Object Oriented Software Development
VerifiedAdded on 2023/03/17
|10
|2054
|50
AI Summary
This document discusses the concept of object oriented software development and explores the creational design patterns such as abstract factory, builder, factory method, prototype, and singleton. It also presents the strategy method and iterator pattern for solving design problems in a shopping application.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
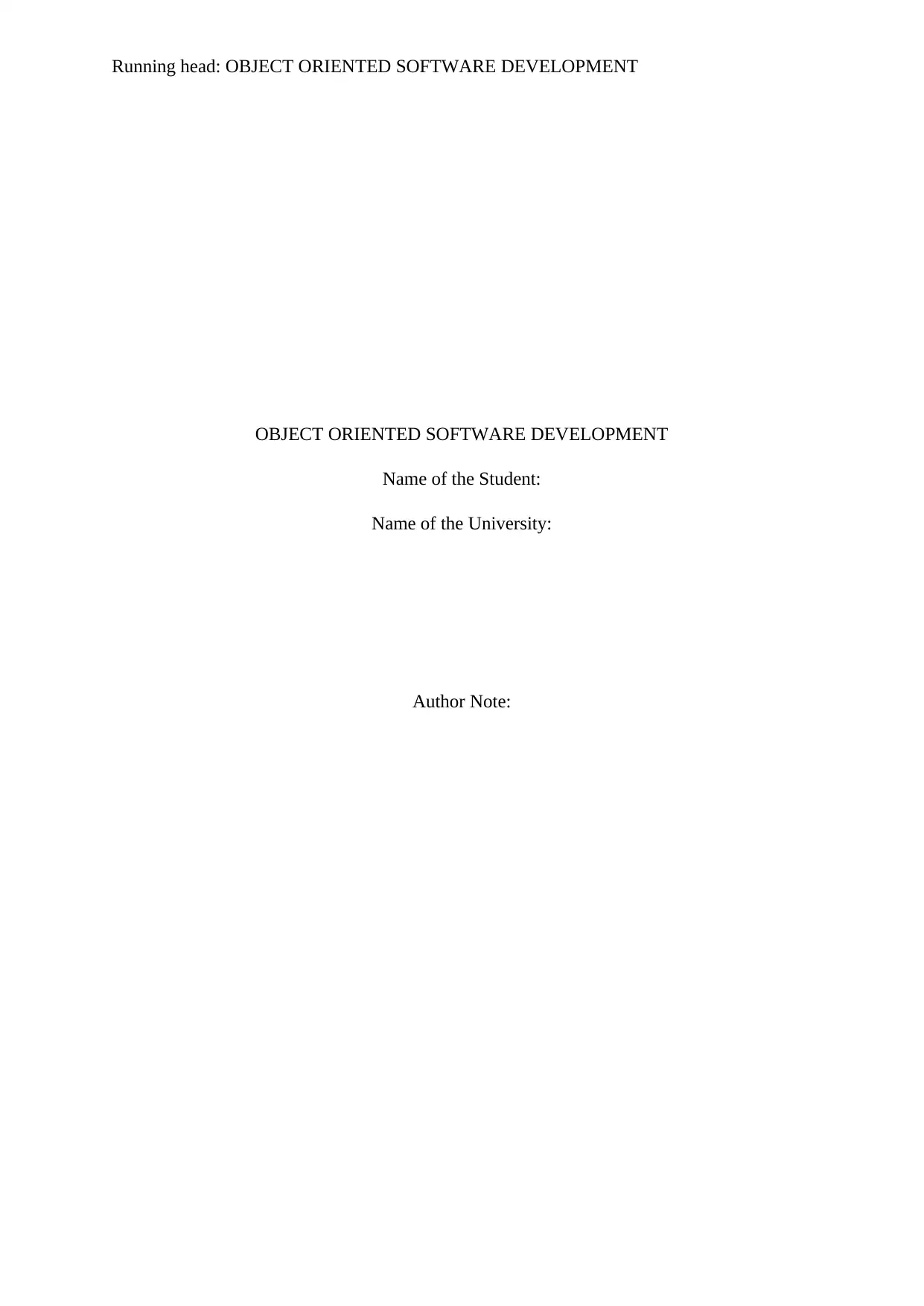
Running head: OBJECT ORIENTED SOFTWARE DEVELOPMENT
OBJECT ORIENTED SOFTWARE DEVELOPMENT
Name of the Student:
Name of the University:
Author Note:
OBJECT ORIENTED SOFTWARE DEVELOPMENT
Name of the Student:
Name of the University:
Author Note:
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
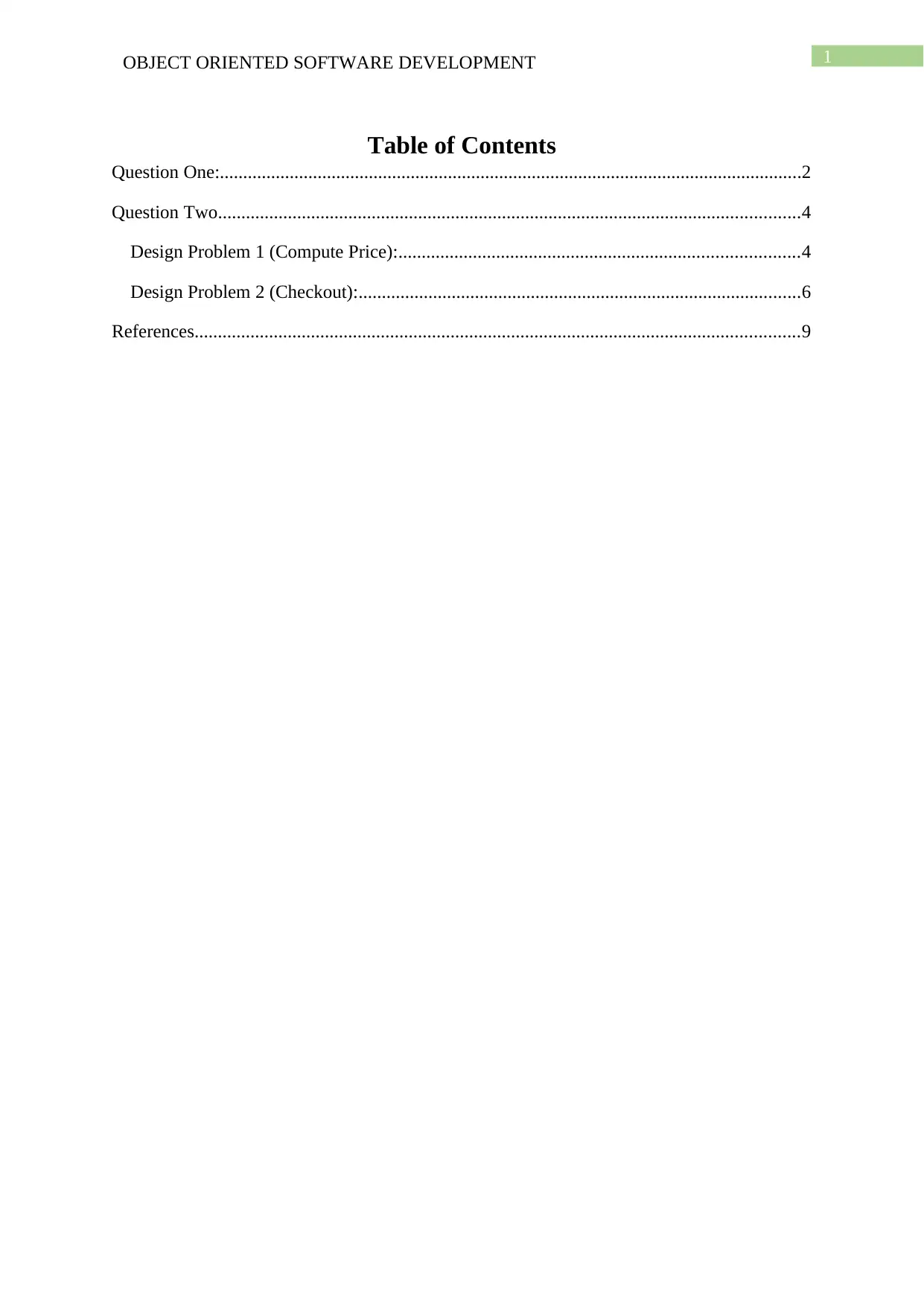
1OBJECT ORIENTED SOFTWARE DEVELOPMENT
Table of Contents
Question One:.............................................................................................................................2
Question Two.............................................................................................................................4
Design Problem 1 (Compute Price):......................................................................................4
Design Problem 2 (Checkout):...............................................................................................6
References..................................................................................................................................9
Table of Contents
Question One:.............................................................................................................................2
Question Two.............................................................................................................................4
Design Problem 1 (Compute Price):......................................................................................4
Design Problem 2 (Checkout):...............................................................................................6
References..................................................................................................................................9
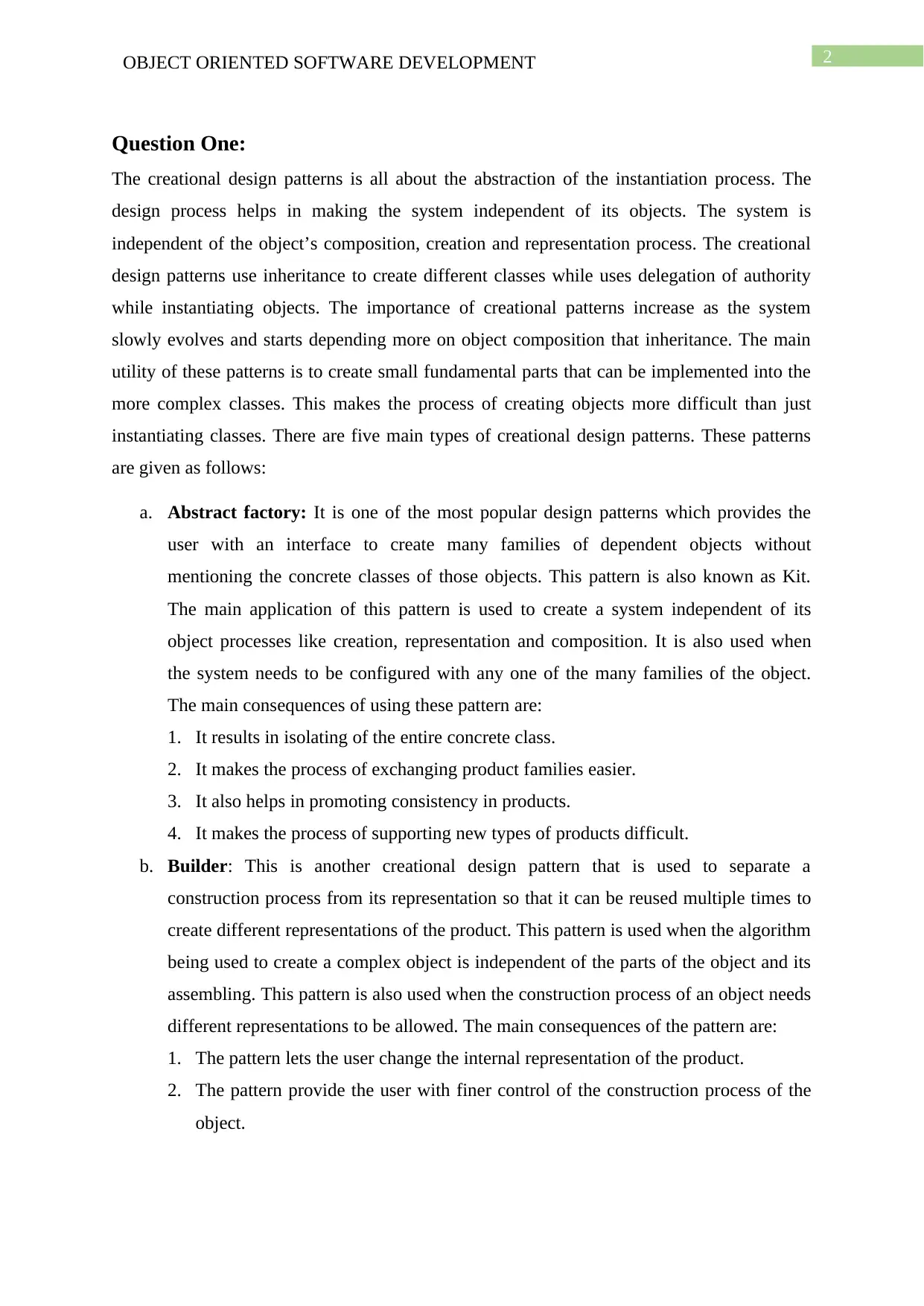
2OBJECT ORIENTED SOFTWARE DEVELOPMENT
Question One:
