Object Oriented Programming: Advanced Scientific Calculator Design and Implementation
Added on 2024-04-25
57 Pages6282 Words499 Views
Object Oriented Programming
1
1
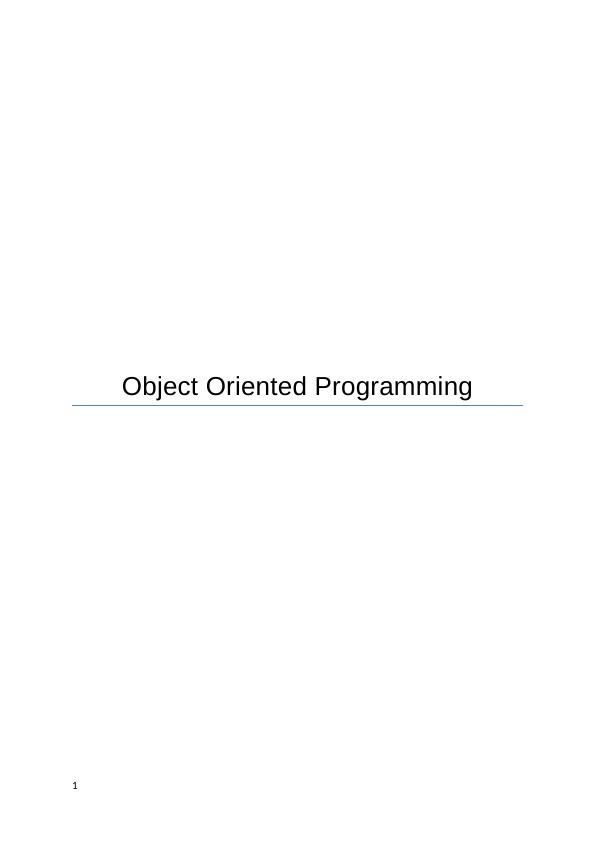
Table of Contents
Introduction..........................................................................................................................................6
LO1 Understand the object-oriented programming principle........................................................7
[P1.1, M1] Discuss the principle, characteristics and features of the object-oriented
programming language..................................................................................................................7
LO2 Be able to design the object-oriented programming solution.............................................12
[P 2.1] Identify the objects and data and file structures required to implement a given
design.............................................................................................................................................12
[P2.2, D1] Show these by drawing a UML class diagram(s) depicting the classes (with
their attributes and methods) and their relationships with each other in the design............18
LO3 Be able to implement object-oriented programming............................................................20
[P3.1, M2] Implement an objected oriented solution based on a prepared design..............20
[P3.2] Programming standards with effective code commenting...........................................32
[P3.3, D2] Implement object behaviors using control structures to meet the design
algorithms......................................................................................................................................36
[P3.4] Make effective use of an Integrated Development Environment (IDE), including
code and screen templates.........................................................................................................38
LO4 Be able to test and document object-oriented programming solutions.............................40
[P4.1, D3] critically reviews and test an object orientated programming solution you
developed for the given scenario................................................................................................40
[P4.2] Analyze actual test results against expected results to identify discrepancies.........46
[P4.3] Evaluate independent feedback on a developed objects oriented programmer
solution and make recommendations for improvements.........................................................48
[P 4.4] Create on-screen help to assist the users of a computer program............................50
[P4.5, M3] Create documentation for the support and maintenance of a computer program
.........................................................................................................................................................52
Conclusion.........................................................................................................................................55
References........................................................................................................................................56
2
Introduction..........................................................................................................................................6
LO1 Understand the object-oriented programming principle........................................................7
[P1.1, M1] Discuss the principle, characteristics and features of the object-oriented
programming language..................................................................................................................7
LO2 Be able to design the object-oriented programming solution.............................................12
[P 2.1] Identify the objects and data and file structures required to implement a given
design.............................................................................................................................................12
[P2.2, D1] Show these by drawing a UML class diagram(s) depicting the classes (with
their attributes and methods) and their relationships with each other in the design............18
LO3 Be able to implement object-oriented programming............................................................20
[P3.1, M2] Implement an objected oriented solution based on a prepared design..............20
[P3.2] Programming standards with effective code commenting...........................................32
[P3.3, D2] Implement object behaviors using control structures to meet the design
algorithms......................................................................................................................................36
