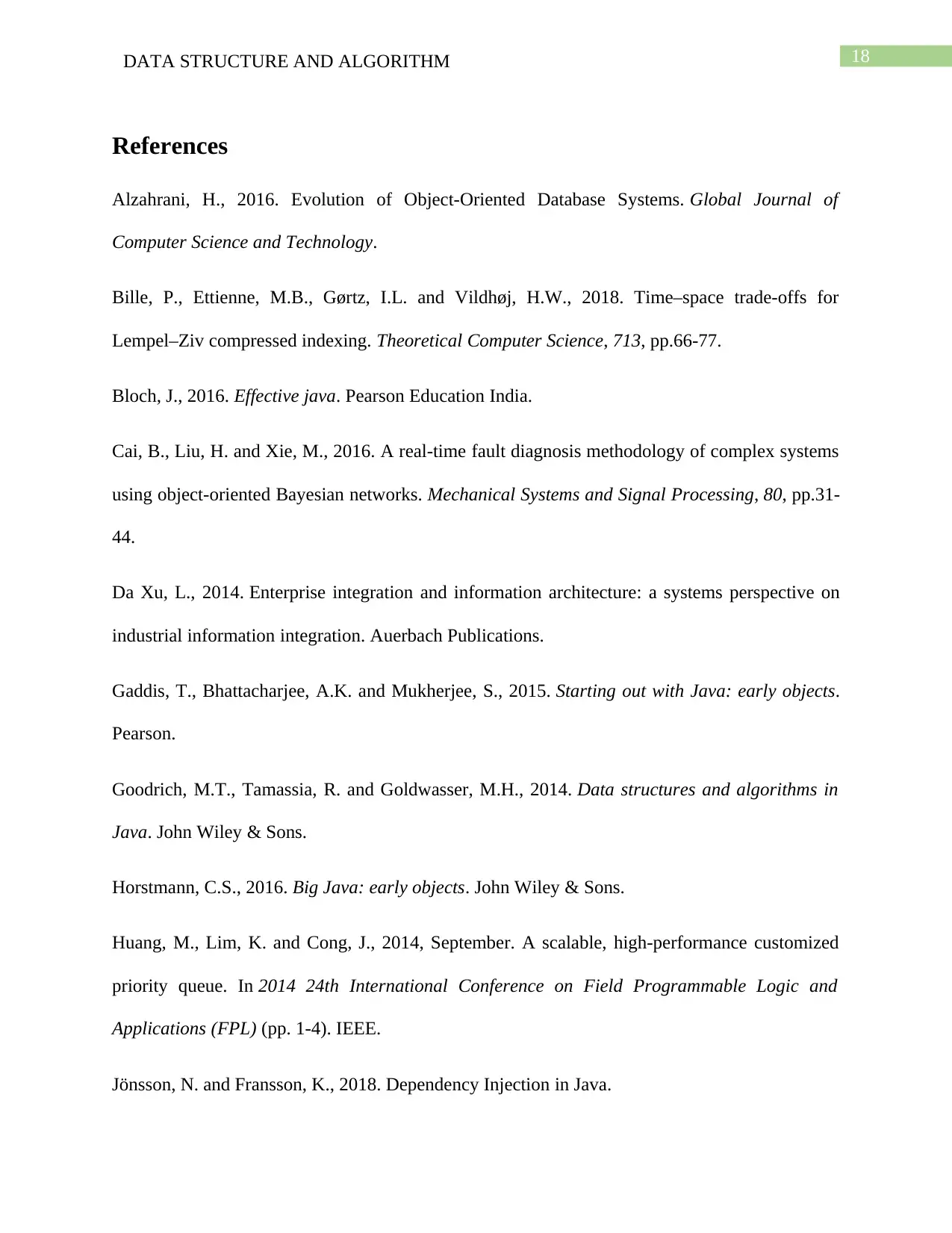
18DATA STRUCTURE AND ALGORITHM
References
Alzahrani, H., 2016. Evolution of Object-Oriented Database Systems. Global Journal of
Computer Science and Technology.