The creational design patterns is all about the abstraction of the instantiation process. The
design process helps in making the system independent of its objects. The system is
independent of the object’s composition, creation and representation process. The creational
design patterns use inheritance to create different classes while uses delegation of authority
while instantiating objects. The importance of creational patterns increase as the system
slowly evolves and starts depending more on object composition that inheritance. The main
utility of these patterns is to create small fundamental parts that can be implemented into the
more complex classes. This makes the process of creating objects more difficult than just
instantiating classes. There are five main types of creational design patterns. These patterns
are given as follows:
a. Abstract factory: It is one of the most popular design patterns which provides the
user with an interface to create many families of dependent objects without
mentioning the concrete classes of those objects. This pattern is also known as Kit.
The main application of this pattern is used to create a system independent of its
object processes like creation, representation and composition. It is also used when
the system needs to be configured with any one of the many families of the object.
The main consequences of using these pattern are:
1. It results in isolating of the entire concrete class.
2. It makes the process of exchanging product families easier.
3. It also helps in promoting consistency in products.
4. It makes the process of supporting new types of products difficult.
b. Builder: This is another creational design pattern that is used to separate a
construction process from its representation so that it can be reused multiple times to
create different representations of the product. This pattern is used when the algorithm
being used to create a complex object is independent of the parts of the object and its
assembling. This pattern is also used when the construction process of an object needs
different representations to be allowed. The main consequences of the pattern are:
1. The pattern lets the user change the internal representation of the product.
2. The pattern provide the user with finer control of the construction process of the
object.