[P3.4] Make effective use of an Integrated Development Environment (IDE), including
code and screen templates.........................................................................................................38
LO4 Be able to test and document object-oriented programming solutions.............................40
[P4.1, D3] critically reviews and test an object orientated programming solution you
developed for the given scenario................................................................................................40
[P4.2] Analyze actual test results against expected results to identify discrepancies.........46
[P4.3] Evaluate independent feedback on a developed objects oriented programmer
solution and make recommendations for improvements.........................................................48
[P 4.4] Create on-screen help to assist the users of a computer program............................50
[P4.5, M3] Create documentation for the support and maintenance of a computer program
.........................................................................................................................................................52
Conclusion.........................................................................................................................................55
References........................................................................................................................................56
2
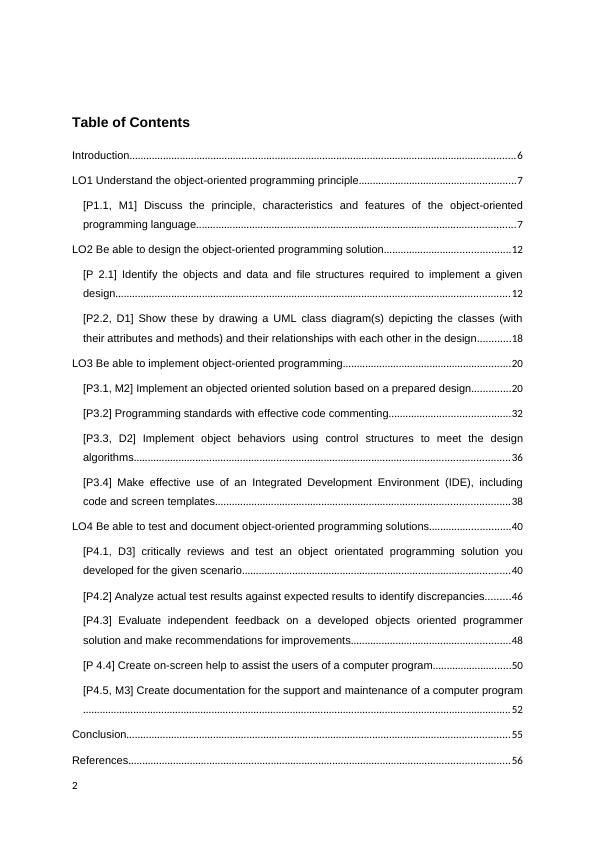
Appendix............................................................................................................................................58
List of Figure
Figure 1 Abstraction Example..................................................................................................8
Figure 2 Encapsulation example..............................................................................................8
Figure 3 Example of exception handling..................................................................................9
Figure 4 Inheritance...............................................................................................................10
Figure 5 Example of Modularity.............................................................................................11
Figure 6 Advanced scientific calculator modularity................................................................11
Figure 7 Objects.....................................................................................................................13
Figure 8 Registration object...................................................................................................14
Figure 9 Class........................................................................................................................14
Figure 10 File Structure..........................................................................................................15
Figure 11 File Structure..........................................................................................................15
Figure 12 Admin screen........................................................................................................16
Figure 13 Login Page.............................................................................................................16
Figure 14 Registration screen................................................................................................16
Figure 15 Calculator Screen..................................................................................................17
Figure 16 Calculator Screen..................................................................................................17
Figure 17 GUI Help page.......................................................................................................18
Figure 18 Methods in program...............................................................................................19
Figure 19 Use case Diagram.................................................................................................20
Figure 20 Login Code.............................................................................................................21
Figure 21 Login Code.............................................................................................................21
Figure 22 Registration screen code.......................................................................................22
Figure 23 Standard screen code 1.........................................................................................22
Figure 24 Standard screen code 2.........................................................................................23
Figure 25 Standard screen code 3.........................................................................................23
