Python Programming Fundamentals: Algorithms, IDEs, Debugging, and Coding Standards
VerifiedAdded on 2024/05/29
|28
|4336
|358
AI Summary
This assignment explores fundamental concepts in Python programming, including algorithm design, the use of Integrated Development Environments (IDEs), debugging techniques, and the importance of coding standards. Through practical examples and code implementations, the document demonstrates the application of these concepts in solving various programming problems. It covers procedural, object-oriented, and event-driven programming paradigms, providing a comprehensive understanding of Python's versatility and its role in software development.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
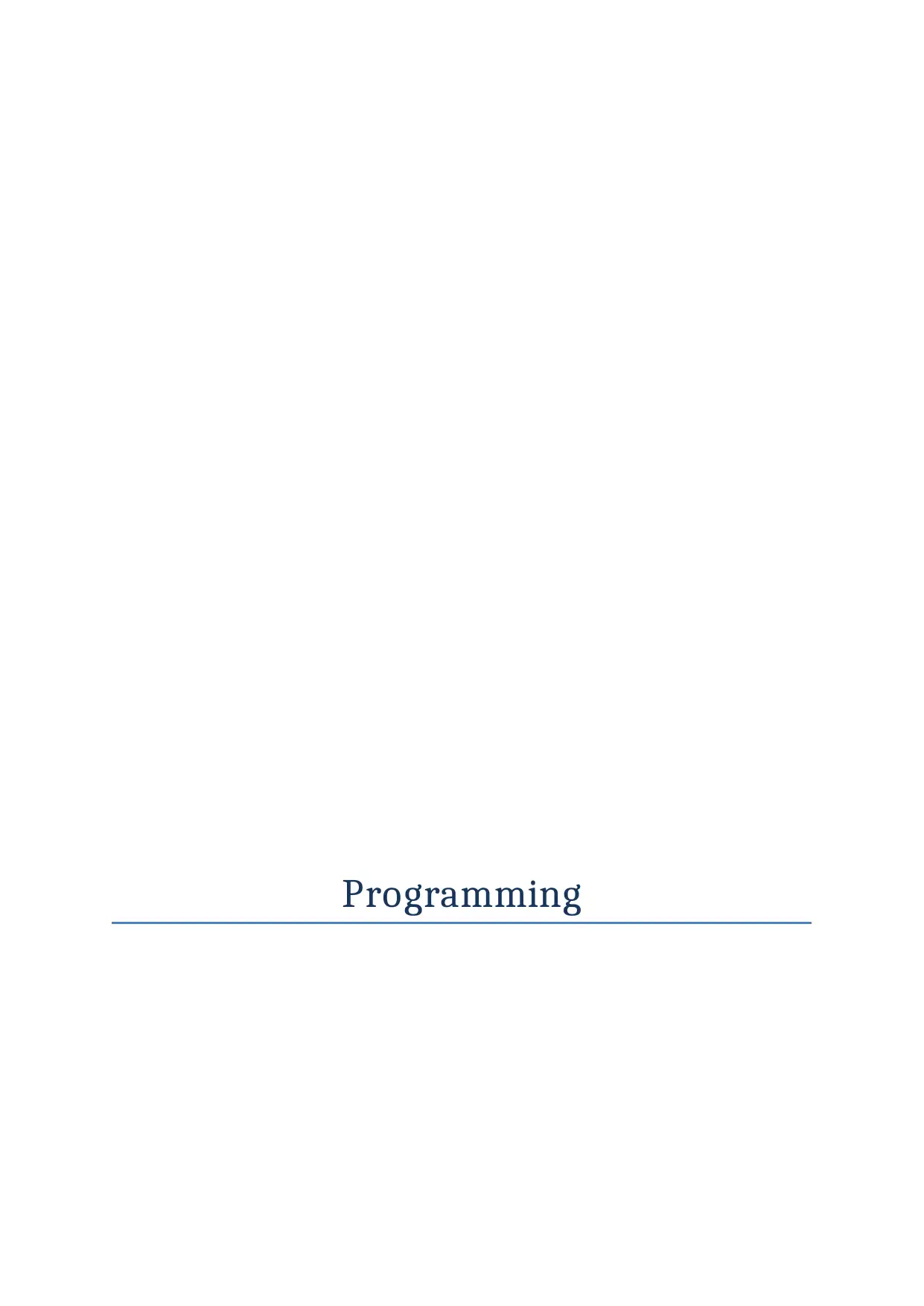
Programming
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
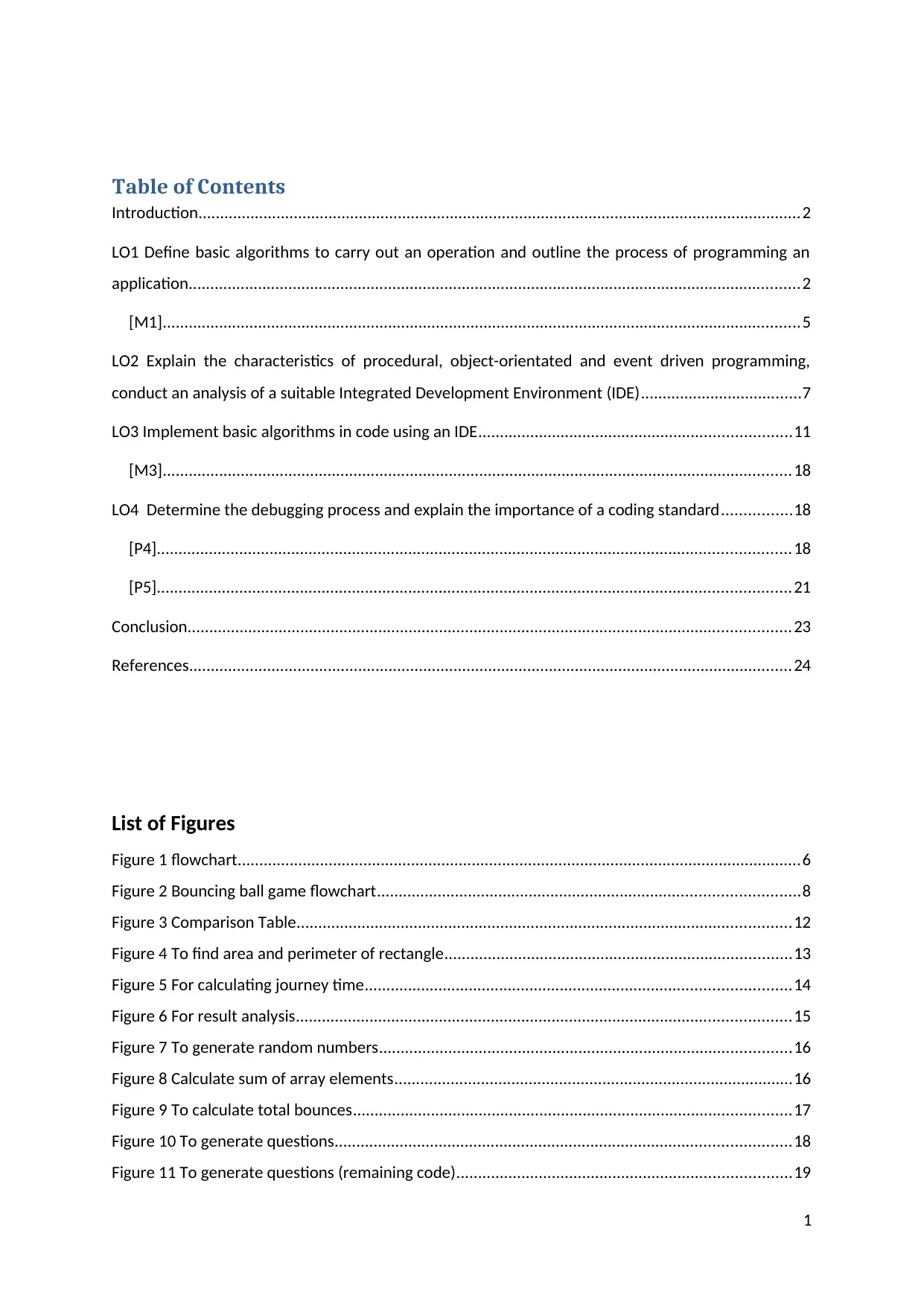
Table of Contents
Introduction...........................................................................................................................................2
LO1 Define basic algorithms to carry out an operation and outline the process of programming an
application.............................................................................................................................................2
[M1]...................................................................................................................................................5
LO2 Explain the characteristics of procedural, object-orientated and event driven programming,
conduct an analysis of a suitable Integrated Development Environment (IDE).....................................7
LO3 Implement basic algorithms in code using an IDE........................................................................11
[M3].................................................................................................................................................18
LO4 Determine the debugging process and explain the importance of a coding standard................18
[P4]..................................................................................................................................................18
[P5]..................................................................................................................................................21
Conclusion...........................................................................................................................................23
References...........................................................................................................................................24
List of Figures
Figure 1 flowchart..................................................................................................................................6
Figure 2 Bouncing ball game flowchart.................................................................................................8
Figure 3 Comparison Table..................................................................................................................12
Figure 4 To find area and perimeter of rectangle................................................................................13
Figure 5 For calculating journey time..................................................................................................14
Figure 6 For result analysis..................................................................................................................15
Figure 7 To generate random numbers...............................................................................................16
Figure 8 Calculate sum of array elements............................................................................................16
Figure 9 To calculate total bounces.....................................................................................................17
Figure 10 To generate questions.........................................................................................................18
Figure 11 To generate questions (remaining code).............................................................................19
1
Introduction...........................................................................................................................................2
LO1 Define basic algorithms to carry out an operation and outline the process of programming an
application.............................................................................................................................................2
[M1]...................................................................................................................................................5
LO2 Explain the characteristics of procedural, object-orientated and event driven programming,
conduct an analysis of a suitable Integrated Development Environment (IDE).....................................7
LO3 Implement basic algorithms in code using an IDE........................................................................11
[M3].................................................................................................................................................18
LO4 Determine the debugging process and explain the importance of a coding standard................18
[P4]..................................................................................................................................................18
[P5]..................................................................................................................................................21
Conclusion...........................................................................................................................................23
References...........................................................................................................................................24
List of Figures
Figure 1 flowchart..................................................................................................................................6
Figure 2 Bouncing ball game flowchart.................................................................................................8
Figure 3 Comparison Table..................................................................................................................12
Figure 4 To find area and perimeter of rectangle................................................................................13
Figure 5 For calculating journey time..................................................................................................14
Figure 6 For result analysis..................................................................................................................15
Figure 7 To generate random numbers...............................................................................................16
Figure 8 Calculate sum of array elements............................................................................................16
Figure 9 To calculate total bounces.....................................................................................................17
Figure 10 To generate questions.........................................................................................................18
Figure 11 To generate questions (remaining code).............................................................................19
1
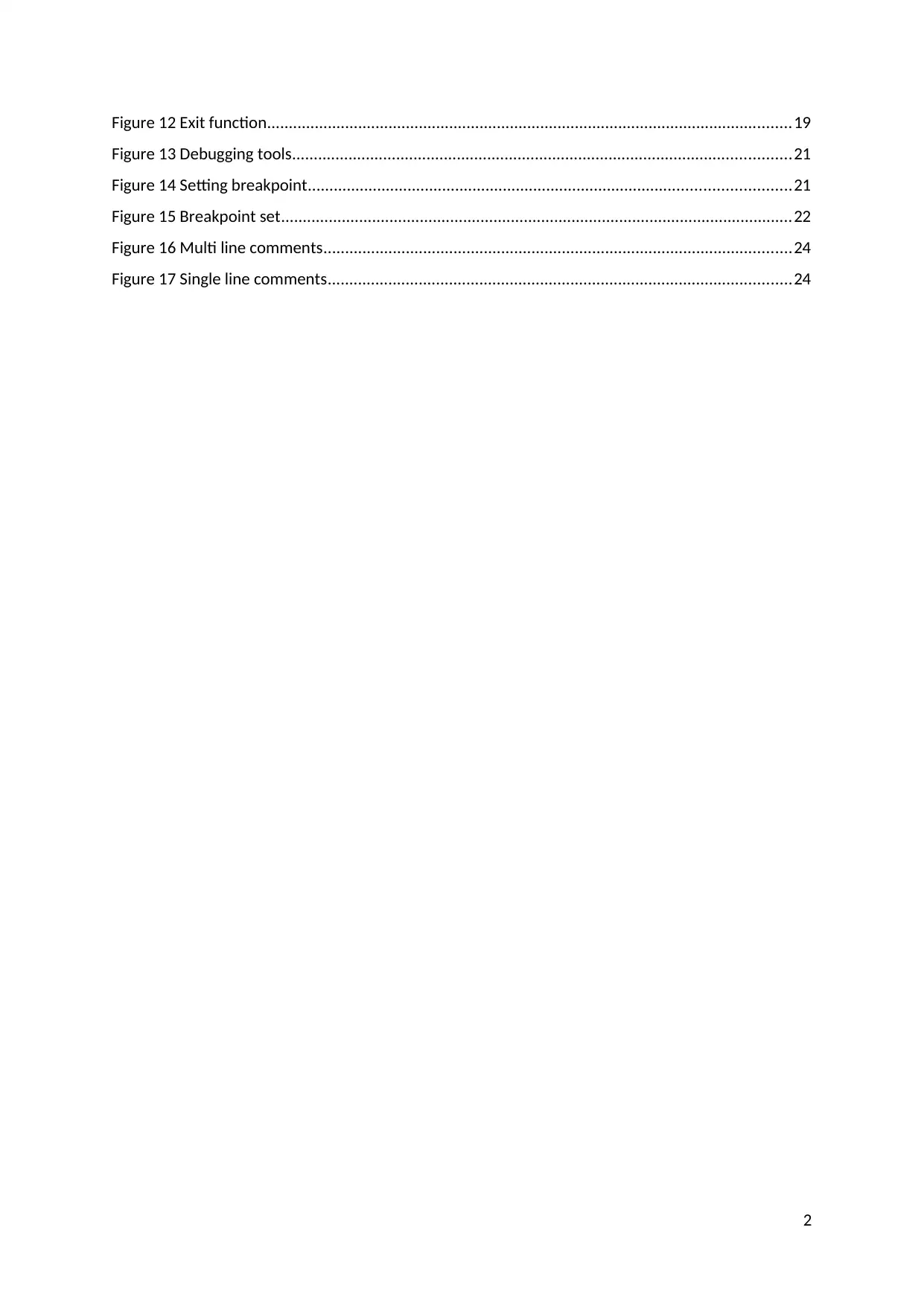
Figure 12 Exit function.........................................................................................................................19
Figure 13 Debugging tools...................................................................................................................21
Figure 14 Setting breakpoint...............................................................................................................21
Figure 15 Breakpoint set......................................................................................................................22
Figure 16 Multi line comments............................................................................................................24
Figure 17 Single line comments...........................................................................................................24
2
Figure 13 Debugging tools...................................................................................................................21
Figure 14 Setting breakpoint...............................................................................................................21
Figure 15 Breakpoint set......................................................................................................................22
Figure 16 Multi line comments............................................................................................................24
Figure 17 Single line comments...........................................................................................................24
2
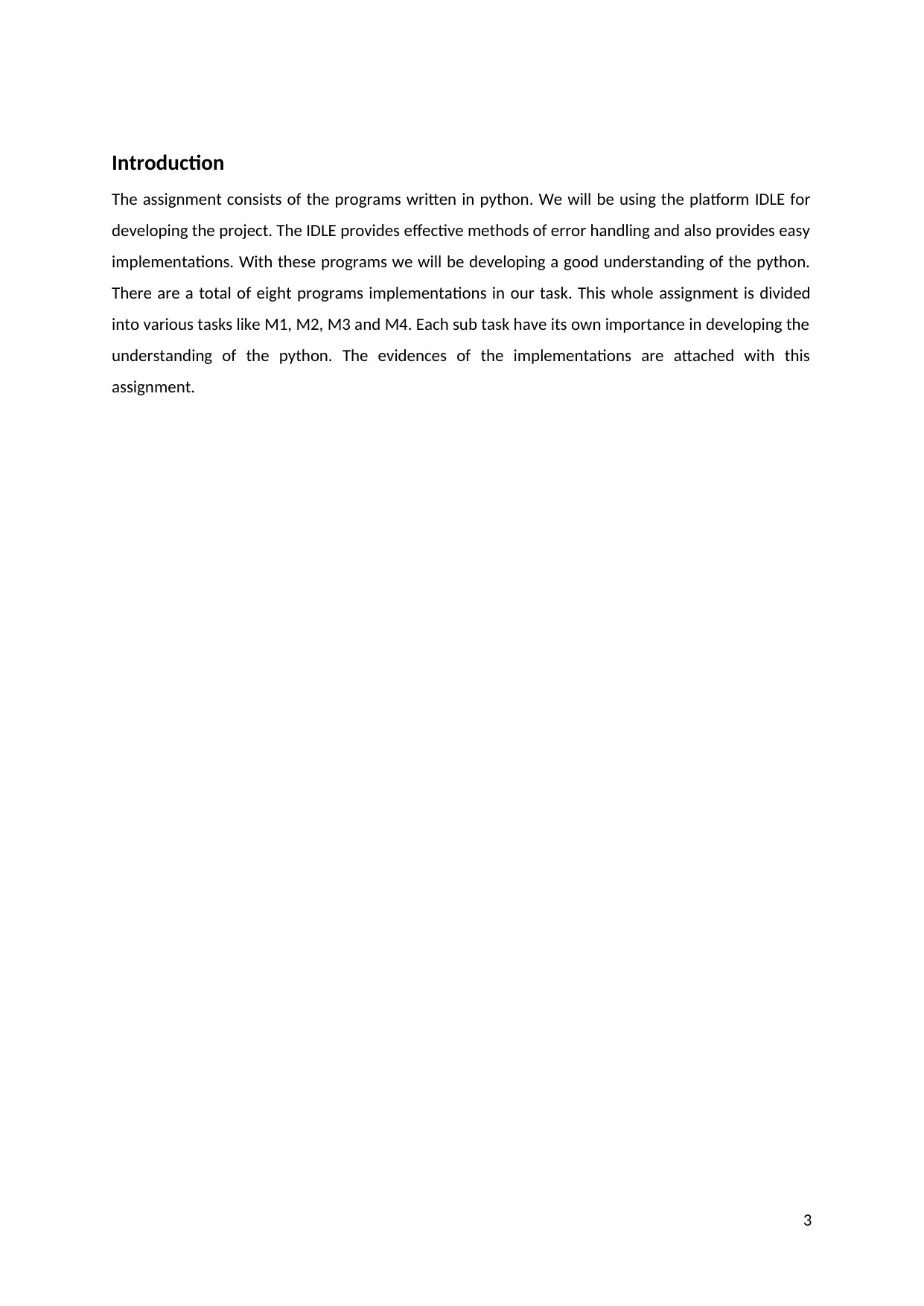
Introduction
The assignment consists of the programs written in python. We will be using the platform IDLE for
developing the project. The IDLE provides effective methods of error handling and also provides easy
implementations. With these programs we will be developing a good understanding of the python.