Question One:
The creational design patterns is all about the abstraction of the instantiation process. The
design process helps in making the system independent of its objects. The system is
independent of the object’s composition, creation and representation process. The creational
design patterns use inheritance to create different classes while uses delegation of authority
while instantiating objects. The importance of creational patterns increase as the system
slowly evolves and starts depending more on object composition that inheritance. The main
utility of these patterns is to create small fundamental parts that can be implemented into the
more complex classes. This makes the process of creating objects more difficult than just
instantiating classes. There are five main types of creational design patterns. These patterns
are given as follows:
a. Abstract factory: It is one of the most popular design patterns which provides the
user with an interface to create many families of dependent objects without
mentioning the concrete classes of those objects. This pattern is also known as Kit.
The main application of this pattern is used to create a system independent of its
object processes like creation, representation and composition. It is also used when
the system needs to be configured with any one of the many families of the object.
The main consequences of using these pattern are:
1. It results in isolating of the entire concrete class.
2. It makes the process of exchanging product families easier.
3. It also helps in promoting consistency in products.
4. It makes the process of supporting new types of products difficult.
b. Builder: This is another creational design pattern that is used to separate a
construction process from its representation so that it can be reused multiple times to
create different representations of the product. This pattern is used when the algorithm
being used to create a complex object is independent of the parts of the object and its
assembling. This pattern is also used when the construction process of an object needs
different representations to be allowed. The main consequences of the pattern are:
1. The pattern lets the user change the internal representation of the product.
2. The pattern provide the user with finer control of the construction process of the
object.
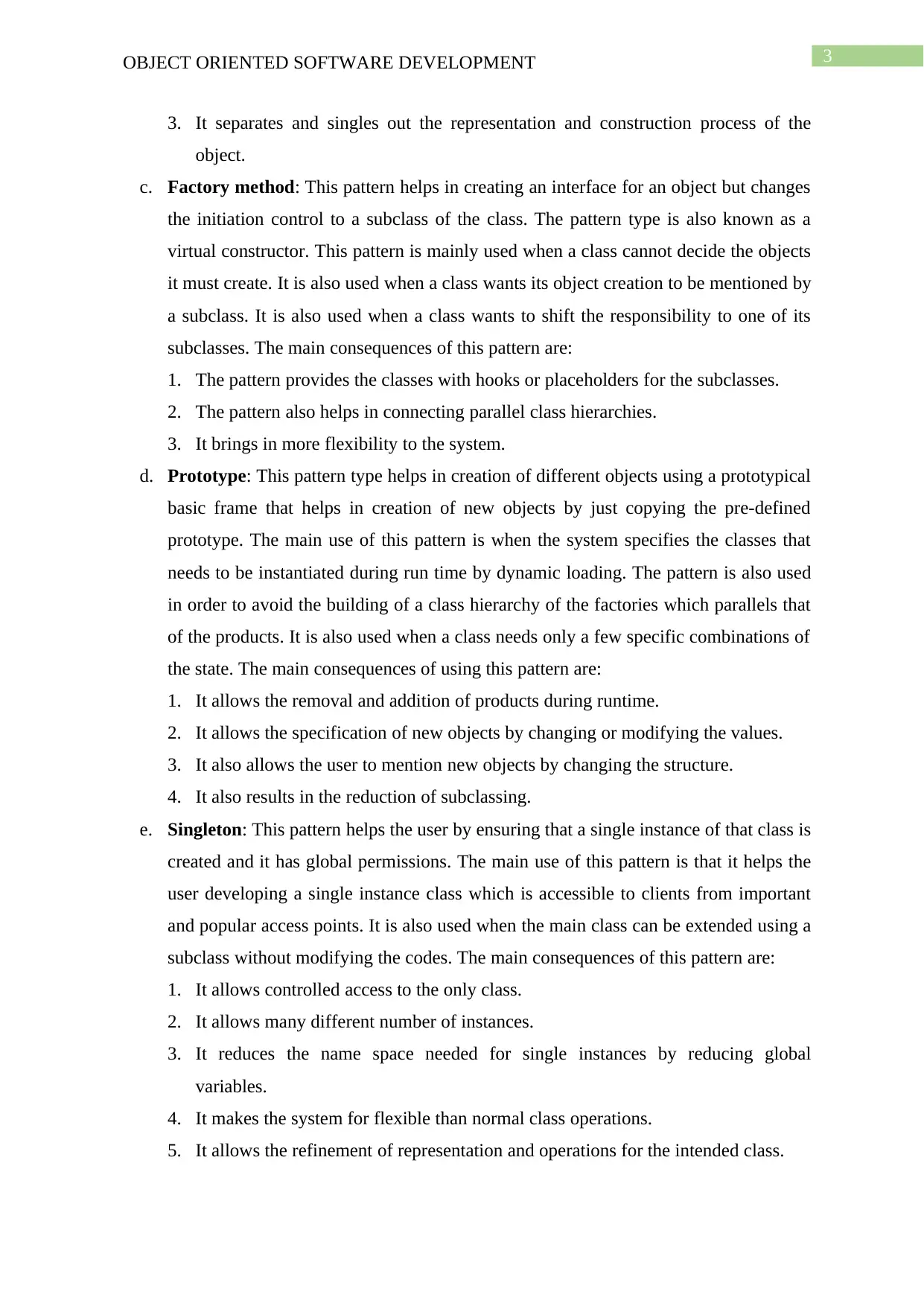
3OBJECT ORIENTED SOFTWARE DEVELOPMENT
3. It separates and singles out the representation and construction process of the
object.
c. Factory method: This pattern helps in creating an interface for an object but changes
the initiation control to a subclass of the class. The pattern type is also known as a
virtual constructor. This pattern is mainly used when a class cannot decide the objects
it must create. It is also used when a class wants its object creation to be mentioned by
a subclass. It is also used when a class wants to shift the responsibility to one of its
subclasses. The main consequences of this pattern are:
1. The pattern provides the classes with hooks or placeholders for the subclasses.
2. The pattern also helps in connecting parallel class hierarchies.
3. It brings in more flexibility to the system.
d. Prototype: This pattern type helps in creation of different objects using a prototypical
basic frame that helps in creation of new objects by just copying the pre-defined
prototype. The main use of this pattern is when the system specifies the classes that
needs to be instantiated during run time by dynamic loading. The pattern is also used
in order to avoid the building of a class hierarchy of the factories which parallels that
of the products. It is also used when a class needs only a few specific combinations of
the state. The main consequences of using this pattern are:
1. It allows the removal and addition of products during runtime.
2. It allows the specification of new objects by changing or modifying the values.
3. It also allows the user to mention new objects by changing the structure.
4. It also results in the reduction of subclassing.
e. Singleton: This pattern helps the user by ensuring that a single instance of that class is
created and it has global permissions. The main use of this pattern is that it helps the
user developing a single instance class which is accessible to clients from important
and popular access points. It is also used when the main class can be extended using a
subclass without modifying the codes. The main consequences of this pattern are:
1. It allows controlled access to the only class.
2. It allows many different number of instances.
3. It reduces the name space needed for single instances by reducing global
variables.