Figure 26 Standard screen code 4.........................................................................................24
Figure 27 Standard screen code 5.........................................................................................24
Figure 28 Advanced Screen code..........................................................................................25
Figure 29 Advanced Screen code..........................................................................................25
Figure 30 Advanced Screen code..........................................................................................26
Figure 31 Advanced Screen code..........................................................................................26
Figure 32 Advanced Screen code..........................................................................................27
3
List of Figure
Figure 1 Abstraction Example..................................................................................................8
Figure 2 Encapsulation example..............................................................................................8
Figure 3 Example of exception handling..................................................................................9
Figure 4 Inheritance...............................................................................................................10
Figure 5 Example of Modularity.............................................................................................11
Figure 6 Advanced scientific calculator modularity................................................................11
Figure 7 Objects.....................................................................................................................13
Figure 8 Registration object...................................................................................................14
Figure 9 Class........................................................................................................................14
Figure 10 File Structure..........................................................................................................15
Figure 11 File Structure..........................................................................................................15
Figure 12 Admin screen........................................................................................................16
Figure 13 Login Page.............................................................................................................16
Figure 14 Registration screen................................................................................................16
Figure 15 Calculator Screen..................................................................................................17
Figure 16 Calculator Screen..................................................................................................17
Figure 17 GUI Help page.......................................................................................................18
Figure 18 Methods in program...............................................................................................19
Figure 19 Use case Diagram.................................................................................................20
Figure 20 Login Code.............................................................................................................21
Figure 21 Login Code.............................................................................................................21
Figure 22 Registration screen code.......................................................................................22
Figure 23 Standard screen code 1.........................................................................................22
Figure 24 Standard screen code 2.........................................................................................23
Figure 25 Standard screen code 3.........................................................................................23
Figure 26 Standard screen code 4.........................................................................................24
Figure 27 Standard screen code 5.........................................................................................24
Figure 28 Advanced Screen code..........................................................................................25
Figure 29 Advanced Screen code..........................................................................................25
Figure 30 Advanced Screen code..........................................................................................26
Figure 31 Advanced Screen code..........................................................................................26
