Python Programming Fundamentals: Algorithms, Paradigms, and Debugging
VerifiedAdded on 2024/05/23
|27
|3721
|248
AI Summary
This report explores the fundamentals of Python programming, covering essential concepts like algorithms, programming paradigms, and debugging techniques. It delves into the characteristics of procedural, object-oriented, and event-driven programming, analyzing the strengths and limitations of each approach. The report also provides a detailed analysis of the Integrated Development Environment (IDE) used for Python development, IDLE, highlighting its features and functionalities. Furthermore, it outlines the debugging process in IDLE, emphasizing the importance of coding standards for creating robust and maintainable code. Through practical examples and code snippets, this report aims to provide a comprehensive understanding of the core principles of Python programming.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
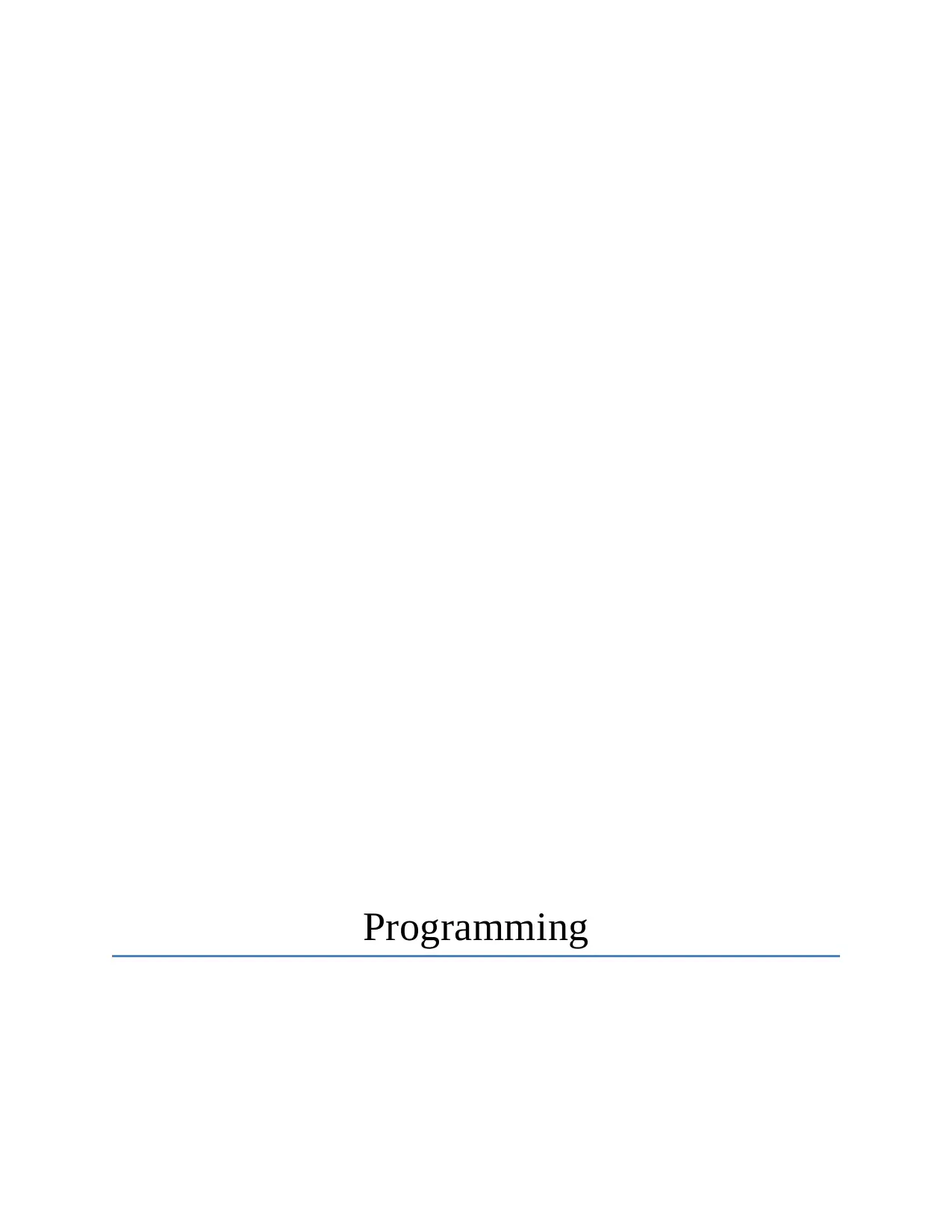
Programming
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
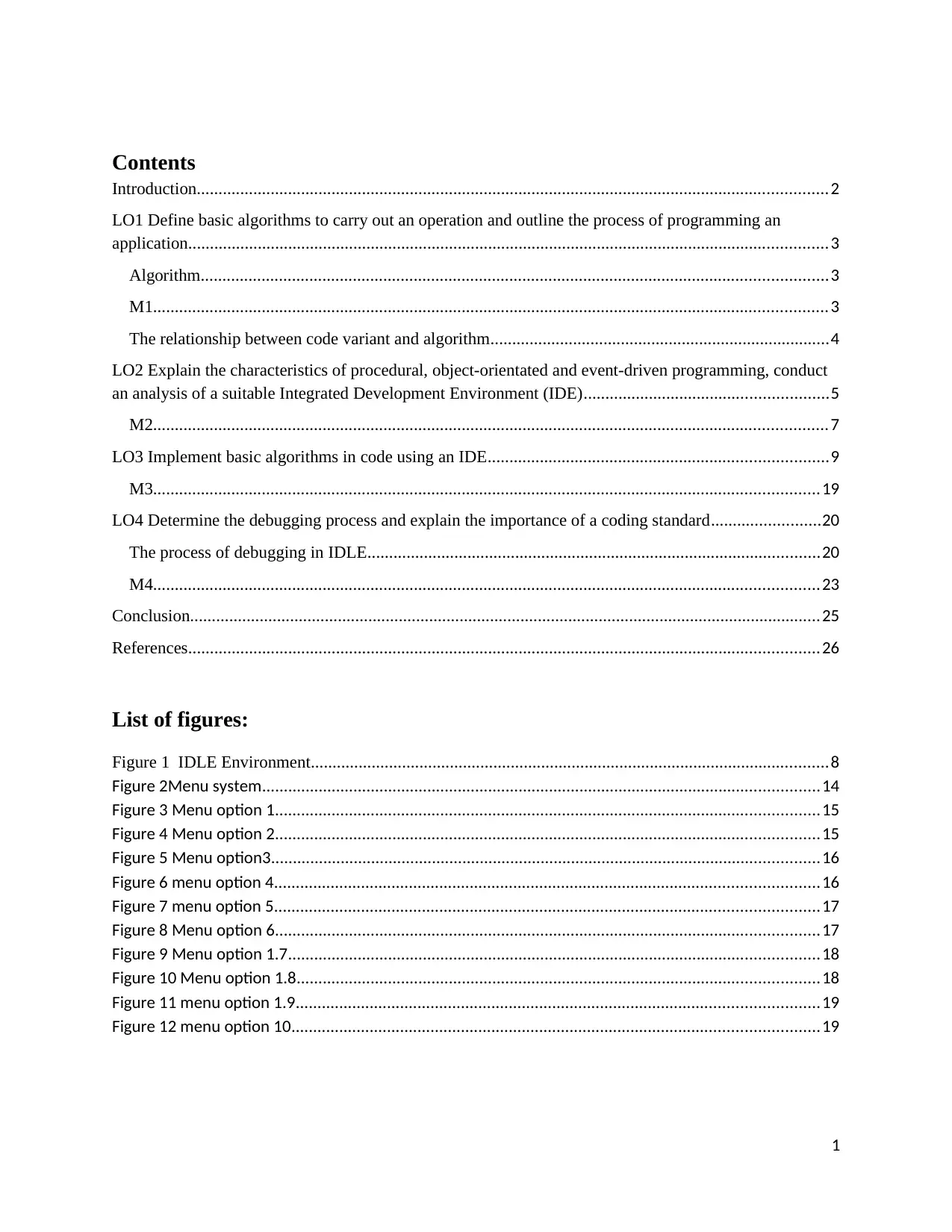
Contents
Introduction.................................................................................................................................................2
LO1 Define basic algorithms to carry out an operation and outline the process of programming an
application...................................................................................................................................................3
Algorithm................................................................................................................................................3
M1...........................................................................................................................................................3
The relationship between code variant and algorithm..............................................................................4
LO2 Explain the characteristics of procedural, object-orientated and event-driven programming, conduct
an analysis of a suitable Integrated Development Environment (IDE)........................................................5
M2...........................................................................................................................................................7
LO3 Implement basic algorithms in code using an IDE..............................................................................9
M3.........................................................................................................................................................19
LO4 Determine the debugging process and explain the importance of a coding standard.........................20
The process of debugging in IDLE........................................................................................................20
M4.........................................................................................................................................................23
Conclusion.................................................................................................................................................25
References.................................................................................................................................................26
List of figures:
Figure 1 IDLE Environment.......................................................................................................................8
Figure 2Menu system................................................................................................................................14
Figure 3 Menu option 1.............................................................................................................................15
Figure 4 Menu option 2.............................................................................................................................15
Figure 5 Menu option3..............................................................................................................................16
Figure 6 menu option 4.............................................................................................................................16
Figure 7 menu option 5.............................................................................................................................17
Figure 8 Menu option 6.............................................................................................................................17
Figure 9 Menu option 1.7..........................................................................................................................18
Figure 10 Menu option 1.8........................................................................................................................18
Figure 11 menu option 1.9........................................................................................................................19
Figure 12 menu option 10.........................................................................................................................19
1
Introduction.................................................................................................................................................2
LO1 Define basic algorithms to carry out an operation and outline the process of programming an
application...................................................................................................................................................3
Algorithm................................................................................................................................................3
M1...........................................................................................................................................................3
The relationship between code variant and algorithm..............................................................................4
LO2 Explain the characteristics of procedural, object-orientated and event-driven programming, conduct
an analysis of a suitable Integrated Development Environment (IDE)........................................................5
M2...........................................................................................................................................................7
LO3 Implement basic algorithms in code using an IDE..............................................................................9
M3.........................................................................................................................................................19
LO4 Determine the debugging process and explain the importance of a coding standard.........................20
The process of debugging in IDLE........................................................................................................20
M4.........................................................................................................................................................23
Conclusion.................................................................................................................................................25
References.................................................................................................................................................26
List of figures:
Figure 1 IDLE Environment.......................................................................................................................8
Figure 2Menu system................................................................................................................................14
Figure 3 Menu option 1.............................................................................................................................15
Figure 4 Menu option 2.............................................................................................................................15
Figure 5 Menu option3..............................................................................................................................16
Figure 6 menu option 4.............................................................................................................................16
Figure 7 menu option 5.............................................................................................................................17
Figure 8 Menu option 6.............................................................................................................................17
Figure 9 Menu option 1.7..........................................................................................................................18
Figure 10 Menu option 1.8........................................................................................................................18
Figure 11 menu option 1.9........................................................................................................................19
Figure 12 menu option 10.........................................................................................................................19
1
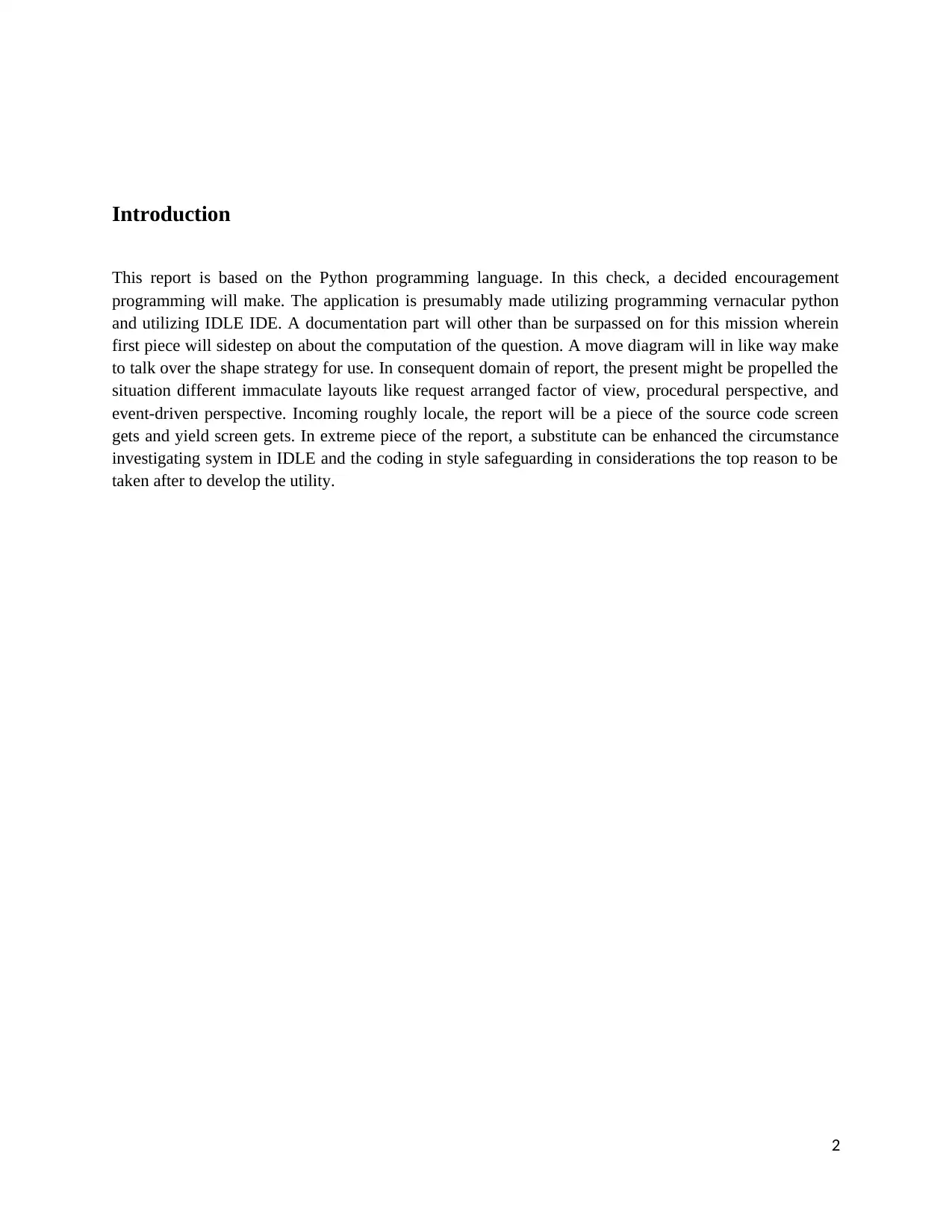
Introduction
This report is based on the Python programming language. In this check, a decided encouragement
programming will make. The application is presumably made utilizing programming vernacular python
and utilizing IDLE IDE. A documentation part will other than be surpassed on for this mission wherein
first piece will sidestep on about the computation of the question. A move diagram will in like way make
to talk over the shape strategy for use. In consequent domain of report, the present might be propelled the
situation different immaculate layouts like request arranged factor of view, procedural perspective, and
event-driven perspective. Incoming roughly locale, the report will be a piece of the source code screen
gets and yield screen gets. In extreme piece of the report, a substitute can be enhanced the circumstance
investigating system in IDLE and the coding in style safeguarding in considerations the top reason to be
taken after to develop the utility.
2
This report is based on the Python programming language. In this check, a decided encouragement
programming will make. The application is presumably made utilizing programming vernacular python
and utilizing IDLE IDE. A documentation part will other than be surpassed on for this mission wherein
first piece will sidestep on about the computation of the question. A move diagram will in like way make
to talk over the shape strategy for use. In consequent domain of report, the present might be propelled the
situation different immaculate layouts like request arranged factor of view, procedural perspective, and
event-driven perspective. Incoming roughly locale, the report will be a piece of the source code screen
gets and yield screen gets. In extreme piece of the report, a substitute can be enhanced the circumstance
investigating system in IDLE and the coding in style safeguarding in considerations the top reason to be
taken after to develop the utility.