4. It makes the system for flexible than normal class operations.
5. It allows the refinement of representation and operations for the intended class.
3. It separates and singles out the representation and construction process of the
object.
c. Factory method: This pattern helps in creating an interface for an object but changes
the initiation control to a subclass of the class. The pattern type is also known as a
virtual constructor. This pattern is mainly used when a class cannot decide the objects
it must create. It is also used when a class wants its object creation to be mentioned by
a subclass. It is also used when a class wants to shift the responsibility to one of its
subclasses. The main consequences of this pattern are:
1. The pattern provides the classes with hooks or placeholders for the subclasses.
2. The pattern also helps in connecting parallel class hierarchies.
3. It brings in more flexibility to the system.
d. Prototype: This pattern type helps in creation of different objects using a prototypical
basic frame that helps in creation of new objects by just copying the pre-defined
prototype. The main use of this pattern is when the system specifies the classes that
needs to be instantiated during run time by dynamic loading. The pattern is also used
in order to avoid the building of a class hierarchy of the factories which parallels that
of the products. It is also used when a class needs only a few specific combinations of
the state. The main consequences of using this pattern are:
1. It allows the removal and addition of products during runtime.
2. It allows the specification of new objects by changing or modifying the values.
3. It also allows the user to mention new objects by changing the structure.
4. It also results in the reduction of subclassing.
e. Singleton: This pattern helps the user by ensuring that a single instance of that class is
created and it has global permissions. The main use of this pattern is that it helps the
user developing a single instance class which is accessible to clients from important
and popular access points. It is also used when the main class can be extended using a
subclass without modifying the codes. The main consequences of this pattern are:
1. It allows controlled access to the only class.
2. It allows many different number of instances.
3. It reduces the name space needed for single instances by reducing global
variables.
4. It makes the system for flexible than normal class operations.
5. It allows the refinement of representation and operations for the intended class.
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
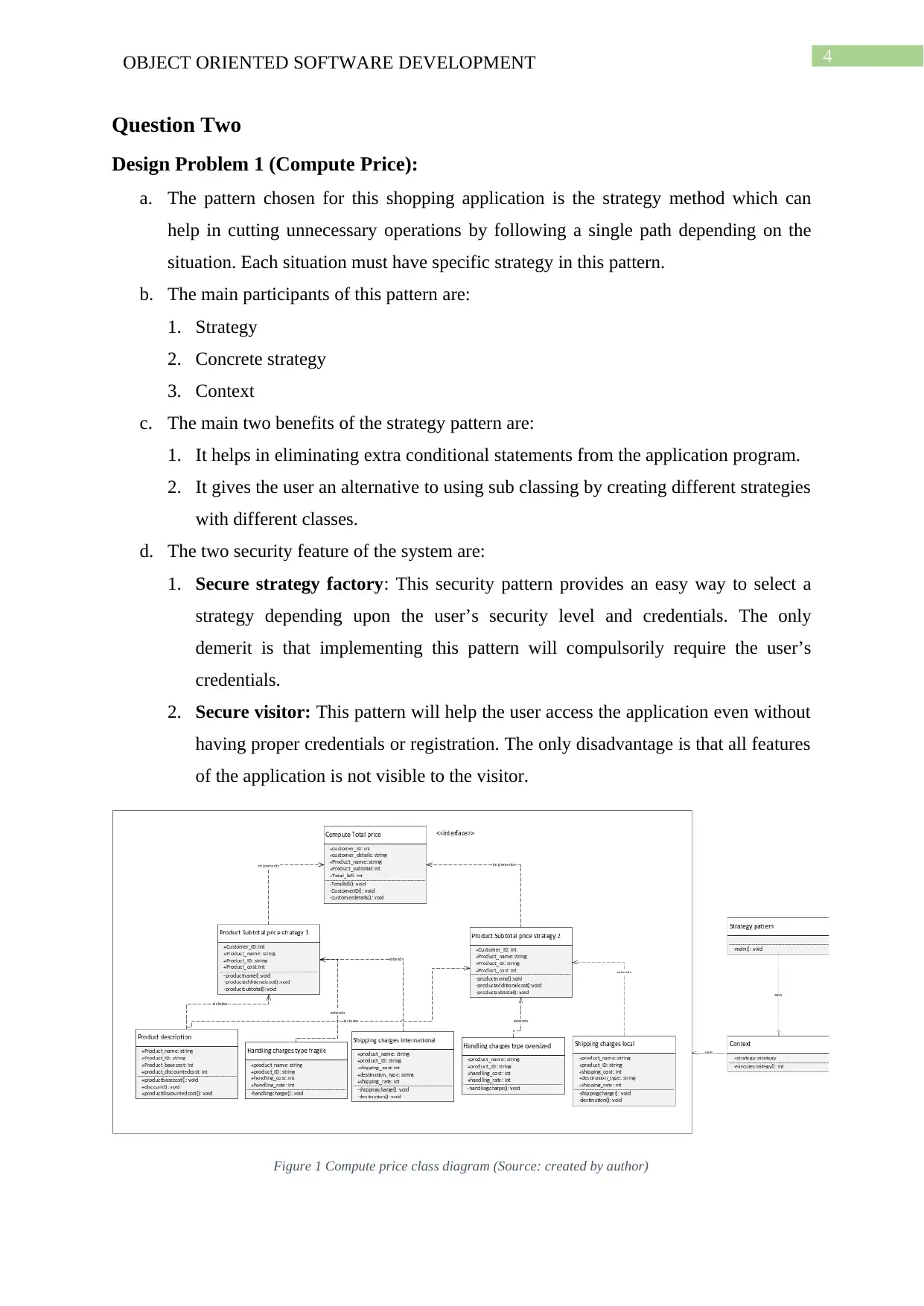
4OBJECT ORIENTED SOFTWARE DEVELOPMENT
Question Two
Design Problem 1 (Compute Price):
a. The pattern chosen for this shopping application is the strategy method which can
help in cutting unnecessary operations by following a single path depending on the
situation. Each situation must have specific strategy in this pattern.
b. The main participants of this pattern are:
1. Strategy
2. Concrete strategy
3. Context
c. The main two benefits of the strategy pattern are:
1. It helps in eliminating extra conditional statements from the application program.
2. It gives the user an alternative to using sub classing by creating different strategies
with different classes.
d. The two security feature of the system are:
1. Secure strategy factory: This security pattern provides an easy way to select a
strategy depending upon the user’s security level and credentials. The only
demerit is that implementing this pattern will compulsorily require the user’s
credentials.
2. Secure visitor: This pattern will help the user access the application even without
having proper credentials or registration. The only disadvantage is that all features
of the application is not visible to the visitor.
Figure 1 Compute price class diagram (Source: created by author)
Question Two
Design Problem 1 (Compute Price):
a. The pattern chosen for this shopping application is the strategy method which can
help in cutting unnecessary operations by following a single path depending on the
situation. Each situation must have specific strategy in this pattern.
b. The main participants of this pattern are:
1. Strategy
2. Concrete strategy
3. Context
c. The main two benefits of the strategy pattern are:
1. It helps in eliminating extra conditional statements from the application program.
2. It gives the user an alternative to using sub classing by creating different strategies
with different classes.
d. The two security feature of the system are:
1. Secure strategy factory: This security pattern provides an easy way to select a
strategy depending upon the user’s security level and credentials. The only
demerit is that implementing this pattern will compulsorily require the user’s
credentials.