There are a total of eight programs implementations in our task. This whole assignment is divided
into various tasks like M1, M2, M3 and M4. Each sub task have its own importance in developing the
understanding of the python. The evidences of the implementations are attached with this
assignment.
3
The assignment consists of the programs written in python. We will be using the platform IDLE for
developing the project. The IDLE provides effective methods of error handling and also provides easy
implementations. With these programs we will be developing a good understanding of the python.
There are a total of eight programs implementations in our task. This whole assignment is divided
into various tasks like M1, M2, M3 and M4. Each sub task have its own importance in developing the
understanding of the python. The evidences of the implementations are attached with this
assignment.
3
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
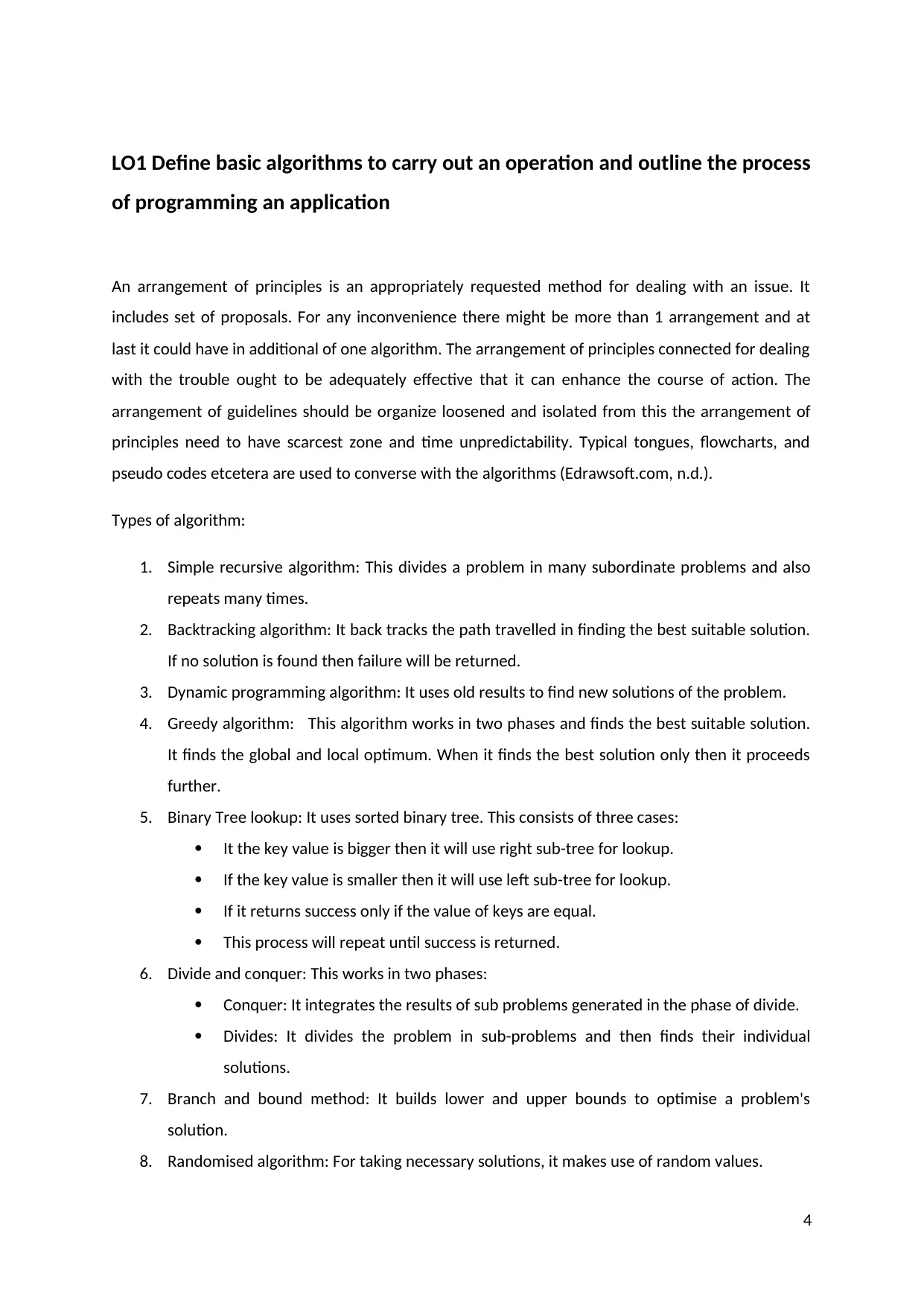
LO1 Define basic algorithms to carry out an operation and outline the process
of programming an application
An arrangement of principles is an appropriately requested method for dealing with an issue. It
includes set of proposals. For any inconvenience there might be more than 1 arrangement and at
last it could have in additional of one algorithm. The arrangement of principles connected for dealing
with the trouble ought to be adequately effective that it can enhance the course of action. The
arrangement of guidelines should be organize loosened and isolated from this the arrangement of
principles need to have scarcest zone and time unpredictability. Typical tongues, flowcharts, and
pseudo codes etcetera are used to converse with the algorithms (Edrawsoft.com, n.d.).
Types of algorithm:
1. Simple recursive algorithm: This divides a problem in many subordinate problems and also
repeats many times.
2. Backtracking algorithm: It back tracks the path travelled in finding the best suitable solution.
If no solution is found then failure will be returned.
3. Dynamic programming algorithm: It uses old results to find new solutions of the problem.
4. Greedy algorithm: This algorithm works in two phases and finds the best suitable solution.
It finds the global and local optimum. When it finds the best solution only then it proceeds
further.
5. Binary Tree lookup: It uses sorted binary tree. This consists of three cases:
It the key value is bigger then it will use right sub-tree for lookup.
If the key value is smaller then it will use left sub-tree for lookup.
If it returns success only if the value of keys are equal.
This process will repeat until success is returned.
6. Divide and conquer: This works in two phases:
Conquer: It integrates the results of sub problems generated in the phase of divide.
Divides: It divides the problem in sub-problems and then finds their individual
solutions.
7. Branch and bound method: It builds lower and upper bounds to optimise a problem's
solution.
8. Randomised algorithm: For taking necessary solutions, it makes use of random values.
4
of programming an application
An arrangement of principles is an appropriately requested method for dealing with an issue. It
includes set of proposals. For any inconvenience there might be more than 1 arrangement and at
last it could have in additional of one algorithm. The arrangement of principles connected for dealing
with the trouble ought to be adequately effective that it can enhance the course of action. The
arrangement of guidelines should be organize loosened and isolated from this the arrangement of
principles need to have scarcest zone and time unpredictability. Typical tongues, flowcharts, and
pseudo codes etcetera are used to converse with the algorithms (Edrawsoft.com, n.d.).
Types of algorithm:
1. Simple recursive algorithm: This divides a problem in many subordinate problems and also
repeats many times.
2. Backtracking algorithm: It back tracks the path travelled in finding the best suitable solution.
If no solution is found then failure will be returned.
3. Dynamic programming algorithm: It uses old results to find new solutions of the problem.
4. Greedy algorithm: This algorithm works in two phases and finds the best suitable solution.
It finds the global and local optimum. When it finds the best solution only then it proceeds
further.
5. Binary Tree lookup: It uses sorted binary tree. This consists of three cases:
It the key value is bigger then it will use right sub-tree for lookup.
If the key value is smaller then it will use left sub-tree for lookup.
If it returns success only if the value of keys are equal.
This process will repeat until success is returned.
6. Divide and conquer: This works in two phases:
Conquer: It integrates the results of sub problems generated in the phase of divide.
Divides: It divides the problem in sub-problems and then finds their individual
solutions.
7. Branch and bound method: It builds lower and upper bounds to optimise a problem's
solution.
8. Randomised algorithm: For taking necessary solutions, it makes use of random values.
4
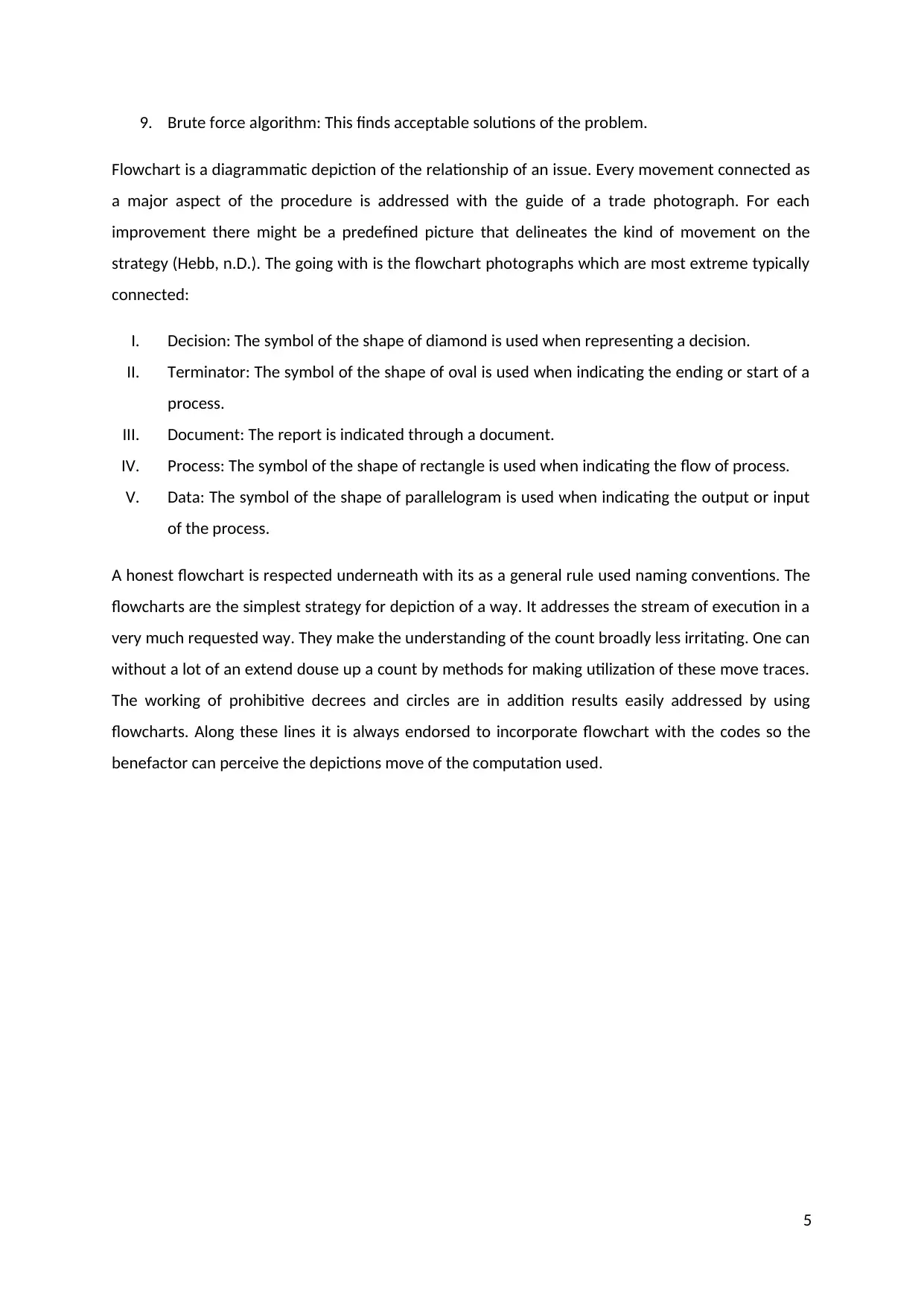
9. Brute force algorithm: This finds acceptable solutions of the problem.
Flowchart is a diagrammatic depiction of the relationship of an issue. Every movement connected as
a major aspect of the procedure is addressed with the guide of a trade photograph. For each
improvement there might be a predefined picture that delineates the kind of movement on the
strategy (Hebb, n.D.). The going with is the flowchart photographs which are most extreme typically
connected:
I. Decision: The symbol of the shape of diamond is used when representing a decision.
II. Terminator: The symbol of the shape of oval is used when indicating the ending or start of a
process.
III. Document: The report is indicated through a document.
IV. Process: The symbol of the shape of rectangle is used when indicating the flow of process.
V. Data: The symbol of the shape of parallelogram is used when indicating the output or input
of the process.
A honest flowchart is respected underneath with its as a general rule used naming conventions. The
flowcharts are the simplest strategy for depiction of a way. It addresses the stream of execution in a
very much requested way. They make the understanding of the count broadly less irritating. One can
without a lot of an extend douse up a count by methods for making utilization of these move traces.
The working of prohibitive decrees and circles are in addition results easily addressed by using
flowcharts. Along these lines it is always endorsed to incorporate flowchart with the codes so the
benefactor can perceive the depictions move of the computation used.
5
Flowchart is a diagrammatic depiction of the relationship of an issue. Every movement connected as
a major aspect of the procedure is addressed with the guide of a trade photograph. For each
improvement there might be a predefined picture that delineates the kind of movement on the
strategy (Hebb, n.D.). The going with is the flowchart photographs which are most extreme typically
connected:
I. Decision: The symbol of the shape of diamond is used when representing a decision.
II. Terminator: The symbol of the shape of oval is used when indicating the ending or start of a
process.
III. Document: The report is indicated through a document.
IV. Process: The symbol of the shape of rectangle is used when indicating the flow of process.
V. Data: The symbol of the shape of parallelogram is used when indicating the output or input
of the process.
A honest flowchart is respected underneath with its as a general rule used naming conventions. The
flowcharts are the simplest strategy for depiction of a way. It addresses the stream of execution in a
very much requested way. They make the understanding of the count broadly less irritating. One can
without a lot of an extend douse up a count by methods for making utilization of these move traces.
The working of prohibitive decrees and circles are in addition results easily addressed by using
flowcharts. Along these lines it is always endorsed to incorporate flowchart with the codes so the
benefactor can perceive the depictions move of the computation used.
5
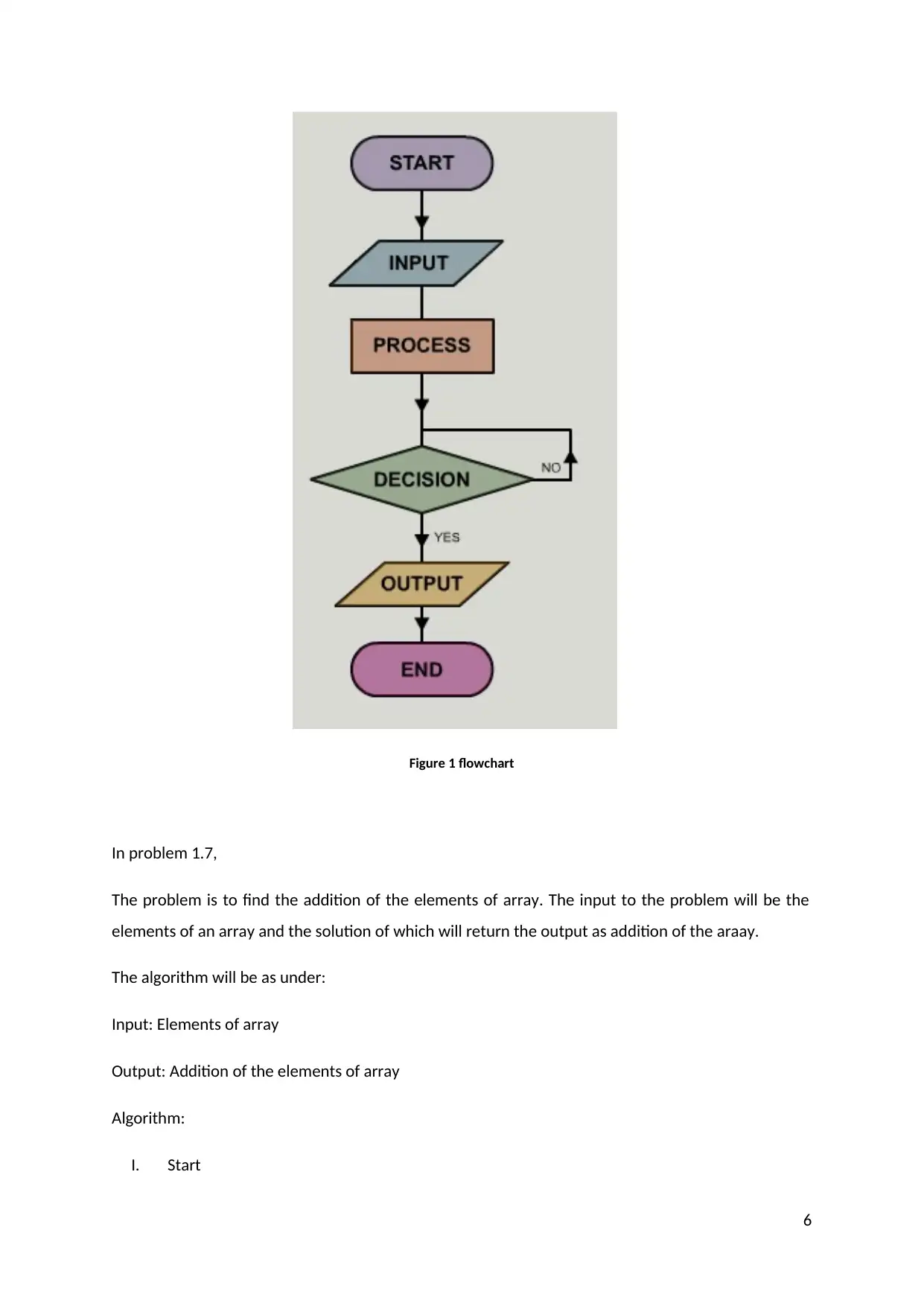
Figure 1 flowchart
In problem 1.7,
The problem is to find the addition of the elements of array. The input to the problem will be the
elements of an array and the solution of which will return the output as addition of the araay.
