Wood Flooring Cost Estimation: A Java Programming Solution
VerifiedAdded on 2024/05/29
|51
|4824
|187
AI Summary
This report details the development and implementation of a Java application designed to calculate wood flooring costs. The application utilizes object-oriented programming principles to estimate area, time, and cost based on user-provided room dimensions. The report includes UML diagrams, code examples, test cases, and user feedback analysis, demonstrating the application's functionality and effectiveness. It also provides technical documentation for deployment and maintenance.
Contribute Materials
Your contribution can guide someone’s learning journey. Share your
documents today.
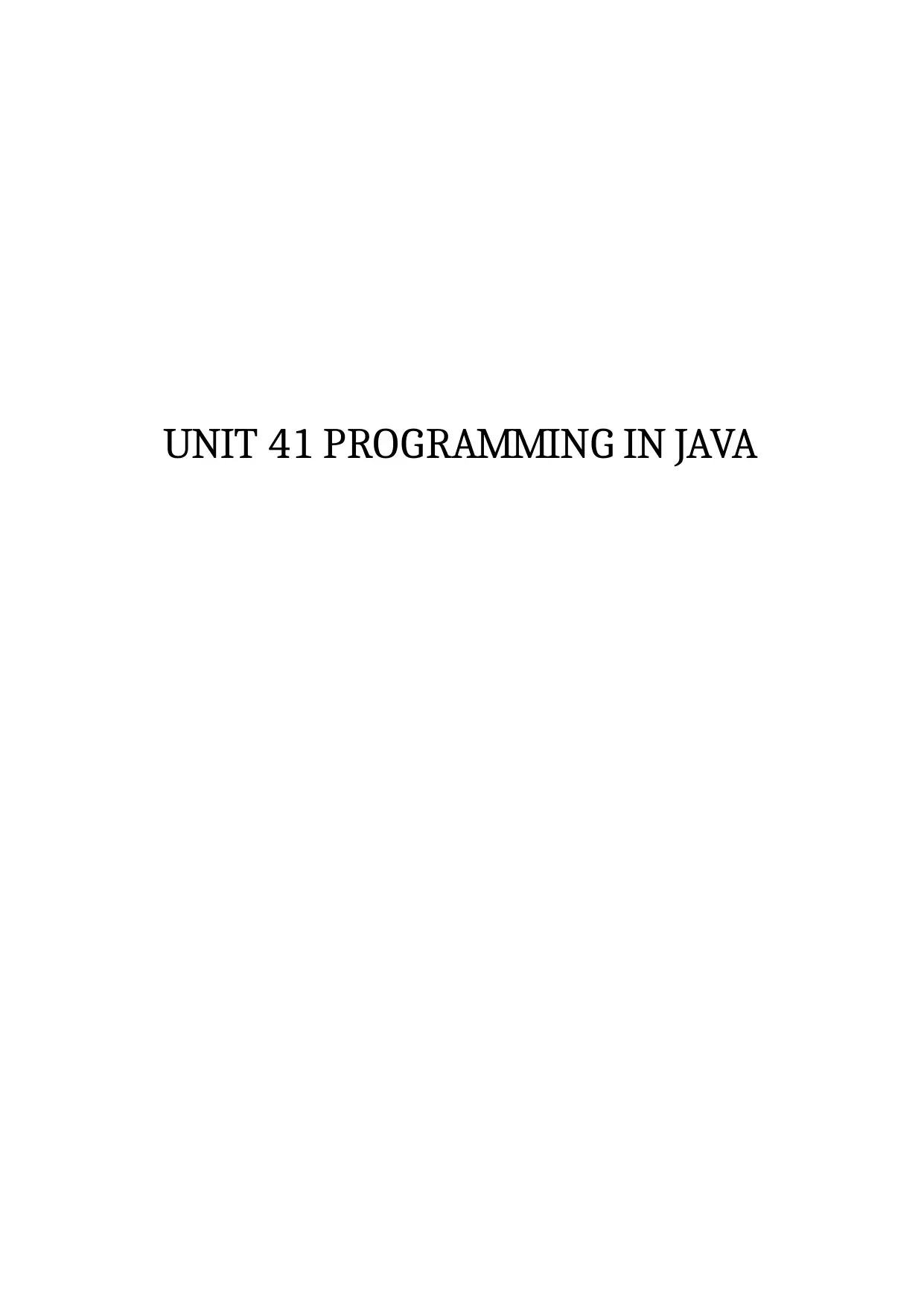
UNIT 41 PROGRAMMING IN JAVA
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
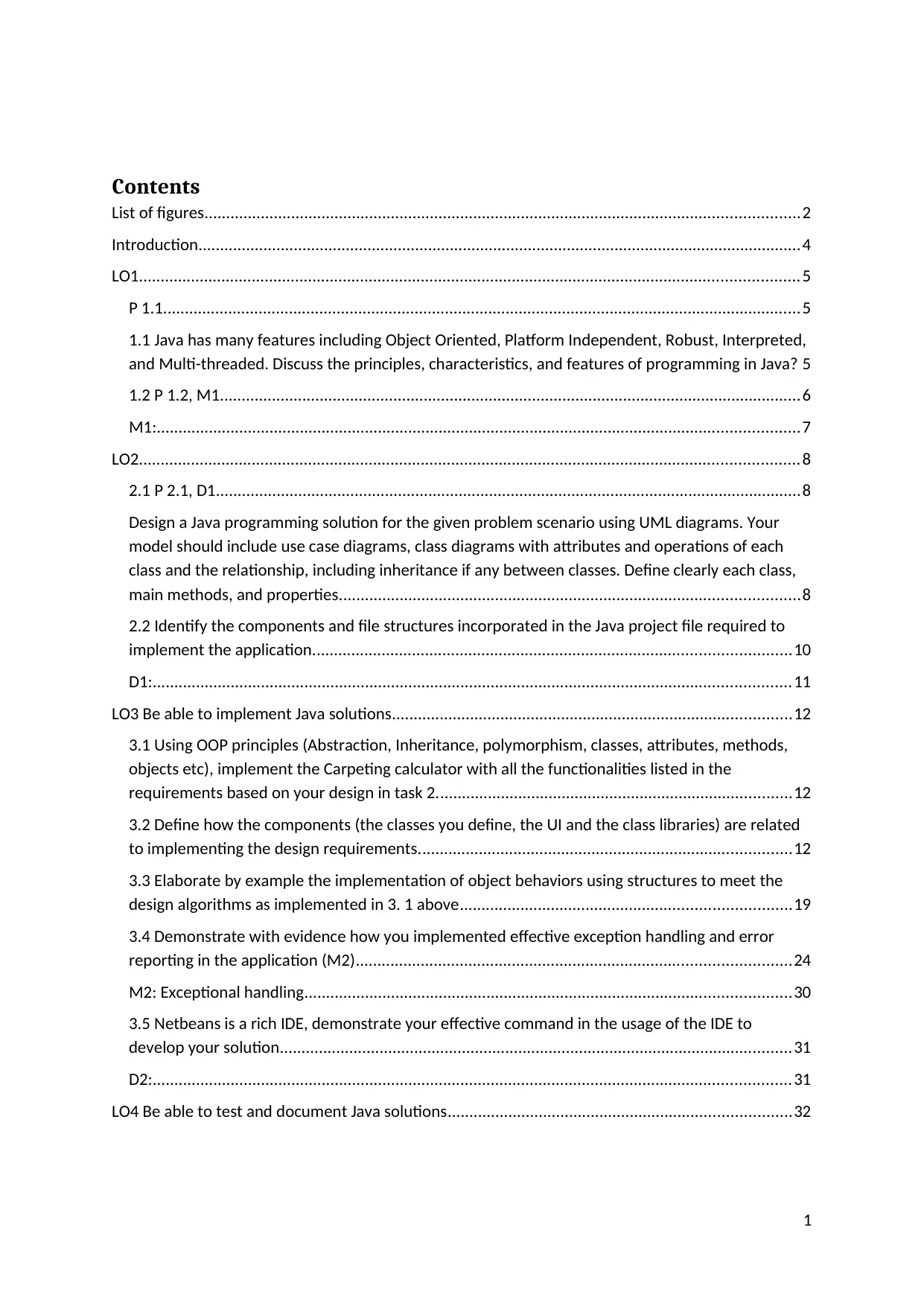
Contents
List of figures.........................................................................................................................................2
Introduction...........................................................................................................................................4
LO1........................................................................................................................................................5
P 1.1...................................................................................................................................................5
1.1 Java has many features including Object Oriented, Platform Independent, Robust, Interpreted,
and Multi-threaded. Discuss the principles, characteristics, and features of programming in Java? 5
1.2 P 1.2, M1......................................................................................................................................6
M1:....................................................................................................................................................7
LO2........................................................................................................................................................8
2.1 P 2.1, D1.......................................................................................................................................8
Design a Java programming solution for the given problem scenario using UML diagrams. Your
model should include use case diagrams, class diagrams with attributes and operations of each
class and the relationship, including inheritance if any between classes. Define clearly each class,
main methods, and properties..........................................................................................................8
2.2 Identify the components and file structures incorporated in the Java project file required to
implement the application..............................................................................................................10
D1:...................................................................................................................................................11
LO3 Be able to implement Java solutions............................................................................................12
3.1 Using OOP principles (Abstraction, Inheritance, polymorphism, classes, attributes, methods,
objects etc), implement the Carpeting calculator with all the functionalities listed in the
requirements based on your design in task 2..................................................................................12
3.2 Define how the components (the classes you define, the UI and the class libraries) are related
to implementing the design requirements......................................................................................12
3.3 Elaborate by example the implementation of object behaviors using structures to meet the
design algorithms as implemented in 3. 1 above............................................................................19
3.4 Demonstrate with evidence how you implemented effective exception handling and error
reporting in the application (M2)....................................................................................................24
M2: Exceptional handling................................................................................................................30
3.5 Netbeans is a rich IDE, demonstrate your effective command in the usage of the IDE to
develop your solution......................................................................................................................31
D2:...................................................................................................................................................31
LO4 Be able to test and document Java solutions...............................................................................32
1
List of figures.........................................................................................................................................2
Introduction...........................................................................................................................................4
LO1........................................................................................................................................................5
P 1.1...................................................................................................................................................5
1.1 Java has many features including Object Oriented, Platform Independent, Robust, Interpreted,
and Multi-threaded. Discuss the principles, characteristics, and features of programming in Java? 5
1.2 P 1.2, M1......................................................................................................................................6
M1:....................................................................................................................................................7
LO2........................................................................................................................................................8
2.1 P 2.1, D1.......................................................................................................................................8
Design a Java programming solution for the given problem scenario using UML diagrams. Your
model should include use case diagrams, class diagrams with attributes and operations of each
class and the relationship, including inheritance if any between classes. Define clearly each class,
main methods, and properties..........................................................................................................8
2.2 Identify the components and file structures incorporated in the Java project file required to
implement the application..............................................................................................................10
D1:...................................................................................................................................................11
LO3 Be able to implement Java solutions............................................................................................12
3.1 Using OOP principles (Abstraction, Inheritance, polymorphism, classes, attributes, methods,
objects etc), implement the Carpeting calculator with all the functionalities listed in the
requirements based on your design in task 2..................................................................................12
3.2 Define how the components (the classes you define, the UI and the class libraries) are related
to implementing the design requirements......................................................................................12
3.3 Elaborate by example the implementation of object behaviors using structures to meet the
design algorithms as implemented in 3. 1 above............................................................................19
3.4 Demonstrate with evidence how you implemented effective exception handling and error
reporting in the application (M2)....................................................................................................24
M2: Exceptional handling................................................................................................................30
3.5 Netbeans is a rich IDE, demonstrate your effective command in the usage of the IDE to
develop your solution......................................................................................................................31
D2:...................................................................................................................................................31
LO4 Be able to test and document Java solutions...............................................................................32
1
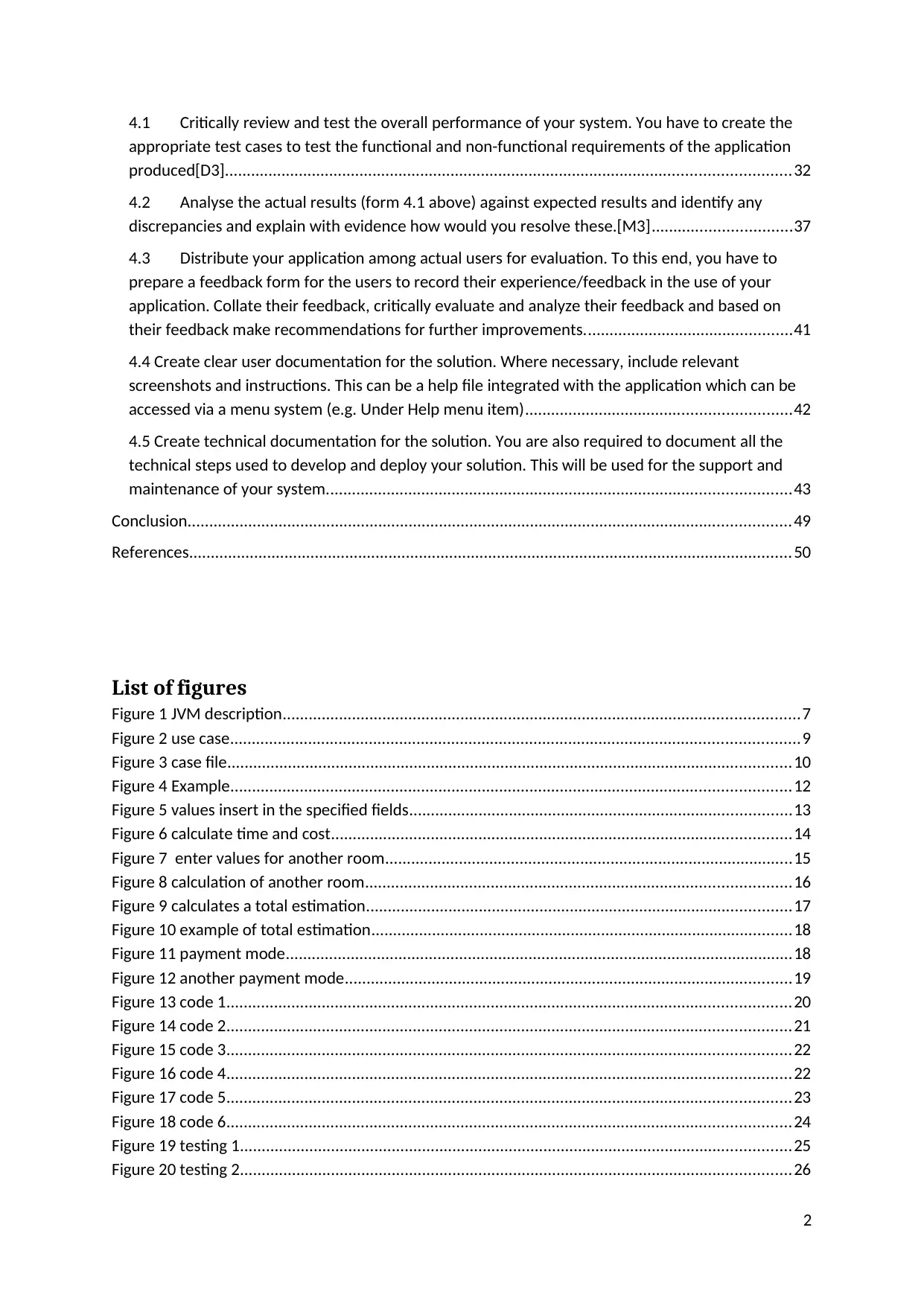
4.1 Critically review and test the overall performance of your system. You have to create the
appropriate test cases to test the functional and non-functional requirements of the application
produced[D3]..................................................................................................................................32
4.2 Analyse the actual results (form 4.1 above) against expected results and identify any
discrepancies and explain with evidence how would you resolve these.[M3]................................37
4.3 Distribute your application among actual users for evaluation. To this end, you have to
prepare a feedback form for the users to record their experience/feedback in the use of your
application. Collate their feedback, critically evaluate and analyze their feedback and based on
their feedback make recommendations for further improvements................................................41
4.4 Create clear user documentation for the solution. Where necessary, include relevant
screenshots and instructions. This can be a help file integrated with the application which can be
accessed via a menu system (e.g. Under Help menu item).............................................................42
4.5 Create technical documentation for the solution. You are also required to document all the
technical steps used to develop and deploy your solution. This will be used for the support and
maintenance of your system...........................................................................................................43
Conclusion...........................................................................................................................................49
References...........................................................................................................................................50
List of figures
Figure 1 JVM description.......................................................................................................................7
Figure 2 use case...................................................................................................................................9
Figure 3 case file..................................................................................................................................10
Figure 4 Example.................................................................................................................................12
Figure 5 values insert in the specified fields........................................................................................13
Figure 6 calculate time and cost..........................................................................................................14
Figure 7 enter values for another room..............................................................................................15
Figure 8 calculation of another room..................................................................................................16
Figure 9 calculates a total estimation..................................................................................................17
Figure 10 example of total estimation.................................................................................................18
Figure 11 payment mode.....................................................................................................................18
Figure 12 another payment mode.......................................................................................................19
Figure 13 code 1..................................................................................................................................20
Figure 14 code 2..................................................................................................................................21
Figure 15 code 3..................................................................................................................................22
Figure 16 code 4..................................................................................................................................22
Figure 17 code 5..................................................................................................................................23
Figure 18 code 6..................................................................................................................................24
Figure 19 testing 1...............................................................................................................................25
Figure 20 testing 2...............................................................................................................................26
2
appropriate test cases to test the functional and non-functional requirements of the application
produced[D3]..................................................................................................................................32
4.2 Analyse the actual results (form 4.1 above) against expected results and identify any
discrepancies and explain with evidence how would you resolve these.[M3]................................37
4.3 Distribute your application among actual users for evaluation. To this end, you have to
prepare a feedback form for the users to record their experience/feedback in the use of your
application. Collate their feedback, critically evaluate and analyze their feedback and based on
their feedback make recommendations for further improvements................................................41
4.4 Create clear user documentation for the solution. Where necessary, include relevant
screenshots and instructions. This can be a help file integrated with the application which can be
accessed via a menu system (e.g. Under Help menu item).............................................................42
4.5 Create technical documentation for the solution. You are also required to document all the
technical steps used to develop and deploy your solution. This will be used for the support and
maintenance of your system...........................................................................................................43
Conclusion...........................................................................................................................................49
References...........................................................................................................................................50
List of figures
Figure 1 JVM description.......................................................................................................................7
Figure 2 use case...................................................................................................................................9
Figure 3 case file..................................................................................................................................10
Figure 4 Example.................................................................................................................................12
Figure 5 values insert in the specified fields........................................................................................13
Figure 6 calculate time and cost..........................................................................................................14
Figure 7 enter values for another room..............................................................................................15
Figure 8 calculation of another room..................................................................................................16
Figure 9 calculates a total estimation..................................................................................................17
Figure 10 example of total estimation.................................................................................................18
Figure 11 payment mode.....................................................................................................................18
Figure 12 another payment mode.......................................................................................................19
Figure 13 code 1..................................................................................................................................20
Figure 14 code 2..................................................................................................................................21
Figure 15 code 3..................................................................................................................................22
Figure 16 code 4..................................................................................................................................22
Figure 17 code 5..................................................................................................................................23
Figure 18 code 6..................................................................................................................................24
Figure 19 testing 1...............................................................................................................................25
Figure 20 testing 2...............................................................................................................................26
2
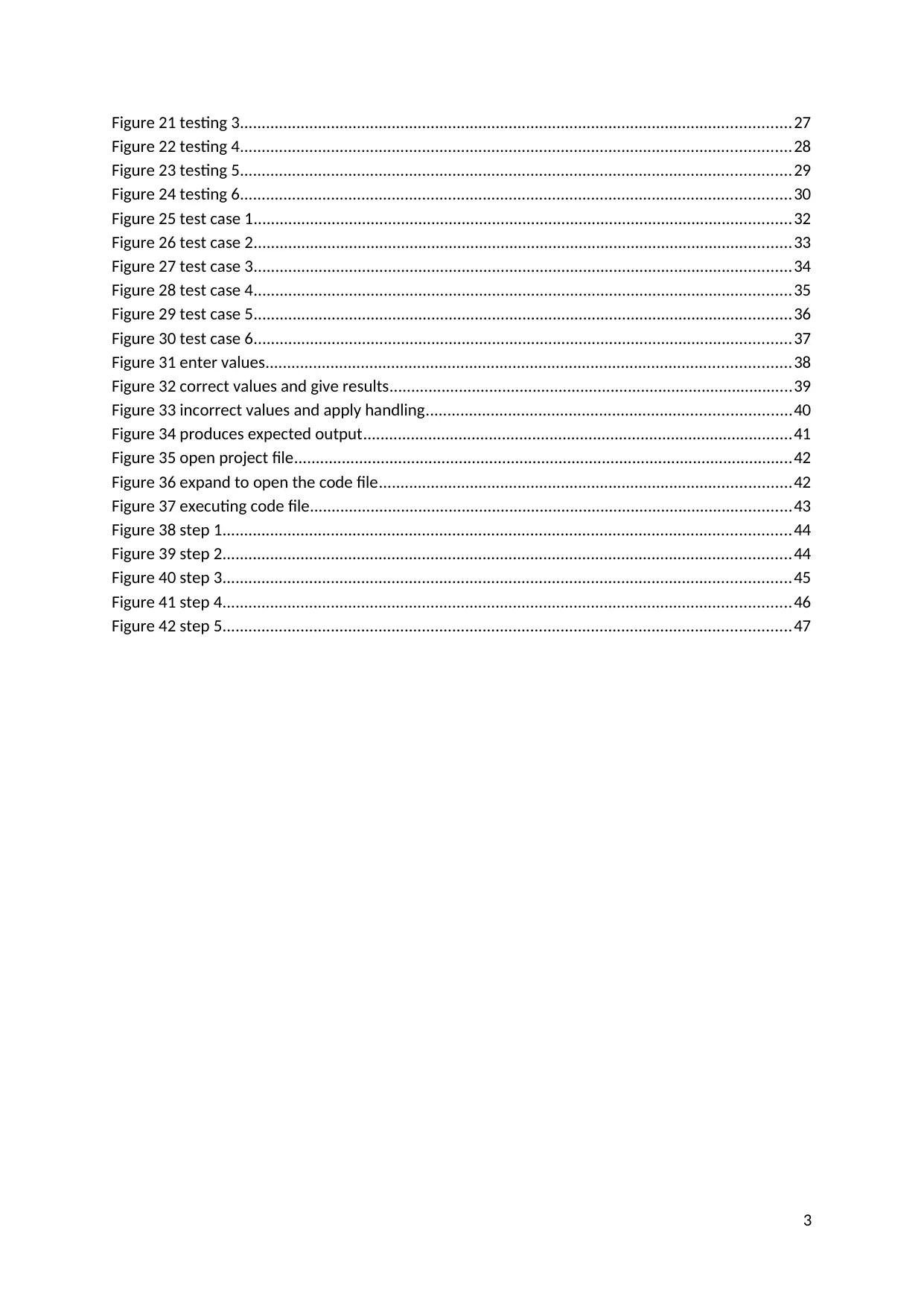
Figure 21 testing 3...............................................................................................................................27
Figure 22 testing 4...............................................................................................................................28
Figure 23 testing 5...............................................................................................................................29
Figure 24 testing 6...............................................................................................................................30
Figure 25 test case 1............................................................................................................................32
Figure 26 test case 2............................................................................................................................33
Figure 27 test case 3............................................................................................................................34
Figure 28 test case 4............................................................................................................................35
Figure 29 test case 5............................................................................................................................36
Figure 30 test case 6............................................................................................................................37
Figure 31 enter values.........................................................................................................................38
Figure 32 correct values and give results.............................................................................................39
Figure 33 incorrect values and apply handling....................................................................................40
Figure 34 produces expected output...................................................................................................41
Figure 35 open project file...................................................................................................................42
Figure 36 expand to open the code file...............................................................................................42
Figure 37 executing code file...............................................................................................................43
Figure 38 step 1...................................................................................................................................44
Figure 39 step 2...................................................................................................................................44
Figure 40 step 3...................................................................................................................................45
Figure 41 step 4...................................................................................................................................46
Figure 42 step 5...................................................................................................................................47
3
Figure 22 testing 4...............................................................................................................................28
Figure 23 testing 5...............................................................................................................................29
Figure 24 testing 6...............................................................................................................................30
Figure 25 test case 1............................................................................................................................32
Figure 26 test case 2............................................................................................................................33
Figure 27 test case 3............................................................................................................................34
Figure 28 test case 4............................................................................................................................35
Figure 29 test case 5............................................................................................................................36
Figure 30 test case 6............................................................................................................................37
Figure 31 enter values.........................................................................................................................38
Figure 32 correct values and give results.............................................................................................39
Figure 33 incorrect values and apply handling....................................................................................40
Figure 34 produces expected output...................................................................................................41
Figure 35 open project file...................................................................................................................42
Figure 36 expand to open the code file...............................................................................................42
Figure 37 executing code file...............................................................................................................43
Figure 38 step 1...................................................................................................................................44
Figure 39 step 2...................................................................................................................................44
Figure 40 step 3...................................................................................................................................45
Figure 41 step 4...................................................................................................................................46
Figure 42 step 5...................................................................................................................................47
3
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
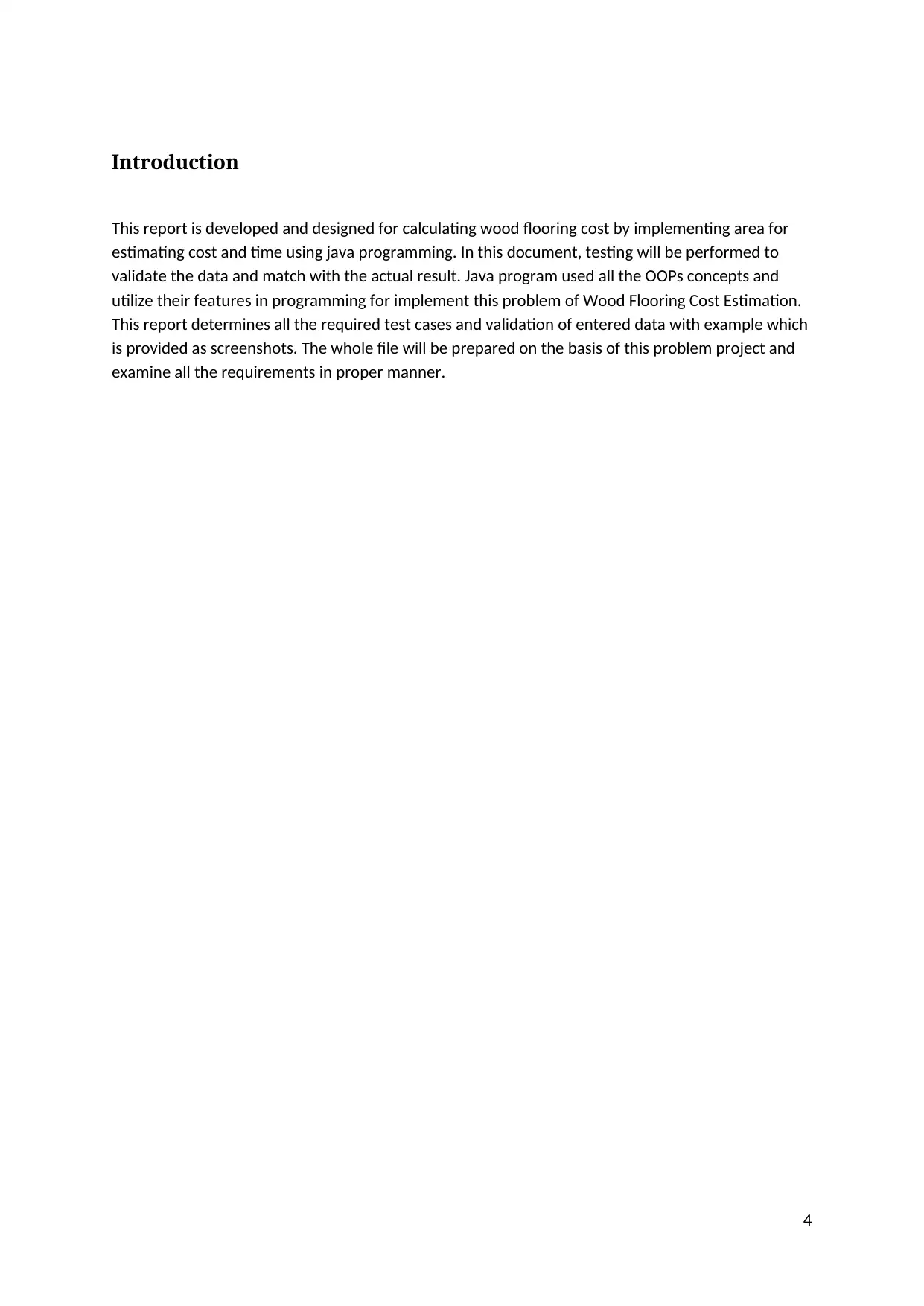
Introduction
This report is developed and designed for calculating wood flooring cost by implementing area for
estimating cost and time using java programming. In this document, testing will be performed to
validate the data and match with the actual result. Java program used all the OOPs concepts and
utilize their features in programming for implement this problem of Wood Flooring Cost Estimation.