2. Secure visitor: This pattern will help the user access the application even without
having proper credentials or registration. The only disadvantage is that all features
of the application is not visible to the visitor.
Figure 1 Compute price class diagram (Source: created by author)
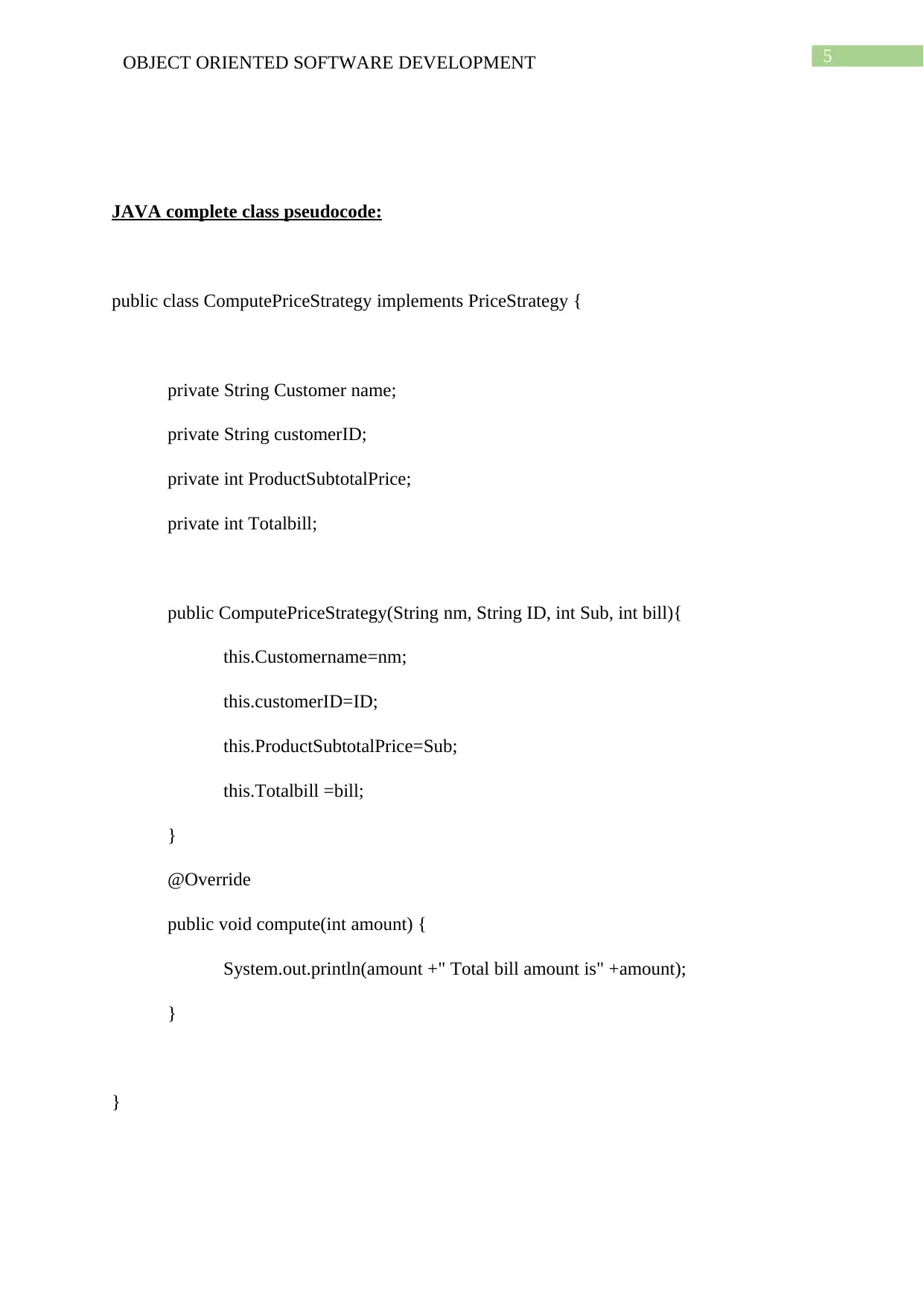
5OBJECT ORIENTED SOFTWARE DEVELOPMENT
JAVA complete class pseudocode:
public class ComputePriceStrategy implements PriceStrategy {
private String Customer name;
private String customerID;
private int ProductSubtotalPrice;
private int Totalbill;
public ComputePriceStrategy(String nm, String ID, int Sub, int bill){
this.Customername=nm;
this.customerID=ID;
this.ProductSubtotalPrice=Sub;
this.Totalbill =bill;
}
@Override
public void compute(int amount) {
System.out.println(amount +" Total bill amount is" +amount);
}
}
JAVA complete class pseudocode:
public class ComputePriceStrategy implements PriceStrategy {
private String Customer name;
private String customerID;
private int ProductSubtotalPrice;
private int Totalbill;
public ComputePriceStrategy(String nm, String ID, int Sub, int bill){
this.Customername=nm;
this.customerID=ID;
this.ProductSubtotalPrice=Sub;
this.Totalbill =bill;
}
@Override
public void compute(int amount) {
System.out.println(amount +" Total bill amount is" +amount);
}
}
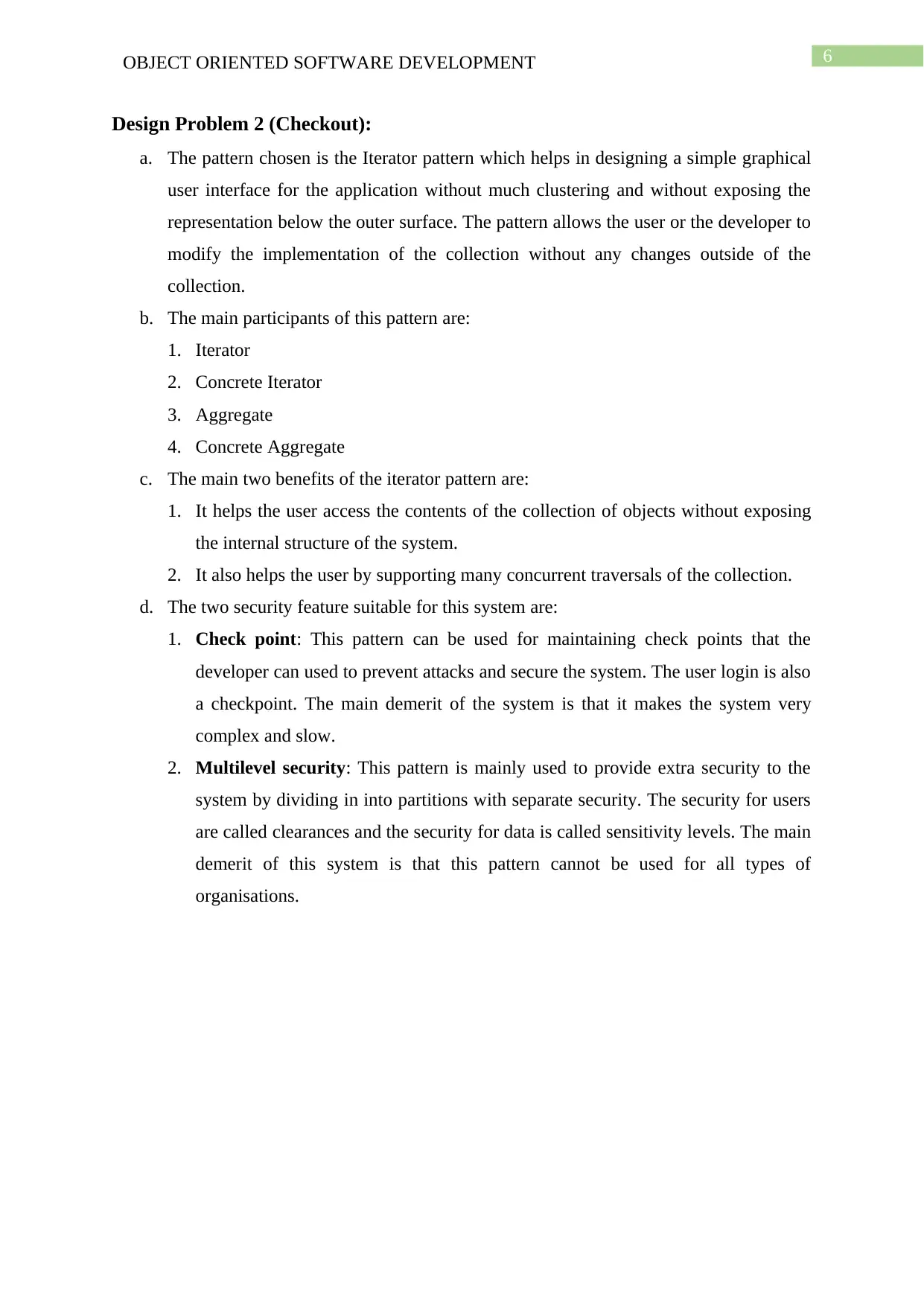
6OBJECT ORIENTED SOFTWARE DEVELOPMENT
Design Problem 2 (Checkout):
a. The pattern chosen is the Iterator pattern which helps in designing a simple graphical
user interface for the application without much clustering and without exposing the
representation below the outer surface. The pattern allows the user or the developer to
modify the implementation of the collection without any changes outside of the
collection.
b. The main participants of this pattern are:
1. Iterator
2. Concrete Iterator
3. Aggregate
4. Concrete Aggregate
