Programming Assignment: Implementing Basic Algorithms in Python
VerifiedAdded on 2024/05/21
|39
|4032
|148
AI Summary
This assignment focuses on implementing basic algorithms in Python using the IDLE IDE. It covers defining algorithms, outlining the programming process, explaining different programming paradigms (procedural, object-oriented, and event-driven), and analyzing the features of IDLE. The assignment includes a detailed documentation section with algorithms, flowcharts, pseudocode, source code screenshots, output screenshots, and a discussion on debugging and coding standards. The application developed is a console-based program that demonstrates the implementation of various algorithms.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
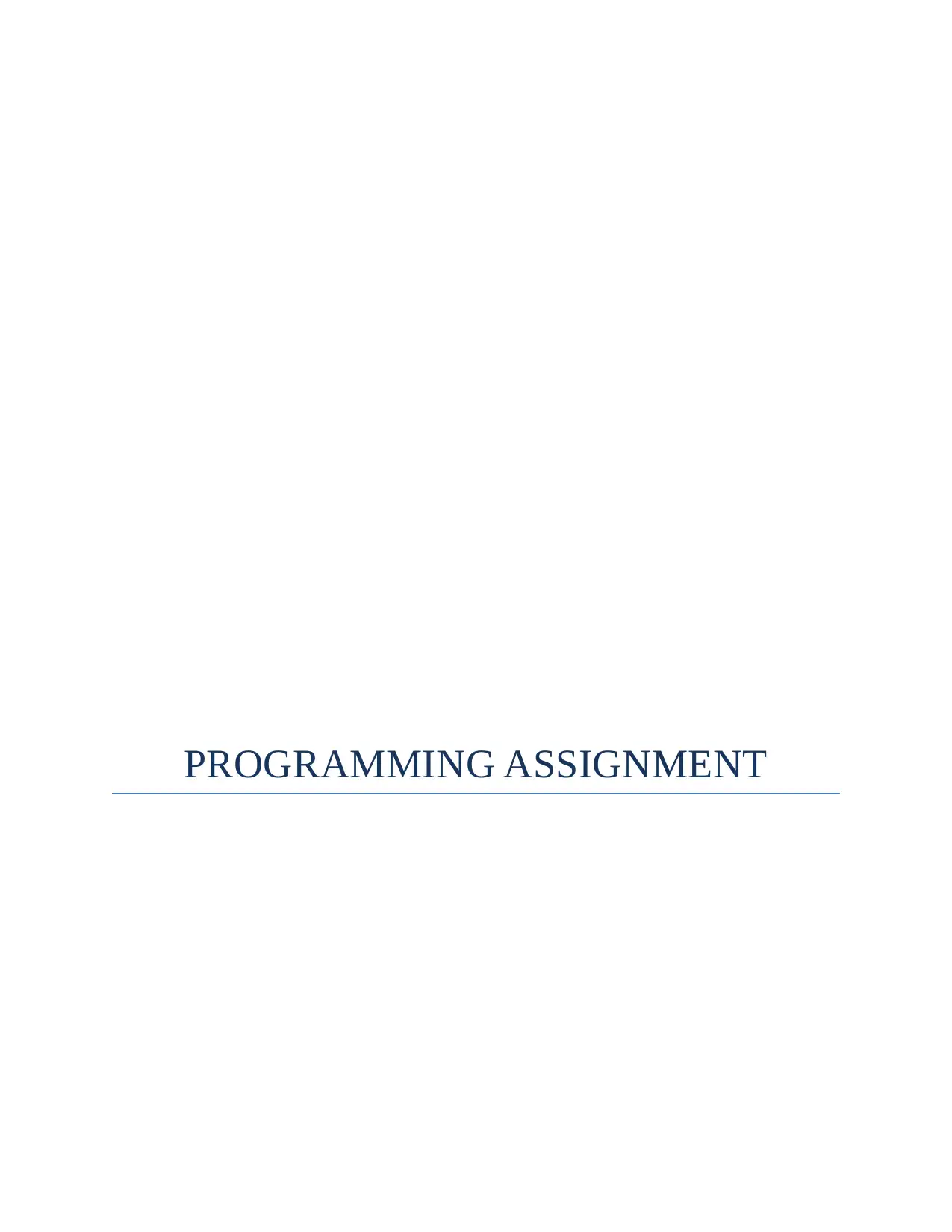
PROGRAMMING ASSIGNMENT
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
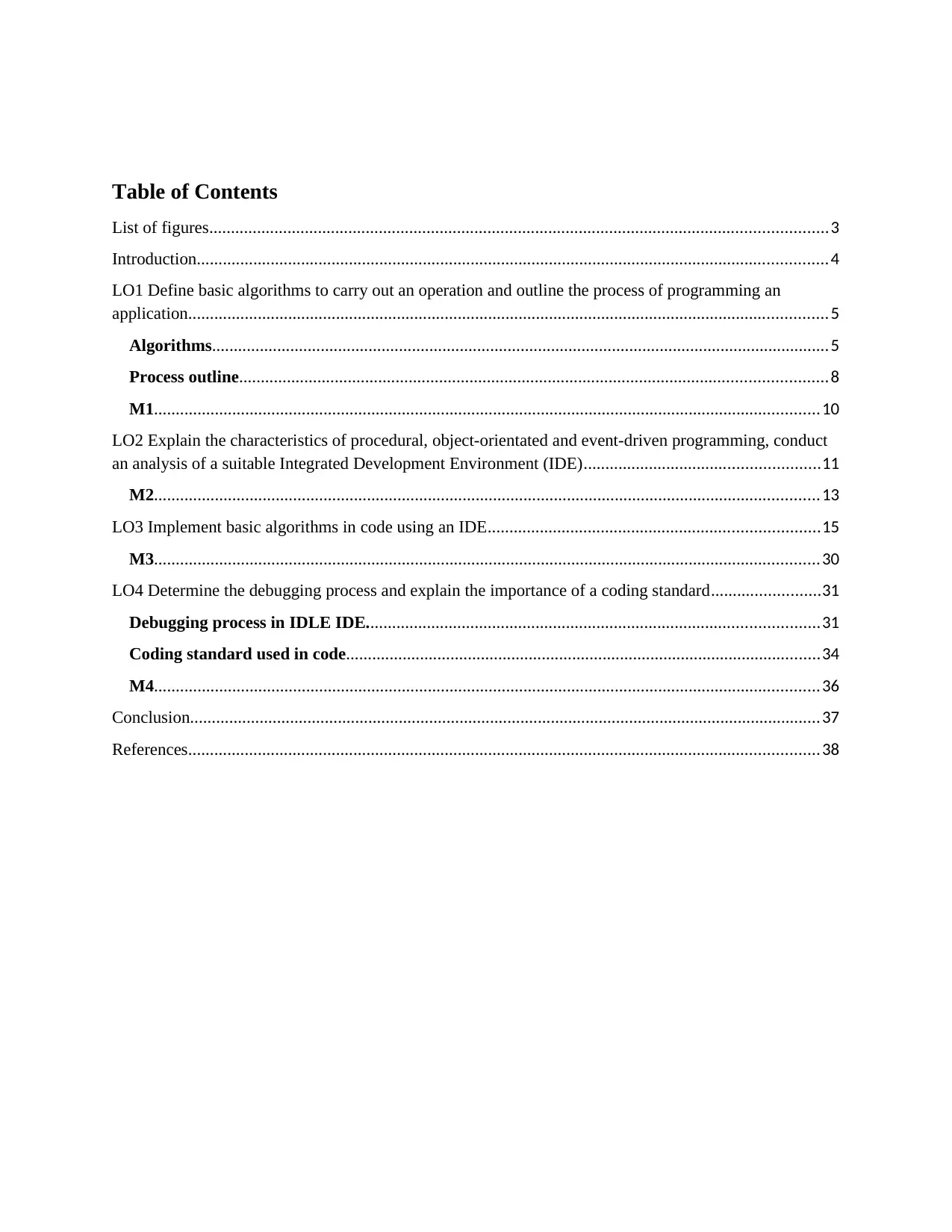
Table of Contents
List of figures..............................................................................................................................................3
Introduction.................................................................................................................................................4
LO1 Define basic algorithms to carry out an operation and outline the process of programming an
application...................................................................................................................................................5
Algorithms..............................................................................................................................................5
Process outline.......................................................................................................................................8
M1.........................................................................................................................................................10
LO2 Explain the characteristics of procedural, object-orientated and event-driven programming, conduct
an analysis of a suitable Integrated Development Environment (IDE)......................................................11
M2.........................................................................................................................................................13
LO3 Implement basic algorithms in code using an IDE............................................................................15
M3.........................................................................................................................................................30
LO4 Determine the debugging process and explain the importance of a coding standard.........................31
Debugging process in IDLE IDE........................................................................................................31
Coding standard used in code.............................................................................................................34
M4.........................................................................................................................................................36
Conclusion.................................................................................................................................................37
References.................................................................................................................................................38
List of figures..............................................................................................................................................3
Introduction.................................................................................................................................................4
LO1 Define basic algorithms to carry out an operation and outline the process of programming an
application...................................................................................................................................................5
Algorithms..............................................................................................................................................5
Process outline.......................................................................................................................................8
M1.........................................................................................................................................................10
LO2 Explain the characteristics of procedural, object-orientated and event-driven programming, conduct
an analysis of a suitable Integrated Development Environment (IDE)......................................................11
M2.........................................................................................................................................................13
LO3 Implement basic algorithms in code using an IDE............................................................................15
M3.........................................................................................................................................................30
LO4 Determine the debugging process and explain the importance of a coding standard.........................31
Debugging process in IDLE IDE........................................................................................................31
Coding standard used in code.............................................................................................................34
M4.........................................................................................................................................................36
Conclusion.................................................................................................................................................37
References.................................................................................................................................................38
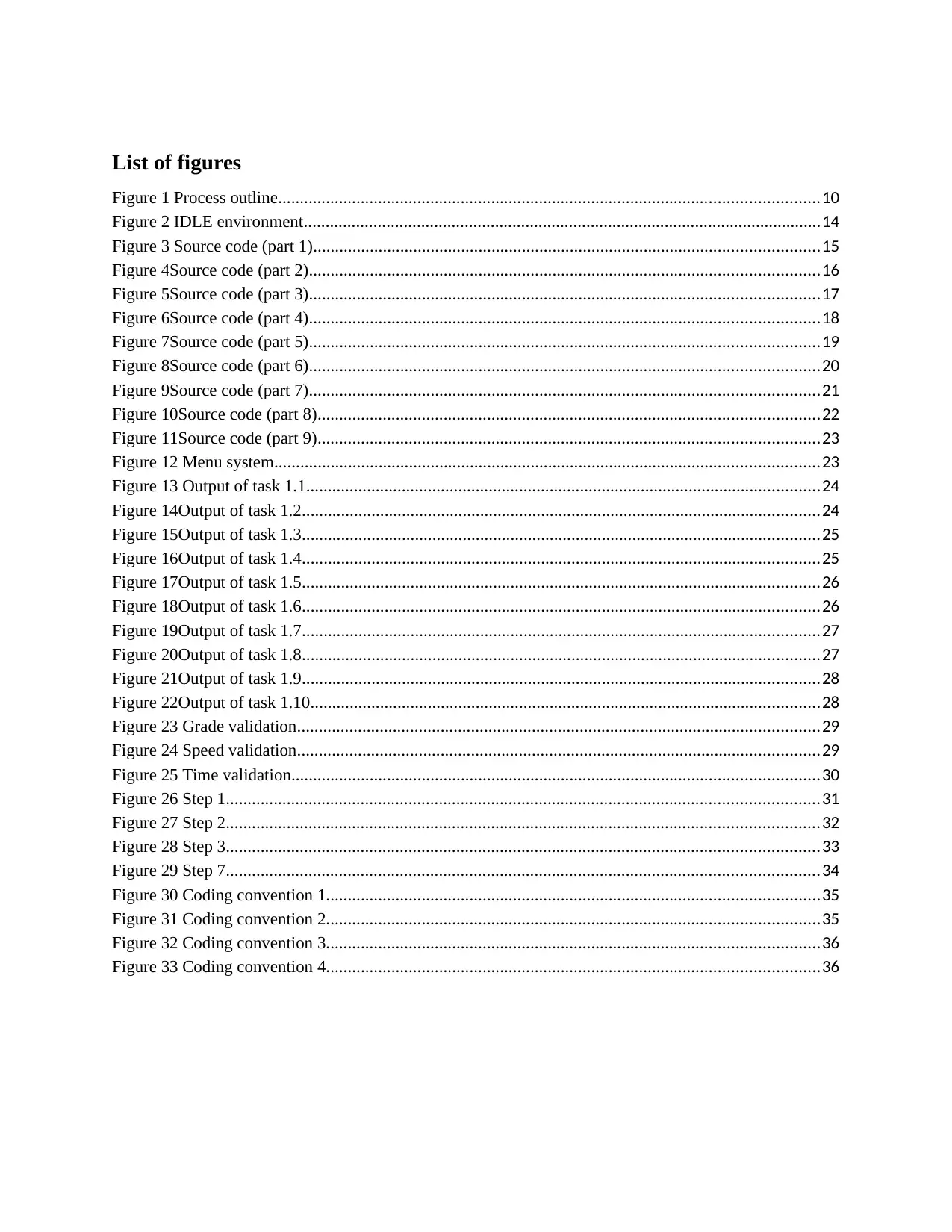
List of figures
Figure 1 Process outline............................................................................................................................10
Figure 2 IDLE environment.......................................................................................................................14
Figure 3 Source code (part 1)....................................................................................................................15
Figure 4Source code (part 2).....................................................................................................................16
Figure 5Source code (part 3).....................................................................................................................17
Figure 6Source code (part 4).....................................................................................................................18
Figure 7Source code (part 5).....................................................................................................................19
Figure 8Source code (part 6).....................................................................................................................20
Figure 9Source code (part 7).....................................................................................................................21
Figure 10Source code (part 8)...................................................................................................................22
Figure 11Source code (part 9)...................................................................................................................23
Figure 12 Menu system.............................................................................................................................23
Figure 13 Output of task 1.1......................................................................................................................24
Figure 14Output of task 1.2.......................................................................................................................24
Figure 15Output of task 1.3.......................................................................................................................25
Figure 16Output of task 1.4.......................................................................................................................25
Figure 17Output of task 1.5.......................................................................................................................26
Figure 18Output of task 1.6.......................................................................................................................26
Figure 19Output of task 1.7.......................................................................................................................27
Figure 20Output of task 1.8.......................................................................................................................27
Figure 21Output of task 1.9.......................................................................................................................28
Figure 22Output of task 1.10.....................................................................................................................28
Figure 23 Grade validation........................................................................................................................29
Figure 24 Speed validation........................................................................................................................29
Figure 25 Time validation.........................................................................................................................30
Figure 26 Step 1........................................................................................................................................31
Figure 27 Step 2........................................................................................................................................32
Figure 28 Step 3........................................................................................................................................33
Figure 29 Step 7........................................................................................................................................34
Figure 30 Coding convention 1.................................................................................................................35
Figure 31 Coding convention 2.................................................................................................................35
Figure 32 Coding convention 3.................................................................................................................36
Figure 33 Coding convention 4.................................................................................................................36
Figure 1 Process outline............................................................................................................................10
Figure 2 IDLE environment.......................................................................................................................14
Figure 3 Source code (part 1)....................................................................................................................15
Figure 4Source code (part 2).....................................................................................................................16
Figure 5Source code (part 3).....................................................................................................................17
Figure 6Source code (part 4).....................................................................................................................18
Figure 7Source code (part 5).....................................................................................................................19
Figure 8Source code (part 6).....................................................................................................................20
Figure 9Source code (part 7).....................................................................................................................21
Figure 10Source code (part 8)...................................................................................................................22
Figure 11Source code (part 9)...................................................................................................................23
Figure 12 Menu system.............................................................................................................................23
Figure 13 Output of task 1.1......................................................................................................................24
Figure 14Output of task 1.2.......................................................................................................................24
Figure 15Output of task 1.3.......................................................................................................................25
Figure 16Output of task 1.4.......................................................................................................................25
Figure 17Output of task 1.5.......................................................................................................................26
Figure 18Output of task 1.6.......................................................................................................................26
Figure 19Output of task 1.7.......................................................................................................................27
Figure 20Output of task 1.8.......................................................................................................................27
Figure 21Output of task 1.9.......................................................................................................................28
Figure 22Output of task 1.10.....................................................................................................................28
Figure 23 Grade validation........................................................................................................................29
Figure 24 Speed validation........................................................................................................................29
Figure 25 Time validation.........................................................................................................................30
Figure 26 Step 1........................................................................................................................................31
Figure 27 Step 2........................................................................................................................................32
Figure 28 Step 3........................................................................................................................................33
Figure 29 Step 7........................................................................................................................................34
Figure 30 Coding convention 1.................................................................................................................35
Figure 31 Coding convention 2.................................................................................................................35
Figure 32 Coding convention 3.................................................................................................................36
Figure 33 Coding convention 4.................................................................................................................36
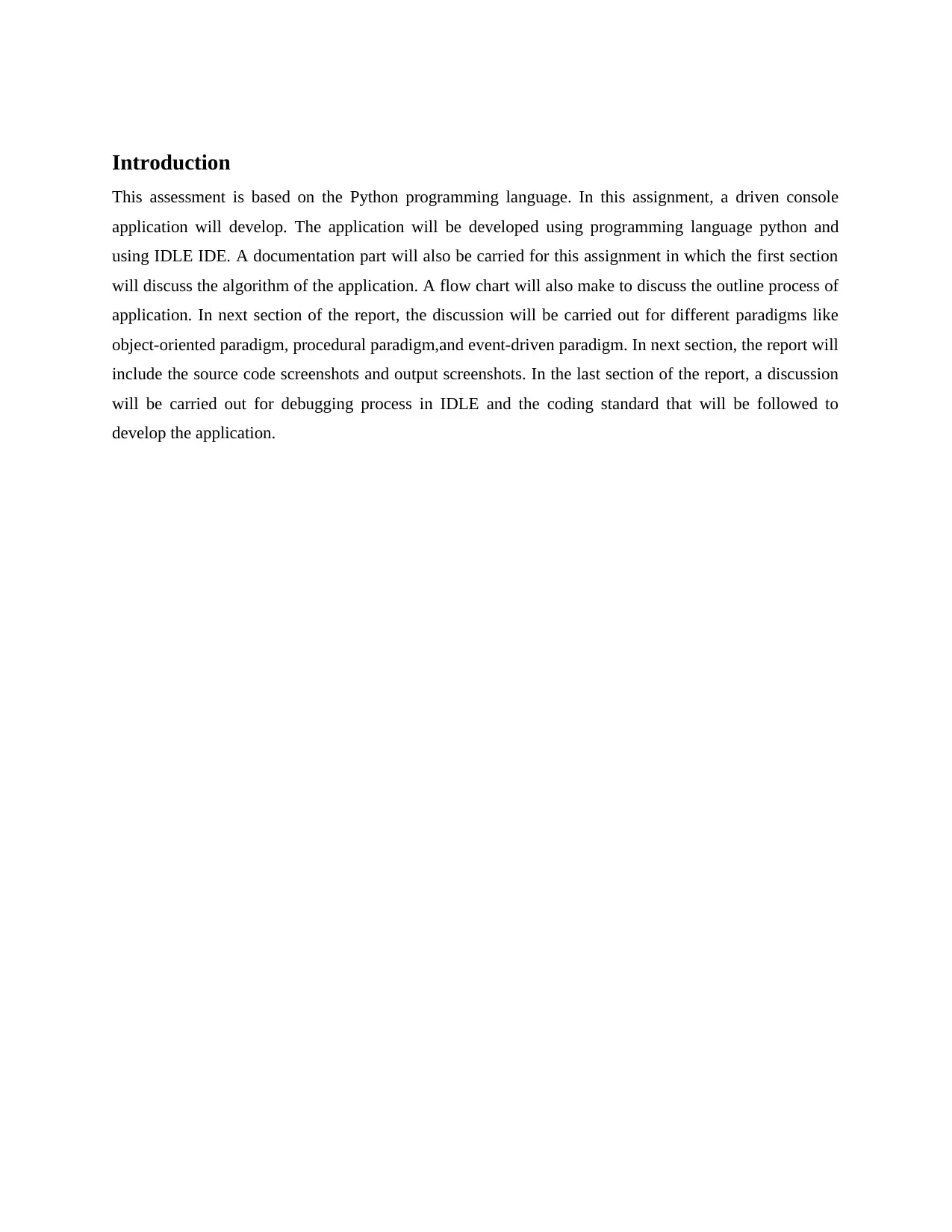
Introduction
This assessment is based on the Python programming language. In this assignment, a driven console
application will develop. The application will be developed using programming language python and
using IDLE IDE. A documentation part will also be carried for this assignment in which the first section
will discuss the algorithm of the application. A flow chart will also make to discuss the outline process of
application. In next section of the report, the discussion will be carried out for different paradigms like
object-oriented paradigm, procedural paradigm,and event-driven paradigm. In next section, the report will
include the source code screenshots and output screenshots. In the last section of the report, a discussion
will be carried out for debugging process in IDLE and the coding standard that will be followed to
develop the application.
This assessment is based on the Python programming language. In this assignment, a driven console
application will develop. The application will be developed using programming language python and
using IDLE IDE. A documentation part will also be carried for this assignment in which the first section
will discuss the algorithm of the application. A flow chart will also make to discuss the outline process of
application. In next section of the report, the discussion will be carried out for different paradigms like
object-oriented paradigm, procedural paradigm,and event-driven paradigm. In next section, the report will
include the source code screenshots and output screenshots. In the last section of the report, a discussion
will be carried out for debugging process in IDLE and the coding standard that will be followed to
develop the application.