Figure 32 Advanced Screen code..........................................................................................27
3
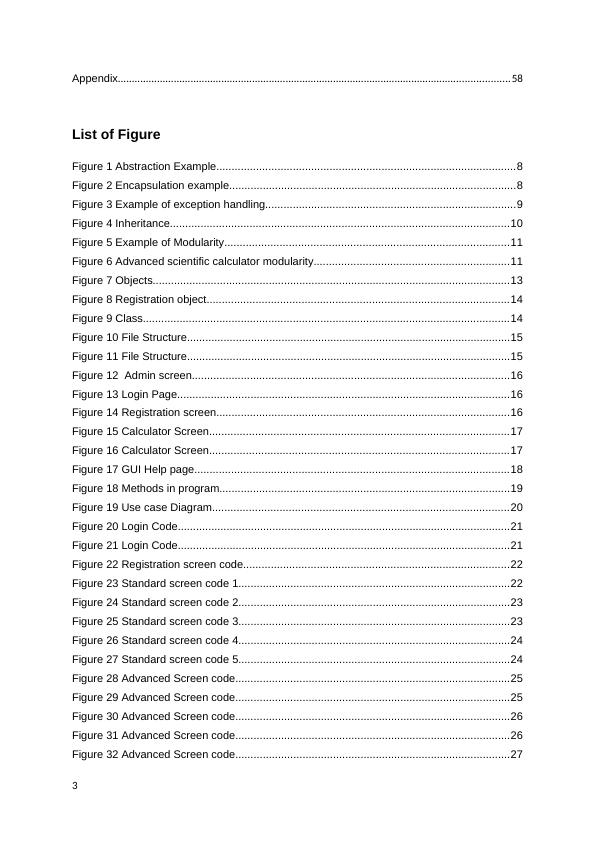
Figure 33 Code for calculator as scientific screen.................................................................27
Figure 34 Code for calculator as scientific screen.................................................................28
Figure 35 Code for calculator as scientific screen.................................................................28
Figure 36 Code for calculator as scientific screen.................................................................29
Figure 37 Code for calculator as scientific screen.................................................................29
Figure 38 Code for calculator as scientific screen.................................................................30
Figure 39 Code of programmer screen..................................................................................30
Figure 40 Code of programmer screen..................................................................................31
Figure 41 Code of programmer screen..................................................................................31
Figure 42 Code of programmer screen..................................................................................32
Figure 43 Code of programmer screen..................................................................................32
Figure 44 Commenting Convention.......................................................................................33
Figure 45 Multiline Handling...................................................................................................34
Figure 46 Single line comments............................................................................................35
Figure 47 Event Handling.......................................................................................................35
Figure 48 data type................................................................................................................36
Figure 49 IF else statements..................................................................................................37
Figure 50 if else......................................................................................................................38
Figure 51 For Loop.................................................................................................................38
Figure 52 Admin Page...........................................................................................................39
Figure 53 Registration Screen...............................................................................................39
Figure 54 Login page.............................................................................................................40
Figure 55 Calculator screen...................................................................................................40
Figure 56 Admin Page...........................................................................................................43
Figure 57 Login Page.............................................................................................................43
Figure 58 Registration page...................................................................................................43
Figure 59 Calculator Screen..................................................................................................44
Figure 60 Admin Screen........................................................................................................44
Figure 61 Login Screen..........................................................................................................44
Figure 62 Calculator Screen..................................................................................................45
Figure 63 Scientific Calculator Screen...................................................................................45
Figure 64 Standard Calculator Screen...................................................................................45
Figure 65 Advanced Calculator Screen.................................................................................46
Figure 66 Test Case 1 and 2..................................................................................................47
Figure 67 Test case 3............................................................................................................48
Figure 68 Test Case 5............................................................................................................48
Figure 69 Test case 6 and 7.................................................................................................48