c. The main two benefits of the iterator pattern are:
1. It helps the user access the contents of the collection of objects without exposing
the internal structure of the system.
2. It also helps the user by supporting many concurrent traversals of the collection.
d. The two security feature suitable for this system are:
1. Check point: This pattern can be used for maintaining check points that the
developer can used to prevent attacks and secure the system. The user login is also
a checkpoint. The main demerit of the system is that it makes the system very
complex and slow.
2. Multilevel security: This pattern is mainly used to provide extra security to the
system by dividing in into partitions with separate security. The security for users
are called clearances and the security for data is called sensitivity levels. The main
demerit of this system is that this pattern cannot be used for all types of
organisations.
Design Problem 2 (Checkout):
a. The pattern chosen is the Iterator pattern which helps in designing a simple graphical
user interface for the application without much clustering and without exposing the
representation below the outer surface. The pattern allows the user or the developer to
modify the implementation of the collection without any changes outside of the
collection.
b. The main participants of this pattern are:
1. Iterator
2. Concrete Iterator
3. Aggregate
4. Concrete Aggregate
c. The main two benefits of the iterator pattern are:
1. It helps the user access the contents of the collection of objects without exposing
the internal structure of the system.
2. It also helps the user by supporting many concurrent traversals of the collection.
d. The two security feature suitable for this system are:
1. Check point: This pattern can be used for maintaining check points that the
developer can used to prevent attacks and secure the system. The user login is also