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
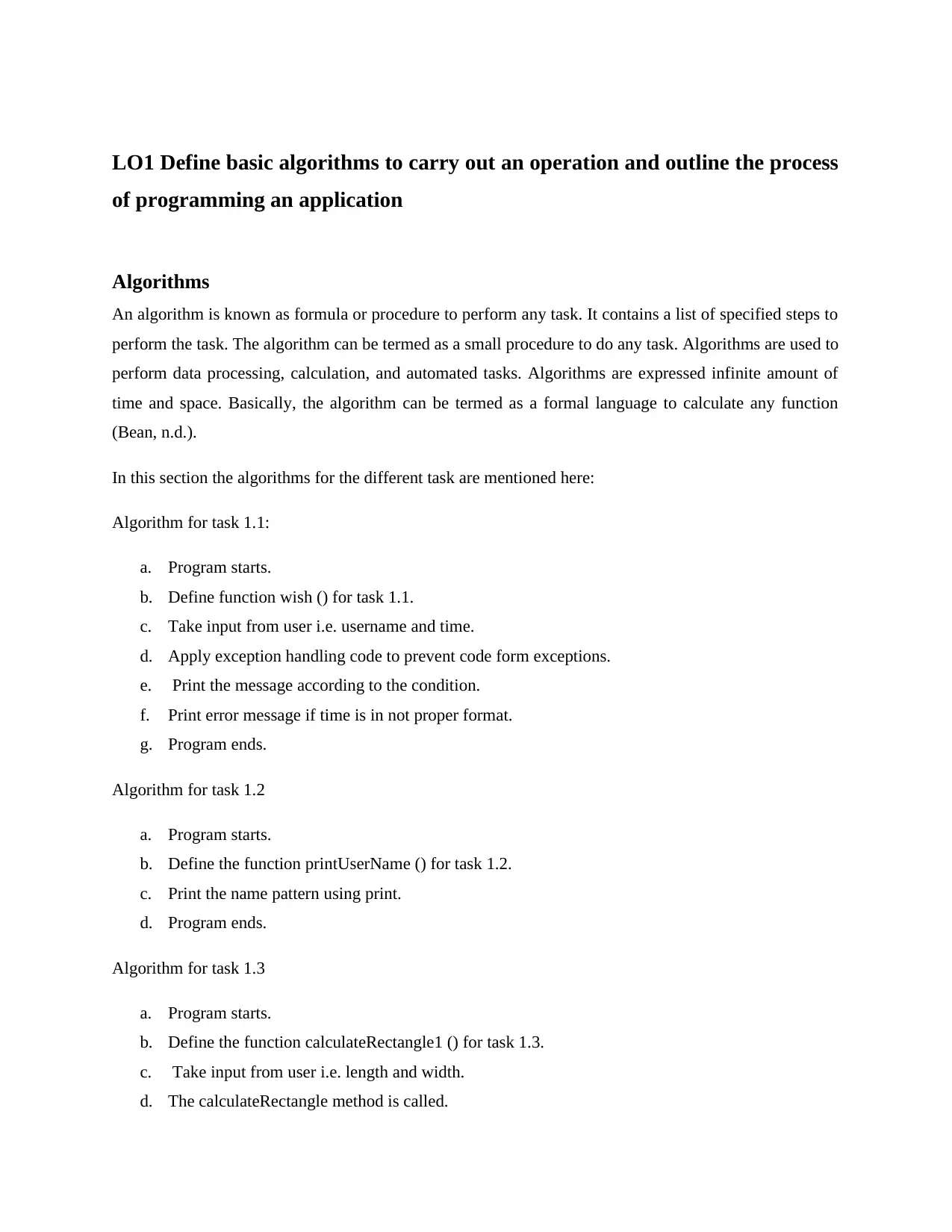
LO1 Define basic algorithms to carry out an operation and outline the process
of programming an application
Algorithms
An algorithm is known as formula or procedure to perform any task. It contains a list of specified steps to
perform the task. The algorithm can be termed as a small procedure to do any task. Algorithms are used to
perform data processing, calculation, and automated tasks. Algorithms are expressed infinite amount of
time and space. Basically, the algorithm can be termed as a formal language to calculate any function
(Bean, n.d.).
In this section the algorithms for the different task are mentioned here:
Algorithm for task 1.1:
a. Program starts.
b. Define function wish () for task 1.1.
c. Take input from user i.e. username and time.
d. Apply exception handling code to prevent code form exceptions.
e. Print the message according to the condition.
f. Print error message if time is in not proper format.
g. Program ends.
Algorithm for task 1.2
a. Program starts.
b. Define the function printUserName () for task 1.2.
c. Print the name pattern using print.
d. Program ends.
Algorithm for task 1.3
a. Program starts.
b. Define the function calculateRectangle1 () for task 1.3.
c. Take input from user i.e. length and width.
d. The calculateRectangle method is called.
of programming an application
Algorithms
An algorithm is known as formula or procedure to perform any task. It contains a list of specified steps to
perform the task. The algorithm can be termed as a small procedure to do any task. Algorithms are used to
perform data processing, calculation, and automated tasks. Algorithms are expressed infinite amount of
time and space. Basically, the algorithm can be termed as a formal language to calculate any function
(Bean, n.d.).
In this section the algorithms for the different task are mentioned here:
Algorithm for task 1.1:
a. Program starts.
b. Define function wish () for task 1.1.
c. Take input from user i.e. username and time.
d. Apply exception handling code to prevent code form exceptions.
e. Print the message according to the condition.
f. Print error message if time is in not proper format.
g. Program ends.
Algorithm for task 1.2
a. Program starts.
b. Define the function printUserName () for task 1.2.
c. Print the name pattern using print.
d. Program ends.
Algorithm for task 1.3
a. Program starts.
b. Define the function calculateRectangle1 () for task 1.3.
c. Take input from user i.e. length and width.
d. The calculateRectangle method is called.
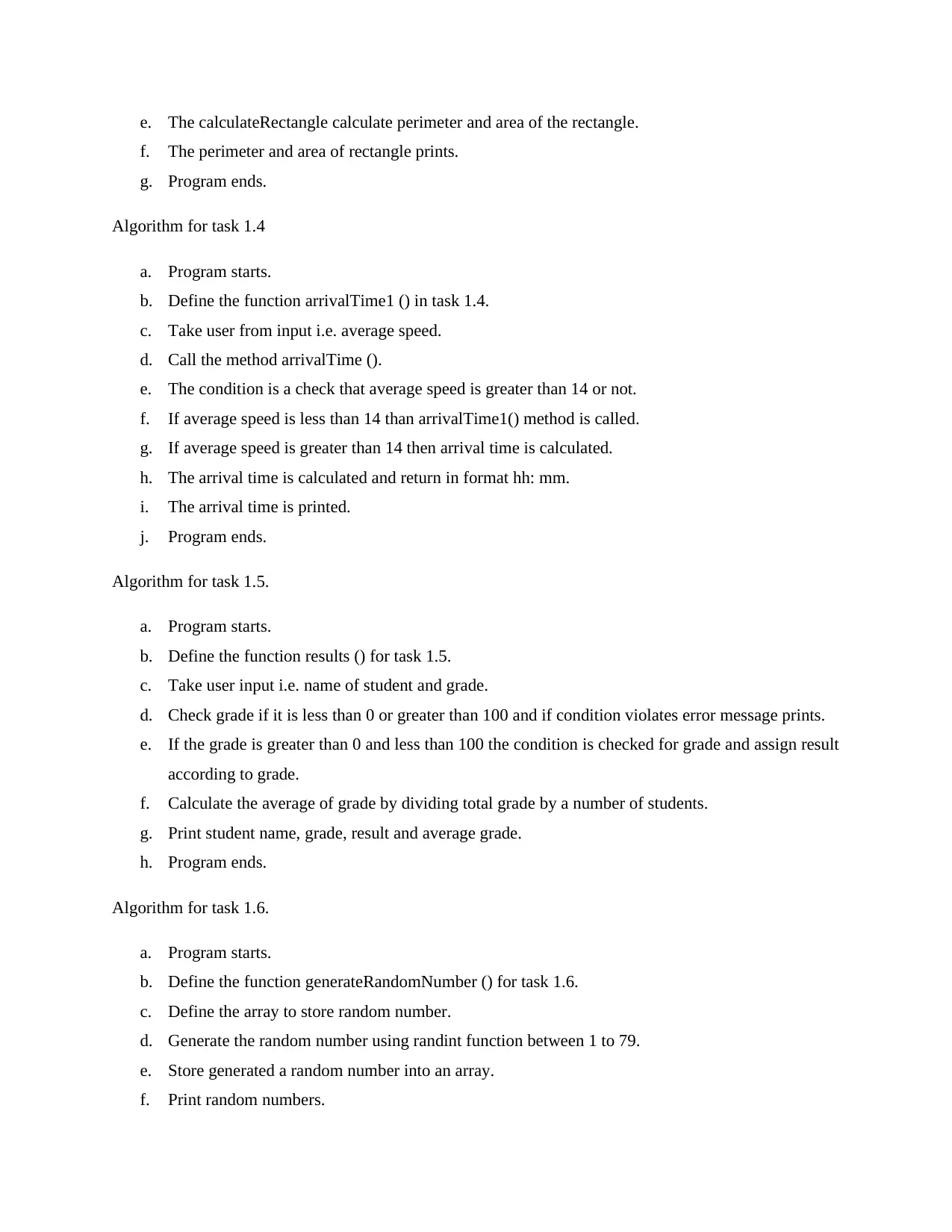
e. The calculateRectangle calculate perimeter and area of the rectangle.
f. The perimeter and area of rectangle prints.
g. Program ends.
Algorithm for task 1.4
a. Program starts.
b. Define the function arrivalTime1 () in task 1.4.
c. Take user from input i.e. average speed.
d. Call the method arrivalTime ().
e. The condition is a check that average speed is greater than 14 or not.
f. If average speed is less than 14 than arrivalTime1() method is called.
g. If average speed is greater than 14 then arrival time is calculated.
h. The arrival time is calculated and return in format hh: mm.
i. The arrival time is printed.
j. Program ends.
Algorithm for task 1.5.
a. Program starts.
b. Define the function results () for task 1.5.
c. Take user input i.e. name of student and grade.
d. Check grade if it is less than 0 or greater than 100 and if condition violates error message prints.
e. If the grade is greater than 0 and less than 100 the condition is checked for grade and assign result
according to grade.
f. Calculate the average of grade by dividing total grade by a number of students.
g. Print student name, grade, result and average grade.
h. Program ends.
Algorithm for task 1.6.
a. Program starts.
b. Define the function generateRandomNumber () for task 1.6.
c. Define the array to store random number.
d. Generate the random number using randint function between 1 to 79.
e. Store generated a random number into an array.
f. Print random numbers.
f. The perimeter and area of rectangle prints.
g. Program ends.
Algorithm for task 1.4
a. Program starts.
b. Define the function arrivalTime1 () in task 1.4.
c. Take user from input i.e. average speed.
d. Call the method arrivalTime ().
e. The condition is a check that average speed is greater than 14 or not.
f. If average speed is less than 14 than arrivalTime1() method is called.
g. If average speed is greater than 14 then arrival time is calculated.
h. The arrival time is calculated and return in format hh: mm.
i. The arrival time is printed.
j. Program ends.
Algorithm for task 1.5.
a. Program starts.
b. Define the function results () for task 1.5.
c. Take user input i.e. name of student and grade.
d. Check grade if it is less than 0 or greater than 100 and if condition violates error message prints.
e. If the grade is greater than 0 and less than 100 the condition is checked for grade and assign result
according to grade.
f. Calculate the average of grade by dividing total grade by a number of students.
g. Print student name, grade, result and average grade.
h. Program ends.
Algorithm for task 1.6.
a. Program starts.
b. Define the function generateRandomNumber () for task 1.6.
c. Define the array to store random number.
d. Generate the random number using randint function between 1 to 79.
e. Store generated a random number into an array.
f. Print random numbers.
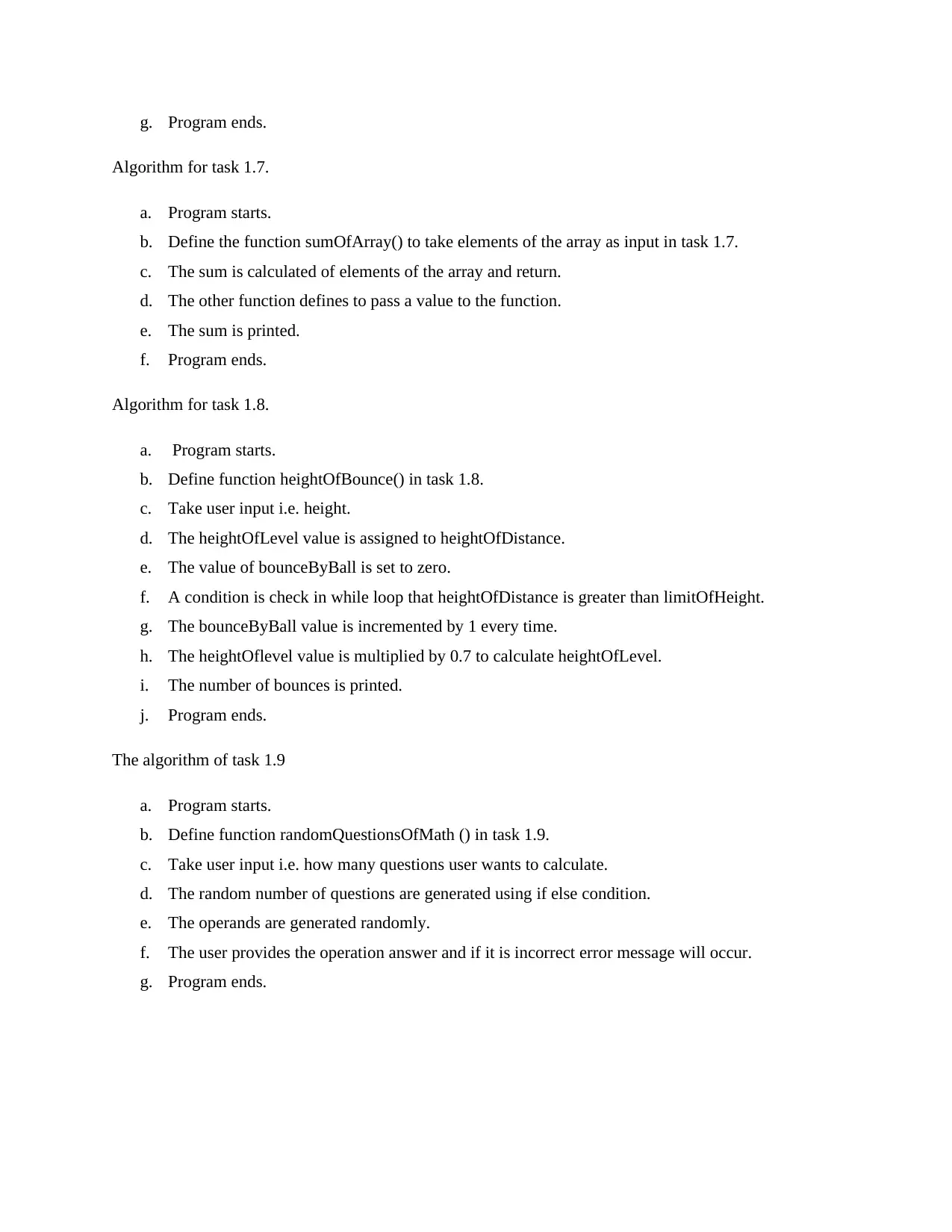
g. Program ends.
Algorithm for task 1.7.
a. Program starts.
b. Define the function sumOfArray() to take elements of the array as input in task 1.7.
c. The sum is calculated of elements of the array and return.
d. The other function defines to pass a value to the function.
e. The sum is printed.
f. Program ends.
Algorithm for task 1.8.
a. Program starts.
b. Define function heightOfBounce() in task 1.8.
c. Take user input i.e. height.
d. The heightOfLevel value is assigned to heightOfDistance.
e. The value of bounceByBall is set to zero.
f. A condition is check in while loop that heightOfDistance is greater than limitOfHeight.
g. The bounceByBall value is incremented by 1 every time.
h. The heightOflevel value is multiplied by 0.7 to calculate heightOfLevel.
i. The number of bounces is printed.
j. Program ends.
The algorithm of task 1.9
a. Program starts.
b. Define function randomQuestionsOfMath () in task 1.9.
c. Take user input i.e. how many questions user wants to calculate.
d. The random number of questions are generated using if else condition.
e. The operands are generated randomly.
f. The user provides the operation answer and if it is incorrect error message will occur.
g. Program ends.
Algorithm for task 1.7.
a. Program starts.
b. Define the function sumOfArray() to take elements of the array as input in task 1.7.
c. The sum is calculated of elements of the array and return.
d. The other function defines to pass a value to the function.
e. The sum is printed.
f. Program ends.
Algorithm for task 1.8.
a. Program starts.
b. Define function heightOfBounce() in task 1.8.
c. Take user input i.e. height.
d. The heightOfLevel value is assigned to heightOfDistance.
e. The value of bounceByBall is set to zero.
f. A condition is check in while loop that heightOfDistance is greater than limitOfHeight.
g. The bounceByBall value is incremented by 1 every time.
h. The heightOflevel value is multiplied by 0.7 to calculate heightOfLevel.
i. The number of bounces is printed.
j. Program ends.
The algorithm of task 1.9
a. Program starts.
b. Define function randomQuestionsOfMath () in task 1.9.
c. Take user input i.e. how many questions user wants to calculate.
d. The random number of questions are generated using if else condition.
e. The operands are generated randomly.
f. The user provides the operation answer and if it is incorrect error message will occur.
g. Program ends.