2
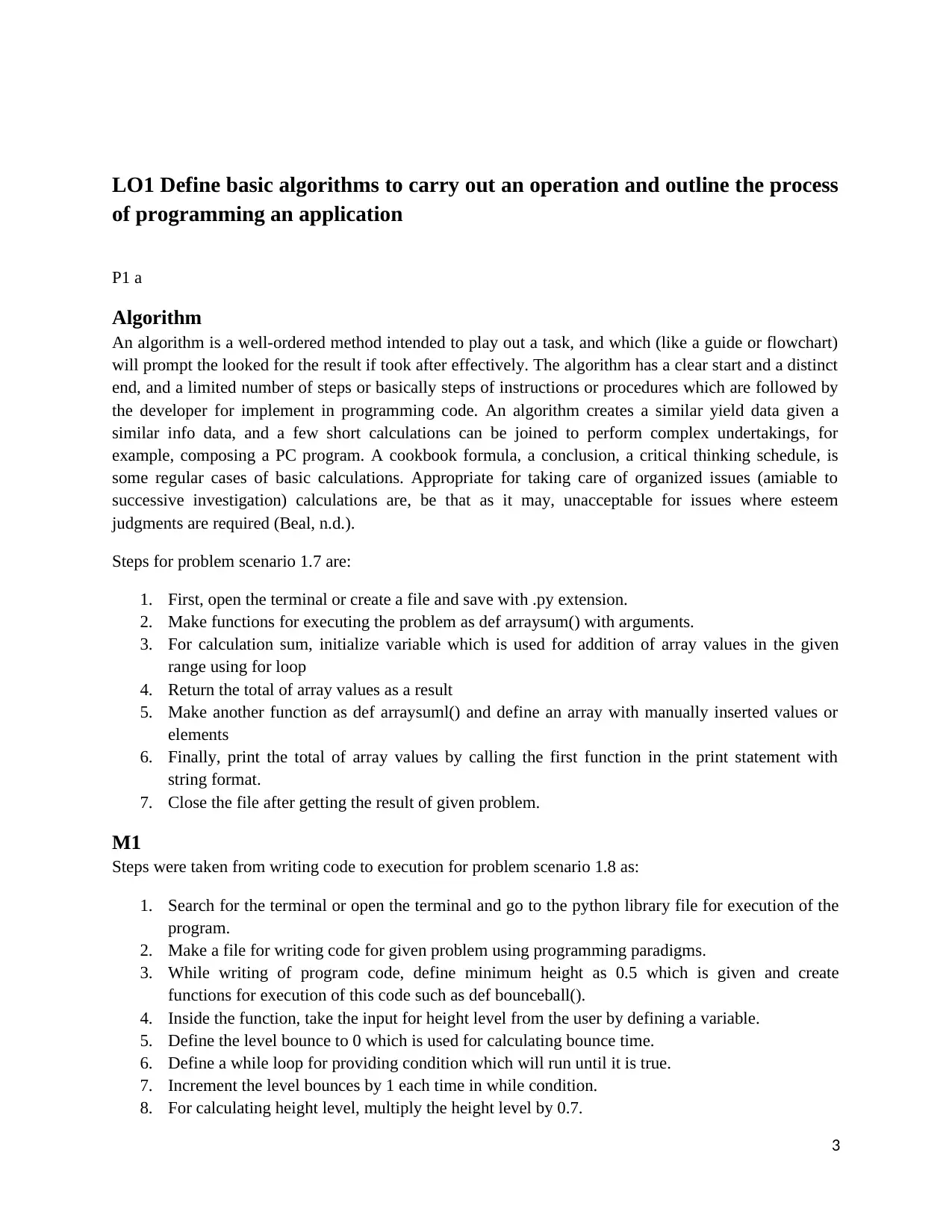
LO1 Define basic algorithms to carry out an operation and outline the process
of programming an application
P1 a
Algorithm
An algorithm is a well-ordered method intended to play out a task, and which (like a guide or flowchart)
will prompt the looked for the result if took after effectively. The algorithm has a clear start and a distinct
end, and a limited number of steps or basically steps of instructions or procedures which are followed by
the developer for implement in programming code. An algorithm creates a similar yield data given a
similar info data, and a few short calculations can be joined to perform complex undertakings, for
example, composing a PC program. A cookbook formula, a conclusion, a critical thinking schedule, is
some regular cases of basic calculations. Appropriate for taking care of organized issues (amiable to
successive investigation) calculations are, be that as it may, unacceptable for issues where esteem
judgments are required (Beal, n.d.).
Steps for problem scenario 1.7 are:
1. First, open the terminal or create a file and save with .py extension.
2. Make functions for executing the problem as def arraysum() with arguments.
3. For calculation sum, initialize variable which is used for addition of array values in the given
range using for loop
4. Return the total of array values as a result
5. Make another function as def arraysuml() and define an array with manually inserted values or
elements
6. Finally, print the total of array values by calling the first function in the print statement with
string format.
7. Close the file after getting the result of given problem.
M1
Steps were taken from writing code to execution for problem scenario 1.8 as:
1. Search for the terminal or open the terminal and go to the python library file for execution of the
program.
2. Make a file for writing code for given problem using programming paradigms.
3. While writing of program code, define minimum height as 0.5 which is given and create
functions for execution of this code such as def bounceball().
4. Inside the function, take the input for height level from the user by defining a variable.
5. Define the level bounce to 0 which is used for calculating bounce time.
6. Define a while loop for providing condition which will run until it is true.
7. Increment the level bounces by 1 each time in while condition.
8. For calculating height level, multiply the height level by 0.7.
3
of programming an application
P1 a
Algorithm
An algorithm is a well-ordered method intended to play out a task, and which (like a guide or flowchart)
will prompt the looked for the result if took after effectively. The algorithm has a clear start and a distinct
end, and a limited number of steps or basically steps of instructions or procedures which are followed by
the developer for implement in programming code. An algorithm creates a similar yield data given a
similar info data, and a few short calculations can be joined to perform complex undertakings, for
example, composing a PC program. A cookbook formula, a conclusion, a critical thinking schedule, is
some regular cases of basic calculations. Appropriate for taking care of organized issues (amiable to
successive investigation) calculations are, be that as it may, unacceptable for issues where esteem
judgments are required (Beal, n.d.).
Steps for problem scenario 1.7 are:
1. First, open the terminal or create a file and save with .py extension.
2. Make functions for executing the problem as def arraysum() with arguments.
3. For calculation sum, initialize variable which is used for addition of array values in the given
range using for loop
4. Return the total of array values as a result
5. Make another function as def arraysuml() and define an array with manually inserted values or
elements
6. Finally, print the total of array values by calling the first function in the print statement with
string format.
7. Close the file after getting the result of given problem.
M1
Steps were taken from writing code to execution for problem scenario 1.8 as:
1. Search for the terminal or open the terminal and go to the python library file for execution of the
program.
2. Make a file for writing code for given problem using programming paradigms.
3. While writing of program code, define minimum height as 0.5 which is given and create
functions for execution of this code such as def bounceball().
4. Inside the function, take the input for height level from the user by defining a variable.
5. Define the level bounce to 0 which is used for calculating bounce time.
6. Define a while loop for providing condition which will run until it is true.
7. Increment the level bounces by 1 each time in while condition.
8. For calculating height level, multiply the height level by 0.7.
3
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
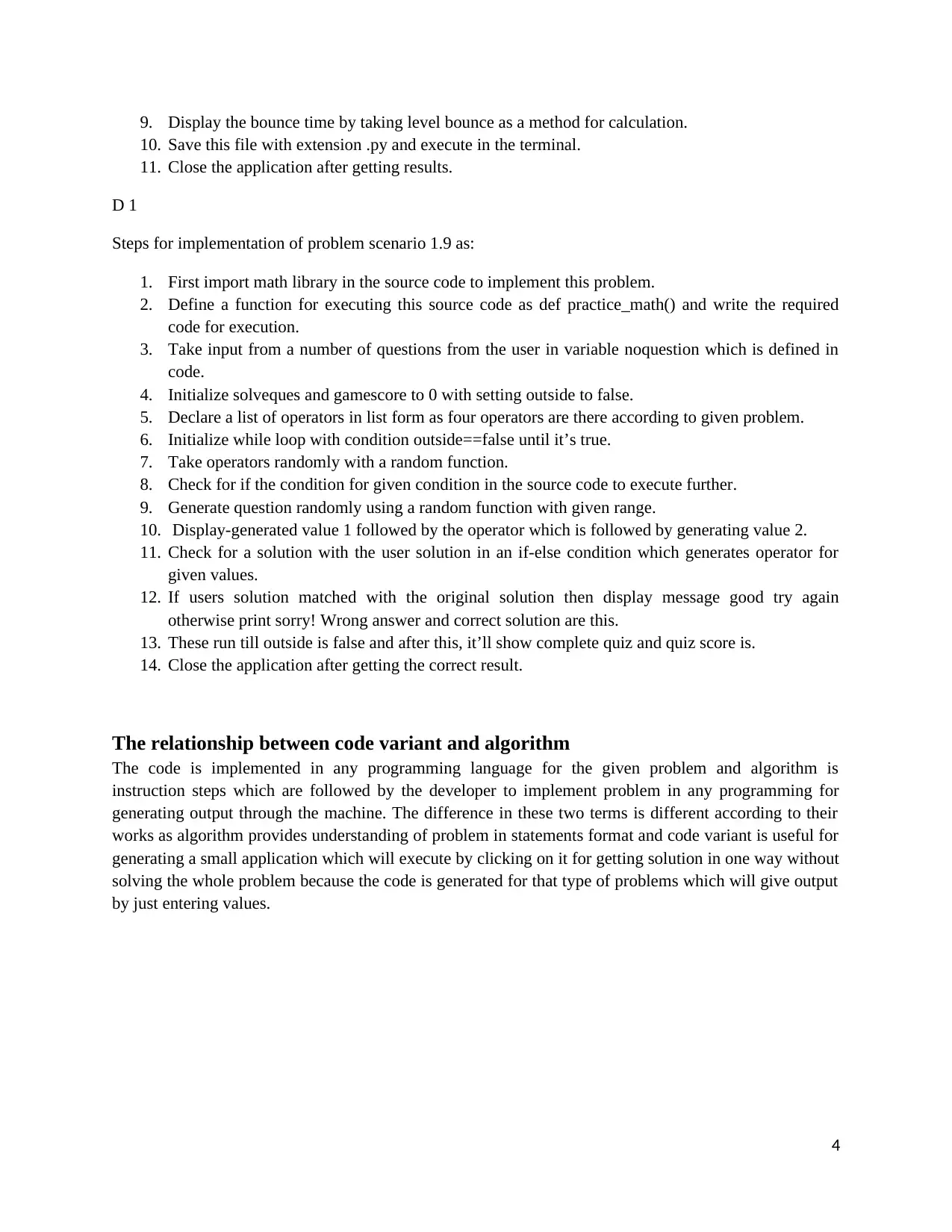
9. Display the bounce time by taking level bounce as a method for calculation.
10. Save this file with extension .py and execute in the terminal.
11. Close the application after getting results.
D 1
Steps for implementation of problem scenario 1.9 as:
1. First import math library in the source code to implement this problem.
2. Define a function for executing this source code as def practice_math() and write the required
code for execution.
3. Take input from a number of questions from the user in variable noquestion which is defined in
code.
4. Initialize solveques and gamescore to 0 with setting outside to false.
5. Declare a list of operators in list form as four operators are there according to given problem.
6. Initialize while loop with condition outside==false until it’s true.
7. Take operators randomly with a random function.
8. Check for if the condition for given condition in the source code to execute further.
9. Generate question randomly using a random function with given range.
10. Display-generated value 1 followed by the operator which is followed by generating value 2.
11. Check for a solution with the user solution in an if-else condition which generates operator for
given values.
12. If users solution matched with the original solution then display message good try again
otherwise print sorry! Wrong answer and correct solution are this.
13. These run till outside is false and after this, it’ll show complete quiz and quiz score is.
14. Close the application after getting the correct result.
The relationship between code variant and algorithm
The code is implemented in any programming language for the given problem and algorithm is
instruction steps which are followed by the developer to implement problem in any programming for
generating output through the machine. The difference in these two terms is different according to their
works as algorithm provides understanding of problem in statements format and code variant is useful for
generating a small application which will execute by clicking on it for getting solution in one way without
solving the whole problem because the code is generated for that type of problems which will give output
by just entering values.
4
10. Save this file with extension .py and execute in the terminal.
11. Close the application after getting results.
D 1
Steps for implementation of problem scenario 1.9 as:
1. First import math library in the source code to implement this problem.
2. Define a function for executing this source code as def practice_math() and write the required
code for execution.
3. Take input from a number of questions from the user in variable noquestion which is defined in
code.
4. Initialize solveques and gamescore to 0 with setting outside to false.
5. Declare a list of operators in list form as four operators are there according to given problem.
6. Initialize while loop with condition outside==false until it’s true.
7. Take operators randomly with a random function.
8. Check for if the condition for given condition in the source code to execute further.
9. Generate question randomly using a random function with given range.
10. Display-generated value 1 followed by the operator which is followed by generating value 2.
11. Check for a solution with the user solution in an if-else condition which generates operator for
given values.
12. If users solution matched with the original solution then display message good try again
otherwise print sorry! Wrong answer and correct solution are this.
13. These run till outside is false and after this, it’ll show complete quiz and quiz score is.
14. Close the application after getting the correct result.
The relationship between code variant and algorithm
The code is implemented in any programming language for the given problem and algorithm is
instruction steps which are followed by the developer to implement problem in any programming for
generating output through the machine. The difference in these two terms is different according to their
works as algorithm provides understanding of problem in statements format and code variant is useful for
generating a small application which will execute by clicking on it for getting solution in one way without
solving the whole problem because the code is generated for that type of problems which will give output
by just entering values.
4
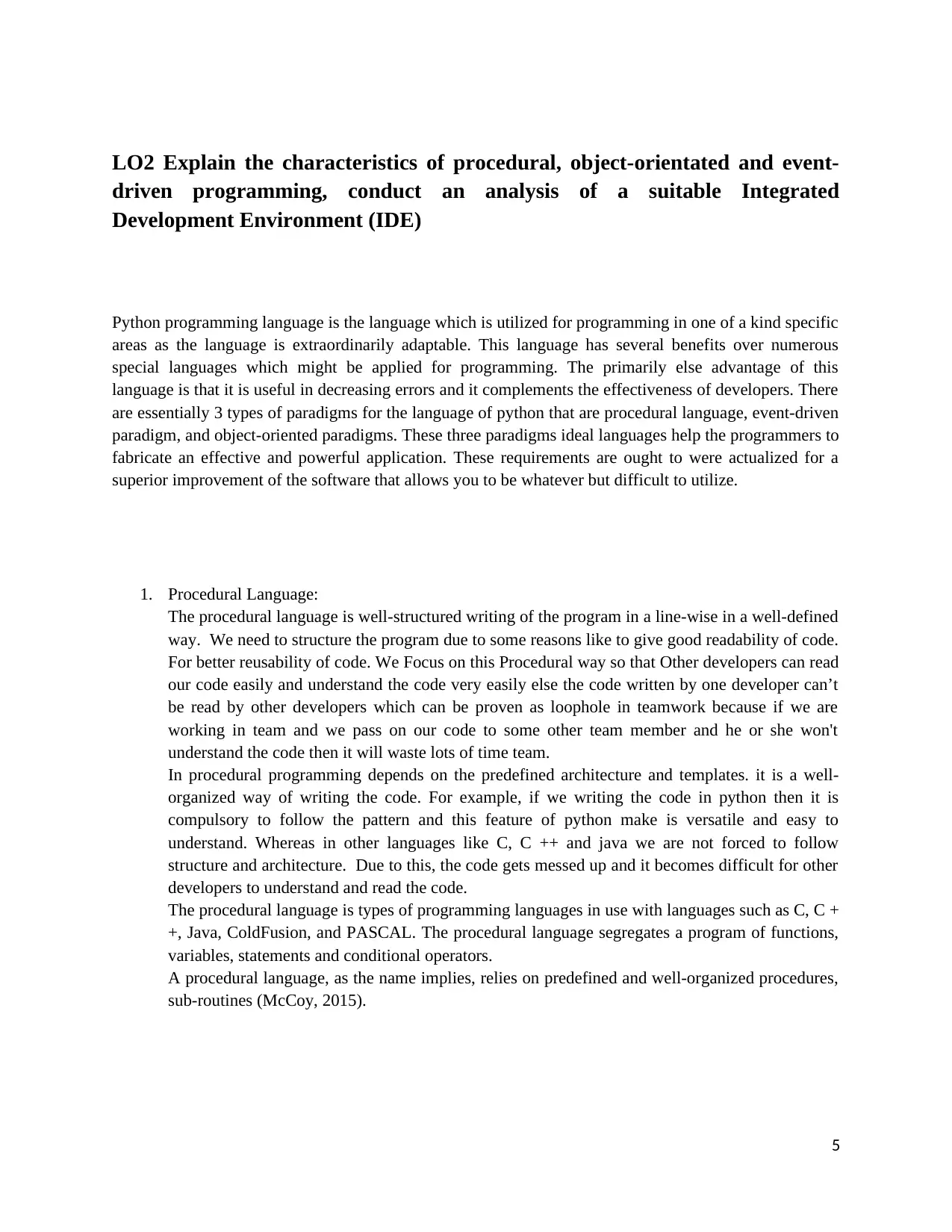
LO2 Explain the characteristics of procedural, object-orientated and event-
driven programming, conduct an analysis of a suitable Integrated
Development Environment (IDE)
Python programming language is the language which is utilized for programming in one of a kind specific
areas as the language is extraordinarily adaptable. This language has several benefits over numerous
special languages which might be applied for programming. The primarily else advantage of this
language is that it is useful in decreasing errors and it complements the effectiveness of developers. There
are essentially 3 types of paradigms for the language of python that are procedural language, event-driven
paradigm, and object-oriented paradigms. These three paradigms ideal languages help the programmers to
fabricate an effective and powerful application. These requirements are ought to were actualized for a
superior improvement of the software that allows you to be whatever but difficult to utilize.
