Algorithm Theory and Programming Paradigms
Create a report on algorithms, programming paradigms, and coding examples for each paradigm.
31 Pages6478 Words184 Views
Added on 2023-05-28
About This Document
This report discusses algorithm theory and programming paradigms including procedural, object-oriented, and event-driven paradigms. It also covers the implementation of an algorithm using an IDE and the advantages of using an IDE. The report evaluates the relationship between written algorithm and the code variant.
Algorithm Theory and Programming Paradigms
Create a report on algorithms, programming paradigms, and coding examples for each paradigm.
Added on 2023-05-28
ShareRelated Documents
PROGRAMMING AND ALGORITHM
REPORT
STUDENT NAME:
STUDENT ID:
1
REPORT
STUDENT NAME:
STUDENT ID:
1
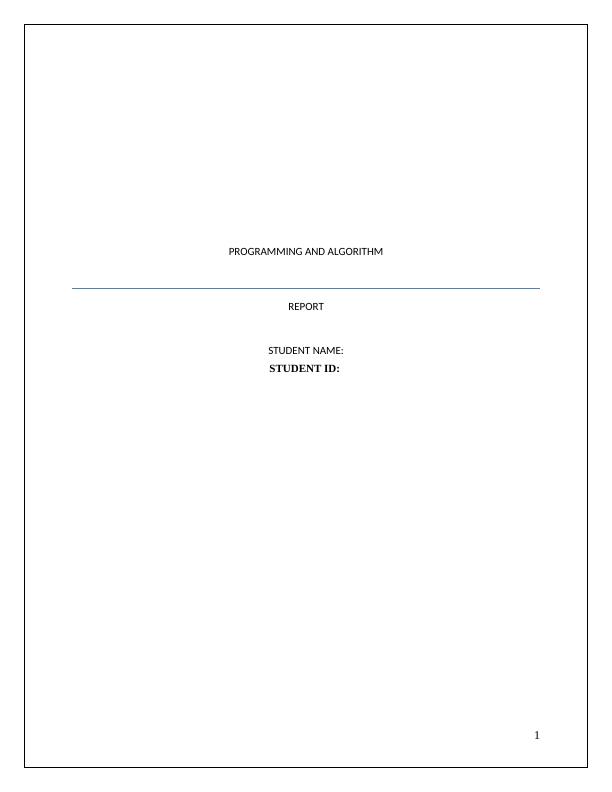
Table of Contents
Algorithm Theory and the process of programming an application................................................3
P1: Definition of Algorithm and Building process of an Application.........................................3
M1: Steps for writing a Code for Execution................................................................................4
D1: Implementation of an algorithm in a suitable language evaluate the relationship between
written algorithm and the code variant........................................................................................4
Programming Paradigms.................................................................................................................6
P2. Discussion of Procedural, Object Oriented and Event Driven Paradigms with their
Characteristics..............................................................................................................................6
Comparison among Procedural, Object Oriented, Even Driven:...............................................12
Implementation..............................................................................................................................14
P3: Program that implements an algorithm using an IDE.........................................................14
Advantages using IDE:..............................................................................................................15
M2. Common features that a developer has access to in an IDE...............................................18
M3: Use the IDE to manage the development process of the program.....................................18
D4: Use of an IDE for development of applications contrasted with not using an IDE............20
Report............................................................................................................................................26
P4. Debugging process:..............................................................................................................26
P5. Coding standard...................................................................................................................27
M4. Evaluate how the debugging process can be used to help develop more secure, robust
applications................................................................................................................................29
D4. Necessity of Coding Standard.................................................................................................29
References:....................................................................................................................................30
2
Algorithm Theory and the process of programming an application................................................3
P1: Definition of Algorithm and Building process of an Application.........................................3
M1: Steps for writing a Code for Execution................................................................................4
D1: Implementation of an algorithm in a suitable language evaluate the relationship between
written algorithm and the code variant........................................................................................4
Programming Paradigms.................................................................................................................6
P2. Discussion of Procedural, Object Oriented and Event Driven Paradigms with their
Characteristics..............................................................................................................................6
Comparison among Procedural, Object Oriented, Even Driven:...............................................12
Implementation..............................................................................................................................14
P3: Program that implements an algorithm using an IDE.........................................................14