Bille, P., Ettienne, M.B., Gørtz, I.L. and Vildhøj, H.W., 2018. Time–space trade-offs for
Lempel–Ziv compressed indexing. Theoretical Computer Science, 713, pp.66-77.
Bloch, J., 2016. Effective java. Pearson Education India.
Cai, B., Liu, H. and Xie, M., 2016. A real-time fault diagnosis methodology of complex systems
using object-oriented Bayesian networks. Mechanical Systems and Signal Processing, 80, pp.31-
44.
Da Xu, L., 2014. Enterprise integration and information architecture: a systems perspective on
industrial information integration. Auerbach Publications.
Gaddis, T., Bhattacharjee, A.K. and Mukherjee, S., 2015. Starting out with Java: early objects.
Pearson.
Goodrich, M.T., Tamassia, R. and Goldwasser, M.H., 2014. Data structures and algorithms in
Java. John Wiley & Sons.
Horstmann, C.S., 2016. Big Java: early objects. John Wiley & Sons.
Huang, M., Lim, K. and Cong, J., 2014, September. A scalable, high-performance customized
priority queue. In 2014 24th International Conference on Field Programmable Logic and
Applications (FPL) (pp. 1-4). IEEE.
Jönsson, N. and Fransson, K., 2018. Dependency Injection in Java.
References
Alzahrani, H., 2016. Evolution of Object-Oriented Database Systems. Global Journal of
Computer Science and Technology.
Bille, P., Ettienne, M.B., Gørtz, I.L. and Vildhøj, H.W., 2018. Time–space trade-offs for
Lempel–Ziv compressed indexing. Theoretical Computer Science, 713, pp.66-77.
Bloch, J., 2016. Effective java. Pearson Education India.
Cai, B., Liu, H. and Xie, M., 2016. A real-time fault diagnosis methodology of complex systems
using object-oriented Bayesian networks. Mechanical Systems and Signal Processing, 80, pp.31-
44.
Da Xu, L., 2014. Enterprise integration and information architecture: a systems perspective on
industrial information integration. Auerbach Publications.
Gaddis, T., Bhattacharjee, A.K. and Mukherjee, S., 2015. Starting out with Java: early objects.
Pearson.
Goodrich, M.T., Tamassia, R. and Goldwasser, M.H., 2014. Data structures and algorithms in