1. Procedural Language:
The procedural language is well-structured writing of the program in a line-wise in a well-defined
way. We need to structure the program due to some reasons like to give good readability of code.
For better reusability of code. We Focus on this Procedural way so that Other developers can read
our code easily and understand the code very easily else the code written by one developer can’t
be read by other developers which can be proven as loophole in teamwork because if we are
working in team and we pass on our code to some other team member and he or she won't
understand the code then it will waste lots of time team.
In procedural programming depends on the predefined architecture and templates. it is a well-
organized way of writing the code. For example, if we writing the code in python then it is
compulsory to follow the pattern and this feature of python make is versatile and easy to
understand. Whereas in other languages like C, C ++ and java we are not forced to follow
structure and architecture. Due to this, the code gets messed up and it becomes difficult for other
developers to understand and read the code.
The procedural language is types of programming languages in use with languages such as C, C +
+, Java, ColdFusion, and PASCAL. The procedural language segregates a program of functions,
variables, statements and conditional operators.
A procedural language, as the name implies, relies on predefined and well-organized procedures,
sub-routines (McCoy, 2015).
5
driven programming, conduct an analysis of a suitable Integrated
Development Environment (IDE)
Python programming language is the language which is utilized for programming in one of a kind specific
areas as the language is extraordinarily adaptable. This language has several benefits over numerous
special languages which might be applied for programming. The primarily else advantage of this
language is that it is useful in decreasing errors and it complements the effectiveness of developers. There
are essentially 3 types of paradigms for the language of python that are procedural language, event-driven
paradigm, and object-oriented paradigms. These three paradigms ideal languages help the programmers to
fabricate an effective and powerful application. These requirements are ought to were actualized for a
superior improvement of the software that allows you to be whatever but difficult to utilize.
1. Procedural Language:
The procedural language is well-structured writing of the program in a line-wise in a well-defined
way. We need to structure the program due to some reasons like to give good readability of code.
For better reusability of code. We Focus on this Procedural way so that Other developers can read
our code easily and understand the code very easily else the code written by one developer can’t
be read by other developers which can be proven as loophole in teamwork because if we are
working in team and we pass on our code to some other team member and he or she won't
understand the code then it will waste lots of time team.
In procedural programming depends on the predefined architecture and templates. it is a well-
organized way of writing the code. For example, if we writing the code in python then it is
compulsory to follow the pattern and this feature of python make is versatile and easy to
understand. Whereas in other languages like C, C ++ and java we are not forced to follow
structure and architecture. Due to this, the code gets messed up and it becomes difficult for other
developers to understand and read the code.
The procedural language is types of programming languages in use with languages such as C, C +
+, Java, ColdFusion, and PASCAL. The procedural language segregates a program of functions,
variables, statements and conditional operators.
A procedural language, as the name implies, relies on predefined and well-organized procedures,
sub-routines (McCoy, 2015).
5
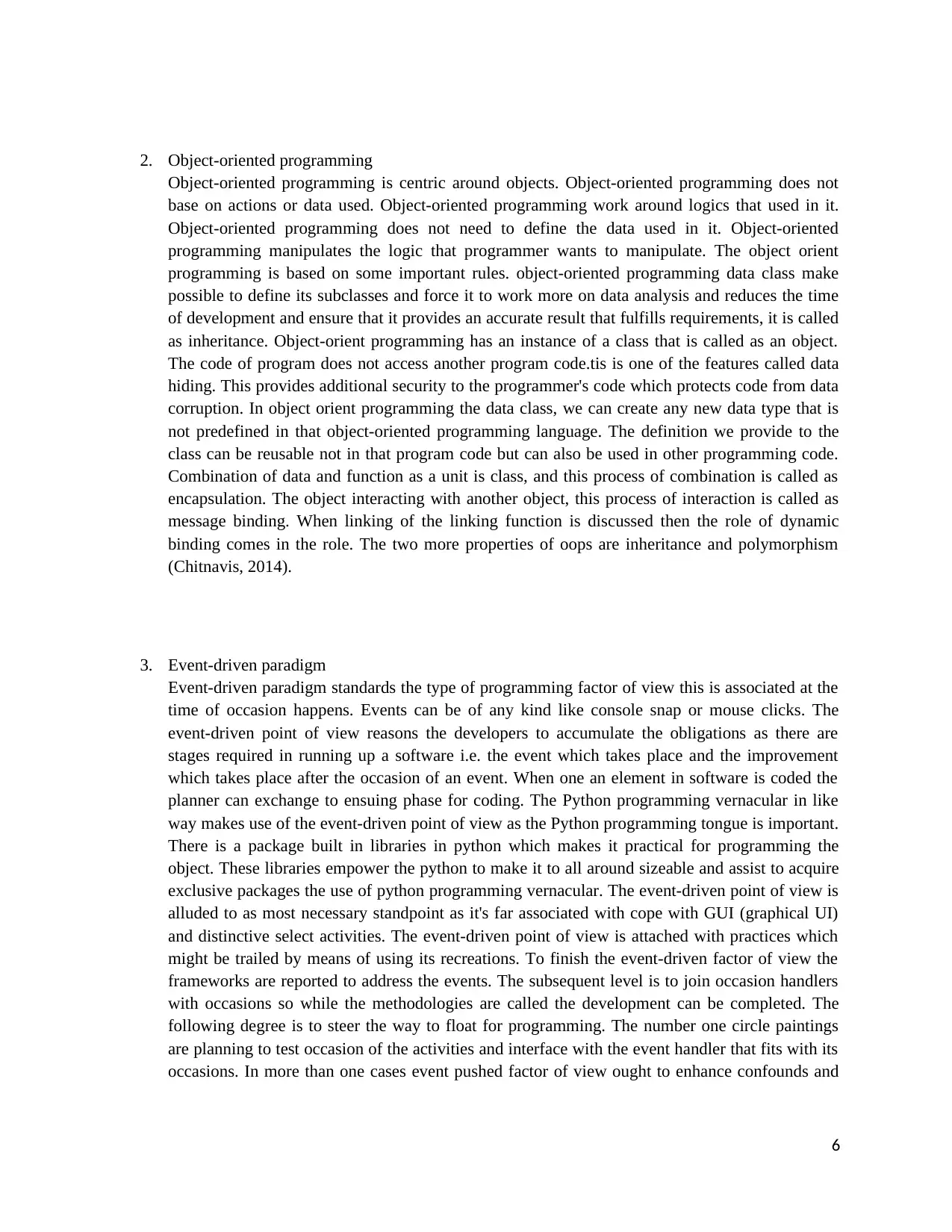
2. Object-oriented programming
Object-oriented programming is centric around objects. Object-oriented programming does not
base on actions or data used. Object-oriented programming work around logics that used in it.
Object-oriented programming does not need to define the data used in it. Object-oriented
programming manipulates the logic that programmer wants to manipulate. The object orient
programming is based on some important rules. object-oriented programming data class make
possible to define its subclasses and force it to work more on data analysis and reduces the time
of development and ensure that it provides an accurate result that fulfills requirements, it is called
as inheritance. Object-orient programming has an instance of a class that is called as an object.
The code of program does not access another program code.tis is one of the features called data
hiding. This provides additional security to the programmer's code which protects code from data
corruption. In object orient programming the data class, we can create any new data type that is
not predefined in that object-oriented programming language. The definition we provide to the
class can be reusable not in that program code but can also be used in other programming code.
Combination of data and function as a unit is class, and this process of combination is called as
encapsulation. The object interacting with another object, this process of interaction is called as
message binding. When linking of the linking function is discussed then the role of dynamic
binding comes in the role. The two more properties of oops are inheritance and polymorphism
(Chitnavis, 2014).
3. Event-driven paradigm
Event-driven paradigm standards the type of programming factor of view this is associated at the
time of occasion happens. Events can be of any kind like console snap or mouse clicks. The
event-driven point of view reasons the developers to accumulate the obligations as there are
stages required in running up a software i.e. the event which takes place and the improvement
which takes place after the occasion of an event. When one an element in software is coded the
planner can exchange to ensuing phase for coding. The Python programming vernacular in like
way makes use of the event-driven point of view as the Python programming tongue is important.
There is a package built in libraries in python which makes it practical for programming the
object. These libraries empower the python to make it to all around sizeable and assist to acquire
exclusive packages the use of python programming vernacular. The event-driven point of view is
alluded to as most necessary standpoint as it's far associated with cope with GUI (graphical UI)
and distinctive select activities. The event-driven point of view is attached with practices which
might be trailed by means of using its recreations. To finish the event-driven factor of view the
frameworks are reported to address the events. The subsequent level is to join occasion handlers
with occasions so while the methodologies are called the development can be completed. The
following degree is to steer the way to float for programming. The number one circle paintings
are planning to test occasion of the activities and interface with the event handler that fits with its
occasions. In more than one cases event pushed factor of view ought to enhance confounds and
6
Object-oriented programming is centric around objects. Object-oriented programming does not
base on actions or data used. Object-oriented programming work around logics that used in it.
Object-oriented programming does not need to define the data used in it. Object-oriented
programming manipulates the logic that programmer wants to manipulate. The object orient
programming is based on some important rules. object-oriented programming data class make
possible to define its subclasses and force it to work more on data analysis and reduces the time
of development and ensure that it provides an accurate result that fulfills requirements, it is called
as inheritance. Object-orient programming has an instance of a class that is called as an object.
The code of program does not access another program code.tis is one of the features called data
hiding. This provides additional security to the programmer's code which protects code from data
corruption. In object orient programming the data class, we can create any new data type that is
not predefined in that object-oriented programming language. The definition we provide to the
class can be reusable not in that program code but can also be used in other programming code.
Combination of data and function as a unit is class, and this process of combination is called as
encapsulation. The object interacting with another object, this process of interaction is called as
message binding. When linking of the linking function is discussed then the role of dynamic
binding comes in the role. The two more properties of oops are inheritance and polymorphism
(Chitnavis, 2014).
3. Event-driven paradigm
Event-driven paradigm standards the type of programming factor of view this is associated at the
time of occasion happens. Events can be of any kind like console snap or mouse clicks. The
event-driven point of view reasons the developers to accumulate the obligations as there are
stages required in running up a software i.e. the event which takes place and the improvement
which takes place after the occasion of an event. When one an element in software is coded the
planner can exchange to ensuing phase for coding. The Python programming vernacular in like
way makes use of the event-driven point of view as the Python programming tongue is important.
There is a package built in libraries in python which makes it practical for programming the
object. These libraries empower the python to make it to all around sizeable and assist to acquire
exclusive packages the use of python programming vernacular. The event-driven point of view is
alluded to as most necessary standpoint as it's far associated with cope with GUI (graphical UI)
and distinctive select activities. The event-driven point of view is attached with practices which
might be trailed by means of using its recreations. To finish the event-driven factor of view the
frameworks are reported to address the events. The subsequent level is to join occasion handlers
with occasions so while the methodologies are called the development can be completed. The
following degree is to steer the way to float for programming. The number one circle paintings
are planning to test occasion of the activities and interface with the event handler that fits with its
occasions. In more than one cases event pushed factor of view ought to enhance confounds and
6
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
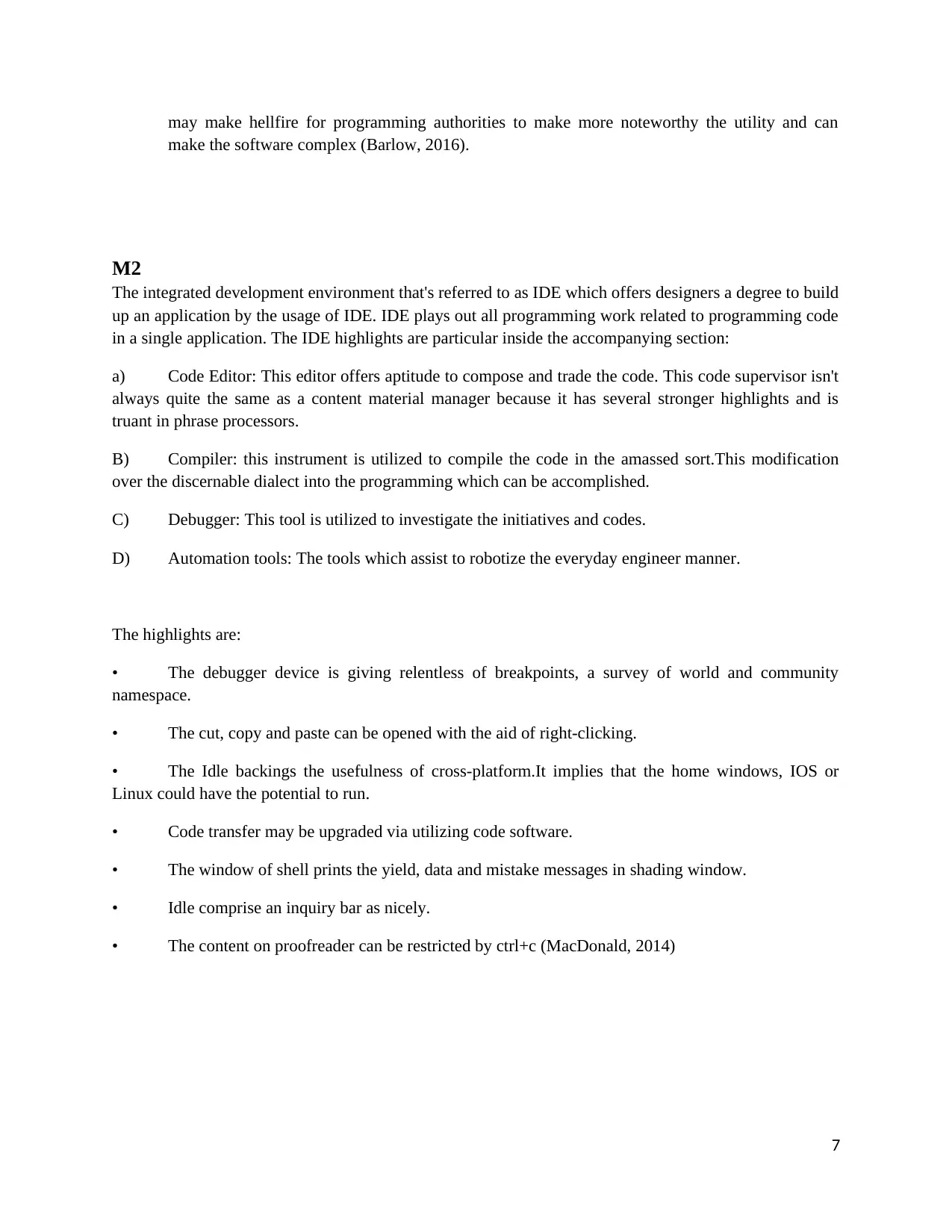
may make hellfire for programming authorities to make more noteworthy the utility and can
make the software complex (Barlow, 2016).
