Chip Credit Card Transaction & IMEI Validation
VerifiedAdded on 2019/09/20
|6
|1854
|462
Report
AI Summary
A programming assignment to implement I/O for a chip credit card transaction using Easy68K I/O tasks, with options to turn off keyboard echo. It also involves verifying the International Mobile Equipment Identity (IMEI) number using the Luhn-10 Algorithm.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
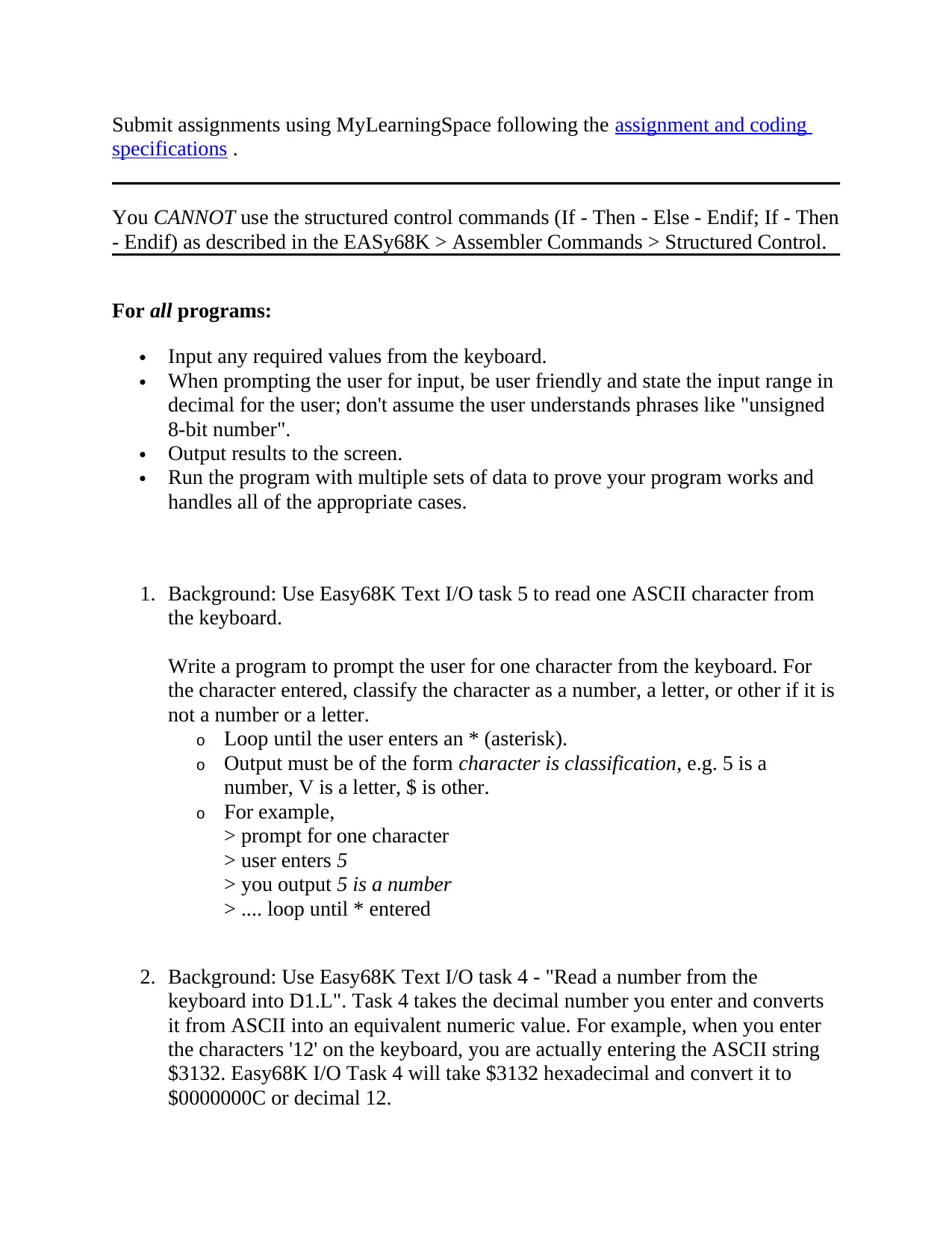
Submit assignments using MyLearningSpace following the assignment and coding
specifications .
You CANNOT use the structured control commands (If - Then - Else - Endif; If - Then
- Endif) as described in the EASy68K > Assembler Commands > Structured Control.
For all programs:
Input any required values from the keyboard.
When prompting the user for input, be user friendly and state the input range in
decimal for the user; don't assume the user understands phrases like "unsigned
8-bit number".
Output results to the screen.
Run the program with multiple sets of data to prove your program works and
handles all of the appropriate cases.
1. Background: Use Easy68K Text I/O task 5 to read one ASCII character from
the keyboard.