This report determines all the required test cases and validation of entered data with example which
is provided as screenshots. The whole file will be prepared on the basis of this problem project and
examine all the requirements in proper manner.
4
This report is developed and designed for calculating wood flooring cost by implementing area for
estimating cost and time using java programming. In this document, testing will be performed to
validate the data and match with the actual result. Java program used all the OOPs concepts and
utilize their features in programming for implement this problem of Wood Flooring Cost Estimation.
This report determines all the required test cases and validation of entered data with example which
is provided as screenshots. The whole file will be prepared on the basis of this problem project and
examine all the requirements in proper manner.
4
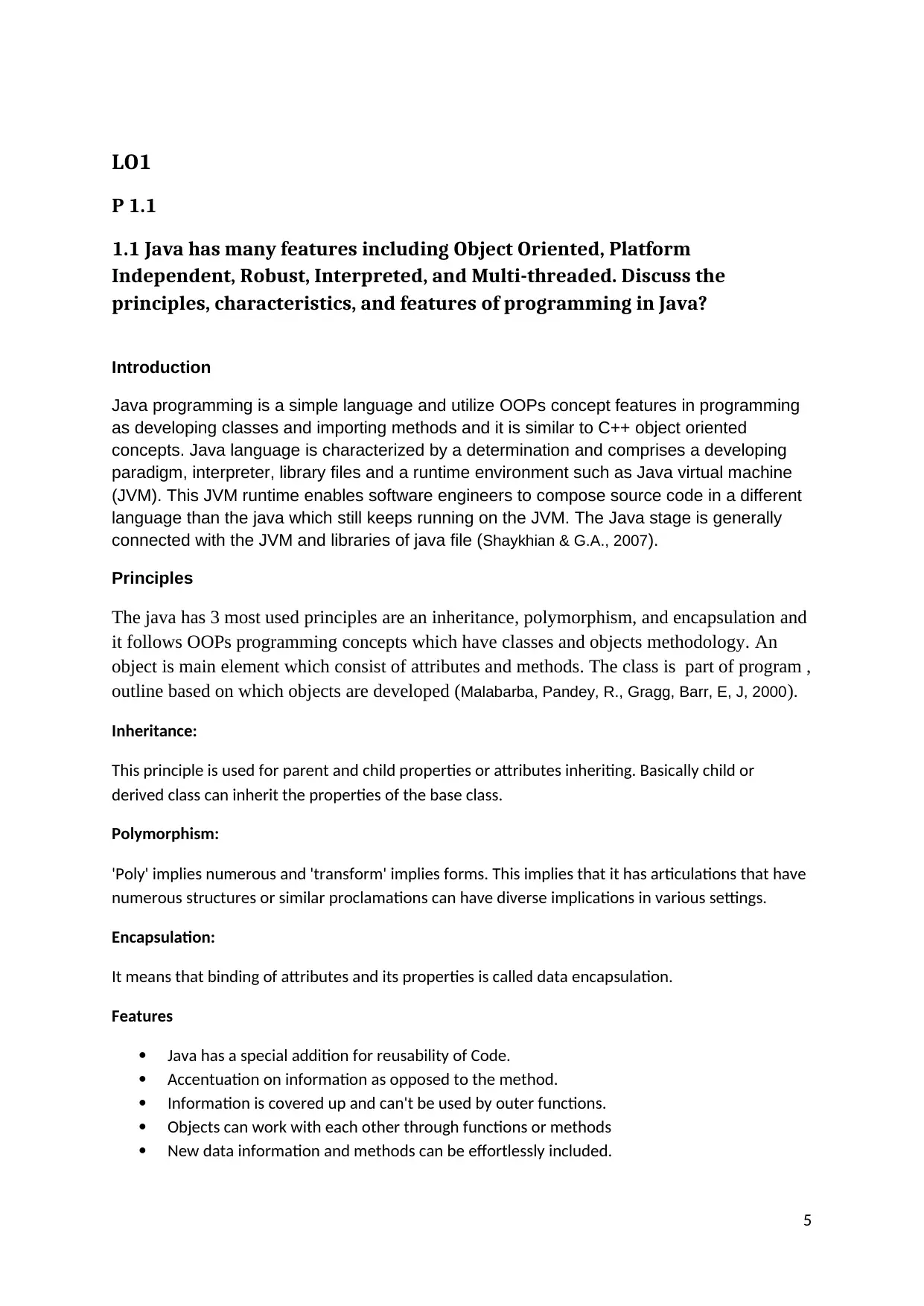
LO1
P 1.1
1.1 Java has many features including Object Oriented, Platform
Independent, Robust, Interpreted, and Multi-threaded. Discuss the
principles, characteristics, and features of programming in Java?
Introduction
Java programming is a simple language and utilize OOPs concept features in programming
as developing classes and importing methods and it is similar to C++ object oriented
concepts. Java language is characterized by a determination and comprises a developing
paradigm, interpreter, library files and a runtime environment such as Java virtual machine
(JVM). This JVM runtime enables software engineers to compose source code in a different
language than the java which still keeps running on the JVM. The Java stage is generally
connected with the JVM and libraries of java file (Shaykhian & G.A., 2007).
Principles
The java has 3 most used principles are an inheritance, polymorphism, and encapsulation and
it follows OOPs programming concepts which have classes and objects methodology. An
object is main element which consist of attributes and methods. The class is part of program ,
outline based on which objects are developed (Malabarba, Pandey, R., Gragg, Barr, E, J, 2000).
Inheritance:
This principle is used for parent and child properties or attributes inheriting. Basically child or
derived class can inherit the properties of the base class.
Polymorphism:
'Poly' implies numerous and 'transform' implies forms. This implies that it has articulations that have
numerous structures or similar proclamations can have diverse implications in various settings.
Encapsulation:
It means that binding of attributes and its properties is called data encapsulation.
Features
Java has a special addition for reusability of Code.
Accentuation on information as opposed to the method.
Information is covered up and can't be used by outer functions.
Objects can work with each other through functions or methods
New data information and methods can be effortlessly included.
5
P 1.1
1.1 Java has many features including Object Oriented, Platform
Independent, Robust, Interpreted, and Multi-threaded. Discuss the
principles, characteristics, and features of programming in Java?
Introduction
Java programming is a simple language and utilize OOPs concept features in programming
as developing classes and importing methods and it is similar to C++ object oriented
concepts. Java language is characterized by a determination and comprises a developing
paradigm, interpreter, library files and a runtime environment such as Java virtual machine
(JVM). This JVM runtime enables software engineers to compose source code in a different
language than the java which still keeps running on the JVM. The Java stage is generally
connected with the JVM and libraries of java file (Shaykhian & G.A., 2007).
Principles
The java has 3 most used principles are an inheritance, polymorphism, and encapsulation and
it follows OOPs programming concepts which have classes and objects methodology. An
object is main element which consist of attributes and methods. The class is part of program ,
outline based on which objects are developed (Malabarba, Pandey, R., Gragg, Barr, E, J, 2000).
Inheritance:
This principle is used for parent and child properties or attributes inheriting. Basically child or
derived class can inherit the properties of the base class.
Polymorphism:
'Poly' implies numerous and 'transform' implies forms. This implies that it has articulations that have
numerous structures or similar proclamations can have diverse implications in various settings.
Encapsulation:
It means that binding of attributes and its properties is called data encapsulation.
Features
Java has a special addition for reusability of Code.
Accentuation on information as opposed to the method.
Information is covered up and can't be used by outer functions.
Objects can work with each other through functions or methods
New data information and methods can be effortlessly included.
5
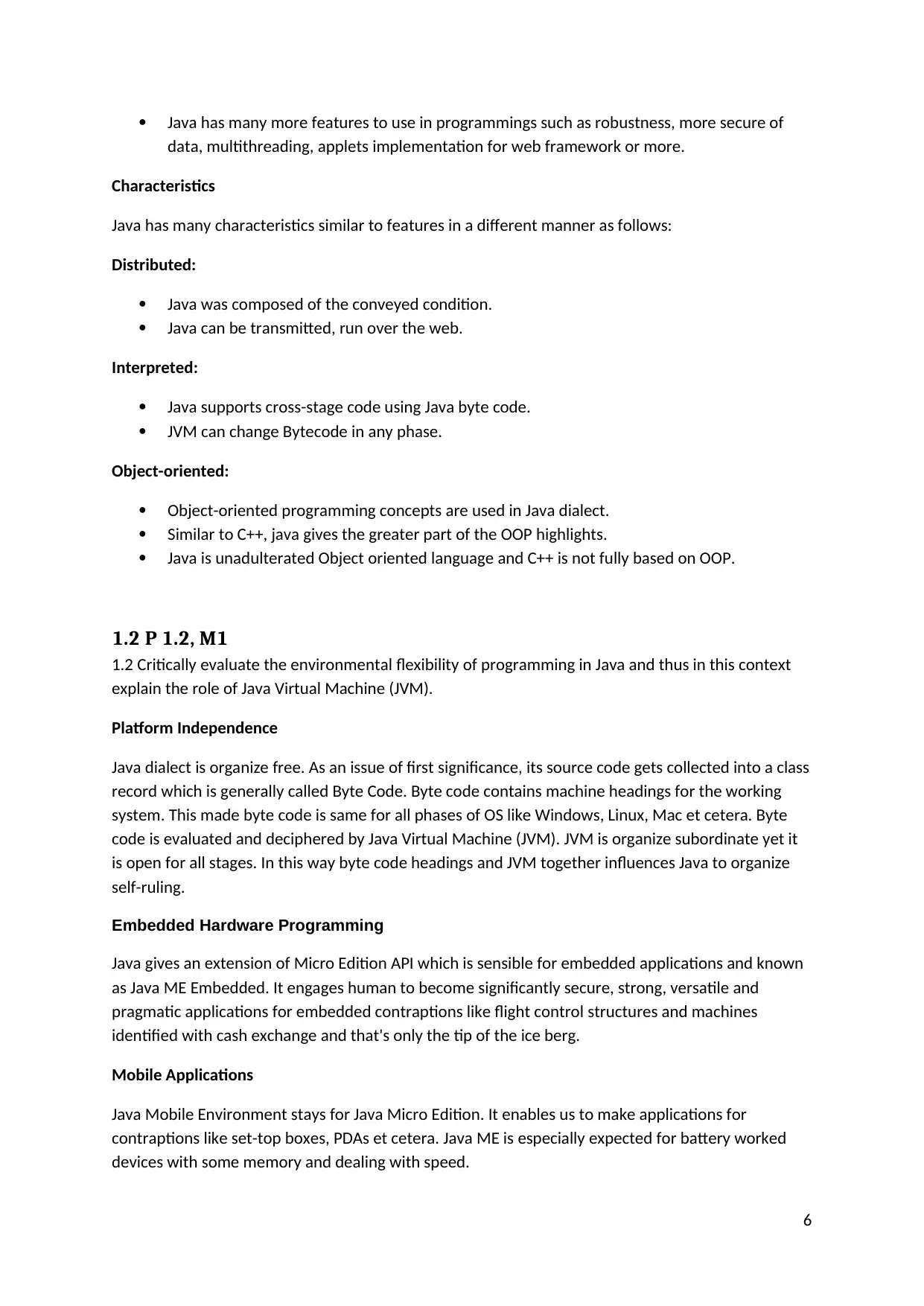
Java has many more features to use in programmings such as robustness, more secure of
data, multithreading, applets implementation for web framework or more.
Characteristics
Java has many characteristics similar to features in a different manner as follows:
Distributed:
Java was composed of the conveyed condition.
Java can be transmitted, run over the web.
Interpreted:
Java supports cross-stage code using Java byte code.
JVM can change Bytecode in any phase.
Object-oriented:
Object-oriented programming concepts are used in Java dialect.
Similar to C++, java gives the greater part of the OOP highlights.
Java is unadulterated Object oriented language and C++ is not fully based on OOP.
1.2 P 1.2, M1
1.2 Critically evaluate the environmental flexibility of programming in Java and thus in this context
explain the role of Java Virtual Machine (JVM).
Platform Independence
Java dialect is organize free. As an issue of first significance, its source code gets collected into a class
record which is generally called Byte Code. Byte code contains machine headings for the working
system. This made byte code is same for all phases of OS like Windows, Linux, Mac et cetera. Byte
code is evaluated and deciphered by Java Virtual Machine (JVM). JVM is organize subordinate yet it
is open for all stages. In this way byte code headings and JVM together influences Java to organize
self-ruling.
Embedded Hardware Programming
Java gives an extension of Micro Edition API which is sensible for embedded applications and known
as Java ME Embedded. It engages human to become significantly secure, strong, versatile and
pragmatic applications for embedded contraptions like flight control structures and machines
identified with cash exchange and that's only the tip of the ice berg.
Mobile Applications
Java Mobile Environment stays for Java Micro Edition. It enables us to make applications for
contraptions like set-top boxes, PDAs et cetera. Java ME is especially expected for battery worked
devices with some memory and dealing with speed.
6
data, multithreading, applets implementation for web framework or more.
Characteristics
Java has many characteristics similar to features in a different manner as follows:
Distributed:
Java was composed of the conveyed condition.
Java can be transmitted, run over the web.
Interpreted:
Java supports cross-stage code using Java byte code.
JVM can change Bytecode in any phase.
Object-oriented:
Object-oriented programming concepts are used in Java dialect.
Similar to C++, java gives the greater part of the OOP highlights.
Java is unadulterated Object oriented language and C++ is not fully based on OOP.
1.2 P 1.2, M1
1.2 Critically evaluate the environmental flexibility of programming in Java and thus in this context
explain the role of Java Virtual Machine (JVM).
Platform Independence
Java dialect is organize free. As an issue of first significance, its source code gets collected into a class
record which is generally called Byte Code. Byte code contains machine headings for the working
system. This made byte code is same for all phases of OS like Windows, Linux, Mac et cetera. Byte
code is evaluated and deciphered by Java Virtual Machine (JVM). JVM is organize subordinate yet it
is open for all stages. In this way byte code headings and JVM together influences Java to organize
self-ruling.
Embedded Hardware Programming
Java gives an extension of Micro Edition API which is sensible for embedded applications and known
as Java ME Embedded. It engages human to become significantly secure, strong, versatile and
pragmatic applications for embedded contraptions like flight control structures and machines
identified with cash exchange and that's only the tip of the ice berg.
Mobile Applications
Java Mobile Environment stays for Java Micro Edition. It enables us to make applications for
contraptions like set-top boxes, PDAs et cetera. Java ME is especially expected for battery worked
devices with some memory and dealing with speed.
6
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
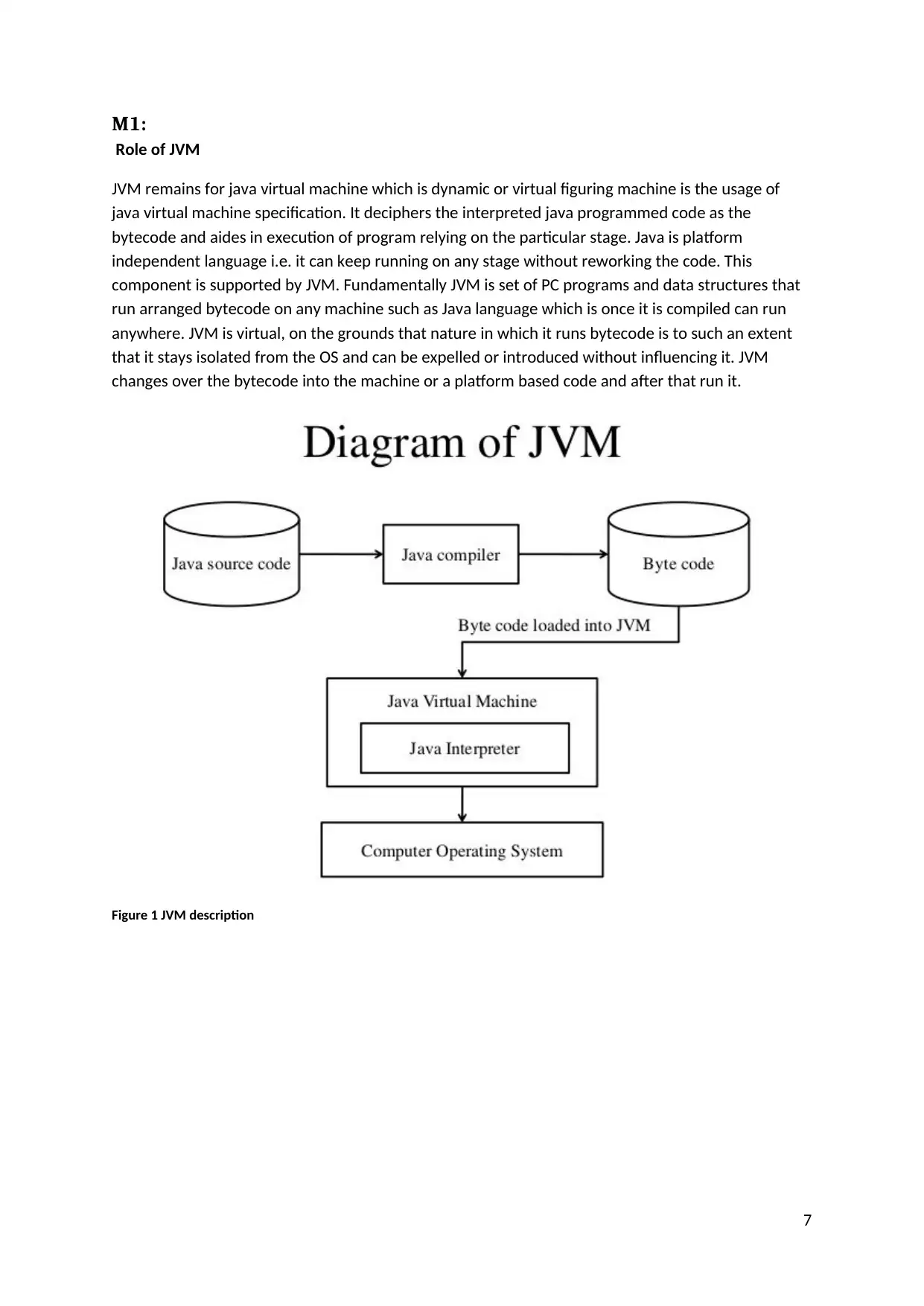
M1:
Role of JVM
JVM remains for java virtual machine which is dynamic or virtual figuring machine is the usage of
java virtual machine specification. It deciphers the interpreted java programmed code as the
bytecode and aides in execution of program relying on the particular stage. Java is platform
independent language i.e. it can keep running on any stage without reworking the code. This
component is supported by JVM. Fundamentally JVM is set of PC programs and data structures that
run arranged bytecode on any machine such as Java language which is once it is compiled can run
anywhere. JVM is virtual, on the grounds that nature in which it runs bytecode is to such an extent
that it stays isolated from the OS and can be expelled or introduced without influencing it. JVM
changes over the bytecode into the machine or a platform based code and after that run it.
Figure 1 JVM description
7
Role of JVM
JVM remains for java virtual machine which is dynamic or virtual figuring machine is the usage of
java virtual machine specification. It deciphers the interpreted java programmed code as the
bytecode and aides in execution of program relying on the particular stage. Java is platform
independent language i.e. it can keep running on any stage without reworking the code. This
component is supported by JVM. Fundamentally JVM is set of PC programs and data structures that
run arranged bytecode on any machine such as Java language which is once it is compiled can run
anywhere. JVM is virtual, on the grounds that nature in which it runs bytecode is to such an extent
that it stays isolated from the OS and can be expelled or introduced without influencing it. JVM
changes over the bytecode into the machine or a platform based code and after that run it.
Figure 1 JVM description
7
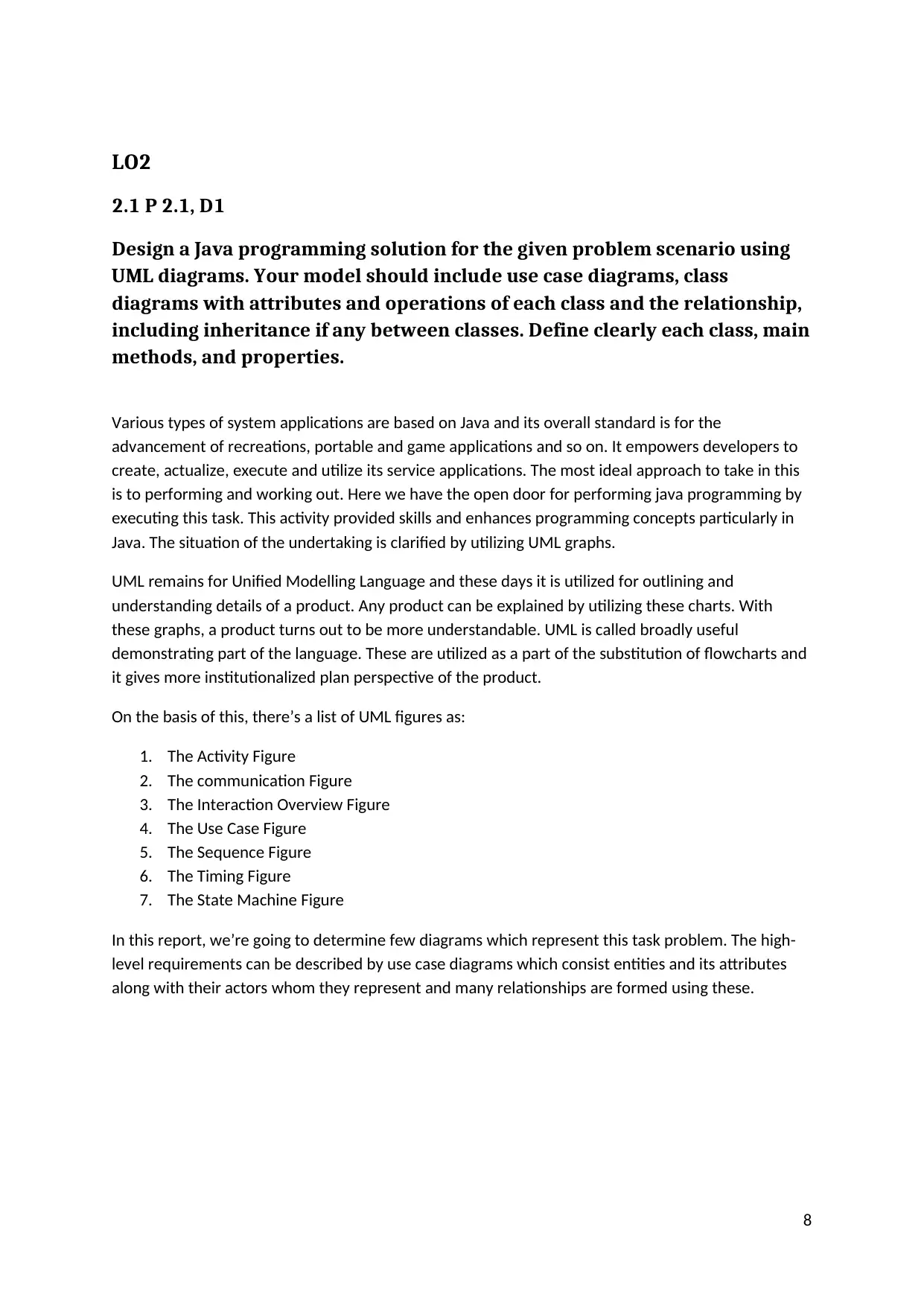
LO2
2.1 P 2.1, D1
Design a Java programming solution for the given problem scenario using
UML diagrams. Your model should include use case diagrams, class
diagrams with attributes and operations of each class and the relationship,
including inheritance if any between classes. Define clearly each class, main
methods, and properties.
Various types of system applications are based on Java and its overall standard is for the
advancement of recreations, portable and game applications and so on. It empowers developers to
create, actualize, execute and utilize its service applications. The most ideal approach to take in this
is to performing and working out. Here we have the open door for performing java programming by
executing this task. This activity provided skills and enhances programming concepts particularly in
Java. The situation of the undertaking is clarified by utilizing UML graphs.
UML remains for Unified Modelling Language and these days it is utilized for outlining and
understanding details of a product. Any product can be explained by utilizing these charts. With
these graphs, a product turns out to be more understandable. UML is called broadly useful
demonstrating part of the language. These are utilized as a part of the substitution of flowcharts and
it gives more institutionalized plan perspective of the product.
On the basis of this, there’s a list of UML figures as:
1. The Activity Figure
2. The communication Figure
3. The Interaction Overview Figure
4. The Use Case Figure
5. The Sequence Figure
6. The Timing Figure
7. The State Machine Figure
In this report, we’re going to determine few diagrams which represent this task problem. The high-
level requirements can be described by use case diagrams which consist entities and its attributes
along with their actors whom they represent and many relationships are formed using these.
8
2.1 P 2.1, D1
Design a Java programming solution for the given problem scenario using
UML diagrams. Your model should include use case diagrams, class
diagrams with attributes and operations of each class and the relationship,
including inheritance if any between classes. Define clearly each class, main
methods, and properties.
Various types of system applications are based on Java and its overall standard is for the
advancement of recreations, portable and game applications and so on. It empowers developers to
create, actualize, execute and utilize its service applications. The most ideal approach to take in this
is to performing and working out. Here we have the open door for performing java programming by
executing this task. This activity provided skills and enhances programming concepts particularly in
Java. The situation of the undertaking is clarified by utilizing UML graphs.
UML remains for Unified Modelling Language and these days it is utilized for outlining and
understanding details of a product. Any product can be explained by utilizing these charts. With
these graphs, a product turns out to be more understandable. UML is called broadly useful
demonstrating part of the language. These are utilized as a part of the substitution of flowcharts and
it gives more institutionalized plan perspective of the product.
On the basis of this, there’s a list of UML figures as:
1. The Activity Figure
2. The communication Figure
3. The Interaction Overview Figure
4. The Use Case Figure
5. The Sequence Figure
6. The Timing Figure
7. The State Machine Figure
In this report, we’re going to determine few diagrams which represent this task problem. The high-
level requirements can be described by use case diagrams which consist entities and its attributes
along with their actors whom they represent and many relationships are formed using these.
8
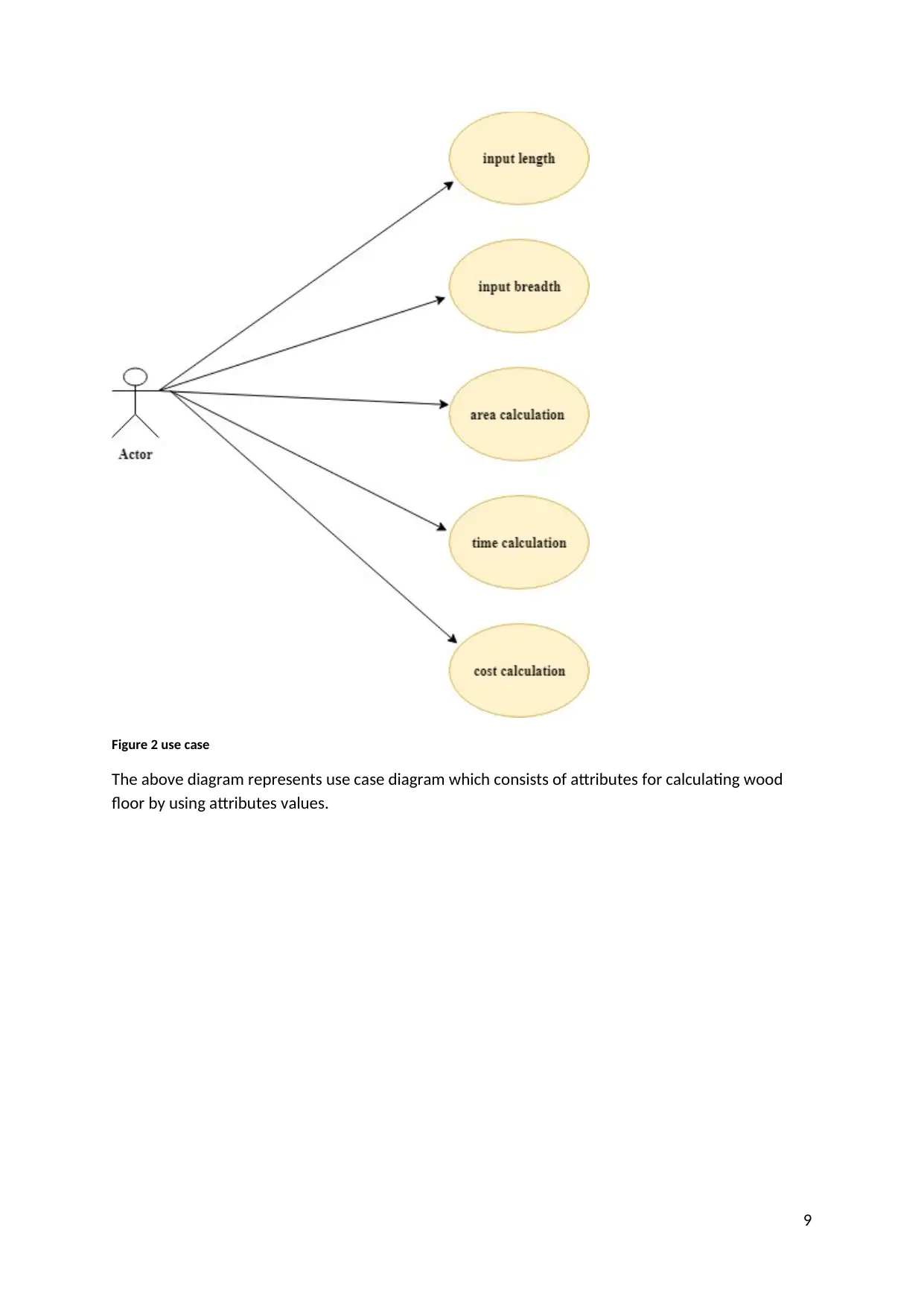
Figure 2 use case
The above diagram represents use case diagram which consists of attributes for calculating wood
floor by using attributes values.