4
Figure 34 Code for calculator as scientific screen.................................................................28
Figure 35 Code for calculator as scientific screen.................................................................28
Figure 36 Code for calculator as scientific screen.................................................................29
Figure 37 Code for calculator as scientific screen.................................................................29
Figure 38 Code for calculator as scientific screen.................................................................30
Figure 39 Code of programmer screen..................................................................................30
Figure 40 Code of programmer screen..................................................................................31
Figure 41 Code of programmer screen..................................................................................31
Figure 42 Code of programmer screen..................................................................................32
Figure 43 Code of programmer screen..................................................................................32
Figure 44 Commenting Convention.......................................................................................33
Figure 45 Multiline Handling...................................................................................................34
Figure 46 Single line comments............................................................................................35
Figure 47 Event Handling.......................................................................................................35
Figure 48 data type................................................................................................................36
Figure 49 IF else statements..................................................................................................37
Figure 50 if else......................................................................................................................38
Figure 51 For Loop.................................................................................................................38
Figure 52 Admin Page...........................................................................................................39
Figure 53 Registration Screen...............................................................................................39
Figure 54 Login page.............................................................................................................40
Figure 55 Calculator screen...................................................................................................40
Figure 56 Admin Page...........................................................................................................43
Figure 57 Login Page.............................................................................................................43
Figure 58 Registration page...................................................................................................43
Figure 59 Calculator Screen..................................................................................................44
Figure 60 Admin Screen........................................................................................................44
Figure 61 Login Screen..........................................................................................................44
Figure 62 Calculator Screen..................................................................................................45
Figure 63 Scientific Calculator Screen...................................................................................45
Figure 64 Standard Calculator Screen...................................................................................45
Figure 65 Advanced Calculator Screen.................................................................................46
Figure 66 Test Case 1 and 2..................................................................................................47
Figure 67 Test case 3............................................................................................................48
Figure 68 Test Case 5............................................................................................................48
Figure 69 Test case 6 and 7.................................................................................................48
4
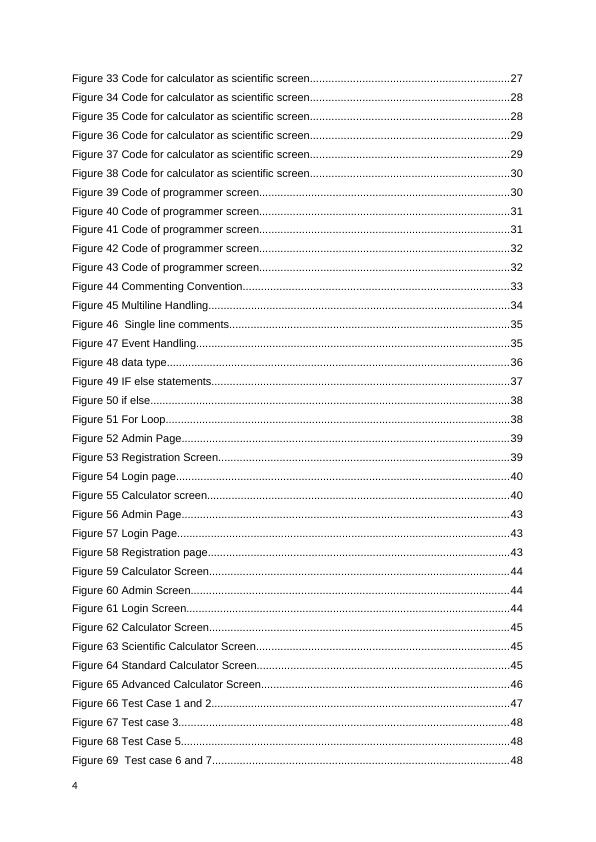
Figure 70 Online Help screen................................................................................................51