a checkpoint. The main demerit of the system is that it makes the system very
complex and slow.
2. Multilevel security: This pattern is mainly used to provide extra security to the
system by dividing in into partitions with separate security. The security for users
are called clearances and the security for data is called sensitivity levels. The main
demerit of this system is that this pattern cannot be used for all types of
organisations.
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
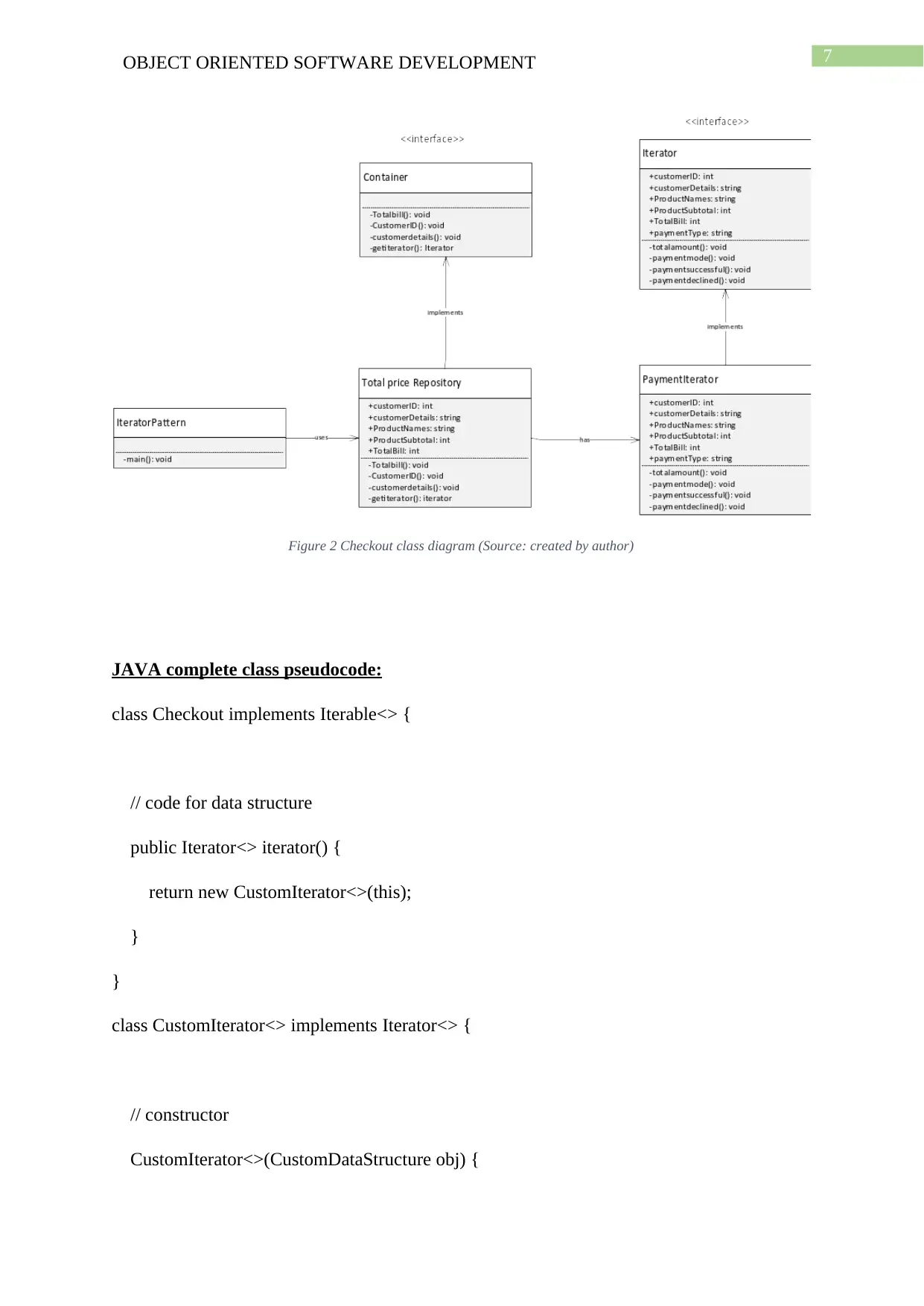
7OBJECT ORIENTED SOFTWARE DEVELOPMENT
Figure 2 Checkout class diagram (Source: created by author)
JAVA complete class pseudocode:
class Checkout implements Iterable<> {
// code for data structure
public Iterator<> iterator() {
return new CustomIterator<>(this);
}
}
class CustomIterator<> implements Iterator<> {
// constructor
CustomIterator<>(CustomDataStructure obj) {
Figure 2 Checkout class diagram (Source: created by author)
JAVA complete class pseudocode:
class Checkout implements Iterable<> {
// code for data structure
public Iterator<> iterator() {
return new CustomIterator<>(this);
}
}
class CustomIterator<> implements Iterator<> {
// constructor
CustomIterator<>(CustomDataStructure obj) {
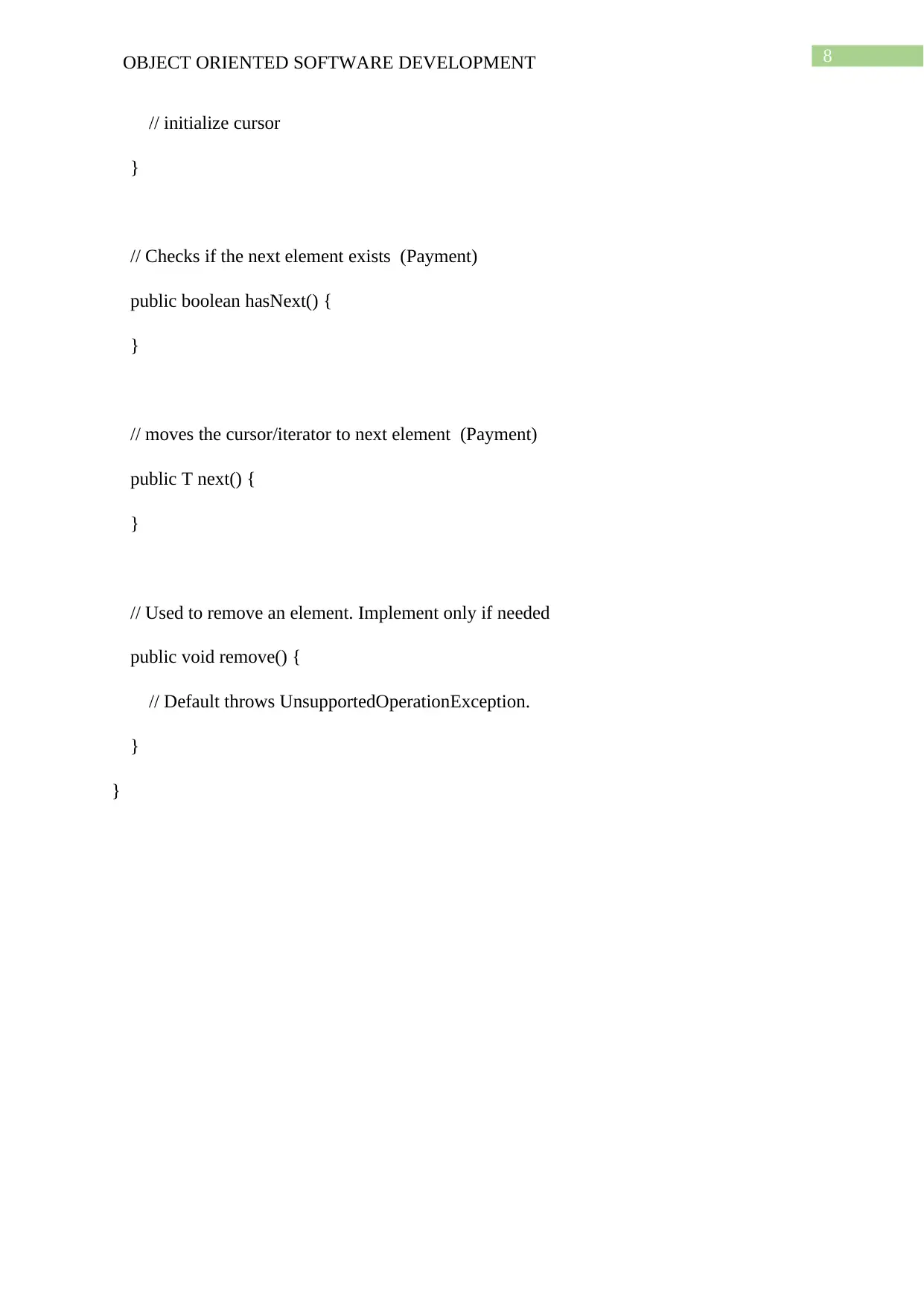
8OBJECT ORIENTED SOFTWARE DEVELOPMENT
// initialize cursor
}
// Checks if the next element exists (Payment)
public boolean hasNext() {
}
// moves the cursor/iterator to next element (Payment)
public T next() {
}
// Used to remove an element. Implement only if needed
public void remove() {
// Default throws UnsupportedOperationException.
}
}
// initialize cursor
}
// Checks if the next element exists (Payment)
public boolean hasNext() {
}
// moves the cursor/iterator to next element (Payment)
public T next() {
}
// Used to remove an element. Implement only if needed
public void remove() {
// Default throws UnsupportedOperationException.