9
The above diagram represents use case diagram which consists of attributes for calculating wood
floor by using attributes values.
9
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
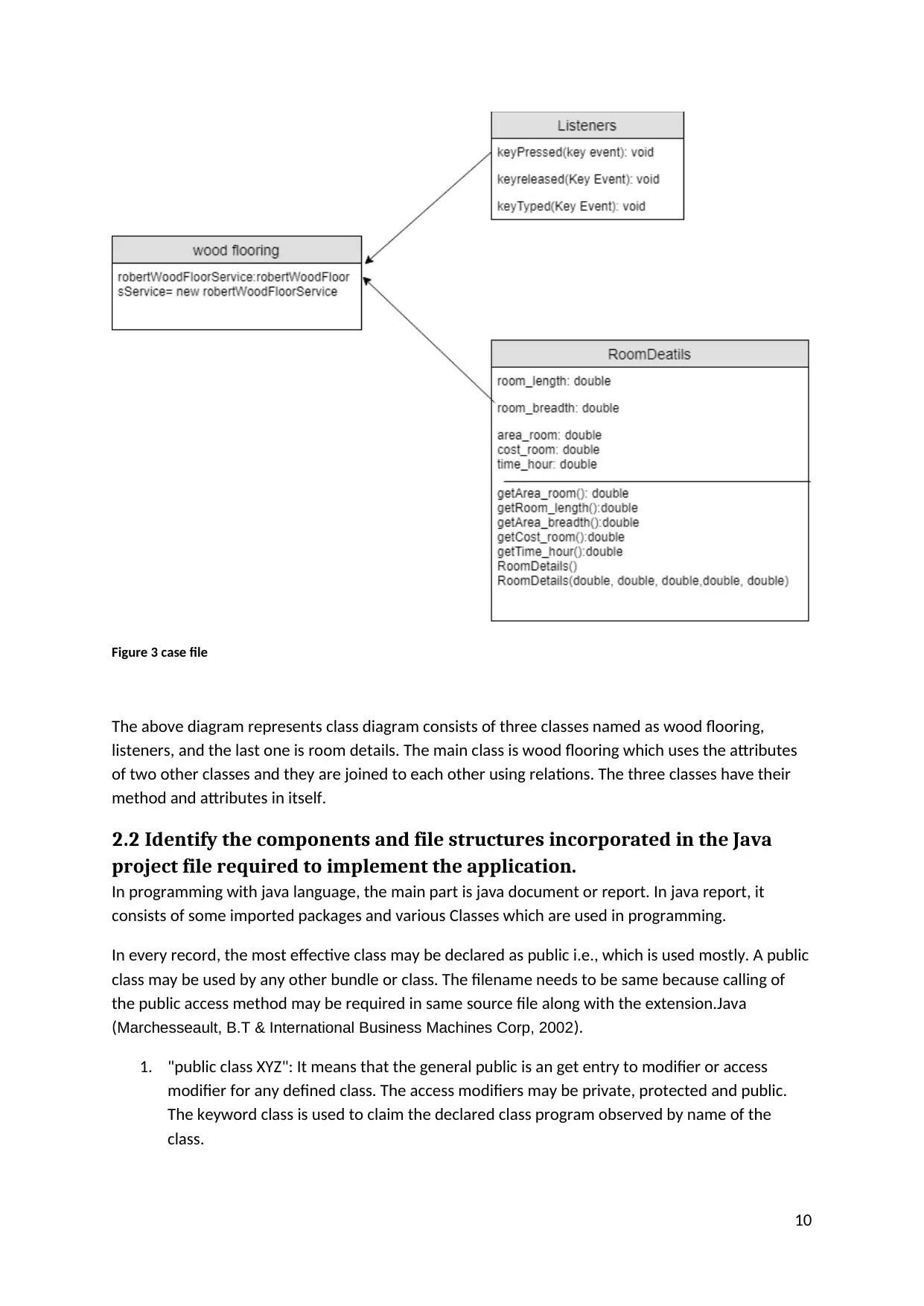
Figure 3 case file
The above diagram represents class diagram consists of three classes named as wood flooring,
listeners, and the last one is room details. The main class is wood flooring which uses the attributes
of two other classes and they are joined to each other using relations. The three classes have their
method and attributes in itself.
2.2 Identify the components and file structures incorporated in the Java
project file required to implement the application.
In programming with java language, the main part is java document or report. In java report, it
consists of some imported packages and various Classes which are used in programming.
In every record, the most effective class may be declared as public i.e., which is used mostly. A public
class may be used by any other bundle or class. The filename needs to be same because calling of
the public access method may be required in same source file along with the extension.Java
(Marchesseault, B.T & International Business Machines Corp, 2002).
1. "public class XYZ": It means that the general public is an get entry to modifier or access
modifier for any defined class. The access modifiers may be private, protected and public.
The keyword class is used to claim the declared class program observed by name of the
class.
10
The above diagram represents class diagram consists of three classes named as wood flooring,
listeners, and the last one is room details. The main class is wood flooring which uses the attributes
of two other classes and they are joined to each other using relations. The three classes have their
method and attributes in itself.
2.2 Identify the components and file structures incorporated in the Java
project file required to implement the application.
In programming with java language, the main part is java document or report. In java report, it
consists of some imported packages and various Classes which are used in programming.
In every record, the most effective class may be declared as public i.e., which is used mostly. A public
class may be used by any other bundle or class. The filename needs to be same because calling of
the public access method may be required in same source file along with the extension.Java
(Marchesseault, B.T & International Business Machines Corp, 2002).
1. "public class XYZ": It means that the general public is an get entry to modifier or access
modifier for any defined class. The access modifiers may be private, protected and public.
The keyword class is used to claim the declared class program observed by name of the
class.
10
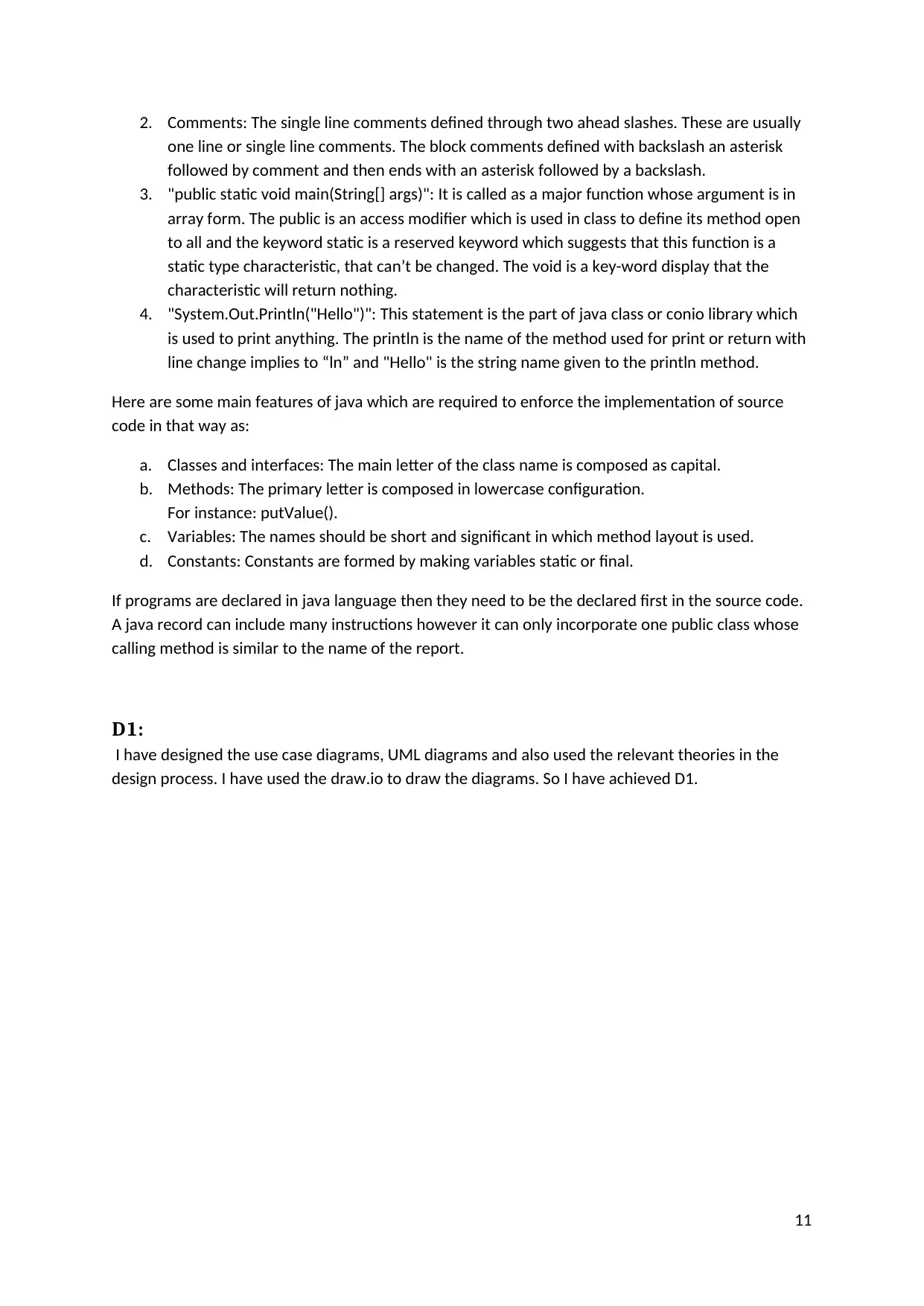
2. Comments: The single line comments defined through two ahead slashes. These are usually
one line or single line comments. The block comments defined with backslash an asterisk
followed by comment and then ends with an asterisk followed by a backslash.
3. "public static void main(String[] args)": It is called as a major function whose argument is in
array form. The public is an access modifier which is used in class to define its method open
to all and the keyword static is a reserved keyword which suggests that this function is a
static type characteristic, that can’t be changed. The void is a key-word display that the
characteristic will return nothing.
4. "System.Out.Println("Hello")": This statement is the part of java class or conio library which
is used to print anything. The println is the name of the method used for print or return with
line change implies to “ln” and "Hello" is the string name given to the println method.
Here are some main features of java which are required to enforce the implementation of source
code in that way as:
a. Classes and interfaces: The main letter of the class name is composed as capital.
b. Methods: The primary letter is composed in lowercase configuration.
For instance: putValue().
c. Variables: The names should be short and significant in which method layout is used.