Java. John Wiley & Sons.
Horstmann, C.S., 2016. Big Java: early objects. John Wiley & Sons.
Huang, M., Lim, K. and Cong, J., 2014, September. A scalable, high-performance customized
priority queue. In 2014 24th International Conference on Field Programmable Logic and
Applications (FPL) (pp. 1-4). IEEE.
Jönsson, N. and Fransson, K., 2018. Dependency Injection in Java.
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
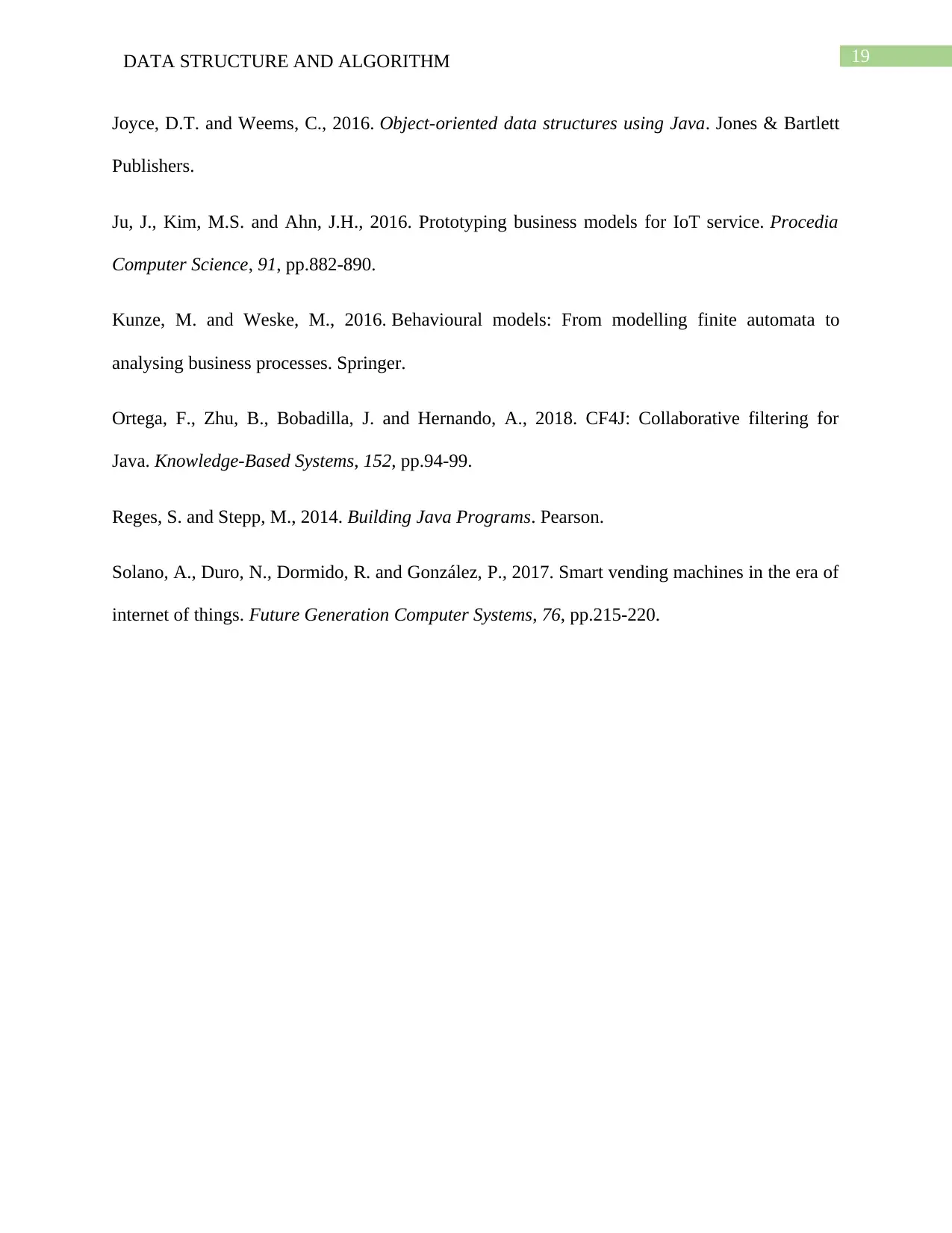
19DATA STRUCTURE AND ALGORITHM
Joyce, D.T. and Weems, C., 2016. Object-oriented data structures using Java. Jones & Bartlett
Publishers.
Ju, J., Kim, M.S. and Ahn, J.H., 2016. Prototyping business models for IoT service. Procedia
Computer Science, 91, pp.882-890.
Kunze, M. and Weske, M., 2016. Behavioural models: From modelling finite automata to
analysing business processes. Springer.
Ortega, F., Zhu, B., Bobadilla, J. and Hernando, A., 2018. CF4J: Collaborative filtering for
Java. Knowledge-Based Systems, 152, pp.94-99.
Reges, S. and Stepp, M., 2014. Building Java Programs. Pearson.
Solano, A., Duro, N., Dormido, R. and González, P., 2017. Smart vending machines in the era of
internet of things. Future Generation Computer Systems, 76, pp.215-220.
Joyce, D.T. and Weems, C., 2016. Object-oriented data structures using Java. Jones & Bartlett
Publishers.
Ju, J., Kim, M.S. and Ahn, J.H., 2016. Prototyping business models for IoT service. Procedia
Computer Science, 91, pp.882-890.
Kunze, M. and Weske, M., 2016. Behavioural models: From modelling finite automata to
analysing business processes. Springer.
Ortega, F., Zhu, B., Bobadilla, J. and Hernando, A., 2018. CF4J: Collaborative filtering for
Java. Knowledge-Based Systems, 152, pp.94-99.
Reges, S. and Stepp, M., 2014. Building Java Programs. Pearson.
Solano, A., Duro, N., Dormido, R. and González, P., 2017. Smart vending machines in the era of
internet of things. Future Generation Computer Systems, 76, pp.215-220.
1 out of 20
Related Documents

Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.