}
}
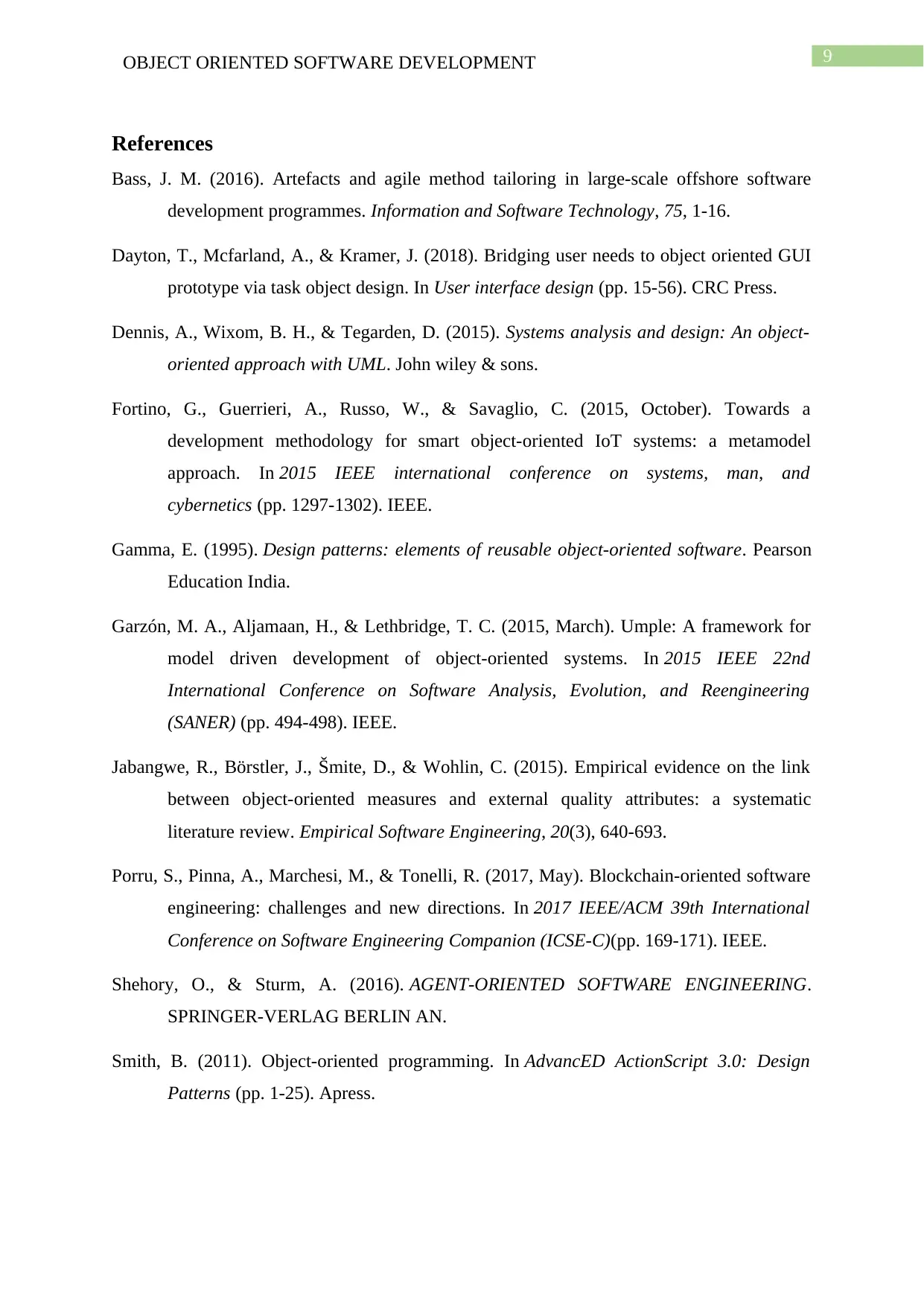
9OBJECT ORIENTED SOFTWARE DEVELOPMENT
References
Bass, J. M. (2016). Artefacts and agile method tailoring in large-scale offshore software
development programmes. Information and Software Technology, 75, 1-16.
Dayton, T., Mcfarland, A., & Kramer, J. (2018). Bridging user needs to object oriented GUI
prototype via task object design. In User interface design (pp. 15-56). CRC Press.
Dennis, A., Wixom, B. H., & Tegarden, D. (2015). Systems analysis and design: An object-
oriented approach with UML. John wiley & sons.
Fortino, G., Guerrieri, A., Russo, W., & Savaglio, C. (2015, October). Towards a
development methodology for smart object-oriented IoT systems: a metamodel
approach. In 2015 IEEE international conference on systems, man, and
cybernetics (pp. 1297-1302). IEEE.
Gamma, E. (1995). Design patterns: elements of reusable object-oriented software. Pearson
Education India.
Garzón, M. A., Aljamaan, H., & Lethbridge, T. C. (2015, March). Umple: A framework for
model driven development of object-oriented systems. In 2015 IEEE 22nd
International Conference on Software Analysis, Evolution, and Reengineering
(SANER) (pp. 494-498). IEEE.
Jabangwe, R., Börstler, J., Šmite, D., & Wohlin, C. (2015). Empirical evidence on the link
between object-oriented measures and external quality attributes: a systematic
literature review. Empirical Software Engineering, 20(3), 640-693.
Porru, S., Pinna, A., Marchesi, M., & Tonelli, R. (2017, May). Blockchain-oriented software
engineering: challenges and new directions. In 2017 IEEE/ACM 39th International
Conference on Software Engineering Companion (ICSE-C)(pp. 169-171). IEEE.
Shehory, O., & Sturm, A. (2016). AGENT-ORIENTED SOFTWARE ENGINEERING.
SPRINGER-VERLAG BERLIN AN.
Smith, B. (2011). Object-oriented programming. In AdvancED ActionScript 3.0: Design
Patterns (pp. 1-25). Apress.
References
Bass, J. M. (2016). Artefacts and agile method tailoring in large-scale offshore software
development programmes. Information and Software Technology, 75, 1-16.
Dayton, T., Mcfarland, A., & Kramer, J. (2018). Bridging user needs to object oriented GUI
prototype via task object design. In User interface design (pp. 15-56). CRC Press.
Dennis, A., Wixom, B. H., & Tegarden, D. (2015). Systems analysis and design: An object-
oriented approach with UML. John wiley & sons.
Fortino, G., Guerrieri, A., Russo, W., & Savaglio, C. (2015, October). Towards a
development methodology for smart object-oriented IoT systems: a metamodel
approach. In 2015 IEEE international conference on systems, man, and
cybernetics (pp. 1297-1302). IEEE.
Gamma, E. (1995). Design patterns: elements of reusable object-oriented software. Pearson
Education India.
Garzón, M. A., Aljamaan, H., & Lethbridge, T. C. (2015, March). Umple: A framework for
model driven development of object-oriented systems. In 2015 IEEE 22nd
International Conference on Software Analysis, Evolution, and Reengineering
(SANER) (pp. 494-498). IEEE.
Jabangwe, R., Börstler, J., Šmite, D., & Wohlin, C. (2015). Empirical evidence on the link
between object-oriented measures and external quality attributes: a systematic
literature review. Empirical Software Engineering, 20(3), 640-693.
Porru, S., Pinna, A., Marchesi, M., & Tonelli, R. (2017, May). Blockchain-oriented software
engineering: challenges and new directions. In 2017 IEEE/ACM 39th International
Conference on Software Engineering Companion (ICSE-C)(pp. 169-171). IEEE.
Shehory, O., & Sturm, A. (2016). AGENT-ORIENTED SOFTWARE ENGINEERING.
SPRINGER-VERLAG BERLIN AN.
Smith, B. (2011). Object-oriented programming. In AdvancED ActionScript 3.0: Design
Patterns (pp. 1-25). Apress.
1 out of 10
Related Documents
![[object Object]](/_next/image/?url=%2F_next%2Fstatic%2Fmedia%2Flogo.6d15ce61.png&w=640&q=75)
Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.