d. Constants: Constants are formed by making variables static or final.
If programs are declared in java language then they need to be the declared first in the source code.
A java record can include many instructions however it can only incorporate one public class whose
calling method is similar to the name of the report.
D1:
I have designed the use case diagrams, UML diagrams and also used the relevant theories in the
design process. I have used the draw.io to draw the diagrams. So I have achieved D1.
11
one line or single line comments. The block comments defined with backslash an asterisk
followed by comment and then ends with an asterisk followed by a backslash.
3. "public static void main(String[] args)": It is called as a major function whose argument is in
array form. The public is an access modifier which is used in class to define its method open
to all and the keyword static is a reserved keyword which suggests that this function is a
static type characteristic, that can’t be changed. The void is a key-word display that the
characteristic will return nothing.
4. "System.Out.Println("Hello")": This statement is the part of java class or conio library which
is used to print anything. The println is the name of the method used for print or return with
line change implies to “ln” and "Hello" is the string name given to the println method.
Here are some main features of java which are required to enforce the implementation of source
code in that way as:
a. Classes and interfaces: The main letter of the class name is composed as capital.
b. Methods: The primary letter is composed in lowercase configuration.
For instance: putValue().
c. Variables: The names should be short and significant in which method layout is used.
d. Constants: Constants are formed by making variables static or final.
If programs are declared in java language then they need to be the declared first in the source code.
A java record can include many instructions however it can only incorporate one public class whose
calling method is similar to the name of the report.
D1:
I have designed the use case diagrams, UML diagrams and also used the relevant theories in the
design process. I have used the draw.io to draw the diagrams. So I have achieved D1.
11
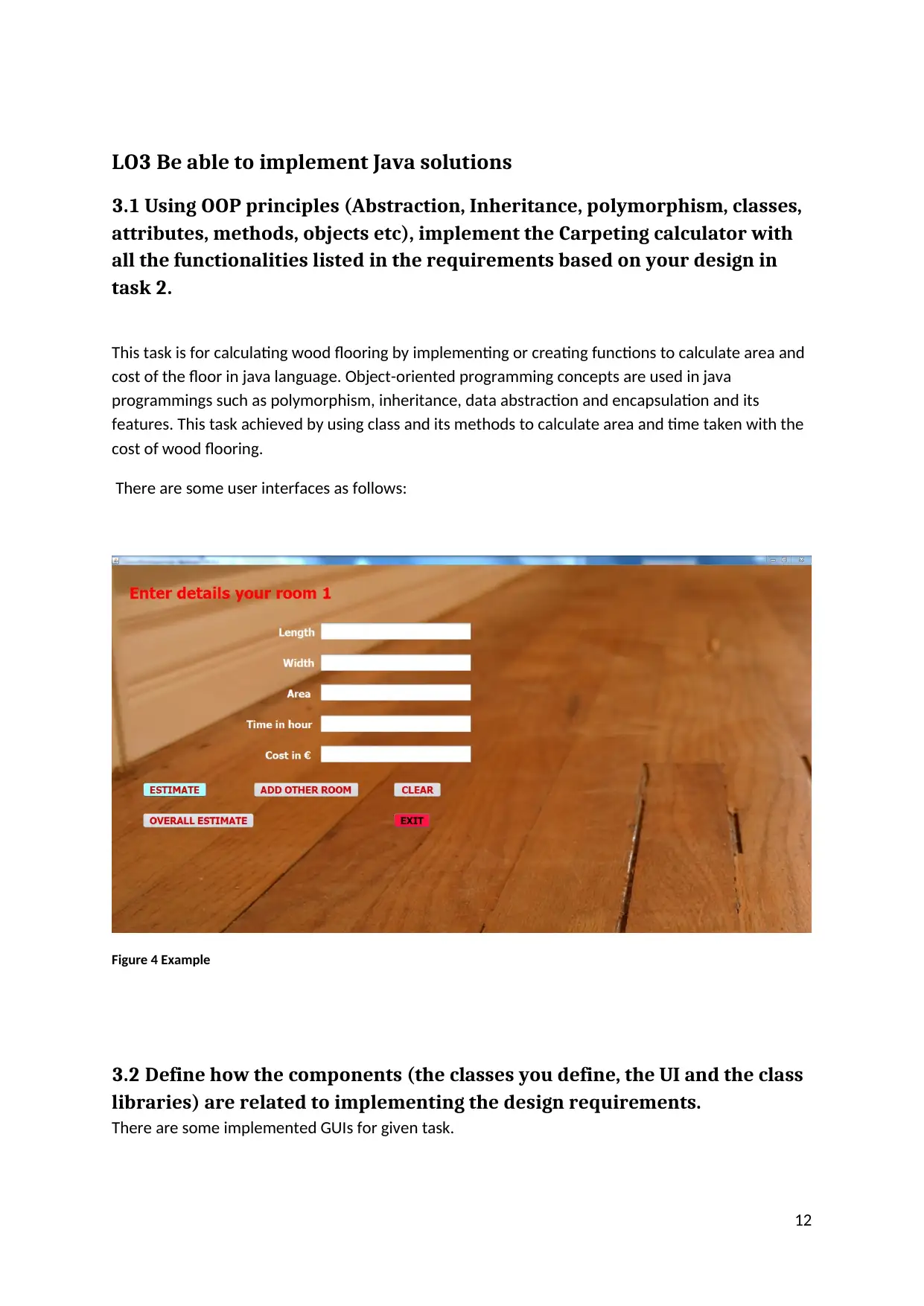
LO3 Be able to implement Java solutions
3.1 Using OOP principles (Abstraction, Inheritance, polymorphism, classes,
attributes, methods, objects etc), implement the Carpeting calculator with
all the functionalities listed in the requirements based on your design in
task 2.
This task is for calculating wood flooring by implementing or creating functions to calculate area and
cost of the floor in java language. Object-oriented programming concepts are used in java
programmings such as polymorphism, inheritance, data abstraction and encapsulation and its
features. This task achieved by using class and its methods to calculate area and time taken with the
cost of wood flooring.
There are some user interfaces as follows:
Figure 4 Example
3.2 Define how the components (the classes you define, the UI and the class
libraries) are related to implementing the design requirements.
There are some implemented GUIs for given task.
12
3.1 Using OOP principles (Abstraction, Inheritance, polymorphism, classes,
attributes, methods, objects etc), implement the Carpeting calculator with
all the functionalities listed in the requirements based on your design in
task 2.
This task is for calculating wood flooring by implementing or creating functions to calculate area and
cost of the floor in java language. Object-oriented programming concepts are used in java
programmings such as polymorphism, inheritance, data abstraction and encapsulation and its
features. This task achieved by using class and its methods to calculate area and time taken with the
cost of wood flooring.
There are some user interfaces as follows:
Figure 4 Example
3.2 Define how the components (the classes you define, the UI and the class
libraries) are related to implementing the design requirements.