M2
The integrated development environment that's referred to as IDE which offers designers a degree to build
up an application by the usage of IDE. IDE plays out all programming work related to programming code
in a single application. The IDE highlights are particular inside the accompanying section:
a) Code Editor: This editor offers aptitude to compose and trade the code. This code supervisor isn't
always quite the same as a content material manager because it has several stronger highlights and is
truant in phrase processors.
B) Compiler: this instrument is utilized to compile the code in the amassed sort.This modification
over the discernable dialect into the programming which can be accomplished.
C) Debugger: This tool is utilized to investigate the initiatives and codes.
D) Automation tools: The tools which assist to robotize the everyday engineer manner.
The highlights are:
• The debugger device is giving relentless of breakpoints, a survey of world and community
namespace.
• The cut, copy and paste can be opened with the aid of right-clicking.
• The Idle backings the usefulness of cross-platform.It implies that the home windows, IOS or
Linux could have the potential to run.
• Code transfer may be upgraded via utilizing code software.
• The window of shell prints the yield, data and mistake messages in shading window.
• Idle comprise an inquiry bar as nicely.
• The content on proofreader can be restricted by ctrl+c (MacDonald, 2014)
7
make the software complex (Barlow, 2016).
M2
The integrated development environment that's referred to as IDE which offers designers a degree to build