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
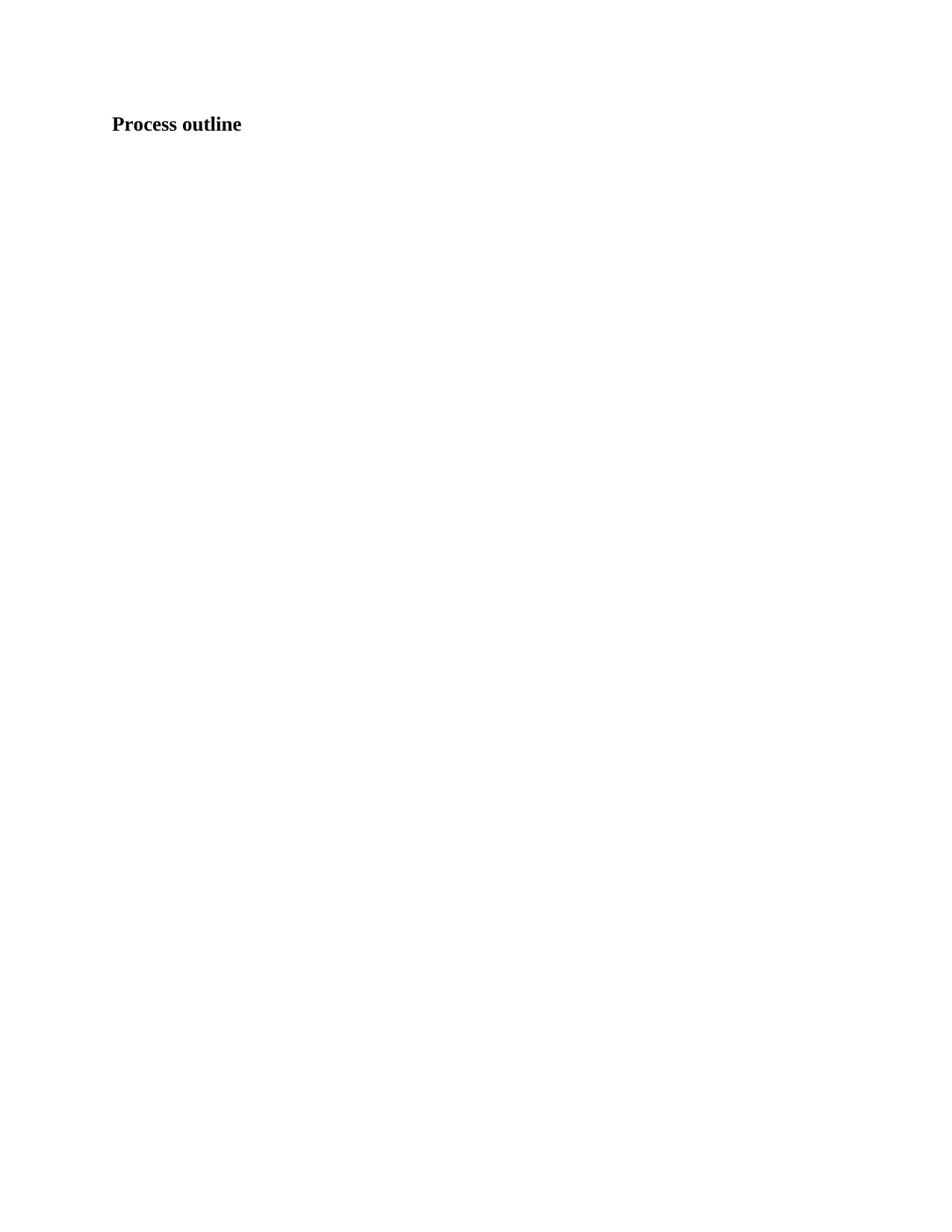
Process outline
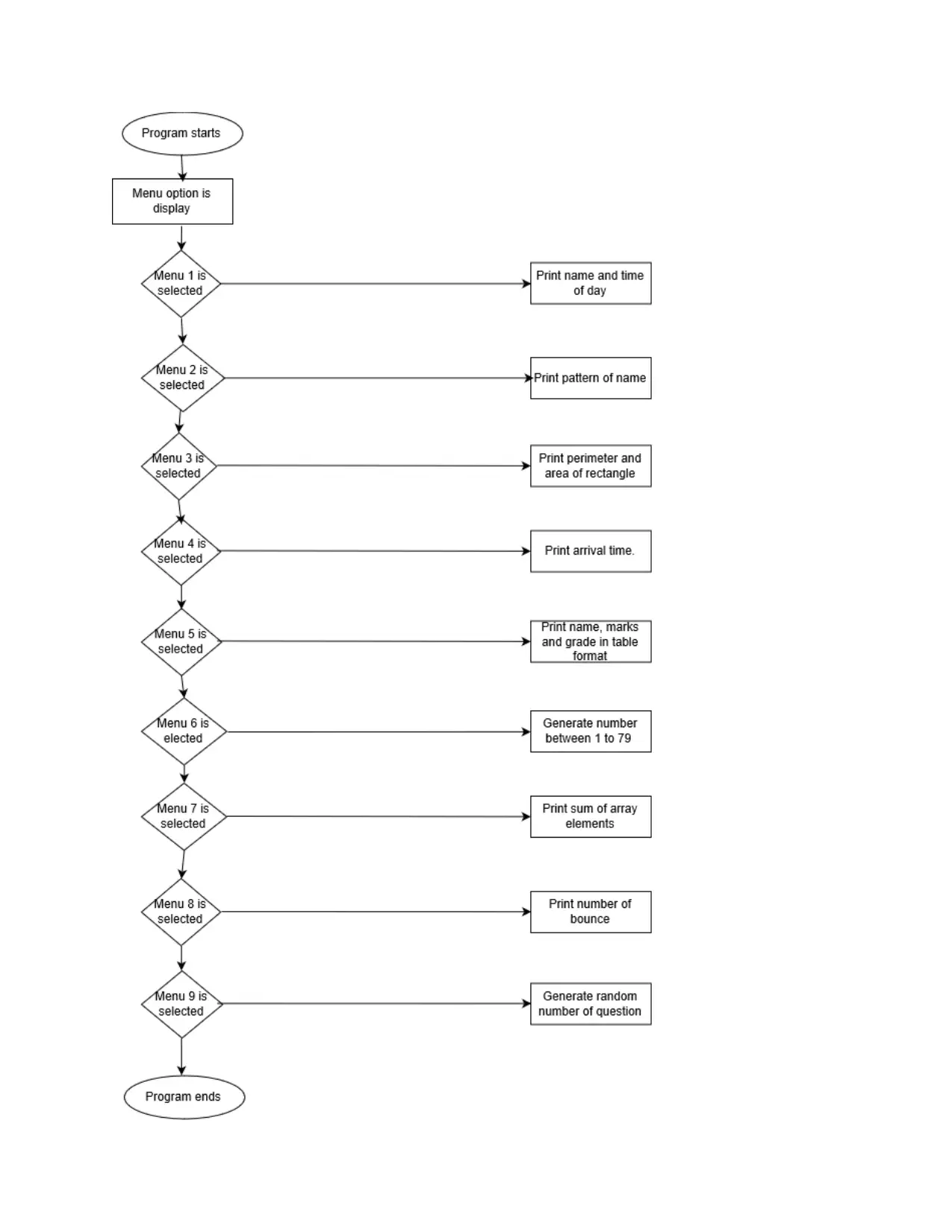
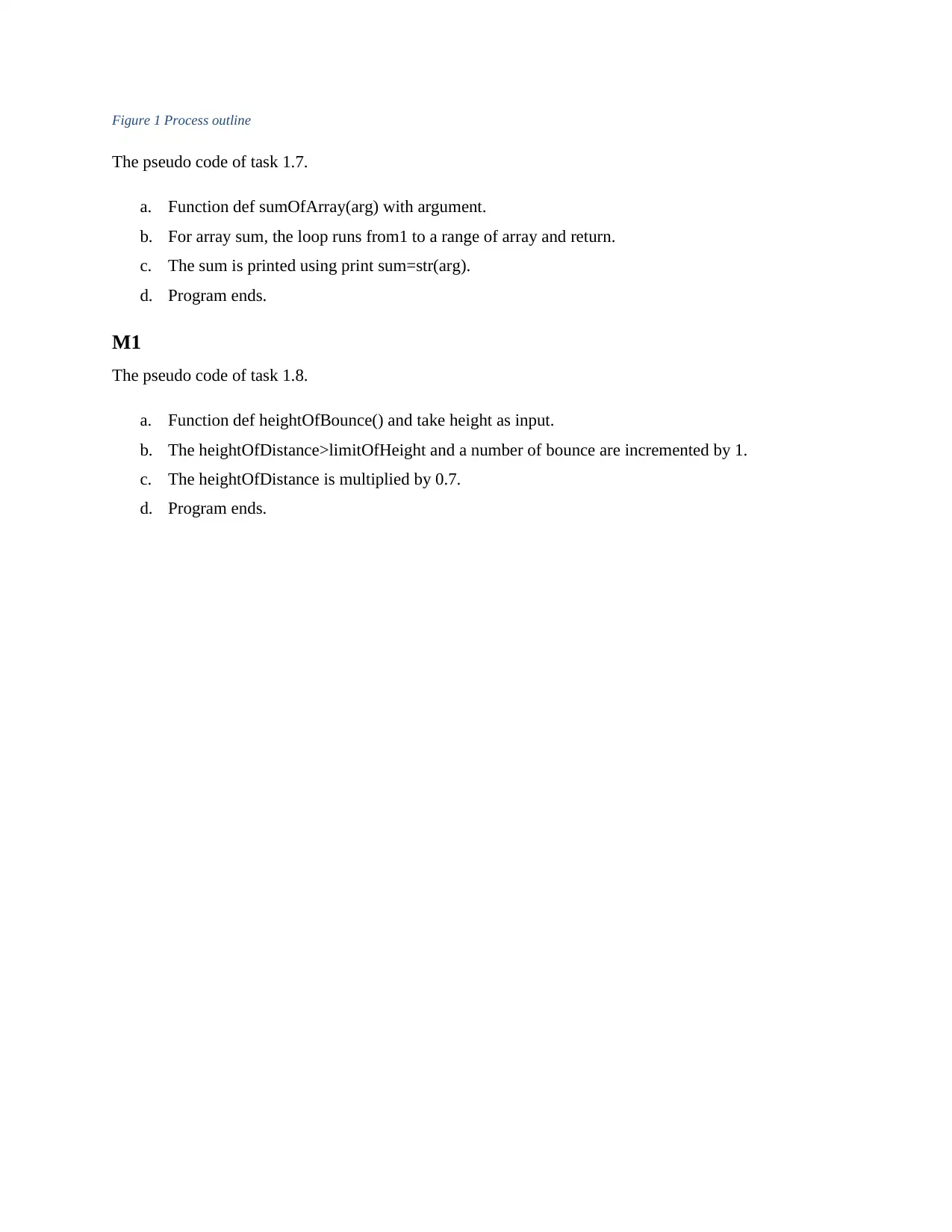
Figure 1 Process outline
The pseudo code of task 1.7.
a. Function def sumOfArray(arg) with argument.
b. For array sum, the loop runs from1 to a range of array and return.
c. The sum is printed using print sum=str(arg).
d. Program ends.
M1
The pseudo code of task 1.8.
a. Function def heightOfBounce() and take height as input.
b. The heightOfDistance>limitOfHeight and a number of bounce are incremented by 1.
c. The heightOfDistance is multiplied by 0.7.
d. Program ends.
The pseudo code of task 1.7.
a. Function def sumOfArray(arg) with argument.
b. For array sum, the loop runs from1 to a range of array and return.
c. The sum is printed using print sum=str(arg).
d. Program ends.
M1
The pseudo code of task 1.8.
a. Function def heightOfBounce() and take height as input.
b. The heightOfDistance>limitOfHeight and a number of bounce are incremented by 1.
c. The heightOfDistance is multiplied by 0.7.
d. Program ends.
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
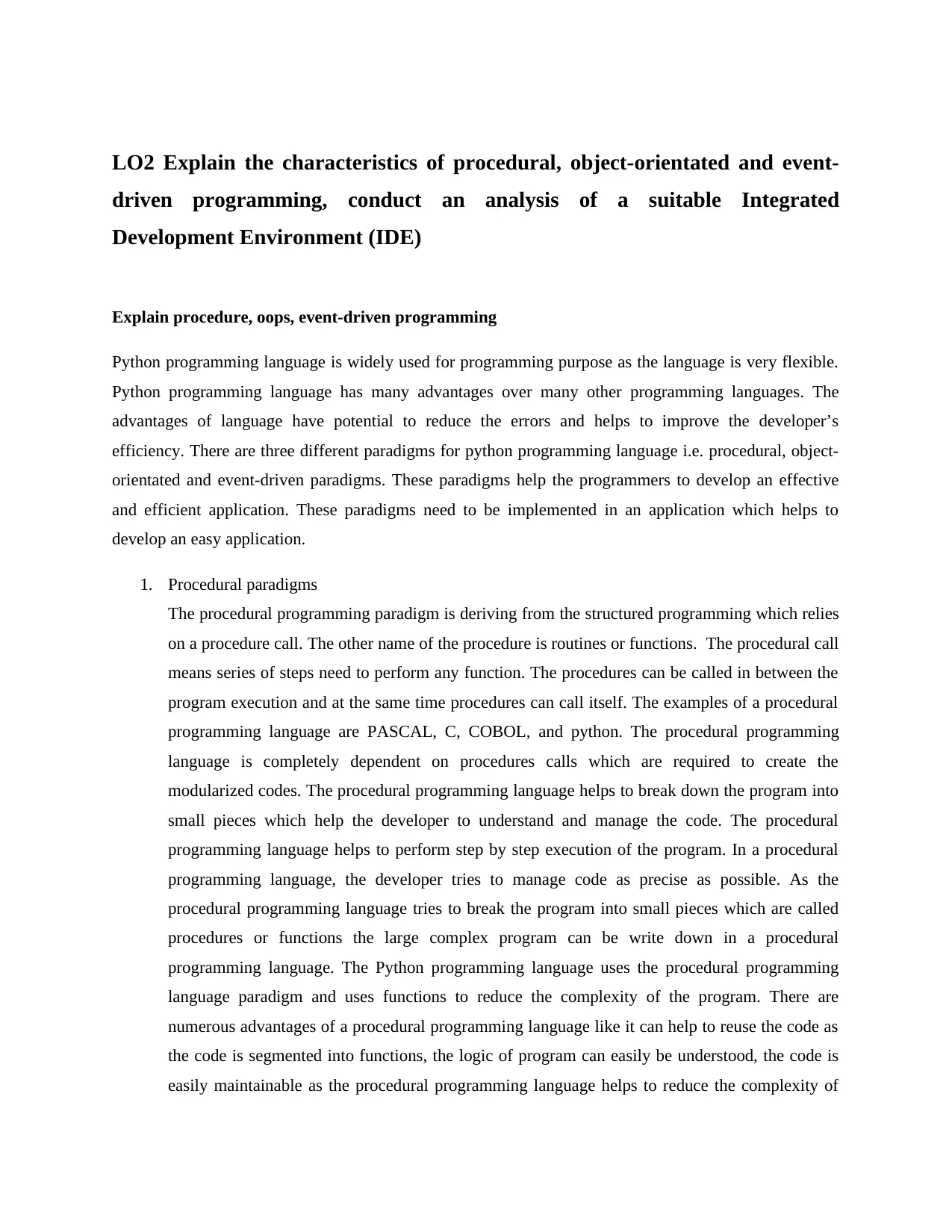
LO2 Explain the characteristics of procedural, object-orientated and event-
driven programming, conduct an analysis of a suitable Integrated
Development Environment (IDE)
Explain procedure, oops, event-driven programming
Python programming language is widely used for programming purpose as the language is very flexible.
Python programming language has many advantages over many other programming languages. The
advantages of language have potential to reduce the errors and helps to improve the developer’s
efficiency. There are three different paradigms for python programming language i.e. procedural, object-
orientated and event-driven paradigms. These paradigms help the programmers to develop an effective
and efficient application. These paradigms need to be implemented in an application which helps to
develop an easy application.
1. Procedural paradigms
The procedural programming paradigm is deriving from the structured programming which relies
on a procedure call. The other name of the procedure is routines or functions. The procedural call
means series of steps need to perform any function. The procedures can be called in between the
program execution and at the same time procedures can call itself. The examples of a procedural
programming language are PASCAL, C, COBOL, and python. The procedural programming
language is completely dependent on procedures calls which are required to create the
modularized codes. The procedural programming language helps to break down the program into
small pieces which help the developer to understand and manage the code. The procedural
programming language helps to perform step by step execution of the program. In a procedural
programming language, the developer tries to manage code as precise as possible. As the
procedural programming language tries to break the program into small pieces which are called
procedures or functions the large complex program can be write down in a procedural
programming language. The Python programming language uses the procedural programming
language paradigm and uses functions to reduce the complexity of the program. There are
numerous advantages of a procedural programming language like it can help to reuse the code as
the code is segmented into functions, the logic of program can easily be understood, the code is
easily maintainable as the procedural programming language helps to reduce the complexity of
driven programming, conduct an analysis of a suitable Integrated
Development Environment (IDE)
Explain procedure, oops, event-driven programming
Python programming language is widely used for programming purpose as the language is very flexible.
Python programming language has many advantages over many other programming languages. The
advantages of language have potential to reduce the errors and helps to improve the developer’s
efficiency. There are three different paradigms for python programming language i.e. procedural, object-
orientated and event-driven paradigms. These paradigms help the programmers to develop an effective
and efficient application. These paradigms need to be implemented in an application which helps to
develop an easy application.
1. Procedural paradigms
The procedural programming paradigm is deriving from the structured programming which relies
on a procedure call. The other name of the procedure is routines or functions. The procedural call
means series of steps need to perform any function. The procedures can be called in between the
program execution and at the same time procedures can call itself. The examples of a procedural
programming language are PASCAL, C, COBOL, and python. The procedural programming
language is completely dependent on procedures calls which are required to create the
modularized codes. The procedural programming language helps to break down the program into
small pieces which help the developer to understand and manage the code. The procedural
programming language helps to perform step by step execution of the program. In a procedural
programming language, the developer tries to manage code as precise as possible. As the
procedural programming language tries to break the program into small pieces which are called
procedures or functions the large complex program can be write down in a procedural
programming language. The Python programming language uses the procedural programming
language paradigm and uses functions to reduce the complexity of the program. There are
numerous advantages of a procedural programming language like it can help to reuse the code as
the code is segmented into functions, the logic of program can easily be understood, the code is
easily maintainable as the procedural programming language helps to reduce the complexity of
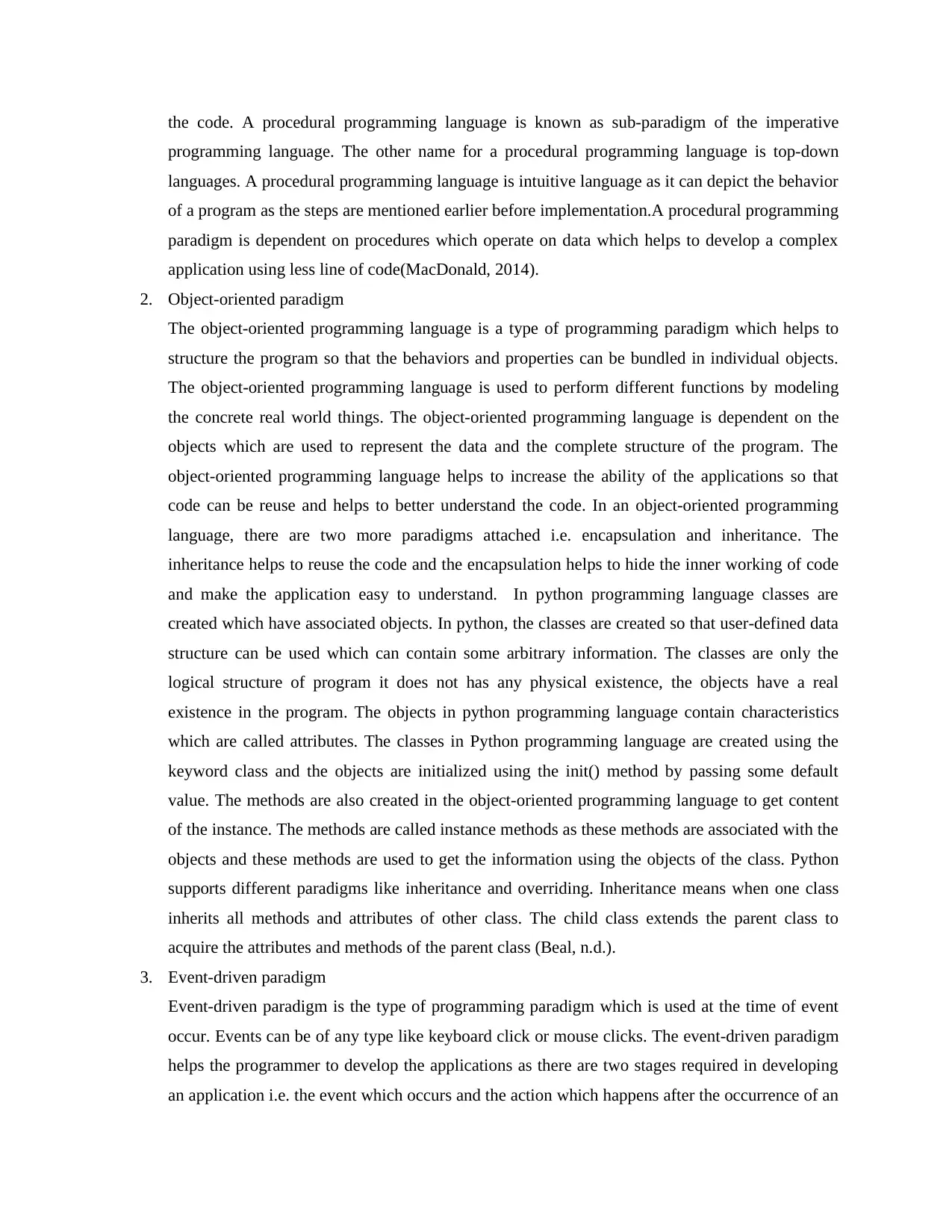
the code. A procedural programming language is known as sub-paradigm of the imperative
programming language. The other name for a procedural programming language is top-down
languages. A procedural programming language is intuitive language as it can depict the behavior
of a program as the steps are mentioned earlier before implementation.A procedural programming
paradigm is dependent on procedures which operate on data which helps to develop a complex
application using less line of code(MacDonald, 2014).
2. Object-oriented paradigm
The object-oriented programming language is a type of programming paradigm which helps to
structure the program so that the behaviors and properties can be bundled in individual objects.
The object-oriented programming language is used to perform different functions by modeling
the concrete real world things. The object-oriented programming language is dependent on the
objects which are used to represent the data and the complete structure of the program. The
object-oriented programming language helps to increase the ability of the applications so that
code can be reuse and helps to better understand the code. In an object-oriented programming
language, there are two more paradigms attached i.e. encapsulation and inheritance. The
inheritance helps to reuse the code and the encapsulation helps to hide the inner working of code
and make the application easy to understand. In python programming language classes are
created which have associated objects. In python, the classes are created so that user-defined data
structure can be used which can contain some arbitrary information. The classes are only the
logical structure of program it does not has any physical existence, the objects have a real
existence in the program. The objects in python programming language contain characteristics
which are called attributes. The classes in Python programming language are created using the
keyword class and the objects are initialized using the init() method by passing some default
value. The methods are also created in the object-oriented programming language to get content
of the instance. The methods are called instance methods as these methods are associated with the
objects and these methods are used to get the information using the objects of the class. Python
supports different paradigms like inheritance and overriding. Inheritance means when one class
inherits all methods and attributes of other class. The child class extends the parent class to
acquire the attributes and methods of the parent class (Beal, n.d.).
3. Event-driven paradigm
Event-driven paradigm is the type of programming paradigm which is used at the time of event
occur. Events can be of any type like keyboard click or mouse clicks. The event-driven paradigm
helps the programmer to develop the applications as there are two stages required in developing
an application i.e. the event which occurs and the action which happens after the occurrence of an
programming language. The other name for a procedural programming language is top-down
languages. A procedural programming language is intuitive language as it can depict the behavior
of a program as the steps are mentioned earlier before implementation.A procedural programming
paradigm is dependent on procedures which operate on data which helps to develop a complex
application using less line of code(MacDonald, 2014).
2. Object-oriented paradigm
The object-oriented programming language is a type of programming paradigm which helps to
structure the program so that the behaviors and properties can be bundled in individual objects.
The object-oriented programming language is used to perform different functions by modeling
the concrete real world things. The object-oriented programming language is dependent on the
objects which are used to represent the data and the complete structure of the program. The
object-oriented programming language helps to increase the ability of the applications so that
code can be reuse and helps to better understand the code. In an object-oriented programming
language, there are two more paradigms attached i.e. encapsulation and inheritance. The
inheritance helps to reuse the code and the encapsulation helps to hide the inner working of code
and make the application easy to understand. In python programming language classes are
created which have associated objects. In python, the classes are created so that user-defined data
structure can be used which can contain some arbitrary information. The classes are only the
logical structure of program it does not has any physical existence, the objects have a real
existence in the program. The objects in python programming language contain characteristics
which are called attributes. The classes in Python programming language are created using the
keyword class and the objects are initialized using the init() method by passing some default
value. The methods are also created in the object-oriented programming language to get content
of the instance. The methods are called instance methods as these methods are associated with the
objects and these methods are used to get the information using the objects of the class. Python
supports different paradigms like inheritance and overriding. Inheritance means when one class
inherits all methods and attributes of other class. The child class extends the parent class to
acquire the attributes and methods of the parent class (Beal, n.d.).