The algorithm will be as under:
Input: Elements of array
Output: Addition of the elements of array
Algorithm:
I. Start
6
In problem 1.7,
The problem is to find the addition of the elements of array. The input to the problem will be the
elements of an array and the solution of which will return the output as addition of the araay.
The algorithm will be as under:
Input: Elements of array
Output: Addition of the elements of array
Algorithm:
I. Start
6
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
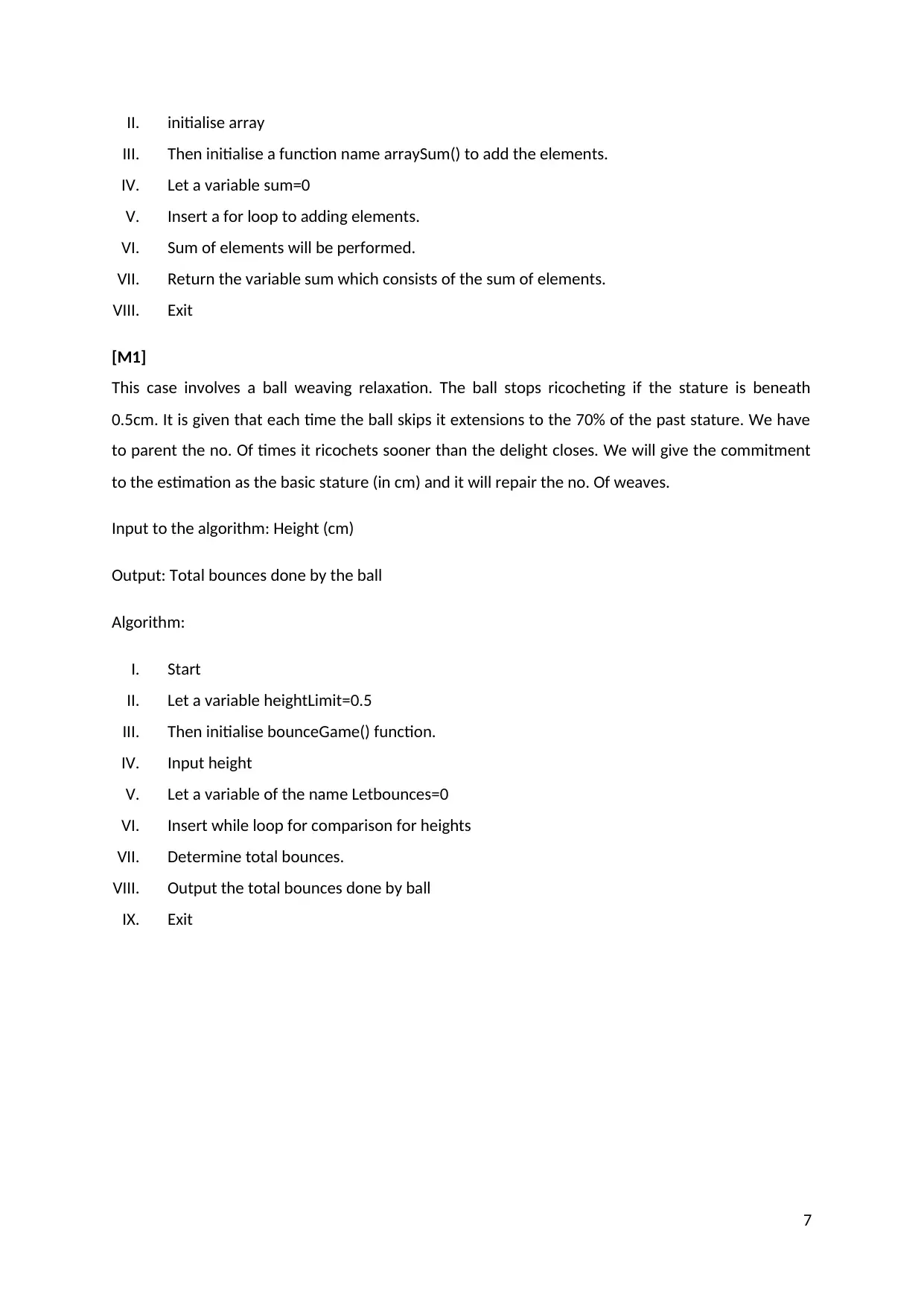
II. initialise array
III. Then initialise a function name arraySum() to add the elements.
IV. Let a variable sum=0
V. Insert a for loop to adding elements.
VI. Sum of elements will be performed.
VII. Return the variable sum which consists of the sum of elements.
VIII. Exit
[M1]
This case involves a ball weaving relaxation. The ball stops ricocheting if the stature is beneath
0.5cm. It is given that each time the ball skips it extensions to the 70% of the past stature. We have
to parent the no. Of times it ricochets sooner than the delight closes. We will give the commitment
to the estimation as the basic stature (in cm) and it will repair the no. Of weaves.
Input to the algorithm: Height (cm)
Output: Total bounces done by the ball
Algorithm:
I. Start
II. Let a variable heightLimit=0.5
III. Then initialise bounceGame() function.
IV. Input height
V. Let a variable of the name Letbounces=0
VI. Insert while loop for comparison for heights
VII. Determine total bounces.
VIII. Output the total bounces done by ball
IX. Exit
7
III. Then initialise a function name arraySum() to add the elements.
IV. Let a variable sum=0
V. Insert a for loop to adding elements.
VI. Sum of elements will be performed.
VII. Return the variable sum which consists of the sum of elements.
VIII. Exit
[M1]
This case involves a ball weaving relaxation. The ball stops ricocheting if the stature is beneath
0.5cm. It is given that each time the ball skips it extensions to the 70% of the past stature. We have
to parent the no. Of times it ricochets sooner than the delight closes. We will give the commitment
to the estimation as the basic stature (in cm) and it will repair the no. Of weaves.
Input to the algorithm: Height (cm)
Output: Total bounces done by the ball
Algorithm:
I. Start
II. Let a variable heightLimit=0.5
III. Then initialise bounceGame() function.
IV. Input height
V. Let a variable of the name Letbounces=0
VI. Insert while loop for comparison for heights
VII. Determine total bounces.
VIII. Output the total bounces done by ball
IX. Exit
7
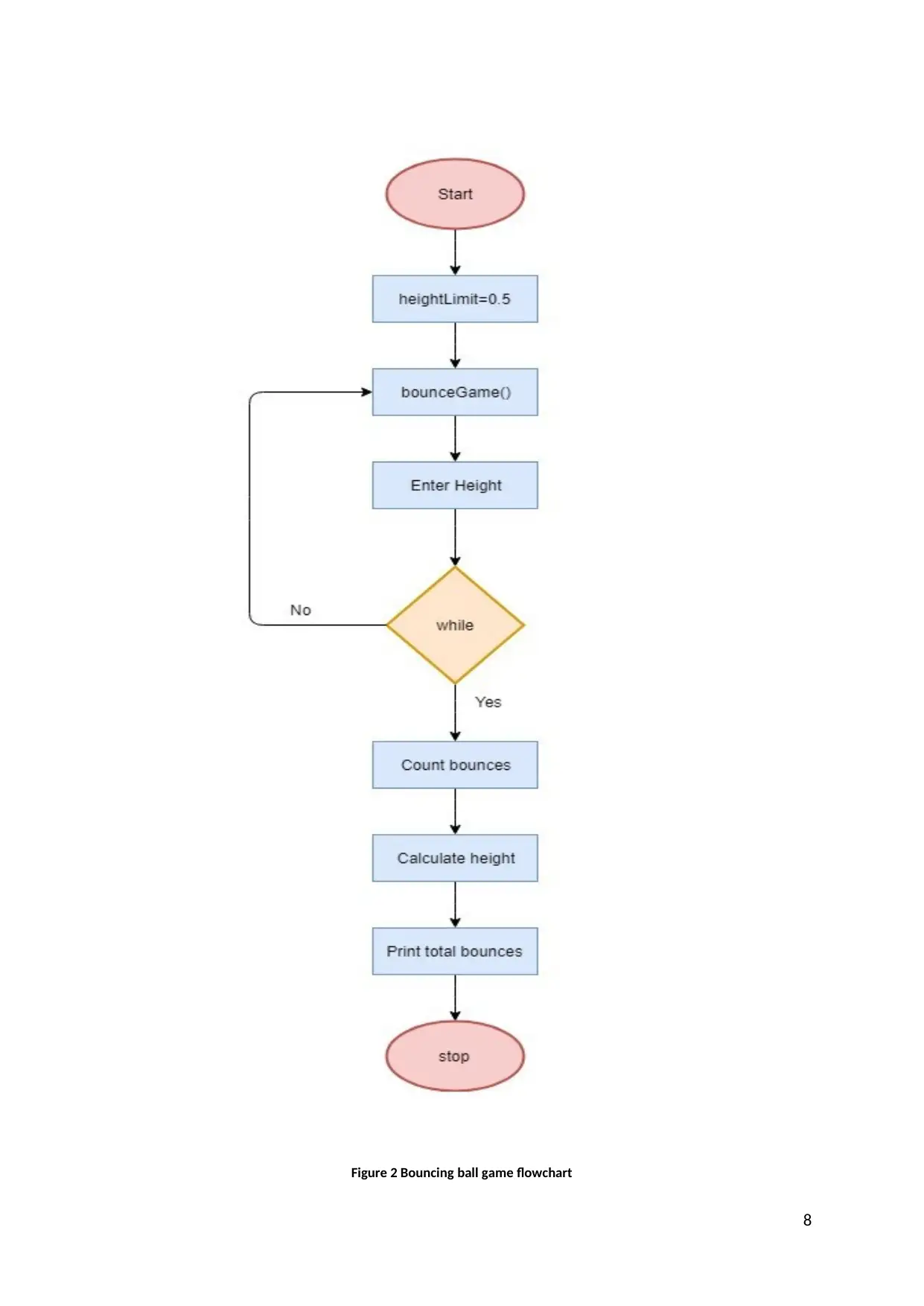
Figure 2 Bouncing ball game flowchart
8
8
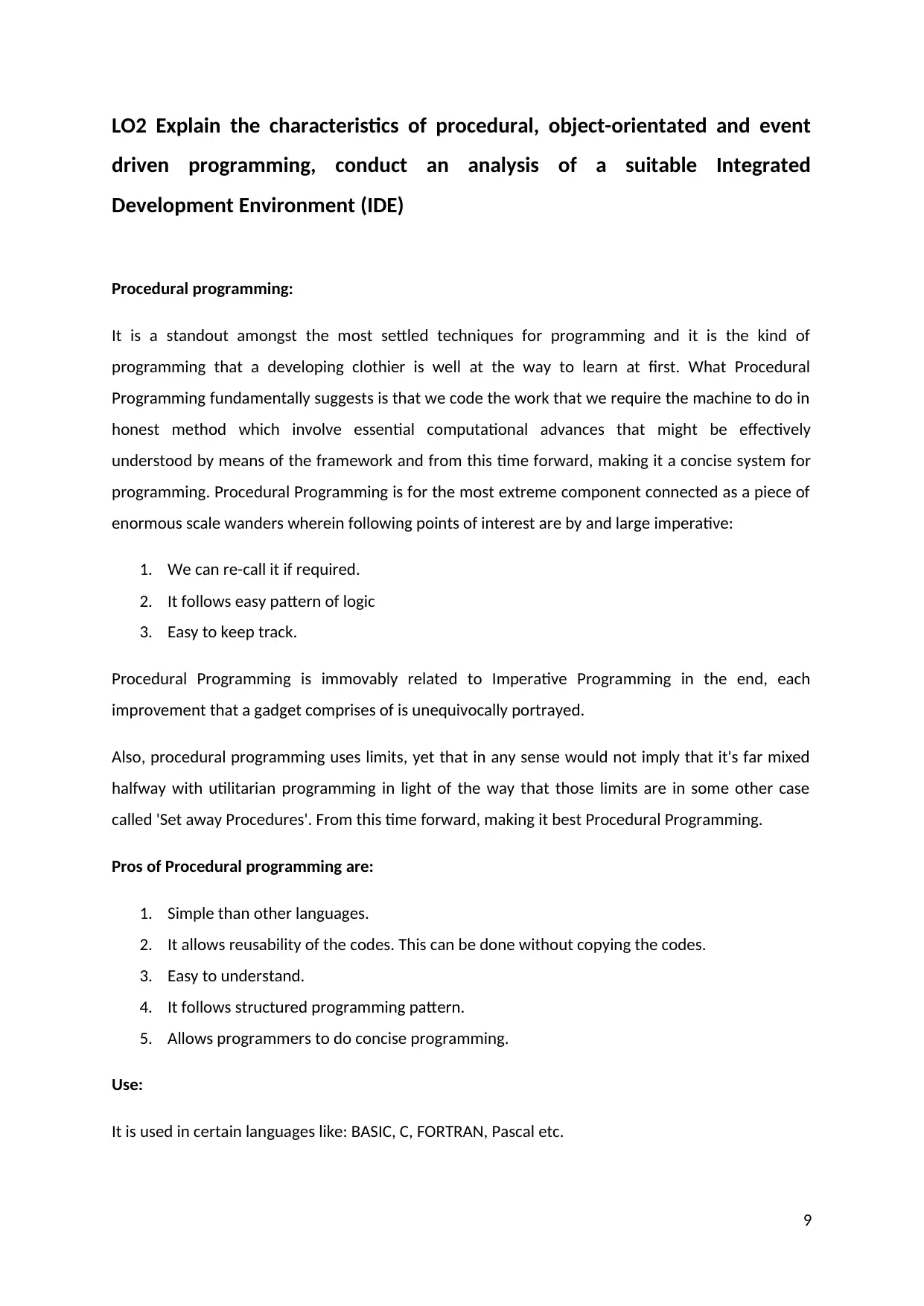
LO2 Explain the characteristics of procedural, object-orientated and event
driven programming, conduct an analysis of a suitable Integrated
Development Environment (IDE)
Procedural programming:
It is a standout amongst the most settled techniques for programming and it is the kind of
programming that a developing clothier is well at the way to learn at first. What Procedural
Programming fundamentally suggests is that we code the work that we require the machine to do in
honest method which involve essential computational advances that might be effectively
understood by means of the framework and from this time forward, making it a concise system for
programming. Procedural Programming is for the most extreme component connected as a piece of
enormous scale wanders wherein following points of interest are by and large imperative:
1. We can re-call it if required.
2. It follows easy pattern of logic
3. Easy to keep track.
Procedural Programming is immovably related to Imperative Programming in the end, each
improvement that a gadget comprises of is unequivocally portrayed.
Also, procedural programming uses limits, yet that in any sense would not imply that it's far mixed
halfway with utilitarian programming in light of the way that those limits are in some other case
called 'Set away Procedures'. From this time forward, making it best Procedural Programming.
Pros of Procedural programming are:
1. Simple than other languages.
2. It allows reusability of the codes. This can be done without copying the codes.
3. Easy to understand.
4. It follows structured programming pattern.
5. Allows programmers to do concise programming.
Use:
It is used in certain languages like: BASIC, C, FORTRAN, Pascal etc.
9
driven programming, conduct an analysis of a suitable Integrated
Development Environment (IDE)
Procedural programming:
It is a standout amongst the most settled techniques for programming and it is the kind of
programming that a developing clothier is well at the way to learn at first. What Procedural
Programming fundamentally suggests is that we code the work that we require the machine to do in
honest method which involve essential computational advances that might be effectively
understood by means of the framework and from this time forward, making it a concise system for
programming. Procedural Programming is for the most extreme component connected as a piece of
enormous scale wanders wherein following points of interest are by and large imperative:
1. We can re-call it if required.
2. It follows easy pattern of logic
3. Easy to keep track.
Procedural Programming is immovably related to Imperative Programming in the end, each
improvement that a gadget comprises of is unequivocally portrayed.
Also, procedural programming uses limits, yet that in any sense would not imply that it's far mixed
halfway with utilitarian programming in light of the way that those limits are in some other case
called 'Set away Procedures'. From this time forward, making it best Procedural Programming.
Pros of Procedural programming are:
1. Simple than other languages.
2. It allows reusability of the codes. This can be done without copying the codes.
3. Easy to understand.
4. It follows structured programming pattern.
5. Allows programmers to do concise programming.
Use:
It is used in certain languages like: BASIC, C, FORTRAN, Pascal etc.