up an application by the usage of IDE. IDE plays out all programming work related to programming code
in a single application. The IDE highlights are particular inside the accompanying section:
a) Code Editor: This editor offers aptitude to compose and trade the code. This code supervisor isn't
always quite the same as a content material manager because it has several stronger highlights and is
truant in phrase processors.
B) Compiler: this instrument is utilized to compile the code in the amassed sort.This modification
over the discernable dialect into the programming which can be accomplished.
C) Debugger: This tool is utilized to investigate the initiatives and codes.
D) Automation tools: The tools which assist to robotize the everyday engineer manner.
The highlights are:
• The debugger device is giving relentless of breakpoints, a survey of world and community
namespace.
• The cut, copy and paste can be opened with the aid of right-clicking.
• The Idle backings the usefulness of cross-platform.It implies that the home windows, IOS or
Linux could have the potential to run.
• Code transfer may be upgraded via utilizing code software.
• The window of shell prints the yield, data and mistake messages in shading window.
• Idle comprise an inquiry bar as nicely.
• The content on proofreader can be restricted by ctrl+c (MacDonald, 2014)
7
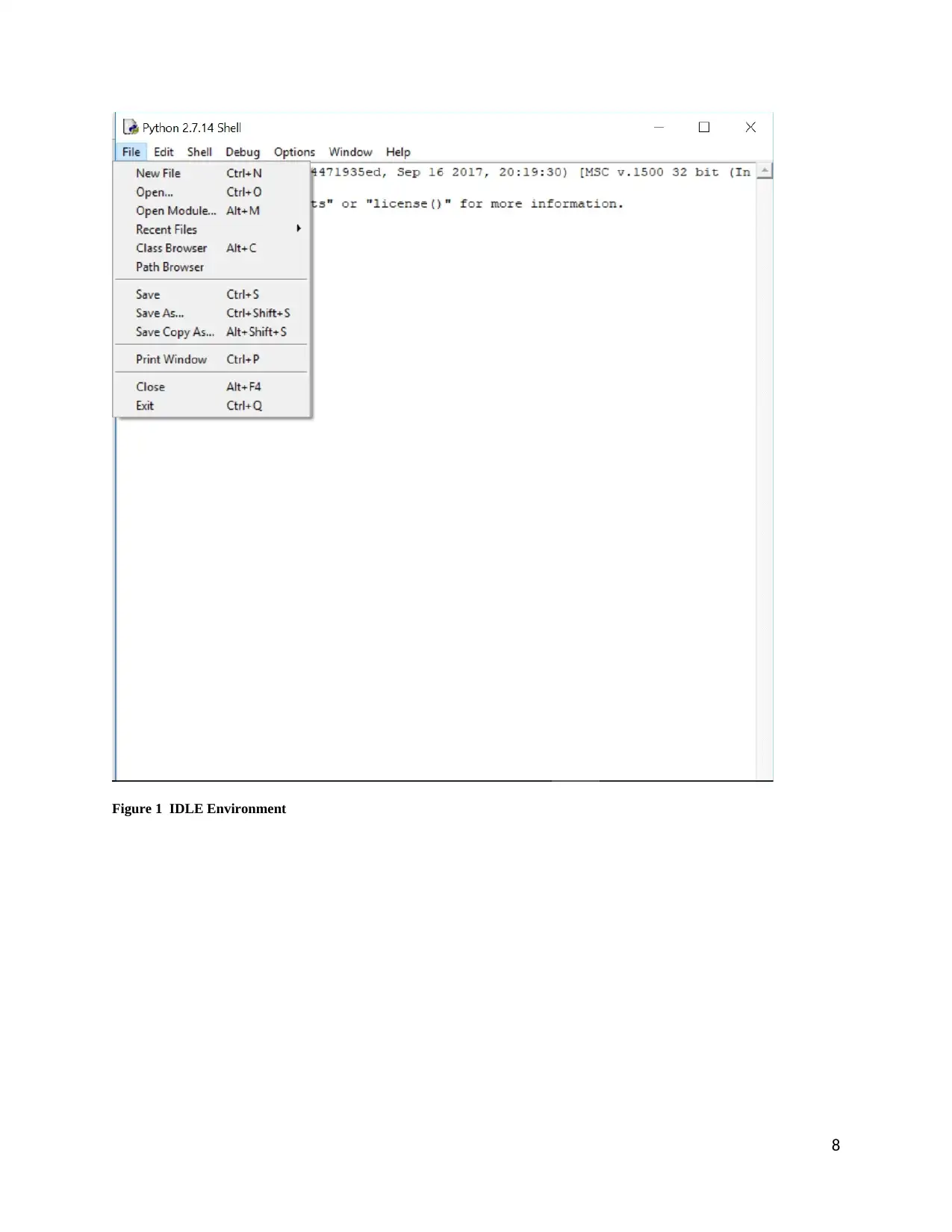
Figure 1 IDLE Environment
8
8
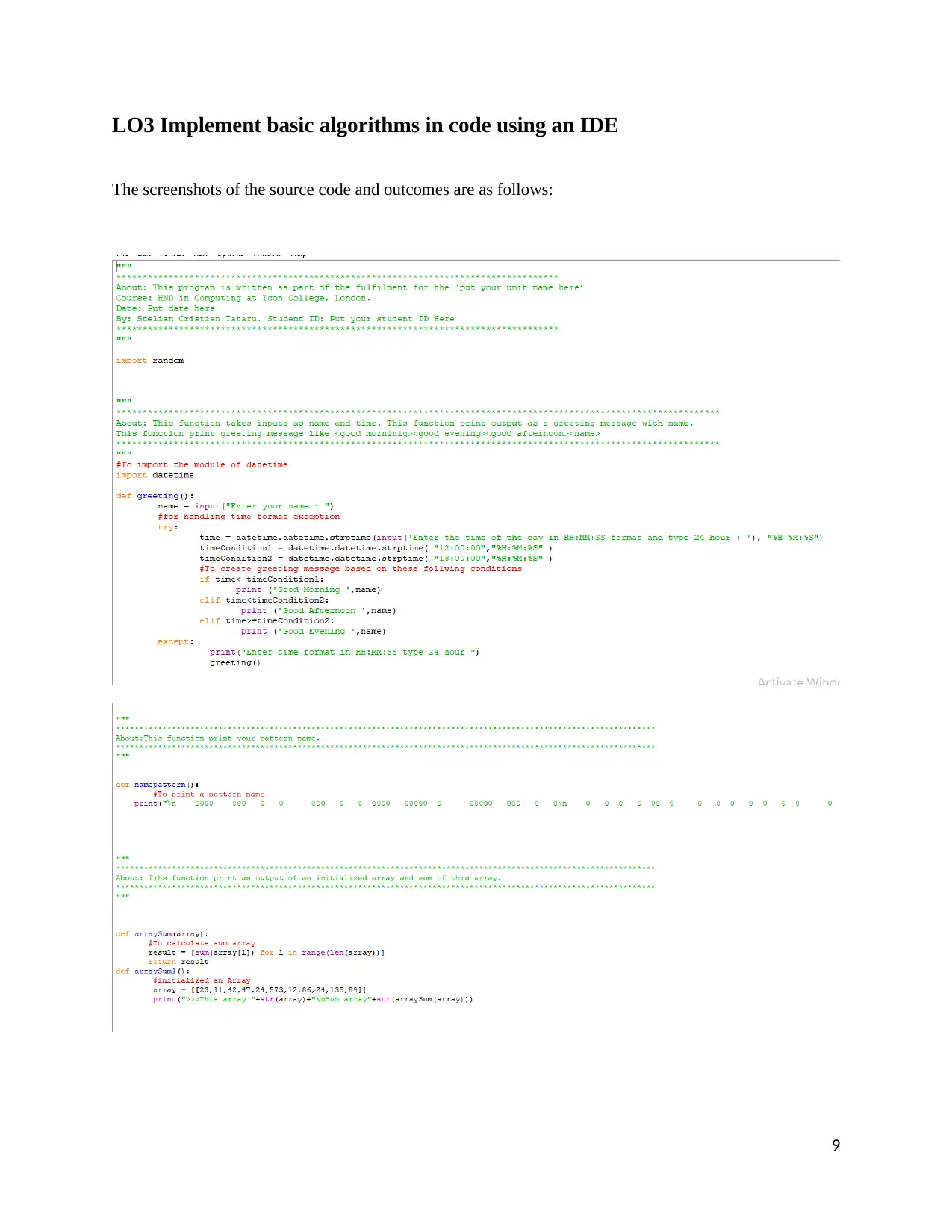
LO3 Implement basic algorithms in code using an IDE
The screenshots of the source code and outcomes are as follows:
9
The screenshots of the source code and outcomes are as follows:
9
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