Write a program to prompt the user for one character from the keyboard. For
the character entered, classify the character as a number, a letter, or other if it is
not a number or a letter.
o Loop until the user enters an * (asterisk).
o Output must be of the form character is classification, e.g. 5 is a
number, V is a letter, $ is other.
o For example,
> prompt for one character
> user enters 5
> you output 5 is a number
> .... loop until * entered
2. Background: Use Easy68K Text I/O task 4 - "Read a number from the
keyboard into D1.L". Task 4 takes the decimal number you enter and converts
it from ASCII into an equivalent numeric value. For example, when you enter
the characters '12' on the keyboard, you are actually entering the ASCII string
$3132. Easy68K I/O Task 4 will take $3132 hexadecimal and convert it to
$0000000C or decimal 12.
specifications .
You CANNOT use the structured control commands (If - Then - Else - Endif; If - Then
- Endif) as described in the EASy68K > Assembler Commands > Structured Control.
For all programs:
Input any required values from the keyboard.
When prompting the user for input, be user friendly and state the input range in
decimal for the user; don't assume the user understands phrases like "unsigned
8-bit number".
Output results to the screen.
Run the program with multiple sets of data to prove your program works and
handles all of the appropriate cases.
1. Background: Use Easy68K Text I/O task 5 to read one ASCII character from
the keyboard.
Write a program to prompt the user for one character from the keyboard. For
the character entered, classify the character as a number, a letter, or other if it is
not a number or a letter.
o Loop until the user enters an * (asterisk).
o Output must be of the form character is classification, e.g. 5 is a
number, V is a letter, $ is other.
o For example,
> prompt for one character
> user enters 5
> you output 5 is a number
> .... loop until * entered
2. Background: Use Easy68K Text I/O task 4 - "Read a number from the
keyboard into D1.L". Task 4 takes the decimal number you enter and converts
it from ASCII into an equivalent numeric value. For example, when you enter
the characters '12' on the keyboard, you are actually entering the ASCII string
$3132. Easy68K I/O Task 4 will take $3132 hexadecimal and convert it to
$0000000C or decimal 12.
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
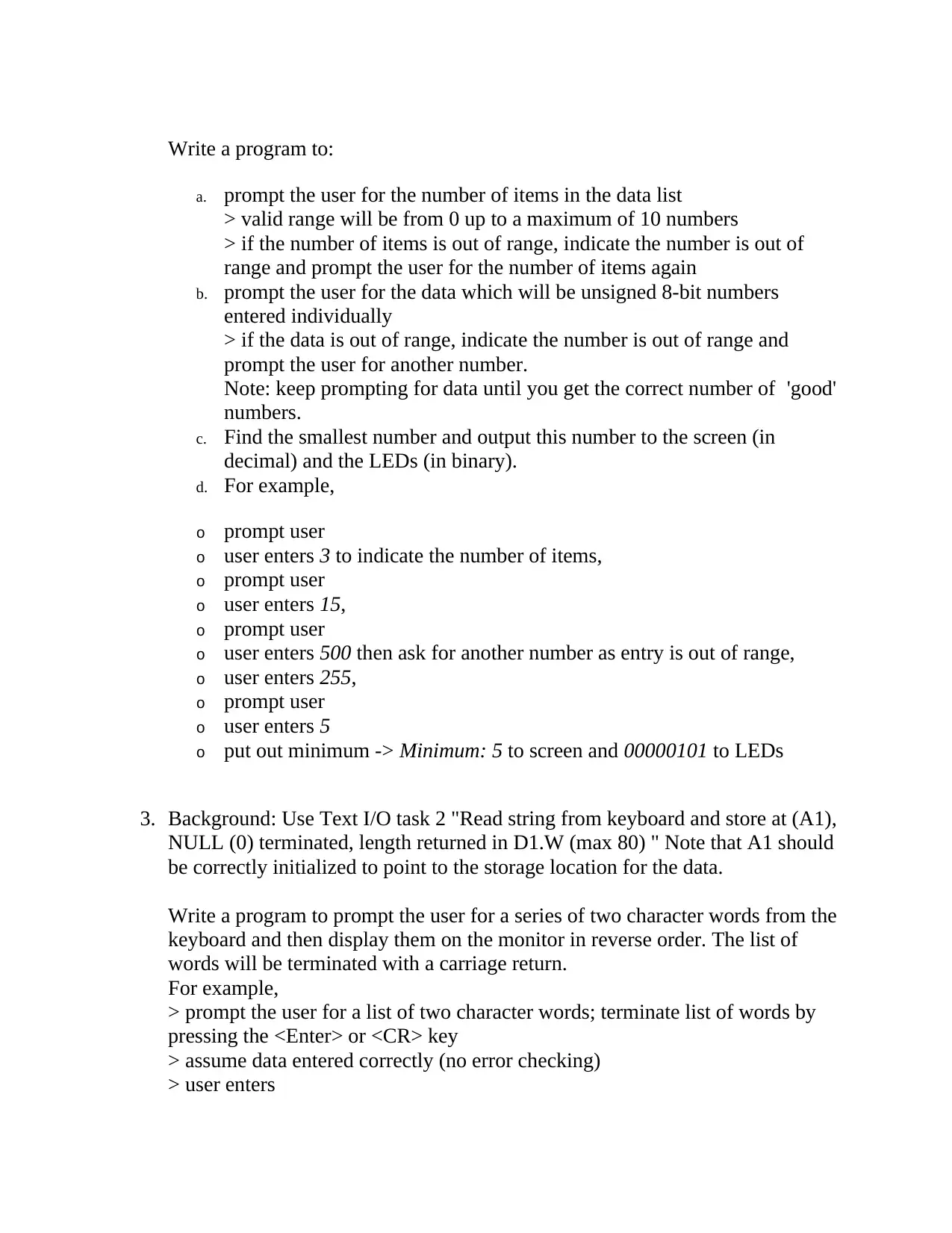
Write a program to:
a. prompt the user for the number of items in the data list
> valid range will be from 0 up to a maximum of 10 numbers
> if the number of items is out of range, indicate the number is out of
range and prompt the user for the number of items again
b. prompt the user for the data which will be unsigned 8-bit numbers
entered individually
> if the data is out of range, indicate the number is out of range and
prompt the user for another number.