Figure 71 Login Help Screen.................................................................................................52
Figure 72 Registration Screen...............................................................................................53
Figure 73 Login Screen..........................................................................................................53
Figure 74 Admin Screen........................................................................................................54
Figure 75 Calculator Screen..................................................................................................54
Figure 76 Calculator screen with programmer module..........................................................54
Figure 77 Scientific calculator screen....................................................................................55
5
Figure 71 Login Help Screen.................................................................................................52
Figure 72 Registration Screen...............................................................................................53
Figure 73 Login Screen..........................................................................................................53
Figure 74 Admin Screen........................................................................................................54
Figure 75 Calculator Screen..................................................................................................54
Figure 76 Calculator screen with programmer module..........................................................54
Figure 77 Scientific calculator screen....................................................................................55
5
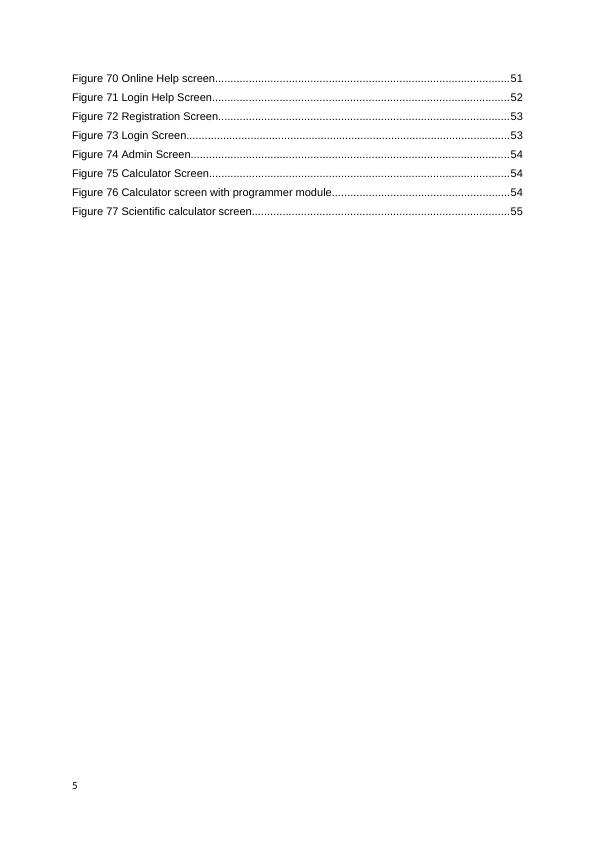
Introduction
This report presents the advanced scientific calculator that solves the various operations of
mathematics such as standard problem, advance problems, scientific problems and
programmer problems. The calculator is developed by using the Microsoft visual studio IDLE
and to understand the program, I will design the UML class diagram and use diagram. This
report is divided into four sections where the first part is discussing the features, principle,
and characteristics of the object-oriented programming language. The second part of this
report discusses the object-oriented programming advantages and used program class, the
object as methods and other principles like inheritance and error handling etc. The third part
of this report presents the implementation of the advanced scientific calculator and in four-
part, I will prepare the test scenario to test the application according to given user and
system requirements.
6
This report presents the advanced scientific calculator that solves the various operations of
mathematics such as standard problem, advance problems, scientific problems and
programmer problems. The calculator is developed by using the Microsoft visual studio IDLE
and to understand the program, I will design the UML class diagram and use diagram. This
report is divided into four sections where the first part is discussing the features, principle,
and characteristics of the object-oriented programming language. The second part of this
report discusses the object-oriented programming advantages and used program class, the
object as methods and other principles like inheritance and error handling etc. The third part
of this report presents the implementation of the advanced scientific calculator and in four-
part, I will prepare the test scenario to test the application according to given user and
system requirements.
6
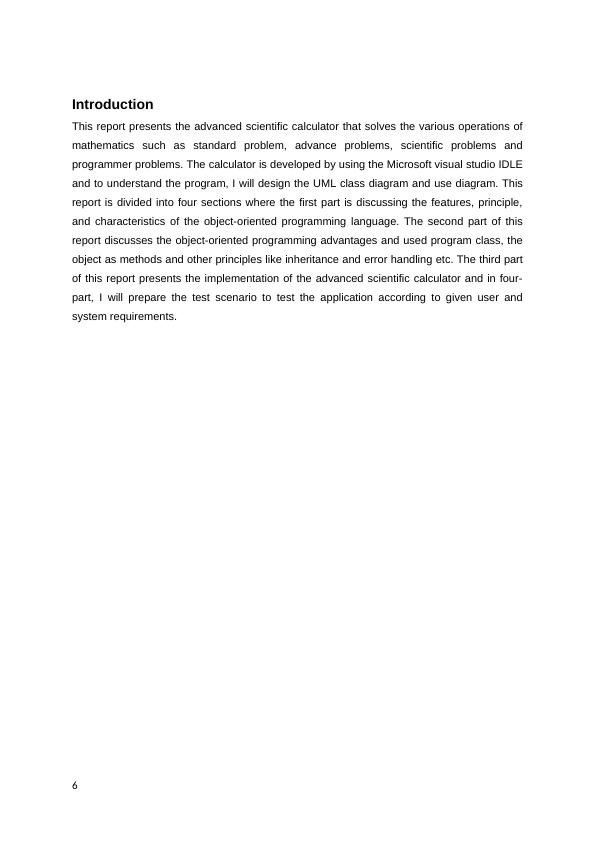
LO1 Understand the object-oriented programming principle
[P1.1, M1] Discuss the principle, characteristics and features of the
object-oriented programming language
Features of the object-oriented programming language
The object-oriented programming language has followed the concept of the objects where
objects are referred to the procedures and attributes. The Opps mainly focus on the data
and objects for taking or implementing action by the application. Object-Oriented
Programming is a software design that is related with the conception, of course, thing and
different other concepts turning around this binary similar, similar to inheritance,
polymorphism, abstraction, encapsulation etc. now, we discuss the various concept of the
object-oriented programming:
Currently, let us converse selected of the foremost features of OOPS which we will be using
in C++ (exactly): Objects, Classes, Abstraction, Encapsulation, Inheritance, Overloading and
Exception handling
1. Objects: - Objects are the simple unit of OOP. They are examples of discussion,
which have facts associates and uses of much associate function to complete
responsibilities.