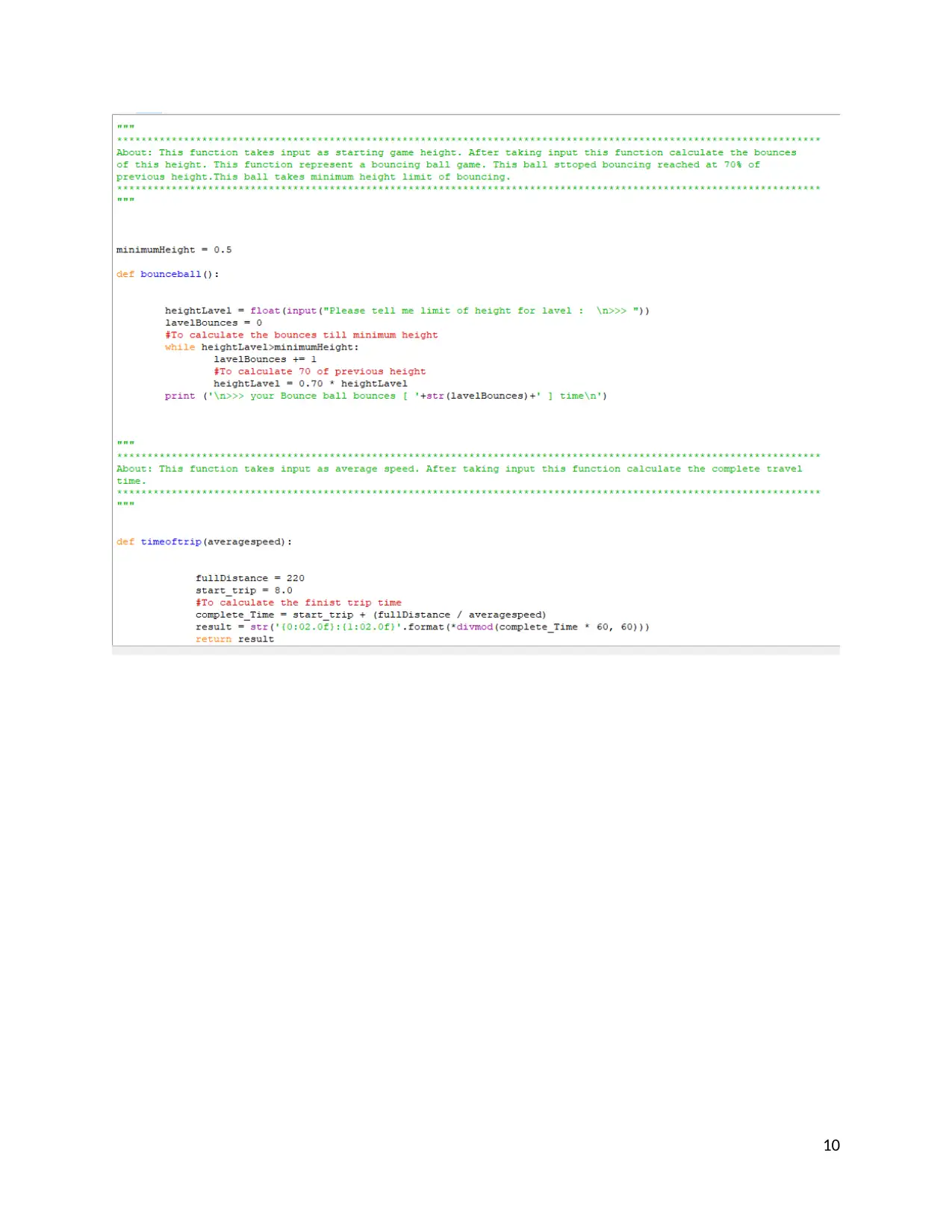
10
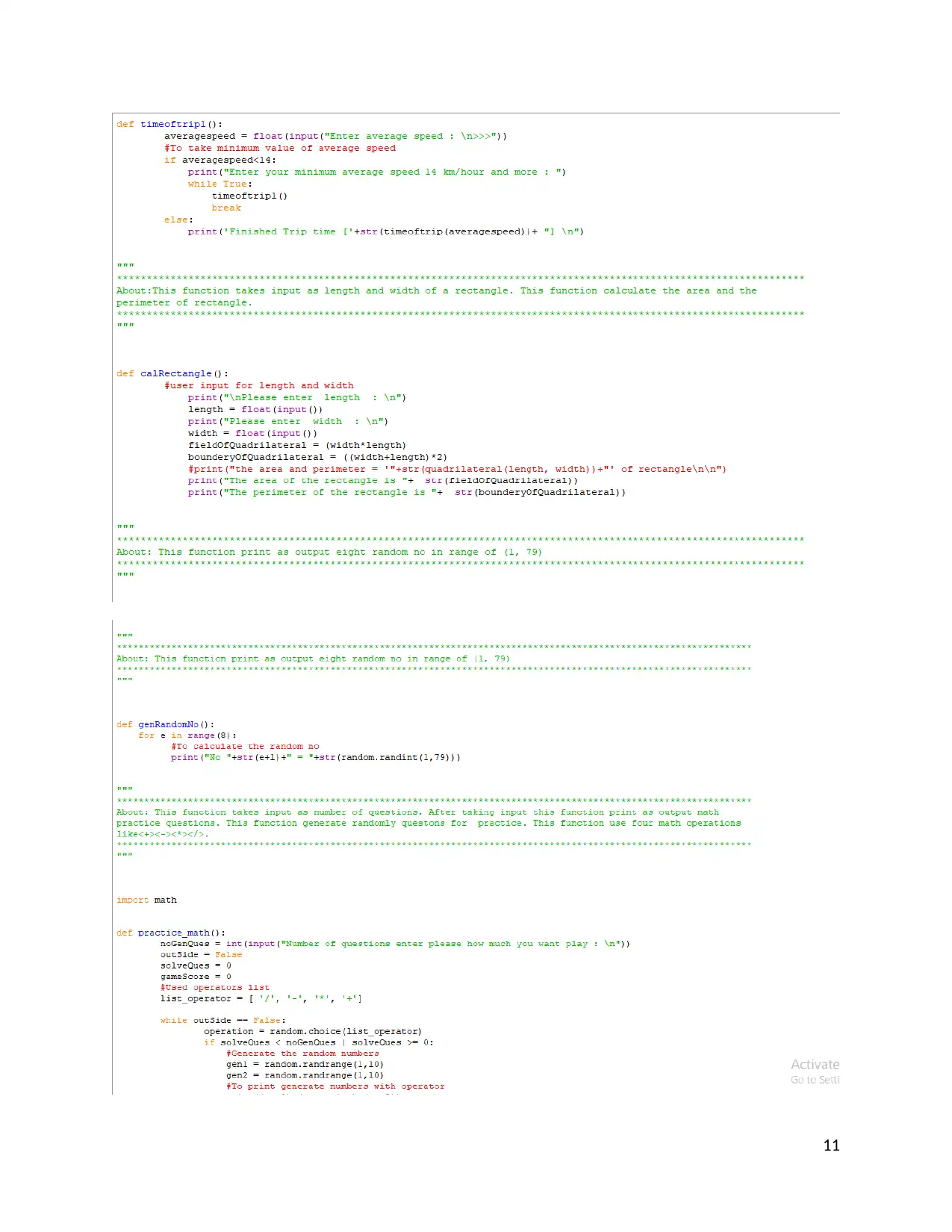
11
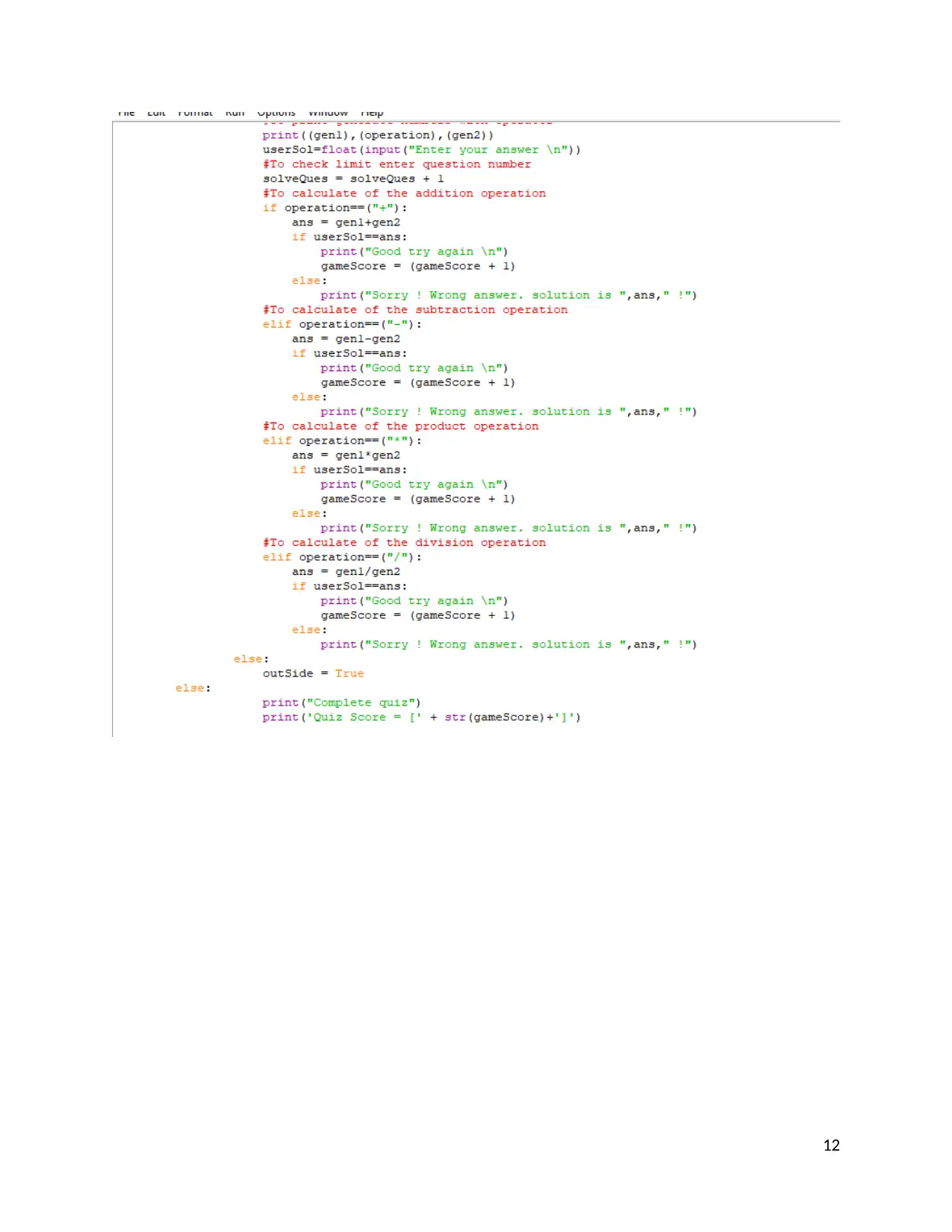
12
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
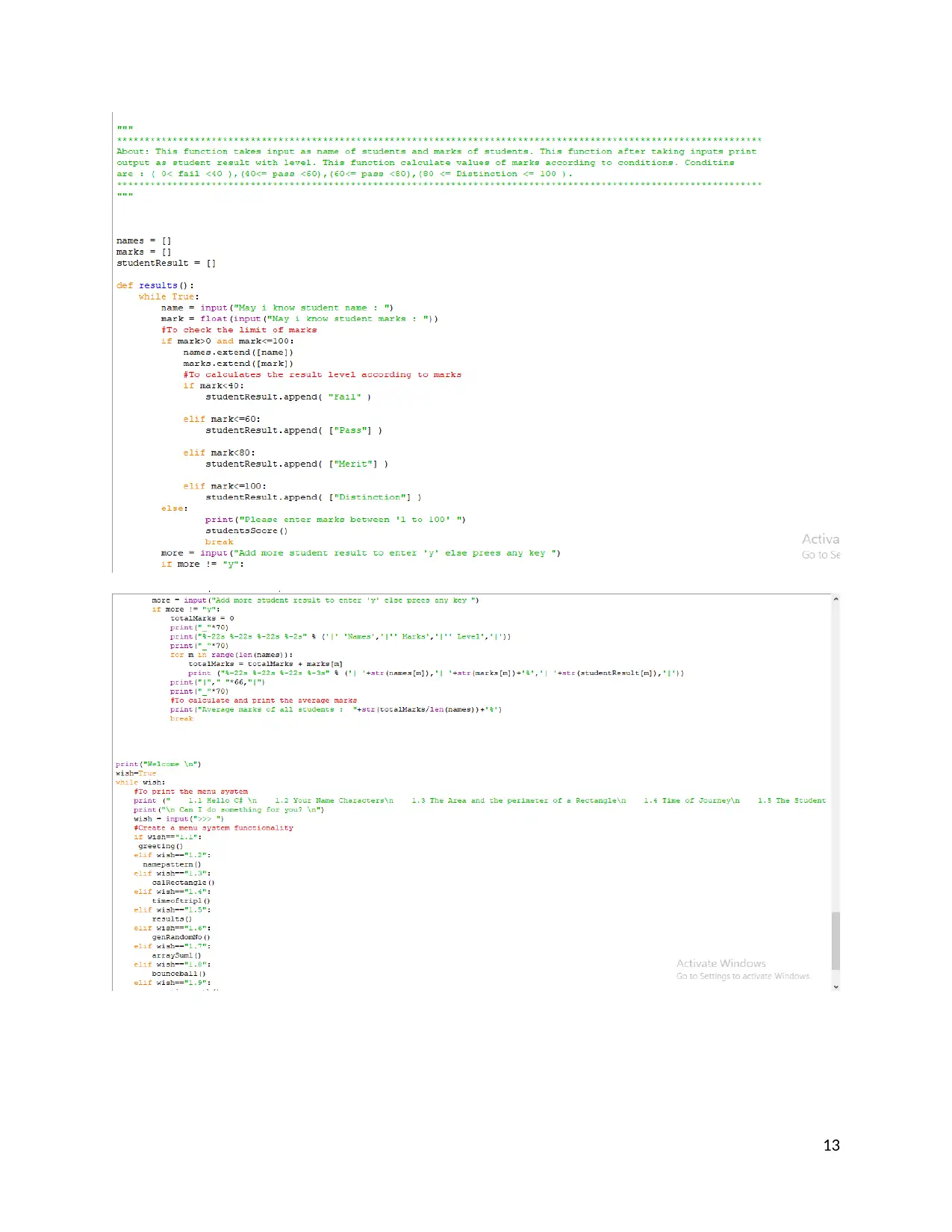
13
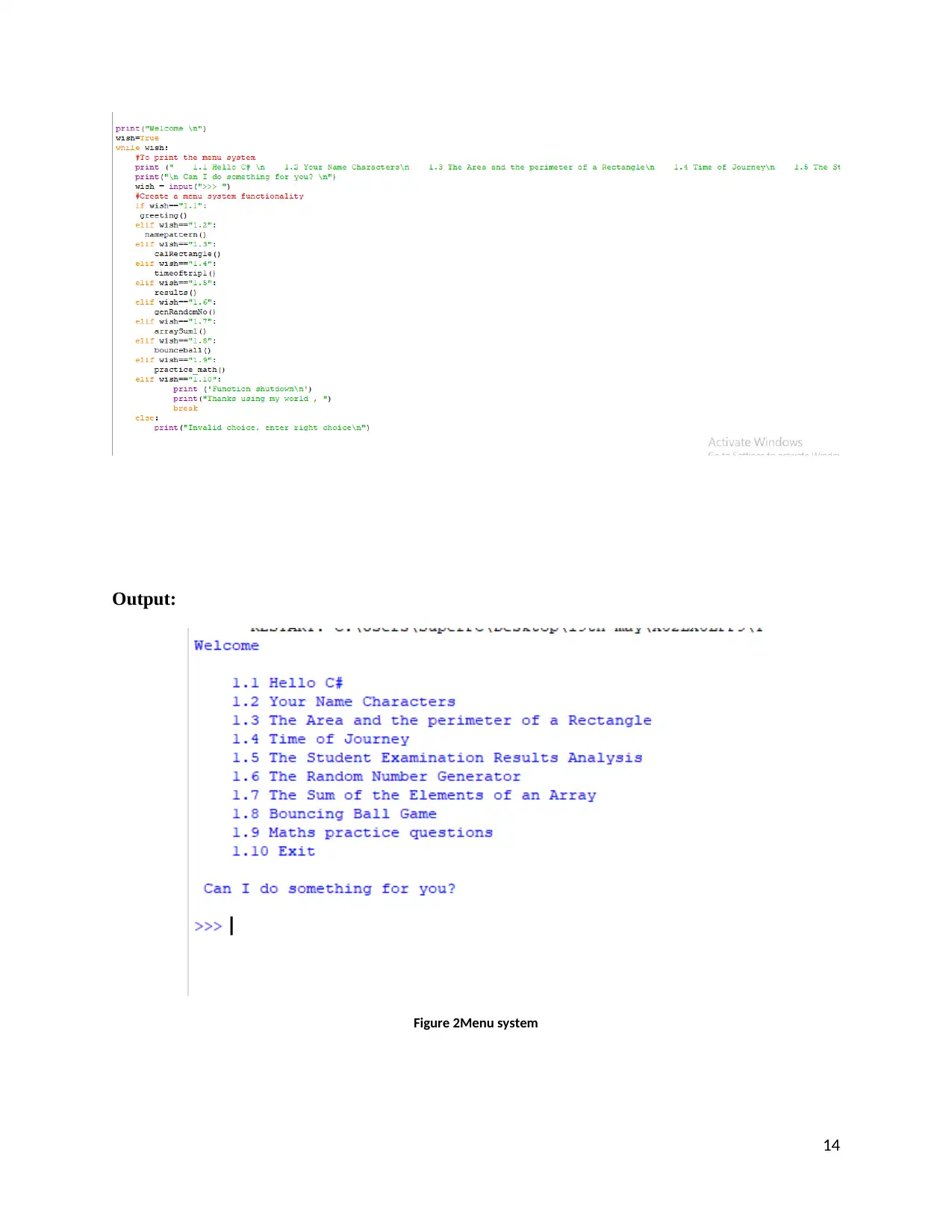
Output:
Figure 2Menu system
14
Figure 2Menu system
14
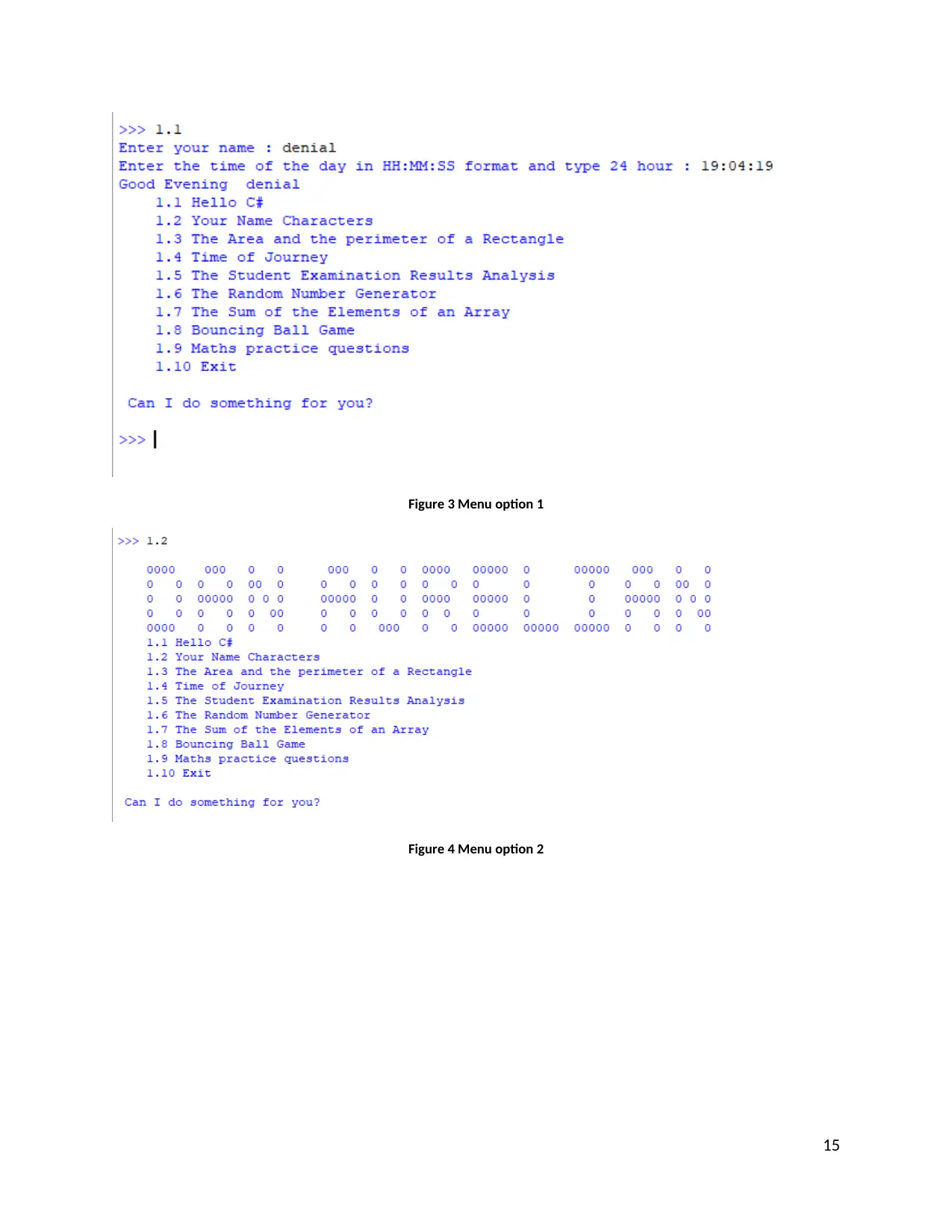
Figure 3 Menu option 1
Figure 4 Menu option 2
15
Figure 4 Menu option 2
15
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
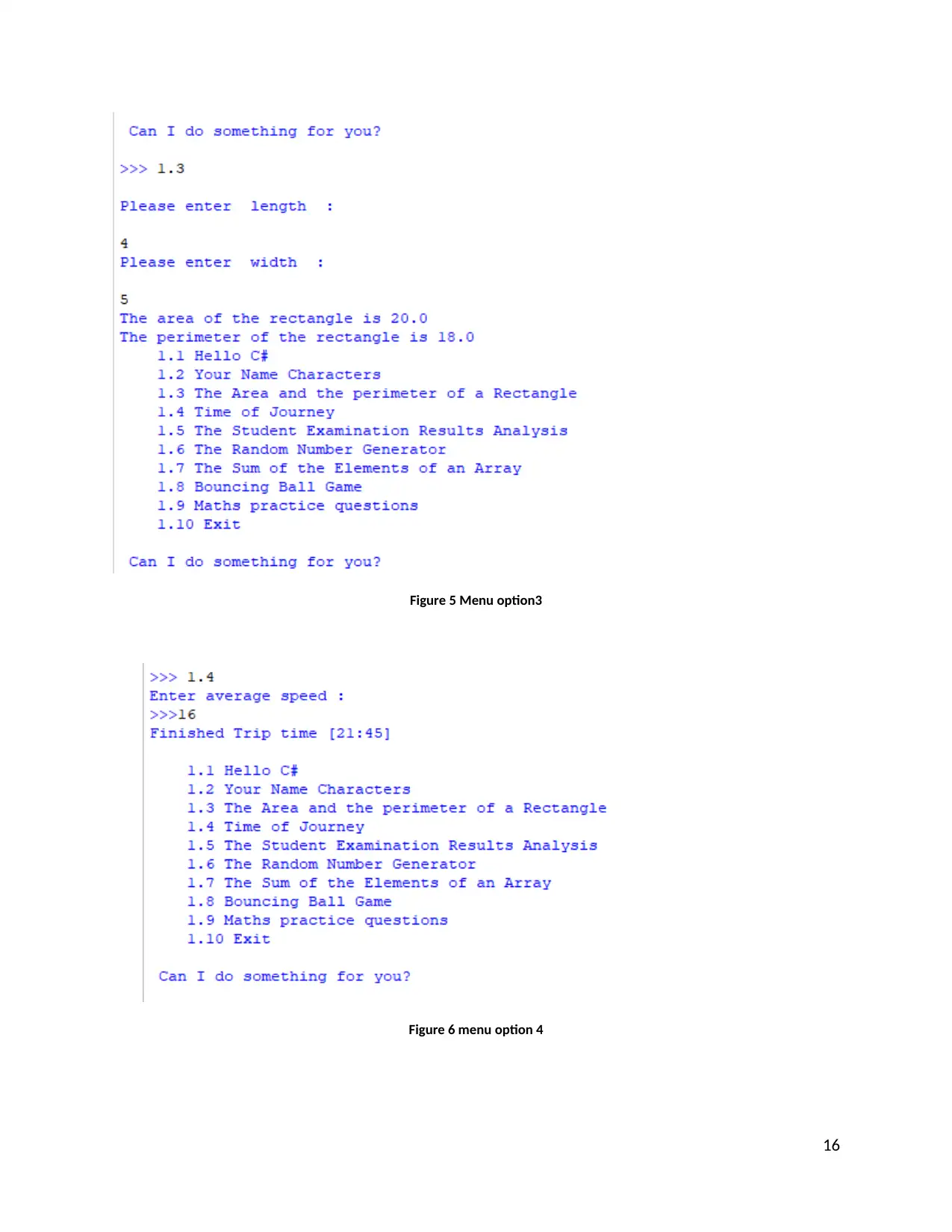
Figure 5 Menu option3
Figure 6 menu option 4
16
Figure 6 menu option 4
16
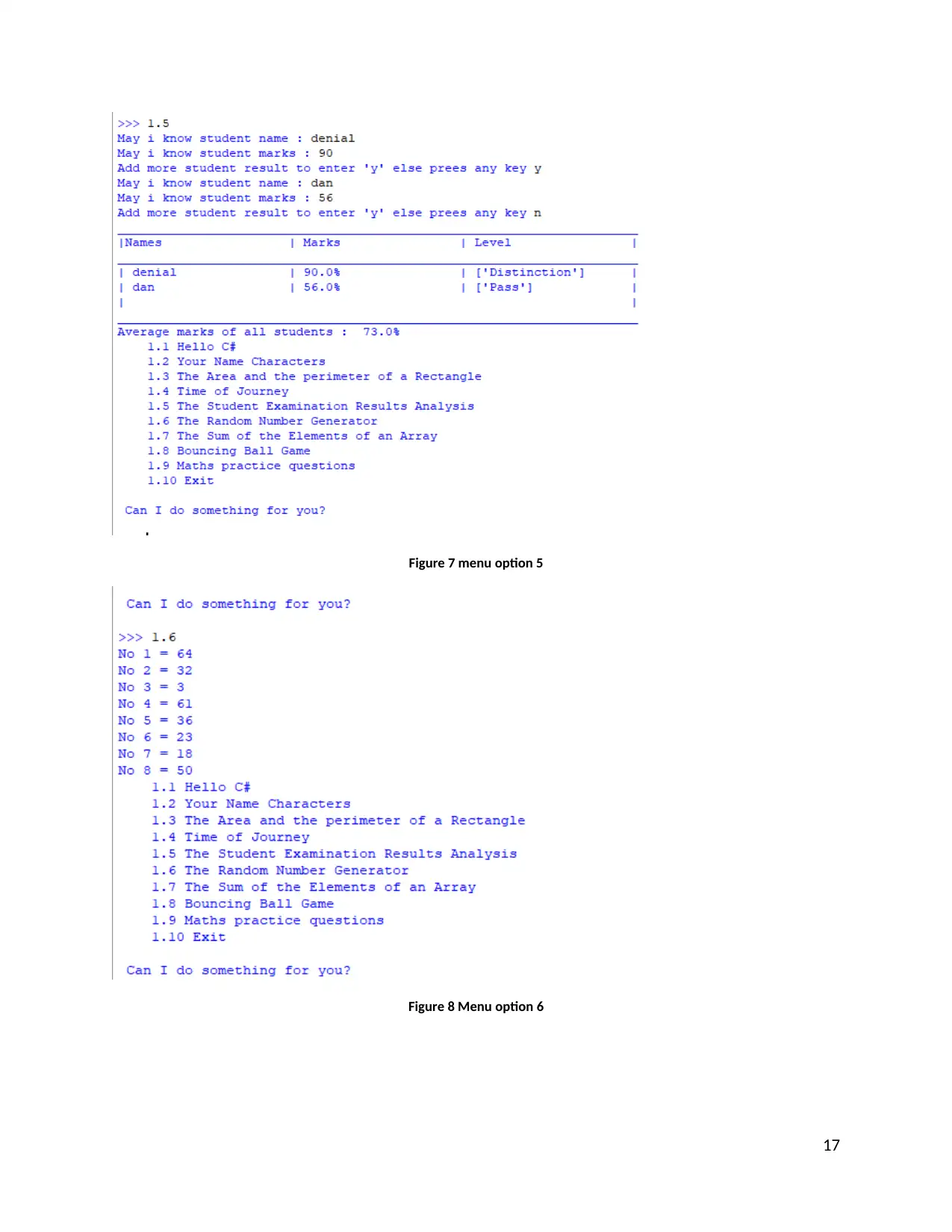
Figure 7 menu option 5
Figure 8 Menu option 6
17
Figure 8 Menu option 6
17
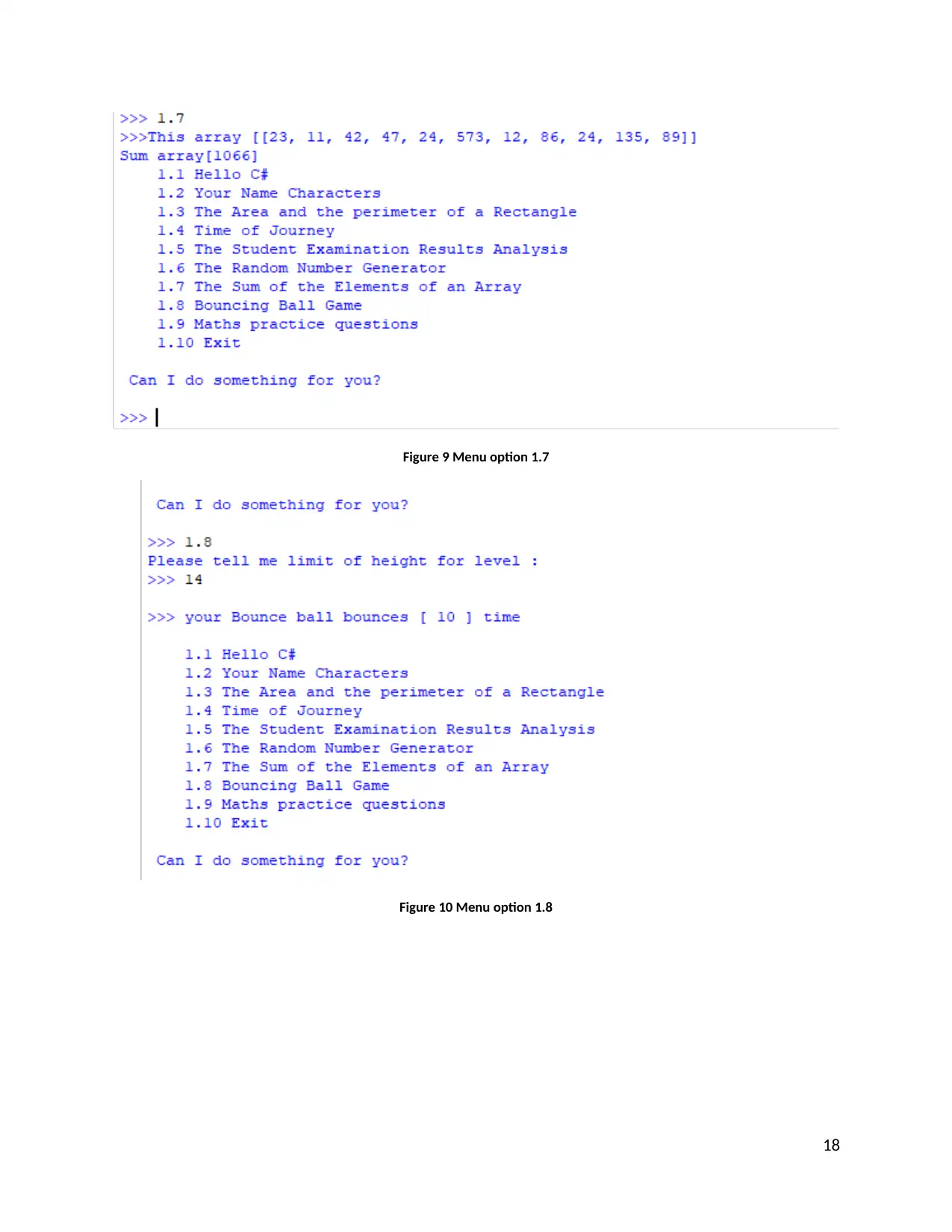
Figure 9 Menu option 1.7
Figure 10 Menu option 1.8
18
Figure 10 Menu option 1.8
18
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
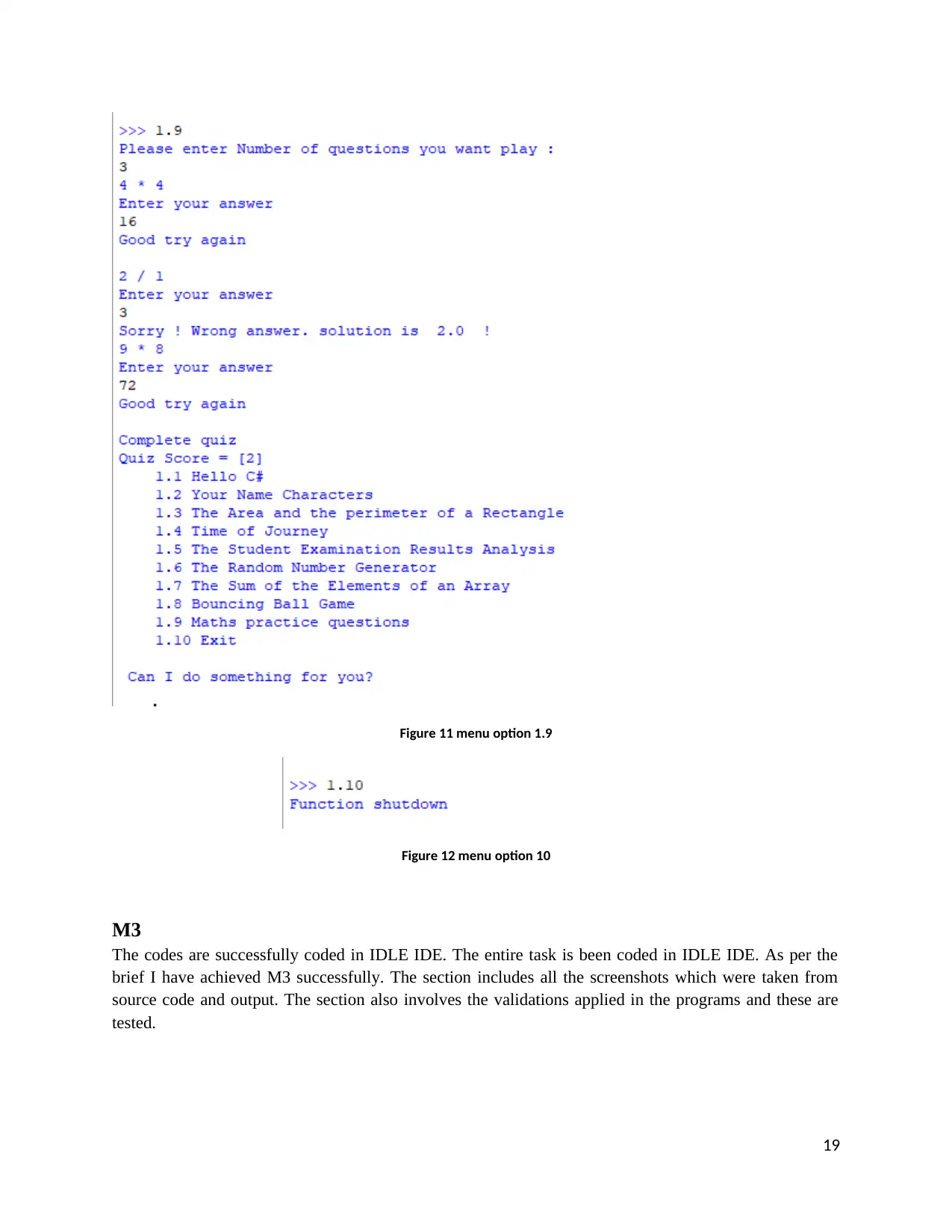
Figure 11 menu option 1.9
Figure 12 menu option 10
M3
The codes are successfully coded in IDLE IDE. The entire task is been coded in IDLE IDE. As per the
brief I have achieved M3 successfully. The section includes all the screenshots which were taken from
source code and output. The section also involves the validations applied in the programs and these are
tested.
19
Figure 12 menu option 10
M3
The codes are successfully coded in IDLE IDE. The entire task is been coded in IDLE IDE. As per the
brief I have achieved M3 successfully. The section includes all the screenshots which were taken from
source code and output. The section also involves the validations applied in the programs and these are
tested.