Note: keep prompting for data until you get the correct number of 'good'
numbers.
c. Find the smallest number and output this number to the screen (in
decimal) and the LEDs (in binary).
d. For example,
o prompt user
o user enters 3 to indicate the number of items,
o prompt user
o user enters 15,
o prompt user
o user enters 500 then ask for another number as entry is out of range,
o user enters 255,
o prompt user
o user enters 5
o put out minimum -> Minimum: 5 to screen and 00000101 to LEDs
3. Background: Use Text I/O task 2 "Read string from keyboard and store at (A1),
NULL (0) terminated, length returned in D1.W (max 80) " Note that A1 should
be correctly initialized to point to the storage location for the data.
Write a program to prompt the user for a series of two character words from the
keyboard and then display them on the monitor in reverse order. The list of
words will be terminated with a carriage return.
For example,
> prompt the user for a list of two character words; terminate list of words by
pressing the <Enter> or <CR> key
> assume data entered correctly (no error checking)
> user enters
a. prompt the user for the number of items in the data list
> valid range will be from 0 up to a maximum of 10 numbers
> if the number of items is out of range, indicate the number is out of
range and prompt the user for the number of items again
b. prompt the user for the data which will be unsigned 8-bit numbers
entered individually
> if the data is out of range, indicate the number is out of range and
prompt the user for another number.
Note: keep prompting for data until you get the correct number of 'good'
numbers.
c. Find the smallest number and output this number to the screen (in
decimal) and the LEDs (in binary).
d. For example,
o prompt user
o user enters 3 to indicate the number of items,
o prompt user
o user enters 15,
o prompt user
o user enters 500 then ask for another number as entry is out of range,
o user enters 255,
o prompt user
o user enters 5
o put out minimum -> Minimum: 5 to screen and 00000101 to LEDs
3. Background: Use Text I/O task 2 "Read string from keyboard and store at (A1),
NULL (0) terminated, length returned in D1.W (max 80) " Note that A1 should
be correctly initialized to point to the storage location for the data.
Write a program to prompt the user for a series of two character words from the
keyboard and then display them on the monitor in reverse order. The list of
words will be terminated with a carriage return.
For example,
> prompt the user for a list of two character words; terminate list of words by