9
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
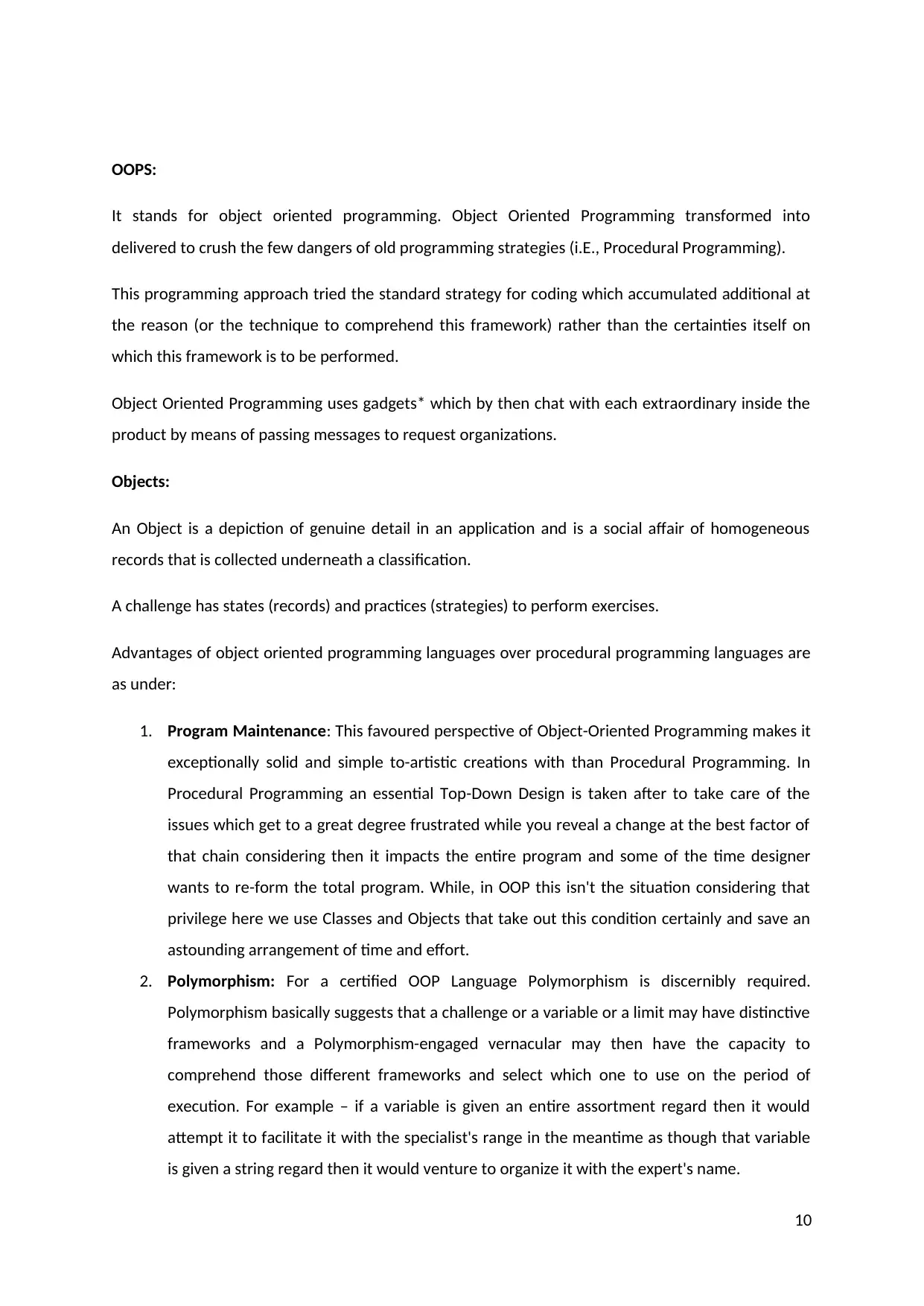
OOPS:
It stands for object oriented programming. Object Oriented Programming transformed into
delivered to crush the few dangers of old programming strategies (i.E., Procedural Programming).
This programming approach tried the standard strategy for coding which accumulated additional at
the reason (or the technique to comprehend this framework) rather than the certainties itself on
which this framework is to be performed.
Object Oriented Programming uses gadgets* which by then chat with each extraordinary inside the
product by means of passing messages to request organizations.
Objects:
An Object is a depiction of genuine detail in an application and is a social affair of homogeneous
records that is collected underneath a classification.
A challenge has states (records) and practices (strategies) to perform exercises.
Advantages of object oriented programming languages over procedural programming languages are
as under:
1. Program Maintenance: This favoured perspective of Object-Oriented Programming makes it
exceptionally solid and simple to-artistic creations with than Procedural Programming. In
Procedural Programming an essential Top-Down Design is taken after to take care of the
issues which get to a great degree frustrated while you reveal a change at the best factor of
that chain considering then it impacts the entire program and some of the time designer
wants to re-form the total program. While, in OOP this isn't the situation considering that
privilege here we use Classes and Objects that take out this condition certainly and save an
astounding arrangement of time and effort.
2. Polymorphism: For a certified OOP Language Polymorphism is discernibly required.
Polymorphism basically suggests that a challenge or a variable or a limit may have distinctive
frameworks and a Polymorphism-engaged vernacular may then have the capacity to
comprehend those different frameworks and select which one to use on the period of
execution. For example – if a variable is given an entire assortment regard then it would
attempt it to facilitate it with the specialist's range in the meantime as though that variable
is given a string regard then it would venture to organize it with the expert's name.
10
It stands for object oriented programming. Object Oriented Programming transformed into
delivered to crush the few dangers of old programming strategies (i.E., Procedural Programming).
This programming approach tried the standard strategy for coding which accumulated additional at
the reason (or the technique to comprehend this framework) rather than the certainties itself on
which this framework is to be performed.
Object Oriented Programming uses gadgets* which by then chat with each extraordinary inside the
product by means of passing messages to request organizations.
Objects:
An Object is a depiction of genuine detail in an application and is a social affair of homogeneous
records that is collected underneath a classification.
A challenge has states (records) and practices (strategies) to perform exercises.
Advantages of object oriented programming languages over procedural programming languages are
as under:
1. Program Maintenance: This favoured perspective of Object-Oriented Programming makes it
exceptionally solid and simple to-artistic creations with than Procedural Programming. In
Procedural Programming an essential Top-Down Design is taken after to take care of the
issues which get to a great degree frustrated while you reveal a change at the best factor of
that chain considering then it impacts the entire program and some of the time designer
wants to re-form the total program. While, in OOP this isn't the situation considering that
privilege here we use Classes and Objects that take out this condition certainly and save an
astounding arrangement of time and effort.
2. Polymorphism: For a certified OOP Language Polymorphism is discernibly required.
Polymorphism basically suggests that a challenge or a variable or a limit may have distinctive
frameworks and a Polymorphism-engaged vernacular may then have the capacity to
comprehend those different frameworks and select which one to use on the period of
execution. For example – if a variable is given an entire assortment regard then it would
attempt it to facilitate it with the specialist's range in the meantime as though that variable
is given a string regard then it would venture to organize it with the expert's name.
10
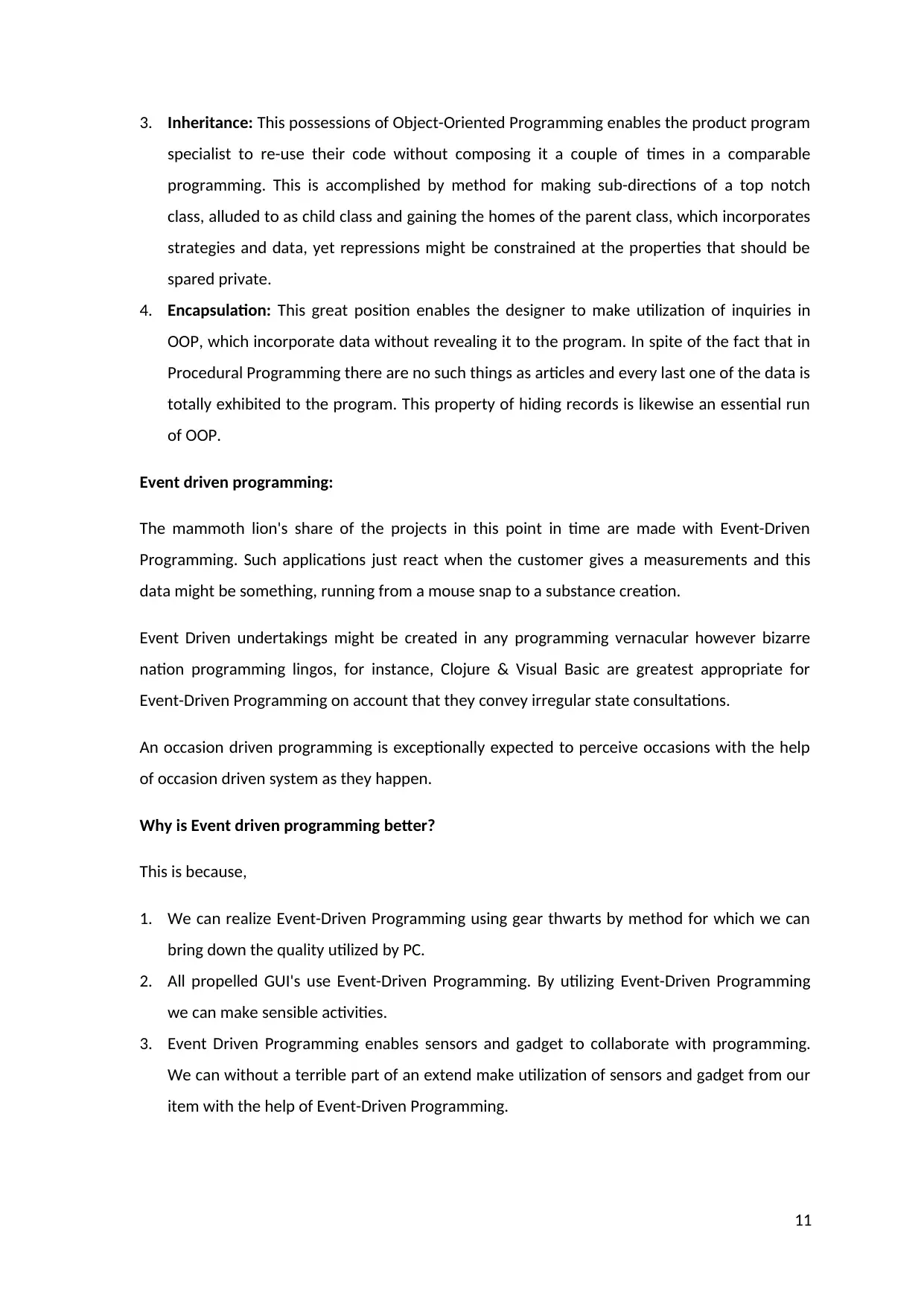
3. Inheritance: This possessions of Object-Oriented Programming enables the product program
specialist to re-use their code without composing it a couple of times in a comparable
programming. This is accomplished by method for making sub-directions of a top notch
class, alluded to as child class and gaining the homes of the parent class, which incorporates
strategies and data, yet repressions might be constrained at the properties that should be
spared private.
4. Encapsulation: This great position enables the designer to make utilization of inquiries in
OOP, which incorporate data without revealing it to the program. In spite of the fact that in
Procedural Programming there are no such things as articles and every last one of the data is
totally exhibited to the program. This property of hiding records is likewise an essential run
of OOP.
Event driven programming:
The mammoth lion's share of the projects in this point in time are made with Event-Driven
Programming. Such applications just react when the customer gives a measurements and this
data might be something, running from a mouse snap to a substance creation.
Event Driven undertakings might be created in any programming vernacular however bizarre
nation programming lingos, for instance, Clojure & Visual Basic are greatest appropriate for
Event-Driven Programming on account that they convey irregular state consultations.
An occasion driven programming is exceptionally expected to perceive occasions with the help
of occasion driven system as they happen.
Why is Event driven programming better?
This is because,
1. We can realize Event-Driven Programming using gear thwarts by method for which we can
bring down the quality utilized by PC.
2. All propelled GUI's use Event-Driven Programming. By utilizing Event-Driven Programming
we can make sensible activities.
3. Event Driven Programming enables sensors and gadget to collaborate with programming.
We can without a terrible part of an extend make utilization of sensors and gadget from our
item with the help of Event-Driven Programming.
11
specialist to re-use their code without composing it a couple of times in a comparable
programming. This is accomplished by method for making sub-directions of a top notch
class, alluded to as child class and gaining the homes of the parent class, which incorporates
strategies and data, yet repressions might be constrained at the properties that should be
spared private.
4. Encapsulation: This great position enables the designer to make utilization of inquiries in
OOP, which incorporate data without revealing it to the program. In spite of the fact that in
Procedural Programming there are no such things as articles and every last one of the data is
totally exhibited to the program. This property of hiding records is likewise an essential run
of OOP.
Event driven programming:
The mammoth lion's share of the projects in this point in time are made with Event-Driven
Programming. Such applications just react when the customer gives a measurements and this
data might be something, running from a mouse snap to a substance creation.
Event Driven undertakings might be created in any programming vernacular however bizarre
nation programming lingos, for instance, Clojure & Visual Basic are greatest appropriate for
Event-Driven Programming on account that they convey irregular state consultations.
An occasion driven programming is exceptionally expected to perceive occasions with the help
of occasion driven system as they happen.
Why is Event driven programming better?
This is because,
1. We can realize Event-Driven Programming using gear thwarts by method for which we can
bring down the quality utilized by PC.
2. All propelled GUI's use Event-Driven Programming. By utilizing Event-Driven Programming
we can make sensible activities.
3. Event Driven Programming enables sensors and gadget to collaborate with programming.
We can without a terrible part of an extend make utilization of sensors and gadget from our
item with the help of Event-Driven Programming.
11
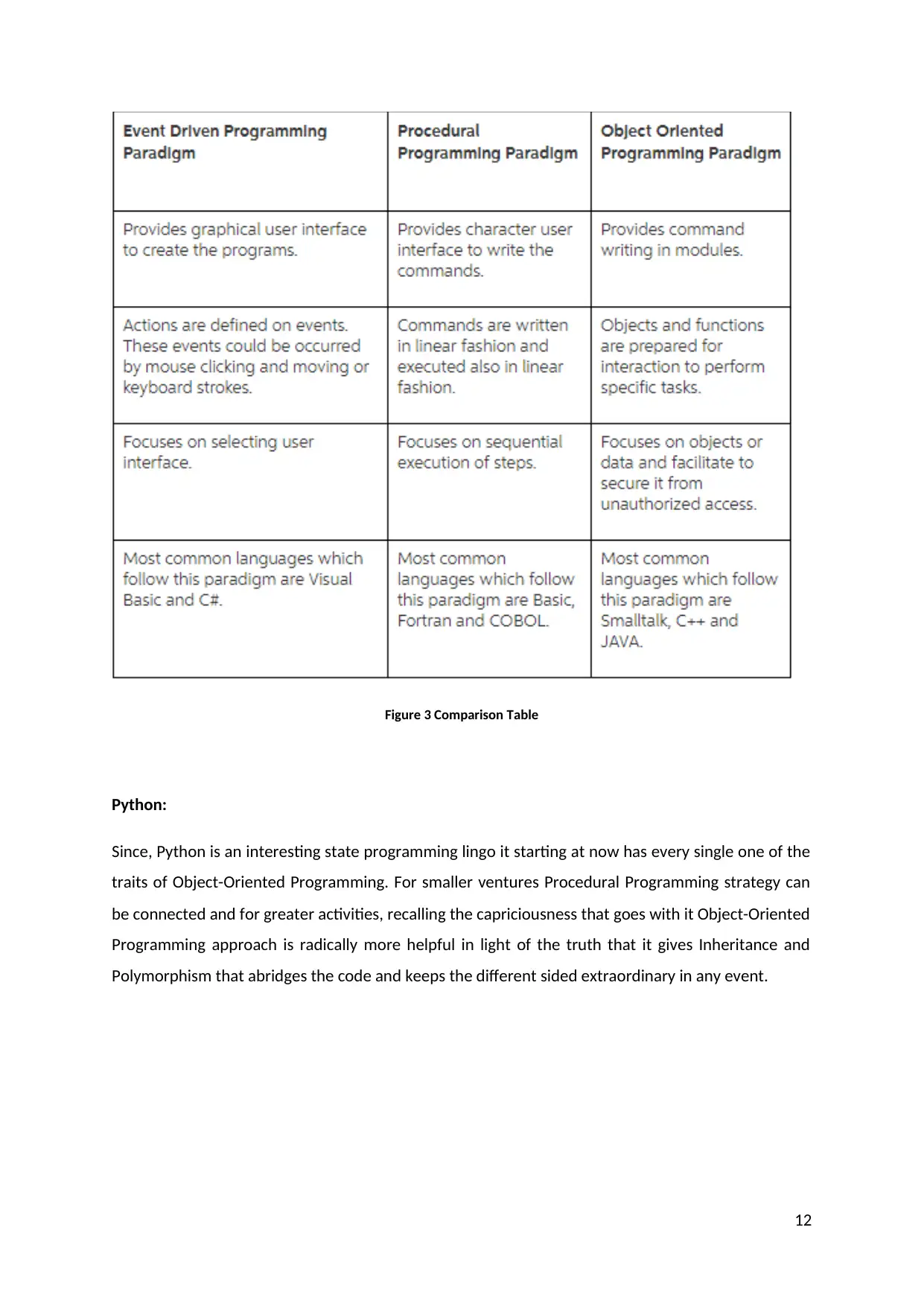
Figure 3 Comparison Table
Python:
Since, Python is an interesting state programming lingo it starting at now has every single one of the
traits of Object-Oriented Programming. For smaller ventures Procedural Programming strategy can
be connected and for greater activities, recalling the capriciousness that goes with it Object-Oriented
Programming approach is radically more helpful in light of the truth that it gives Inheritance and
Polymorphism that abridges the code and keeps the different sided extraordinary in any event.
12
Python:
Since, Python is an interesting state programming lingo it starting at now has every single one of the
traits of Object-Oriented Programming. For smaller ventures Procedural Programming strategy can
be connected and for greater activities, recalling the capriciousness that goes with it Object-Oriented
Programming approach is radically more helpful in light of the truth that it gives Inheritance and
Polymorphism that abridges the code and keeps the different sided extraordinary in any event.