Here, I have presents the objects that are used in a program that is developed for
calculator application:
2. Class: It is comparable to the arrangement in C verbal. The class can likewise be
sharp as operator sharp facts category but it similarly contains occupations in this
one. The class contains the three main components of the program such as name,
attributes, and methods (Exforsys, 2006).
Here, I have presents the classes that are used in a program that is developed for
calculator application:
Principle and characteristics of the Object-oriented programming language
There are various principles of the object-oriented language which is followed by the
programming to develop an application. Let discuss the principle of the object-oriented
programming language:
7
[P1.1, M1] Discuss the principle, characteristics and features of the
object-oriented programming language
Features of the object-oriented programming language
The object-oriented programming language has followed the concept of the objects where
objects are referred to the procedures and attributes. The Opps mainly focus on the data
and objects for taking or implementing action by the application. Object-Oriented
Programming is a software design that is related with the conception, of course, thing and
different other concepts turning around this binary similar, similar to inheritance,
polymorphism, abstraction, encapsulation etc. now, we discuss the various concept of the
object-oriented programming:
Currently, let us converse selected of the foremost features of OOPS which we will be using
in C++ (exactly): Objects, Classes, Abstraction, Encapsulation, Inheritance, Overloading and
Exception handling
1. Objects: - Objects are the simple unit of OOP. They are examples of discussion,
which have facts associates and uses of much associate function to complete
responsibilities.
Here, I have presents the objects that are used in a program that is developed for
calculator application:
2. Class: It is comparable to the arrangement in C verbal. The class can likewise be
sharp as operator sharp facts category but it similarly contains occupations in this
one. The class contains the three main components of the program such as name,
attributes, and methods (Exforsys, 2006).
Here, I have presents the classes that are used in a program that is developed for
calculator application:
Principle and characteristics of the Object-oriented programming language
There are various principles of the object-oriented language which is followed by the
programming to develop an application. Let discuss the principle of the object-oriented
programming language:
7
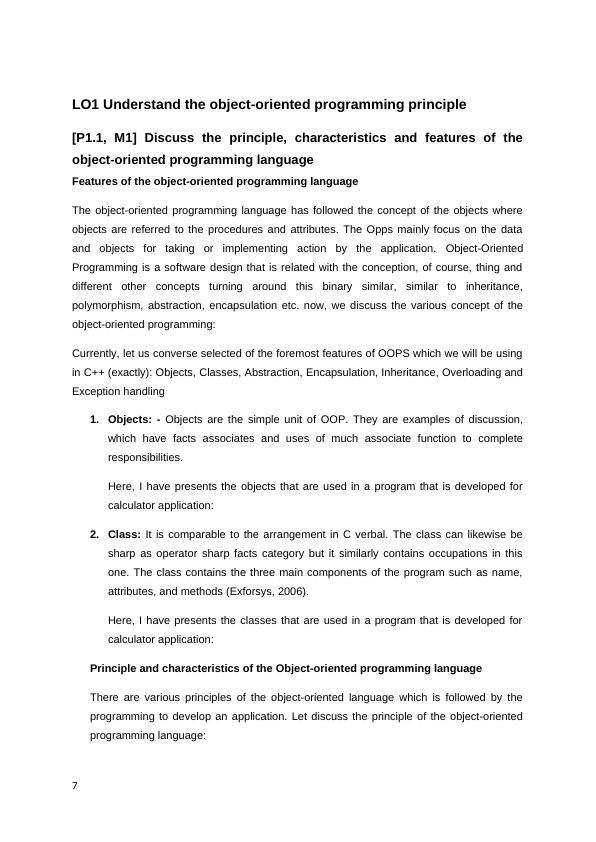
1. Abstraction: Abstraction mentions to viewing simply the important features of the
submission and hiding the particulars. The abstraction is the process that hides the
useful and important data from the users. With the help of abstraction process, the
program complexity will be reduced.