There are some implemented GUIs for given task.
12
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
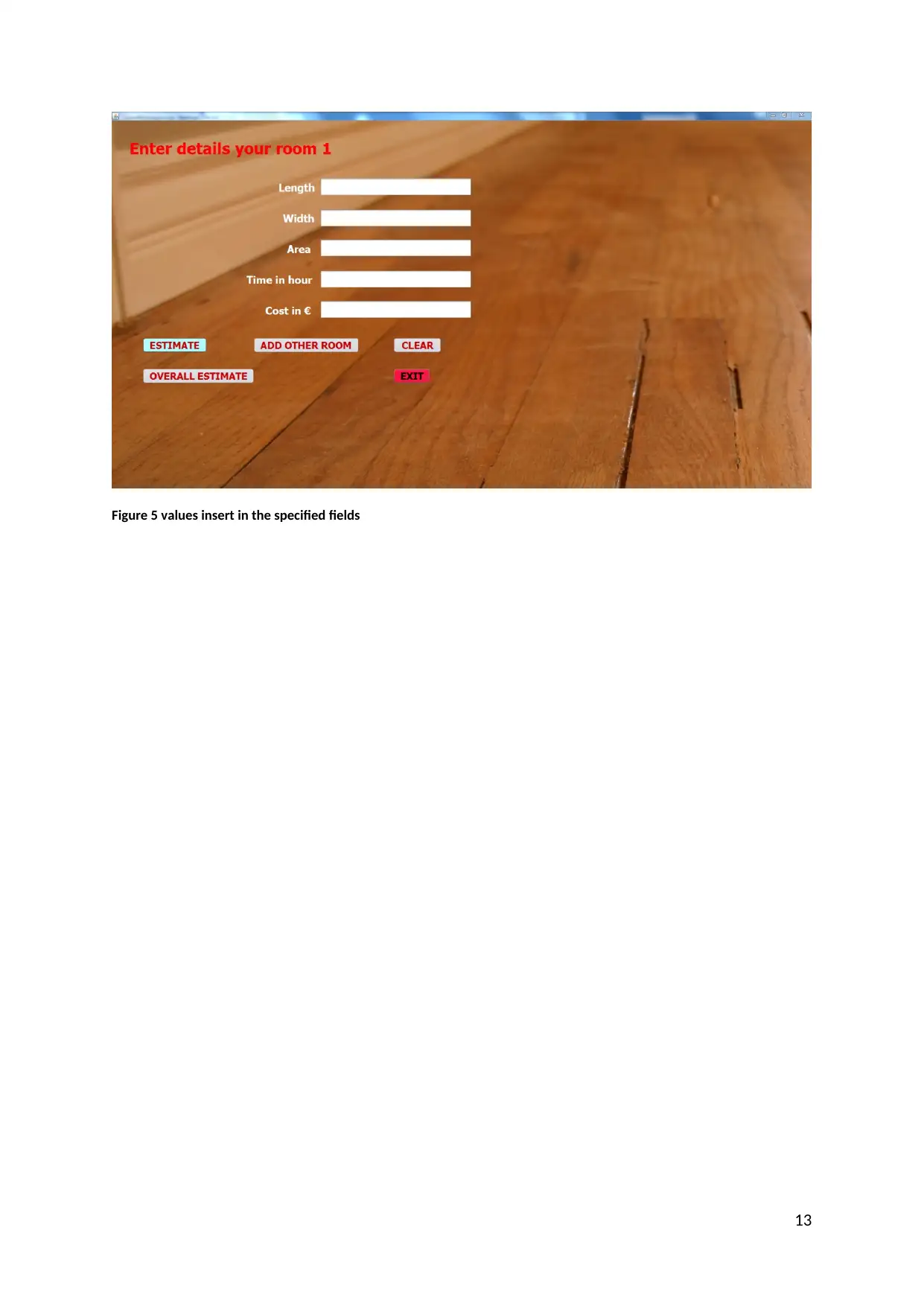
Figure 5 values insert in the specified fields
13
13
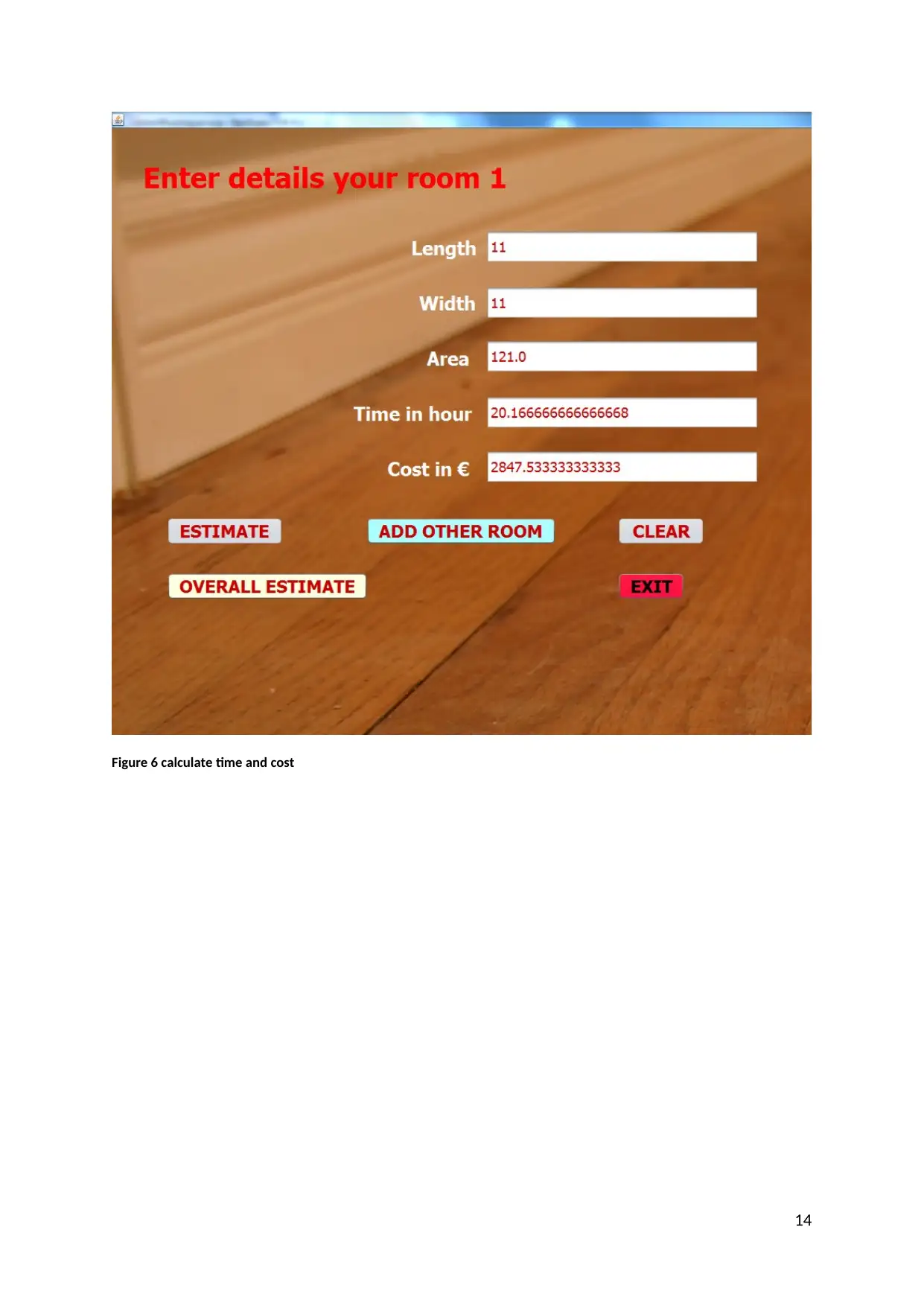
Figure 6 calculate time and cost
14
14
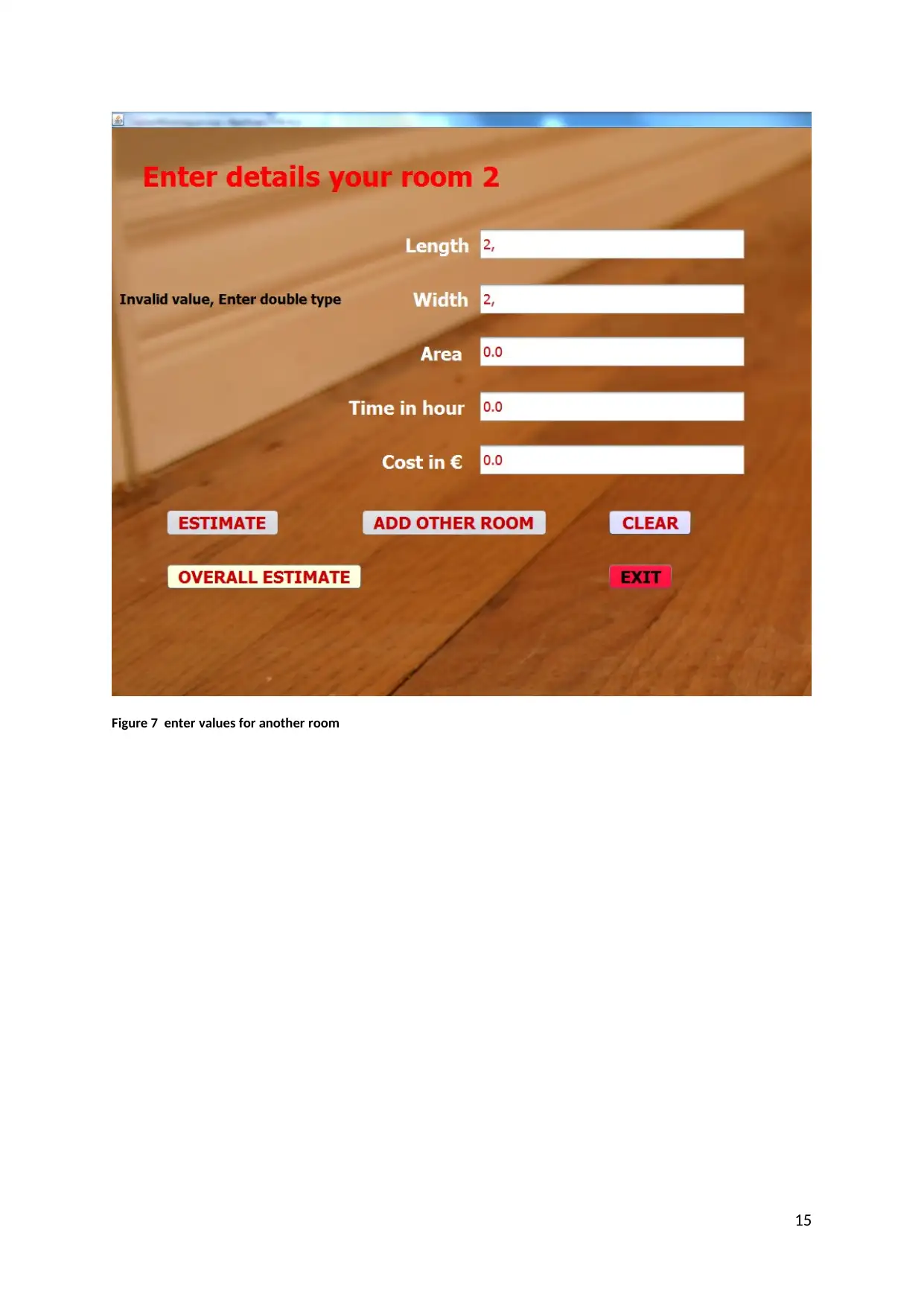
Figure 7 enter values for another room
15
15
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
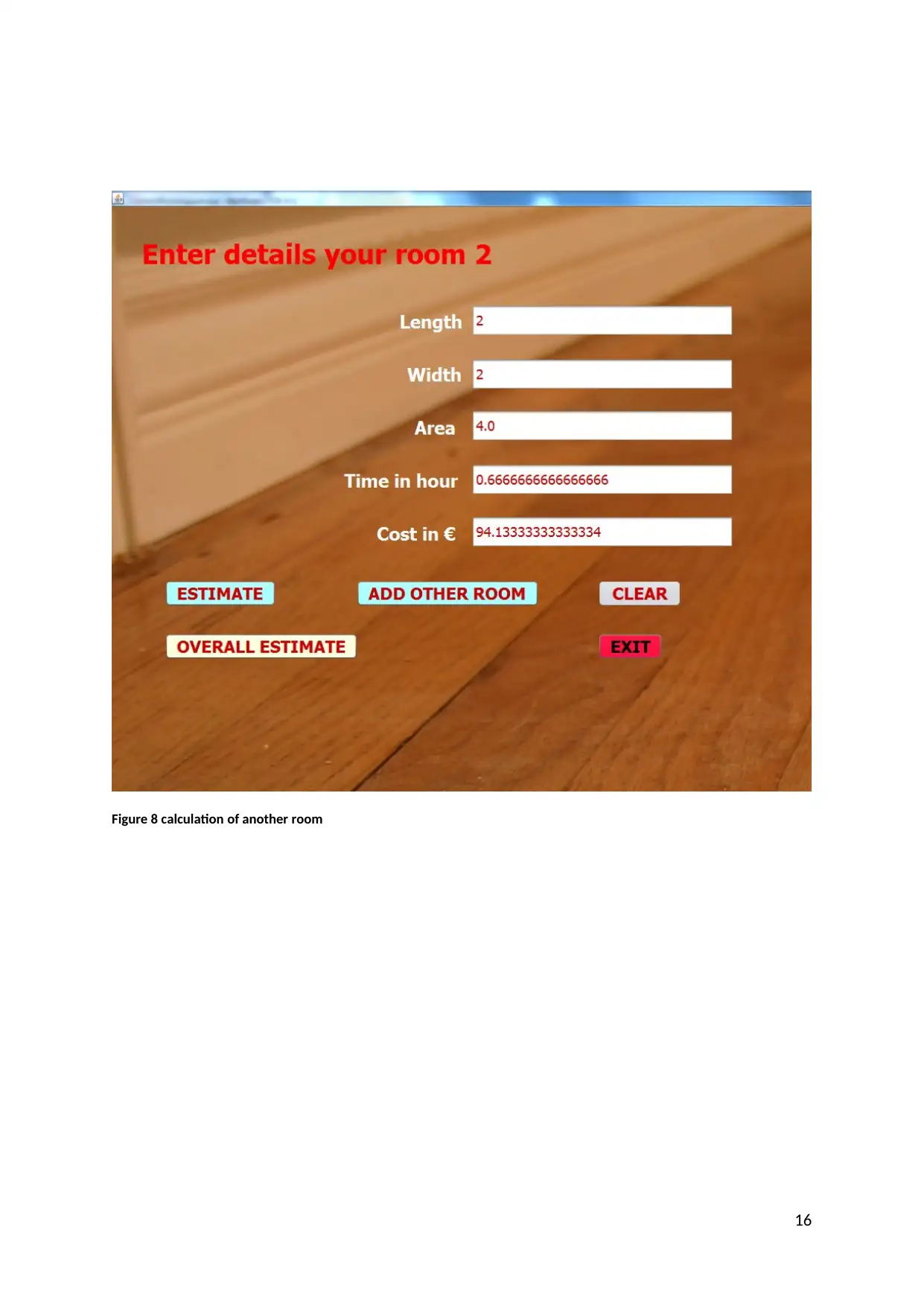
Figure 8 calculation of another room
16
16
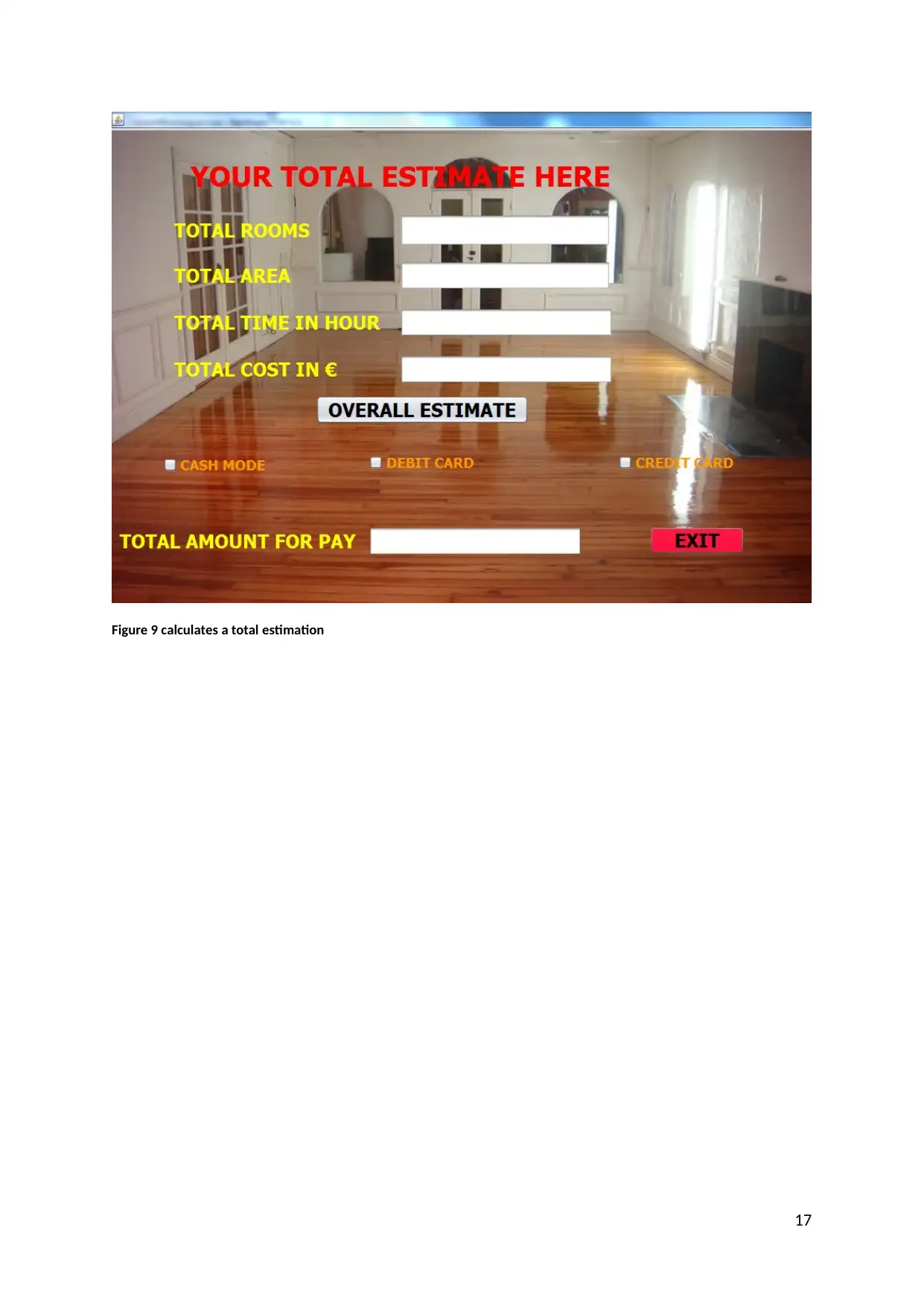
Figure 9 calculates a total estimation
17
17
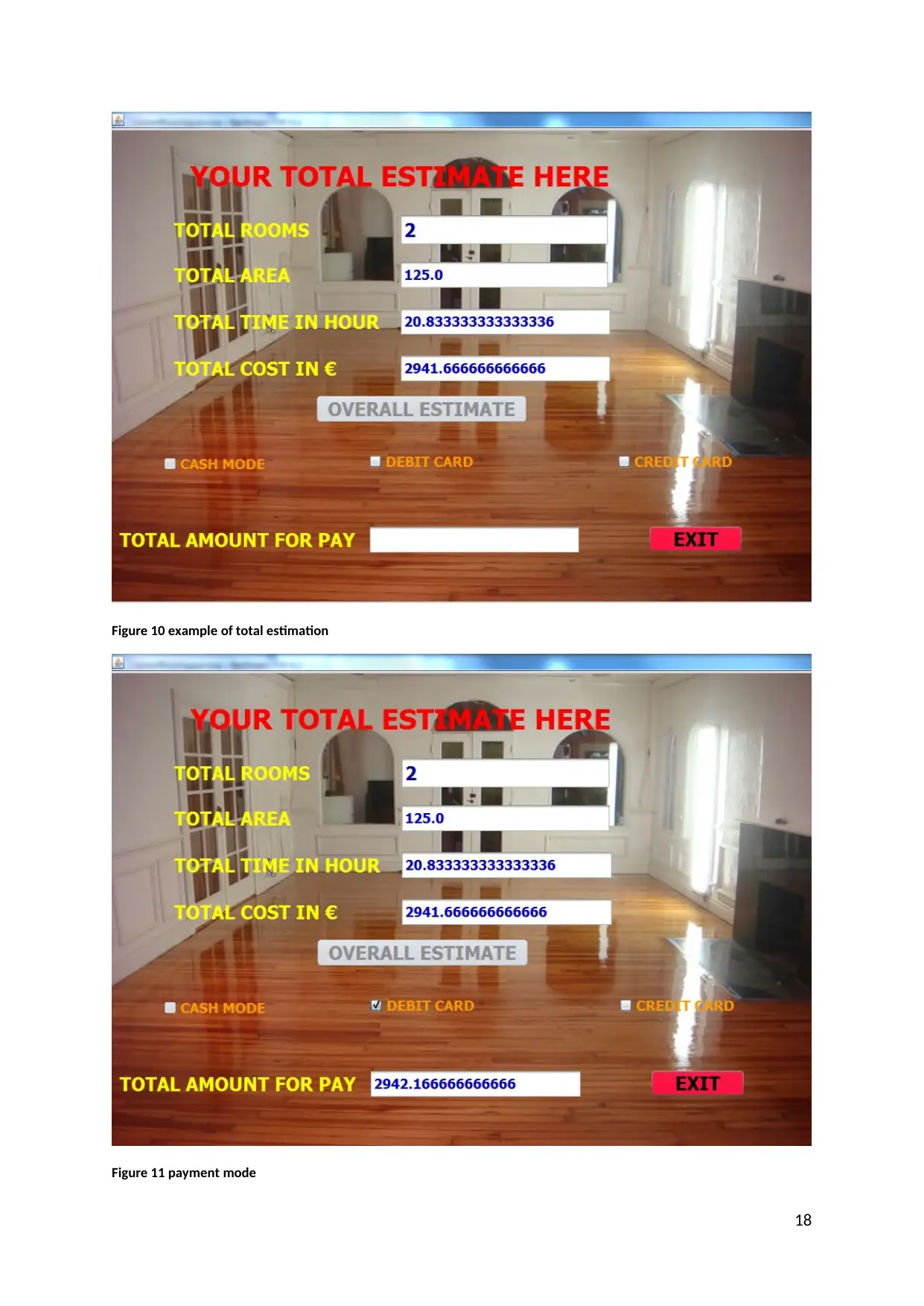
Figure 10 example of total estimation
Figure 11 payment mode
18
Figure 11 payment mode
18
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
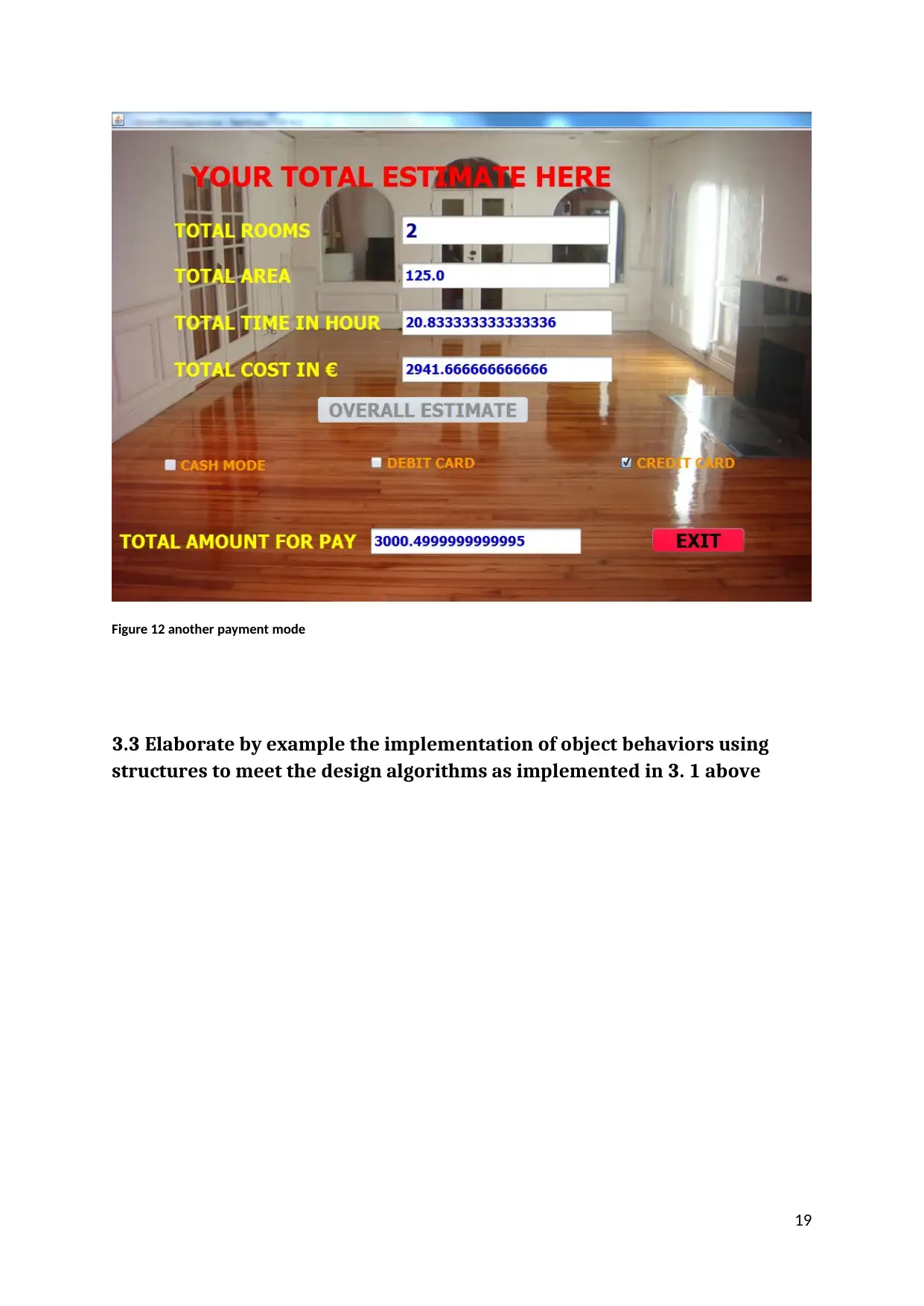
Figure 12 another payment mode
3.3 Elaborate by example the implementation of object behaviors using
structures to meet the design algorithms as implemented in 3. 1 above
19
3.3 Elaborate by example the implementation of object behaviors using
structures to meet the design algorithms as implemented in 3. 1 above
19
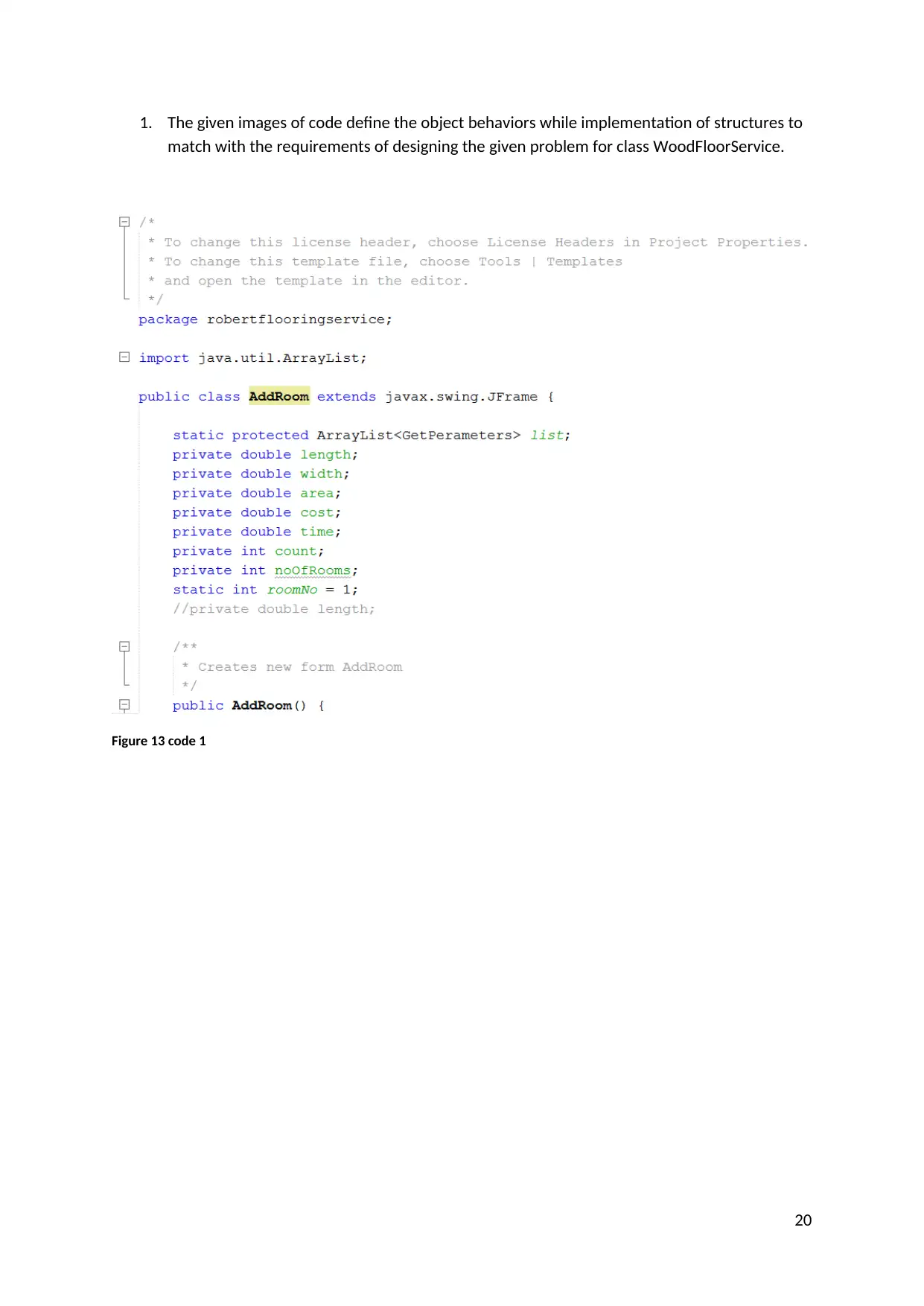
1. The given images of code define the object behaviors while implementation of structures to
match with the requirements of designing the given problem for class WoodFloorService.
Figure 13 code 1
20
match with the requirements of designing the given problem for class WoodFloorService.
Figure 13 code 1
20
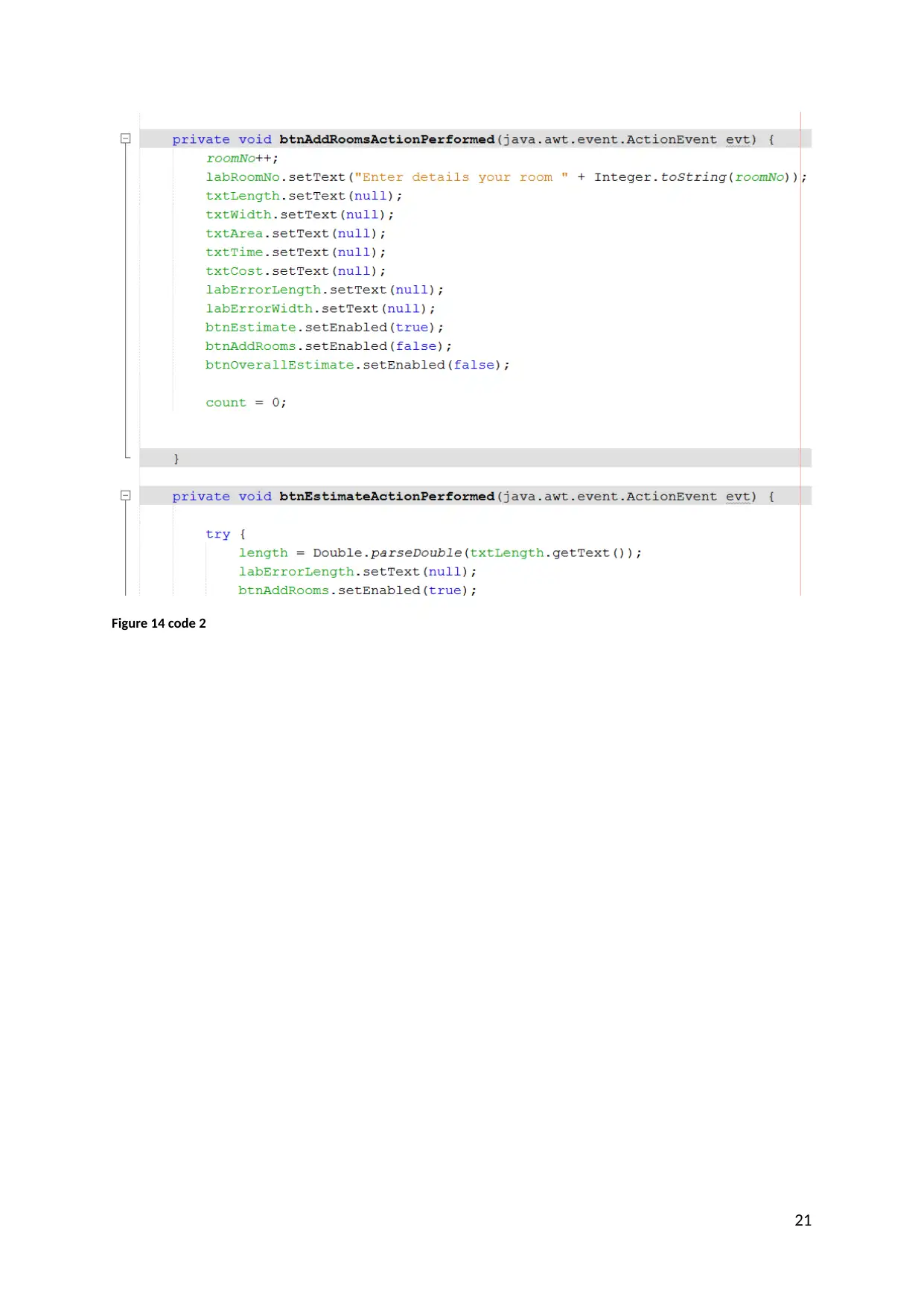
Figure 14 code 2
21
21
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
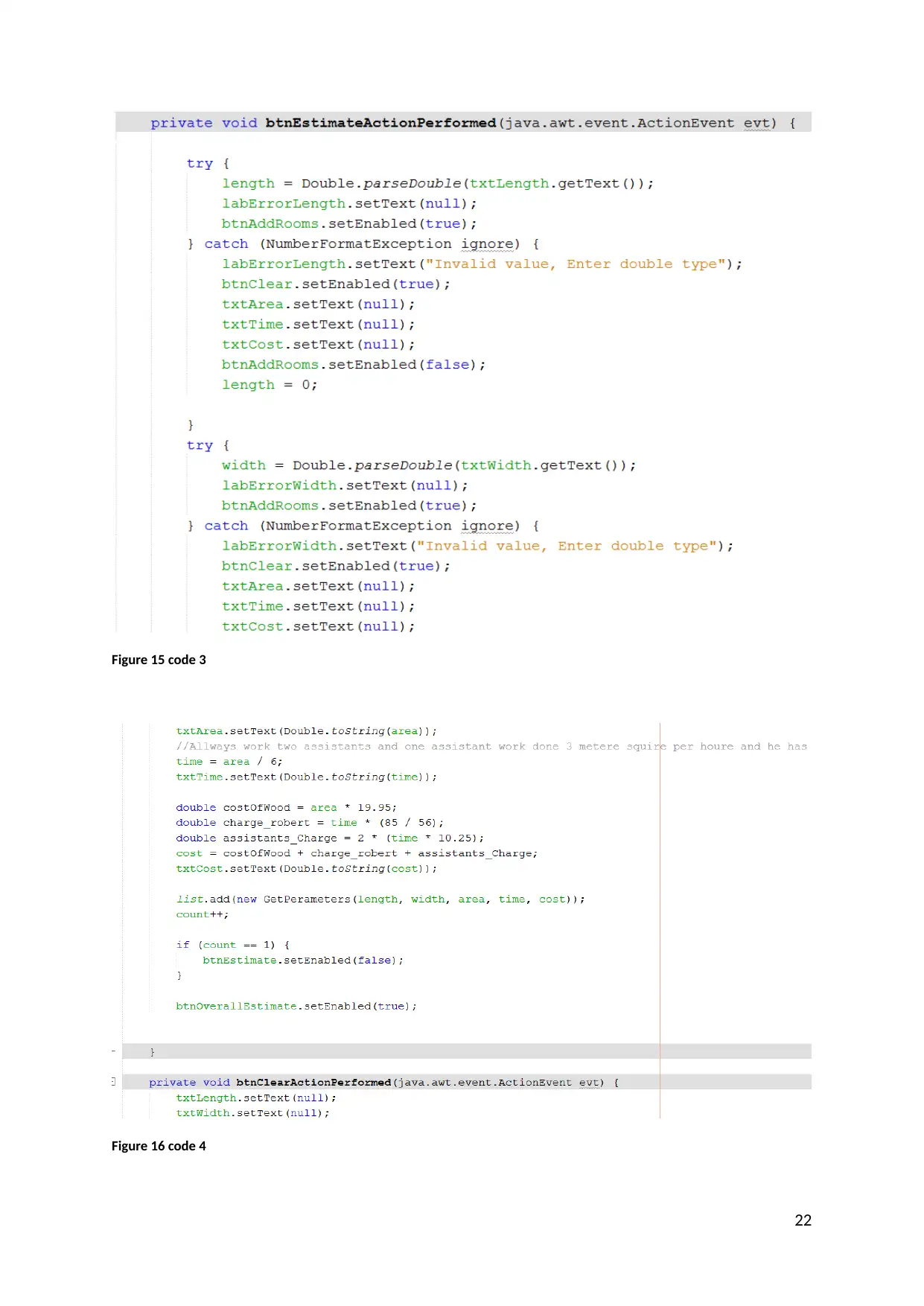
Figure 15 code 3
Figure 16 code 4
22
Figure 16 code 4
22
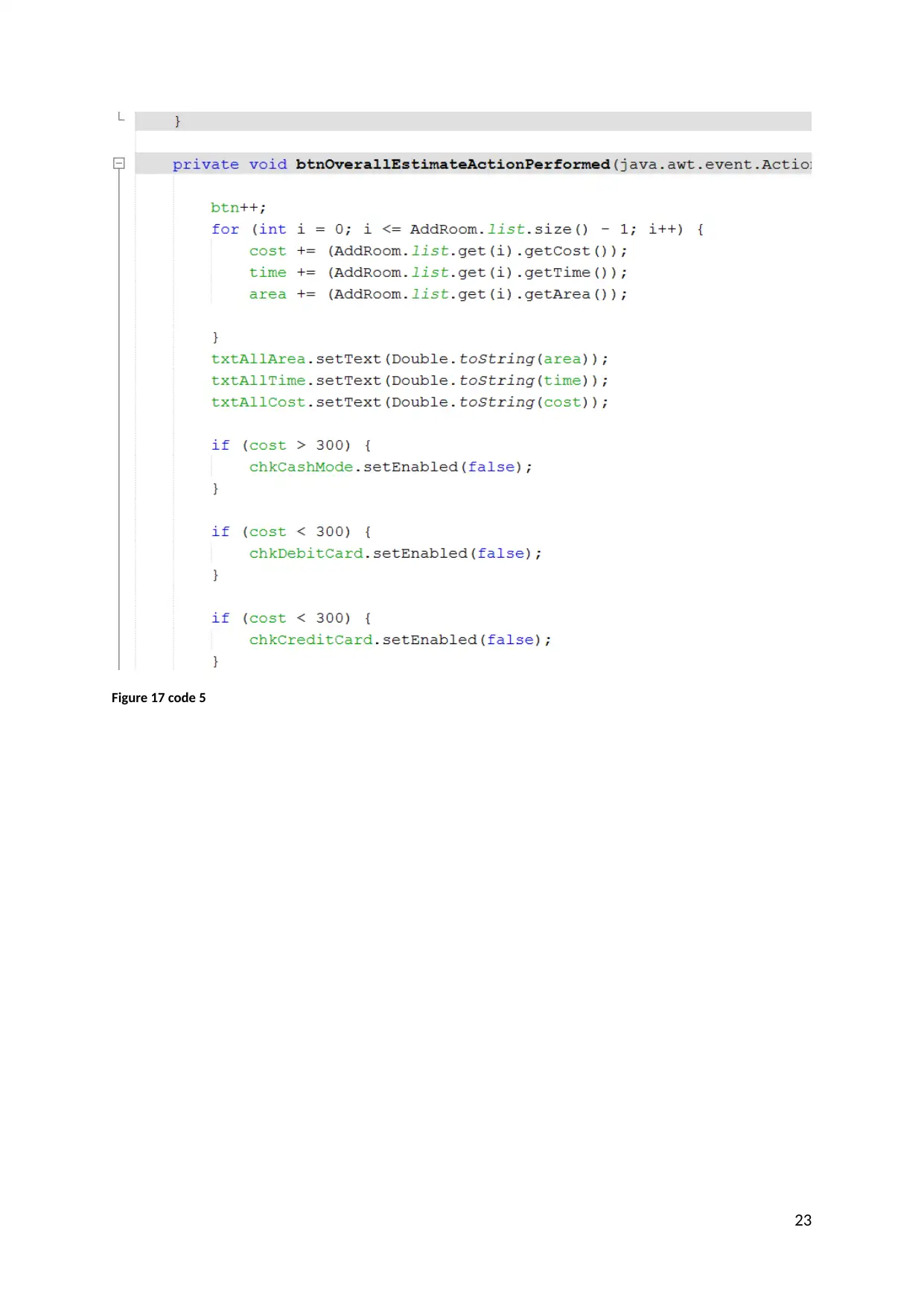
Figure 17 code 5
23
23
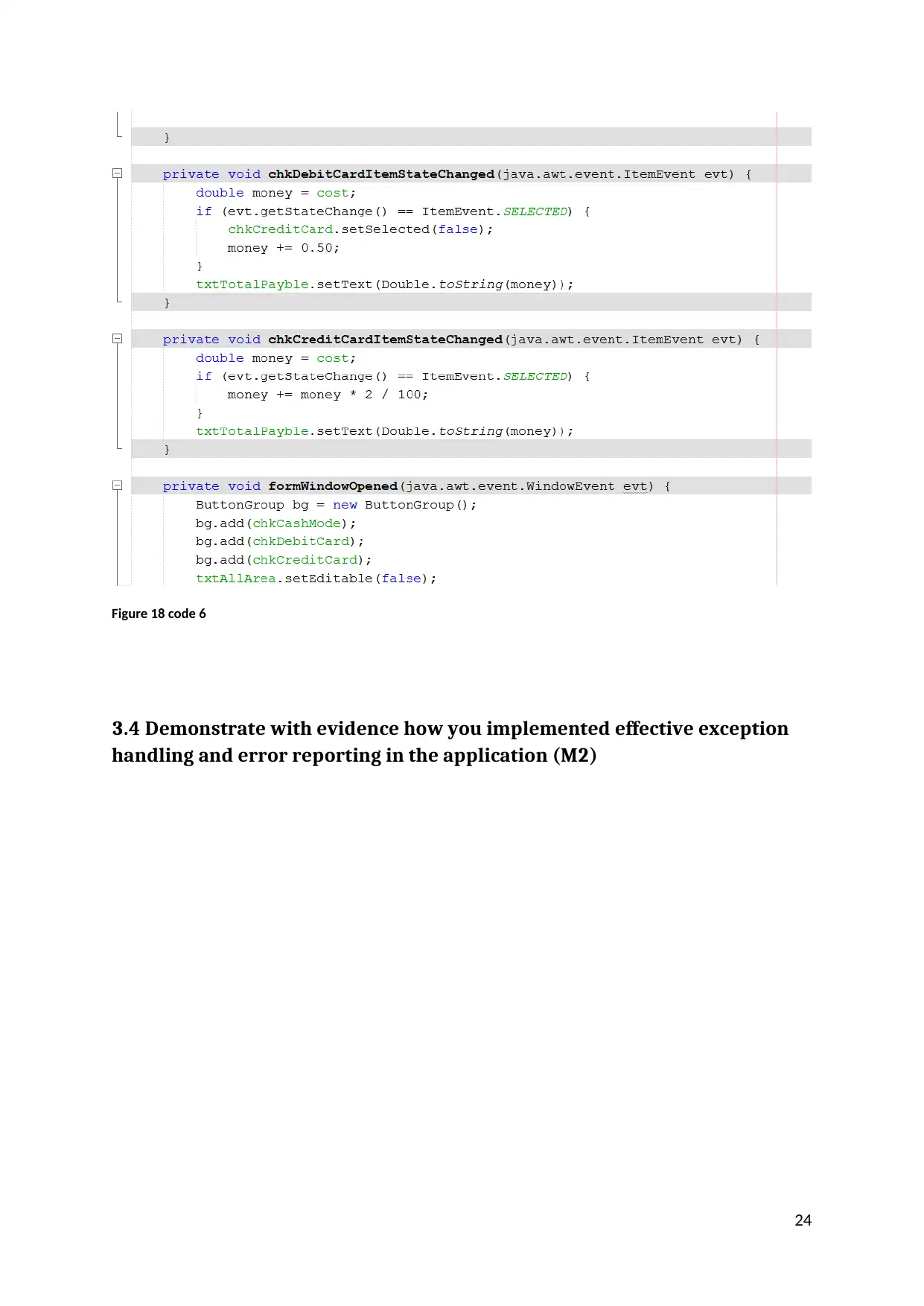
Figure 18 code 6
3.4 Demonstrate with evidence how you implemented effective exception
handling and error reporting in the application (M2)
24
3.4 Demonstrate with evidence how you implemented effective exception
handling and error reporting in the application (M2)
24
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
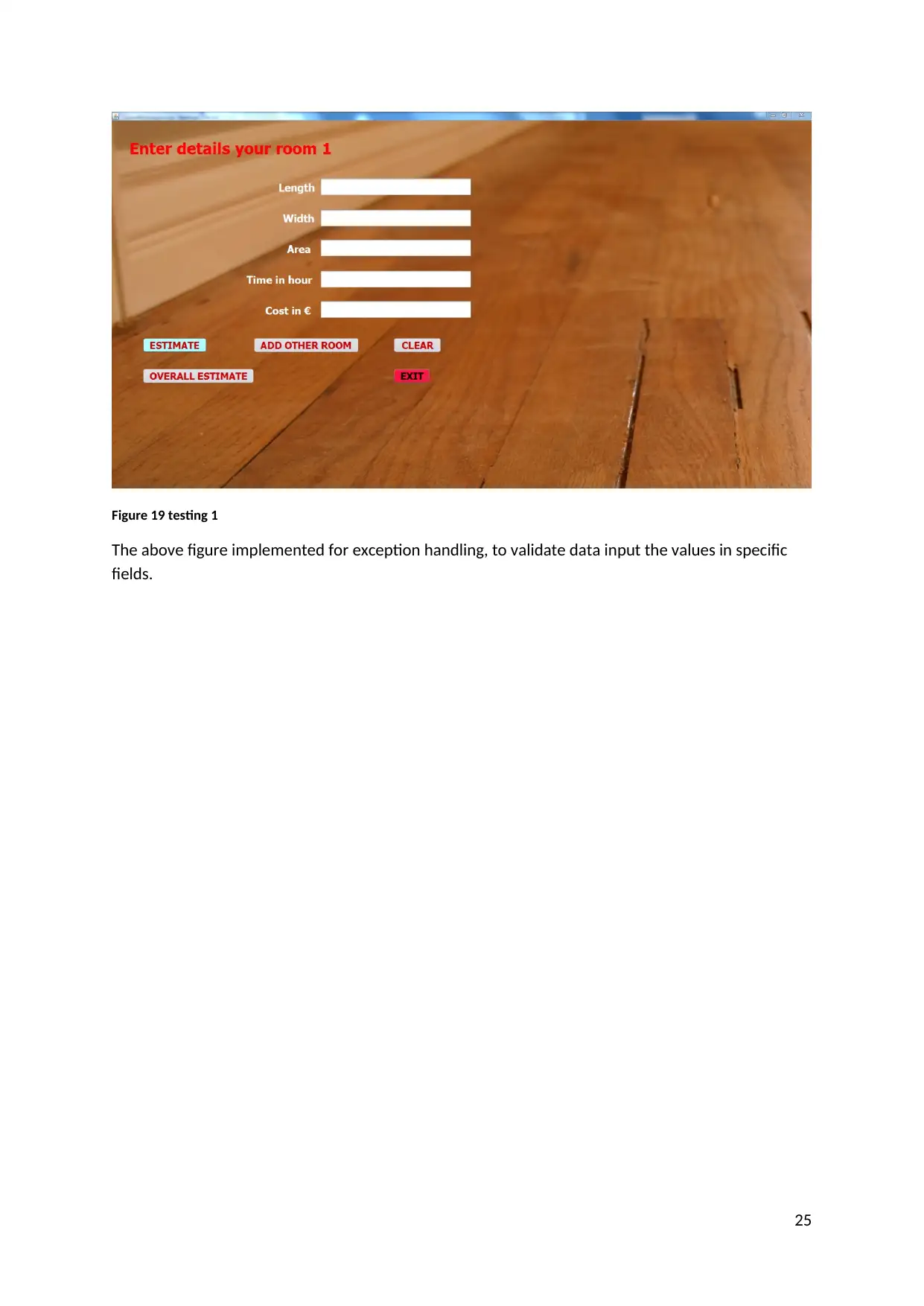
Figure 19 testing 1
The above figure implemented for exception handling, to validate data input the values in specific
fields.
25
The above figure implemented for exception handling, to validate data input the values in specific
fields.
25
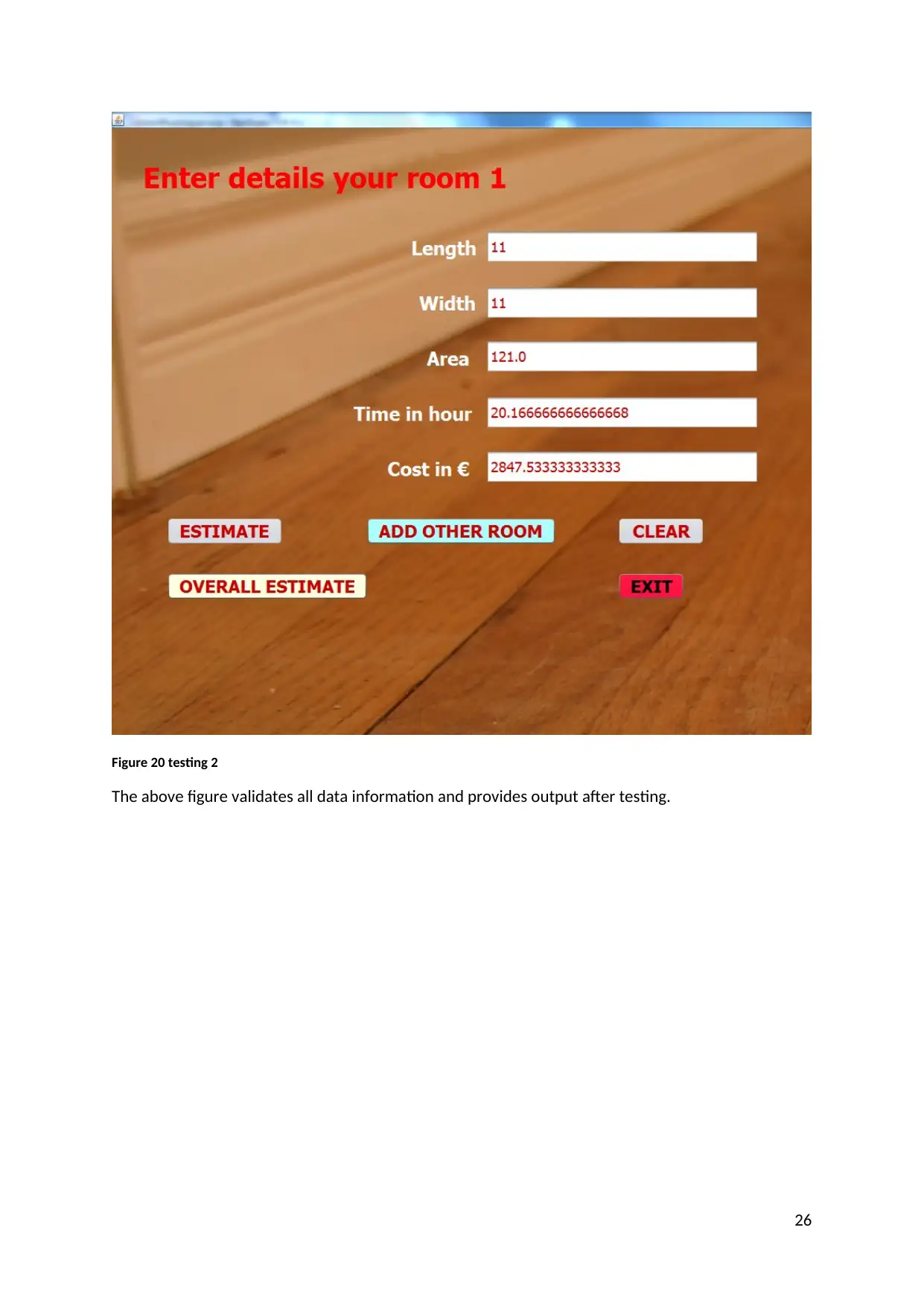
Figure 20 testing 2
The above figure validates all data information and provides output after testing.
26
The above figure validates all data information and provides output after testing.
26
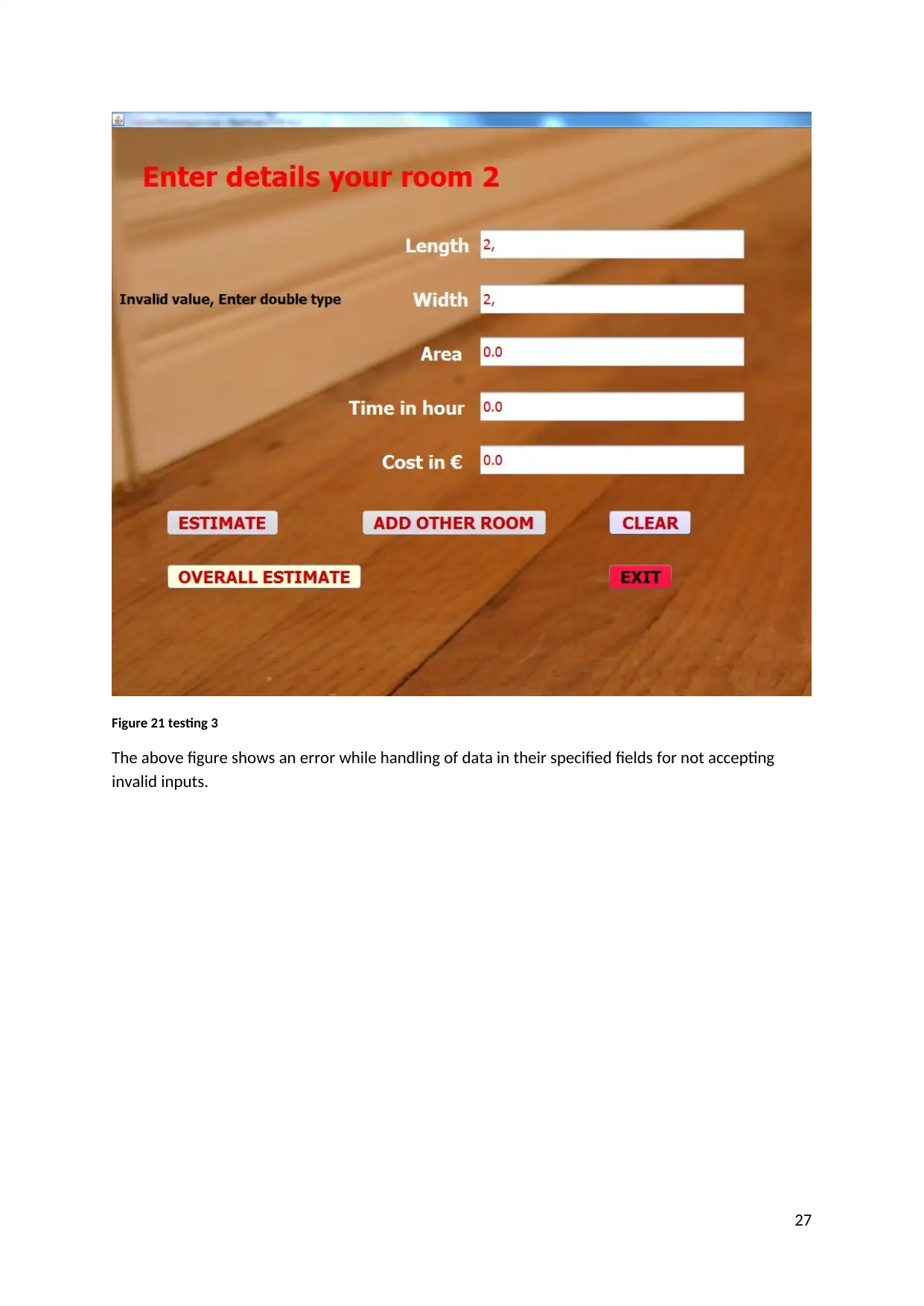
Figure 21 testing 3
The above figure shows an error while handling of data in their specified fields for not accepting
invalid inputs.
27
The above figure shows an error while handling of data in their specified fields for not accepting
invalid inputs.
27
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
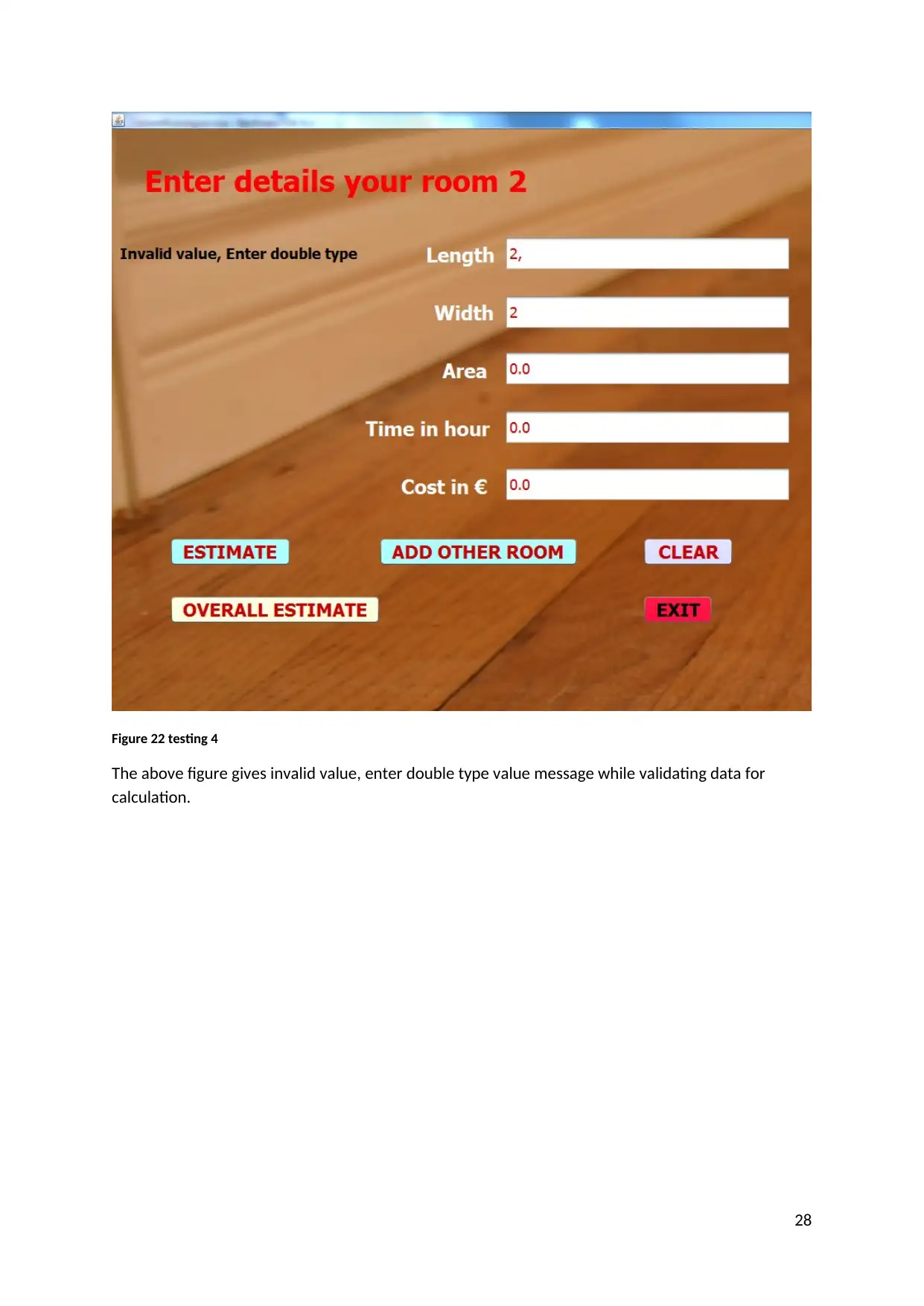
Figure 22 testing 4
The above figure gives invalid value, enter double type value message while validating data for
calculation.
28
The above figure gives invalid value, enter double type value message while validating data for
calculation.
28
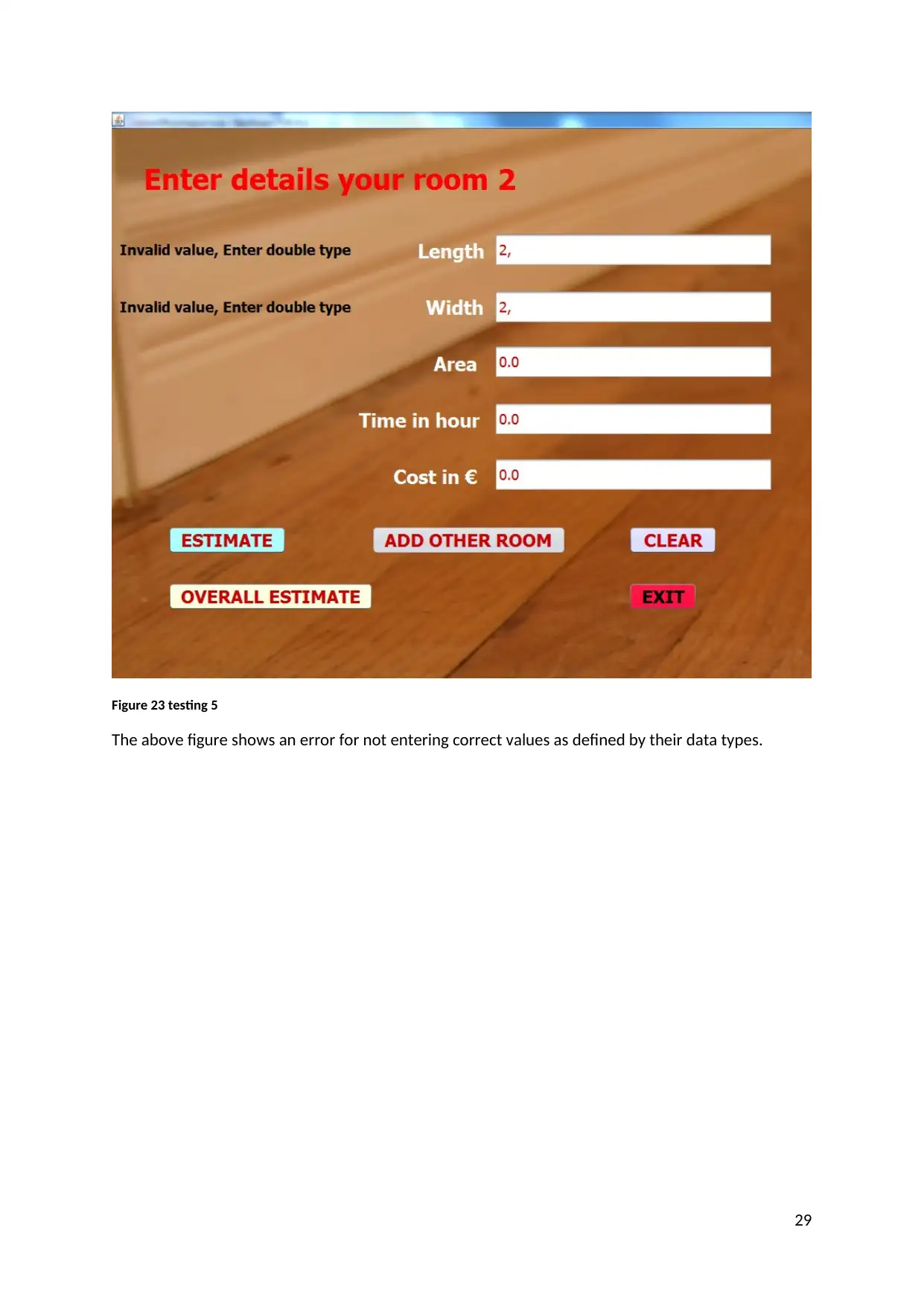
Figure 23 testing 5
The above figure shows an error for not entering correct values as defined by their data types.
29
The above figure shows an error for not entering correct values as defined by their data types.
29
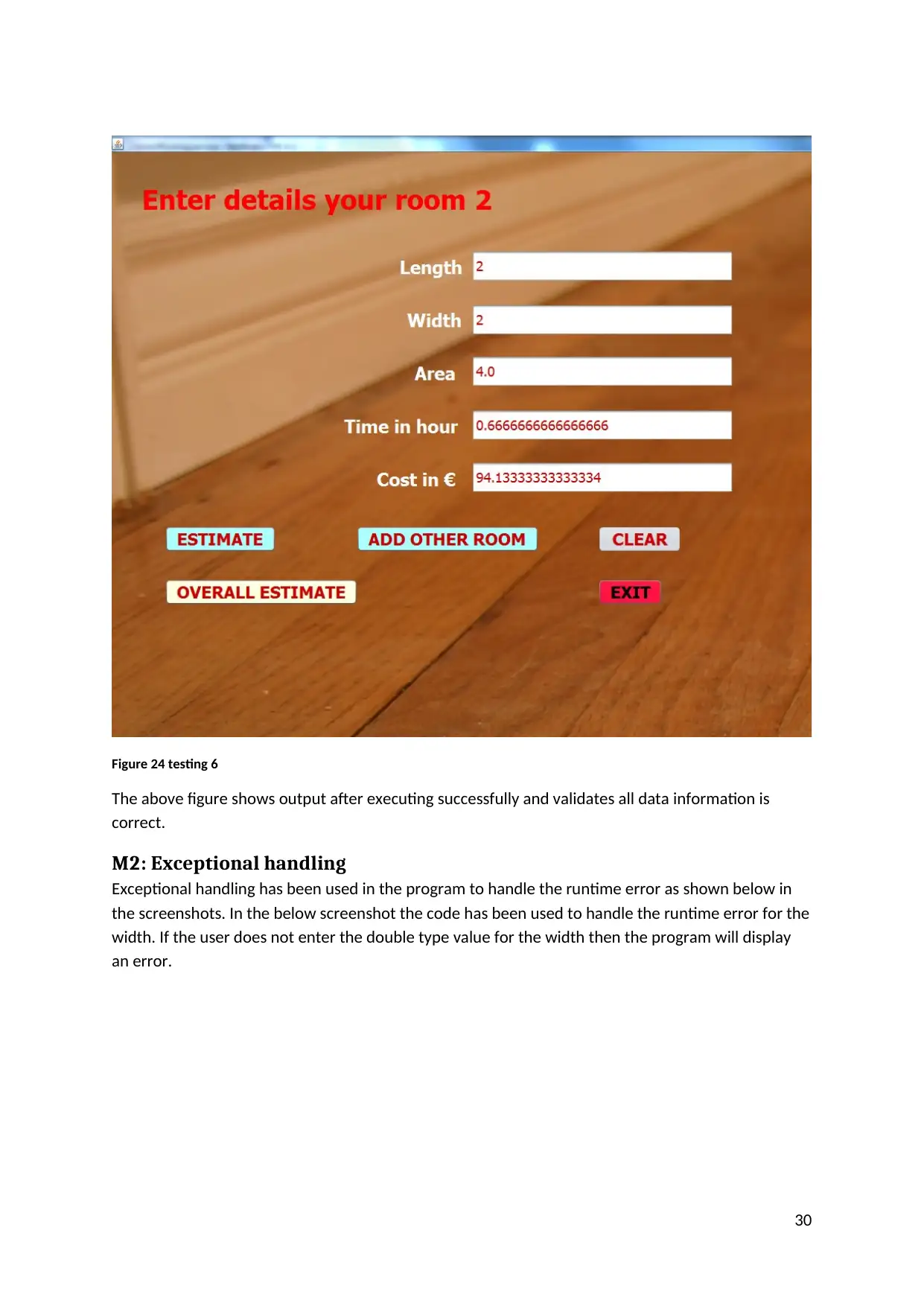
Figure 24 testing 6
The above figure shows output after executing successfully and validates all data information is
correct.
M2: Exceptional handling
Exceptional handling has been used in the program to handle the runtime error as shown below in
the screenshots. In the below screenshot the code has been used to handle the runtime error for the
width. If the user does not enter the double type value for the width then the program will display
an error.
30
The above figure shows output after executing successfully and validates all data information is
correct.