pressing the <Enter> or <CR> key
> assume data entered correctly (no error checking)
> user enters
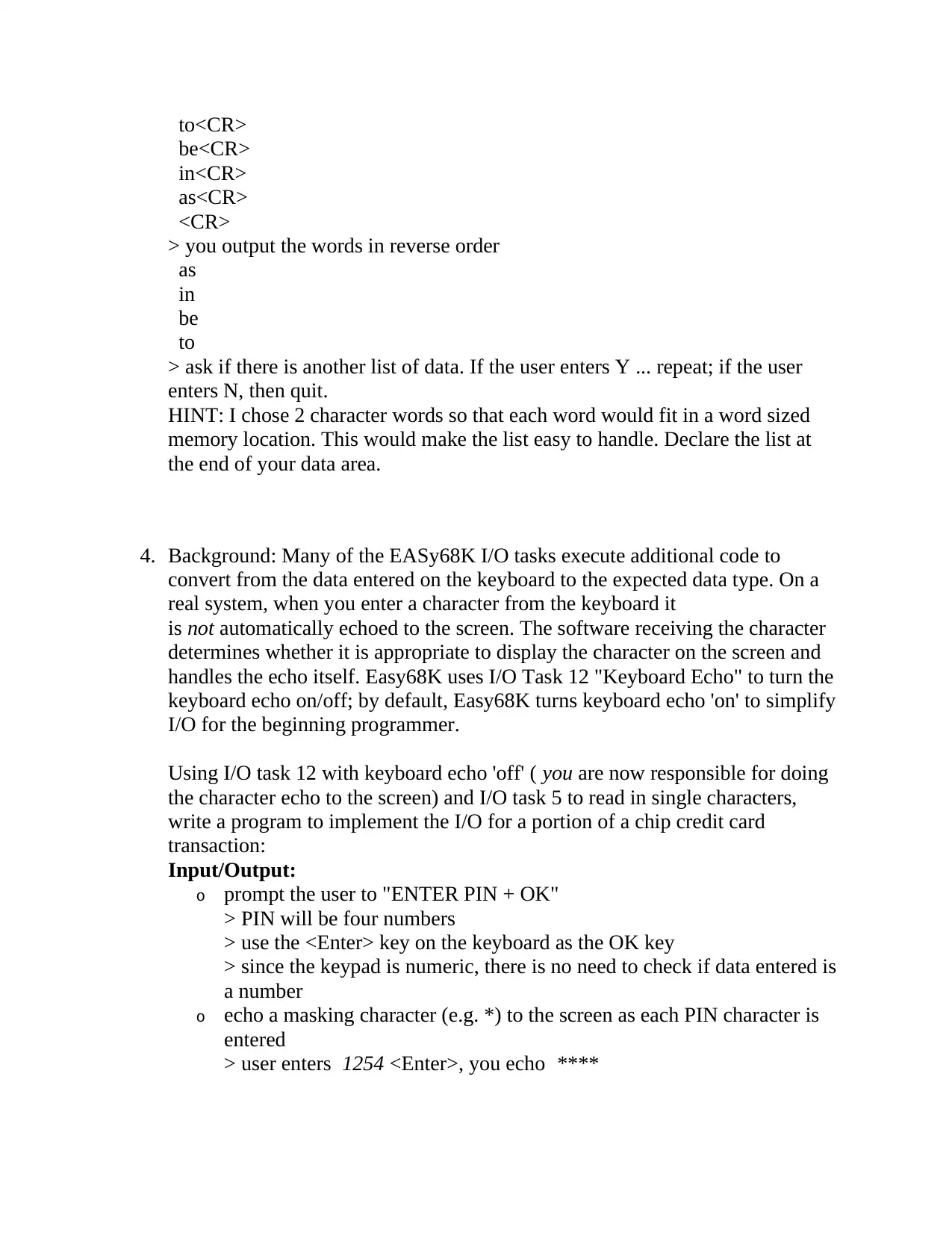
to<CR>
be<CR>
in<CR>
as<CR>
<CR>
> you output the words in reverse order
as
in
be
to
> ask if there is another list of data. If the user enters Y ... repeat; if the user
enters N, then quit.
HINT: I chose 2 character words so that each word would fit in a word sized
memory location. This would make the list easy to handle. Declare the list at
the end of your data area.
4. Background: Many of the EASy68K I/O tasks execute additional code to
convert from the data entered on the keyboard to the expected data type. On a
real system, when you enter a character from the keyboard it
is not automatically echoed to the screen. The software receiving the character
determines whether it is appropriate to display the character on the screen and
handles the echo itself. Easy68K uses I/O Task 12 "Keyboard Echo" to turn the
keyboard echo on/off; by default, Easy68K turns keyboard echo 'on' to simplify
I/O for the beginning programmer.
Using I/O task 12 with keyboard echo 'off' ( you are now responsible for doing
the character echo to the screen) and I/O task 5 to read in single characters,
write a program to implement the I/O for a portion of a chip credit card
transaction:
Input/Output:
o prompt the user to "ENTER PIN + OK"
> PIN will be four numbers
> use the <Enter> key on the keyboard as the OK key
> since the keypad is numeric, there is no need to check if data entered is
a number
o echo a masking character (e.g. *) to the screen as each PIN character is
entered
> user enters 1254 <Enter>, you echo ****
be<CR>
in<CR>
as<CR>
<CR>
> you output the words in reverse order
as
in
be
to
> ask if there is another list of data. If the user enters Y ... repeat; if the user
enters N, then quit.
HINT: I chose 2 character words so that each word would fit in a word sized
memory location. This would make the list easy to handle. Declare the list at
the end of your data area.
4. Background: Many of the EASy68K I/O tasks execute additional code to
convert from the data entered on the keyboard to the expected data type. On a
real system, when you enter a character from the keyboard it
is not automatically echoed to the screen. The software receiving the character
determines whether it is appropriate to display the character on the screen and
handles the echo itself. Easy68K uses I/O Task 12 "Keyboard Echo" to turn the
keyboard echo on/off; by default, Easy68K turns keyboard echo 'on' to simplify
I/O for the beginning programmer.