3. Event-driven paradigm
Event-driven paradigm is the type of programming paradigm which is used at the time of event
occur. Events can be of any type like keyboard click or mouse clicks. The event-driven paradigm
helps the programmer to develop the applications as there are two stages required in developing
an application i.e. the event which occurs and the action which happens after the occurrence of an
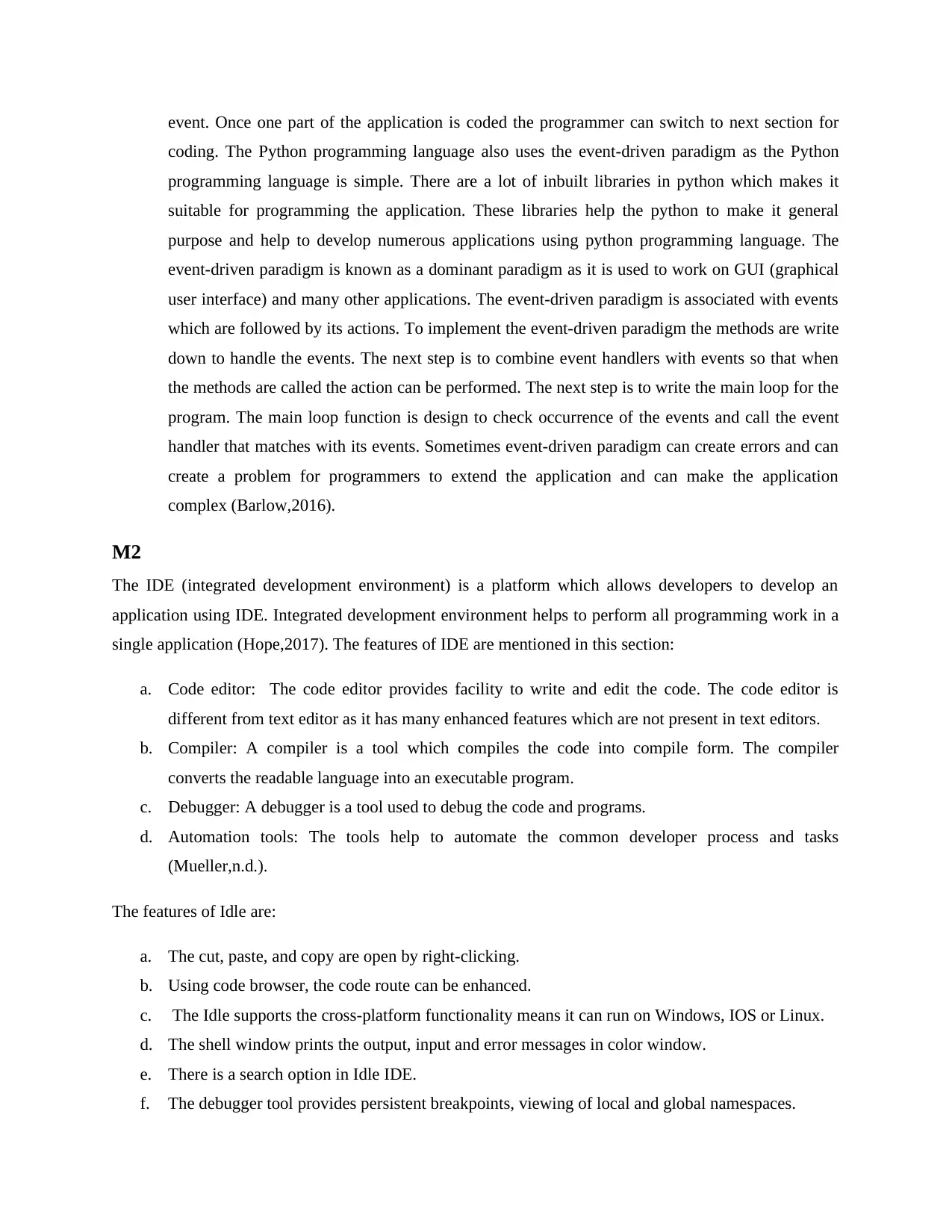
event. Once one part of the application is coded the programmer can switch to next section for
coding. The Python programming language also uses the event-driven paradigm as the Python
programming language is simple. There are a lot of inbuilt libraries in python which makes it
suitable for programming the application. These libraries help the python to make it general
purpose and help to develop numerous applications using python programming language. The
event-driven paradigm is known as a dominant paradigm as it is used to work on GUI (graphical
user interface) and many other applications. The event-driven paradigm is associated with events
which are followed by its actions. To implement the event-driven paradigm the methods are write
down to handle the events. The next step is to combine event handlers with events so that when
the methods are called the action can be performed. The next step is to write the main loop for the
program. The main loop function is design to check occurrence of the events and call the event
handler that matches with its events. Sometimes event-driven paradigm can create errors and can
create a problem for programmers to extend the application and can make the application
complex (Barlow,2016).
M2
The IDE (integrated development environment) is a platform which allows developers to develop an
application using IDE. Integrated development environment helps to perform all programming work in a
single application (Hope,2017). The features of IDE are mentioned in this section:
a. Code editor: The code editor provides facility to write and edit the code. The code editor is
different from text editor as it has many enhanced features which are not present in text editors.
b. Compiler: A compiler is a tool which compiles the code into compile form. The compiler
converts the readable language into an executable program.
c. Debugger: A debugger is a tool used to debug the code and programs.
d. Automation tools: The tools help to automate the common developer process and tasks
(Mueller,n.d.).
The features of Idle are:
a. The cut, paste, and copy are open by right-clicking.
b. Using code browser, the code route can be enhanced.