19
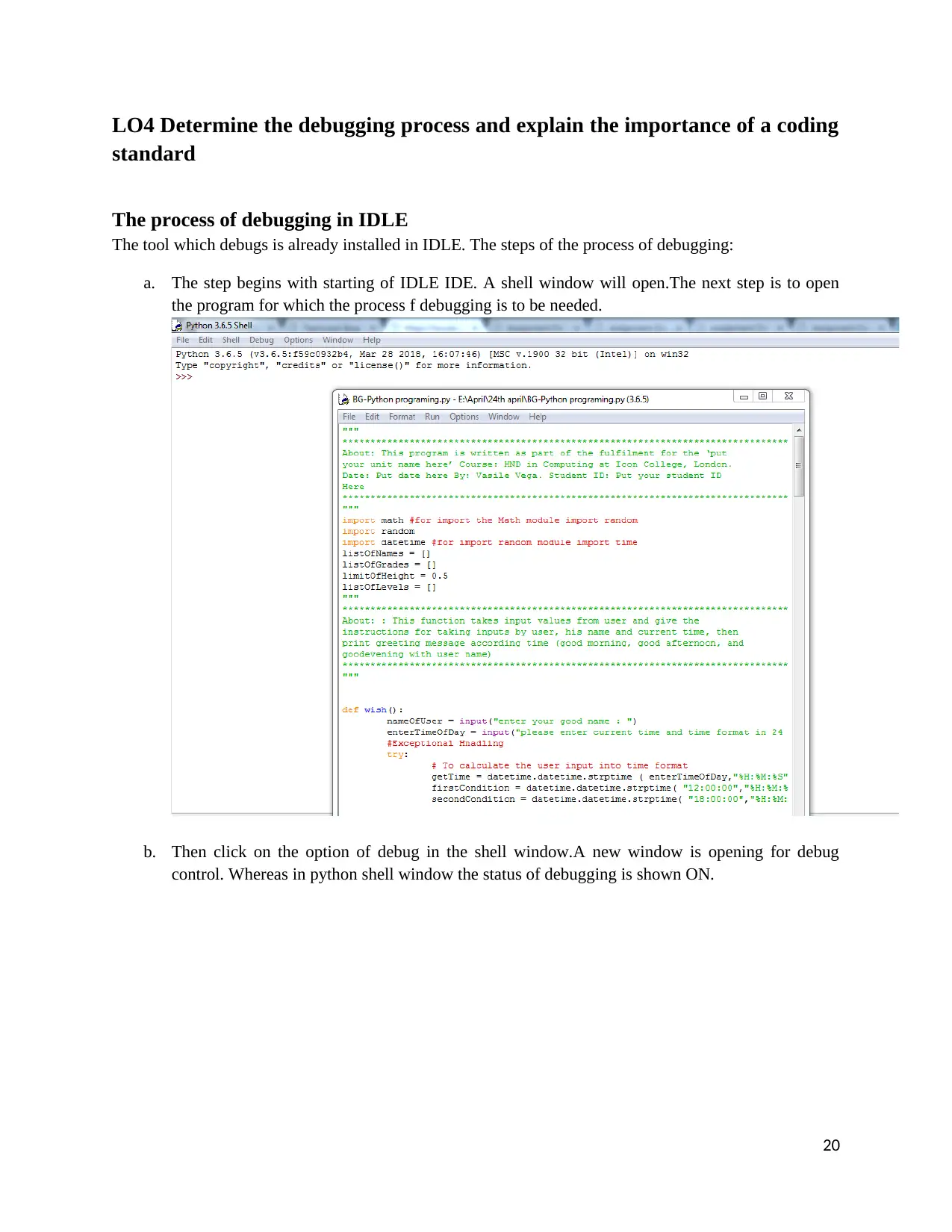
LO4 Determine the debugging process and explain the importance of a coding
standard
The process of debugging in IDLE
The tool which debugs is already installed in IDLE. The steps of the process of debugging:
a. The step begins with starting of IDLE IDE. A shell window will open.The next step is to open
the program for which the process f debugging is to be needed.
b. Then click on the option of debug in the shell window.A new window is opening for debug
control. Whereas in python shell window the status of debugging is shown ON.
20
standard
The process of debugging in IDLE
The tool which debugs is already installed in IDLE. The steps of the process of debugging:
a. The step begins with starting of IDLE IDE. A shell window will open.The next step is to open
the program for which the process f debugging is to be needed.
b. Then click on the option of debug in the shell window.A new window is opening for debug
control. Whereas in python shell window the status of debugging is shown ON.
20
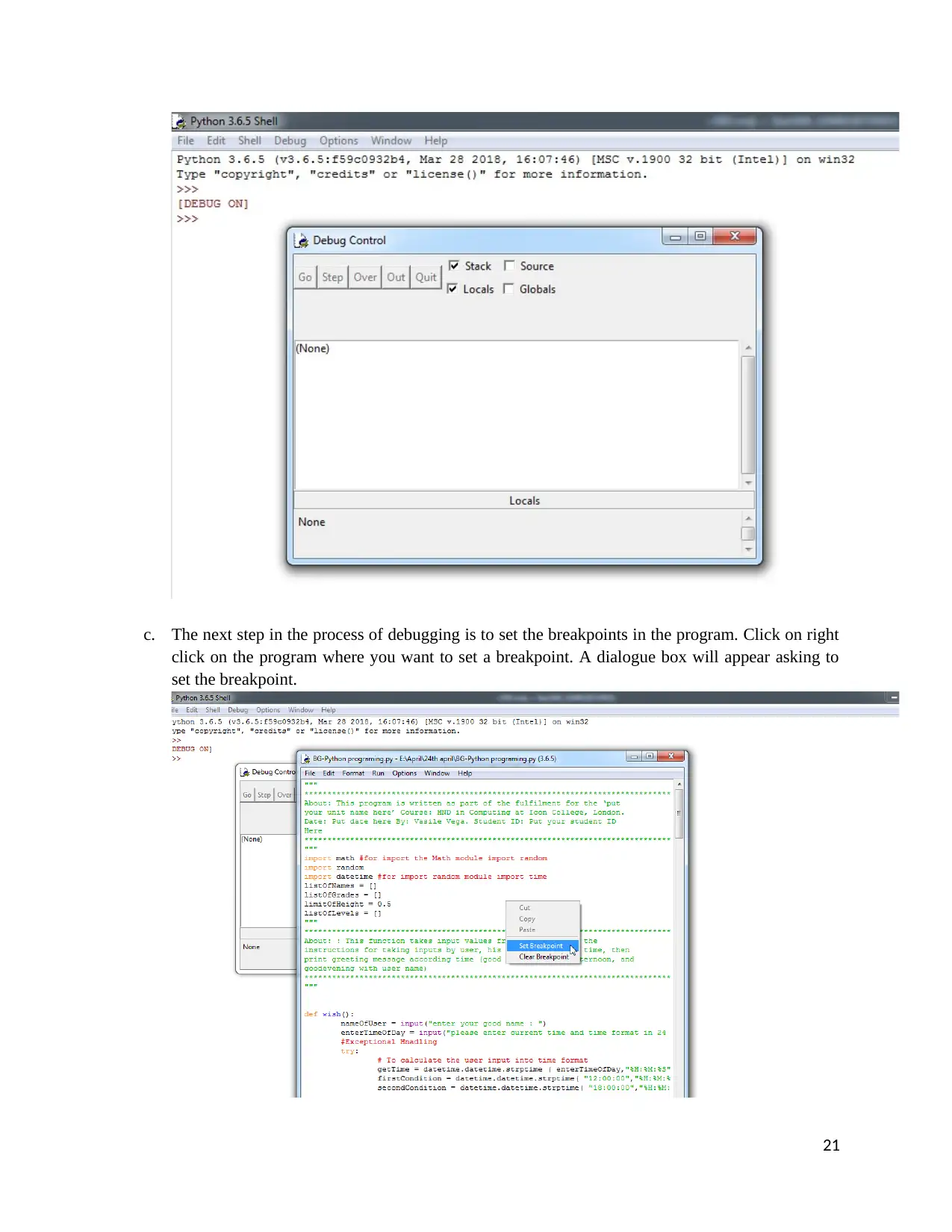
c. The next step in the process of debugging is to set the breakpoints in the program. Click on right
click on the program where you want to set a breakpoint. A dialogue box will appear asking to
set the breakpoint.
21
click on the program where you want to set a breakpoint. A dialogue box will appear asking to
set the breakpoint.
21
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
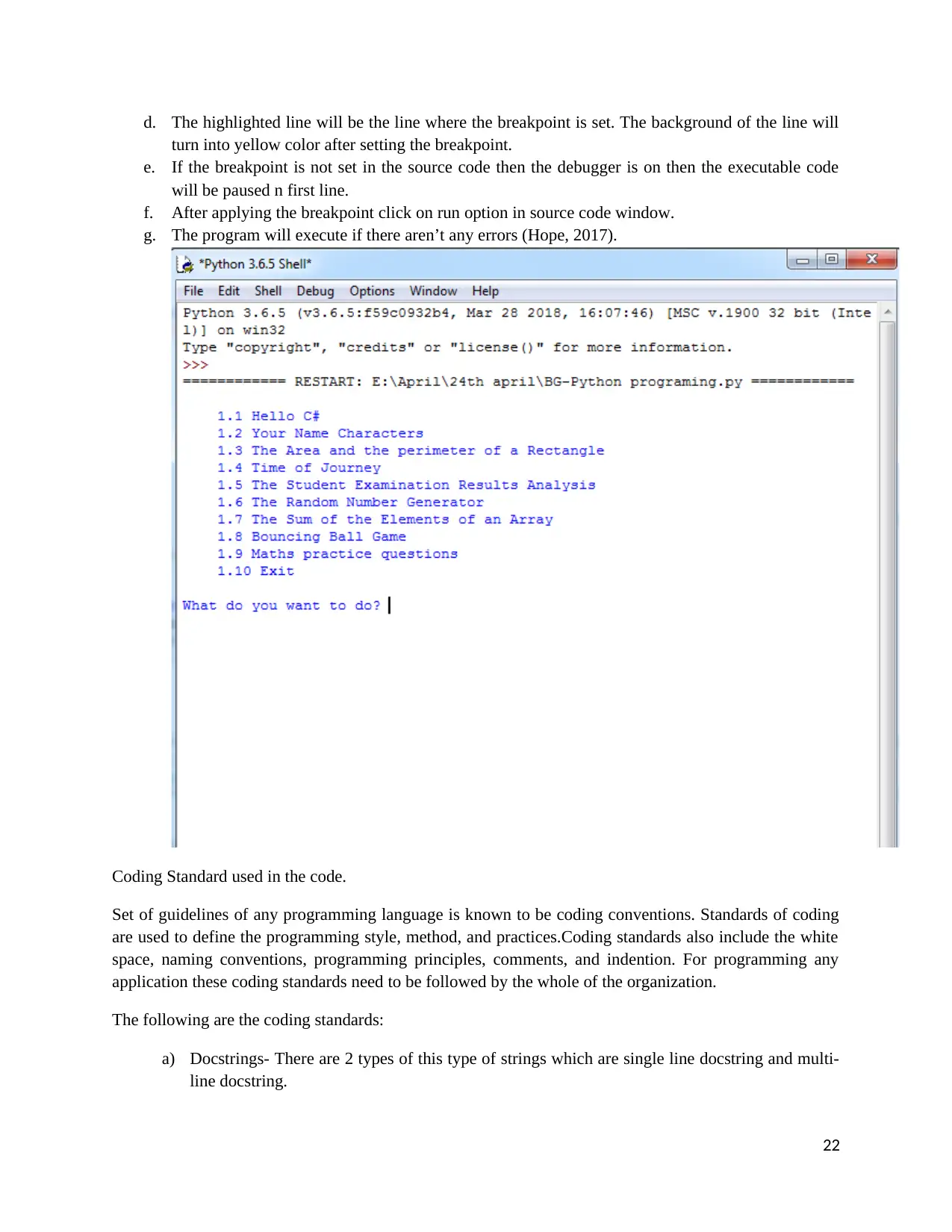
d. The highlighted line will be the line where the breakpoint is set. The background of the line will
turn into yellow color after setting the breakpoint.
e. If the breakpoint is not set in the source code then the debugger is on then the executable code
will be paused n first line.
f. After applying the breakpoint click on run option in source code window.
g. The program will execute if there aren’t any errors (Hope, 2017).
Coding Standard used in the code.
Set of guidelines of any programming language is known to be coding conventions. Standards of coding
are used to define the programming style, method, and practices.Coding standards also include the white
space, naming conventions, programming principles, comments, and indention. For programming any
application these coding standards need to be followed by the whole of the organization.
The following are the coding standards:
a) Docstrings- There are 2 types of this type of strings which are single line docstring and multi-
line docstring.
22
turn into yellow color after setting the breakpoint.
e. If the breakpoint is not set in the source code then the debugger is on then the executable code
will be paused n first line.
f. After applying the breakpoint click on run option in source code window.
g. The program will execute if there aren’t any errors (Hope, 2017).
Coding Standard used in the code.
Set of guidelines of any programming language is known to be coding conventions. Standards of coding
are used to define the programming style, method, and practices.Coding standards also include the white
space, naming conventions, programming principles, comments, and indention. For programming any
application these coding standards need to be followed by the whole of the organization.
The following are the coding standards:
a) Docstrings- There are 2 types of this type of strings which are single line docstring and multi-
line docstring.
22
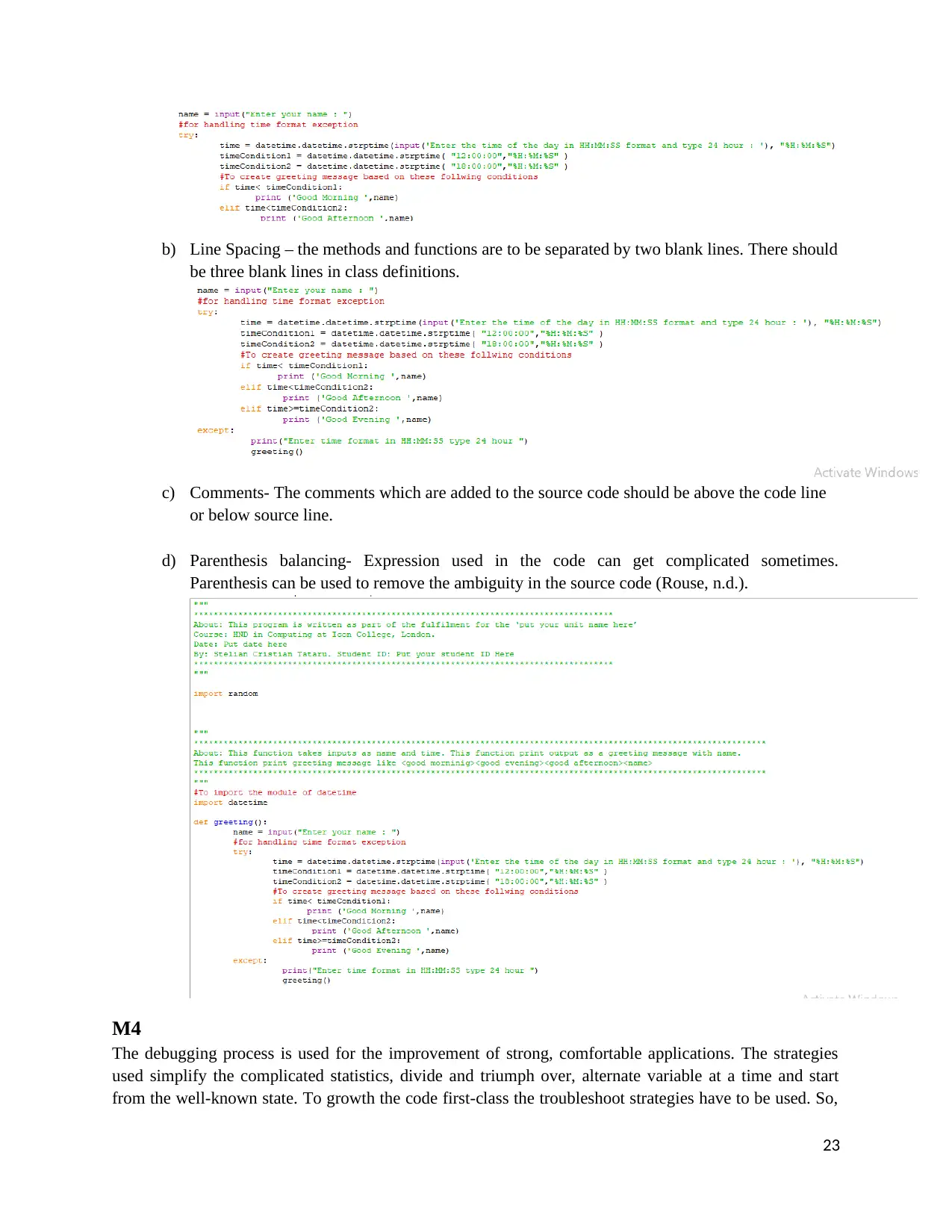
b) Line Spacing – the methods and functions are to be separated by two blank lines. There should
be three blank lines in class definitions.
c) Comments- The comments which are added to the source code should be above the code line
or below source line.
d) Parenthesis balancing- Expression used in the code can get complicated sometimes.
Parenthesis can be used to remove the ambiguity in the source code (Rouse, n.d.).
M4
The debugging process is used for the improvement of strong, comfortable applications. The strategies
used simplify the complicated statistics, divide and triumph over, alternate variable at a time and start
from the well-known state. To growth the code first-class the troubleshoot strategies have to be used. So,
23
be three blank lines in class definitions.
c) Comments- The comments which are added to the source code should be above the code line
or below source line.
d) Parenthesis balancing- Expression used in the code can get complicated sometimes.
Parenthesis can be used to remove the ambiguity in the source code (Rouse, n.d.).