Figure 1 Abstraction Example
2. Encapsulation: Encapsulation is all almost compulsory the facts variables and
occupations together in discussion. It is a combined methods and data in a single
unit and the encapsulation example is class which is used to create the program.
Figure 2 Encapsulation example
8
submission and hiding the particulars. The abstraction is the process that hides the
useful and important data from the users. With the help of abstraction process, the
program complexity will be reduced.
Figure 1 Abstraction Example
2. Encapsulation: Encapsulation is all almost compulsory the facts variables and
occupations together in discussion. It is a combined methods and data in a single
unit and the encapsulation example is class which is used to create the program.
Figure 2 Encapsulation example
8
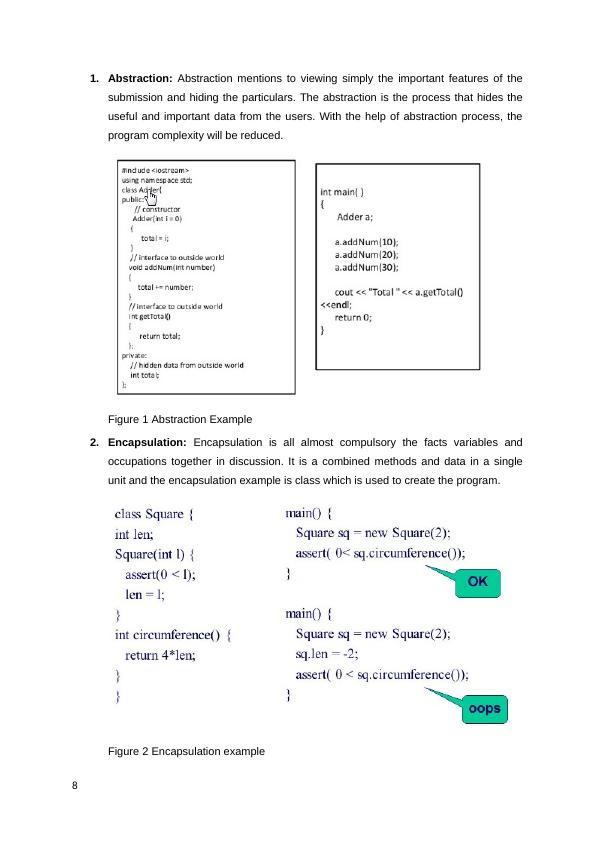
End of preview
Want to access all the pages? Upload your documents or become a member.
Related Documents
Object-Oriented Programming Implementation and Testinglg...
|51
|4099
|332
Order and Components - Thesis and Dissertation Guidelg...
|8
|967
|75
Design and Development of Quadcopter for Advanced Manufacturing-IIlg...
|42
|11891
|479
Unit 1: Programming Submission PDFlg...
|36
|7565
|62
The assignment submission is entirely my own work and I understand the consequences of plagiarismlg...
|75
|6901
|487
Database Design & Development Assignment 2lg...
|75
|6962
|200