c. The Idle supports the cross-platform functionality means it can run on Windows, IOS or Linux.
d. The shell window prints the output, input and error messages in color window.
e. There is a search option in Idle IDE.
f. The debugger tool provides persistent breakpoints, viewing of local and global namespaces.
coding. The Python programming language also uses the event-driven paradigm as the Python
programming language is simple. There are a lot of inbuilt libraries in python which makes it
suitable for programming the application. These libraries help the python to make it general
purpose and help to develop numerous applications using python programming language. The
event-driven paradigm is known as a dominant paradigm as it is used to work on GUI (graphical
user interface) and many other applications. The event-driven paradigm is associated with events
which are followed by its actions. To implement the event-driven paradigm the methods are write
down to handle the events. The next step is to combine event handlers with events so that when
the methods are called the action can be performed. The next step is to write the main loop for the
program. The main loop function is design to check occurrence of the events and call the event
handler that matches with its events. Sometimes event-driven paradigm can create errors and can
create a problem for programmers to extend the application and can make the application
complex (Barlow,2016).
M2
The IDE (integrated development environment) is a platform which allows developers to develop an
application using IDE. Integrated development environment helps to perform all programming work in a
single application (Hope,2017). The features of IDE are mentioned in this section:
a. Code editor: The code editor provides facility to write and edit the code. The code editor is
different from text editor as it has many enhanced features which are not present in text editors.
b. Compiler: A compiler is a tool which compiles the code into compile form. The compiler
converts the readable language into an executable program.
c. Debugger: A debugger is a tool used to debug the code and programs.
d. Automation tools: The tools help to automate the common developer process and tasks
(Mueller,n.d.).
The features of Idle are:
a. The cut, paste, and copy are open by right-clicking.
b. Using code browser, the code route can be enhanced.
c. The Idle supports the cross-platform functionality means it can run on Windows, IOS or Linux.
d. The shell window prints the output, input and error messages in color window.