Using I/O task 12 with keyboard echo 'off' ( you are now responsible for doing
the character echo to the screen) and I/O task 5 to read in single characters,
write a program to implement the I/O for a portion of a chip credit card
transaction:
Input/Output:
o prompt the user to "ENTER PIN + OK"
> PIN will be four numbers
> use the <Enter> key on the keyboard as the OK key
> since the keypad is numeric, there is no need to check if data entered is
a number
o echo a masking character (e.g. *) to the screen as each PIN character is
entered
> user enters 1254 <Enter>, you echo ****
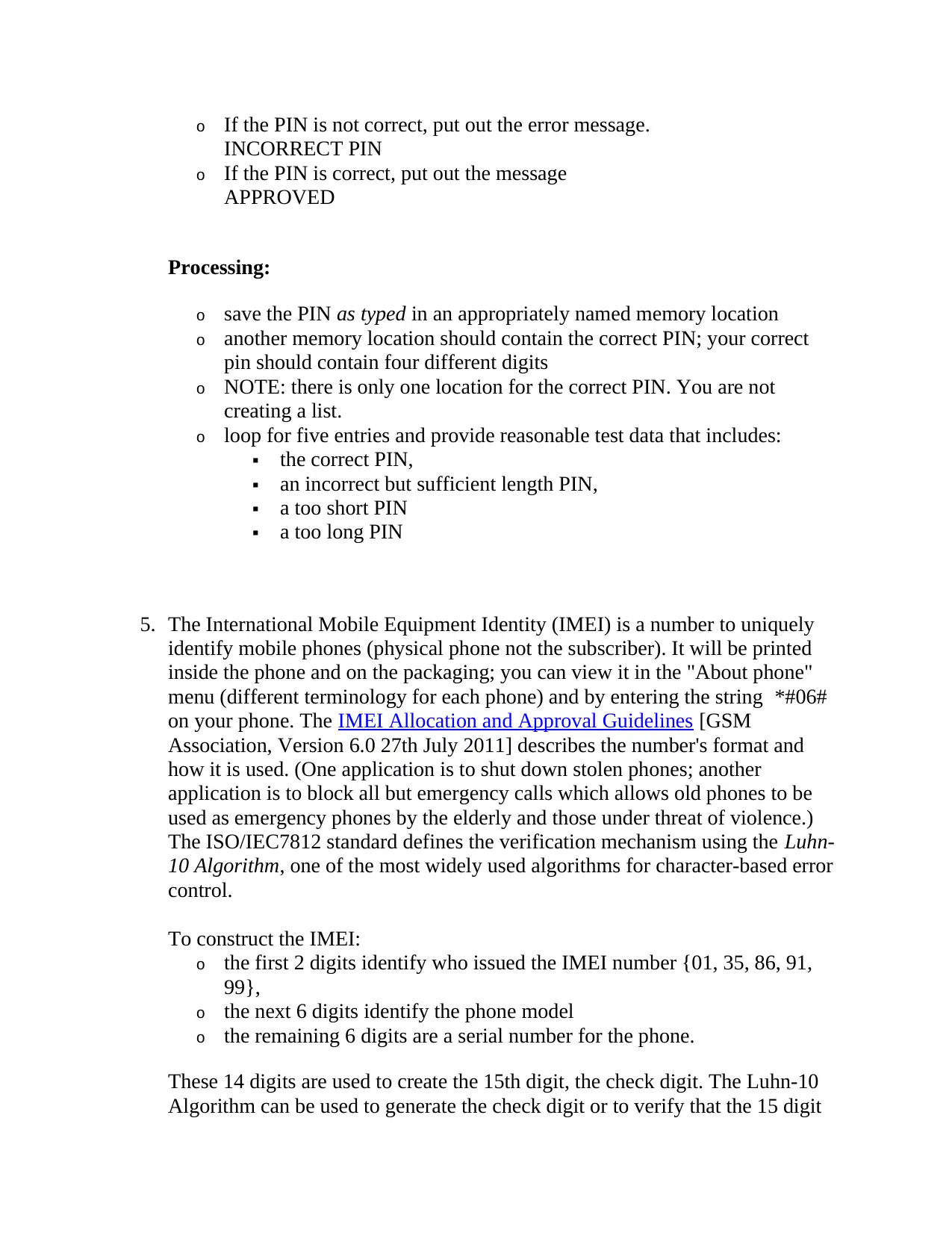
o If the PIN is not correct, put out the error message.
INCORRECT PIN
o If the PIN is correct, put out the message
APPROVED
Processing:
o save the PIN as typed in an appropriately named memory location
o another memory location should contain the correct PIN; your correct
pin should contain four different digits
o NOTE: there is only one location for the correct PIN. You are not
creating a list.
o loop for five entries and provide reasonable test data that includes:
the correct PIN,
an incorrect but sufficient length PIN,
a too short PIN
a too long PIN
5. The International Mobile Equipment Identity (IMEI) is a number to uniquely
identify mobile phones (physical phone not the subscriber). It will be printed
inside the phone and on the packaging; you can view it in the "About phone"
menu (different terminology for each phone) and by entering the string *#06#
on your phone. The IMEI Allocation and Approval Guidelines [GSM
Association, Version 6.0 27th July 2011] describes the number's format and
how it is used. (One application is to shut down stolen phones; another
application is to block all but emergency calls which allows old phones to be
used as emergency phones by the elderly and those under threat of violence.)