M2: Exceptional handling
Exceptional handling has been used in the program to handle the runtime error as shown below in
the screenshots. In the below screenshot the code has been used to handle the runtime error for the
width. If the user does not enter the double type value for the width then the program will display
an error.
30
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
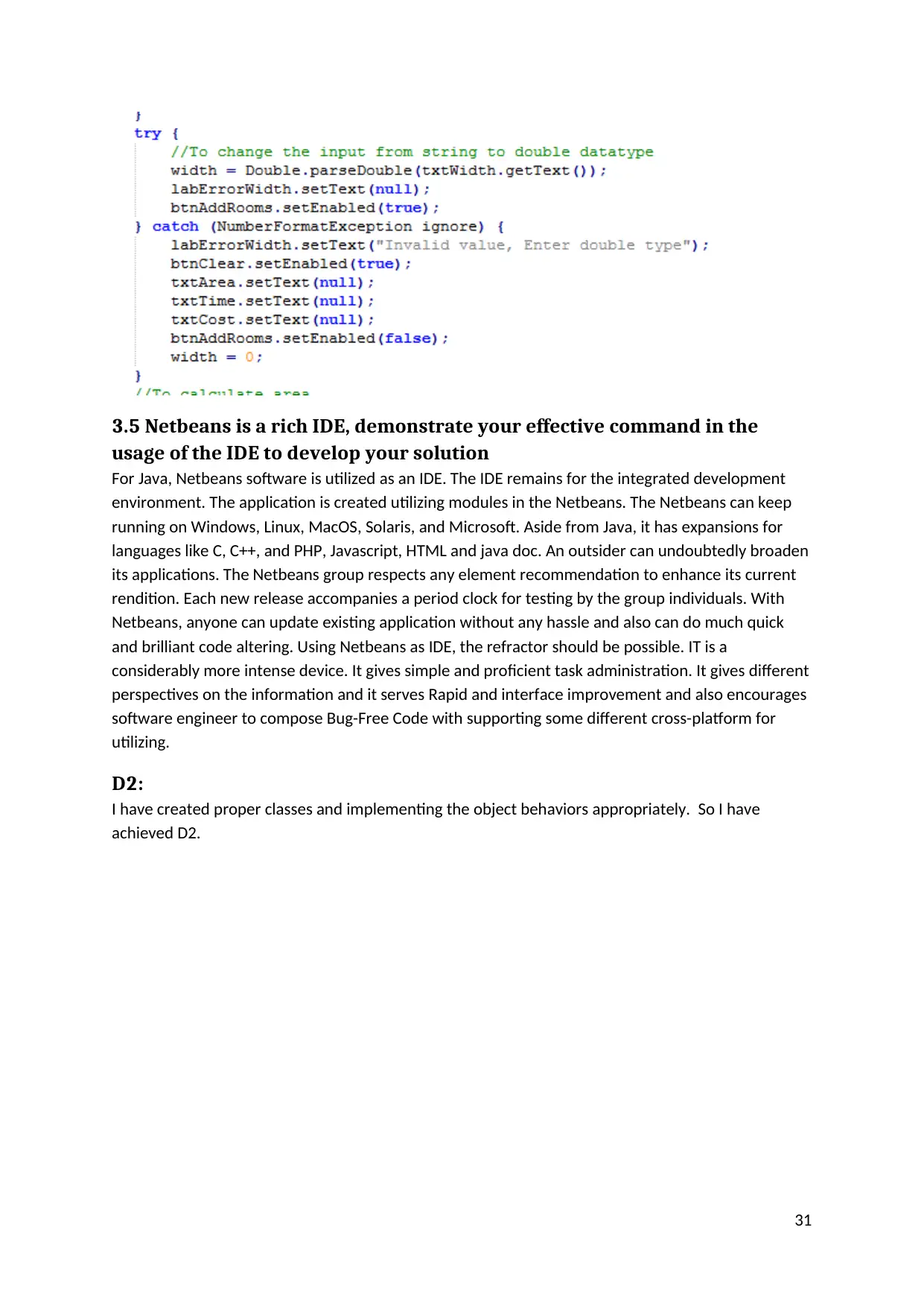
3.5 Netbeans is a rich IDE, demonstrate your effective command in the
usage of the IDE to develop your solution
For Java, Netbeans software is utilized as an IDE. The IDE remains for the integrated development
environment. The application is created utilizing modules in the Netbeans. The Netbeans can keep
running on Windows, Linux, MacOS, Solaris, and Microsoft. Aside from Java, it has expansions for
languages like C, C++, and PHP, Javascript, HTML and java doc. An outsider can undoubtedly broaden
its applications. The Netbeans group respects any element recommendation to enhance its current
rendition. Each new release accompanies a period clock for testing by the group individuals. With
Netbeans, anyone can update existing application without any hassle and also can do much quick
and brilliant code altering. Using Netbeans as IDE, the refractor should be possible. IT is a
considerably more intense device. It gives simple and proficient task administration. It gives different
perspectives on the information and it serves Rapid and interface improvement and also encourages
software engineer to compose Bug-Free Code with supporting some different cross-platform for
utilizing.
D2:
I have created proper classes and implementing the object behaviors appropriately. So I have
achieved D2.
31
usage of the IDE to develop your solution
For Java, Netbeans software is utilized as an IDE. The IDE remains for the integrated development
environment. The application is created utilizing modules in the Netbeans. The Netbeans can keep
running on Windows, Linux, MacOS, Solaris, and Microsoft. Aside from Java, it has expansions for
languages like C, C++, and PHP, Javascript, HTML and java doc. An outsider can undoubtedly broaden
its applications. The Netbeans group respects any element recommendation to enhance its current
rendition. Each new release accompanies a period clock for testing by the group individuals. With
Netbeans, anyone can update existing application without any hassle and also can do much quick
and brilliant code altering. Using Netbeans as IDE, the refractor should be possible. IT is a
considerably more intense device. It gives simple and proficient task administration. It gives different
perspectives on the information and it serves Rapid and interface improvement and also encourages
software engineer to compose Bug-Free Code with supporting some different cross-platform for
utilizing.
D2:
I have created proper classes and implementing the object behaviors appropriately. So I have
achieved D2.
31
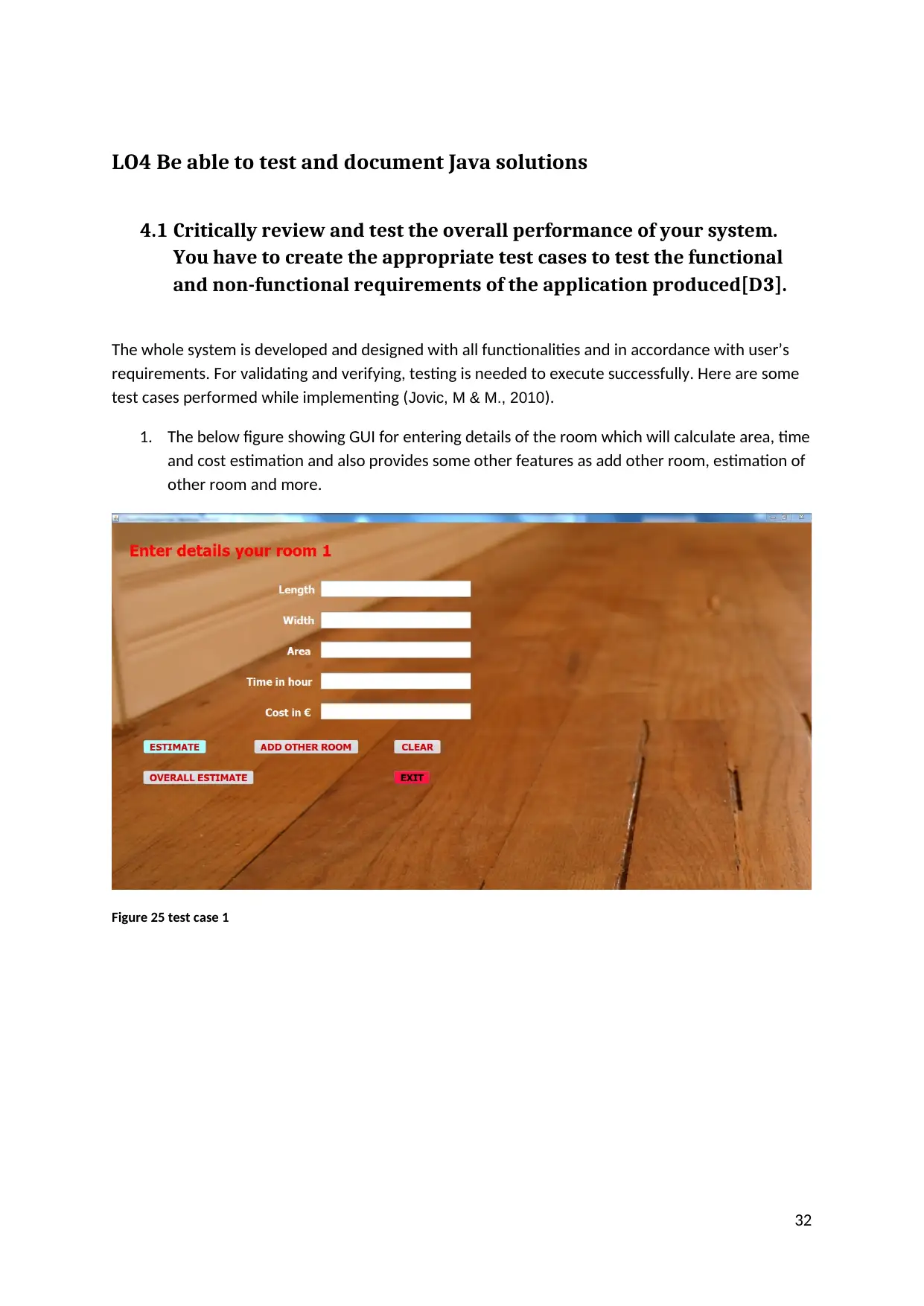
LO4 Be able to test and document Java solutions
4.1 Critically review and test the overall performance of your system.
You have to create the appropriate test cases to test the functional
and non-functional requirements of the application produced[D3].
The whole system is developed and designed with all functionalities and in accordance with user’s
requirements. For validating and verifying, testing is needed to execute successfully. Here are some
test cases performed while implementing (Jovic, M & M., 2010).
1. The below figure showing GUI for entering details of the room which will calculate area, time
and cost estimation and also provides some other features as add other room, estimation of
other room and more.
Figure 25 test case 1
32
4.1 Critically review and test the overall performance of your system.
You have to create the appropriate test cases to test the functional
and non-functional requirements of the application produced[D3].
The whole system is developed and designed with all functionalities and in accordance with user’s
requirements. For validating and verifying, testing is needed to execute successfully. Here are some
test cases performed while implementing (Jovic, M & M., 2010).
1. The below figure showing GUI for entering details of the room which will calculate area, time
and cost estimation and also provides some other features as add other room, estimation of
other room and more.
Figure 25 test case 1
32
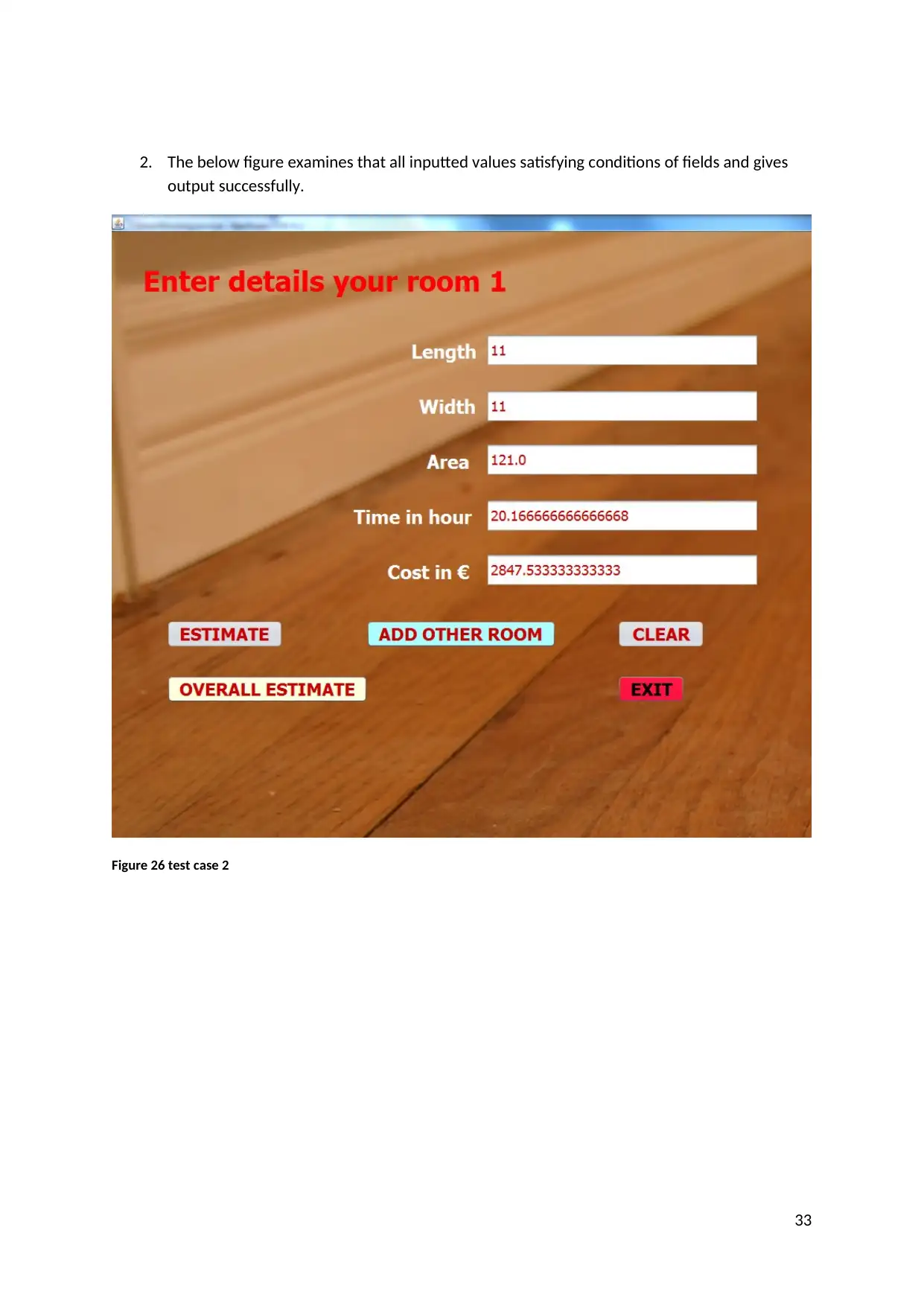
2. The below figure examines that all inputted values satisfying conditions of fields and gives
output successfully.
Figure 26 test case 2
33
output successfully.
Figure 26 test case 2
33
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
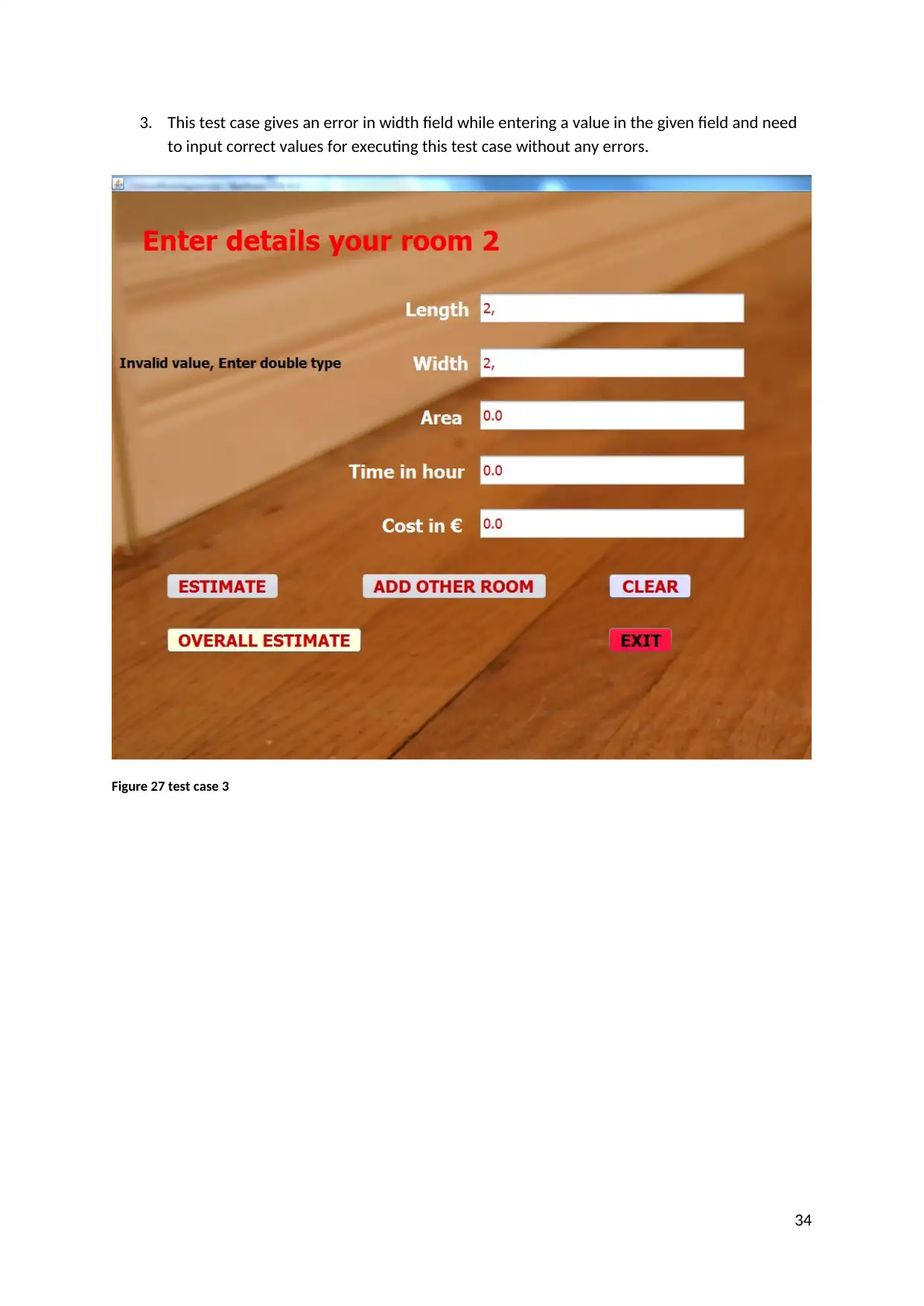
3. This test case gives an error in width field while entering a value in the given field and need
to input correct values for executing this test case without any errors.
Figure 27 test case 3
34
to input correct values for executing this test case without any errors.
Figure 27 test case 3
34
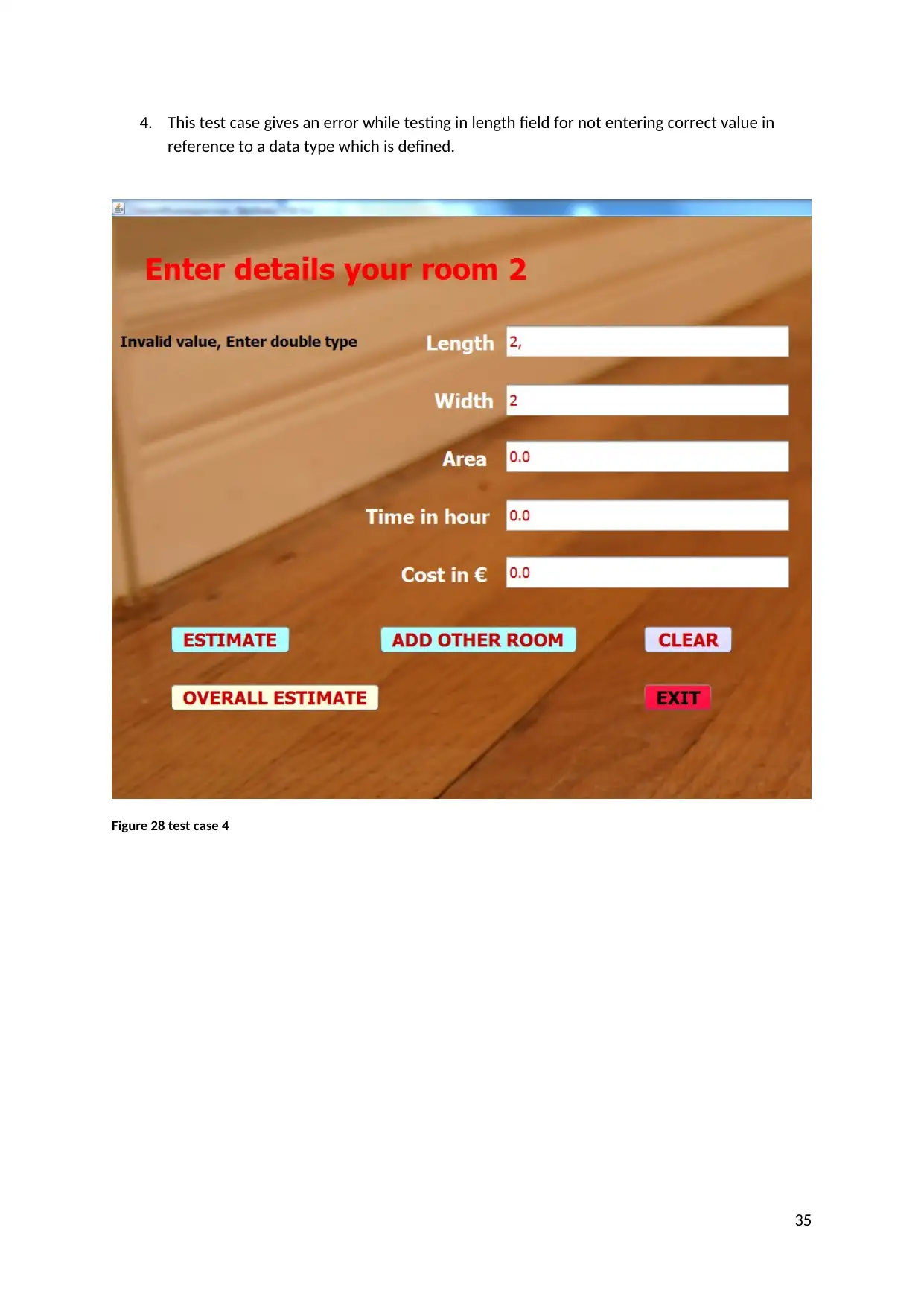
4. This test case gives an error while testing in length field for not entering correct value in
reference to a data type which is defined.
Figure 28 test case 4
35
reference to a data type which is defined.
Figure 28 test case 4
35
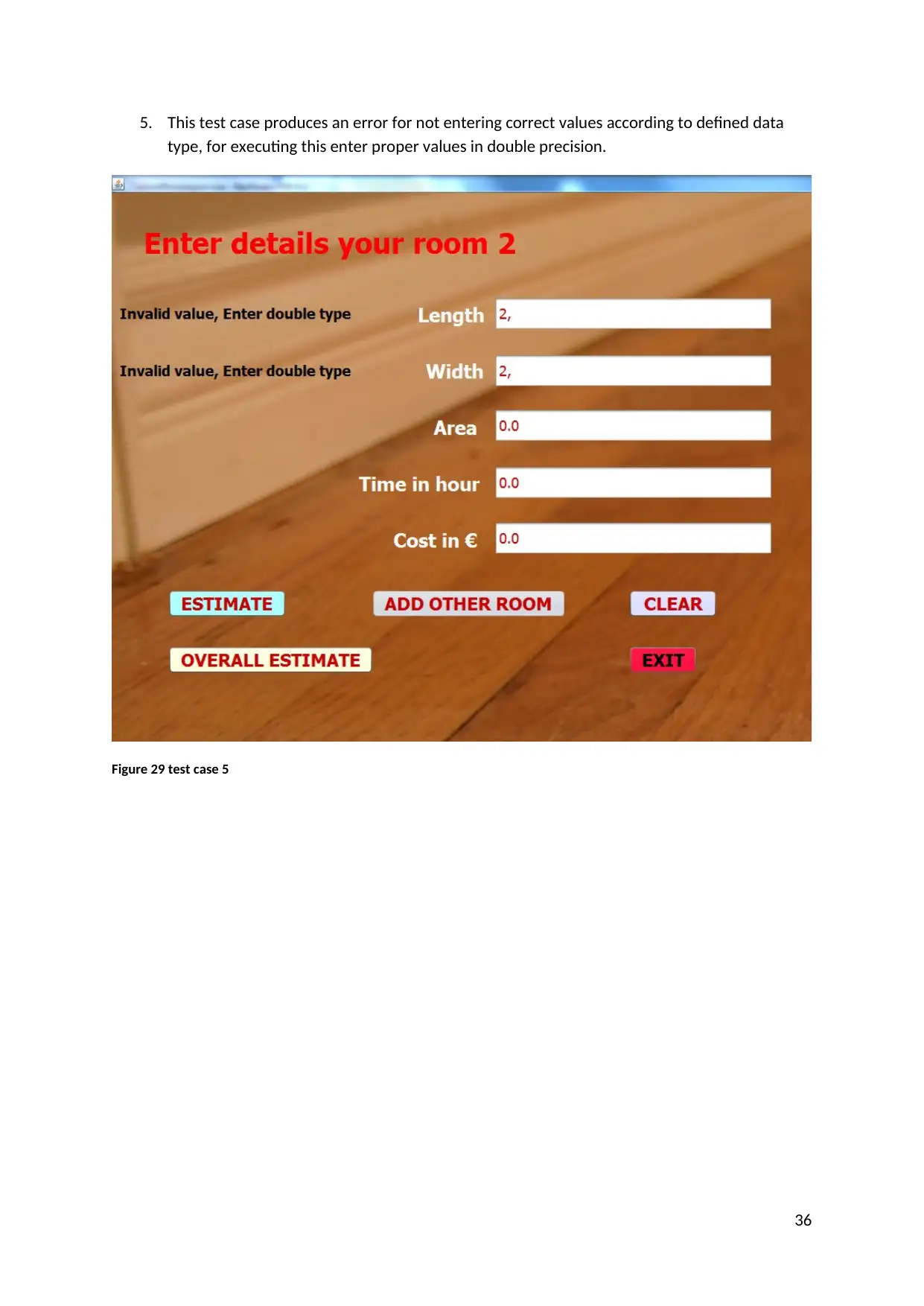
5. This test case produces an error for not entering correct values according to defined data
type, for executing this enter proper values in double precision.
Figure 29 test case 5
36
type, for executing this enter proper values in double precision.
Figure 29 test case 5
36
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
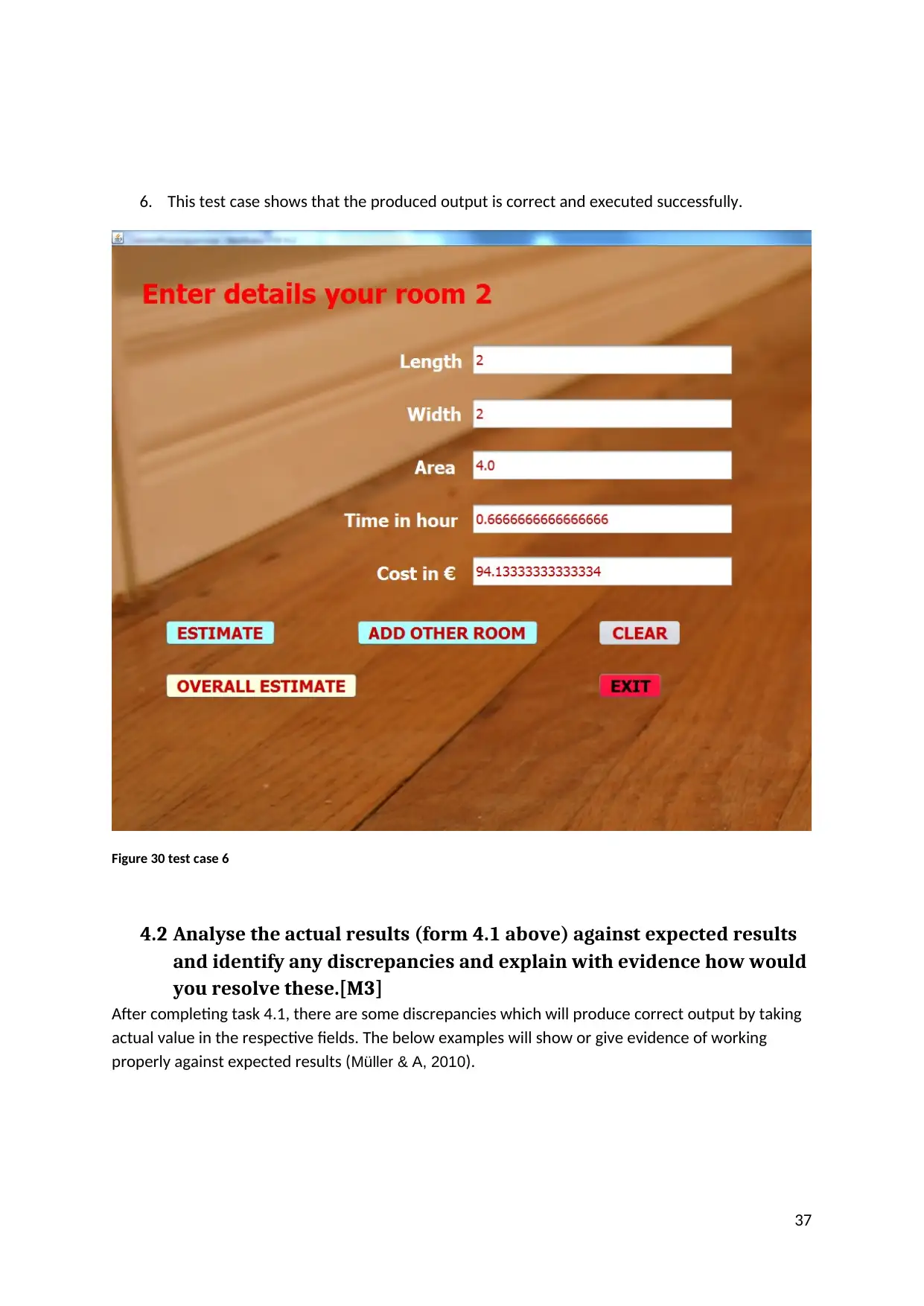
6. This test case shows that the produced output is correct and executed successfully.
Figure 30 test case 6
4.2 Analyse the actual results (form 4.1 above) against expected results
and identify any discrepancies and explain with evidence how would
you resolve these.[M3]
After completing task 4.1, there are some discrepancies which will produce correct output by taking
actual value in the respective fields. The below examples will show or give evidence of working
properly against expected results (Müller & A, 2010).