Advantages using IDE:..............................................................................................................15
M2. Common features that a developer has access to in an IDE...............................................18
M3: Use the IDE to manage the development process of the program.....................................18
D4: Use of an IDE for development of applications contrasted with not using an IDE............20
Report............................................................................................................................................26
P4. Debugging process:..............................................................................................................26
P5. Coding standard...................................................................................................................27
M4. Evaluate how the debugging process can be used to help develop more secure, robust
applications................................................................................................................................29
D4. Necessity of Coding Standard.................................................................................................29
References:....................................................................................................................................30
2
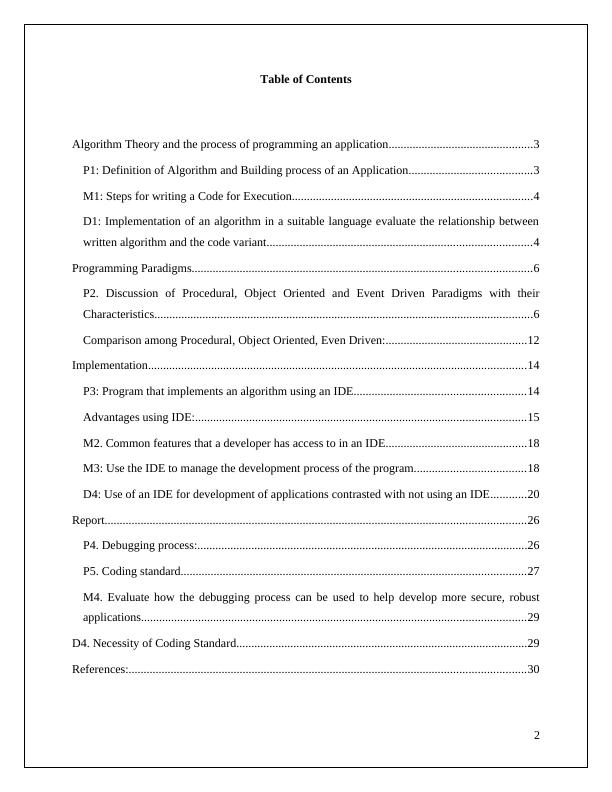
Algorithm Theory and the process of programming an application
P1: Definition of Algorithm and Building process of an Application
Definition:Algorithm is a set of instruction which is written sequentially to write a code for
solving of a problem.
There are some distinct features of algorithm (Brunsch et al. 2013, p.185)
Precise declaration and process to input and output.
Algorithmic steps must be clear enough to understand as they are essentially required to
build a good and systematic code
It should include a clear and dedicated ways to understand and solve the problem
capacity.
Algorithm should be written in language and not include any source code. Algorithm
actually describes the instruction and guide to make the source code. So it should be clear
and hyper enough so that the programmer can understand to way and objective to build
the code.
It should be written in stepwise by labeling them with step number sequentially.
When we are coding some objectives, some computations are necessary. In algorithm, the
computation process should be declared precisely.
Algorithm writing should be started with “START” and ends with “STOP”.
Building process: While building or creating an application, the work must start with writing the
process that is the algorithm. There should be a distinct overview which will provide the building
process of an application. The following steps are included like (Sanghavi et al. 2009, p. 4825)
Start of Algorithm: It is the starting point of an algorithm. It will decide how the program
will be started and how it will be preceded. Depending upon this, the programmer will
have to decide for their coding types for creating application.
Structure of Algorithm: Here is another important part is the structure. For particular
point of view and particular objective, there may be different methods or algorithms are
3
P1: Definition of Algorithm and Building process of an Application
Definition:Algorithm is a set of instruction which is written sequentially to write a code for
solving of a problem.
There are some distinct features of algorithm (Brunsch et al. 2013, p.185)
Precise declaration and process to input and output.
Algorithmic steps must be clear enough to understand as they are essentially required to
build a good and systematic code
It should include a clear and dedicated ways to understand and solve the problem
capacity.
Algorithm should be written in language and not include any source code. Algorithm
actually describes the instruction and guide to make the source code. So it should be clear
and hyper enough so that the programmer can understand to way and objective to build
the code.
It should be written in stepwise by labeling them with step number sequentially.
When we are coding some objectives, some computations are necessary. In algorithm, the
computation process should be declared precisely.
Algorithm writing should be started with “START” and ends with “STOP”.
Building process: While building or creating an application, the work must start with writing the
process that is the algorithm. There should be a distinct overview which will provide the building
process of an application. The following steps are included like (Sanghavi et al. 2009, p. 4825)
Start of Algorithm: It is the starting point of an algorithm. It will decide how the program
will be started and how it will be preceded. Depending upon this, the programmer will
have to decide for their coding types for creating application.