12
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
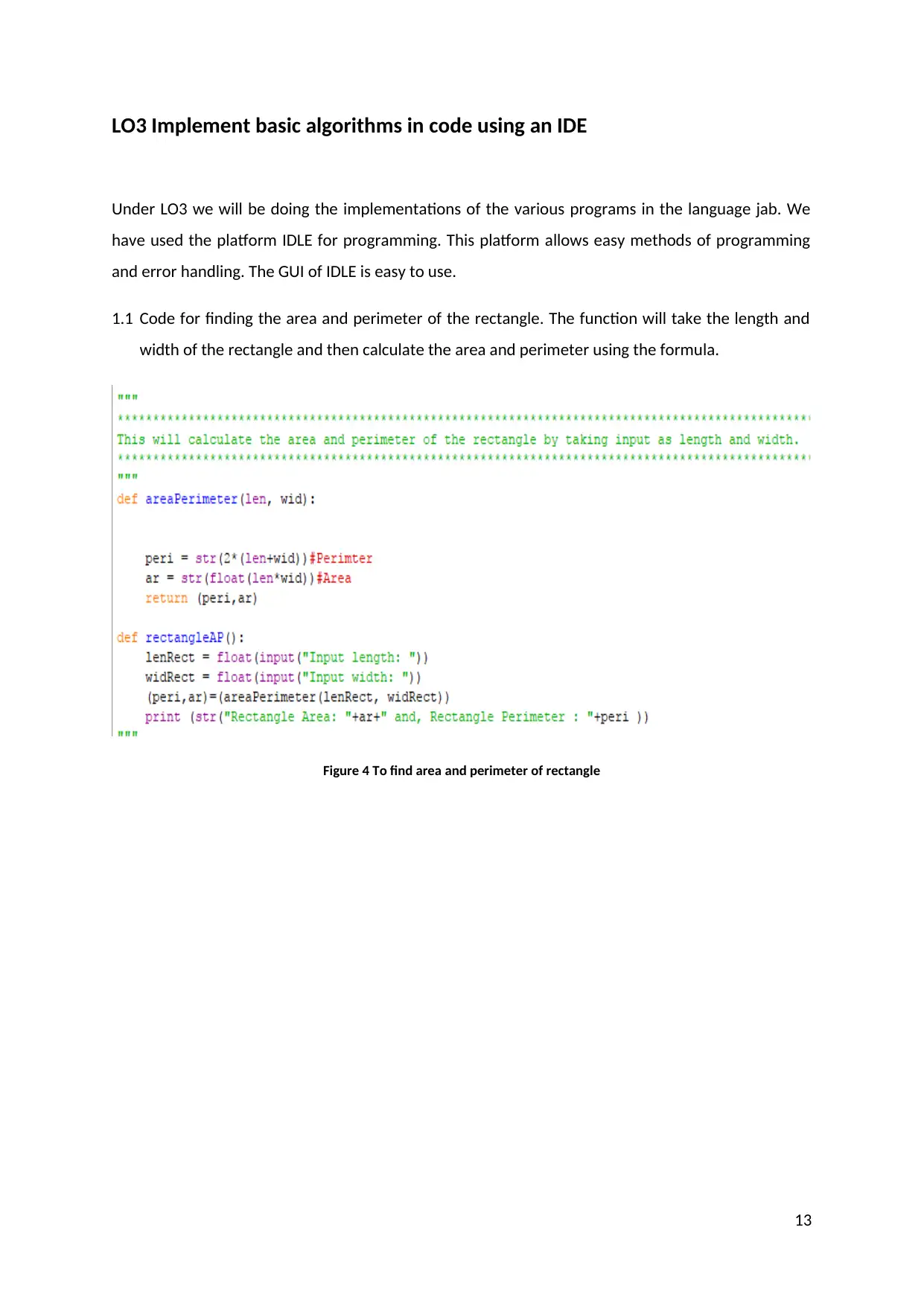
LO3 Implement basic algorithms in code using an IDE
Under LO3 we will be doing the implementations of the various programs in the language jab. We
have used the platform IDLE for programming. This platform allows easy methods of programming
and error handling. The GUI of IDLE is easy to use.
1.1 Code for finding the area and perimeter of the rectangle. The function will take the length and
width of the rectangle and then calculate the area and perimeter using the formula.
Figure 4 To find area and perimeter of rectangle
13
Under LO3 we will be doing the implementations of the various programs in the language jab. We
have used the platform IDLE for programming. This platform allows easy methods of programming
and error handling. The GUI of IDLE is easy to use.
1.1 Code for finding the area and perimeter of the rectangle. The function will take the length and
width of the rectangle and then calculate the area and perimeter using the formula.
Figure 4 To find area and perimeter of rectangle
13
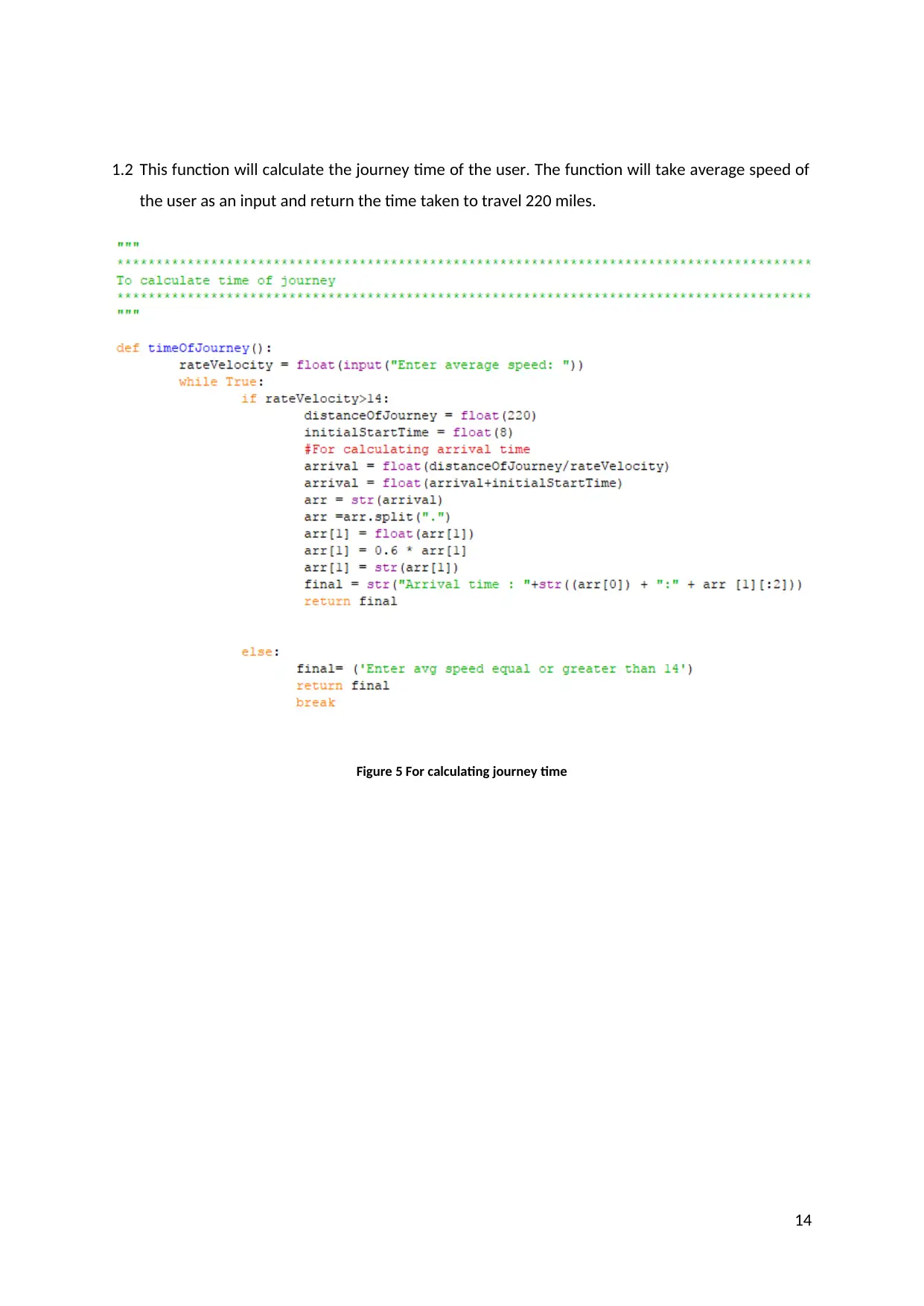
1.2 This function will calculate the journey time of the user. The function will take average speed of
the user as an input and return the time taken to travel 220 miles.
Figure 5 For calculating journey time
14
the user as an input and return the time taken to travel 220 miles.
Figure 5 For calculating journey time
14
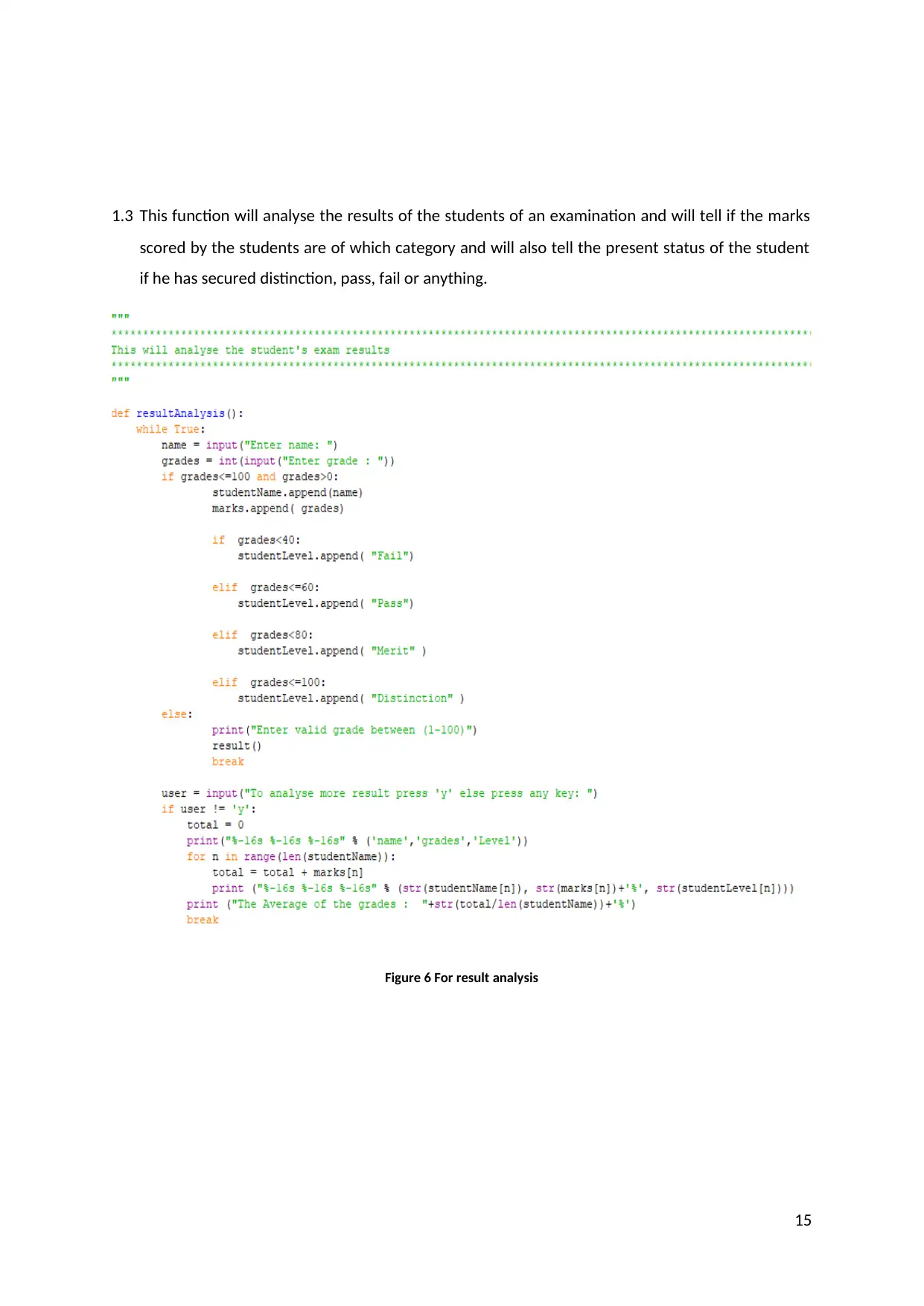
1.3 This function will analyse the results of the students of an examination and will tell if the marks
scored by the students are of which category and will also tell the present status of the student
if he has secured distinction, pass, fail or anything.
Figure 6 For result analysis
15
scored by the students are of which category and will also tell the present status of the student
if he has secured distinction, pass, fail or anything.
Figure 6 For result analysis
15
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
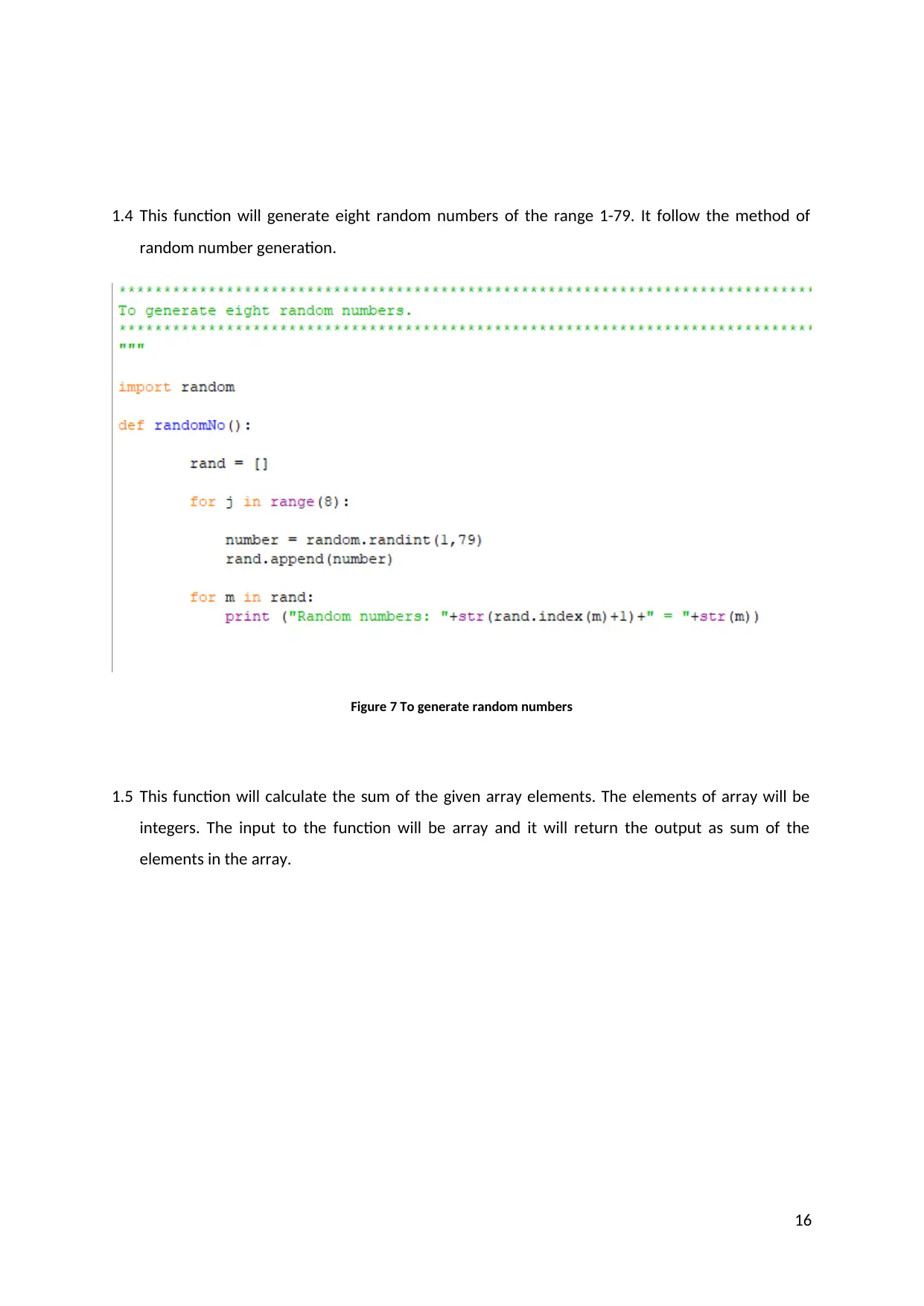
1.4 This function will generate eight random numbers of the range 1-79. It follow the method of
random number generation.
Figure 7 To generate random numbers
1.5 This function will calculate the sum of the given array elements. The elements of array will be
integers. The input to the function will be array and it will return the output as sum of the
elements in the array.
16
random number generation.
Figure 7 To generate random numbers
1.5 This function will calculate the sum of the given array elements. The elements of array will be
integers. The input to the function will be array and it will return the output as sum of the
elements in the array.