37
Figure 30 test case 6
4.2 Analyse the actual results (form 4.1 above) against expected results
and identify any discrepancies and explain with evidence how would
you resolve these.[M3]
After completing task 4.1, there are some discrepancies which will produce correct output by taking
actual value in the respective fields. The below examples will show or give evidence of working
properly against expected results (Müller & A, 2010).
37
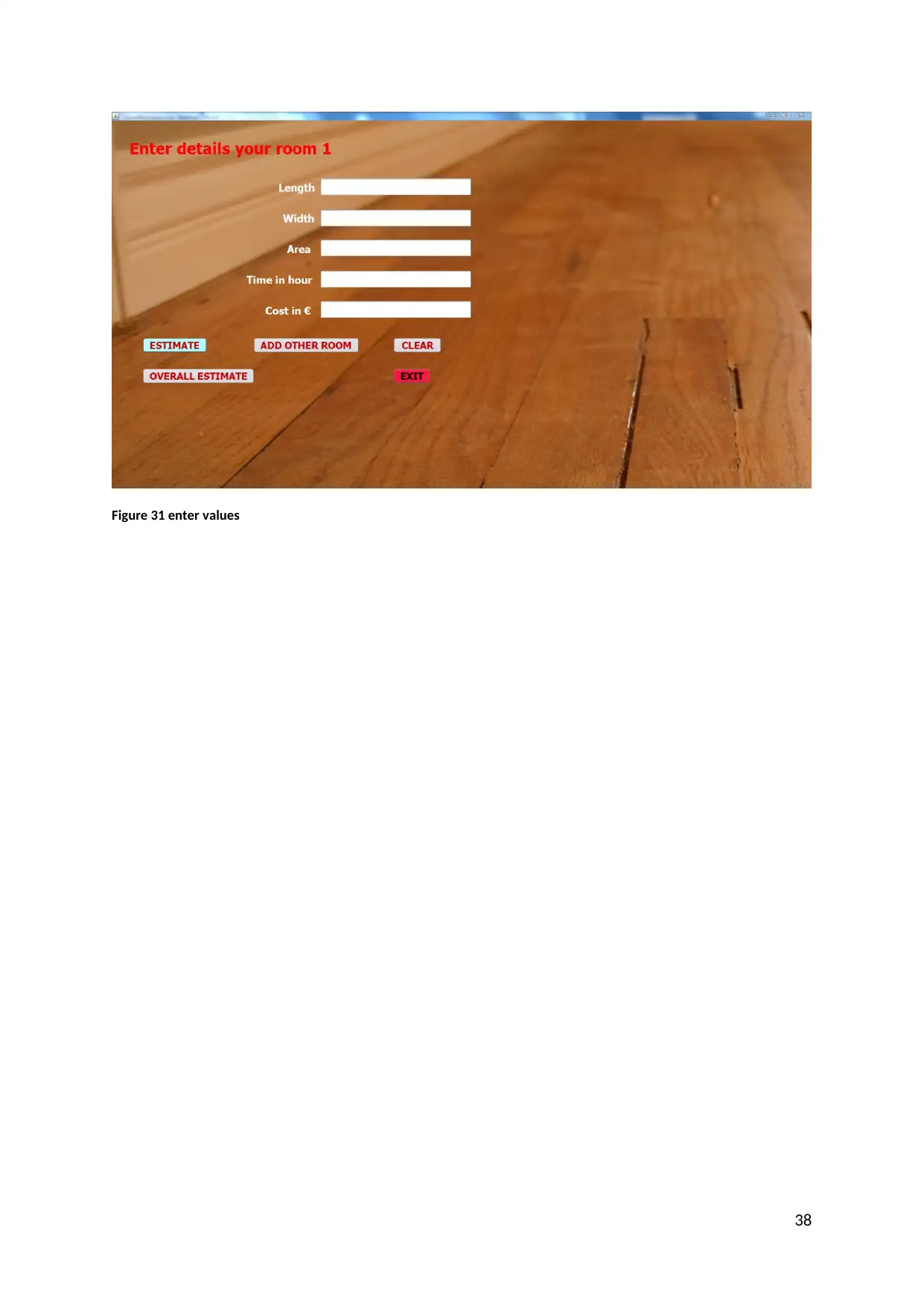
Figure 31 enter values
38
38
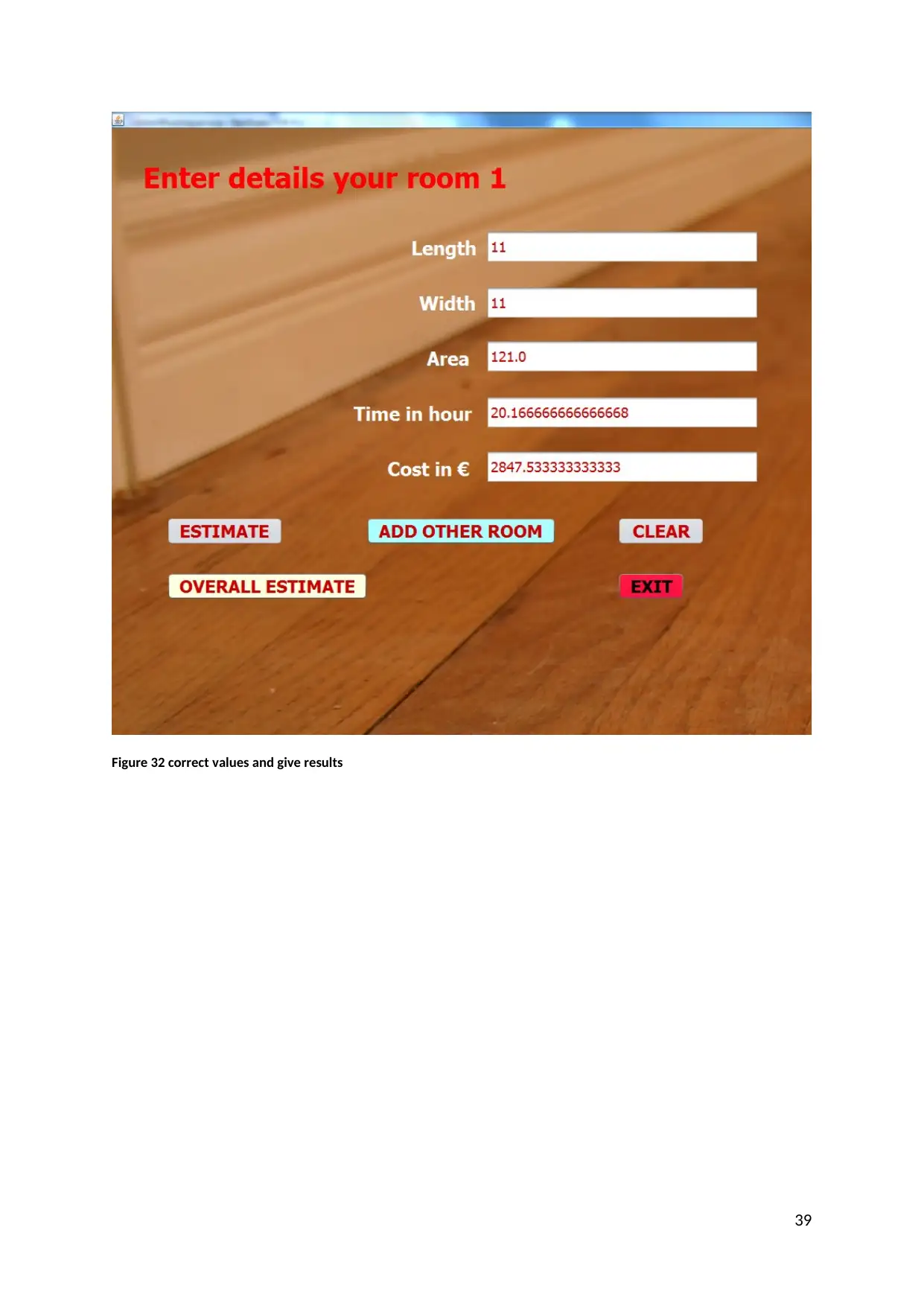
Figure 32 correct values and give results
39
39
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
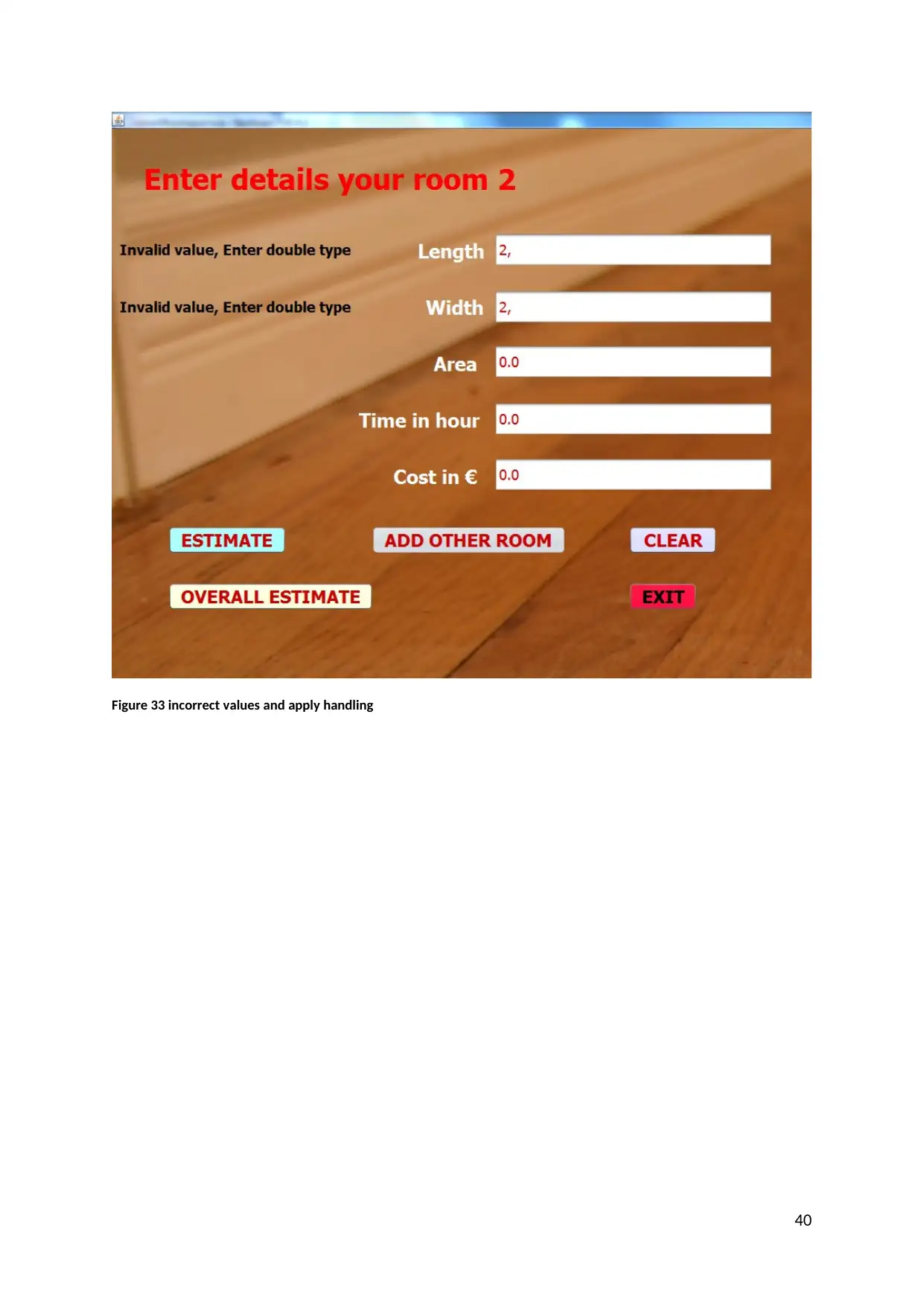
Figure 33 incorrect values and apply handling
40
40
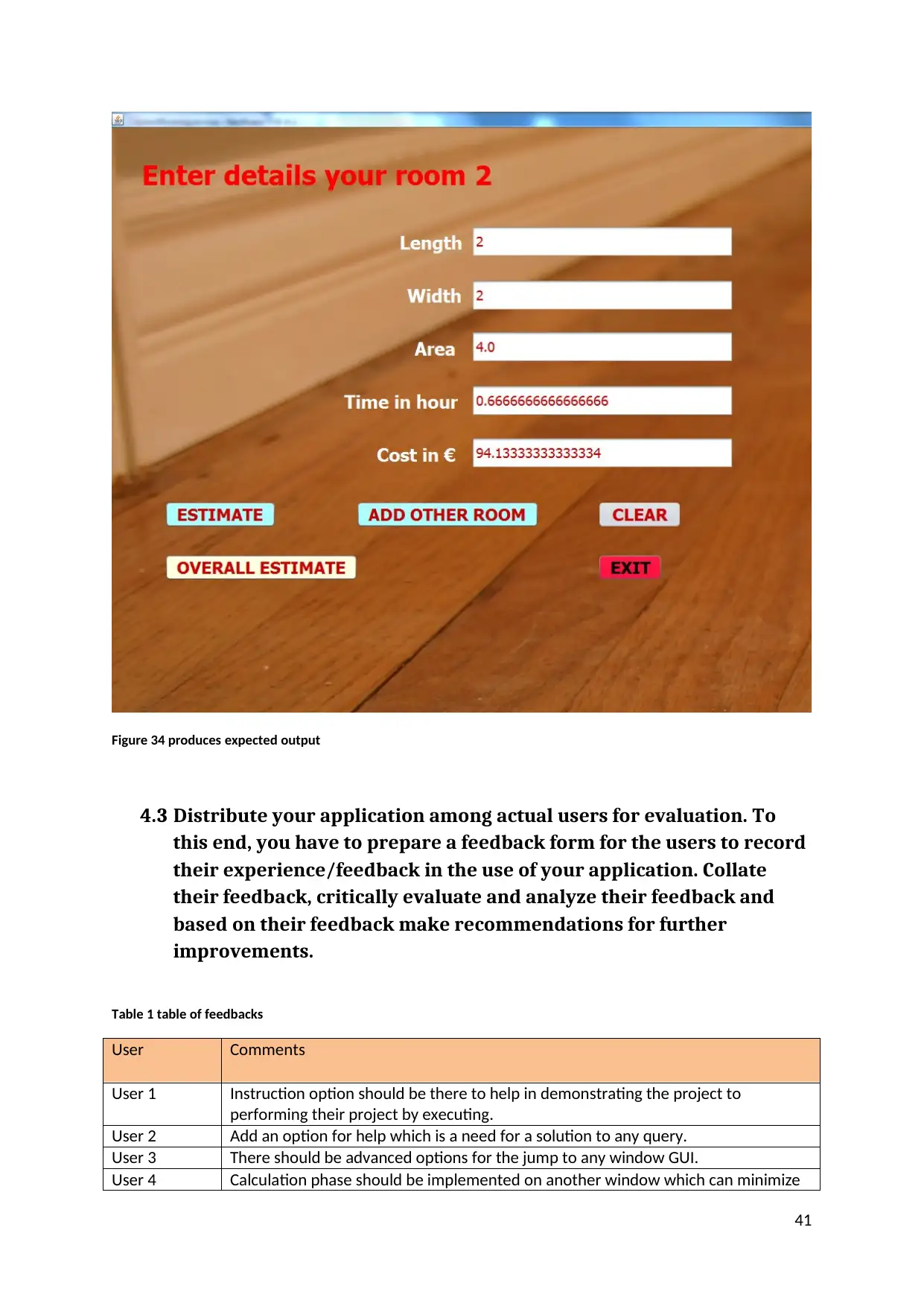
Figure 34 produces expected output
4.3 Distribute your application among actual users for evaluation. To
this end, you have to prepare a feedback form for the users to record
their experience/feedback in the use of your application. Collate
their feedback, critically evaluate and analyze their feedback and
based on their feedback make recommendations for further
improvements.
Table 1 table of feedbacks
User Comments
User 1 Instruction option should be there to help in demonstrating the project to
performing their project by executing.
User 2 Add an option for help which is a need for a solution to any query.
User 3 There should be advanced options for the jump to any window GUI.
User 4 Calculation phase should be implemented on another window which can minimize
41
4.3 Distribute your application among actual users for evaluation. To
this end, you have to prepare a feedback form for the users to record
their experience/feedback in the use of your application. Collate
their feedback, critically evaluate and analyze their feedback and
based on their feedback make recommendations for further
improvements.
Table 1 table of feedbacks
User Comments
User 1 Instruction option should be there to help in demonstrating the project to
performing their project by executing.
User 2 Add an option for help which is a need for a solution to any query.
User 3 There should be advanced options for the jump to any window GUI.
User 4 Calculation phase should be implemented on another window which can minimize
41
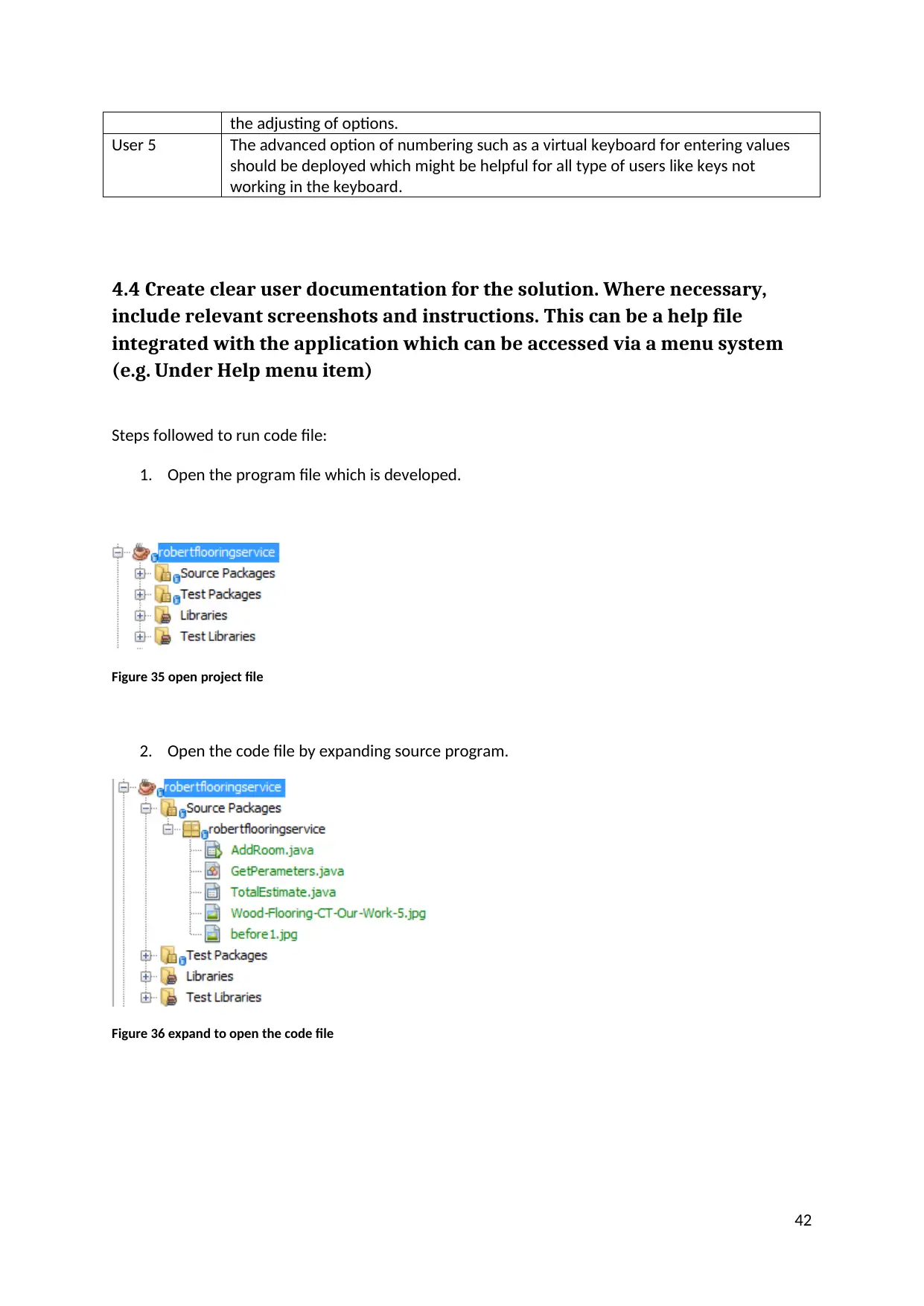
the adjusting of options.
User 5 The advanced option of numbering such as a virtual keyboard for entering values
should be deployed which might be helpful for all type of users like keys not
working in the keyboard.
4.4 Create clear user documentation for the solution. Where necessary,
include relevant screenshots and instructions. This can be a help file
integrated with the application which can be accessed via a menu system
(e.g. Under Help menu item)
Steps followed to run code file:
1. Open the program file which is developed.
Figure 35 open project file
2. Open the code file by expanding source program.
Figure 36 expand to open the code file
42
User 5 The advanced option of numbering such as a virtual keyboard for entering values
should be deployed which might be helpful for all type of users like keys not
working in the keyboard.
4.4 Create clear user documentation for the solution. Where necessary,
include relevant screenshots and instructions. This can be a help file
integrated with the application which can be accessed via a menu system
(e.g. Under Help menu item)
Steps followed to run code file:
1. Open the program file which is developed.
Figure 35 open project file
2. Open the code file by expanding source program.
Figure 36 expand to open the code file
42
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
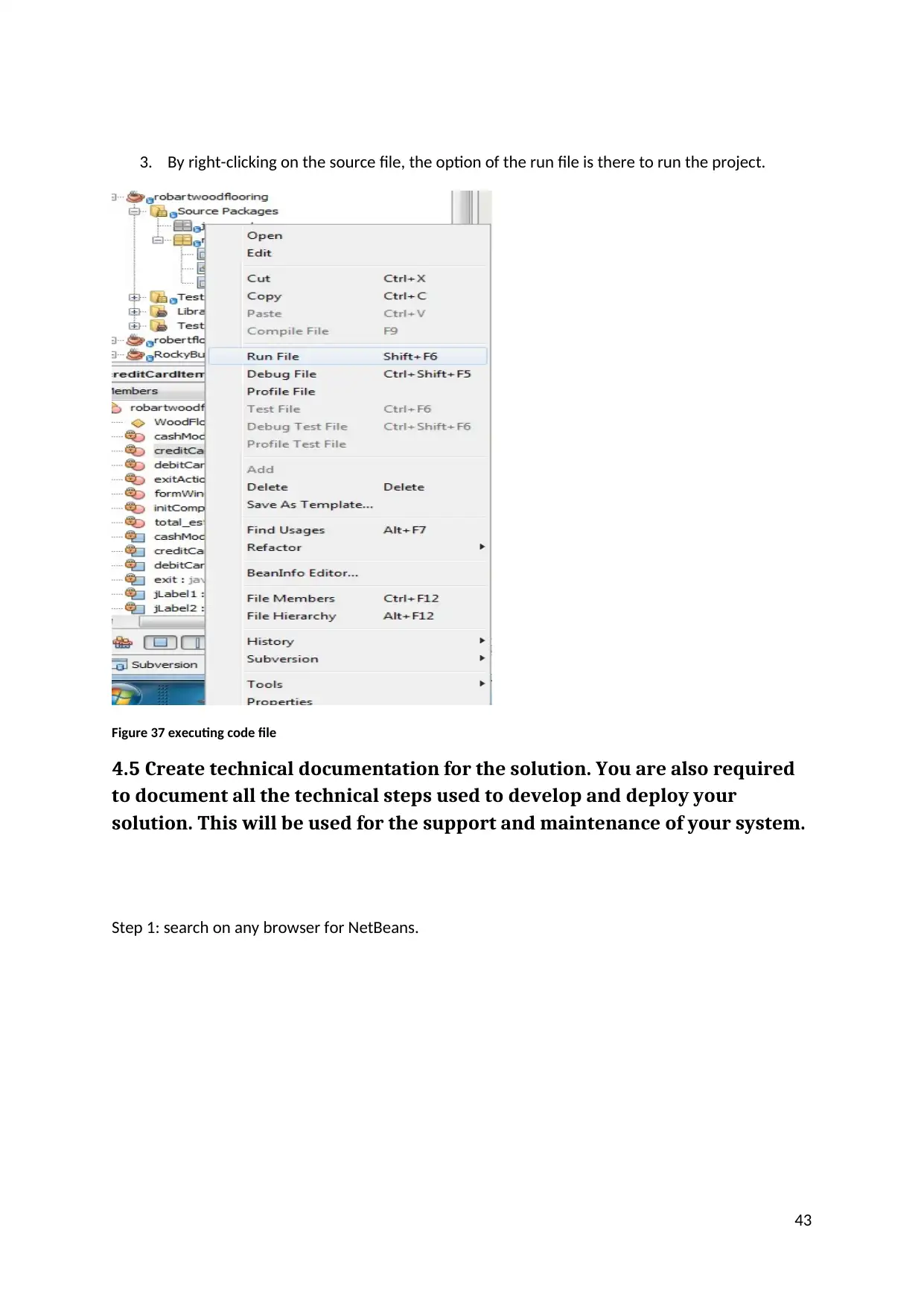
3. By right-clicking on the source file, the option of the run file is there to run the project.
Figure 37 executing code file
4.5 Create technical documentation for the solution. You are also required
to document all the technical steps used to develop and deploy your
solution. This will be used for the support and maintenance of your system.
Step 1: search on any browser for NetBeans.
43
Figure 37 executing code file
4.5 Create technical documentation for the solution. You are also required
to document all the technical steps used to develop and deploy your
solution. This will be used for the support and maintenance of your system.
Step 1: search on any browser for NetBeans.
43
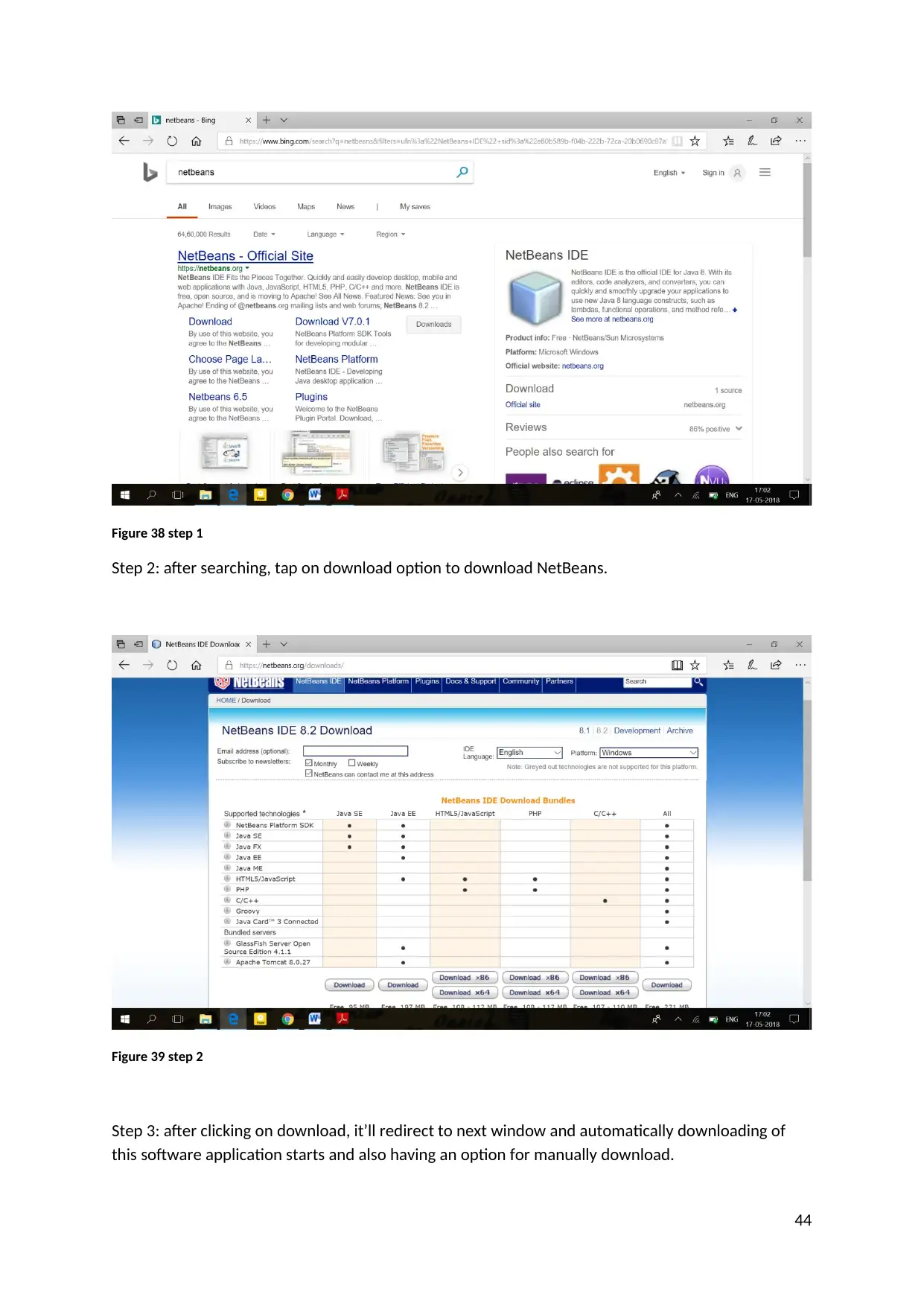
Figure 38 step 1
Step 2: after searching, tap on download option to download NetBeans.