e. There is a search option in Idle IDE.
f. The debugger tool provides persistent breakpoints, viewing of local and global namespaces.
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
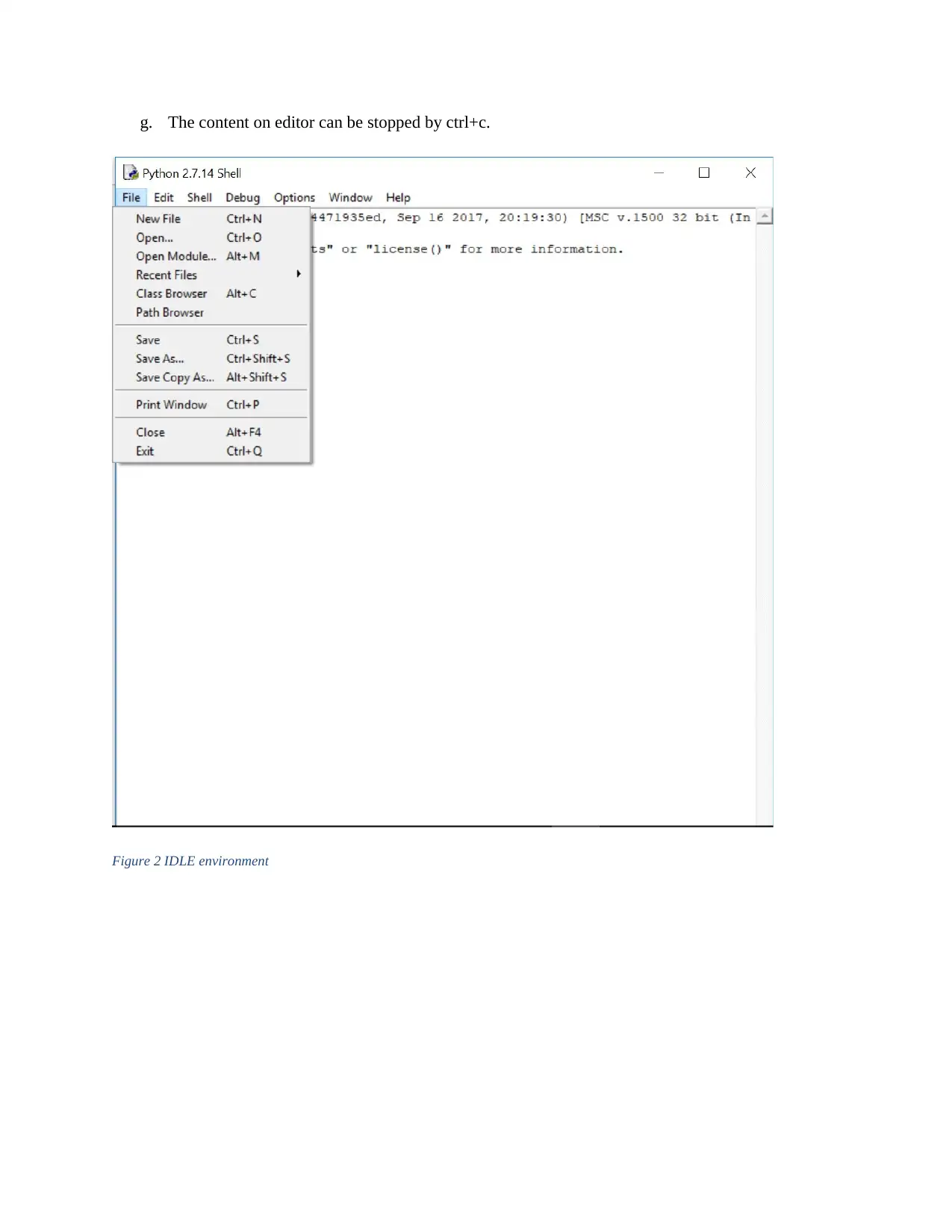
g. The content on editor can be stopped by ctrl+c.
Figure 2 IDLE environment
Figure 2 IDLE environment
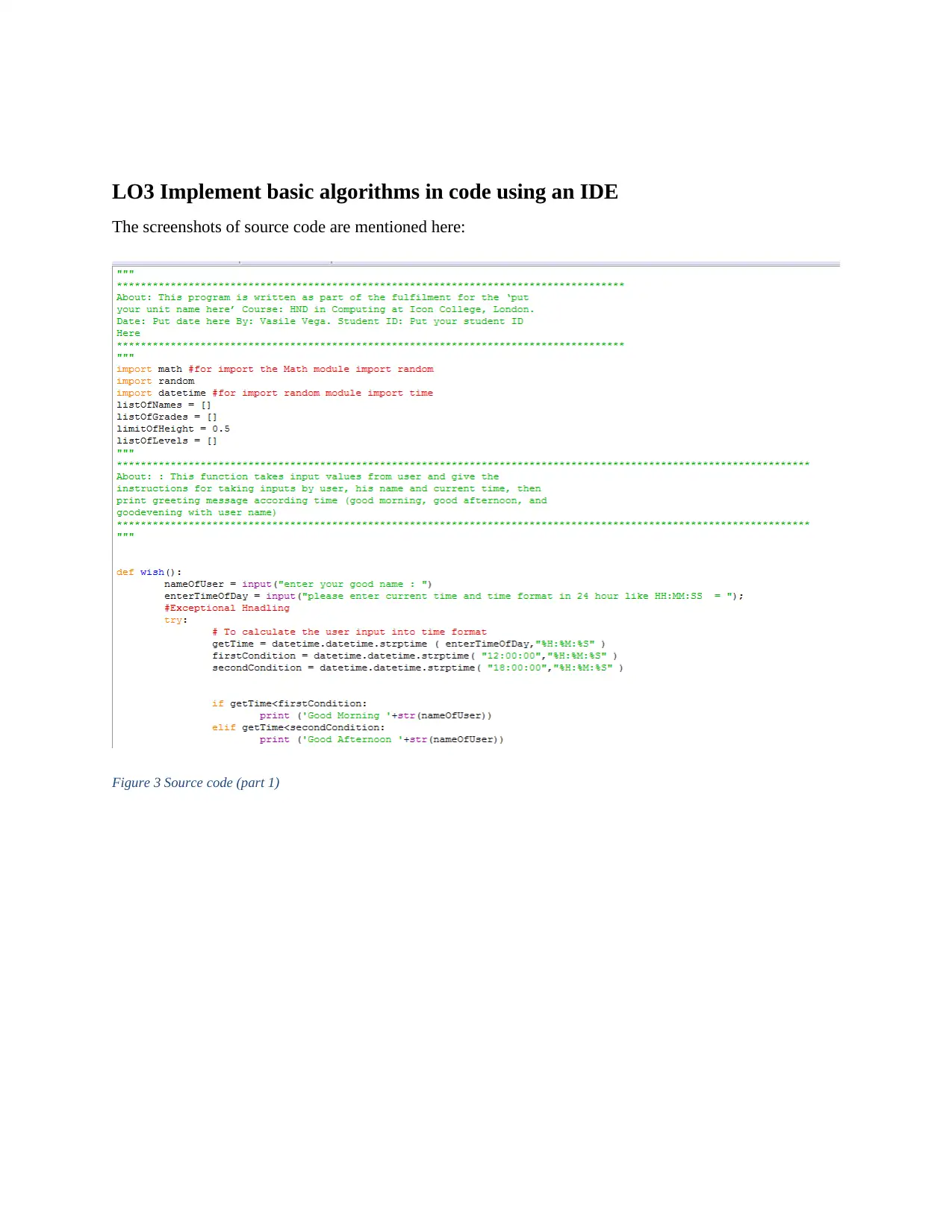
LO3 Implement basic algorithms in code using an IDE
The screenshots of source code are mentioned here:
Figure 3 Source code (part 1)
The screenshots of source code are mentioned here:
Figure 3 Source code (part 1)
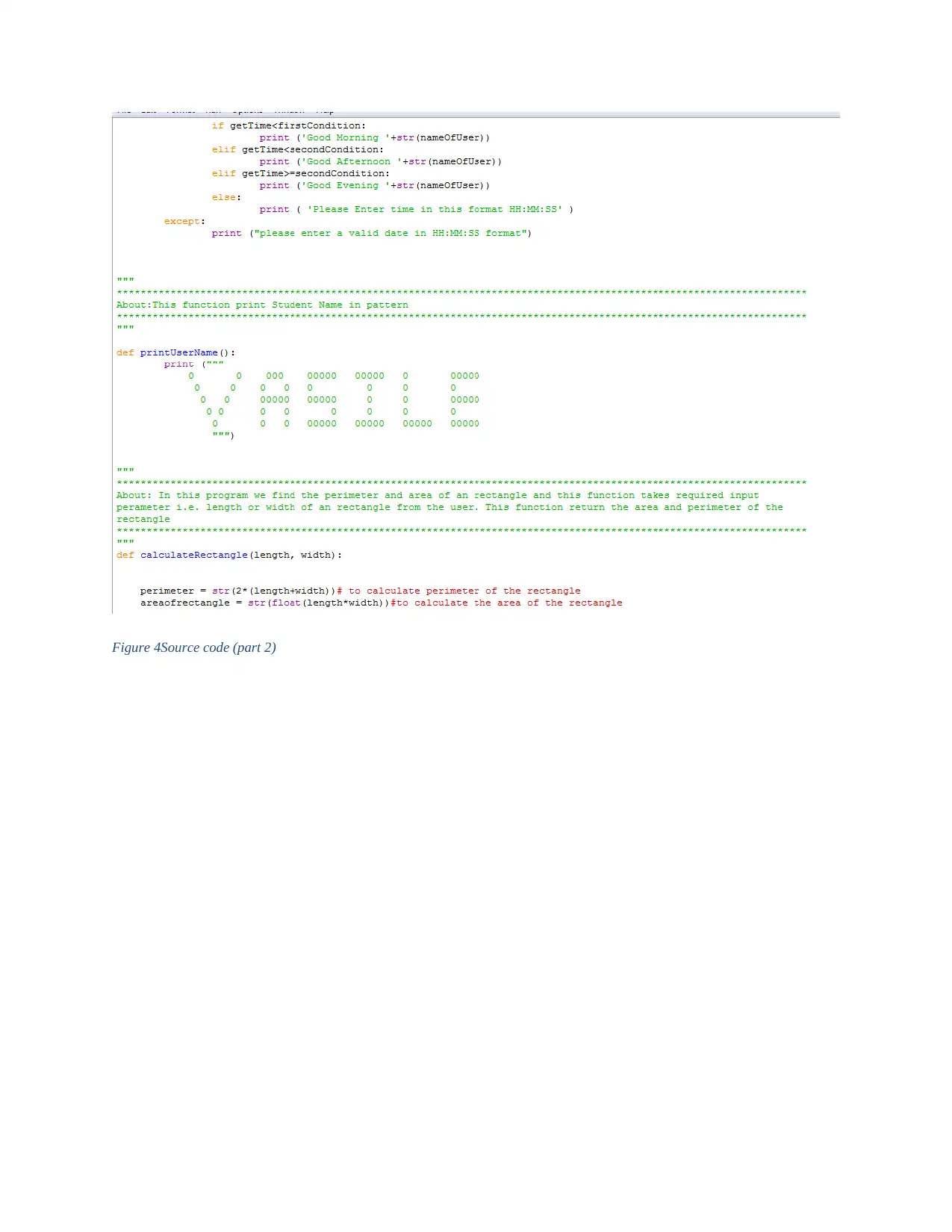
Figure 4Source code (part 2)
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
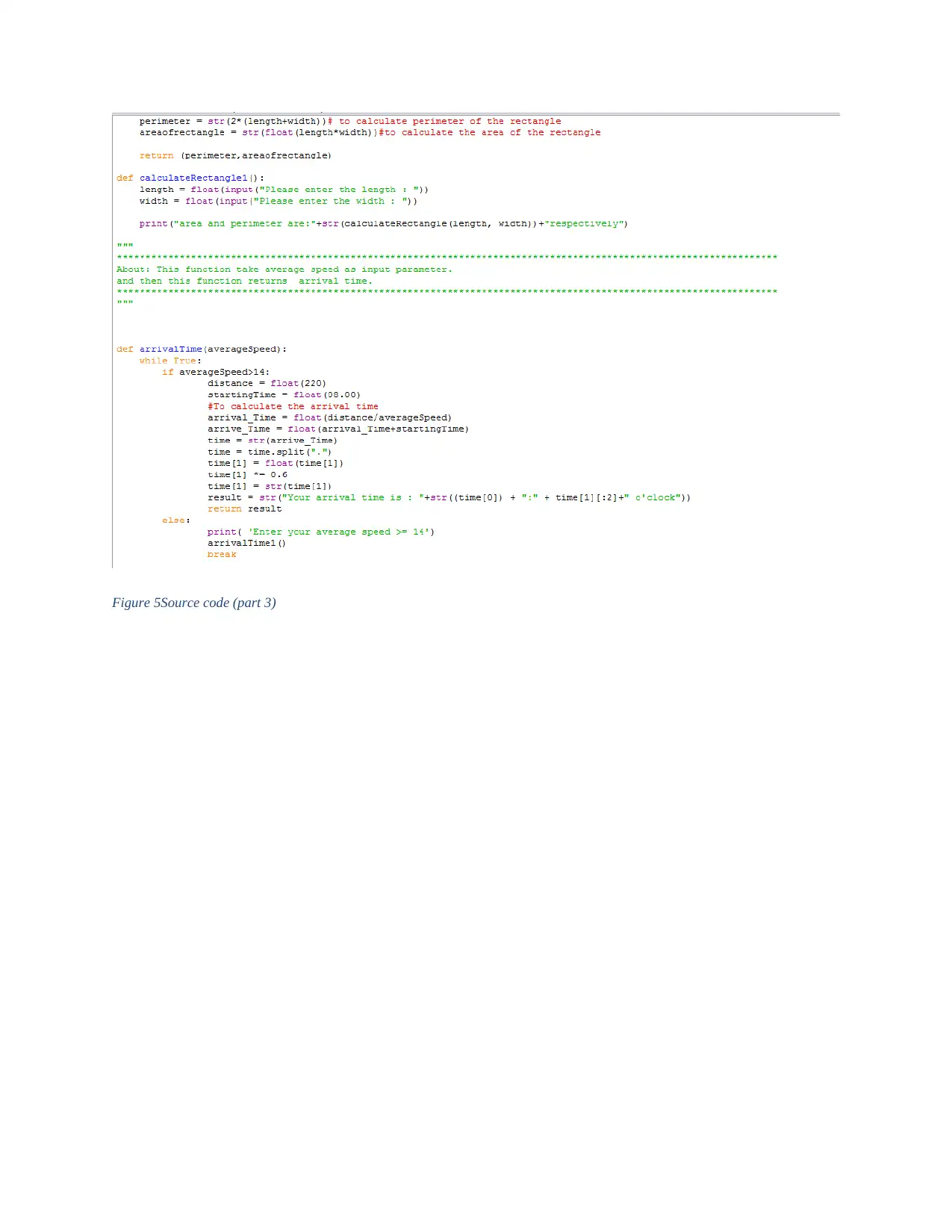
Figure 5Source code (part 3)
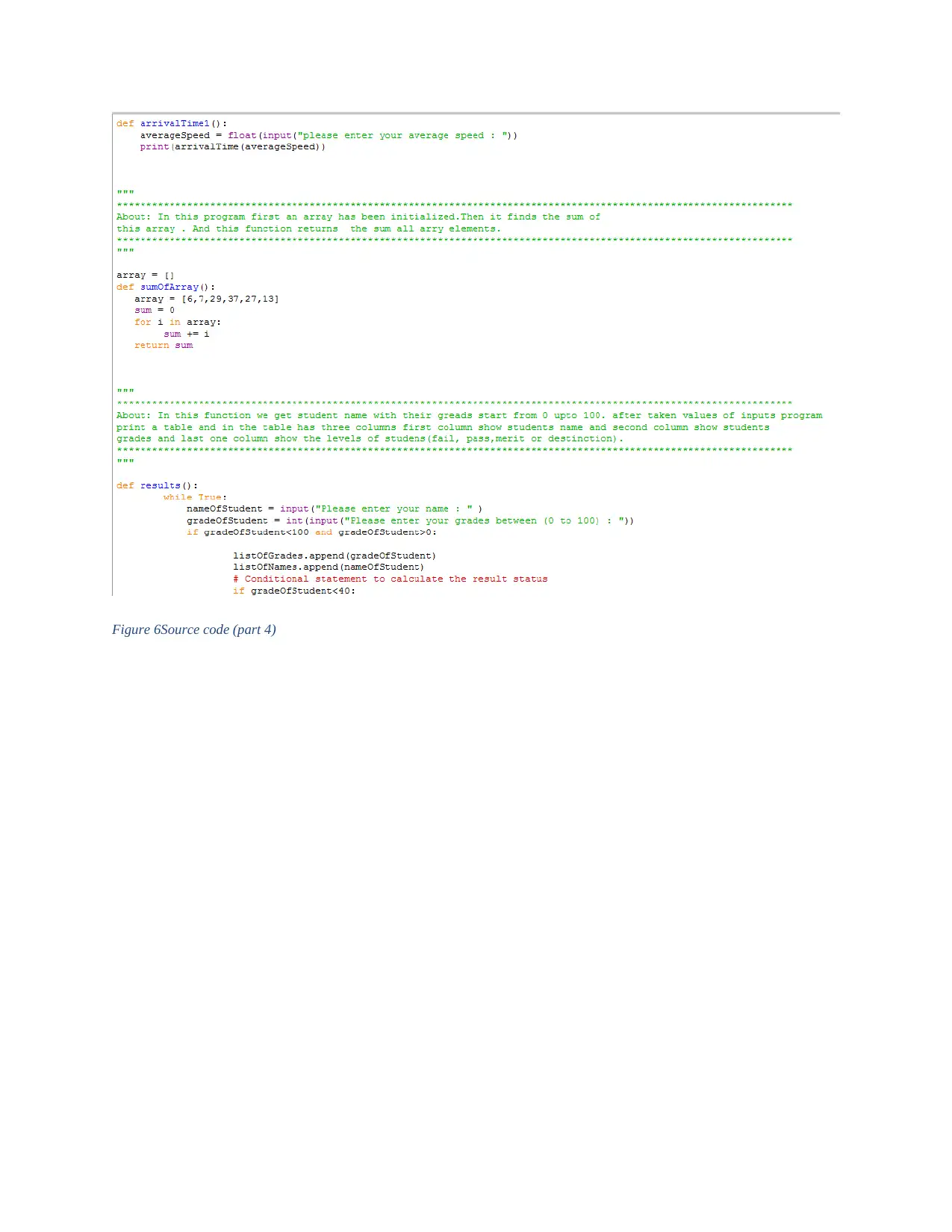
Figure 6Source code (part 4)
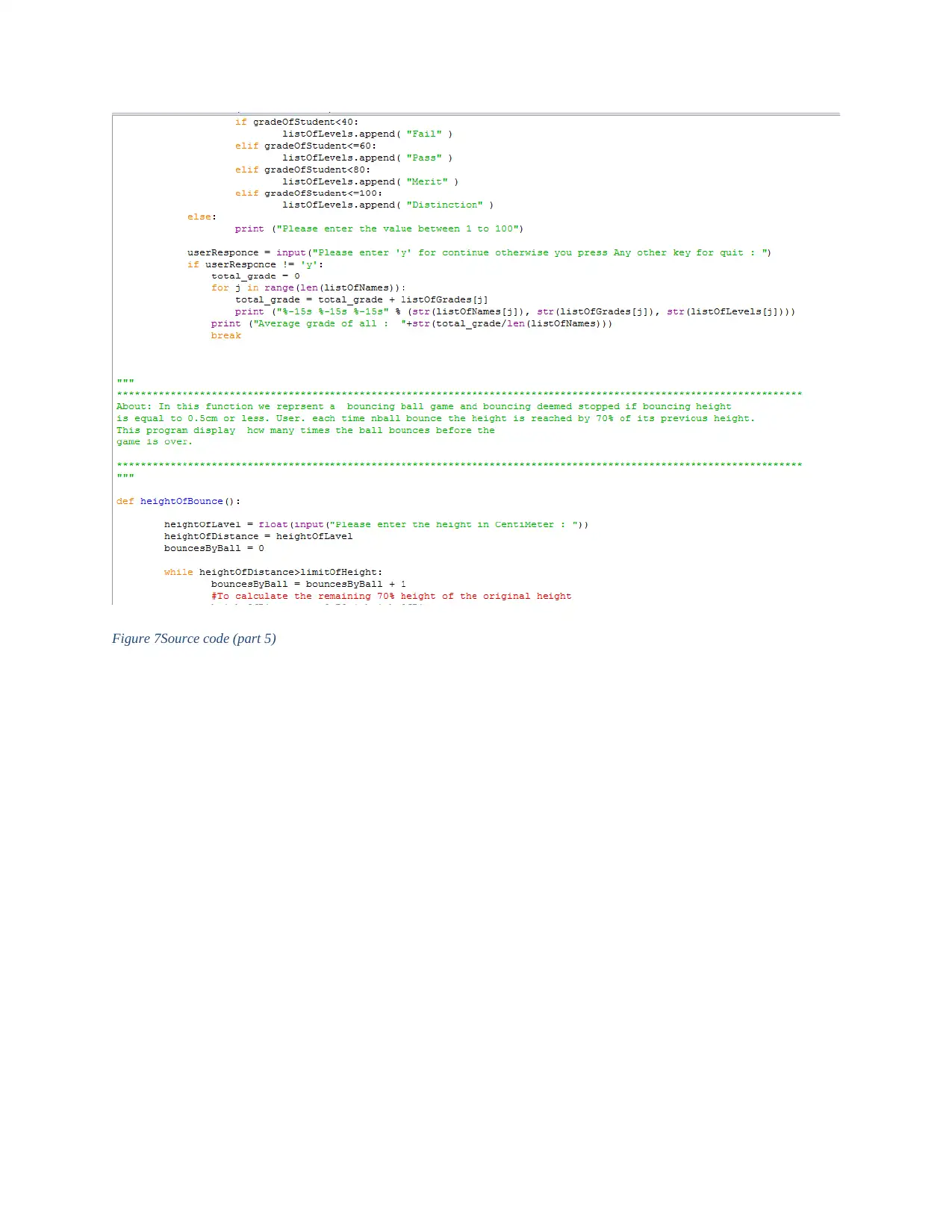
Figure 7Source code (part 5)
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
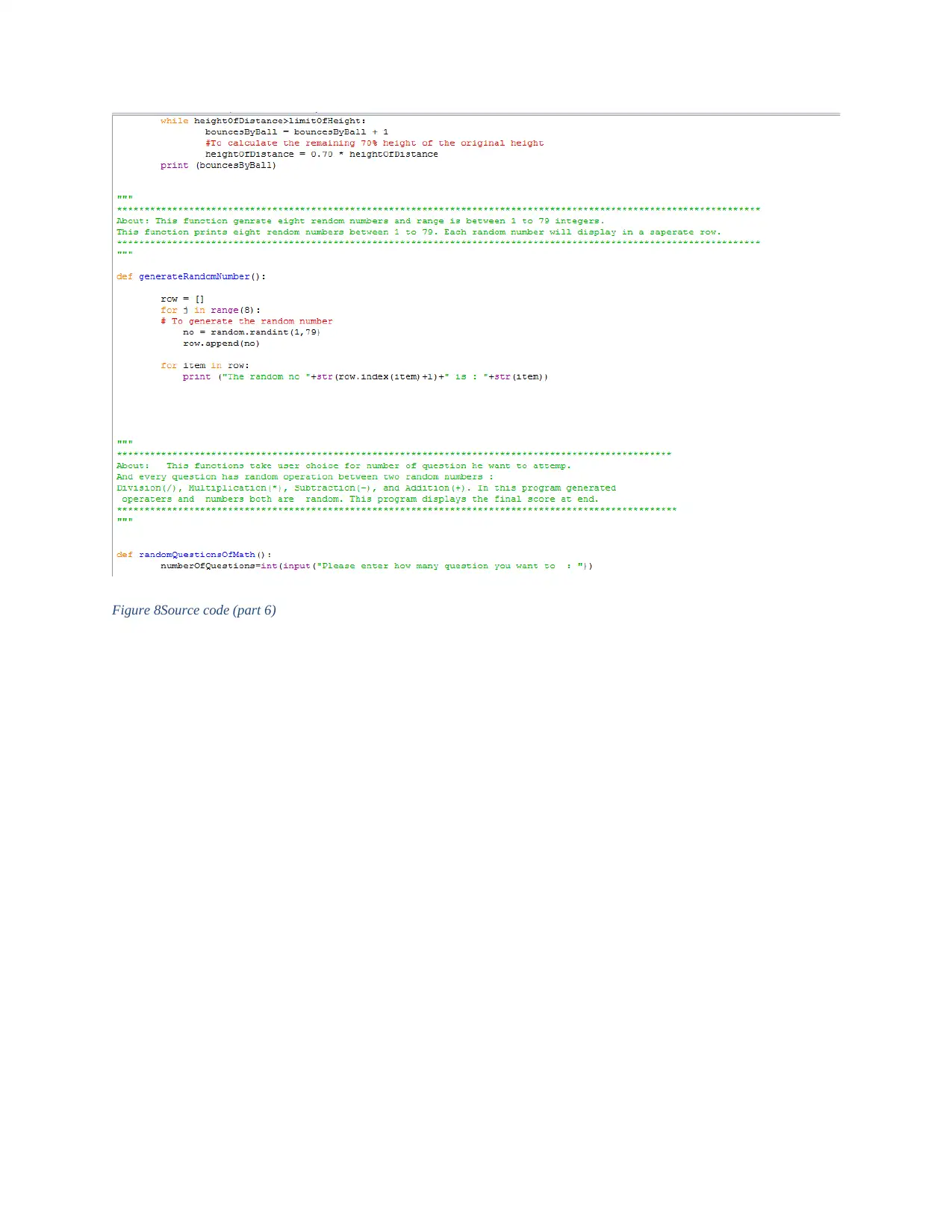
Figure 8Source code (part 6)
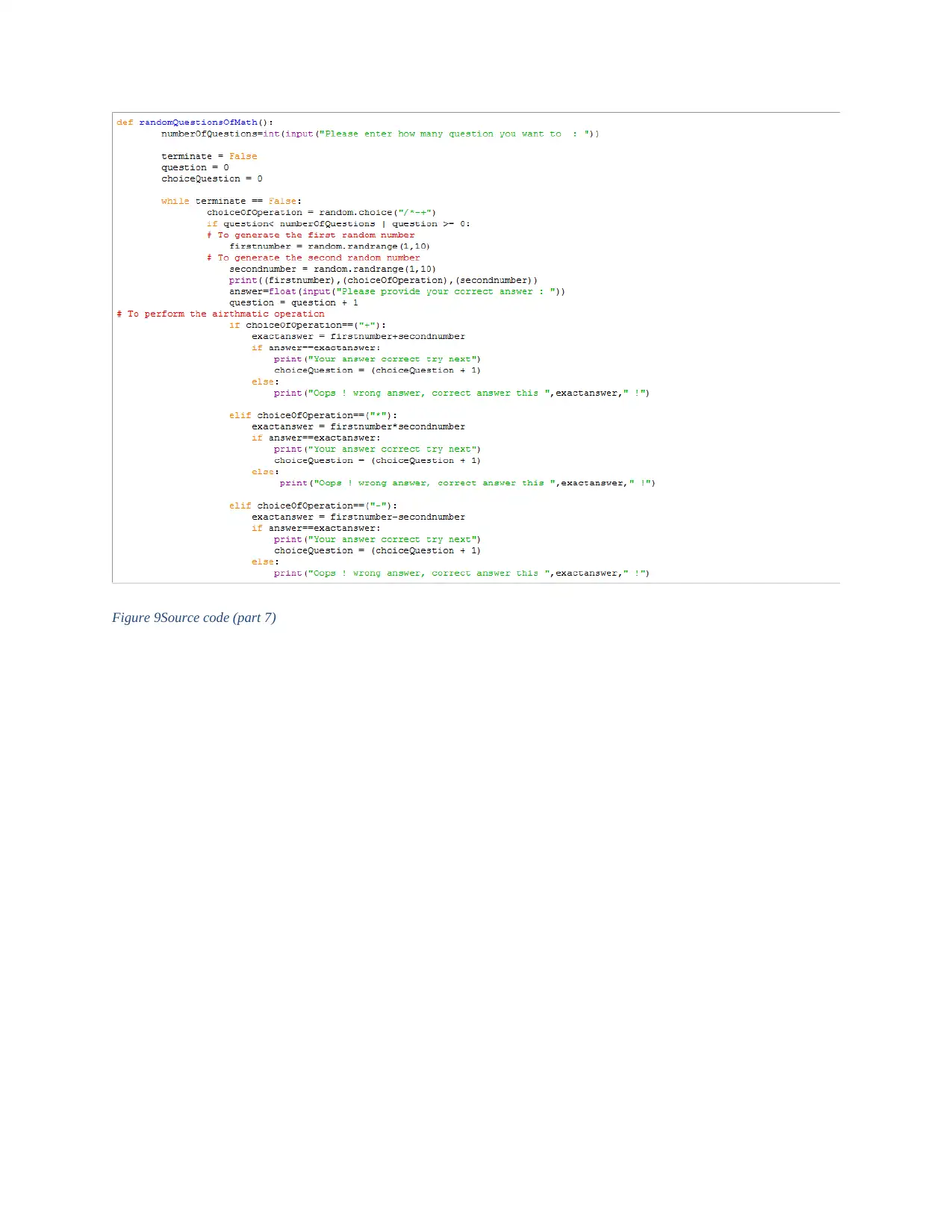
Figure 9Source code (part 7)
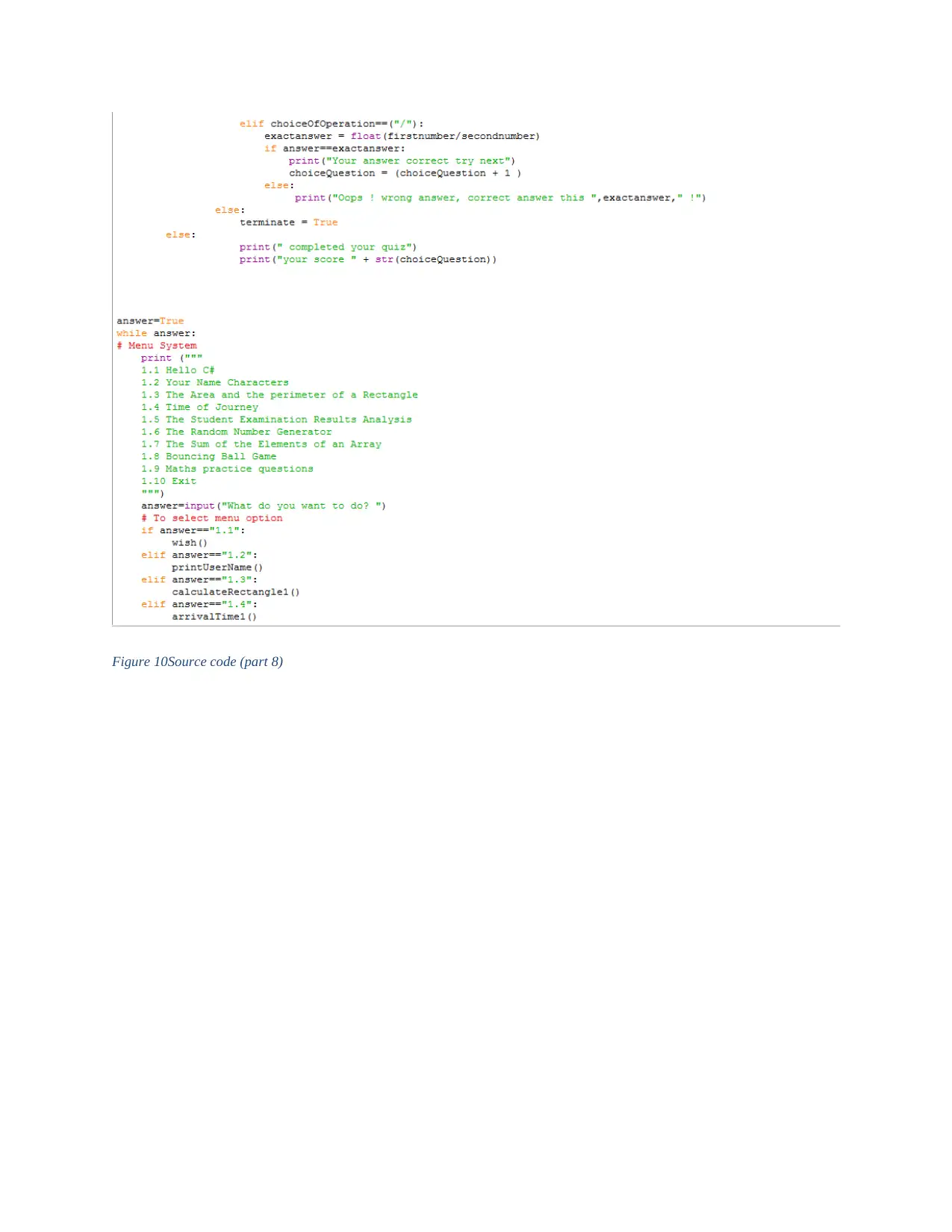
Figure 10Source code (part 8)
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
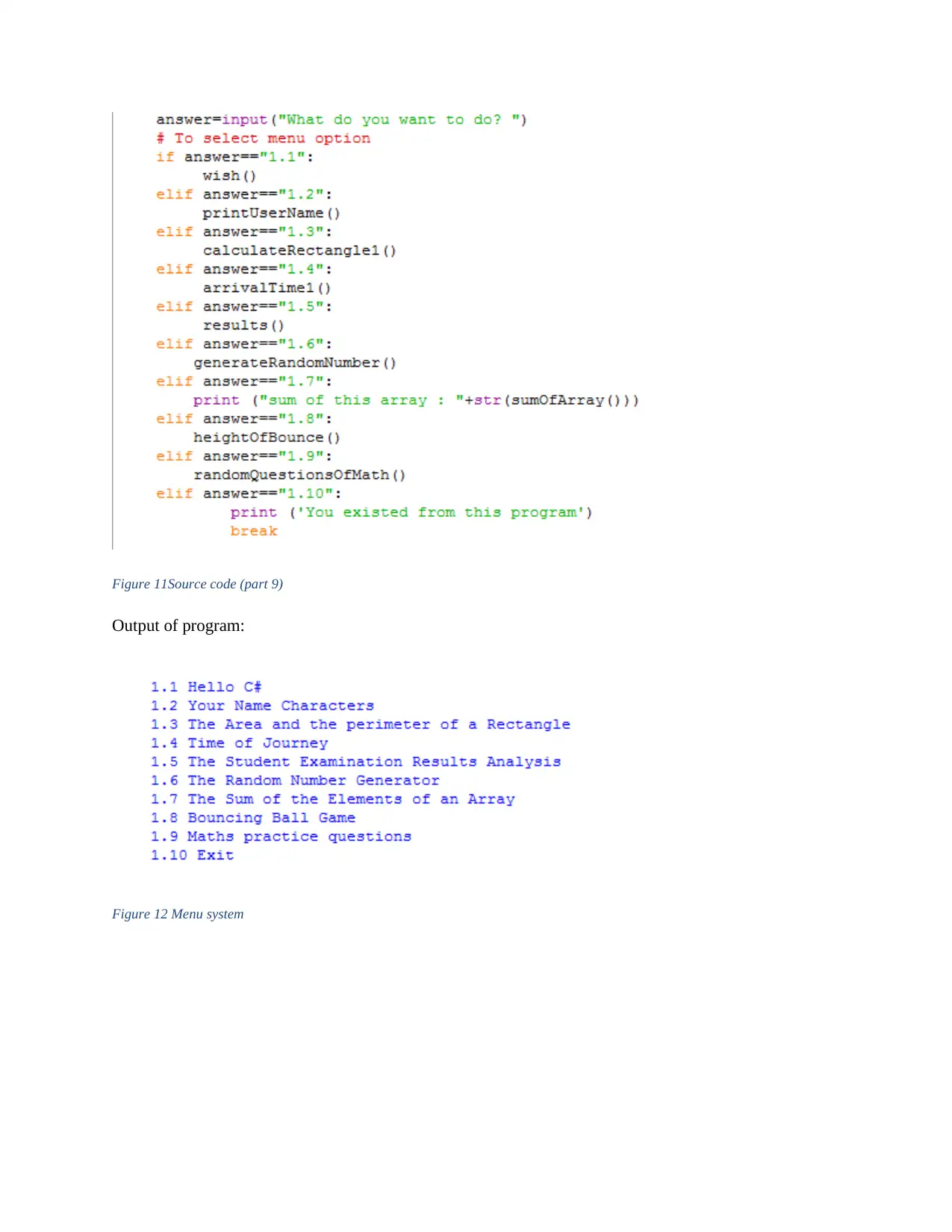
Figure 11Source code (part 9)
Output of program:
Figure 12 Menu system
Output of program:
Figure 12 Menu system
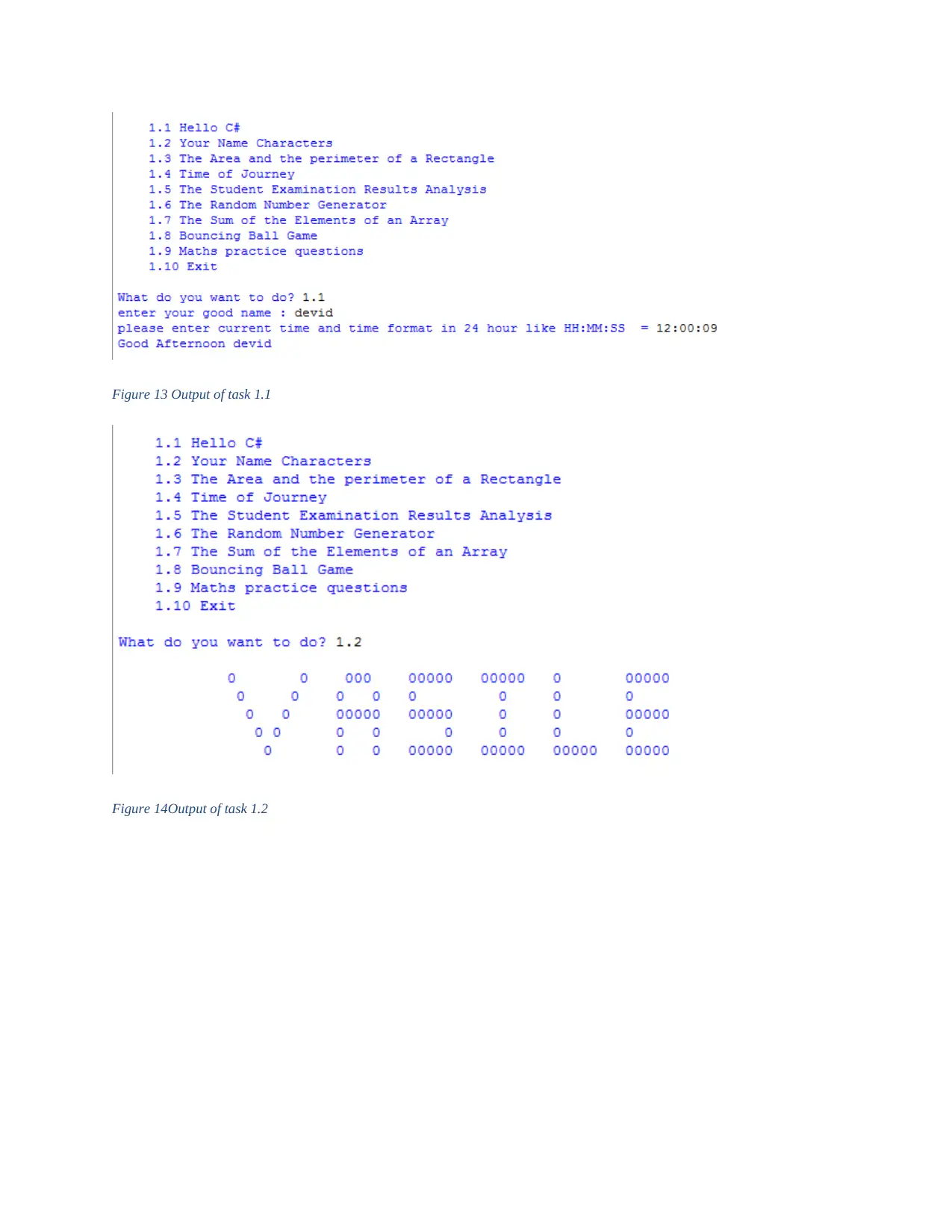
Figure 13 Output of task 1.1
Figure 14Output of task 1.2
Figure 14Output of task 1.2
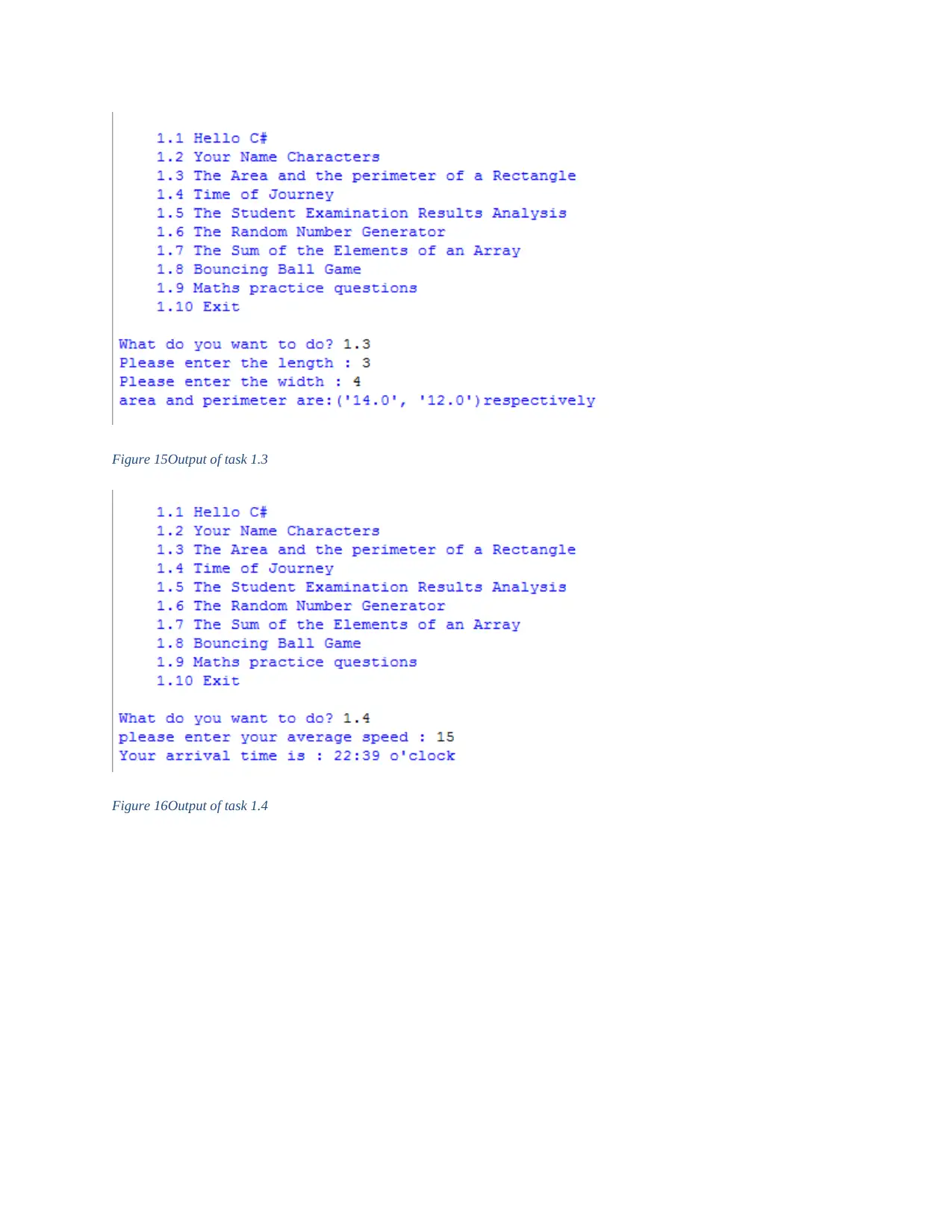
Figure 15Output of task 1.3
Figure 16Output of task 1.4
Figure 16Output of task 1.4
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
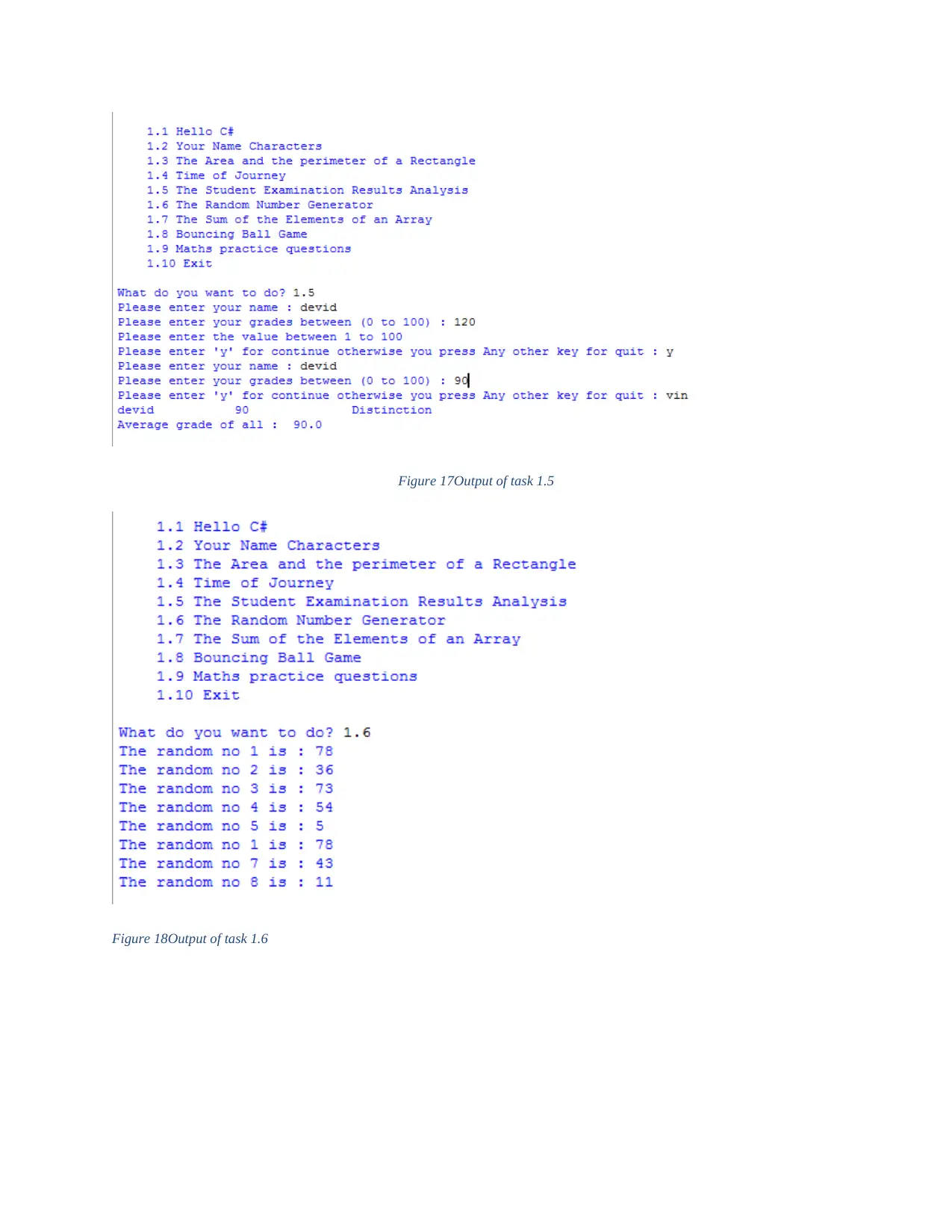
Figure 17Output of task 1.5
Figure 18Output of task 1.6
Figure 18Output of task 1.6
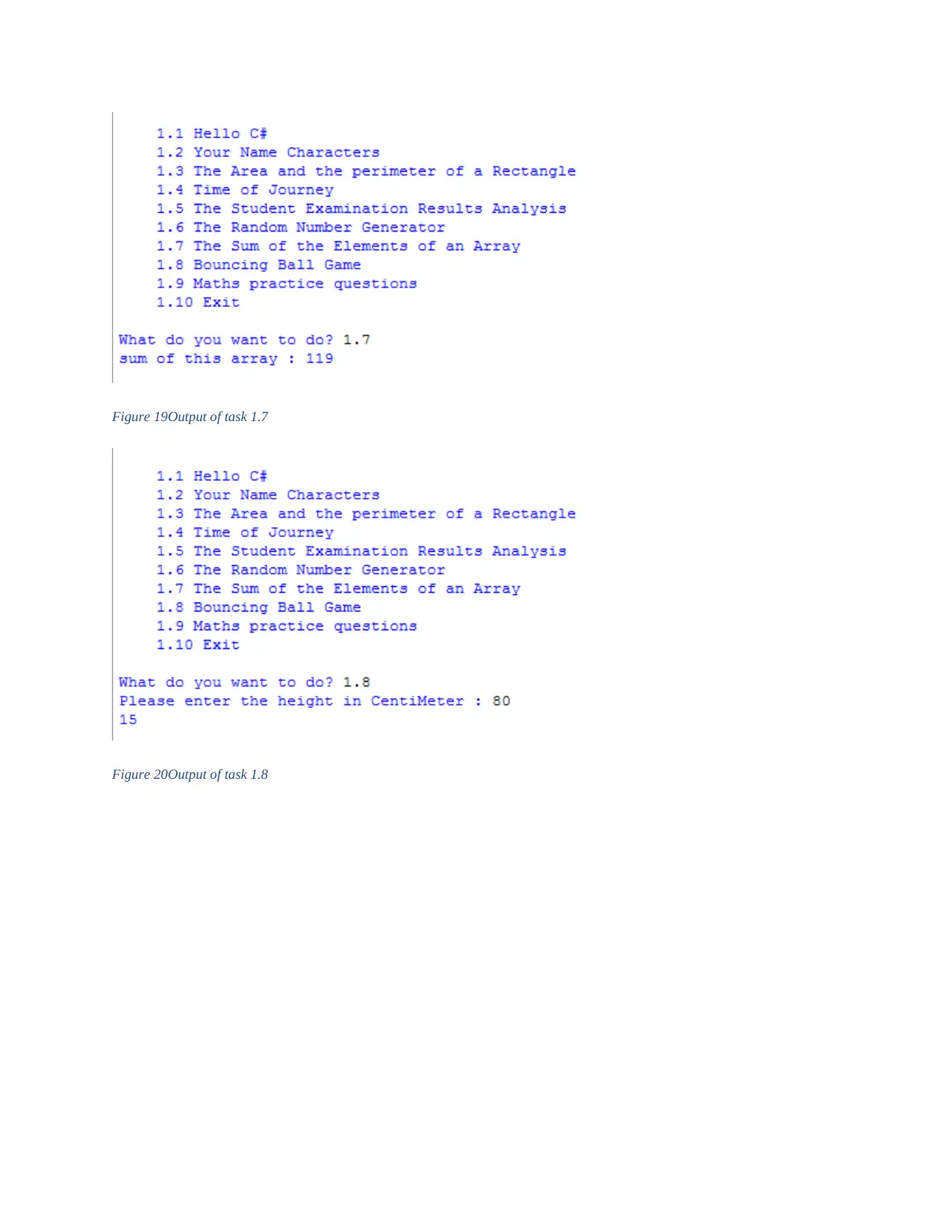
Figure 19Output of task 1.7
Figure 20Output of task 1.8
Figure 20Output of task 1.8
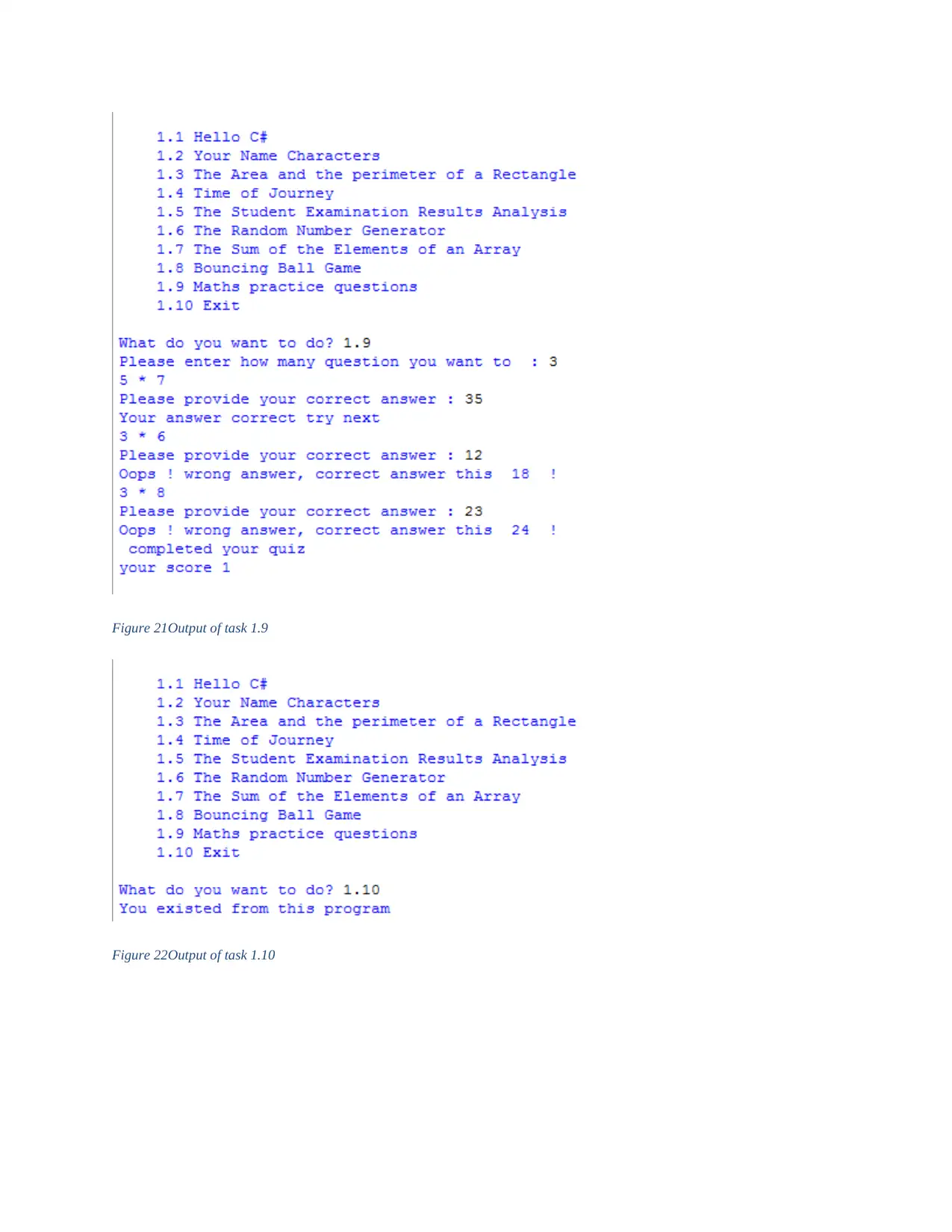
Figure 21Output of task 1.9
Figure 22Output of task 1.10
Figure 22Output of task 1.10
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
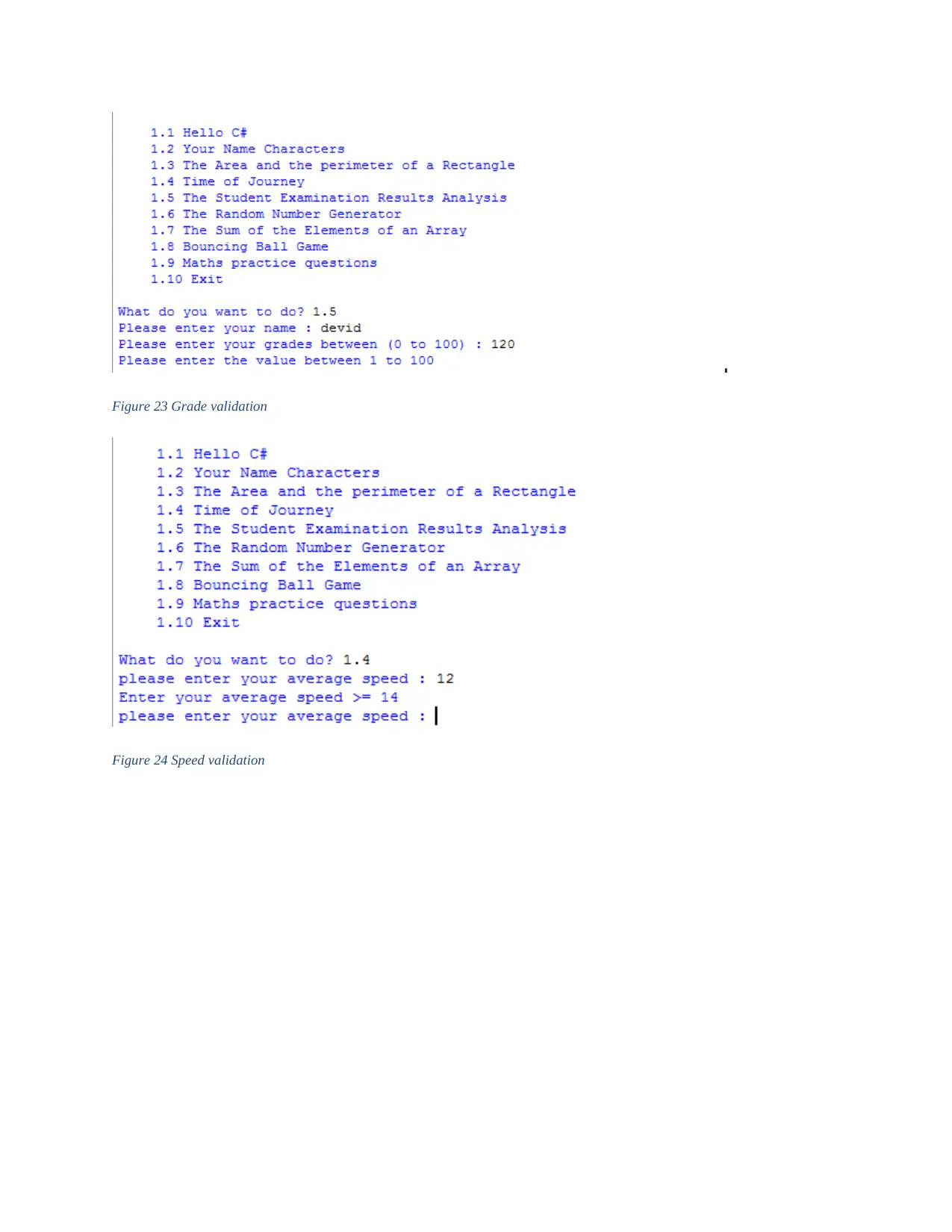
Figure 23 Grade validation
Figure 24 Speed validation
Figure 24 Speed validation
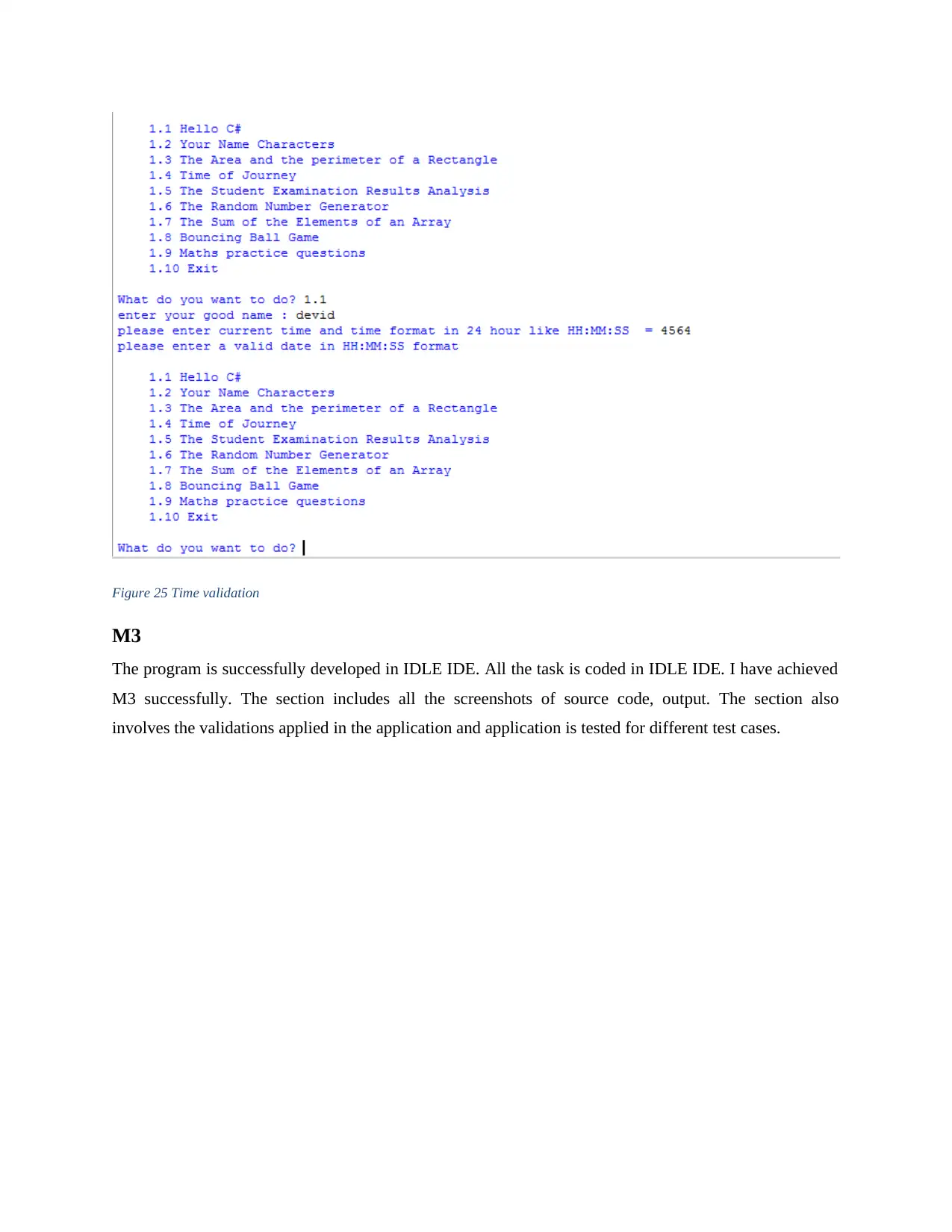
Figure 25 Time validation
M3
The program is successfully developed in IDLE IDE. All the task is coded in IDLE IDE. I have achieved
M3 successfully. The section includes all the screenshots of source code, output. The section also
involves the validations applied in the application and application is tested for different test cases.