16
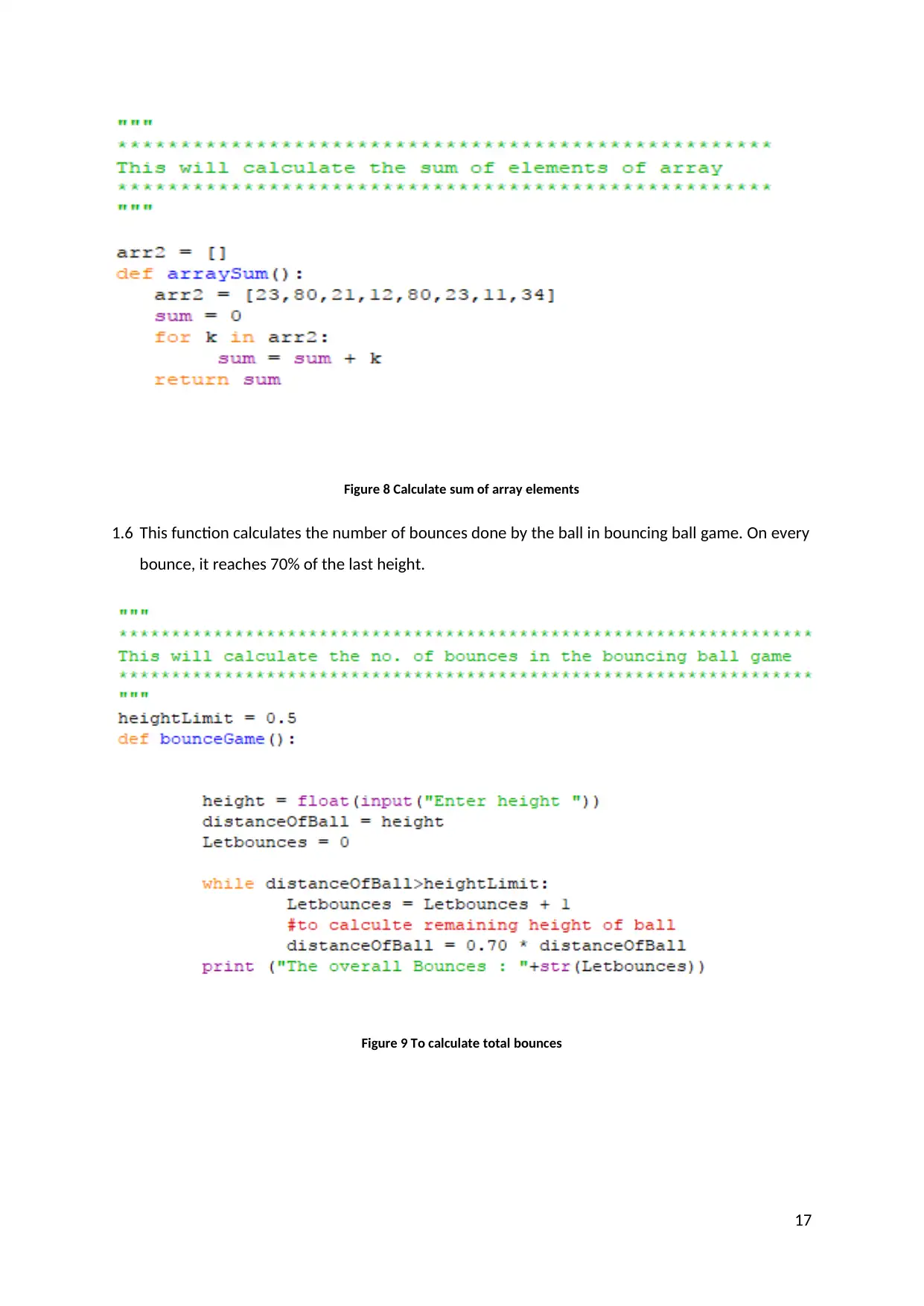
Figure 8 Calculate sum of array elements
1.6 This function calculates the number of bounces done by the ball in bouncing ball game. On every
bounce, it reaches 70% of the last height.
Figure 9 To calculate total bounces
17
1.6 This function calculates the number of bounces done by the ball in bouncing ball game. On every
bounce, it reaches 70% of the last height.
Figure 9 To calculate total bounces
17
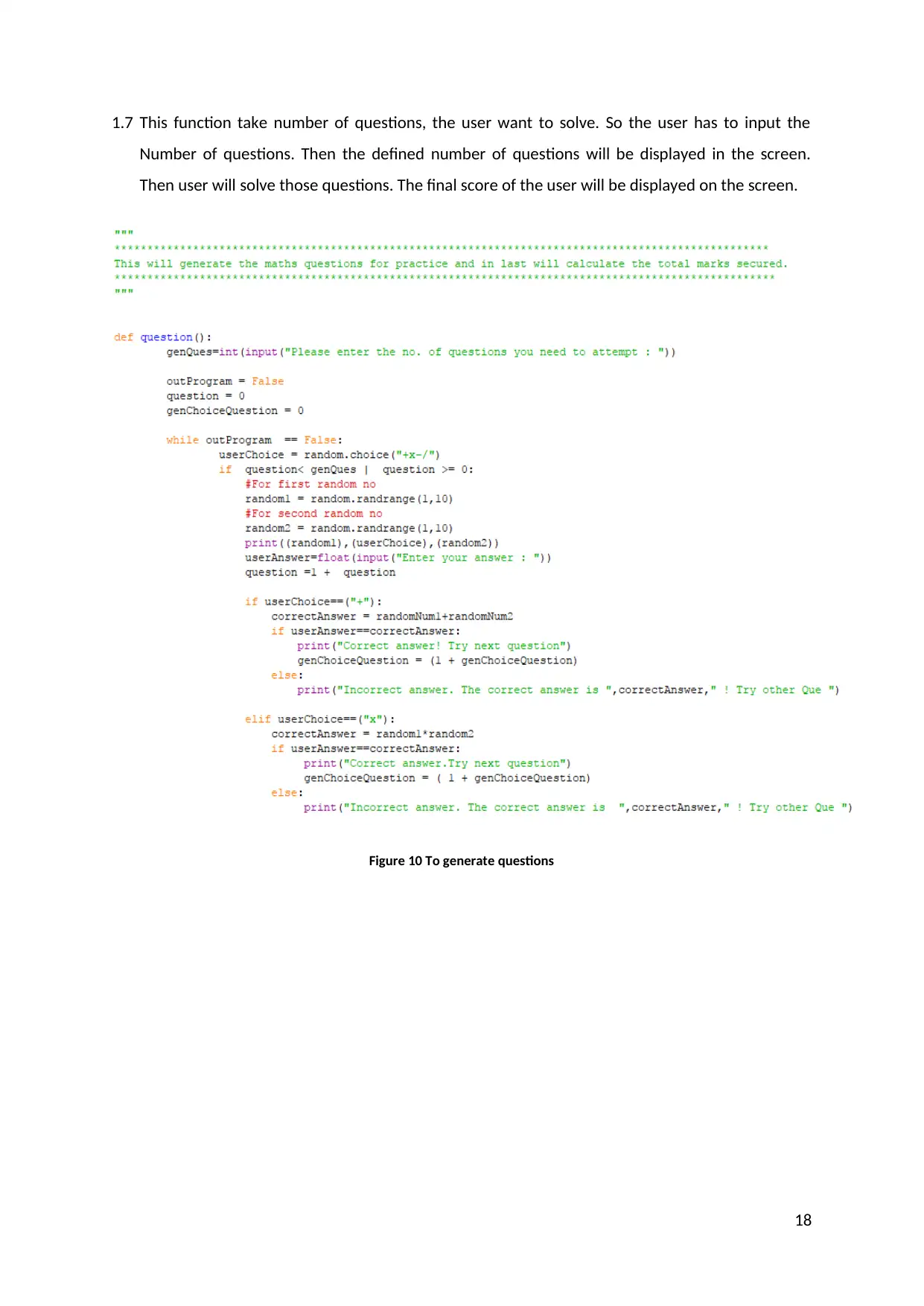
1.7 This function take number of questions, the user want to solve. So the user has to input the
Number of questions. Then the defined number of questions will be displayed in the screen.
Then user will solve those questions. The final score of the user will be displayed on the screen.
Figure 10 To generate questions
18
Number of questions. Then the defined number of questions will be displayed in the screen.
Then user will solve those questions. The final score of the user will be displayed on the screen.
Figure 10 To generate questions
18
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
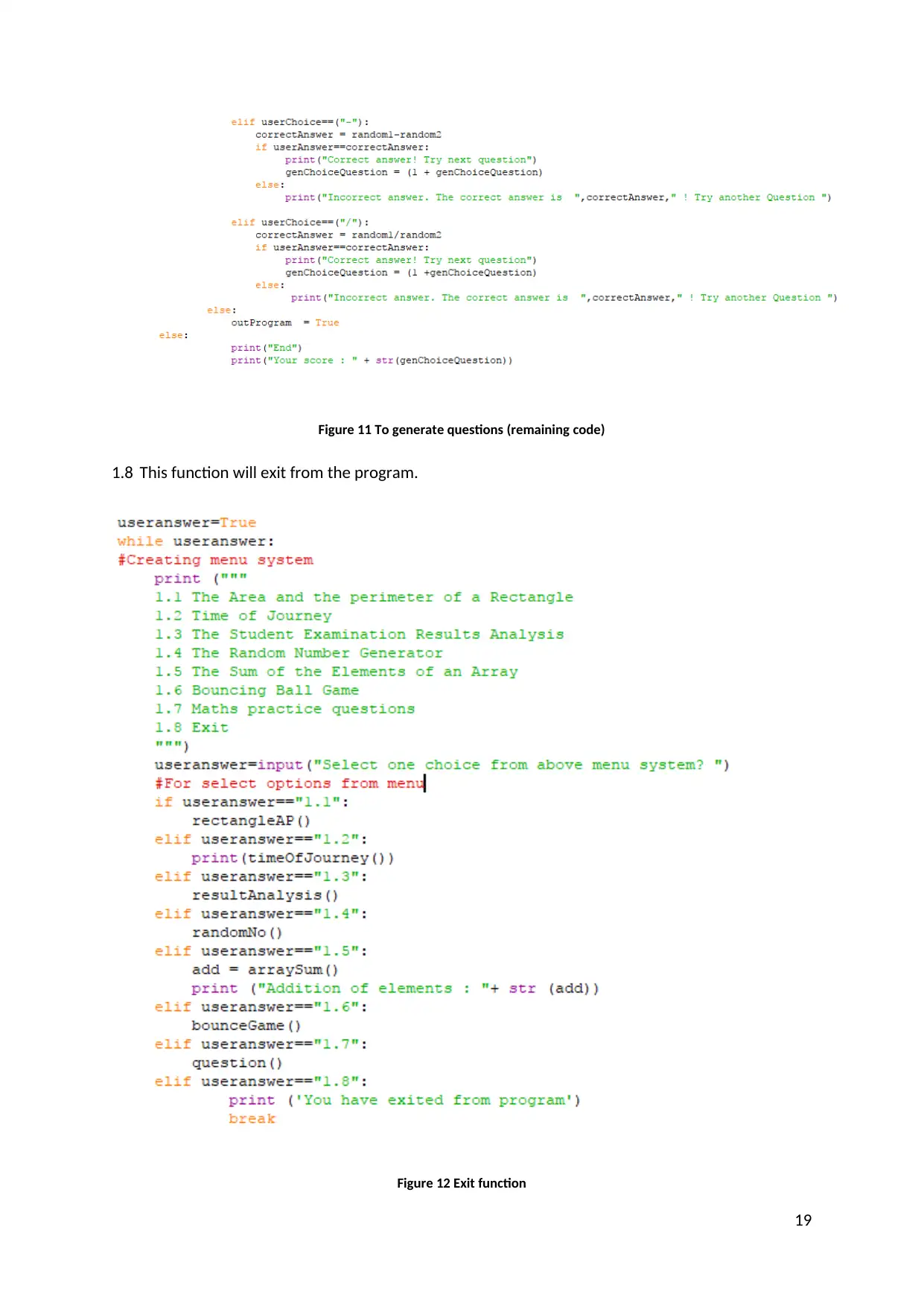
Figure 11 To generate questions (remaining code)
1.8 This function will exit from the program.
Figure 12 Exit function
19
1.8 This function will exit from the program.
Figure 12 Exit function
19
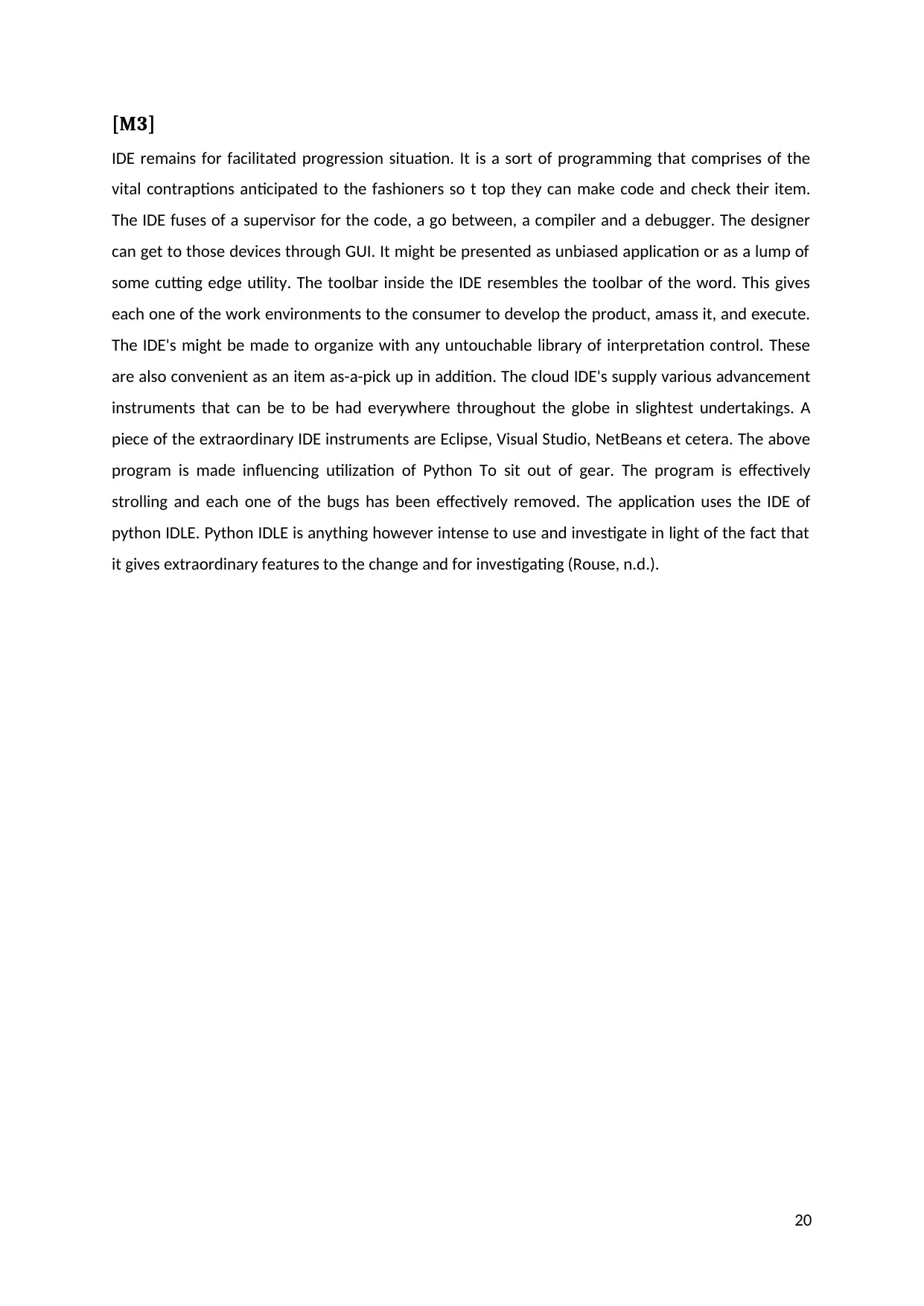
[M3]
IDE remains for facilitated progression situation. It is a sort of programming that comprises of the
vital contraptions anticipated to the fashioners so t top they can make code and check their item.
The IDE fuses of a supervisor for the code, a go between, a compiler and a debugger. The designer
can get to those devices through GUI. It might be presented as unbiased application or as a lump of
some cutting edge utility. The toolbar inside the IDE resembles the toolbar of the word. This gives
each one of the work environments to the consumer to develop the product, amass it, and execute.
The IDE's might be made to organize with any untouchable library of interpretation control. These
are also convenient as an item as-a-pick up in addition. The cloud IDE's supply various advancement
instruments that can be to be had everywhere throughout the globe in slightest undertakings. A
piece of the extraordinary IDE instruments are Eclipse, Visual Studio, NetBeans et cetera. The above
program is made influencing utilization of Python To sit out of gear. The program is effectively
strolling and each one of the bugs has been effectively removed. The application uses the IDE of
python IDLE. Python IDLE is anything however intense to use and investigate in light of the fact that
it gives extraordinary features to the change and for investigating (Rouse, n.d.).
20
IDE remains for facilitated progression situation. It is a sort of programming that comprises of the
vital contraptions anticipated to the fashioners so t top they can make code and check their item.
The IDE fuses of a supervisor for the code, a go between, a compiler and a debugger. The designer
can get to those devices through GUI. It might be presented as unbiased application or as a lump of
some cutting edge utility. The toolbar inside the IDE resembles the toolbar of the word. This gives
each one of the work environments to the consumer to develop the product, amass it, and execute.
The IDE's might be made to organize with any untouchable library of interpretation control. These
are also convenient as an item as-a-pick up in addition. The cloud IDE's supply various advancement
instruments that can be to be had everywhere throughout the globe in slightest undertakings. A
piece of the extraordinary IDE instruments are Eclipse, Visual Studio, NetBeans et cetera. The above
program is made influencing utilization of Python To sit out of gear. The program is effectively
strolling and each one of the bugs has been effectively removed. The application uses the IDE of
python IDLE. Python IDLE is anything however intense to use and investigate in light of the fact that
it gives extraordinary features to the change and for investigating (Rouse, n.d.).