Figure 39 step 2
Step 3: after clicking on download, it’ll redirect to next window and automatically downloading of
this software application starts and also having an option for manually download.
44
Step 2: after searching, tap on download option to download NetBeans.
Figure 39 step 2
Step 3: after clicking on download, it’ll redirect to next window and automatically downloading of
this software application starts and also having an option for manually download.
44
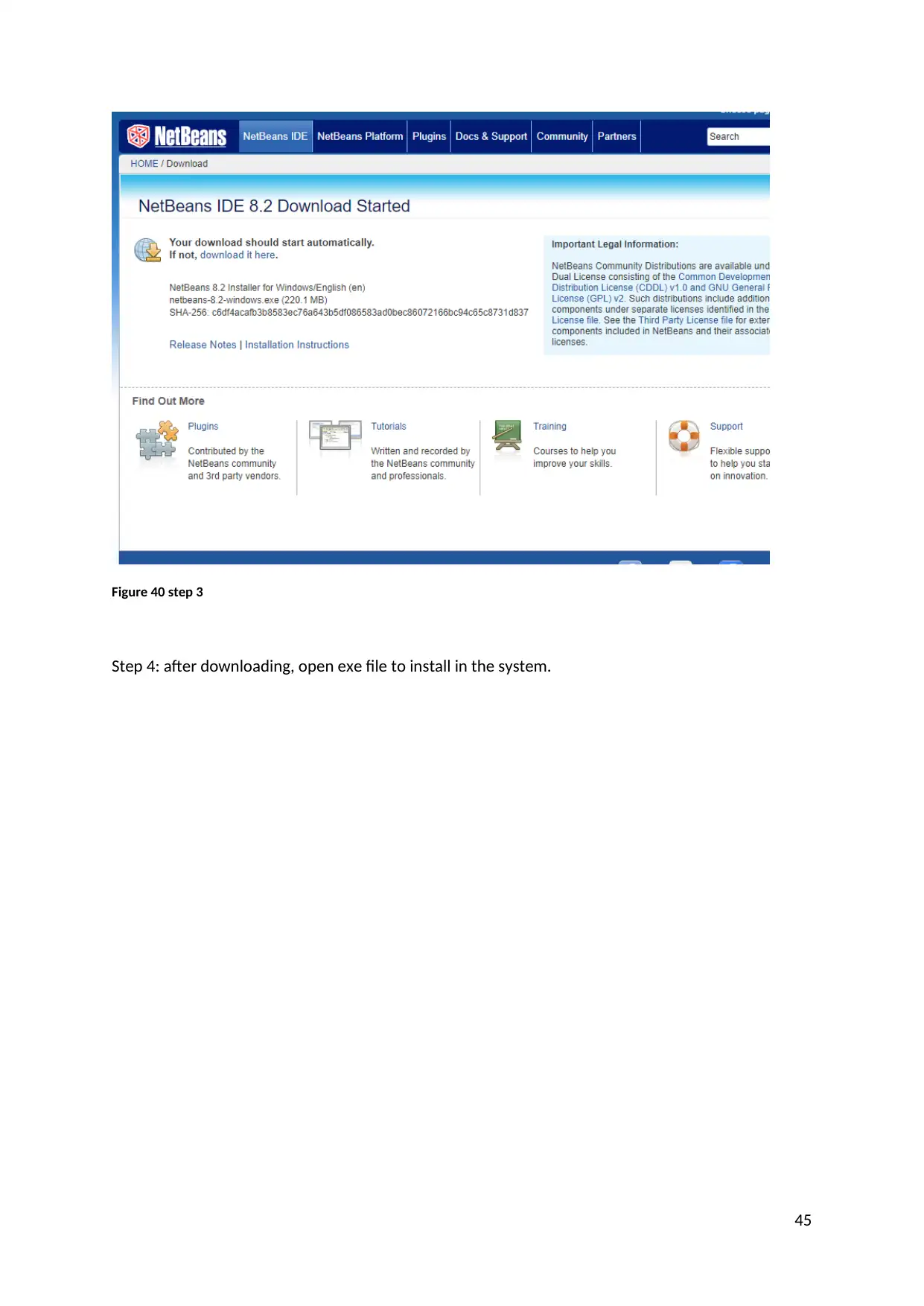
Figure 40 step 3
Step 4: after downloading, open exe file to install in the system.
45
Step 4: after downloading, open exe file to install in the system.
45
Secure Best Marks with AI Grader
Need help grading? Try our AI Grader for instant feedback on your assignments.
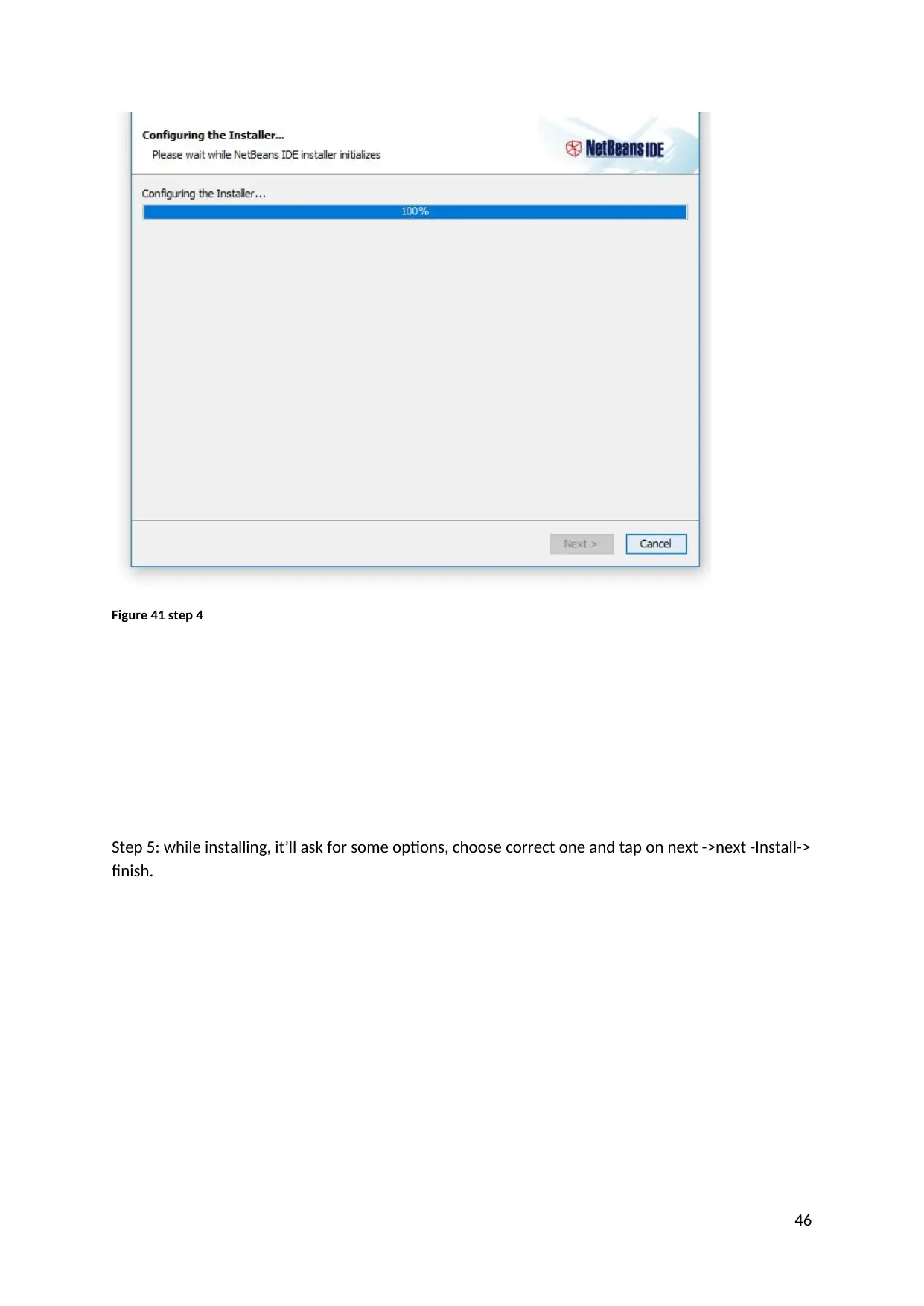
Figure 41 step 4
Step 5: while installing, it’ll ask for some options, choose correct one and tap on next ->next -Install->
finish.
46
Step 5: while installing, it’ll ask for some options, choose correct one and tap on next ->next -Install->
finish.
46
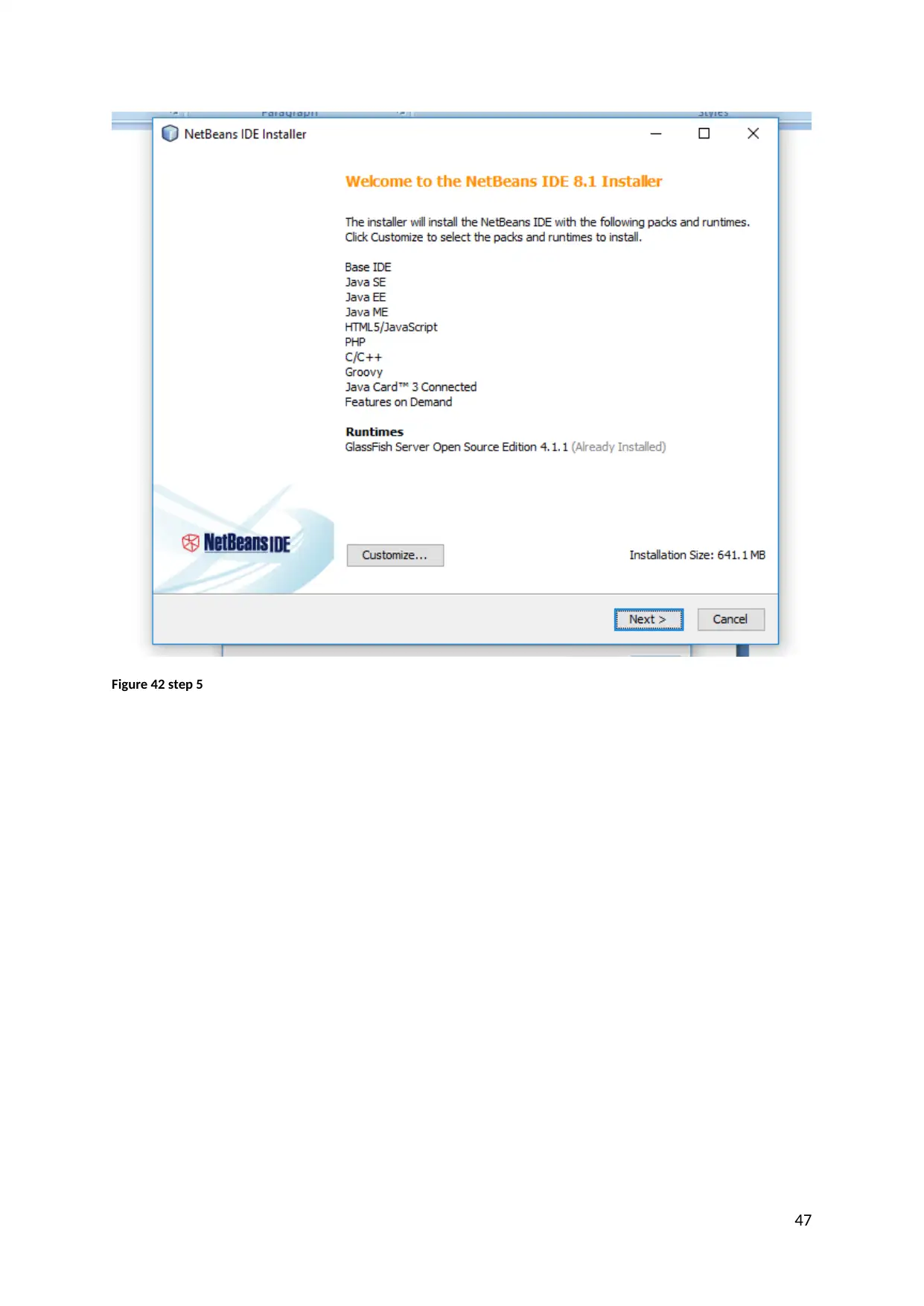
Figure 42 step 5
47
47
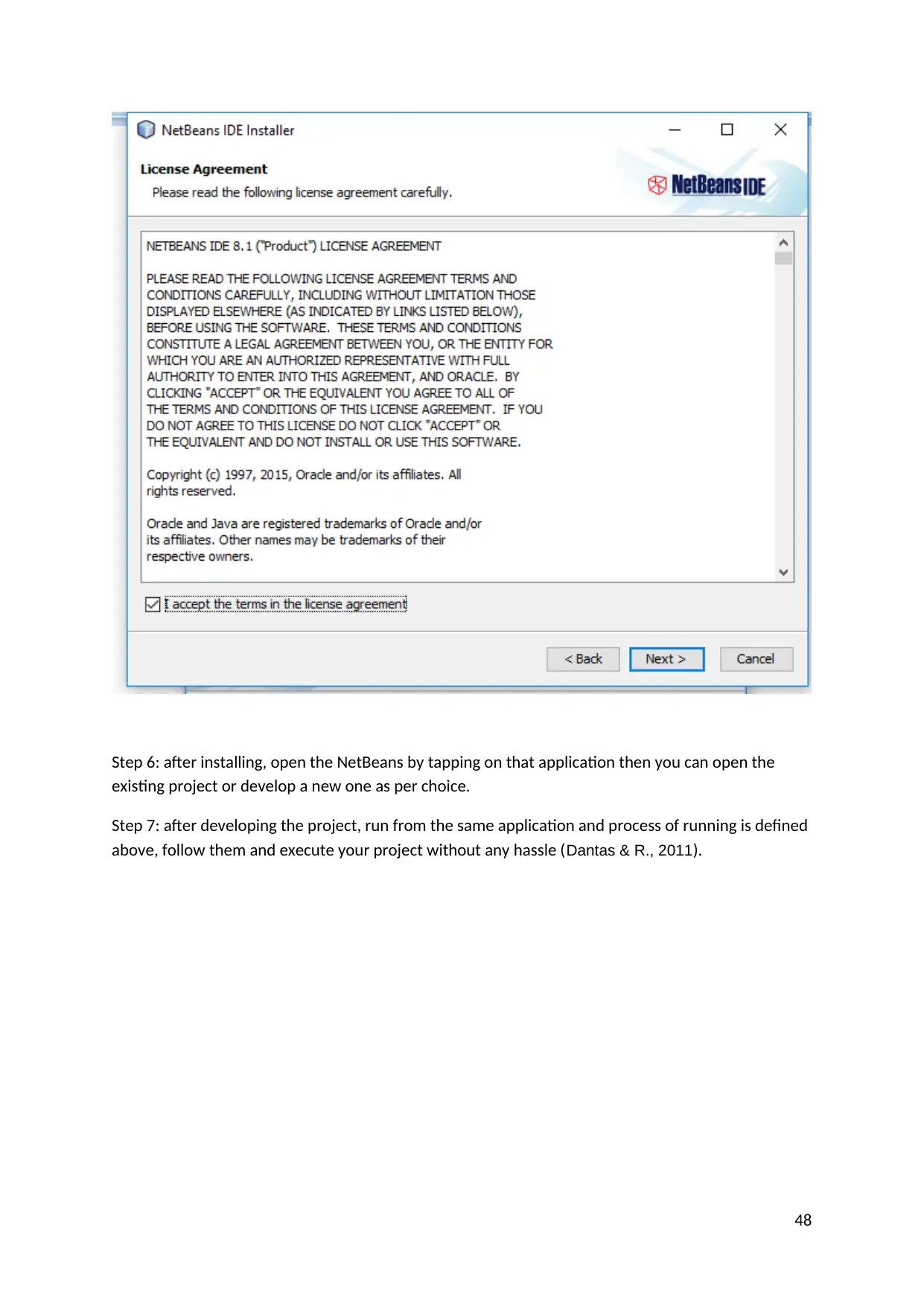
Step 6: after installing, open the NetBeans by tapping on that application then you can open the
existing project or develop a new one as per choice.
Step 7: after developing the project, run from the same application and process of running is defined
above, follow them and execute your project without any hassle (Dantas & R., 2011).
48
existing project or develop a new one as per choice.
Step 7: after developing the project, run from the same application and process of running is defined
above, follow them and execute your project without any hassle (Dantas & R., 2011).
48
Paraphrase This Document
Need a fresh take? Get an instant paraphrase of this document with our AI Paraphraser
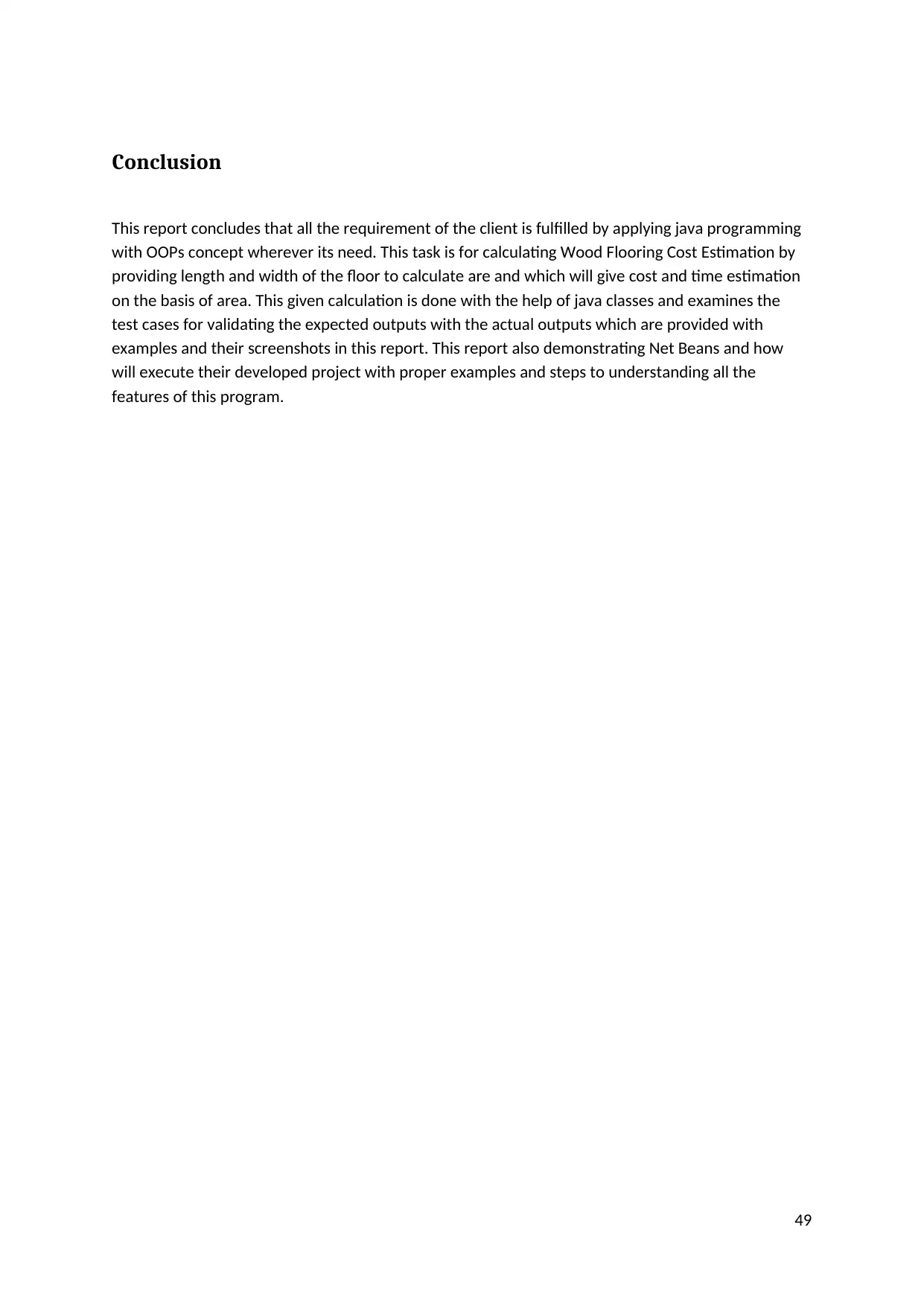
Conclusion
This report concludes that all the requirement of the client is fulfilled by applying java programming
with OOPs concept wherever its need. This task is for calculating Wood Flooring Cost Estimation by
providing length and width of the floor to calculate are and which will give cost and time estimation
on the basis of area. This given calculation is done with the help of java classes and examines the
test cases for validating the expected outputs with the actual outputs which are provided with
examples and their screenshots in this report. This report also demonstrating Net Beans and how
will execute their developed project with proper examples and steps to understanding all the
features of this program.
49
This report concludes that all the requirement of the client is fulfilled by applying java programming
with OOPs concept wherever its need. This task is for calculating Wood Flooring Cost Estimation by
providing length and width of the floor to calculate are and which will give cost and time estimation
on the basis of area. This given calculation is done with the help of java classes and examines the
test cases for validating the expected outputs with the actual outputs which are provided with
examples and their screenshots in this report. This report also demonstrating Net Beans and how
will execute their developed project with proper examples and steps to understanding all the
features of this program.
49
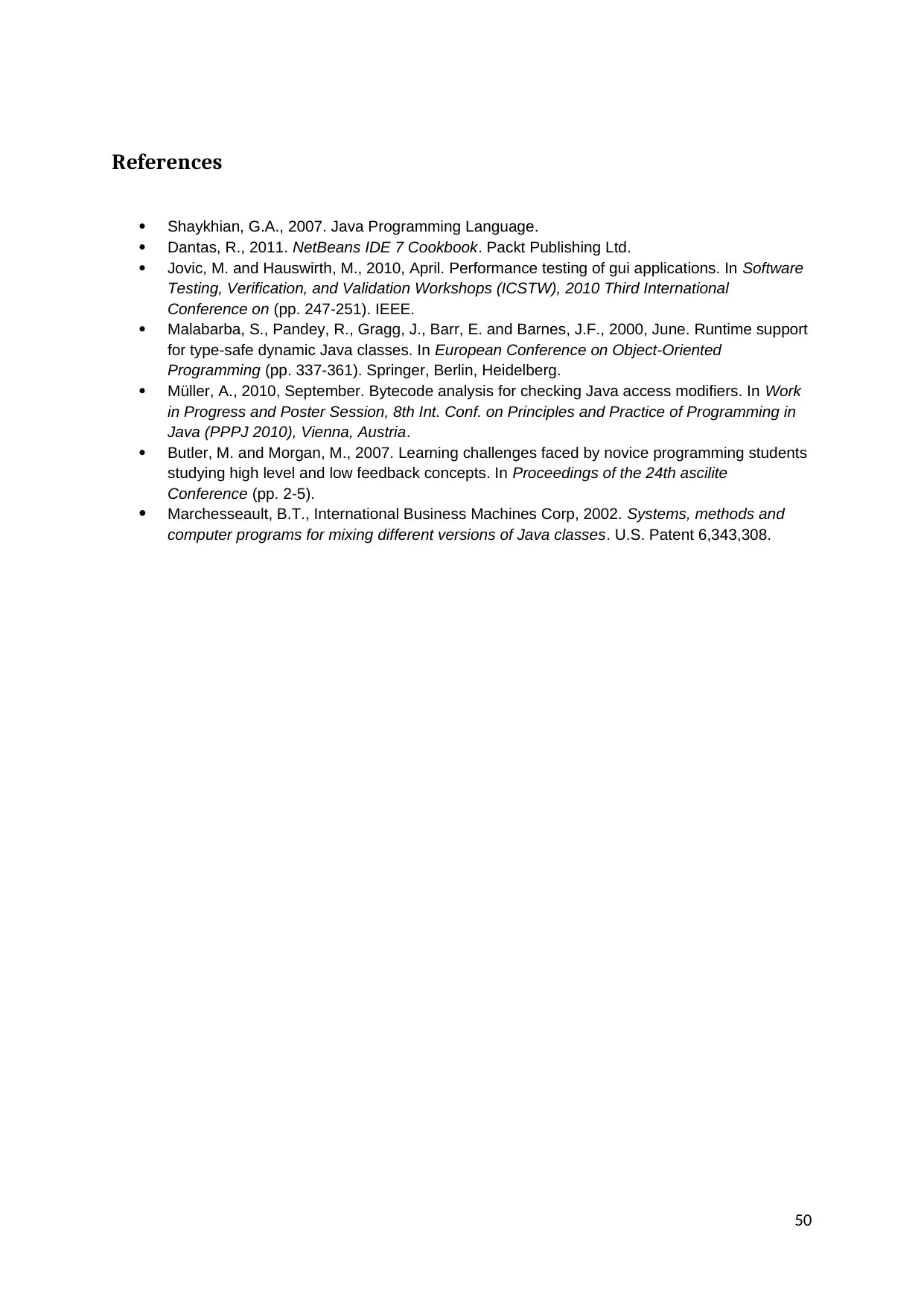
References
Shaykhian, G.A., 2007. Java Programming Language.
Dantas, R., 2011. NetBeans IDE 7 Cookbook. Packt Publishing Ltd.
Jovic, M. and Hauswirth, M., 2010, April. Performance testing of gui applications. In Software
Testing, Verification, and Validation Workshops (ICSTW), 2010 Third International
Conference on (pp. 247-251). IEEE.
Malabarba, S., Pandey, R., Gragg, J., Barr, E. and Barnes, J.F., 2000, June. Runtime support
for type-safe dynamic Java classes. In European Conference on Object-Oriented
Programming (pp. 337-361). Springer, Berlin, Heidelberg.
Müller, A., 2010, September. Bytecode analysis for checking Java access modifiers. In Work
in Progress and Poster Session, 8th Int. Conf. on Principles and Practice of Programming in
Java (PPPJ 2010), Vienna, Austria.
Butler, M. and Morgan, M., 2007. Learning challenges faced by novice programming students
studying high level and low feedback concepts. In Proceedings of the 24th ascilite
Conference (pp. 2-5).
Marchesseault, B.T., International Business Machines Corp, 2002. Systems, methods and
computer programs for mixing different versions of Java classes. U.S. Patent 6,343,308.
50
Shaykhian, G.A., 2007. Java Programming Language.
Dantas, R., 2011. NetBeans IDE 7 Cookbook. Packt Publishing Ltd.
Jovic, M. and Hauswirth, M., 2010, April. Performance testing of gui applications. In Software
Testing, Verification, and Validation Workshops (ICSTW), 2010 Third International
Conference on (pp. 247-251). IEEE.
Malabarba, S., Pandey, R., Gragg, J., Barr, E. and Barnes, J.F., 2000, June. Runtime support
for type-safe dynamic Java classes. In European Conference on Object-Oriented
Programming (pp. 337-361). Springer, Berlin, Heidelberg.
Müller, A., 2010, September. Bytecode analysis for checking Java access modifiers. In Work
in Progress and Poster Session, 8th Int. Conf. on Principles and Practice of Programming in
Java (PPPJ 2010), Vienna, Austria.
Butler, M. and Morgan, M., 2007. Learning challenges faced by novice programming students
studying high level and low feedback concepts. In Proceedings of the 24th ascilite
Conference (pp. 2-5).
Marchesseault, B.T., International Business Machines Corp, 2002. Systems, methods and
computer programs for mixing different versions of Java classes. U.S. Patent 6,343,308.
50
1 out of 51
Related Documents
![[object Object]](/_next/image/?url=%2F_next%2Fstatic%2Fmedia%2Flogo.6d15ce61.png&w=640&q=75)
Your All-in-One AI-Powered Toolkit for Academic Success.
+13062052269
info@desklib.com
Available 24*7 on WhatsApp / Email
Unlock your academic potential
© 2024 | Zucol Services PVT LTD | All rights reserved.