Structure of Algorithm: Here is another important part is the structure. For particular
point of view and particular objective, there may be different methods or algorithms are
3
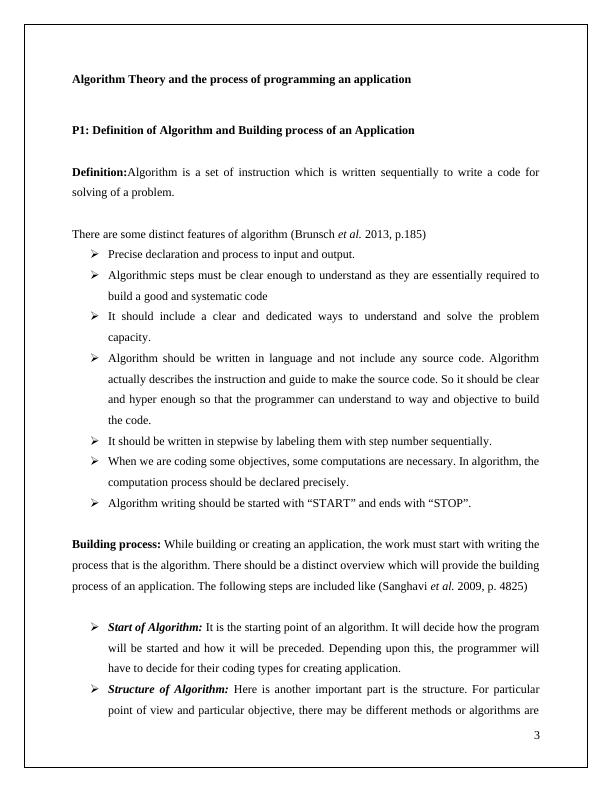
available. For example, there are different methods of sorting an array like bubble sort,
merge sort etc. But which type of sorting algorithm will be used, that depends upon the
environment variables and programmer. So, depending upon that, a proper algorithm will
be built.
Computation: Once the structure is designed, the computation part must be sufficiently
clear and distinct so that depending upon such a good coding can be built up.
Climax: At the end of algorithm, all the variables should be properly executed so that no
error should be faced at the last moment.
M1: Steps for writing a Code for Execution
While writing the algorithm, we have to simulate this and finally execute. So there should be
connected steps behind the all. The steps are as follows:
Step-1: Write algorithm
Step-2: Prepare Flow Chart
Step-3: Test the flow chart with simulation
Step-4: Choose preferred language to write the code
Step-5: Write the code
Step-6: Compile the code
Step-7: Run the code.
D1: Implementation of an algorithm in a suitable language evaluates the relationship
between written algorithm and the code variant.
Let we discuss the steps for writing the code in suitable language to execution depending upon
algorithm. In this context, we are taking an example algorithm for the determination of prime
number
Algorithm:
4
merge sort etc. But which type of sorting algorithm will be used, that depends upon the
environment variables and programmer. So, depending upon that, a proper algorithm will
be built.
Computation: Once the structure is designed, the computation part must be sufficiently
clear and distinct so that depending upon such a good coding can be built up.
Climax: At the end of algorithm, all the variables should be properly executed so that no
error should be faced at the last moment.
M1: Steps for writing a Code for Execution
While writing the algorithm, we have to simulate this and finally execute. So there should be
connected steps behind the all. The steps are as follows:
Step-1: Write algorithm
Step-2: Prepare Flow Chart
Step-3: Test the flow chart with simulation
Step-4: Choose preferred language to write the code
Step-5: Write the code
Step-6: Compile the code
Step-7: Run the code.
D1: Implementation of an algorithm in a suitable language evaluates the relationship
between written algorithm and the code variant.
Let we discuss the steps for writing the code in suitable language to execution depending upon
algorithm. In this context, we are taking an example algorithm for the determination of prime
number
Algorithm:
4
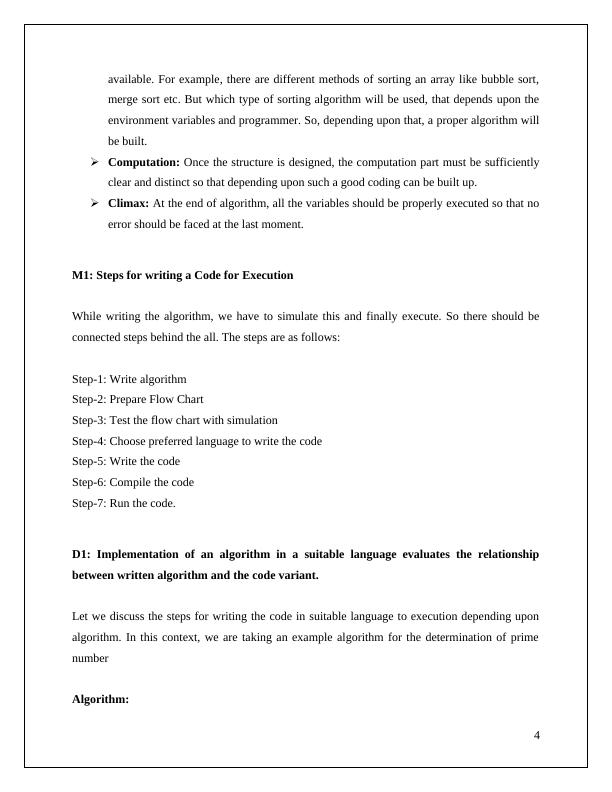
Step 1: Start the process
Step 2: Declare variables with name: i,n,flag.