20
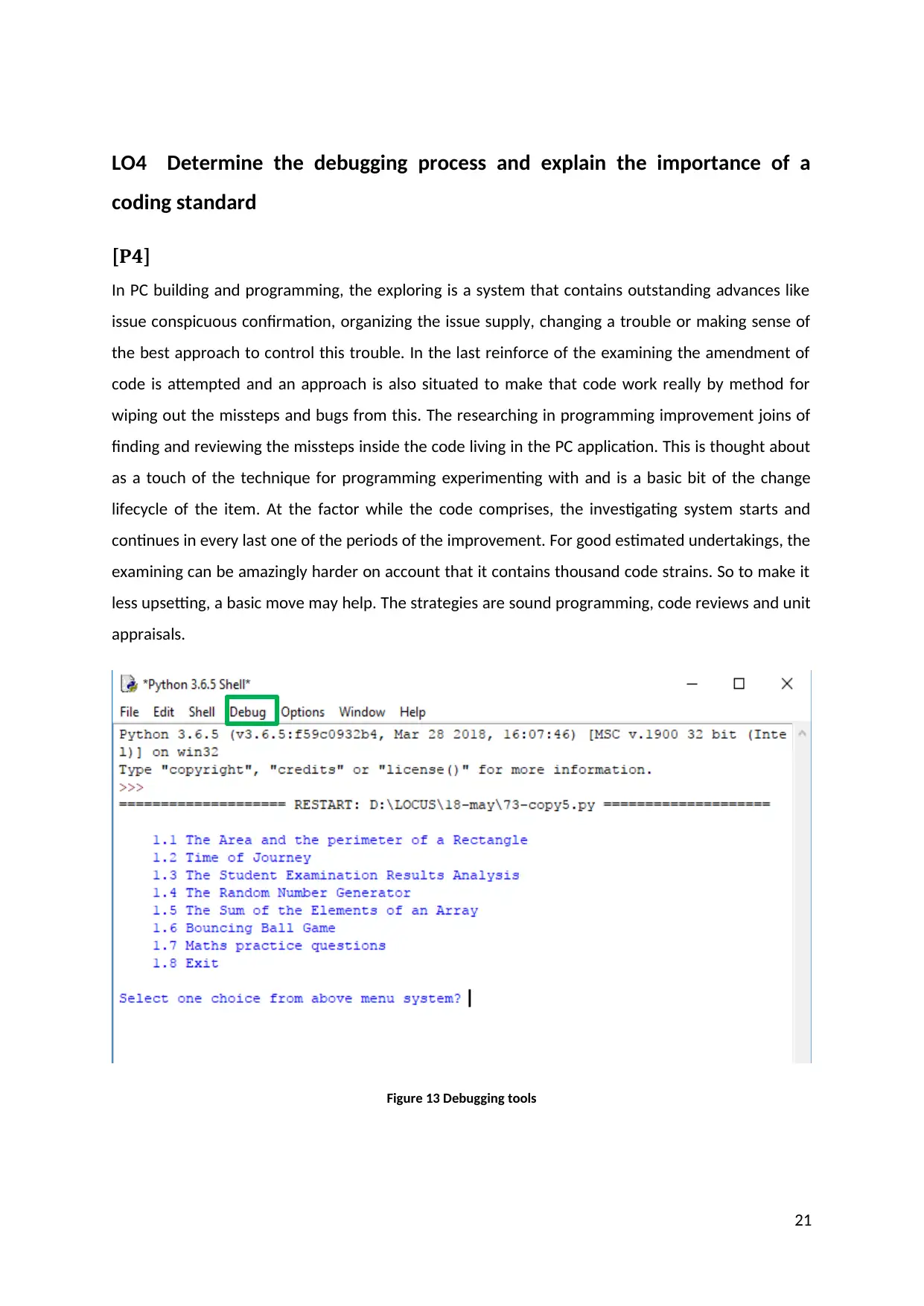
LO4 Determine the debugging process and explain the importance of a
coding standard
[P4]
In PC building and programming, the exploring is a system that contains outstanding advances like
issue conspicuous confirmation, organizing the issue supply, changing a trouble or making sense of
the best approach to control this trouble. In the last reinforce of the examining the amendment of
code is attempted and an approach is also situated to make that code work really by method for
wiping out the missteps and bugs from this. The researching in programming improvement joins of
finding and reviewing the missteps inside the code living in the PC application. This is thought about
as a touch of the technique for programming experimenting with and is a basic bit of the change
lifecycle of the item. At the factor while the code comprises, the investigating system starts and
continues in every last one of the periods of the improvement. For good estimated undertakings, the
examining can be amazingly harder on account that it contains thousand code strains. So to make it
less upsetting, a basic move may help. The strategies are sound programming, code reviews and unit
appraisals.
Figure 13 Debugging tools
21
coding standard
[P4]
In PC building and programming, the exploring is a system that contains outstanding advances like
issue conspicuous confirmation, organizing the issue supply, changing a trouble or making sense of
the best approach to control this trouble. In the last reinforce of the examining the amendment of
code is attempted and an approach is also situated to make that code work really by method for
wiping out the missteps and bugs from this. The researching in programming improvement joins of
finding and reviewing the missteps inside the code living in the PC application. This is thought about
as a touch of the technique for programming experimenting with and is a basic bit of the change
lifecycle of the item. At the factor while the code comprises, the investigating system starts and
continues in every last one of the periods of the improvement. For good estimated undertakings, the
examining can be amazingly harder on account that it contains thousand code strains. So to make it
less upsetting, a basic move may help. The strategies are sound programming, code reviews and unit
appraisals.
Figure 13 Debugging tools
21
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
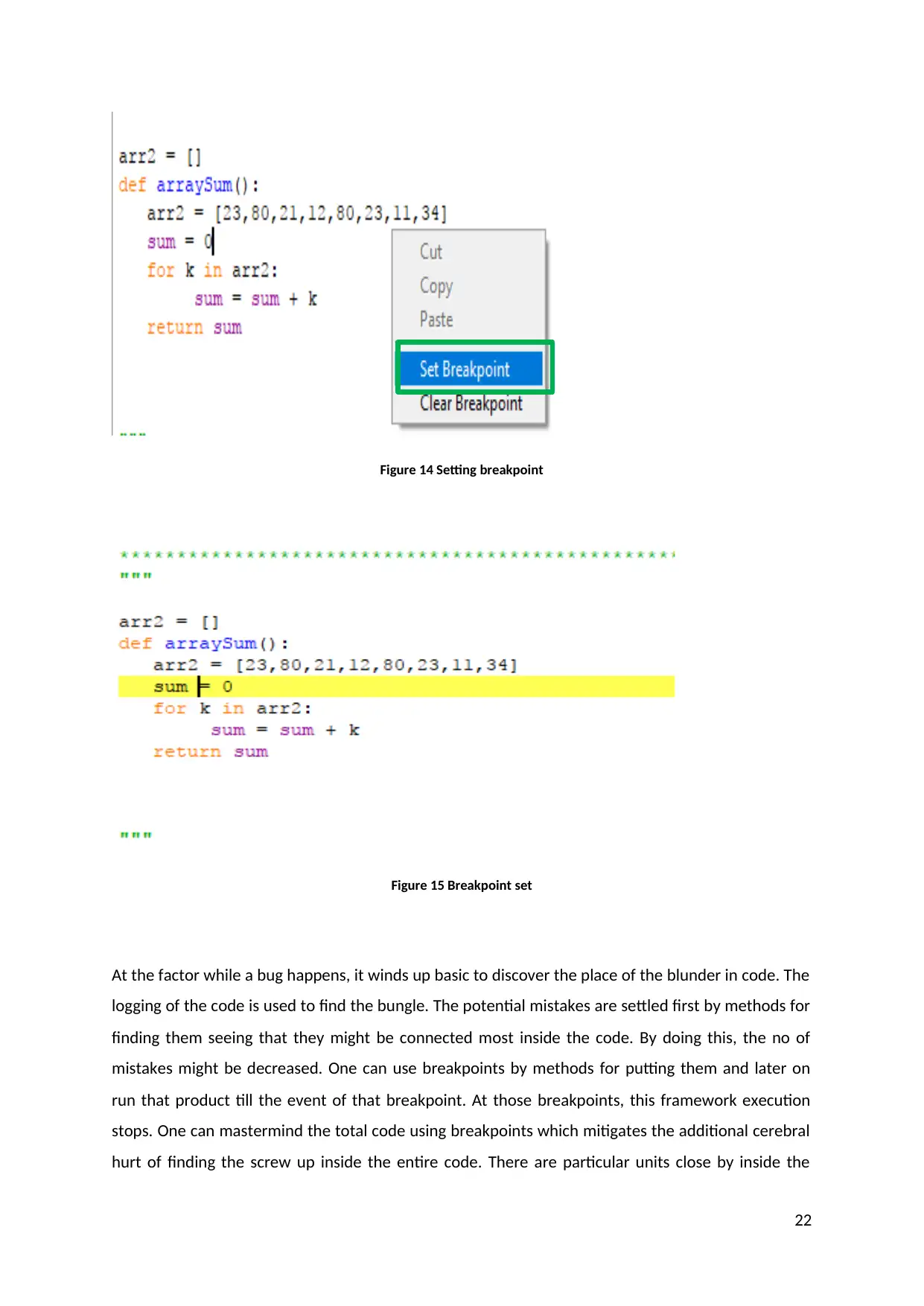
Figure 14 Setting breakpoint
Figure 15 Breakpoint set
At the factor while a bug happens, it winds up basic to discover the place of the blunder in code. The
logging of the code is used to find the bungle. The potential mistakes are settled first by methods for
finding them seeing that they might be connected most inside the code. By doing this, the no of
mistakes might be decreased. One can use breakpoints by methods for putting them and later on
run that product till the event of that breakpoint. At those breakpoints, this framework execution
stops. One can mastermind the total code using breakpoints which mitigates the additional cerebral
hurt of finding the screw up inside the entire code. There are particular units close by inside the
22
Figure 15 Breakpoint set
At the factor while a bug happens, it winds up basic to discover the place of the blunder in code. The
logging of the code is used to find the bungle. The potential mistakes are settled first by methods for
finding them seeing that they might be connected most inside the code. By doing this, the no of
mistakes might be decreased. One can use breakpoints by methods for putting them and later on
run that product till the event of that breakpoint. At those breakpoints, this framework execution
stops. One can mastermind the total code using breakpoints which mitigates the additional cerebral
hurt of finding the screw up inside the entire code. There are particular units close by inside the
22
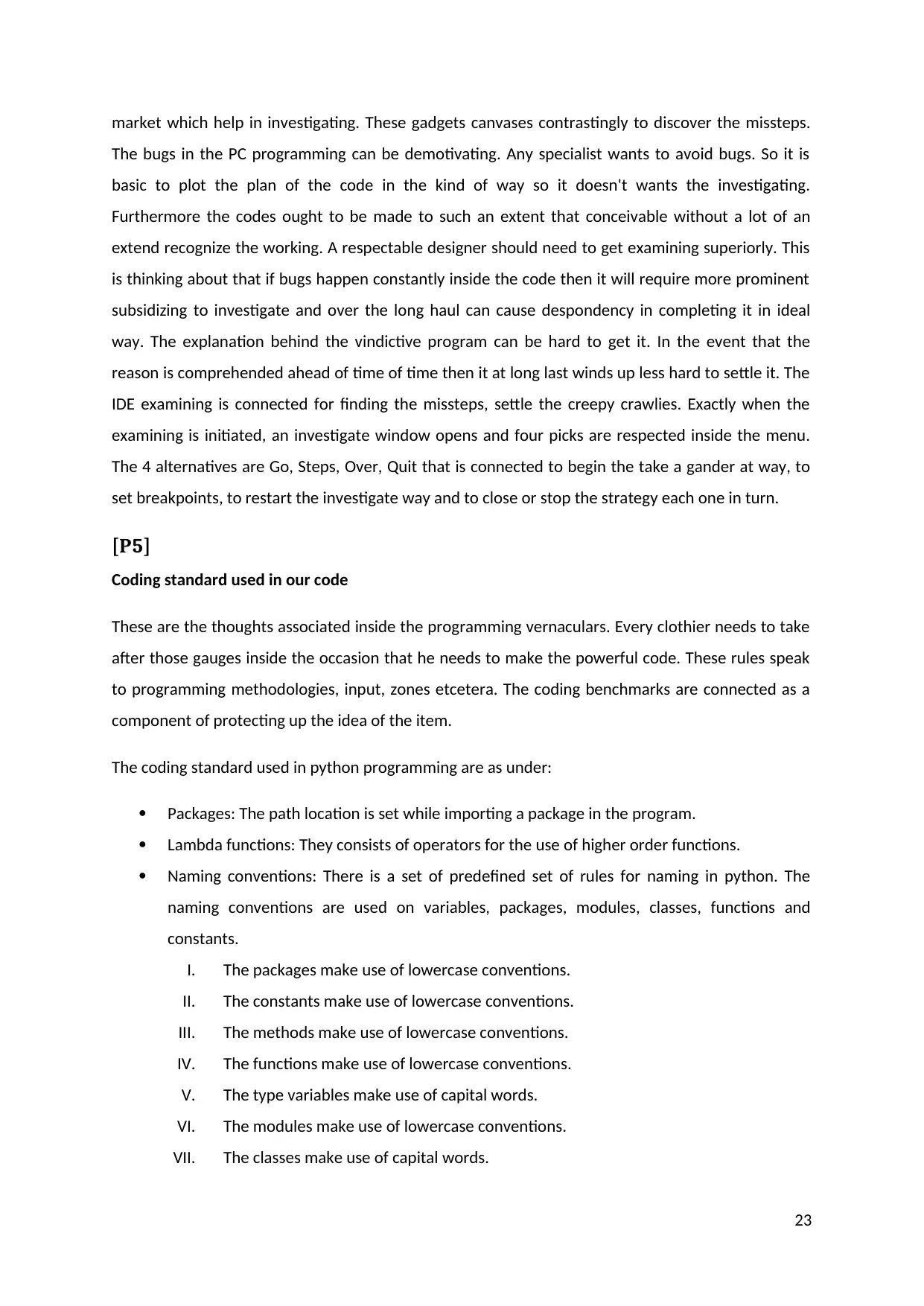
market which help in investigating. These gadgets canvases contrastingly to discover the missteps.
The bugs in the PC programming can be demotivating. Any specialist wants to avoid bugs. So it is
basic to plot the plan of the code in the kind of way so it doesn't wants the investigating.
Furthermore the codes ought to be made to such an extent that conceivable without a lot of an
extend recognize the working. A respectable designer should need to get examining superiorly. This
is thinking about that if bugs happen constantly inside the code then it will require more prominent
subsidizing to investigate and over the long haul can cause despondency in completing it in ideal
way. The explanation behind the vindictive program can be hard to get it. In the event that the
reason is comprehended ahead of time of time then it at long last winds up less hard to settle it. The
IDE examining is connected for finding the missteps, settle the creepy crawlies. Exactly when the
examining is initiated, an investigate window opens and four picks are respected inside the menu.
The 4 alternatives are Go, Steps, Over, Quit that is connected to begin the take a gander at way, to
set breakpoints, to restart the investigate way and to close or stop the strategy each one in turn.
[P5]
Coding standard used in our code
These are the thoughts associated inside the programming vernaculars. Every clothier needs to take
after those gauges inside the occasion that he needs to make the powerful code. These rules speak
to programming methodologies, input, zones etcetera. The coding benchmarks are connected as a
component of protecting up the idea of the item.
The coding standard used in python programming are as under:
Packages: The path location is set while importing a package in the program.
Lambda functions: They consists of operators for the use of higher order functions.
Naming conventions: There is a set of predefined set of rules for naming in python. The
naming conventions are used on variables, packages, modules, classes, functions and
constants.
I. The packages make use of lowercase conventions.
II. The constants make use of lowercase conventions.
III. The methods make use of lowercase conventions.
IV. The functions make use of lowercase conventions.
V. The type variables make use of capital words.
VI. The modules make use of lowercase conventions.
VII. The classes make use of capital words.
23
The bugs in the PC programming can be demotivating. Any specialist wants to avoid bugs. So it is
basic to plot the plan of the code in the kind of way so it doesn't wants the investigating.
Furthermore the codes ought to be made to such an extent that conceivable without a lot of an
extend recognize the working. A respectable designer should need to get examining superiorly. This
is thinking about that if bugs happen constantly inside the code then it will require more prominent
subsidizing to investigate and over the long haul can cause despondency in completing it in ideal
way. The explanation behind the vindictive program can be hard to get it. In the event that the
reason is comprehended ahead of time of time then it at long last winds up less hard to settle it. The
IDE examining is connected for finding the missteps, settle the creepy crawlies. Exactly when the
examining is initiated, an investigate window opens and four picks are respected inside the menu.
The 4 alternatives are Go, Steps, Over, Quit that is connected to begin the take a gander at way, to
set breakpoints, to restart the investigate way and to close or stop the strategy each one in turn.
[P5]
Coding standard used in our code
These are the thoughts associated inside the programming vernaculars. Every clothier needs to take
after those gauges inside the occasion that he needs to make the powerful code. These rules speak
to programming methodologies, input, zones etcetera. The coding benchmarks are connected as a
component of protecting up the idea of the item.
The coding standard used in python programming are as under:
Packages: The path location is set while importing a package in the program.
Lambda functions: They consists of operators for the use of higher order functions.
Naming conventions: There is a set of predefined set of rules for naming in python. The
naming conventions are used on variables, packages, modules, classes, functions and
constants.