M4
The debugging process is used for the improvement of strong, comfortable applications. The strategies
used simplify the complicated statistics, divide and triumph over, alternate variable at a time and start
from the well-known state. To growth the code first-class the troubleshoot strategies have to be used. So,
23
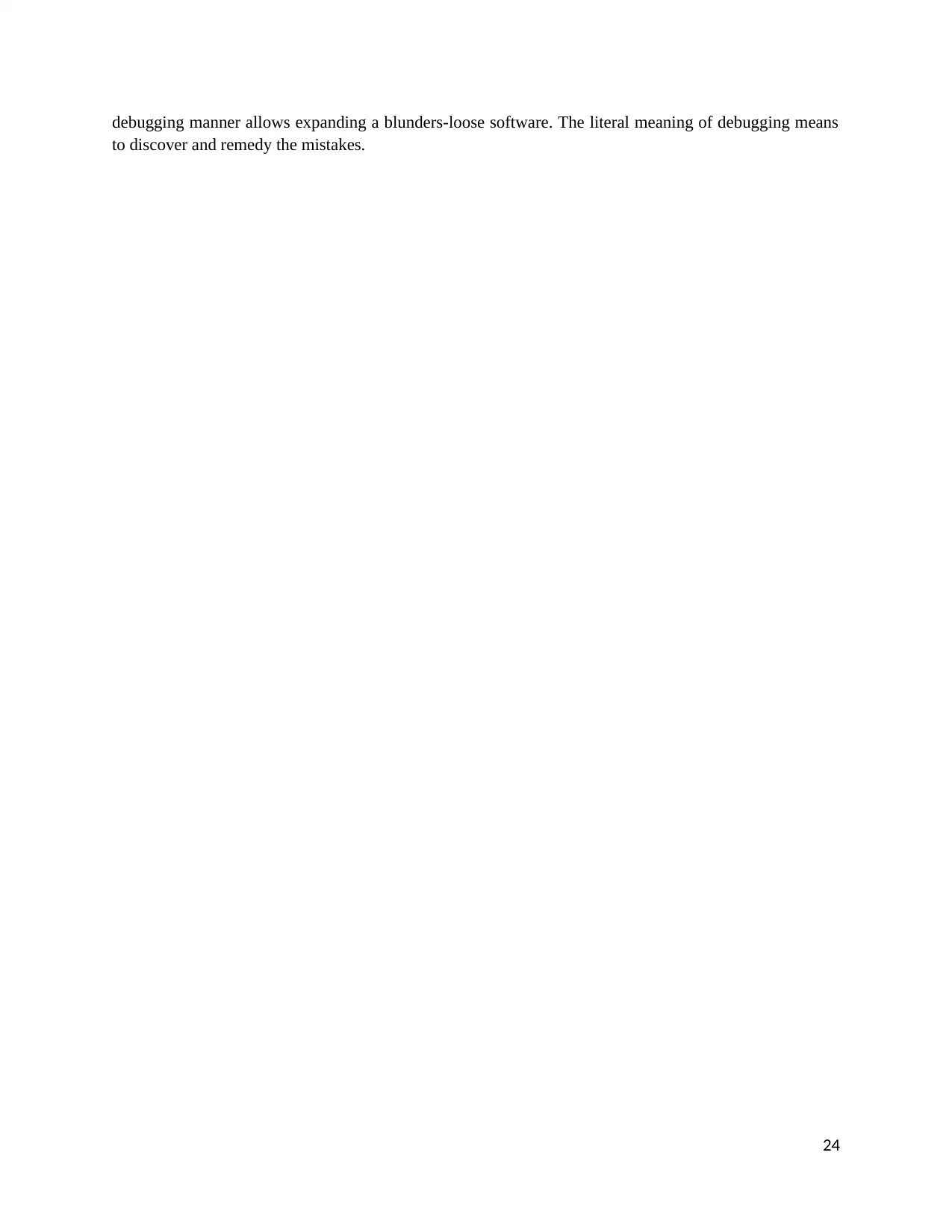
debugging manner allows expanding a blunders-loose software. The literal meaning of debugging means
to discover and remedy the mistakes.
24
to discover and remedy the mistakes.
24
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
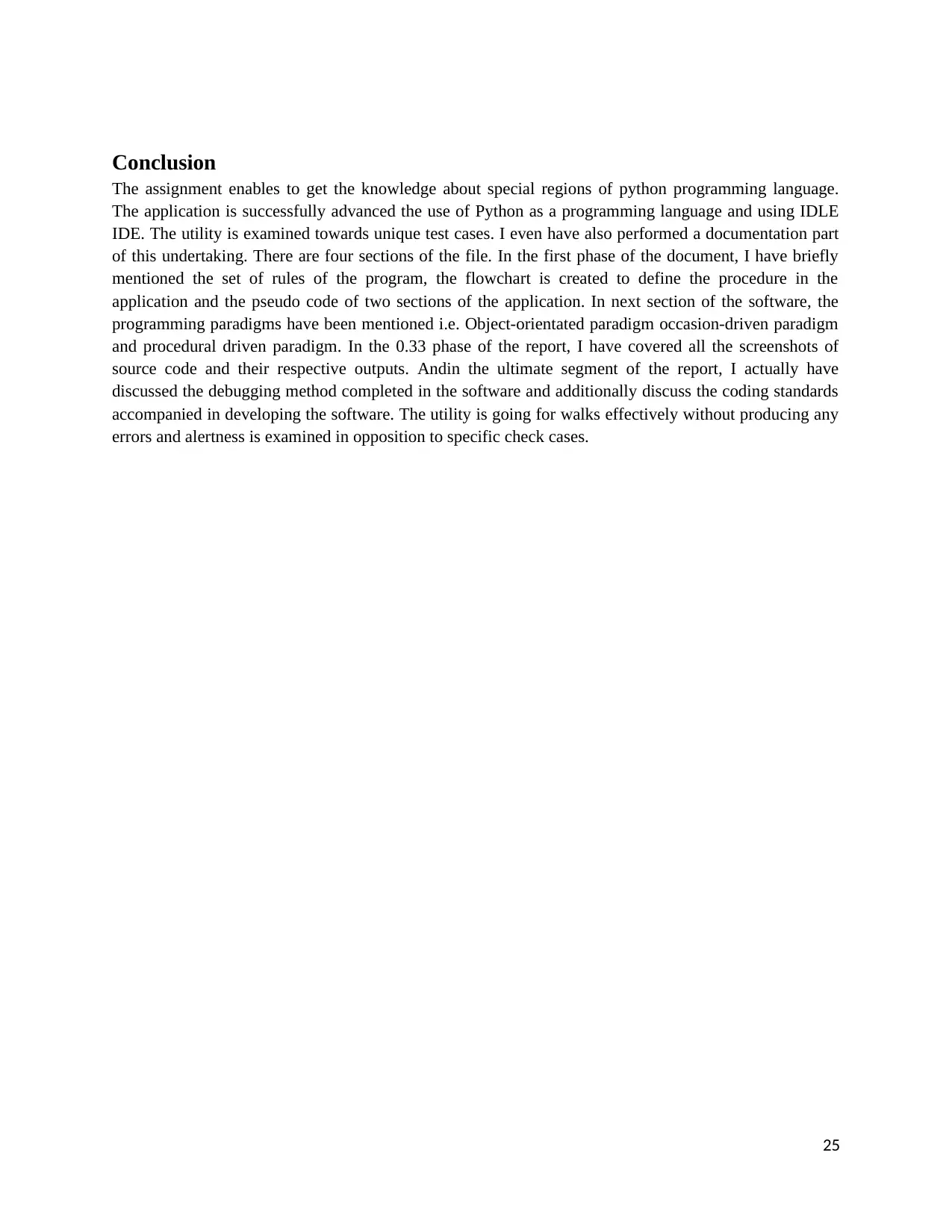
Conclusion
The assignment enables to get the knowledge about special regions of python programming language.
The application is successfully advanced the use of Python as a programming language and using IDLE
IDE. The utility is examined towards unique test cases. I even have also performed a documentation part
of this undertaking. There are four sections of the file. In the first phase of the document, I have briefly
mentioned the set of rules of the program, the flowchart is created to define the procedure in the
application and the pseudo code of two sections of the application. In next section of the software, the
programming paradigms have been mentioned i.e. Object-orientated paradigm occasion-driven paradigm
and procedural driven paradigm. In the 0.33 phase of the report, I have covered all the screenshots of
source code and their respective outputs. Andin the ultimate segment of the report, I actually have
discussed the debugging method completed in the software and additionally discuss the coding standards
accompanied in developing the software. The utility is going for walks effectively without producing any
errors and alertness is examined in opposition to specific check cases.
25
The assignment enables to get the knowledge about special regions of python programming language.
The application is successfully advanced the use of Python as a programming language and using IDLE
IDE. The utility is examined towards unique test cases. I even have also performed a documentation part
of this undertaking. There are four sections of the file. In the first phase of the document, I have briefly
mentioned the set of rules of the program, the flowchart is created to define the procedure in the
application and the pseudo code of two sections of the application. In next section of the software, the
programming paradigms have been mentioned i.e. Object-orientated paradigm occasion-driven paradigm
and procedural driven paradigm. In the 0.33 phase of the report, I have covered all the screenshots of
source code and their respective outputs. Andin the ultimate segment of the report, I actually have
discussed the debugging method completed in the software and additionally discuss the coding standards
accompanied in developing the software. The utility is going for walks effectively without producing any
errors and alertness is examined in opposition to specific check cases.
25
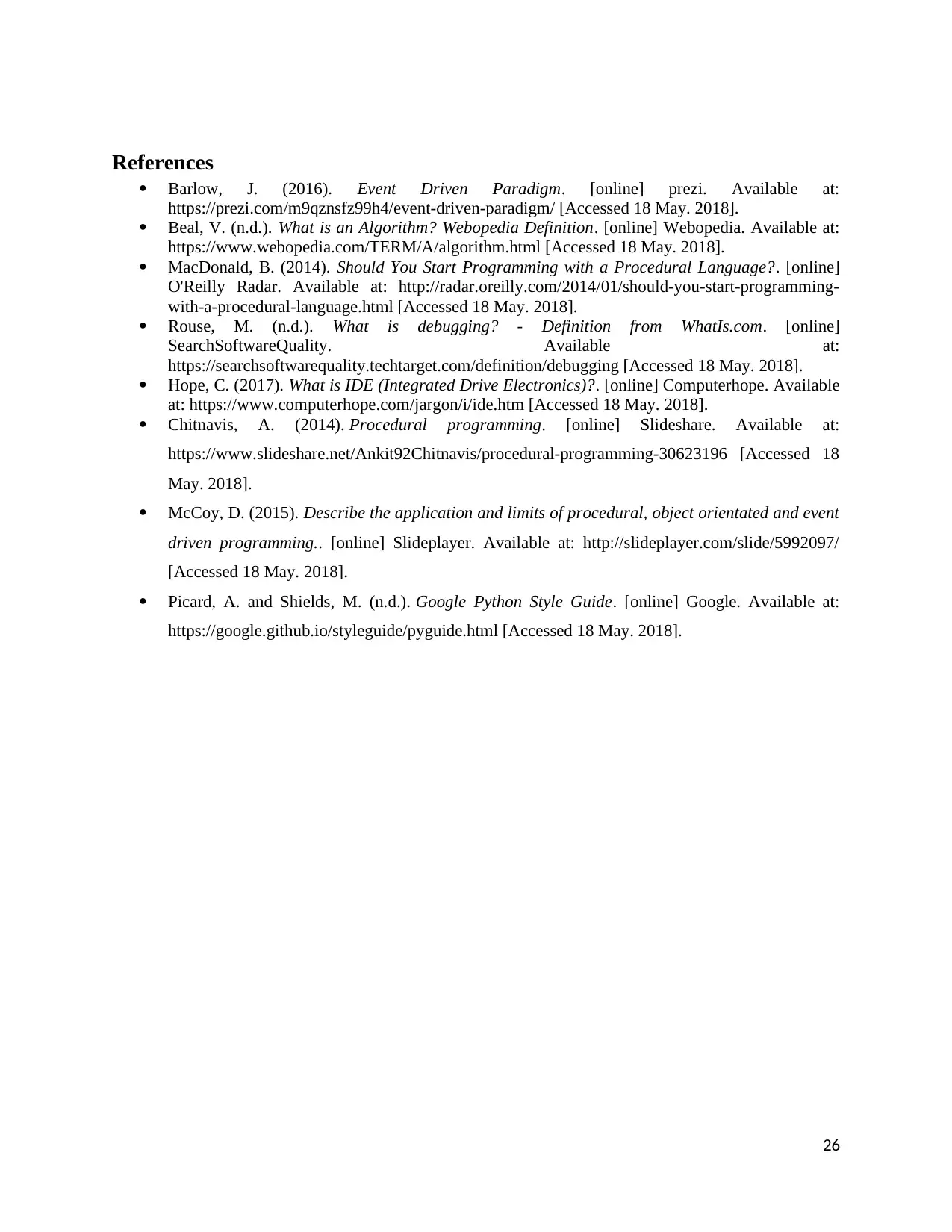
References
Barlow, J. (2016). Event Driven Paradigm. [online] prezi. Available at:
https://prezi.com/m9qznsfz99h4/event-driven-paradigm/ [Accessed 18 May. 2018].
Beal, V. (n.d.). What is an Algorithm? Webopedia Definition. [online] Webopedia. Available at:
https://www.webopedia.com/TERM/A/algorithm.html [Accessed 18 May. 2018].
MacDonald, B. (2014). Should You Start Programming with a Procedural Language?. [online]
O'Reilly Radar. Available at: http://radar.oreilly.com/2014/01/should-you-start-programming-
with-a-procedural-language.html [Accessed 18 May. 2018].
Rouse, M. (n.d.). What is debugging? - Definition from WhatIs.com. [online]
SearchSoftwareQuality. Available at:
https://searchsoftwarequality.techtarget.com/definition/debugging [Accessed 18 May. 2018].
Hope, C. (2017). What is IDE (Integrated Drive Electronics)?. [online] Computerhope. Available
at: https://www.computerhope.com/jargon/i/ide.htm [Accessed 18 May. 2018].
Chitnavis, A. (2014). Procedural programming. [online] Slideshare. Available at:
https://www.slideshare.net/Ankit92Chitnavis/procedural-programming-30623196 [Accessed 18
May. 2018].
McCoy, D. (2015). Describe the application and limits of procedural, object orientated and event
driven programming.. [online] Slideplayer. Available at: http://slideplayer.com/slide/5992097/
[Accessed 18 May. 2018].
Picard, A. and Shields, M. (n.d.). Google Python Style Guide. [online] Google. Available at:
https://google.github.io/styleguide/pyguide.html [Accessed 18 May. 2018].
26
Barlow, J. (2016). Event Driven Paradigm. [online] prezi. Available at:
https://prezi.com/m9qznsfz99h4/event-driven-paradigm/ [Accessed 18 May. 2018].
Beal, V. (n.d.). What is an Algorithm? Webopedia Definition. [online] Webopedia. Available at:
https://www.webopedia.com/TERM/A/algorithm.html [Accessed 18 May. 2018].
MacDonald, B. (2014). Should You Start Programming with a Procedural Language?. [online]
O'Reilly Radar. Available at: http://radar.oreilly.com/2014/01/should-you-start-programming-
with-a-procedural-language.html [Accessed 18 May. 2018].
Rouse, M. (n.d.). What is debugging? - Definition from WhatIs.com. [online]
SearchSoftwareQuality. Available at:
https://searchsoftwarequality.techtarget.com/definition/debugging [Accessed 18 May. 2018].
Hope, C. (2017). What is IDE (Integrated Drive Electronics)?. [online] Computerhope. Available
at: https://www.computerhope.com/jargon/i/ide.htm [Accessed 18 May. 2018].
Chitnavis, A. (2014). Procedural programming. [online] Slideshare. Available at:
https://www.slideshare.net/Ankit92Chitnavis/procedural-programming-30623196 [Accessed 18
May. 2018].
McCoy, D. (2015). Describe the application and limits of procedural, object orientated and event
driven programming.. [online] Slideplayer. Available at: http://slideplayer.com/slide/5992097/
[Accessed 18 May. 2018].
Picard, A. and Shields, M. (n.d.). Google Python Style Guide. [online] Google. Available at:
https://google.github.io/styleguide/pyguide.html [Accessed 18 May. 2018].
26
1 out of 27
Related Documents

Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.