M3
The program is successfully developed in IDLE IDE. All the task is coded in IDLE IDE. I have achieved
M3 successfully. The section includes all the screenshots of source code, output. The section also
involves the validations applied in the application and application is tested for different test cases.
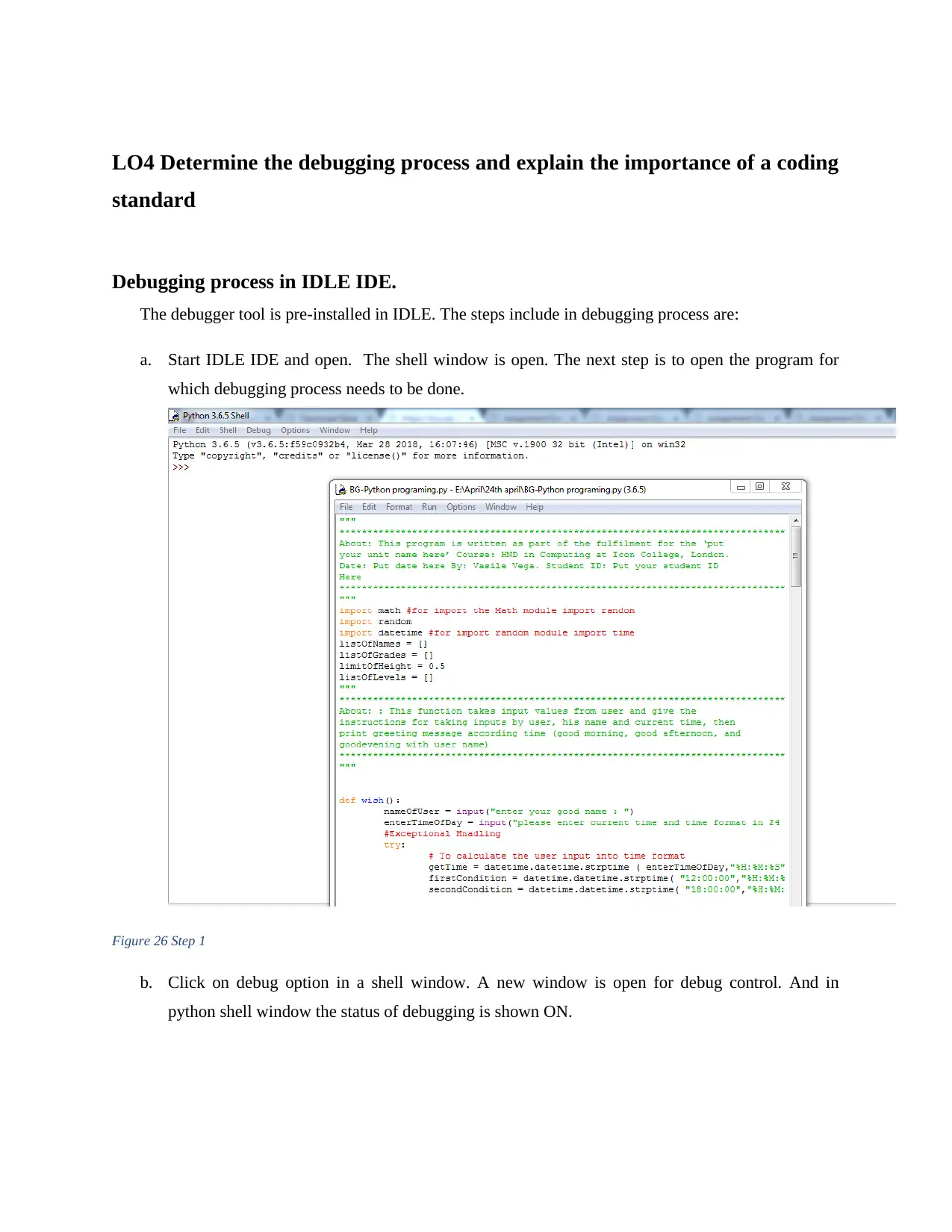
LO4 Determine the debugging process and explain the importance of a coding
standard
Debugging process in IDLE IDE.
The debugger tool is pre-installed in IDLE. The steps include in debugging process are:
a. Start IDLE IDE and open. The shell window is open. The next step is to open the program for
which debugging process needs to be done.
Figure 26 Step 1
b. Click on debug option in a shell window. A new window is open for debug control. And in
python shell window the status of debugging is shown ON.
standard
Debugging process in IDLE IDE.
The debugger tool is pre-installed in IDLE. The steps include in debugging process are:
a. Start IDLE IDE and open. The shell window is open. The next step is to open the program for
which debugging process needs to be done.
Figure 26 Step 1
b. Click on debug option in a shell window. A new window is open for debug control. And in
python shell window the status of debugging is shown ON.
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
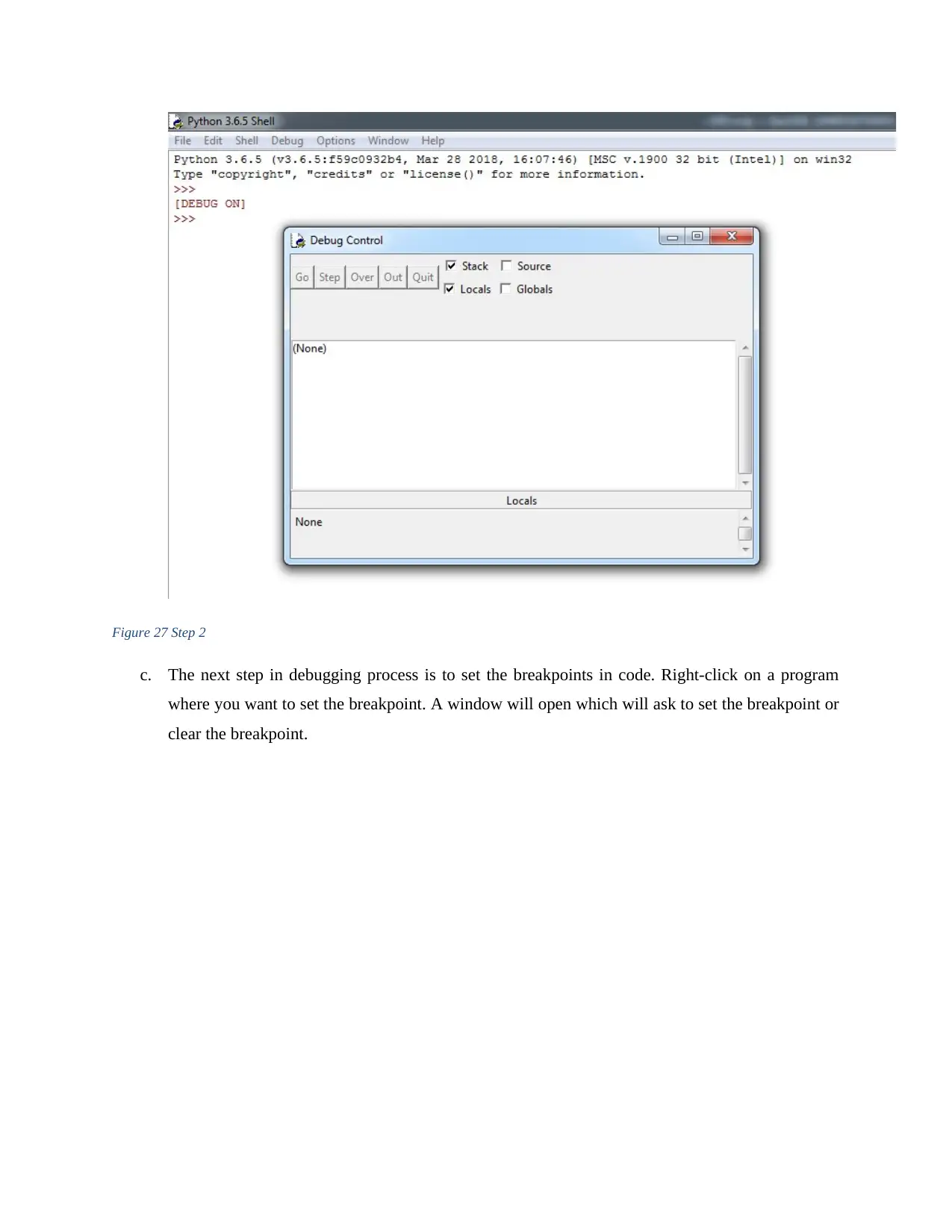
Figure 27 Step 2
c. The next step in debugging process is to set the breakpoints in code. Right-click on a program
where you want to set the breakpoint. A window will open which will ask to set the breakpoint or
clear the breakpoint.
c. The next step in debugging process is to set the breakpoints in code. Right-click on a program
where you want to set the breakpoint. A window will open which will ask to set the breakpoint or
clear the breakpoint.
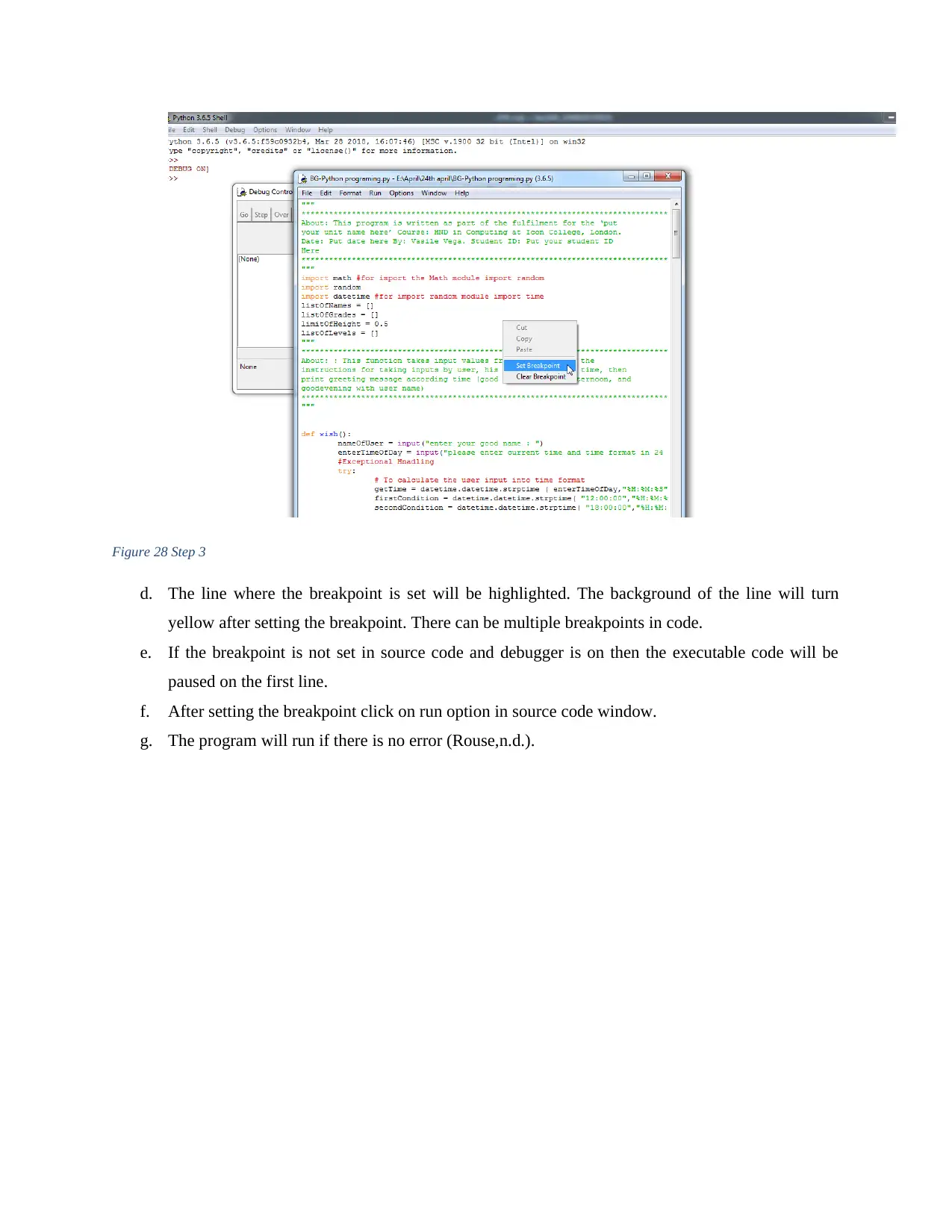
Figure 28 Step 3
d. The line where the breakpoint is set will be highlighted. The background of the line will turn
yellow after setting the breakpoint. There can be multiple breakpoints in code.
e. If the breakpoint is not set in source code and debugger is on then the executable code will be
paused on the first line.
f. After setting the breakpoint click on run option in source code window.
g. The program will run if there is no error (Rouse,n.d.).
d. The line where the breakpoint is set will be highlighted. The background of the line will turn
yellow after setting the breakpoint. There can be multiple breakpoints in code.
e. If the breakpoint is not set in source code and debugger is on then the executable code will be
paused on the first line.
f. After setting the breakpoint click on run option in source code window.
g. The program will run if there is no error (Rouse,n.d.).
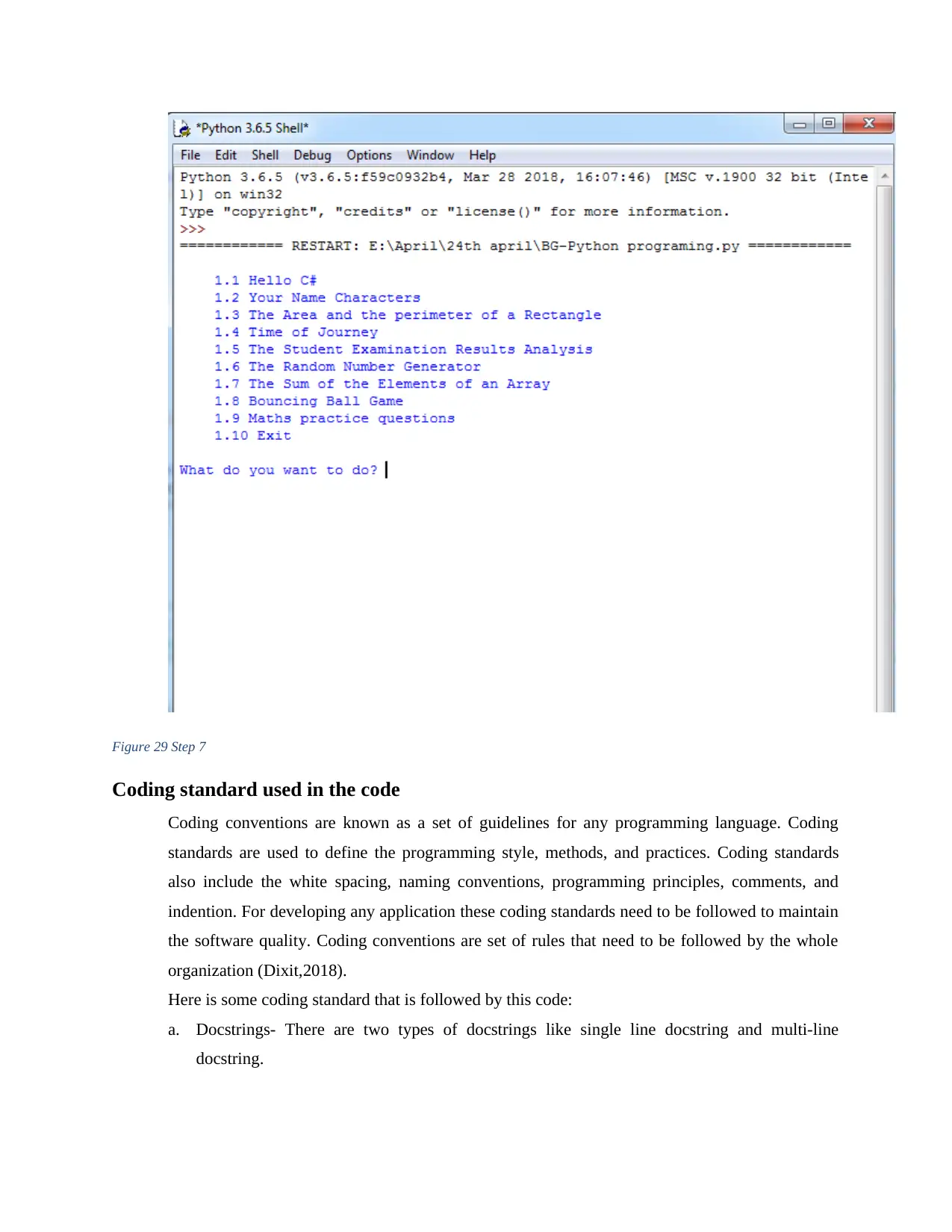
Figure 29 Step 7
Coding standard used in the code
Coding conventions are known as a set of guidelines for any programming language. Coding
standards are used to define the programming style, methods, and practices. Coding standards
also include the white spacing, naming conventions, programming principles, comments, and
indention. For developing any application these coding standards need to be followed to maintain
the software quality. Coding conventions are set of rules that need to be followed by the whole
organization (Dixit,2018).
Here is some coding standard that is followed by this code:
a. Docstrings- There are two types of docstrings like single line docstring and multi-line
docstring.
Coding standard used in the code
Coding conventions are known as a set of guidelines for any programming language. Coding
standards are used to define the programming style, methods, and practices. Coding standards
also include the white spacing, naming conventions, programming principles, comments, and
indention. For developing any application these coding standards need to be followed to maintain
the software quality. Coding conventions are set of rules that need to be followed by the whole
organization (Dixit,2018).
Here is some coding standard that is followed by this code:
a. Docstrings- There are two types of docstrings like single line docstring and multi-line
docstring.
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
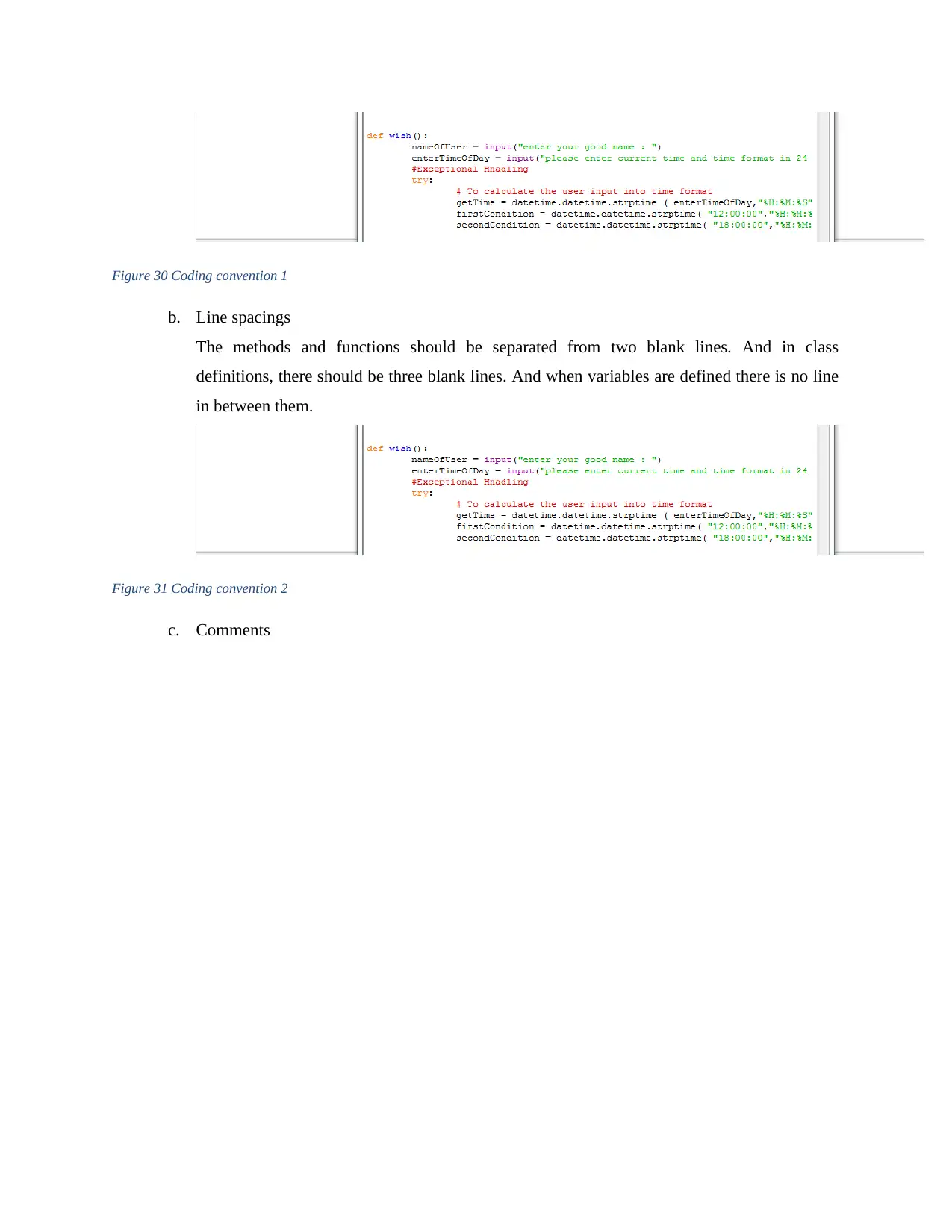
Figure 30 Coding convention 1
b. Line spacings
The methods and functions should be separated from two blank lines. And in class
definitions, there should be three blank lines. And when variables are defined there is no line
in between them.