I. The packages make use of lowercase conventions.
II. The constants make use of lowercase conventions.
III. The methods make use of lowercase conventions.
IV. The functions make use of lowercase conventions.
V. The type variables make use of capital words.
VI. The modules make use of lowercase conventions.
VII. The classes make use of capital words.
23
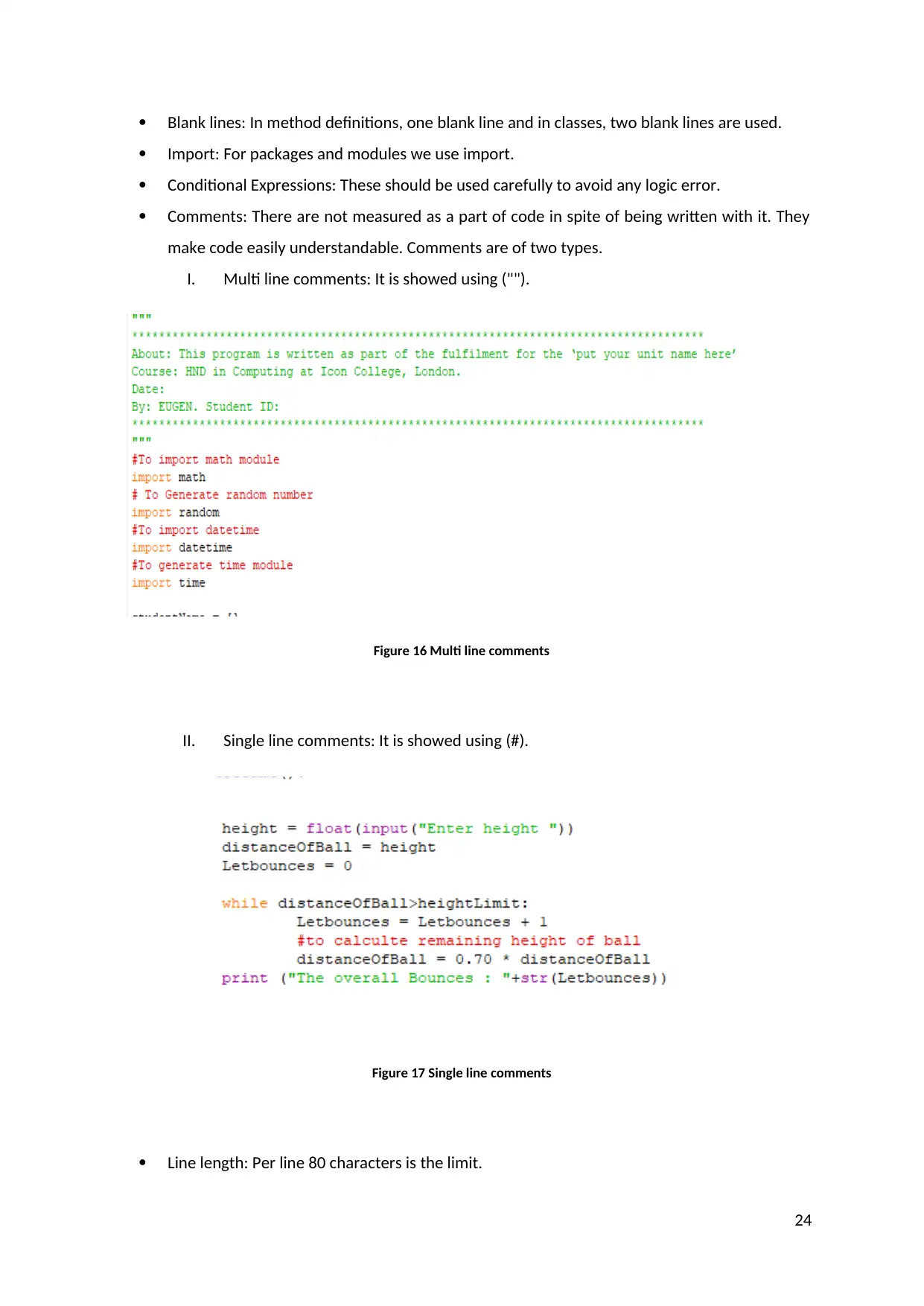
Blank lines: In method definitions, one blank line and in classes, two blank lines are used.
Import: For packages and modules we use import.
Conditional Expressions: These should be used carefully to avoid any logic error.
Comments: There are not measured as a part of code in spite of being written with it. They
make code easily understandable. Comments are of two types.
I. Multi line comments: It is showed using ("").
Figure 16 Multi line comments
II. Single line comments: It is showed using (#).
Figure 17 Single line comments
Line length: Per line 80 characters is the limit.
24
Import: For packages and modules we use import.
Conditional Expressions: These should be used carefully to avoid any logic error.
Comments: There are not measured as a part of code in spite of being written with it. They
make code easily understandable. Comments are of two types.
I. Multi line comments: It is showed using ("").
Figure 16 Multi line comments
II. Single line comments: It is showed using (#).
Figure 17 Single line comments
Line length: Per line 80 characters is the limit.
24
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
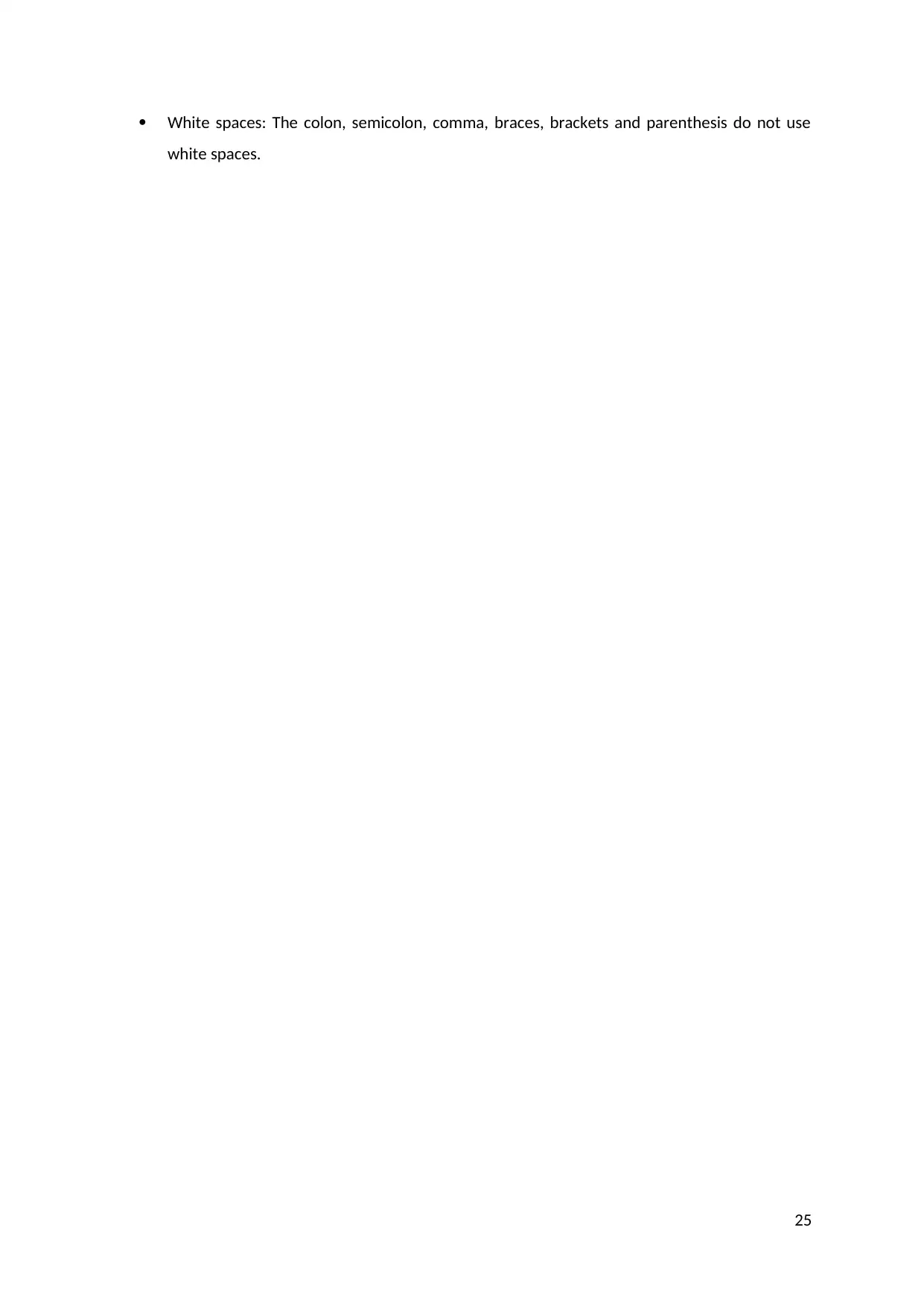
White spaces: The colon, semicolon, comma, braces, brackets and parenthesis do not use
white spaces.
25
white spaces.
25
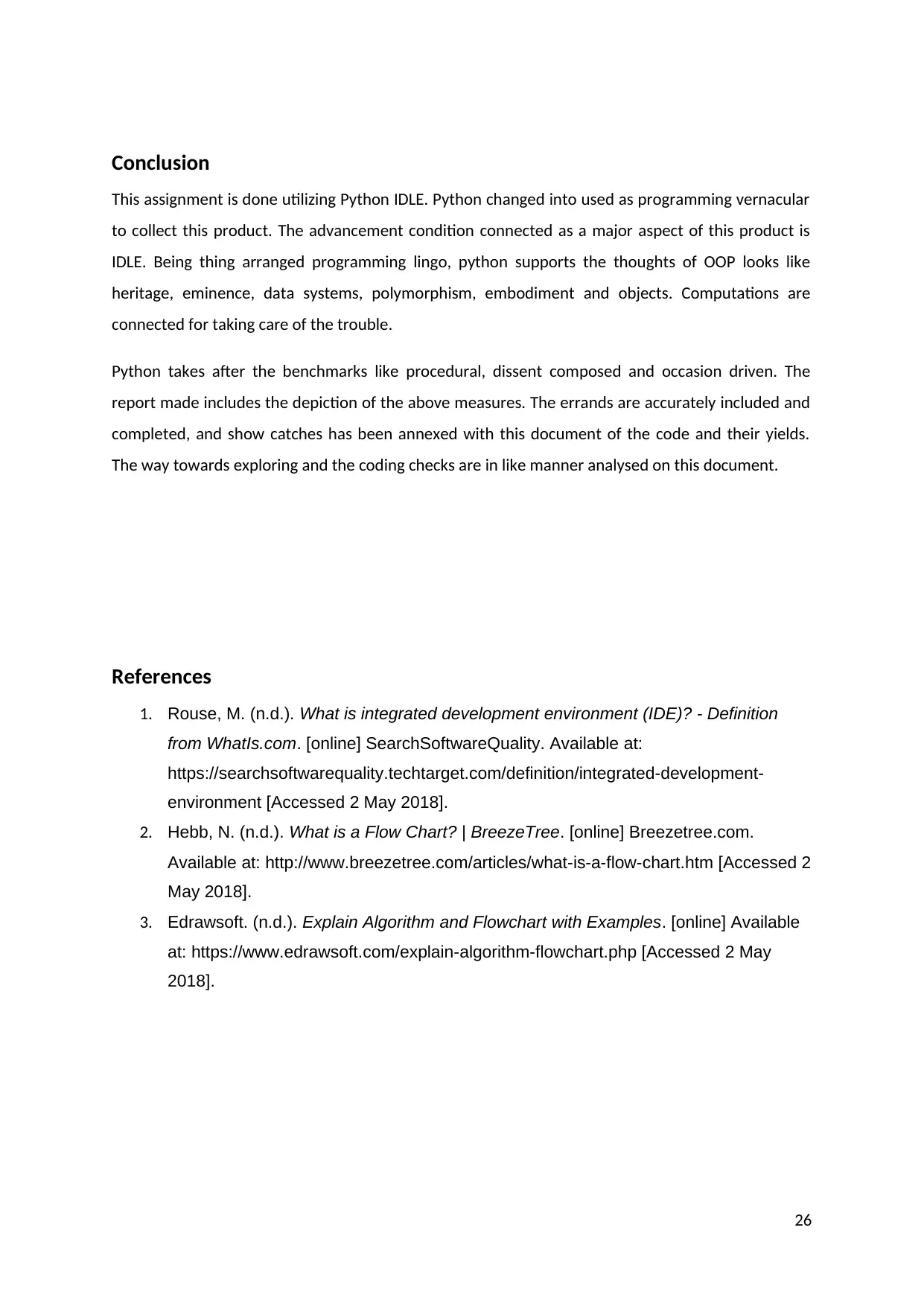
Conclusion
This assignment is done utilizing Python IDLE. Python changed into used as programming vernacular
to collect this product. The advancement condition connected as a major aspect of this product is
IDLE. Being thing arranged programming lingo, python supports the thoughts of OOP looks like
heritage, eminence, data systems, polymorphism, embodiment and objects. Computations are
connected for taking care of the trouble.
Python takes after the benchmarks like procedural, dissent composed and occasion driven. The
report made includes the depiction of the above measures. The errands are accurately included and
completed, and show catches has been annexed with this document of the code and their yields.
The way towards exploring and the coding checks are in like manner analysed on this document.
References
1. Rouse, M. (n.d.). What is integrated development environment (IDE)? - Definition
from WhatIs.com. [online] SearchSoftwareQuality. Available at:
https://searchsoftwarequality.techtarget.com/definition/integrated-development-
environment [Accessed 2 May 2018].
2. Hebb, N. (n.d.). What is a Flow Chart? | BreezeTree. [online] Breezetree.com.
Available at: http://www.breezetree.com/articles/what-is-a-flow-chart.htm [Accessed 2
May 2018].
3. Edrawsoft. (n.d.). Explain Algorithm and Flowchart with Examples. [online] Available
at: https://www.edrawsoft.com/explain-algorithm-flowchart.php [Accessed 2 May
2018].
26
This assignment is done utilizing Python IDLE. Python changed into used as programming vernacular
to collect this product. The advancement condition connected as a major aspect of this product is
IDLE. Being thing arranged programming lingo, python supports the thoughts of OOP looks like
heritage, eminence, data systems, polymorphism, embodiment and objects. Computations are
connected for taking care of the trouble.
Python takes after the benchmarks like procedural, dissent composed and occasion driven. The
report made includes the depiction of the above measures. The errands are accurately included and
completed, and show catches has been annexed with this document of the code and their yields.
The way towards exploring and the coding checks are in like manner analysed on this document.
References
1. Rouse, M. (n.d.). What is integrated development environment (IDE)? - Definition
from WhatIs.com. [online] SearchSoftwareQuality. Available at:
https://searchsoftwarequality.techtarget.com/definition/integrated-development-
environment [Accessed 2 May 2018].
2. Hebb, N. (n.d.). What is a Flow Chart? | BreezeTree. [online] Breezetree.com.
Available at: http://www.breezetree.com/articles/what-is-a-flow-chart.htm [Accessed 2
May 2018].
3. Edrawsoft. (n.d.). Explain Algorithm and Flowchart with Examples. [online] Available
at: https://www.edrawsoft.com/explain-algorithm-flowchart.php [Accessed 2 May
2018].
26
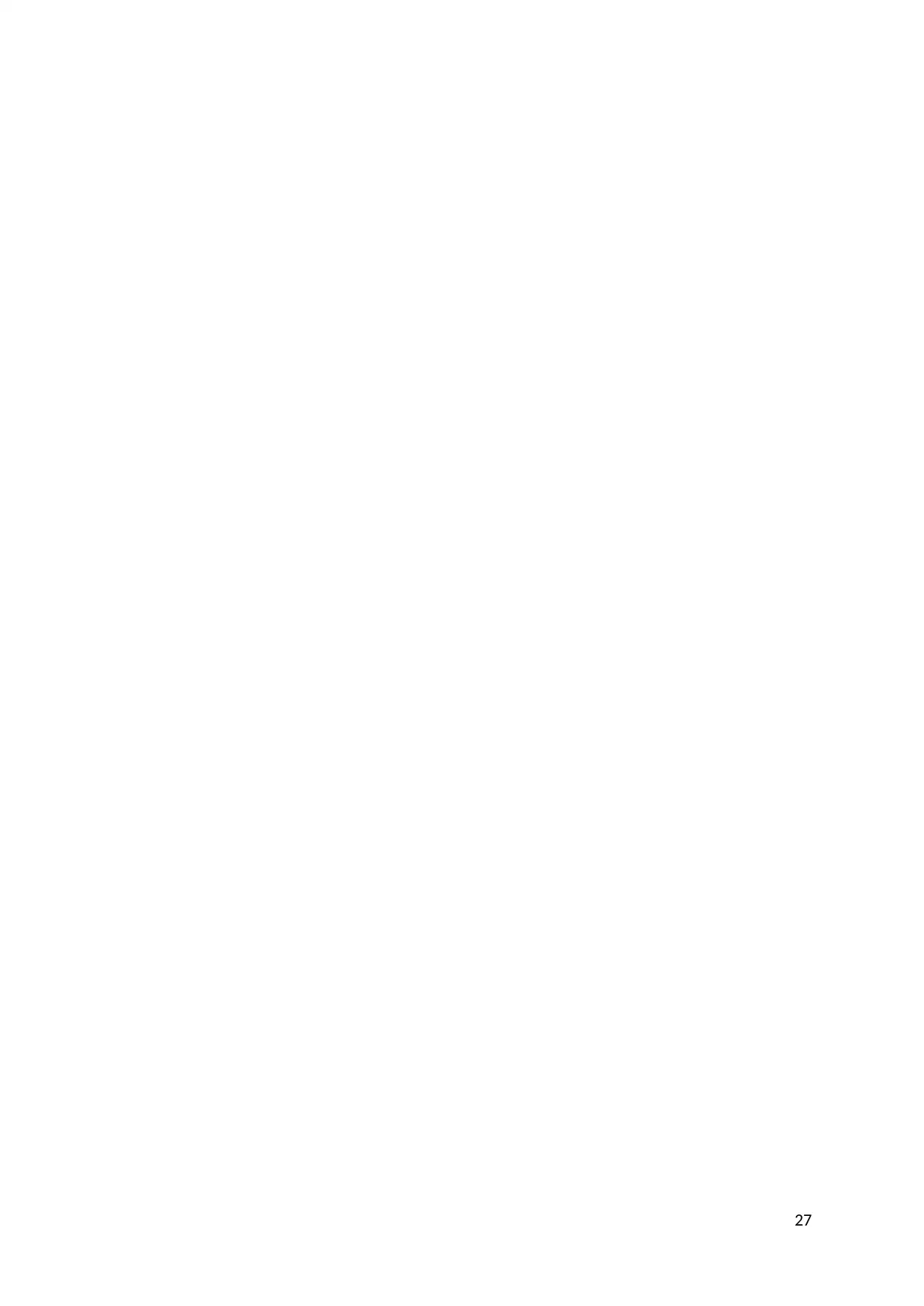
27
1 out of 28
Related Documents

Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.