The ISO/IEC7812 standard defines the verification mechanism using the Luhn-
10 Algorithm, one of the most widely used algorithms for character-based error
control.
To construct the IMEI:
o the first 2 digits identify who issued the IMEI number {01, 35, 86, 91,
99},
o the next 6 digits identify the phone model
o the remaining 6 digits are a serial number for the phone.
These 14 digits are used to create the 15th digit, the check digit. The Luhn-10
Algorithm can be used to generate the check digit or to verify that the 15 digit
INCORRECT PIN
o If the PIN is correct, put out the message
APPROVED
Processing:
o save the PIN as typed in an appropriately named memory location
o another memory location should contain the correct PIN; your correct
pin should contain four different digits
o NOTE: there is only one location for the correct PIN. You are not
creating a list.
o loop for five entries and provide reasonable test data that includes:
the correct PIN,
an incorrect but sufficient length PIN,
a too short PIN
a too long PIN
5. The International Mobile Equipment Identity (IMEI) is a number to uniquely
identify mobile phones (physical phone not the subscriber). It will be printed
inside the phone and on the packaging; you can view it in the "About phone"
menu (different terminology for each phone) and by entering the string *#06#
on your phone. The IMEI Allocation and Approval Guidelines [GSM
Association, Version 6.0 27th July 2011] describes the number's format and
how it is used. (One application is to shut down stolen phones; another
application is to block all but emergency calls which allows old phones to be
used as emergency phones by the elderly and those under threat of violence.)
The ISO/IEC7812 standard defines the verification mechanism using the Luhn-
10 Algorithm, one of the most widely used algorithms for character-based error
control.
To construct the IMEI:
o the first 2 digits identify who issued the IMEI number {01, 35, 86, 91,
99},
o the next 6 digits identify the phone model
o the remaining 6 digits are a serial number for the phone.
These 14 digits are used to create the 15th digit, the check digit. The Luhn-10
Algorithm can be used to generate the check digit or to verify that the 15 digit
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
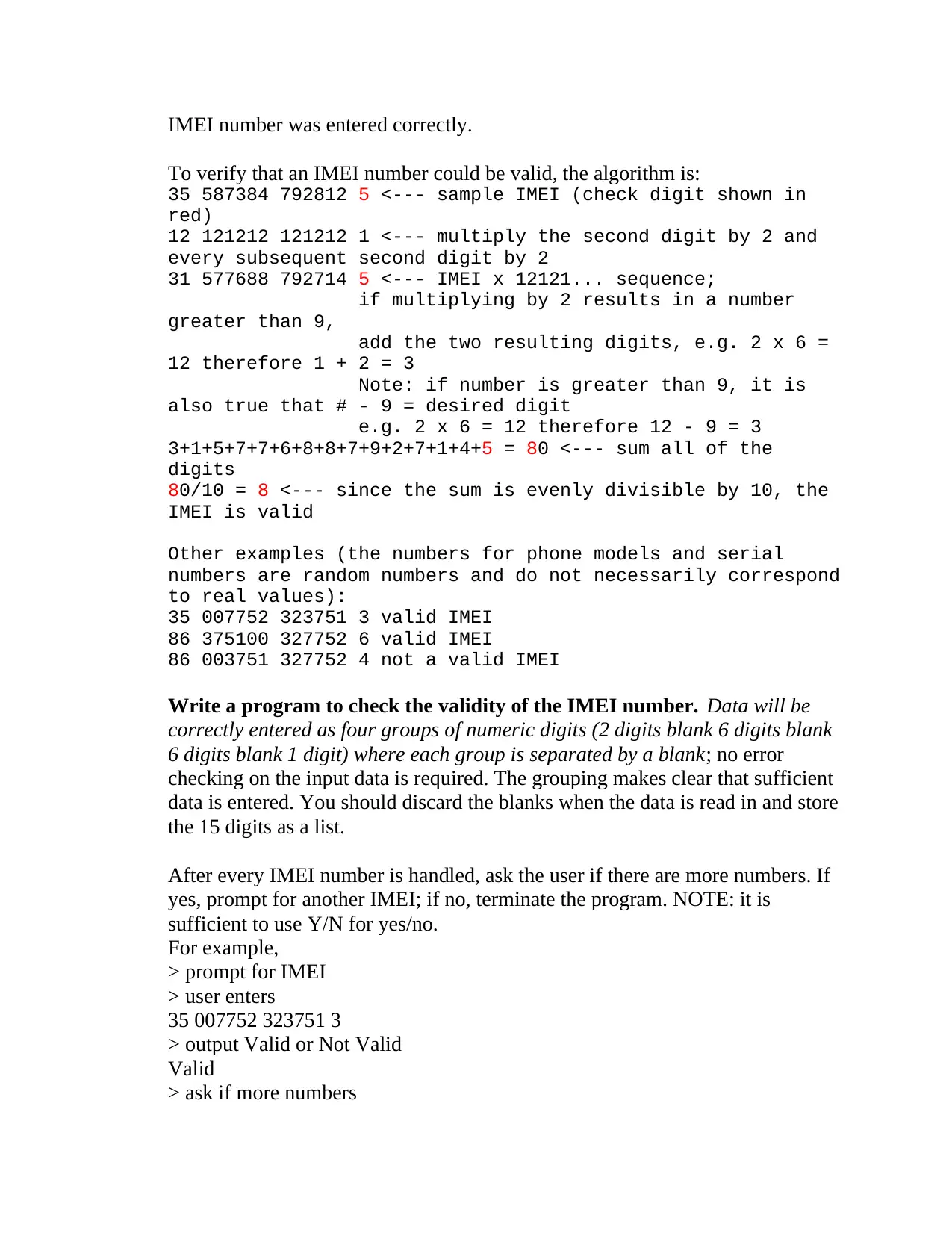
IMEI number was entered correctly.