Figure 31 Coding convention 2
c. Comments
b. Line spacings
The methods and functions should be separated from two blank lines. And in class
definitions, there should be three blank lines. And when variables are defined there is no line
in between them.
Figure 31 Coding convention 2
c. Comments
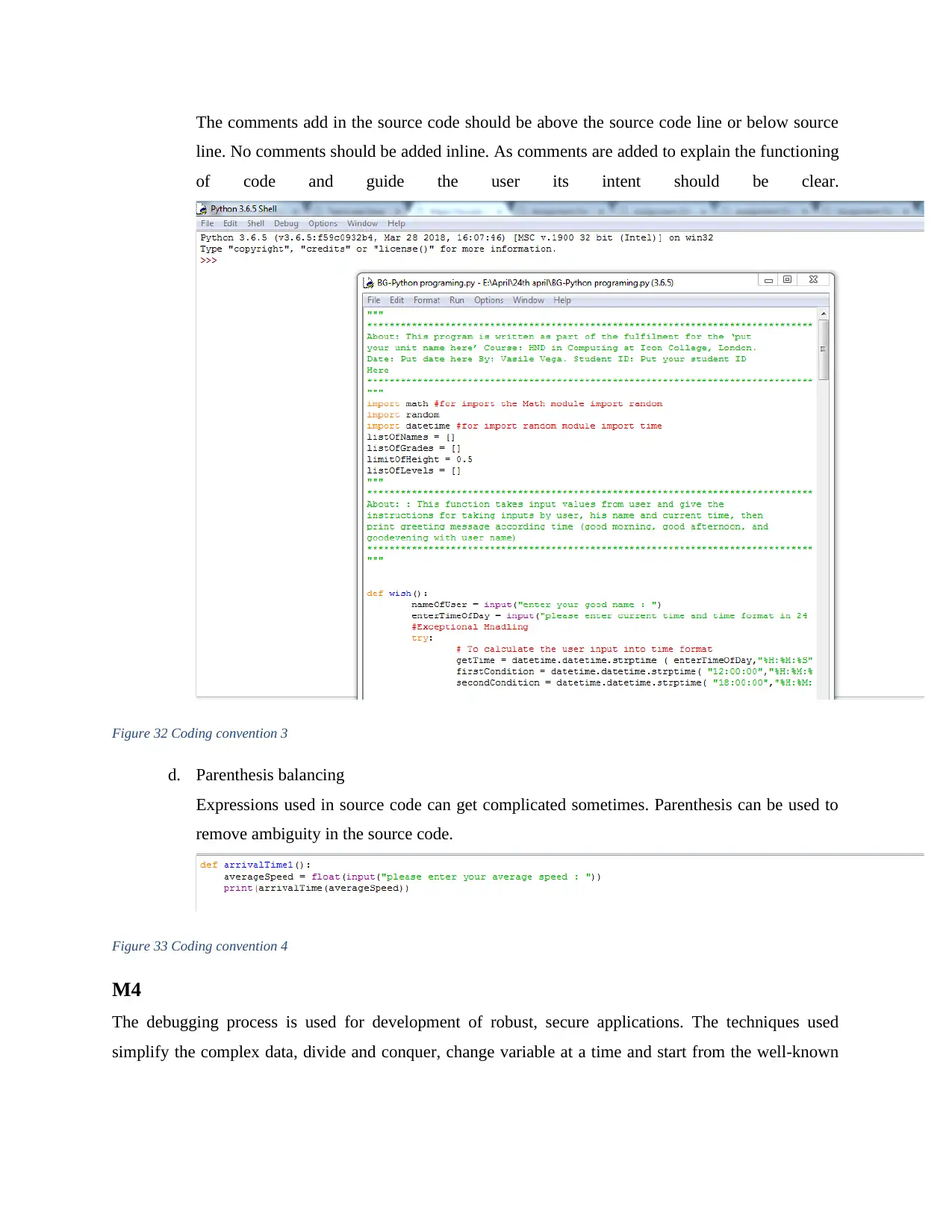
The comments add in the source code should be above the source code line or below source
line. No comments should be added inline. As comments are added to explain the functioning
of code and guide the user its intent should be clear.
Figure 32 Coding convention 3
d. Parenthesis balancing
Expressions used in source code can get complicated sometimes. Parenthesis can be used to
remove ambiguity in the source code.
Figure 33 Coding convention 4
M4
The debugging process is used for development of robust, secure applications. The techniques used
simplify the complex data, divide and conquer, change variable at a time and start from the well-known
line. No comments should be added inline. As comments are added to explain the functioning
of code and guide the user its intent should be clear.
Figure 32 Coding convention 3
d. Parenthesis balancing
Expressions used in source code can get complicated sometimes. Parenthesis can be used to
remove ambiguity in the source code.
Figure 33 Coding convention 4
M4
The debugging process is used for development of robust, secure applications. The techniques used
simplify the complex data, divide and conquer, change variable at a time and start from the well-known
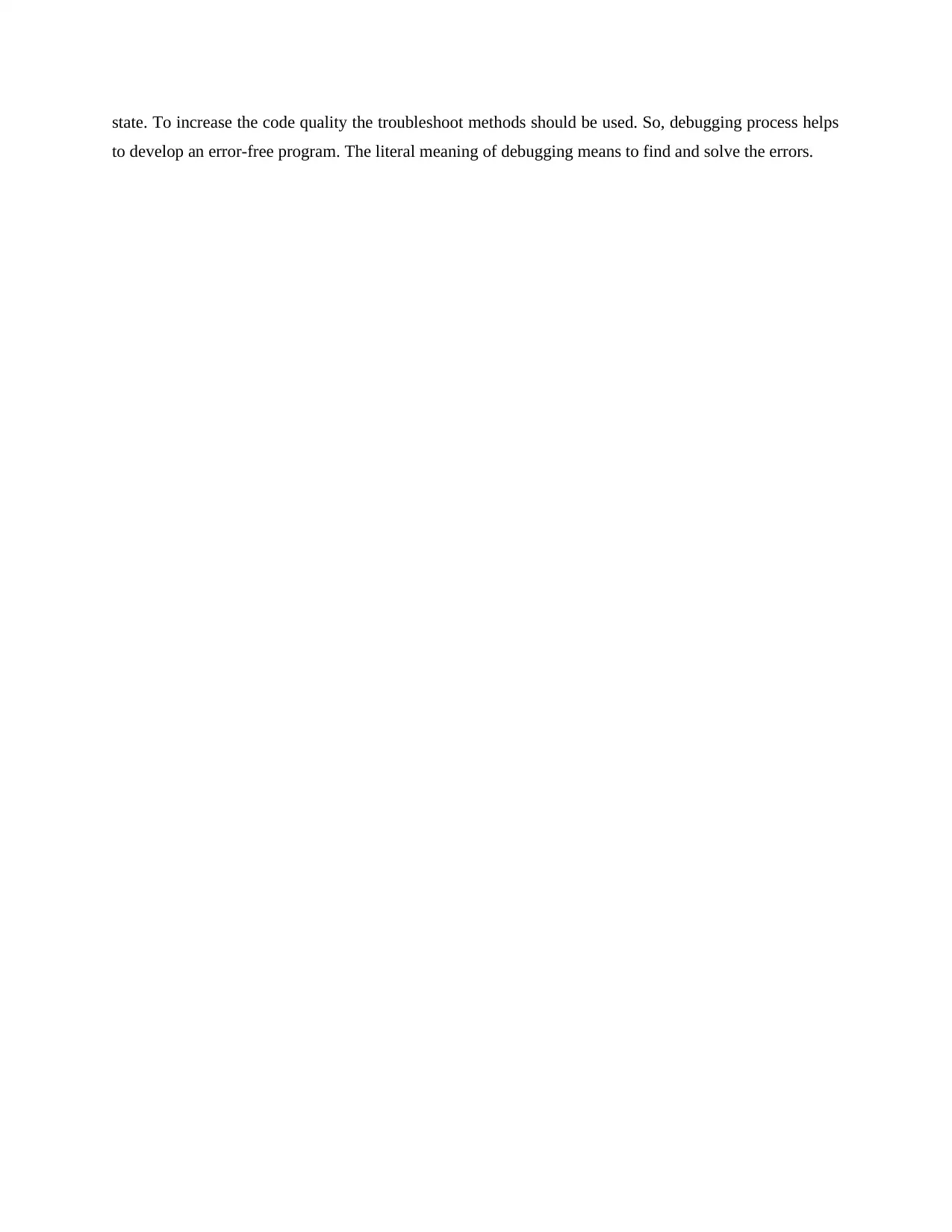
state. To increase the code quality the troubleshoot methods should be used. So, debugging process helps
to develop an error-free program. The literal meaning of debugging means to find and solve the errors.
to develop an error-free program. The literal meaning of debugging means to find and solve the errors.
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
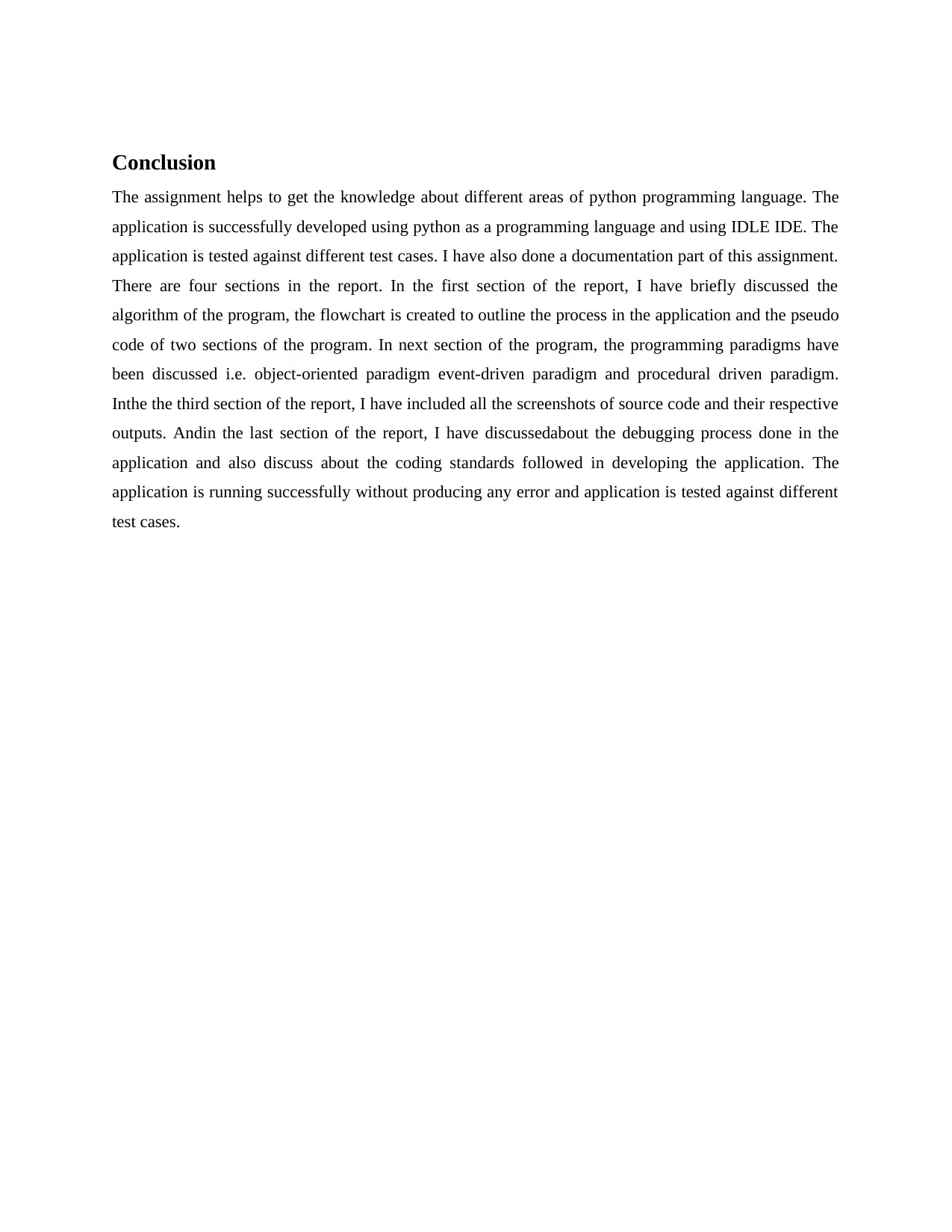
Conclusion
The assignment helps to get the knowledge about different areas of python programming language. The
application is successfully developed using python as a programming language and using IDLE IDE. The
application is tested against different test cases. I have also done a documentation part of this assignment.
There are four sections in the report. In the first section of the report, I have briefly discussed the
algorithm of the program, the flowchart is created to outline the process in the application and the pseudo
code of two sections of the program. In next section of the program, the programming paradigms have
been discussed i.e. object-oriented paradigm event-driven paradigm and procedural driven paradigm.
Inthe the third section of the report, I have included all the screenshots of source code and their respective
outputs. Andin the last section of the report, I have discussedabout the debugging process done in the
application and also discuss about the coding standards followed in developing the application. The
application is running successfully without producing any error and application is tested against different
test cases.
The assignment helps to get the knowledge about different areas of python programming language. The
application is successfully developed using python as a programming language and using IDLE IDE. The
application is tested against different test cases. I have also done a documentation part of this assignment.
There are four sections in the report. In the first section of the report, I have briefly discussed the
algorithm of the program, the flowchart is created to outline the process in the application and the pseudo
code of two sections of the program. In next section of the program, the programming paradigms have
been discussed i.e. object-oriented paradigm event-driven paradigm and procedural driven paradigm.
Inthe the third section of the report, I have included all the screenshots of source code and their respective
outputs. Andin the last section of the report, I have discussedabout the debugging process done in the
application and also discuss about the coding standards followed in developing the application. The
application is running successfully without producing any error and application is tested against different
test cases.
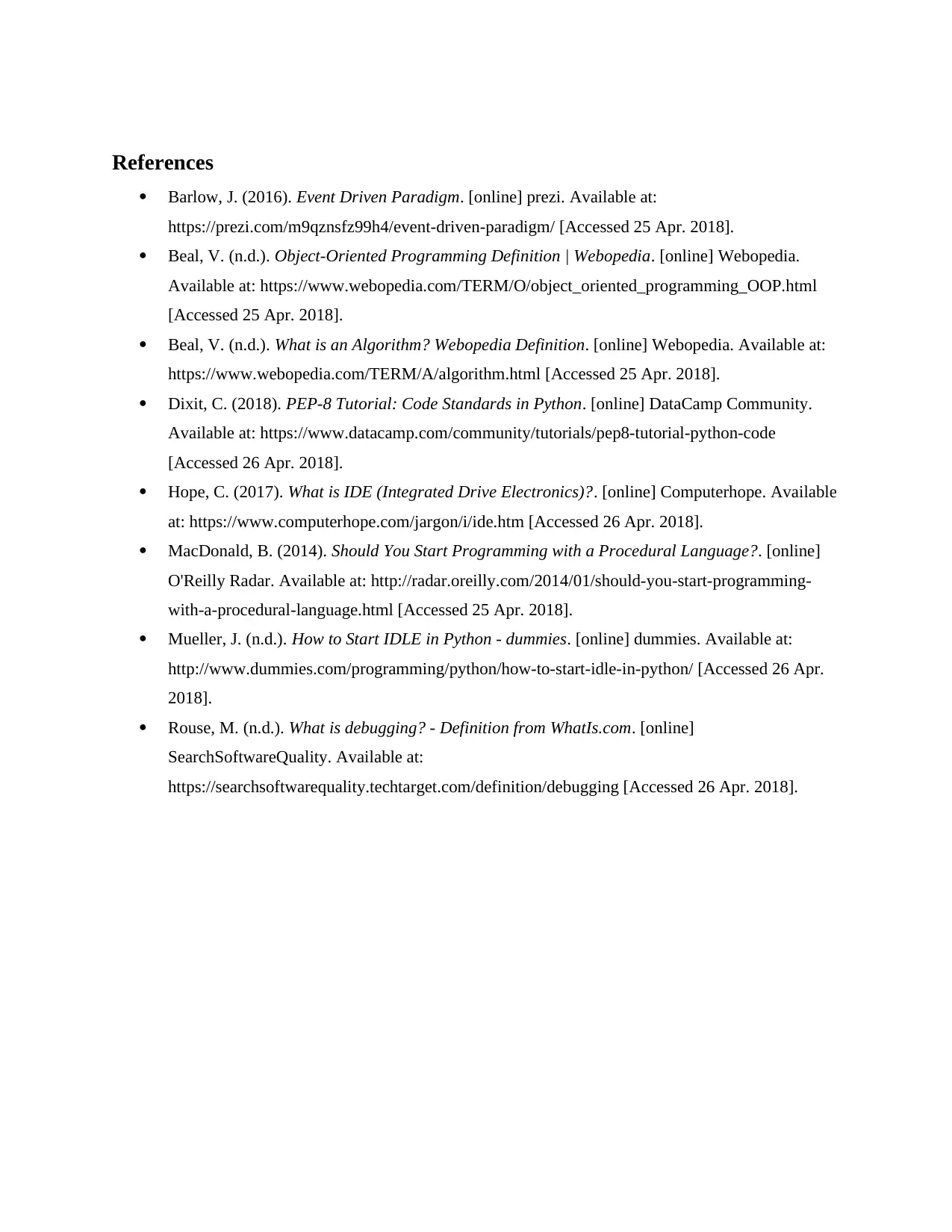
References
Barlow, J. (2016). Event Driven Paradigm. [online] prezi. Available at:
https://prezi.com/m9qznsfz99h4/event-driven-paradigm/ [Accessed 25 Apr. 2018].
Beal, V. (n.d.). Object-Oriented Programming Definition | Webopedia. [online] Webopedia.
Available at: https://www.webopedia.com/TERM/O/object_oriented_programming_OOP.html
[Accessed 25 Apr. 2018].
Beal, V. (n.d.). What is an Algorithm? Webopedia Definition. [online] Webopedia. Available at:
https://www.webopedia.com/TERM/A/algorithm.html [Accessed 25 Apr. 2018].
Dixit, C. (2018). PEP-8 Tutorial: Code Standards in Python. [online] DataCamp Community.
Available at: https://www.datacamp.com/community/tutorials/pep8-tutorial-python-code
[Accessed 26 Apr. 2018].
Hope, C. (2017). What is IDE (Integrated Drive Electronics)?. [online] Computerhope. Available
at: https://www.computerhope.com/jargon/i/ide.htm [Accessed 26 Apr. 2018].
MacDonald, B. (2014). Should You Start Programming with a Procedural Language?. [online]
O'Reilly Radar. Available at: http://radar.oreilly.com/2014/01/should-you-start-programming-
with-a-procedural-language.html [Accessed 25 Apr. 2018].
Mueller, J. (n.d.). How to Start IDLE in Python - dummies. [online] dummies. Available at:
http://www.dummies.com/programming/python/how-to-start-idle-in-python/ [Accessed 26 Apr.
2018].
Rouse, M. (n.d.). What is debugging? - Definition from WhatIs.com. [online]
SearchSoftwareQuality. Available at:
https://searchsoftwarequality.techtarget.com/definition/debugging [Accessed 26 Apr. 2018].
Barlow, J. (2016). Event Driven Paradigm. [online] prezi. Available at:
https://prezi.com/m9qznsfz99h4/event-driven-paradigm/ [Accessed 25 Apr. 2018].
Beal, V. (n.d.). Object-Oriented Programming Definition | Webopedia. [online] Webopedia.
Available at: https://www.webopedia.com/TERM/O/object_oriented_programming_OOP.html
[Accessed 25 Apr. 2018].
Beal, V. (n.d.). What is an Algorithm? Webopedia Definition. [online] Webopedia. Available at:
https://www.webopedia.com/TERM/A/algorithm.html [Accessed 25 Apr. 2018].
Dixit, C. (2018). PEP-8 Tutorial: Code Standards in Python. [online] DataCamp Community.
Available at: https://www.datacamp.com/community/tutorials/pep8-tutorial-python-code
[Accessed 26 Apr. 2018].
Hope, C. (2017). What is IDE (Integrated Drive Electronics)?. [online] Computerhope. Available
at: https://www.computerhope.com/jargon/i/ide.htm [Accessed 26 Apr. 2018].
MacDonald, B. (2014). Should You Start Programming with a Procedural Language?. [online]
O'Reilly Radar. Available at: http://radar.oreilly.com/2014/01/should-you-start-programming-
with-a-procedural-language.html [Accessed 25 Apr. 2018].
Mueller, J. (n.d.). How to Start IDLE in Python - dummies. [online] dummies. Available at:
http://www.dummies.com/programming/python/how-to-start-idle-in-python/ [Accessed 26 Apr.
2018].
Rouse, M. (n.d.). What is debugging? - Definition from WhatIs.com. [online]
SearchSoftwareQuality. Available at:
https://searchsoftwarequality.techtarget.com/definition/debugging [Accessed 26 Apr. 2018].
1 out of 39
Related Documents
![[object Object]](/_next/image/?url=%2F_next%2Fstatic%2Fmedia%2Flogo.6d15ce61.png&w=640&q=75)
Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.