To verify that an IMEI number could be valid, the algorithm is:
35 587384 792812 5 <--- sample IMEI (check digit shown in
red)
12 121212 121212 1 <--- multiply the second digit by 2 and
every subsequent second digit by 2
31 577688 792714 5 <--- IMEI x 12121... sequence;
if multiplying by 2 results in a number
greater than 9,
add the two resulting digits, e.g. 2 x 6 =
12 therefore 1 + 2 = 3
Note: if number is greater than 9, it is
also true that # - 9 = desired digit
e.g. 2 x 6 = 12 therefore 12 - 9 = 3
3+1+5+7+7+6+8+8+7+9+2+7+1+4+5 = 80 <--- sum all of the
digits
80/10 = 8 <--- since the sum is evenly divisible by 10, the
IMEI is valid
Other examples (the numbers for phone models and serial
numbers are random numbers and do not necessarily correspond
to real values):
35 007752 323751 3 valid IMEI
86 375100 327752 6 valid IMEI
86 003751 327752 4 not a valid IMEI
Write a program to check the validity of the IMEI number. Data will be
correctly entered as four groups of numeric digits (2 digits blank 6 digits blank
6 digits blank 1 digit) where each group is separated by a blank; no error
checking on the input data is required. The grouping makes clear that sufficient
data is entered. You should discard the blanks when the data is read in and store
the 15 digits as a list.
After every IMEI number is handled, ask the user if there are more numbers. If
yes, prompt for another IMEI; if no, terminate the program. NOTE: it is
sufficient to use Y/N for yes/no.
For example,
> prompt for IMEI
> user enters
35 007752 323751 3
> output Valid or Not Valid
Valid
> ask if more numbers
To verify that an IMEI number could be valid, the algorithm is:
35 587384 792812 5 <--- sample IMEI (check digit shown in
red)
12 121212 121212 1 <--- multiply the second digit by 2 and
every subsequent second digit by 2
31 577688 792714 5 <--- IMEI x 12121... sequence;
if multiplying by 2 results in a number
greater than 9,
add the two resulting digits, e.g. 2 x 6 =
12 therefore 1 + 2 = 3
Note: if number is greater than 9, it is
also true that # - 9 = desired digit
e.g. 2 x 6 = 12 therefore 12 - 9 = 3
3+1+5+7+7+6+8+8+7+9+2+7+1+4+5 = 80 <--- sum all of the
digits
80/10 = 8 <--- since the sum is evenly divisible by 10, the
IMEI is valid
Other examples (the numbers for phone models and serial
numbers are random numbers and do not necessarily correspond
to real values):
35 007752 323751 3 valid IMEI
86 375100 327752 6 valid IMEI
86 003751 327752 4 not a valid IMEI
Write a program to check the validity of the IMEI number. Data will be
correctly entered as four groups of numeric digits (2 digits blank 6 digits blank
6 digits blank 1 digit) where each group is separated by a blank; no error
checking on the input data is required. The grouping makes clear that sufficient
data is entered. You should discard the blanks when the data is read in and store
the 15 digits as a list.
After every IMEI number is handled, ask the user if there are more numbers. If
yes, prompt for another IMEI; if no, terminate the program. NOTE: it is
sufficient to use Y/N for yes/no.
For example,
> prompt for IMEI
> user enters
35 007752 323751 3
> output Valid or Not Valid
Valid
> ask if more numbers
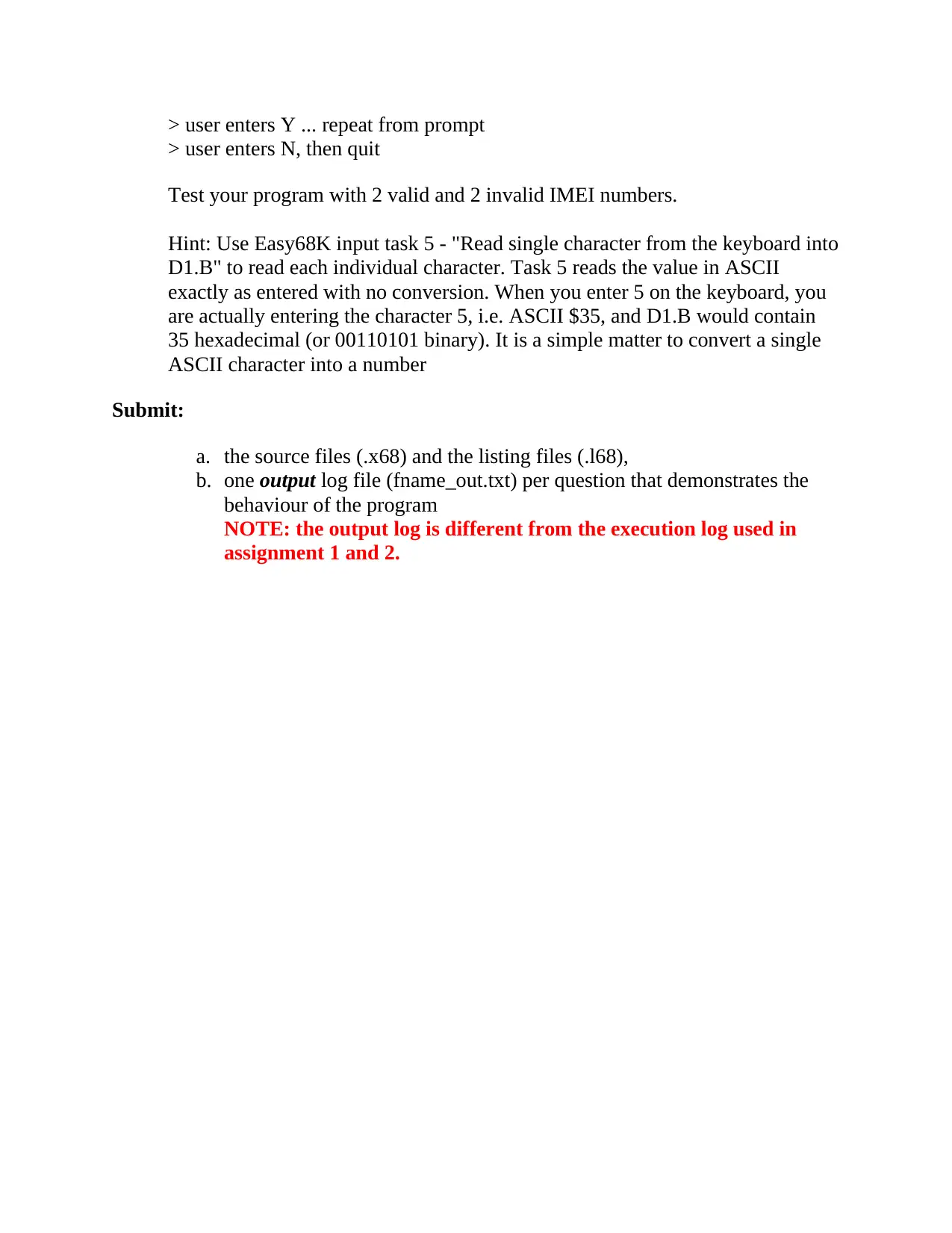
> user enters Y ... repeat from prompt
> user enters N, then quit
Test your program with 2 valid and 2 invalid IMEI numbers.
Hint: Use Easy68K input task 5 - "Read single character from the keyboard into
D1.B" to read each individual character. Task 5 reads the value in ASCII
exactly as entered with no conversion. When you enter 5 on the keyboard, you
are actually entering the character 5, i.e. ASCII $35, and D1.B would contain
35 hexadecimal (or 00110101 binary). It is a simple matter to convert a single
ASCII character into a number
Submit:
a. the source files (.x68) and the listing files (.l68),
b. one output log file (fname_out.txt) per question that demonstrates the
behaviour of the program
NOTE: the output log is different from the execution log used in
assignment 1 and 2.
> user enters N, then quit
Test your program with 2 valid and 2 invalid IMEI numbers.
Hint: Use Easy68K input task 5 - "Read single character from the keyboard into
D1.B" to read each individual character. Task 5 reads the value in ASCII
exactly as entered with no conversion. When you enter 5 on the keyboard, you
are actually entering the character 5, i.e. ASCII $35, and D1.B would contain
35 hexadecimal (or 00110101 binary). It is a simple matter to convert a single
ASCII character into a number
Submit:
a. the source files (.x68) and the listing files (.l68),
b. one output log file (fname_out.txt) per question that demonstrates the
behaviour of the program
NOTE: the output log is different from the execution log used in
assignment 1 and 2.
1 out of 6
Related Documents
![[object Object]](/_next/image/?url=%2F_next%2Fstatic%2Fmedia%2Flogo.6d15ce61.png&w=640&q=75)
Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.