Step 3: Initialize those variables
flag←1
i←2
Step 4: Read the value of ‘n’ from use for checking the Prime number.
Step 5: Repeat the steps until i<(n/2)
If remainder of n÷i equals 0
flag←0
Go to step 6
i←i+1
Step 6: If flag=0
Display n is not prime
else
Display n is prime
Step 7: Stop Process
Corresponding Program(in Java):
After successful writing the algorithm, we have to write the code stepwise as declared in the
algorithmic part.
1.class PrimeCheck{
2. public static void main(String args[]){
3. int i,m=0,flag=0;
4. Scanner sc=new Scanner(System.in);
5. n=sc.nextInt();
6. m=n/2;
7. if(n==0||n==1){
8. System.out.println(n+" is not prime number");
9. }
5
Step 2: Declare variables with name: i,n,flag.
Step 3: Initialize those variables
flag←1
i←2
Step 4: Read the value of ‘n’ from use for checking the Prime number.
Step 5: Repeat the steps until i<(n/2)
If remainder of n÷i equals 0
flag←0
Go to step 6
i←i+1
Step 6: If flag=0
Display n is not prime
else
Display n is prime
Step 7: Stop Process
Corresponding Program(in Java):
After successful writing the algorithm, we have to write the code stepwise as declared in the
algorithmic part.
1.class PrimeCheck{
2. public static void main(String args[]){
3. int i,m=0,flag=0;
4. Scanner sc=new Scanner(System.in);
5. n=sc.nextInt();
6. m=n/2;
7. if(n==0||n==1){
8. System.out.println(n+" is not prime number");
9. }
5
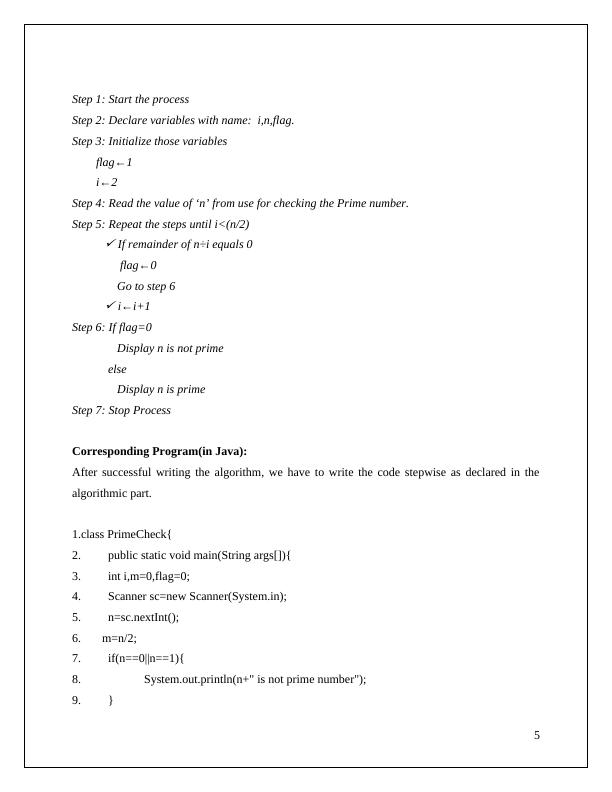
10. else{
11. for(i=2;i<=m;i++){
12. if(n%i==0){
13. System.out.println(n+" is not prime number");
14. flag=1;
15. break;
16. }
17. }
18. if(flag==0)
19. {
20. System.out.println(n+" is prime number"); }
21. }
22. }
23. }
So, in this coding, the correspondence of algorithmic steps are:
Line 1-6: Declaration & Initialization of variables
Line 7-17: Checking (Step-5)
Line 18-21: Decision for being a prime number
Line 22-23: End of Code
Programming Paradigms
P2. Discussion of Procedural, Object Oriented and Event Driven Paradigms with their
Characteristics
6
11. for(i=2;i<=m;i++){
12. if(n%i==0){
13. System.out.println(n+" is not prime number");
14. flag=1;
15. break;
16. }
17. }
18. if(flag==0)
19. {
20. System.out.println(n+" is prime number"); }
21. }
22. }
23. }
So, in this coding, the correspondence of algorithmic steps are:
Line 1-6: Declaration & Initialization of variables
Line 7-17: Checking (Step-5)
Line 18-21: Decision for being a prime number
Line 22-23: End of Code
Programming Paradigms
P2. Discussion of Procedural, Object Oriented and Event Driven Paradigms with their
Characteristics
6
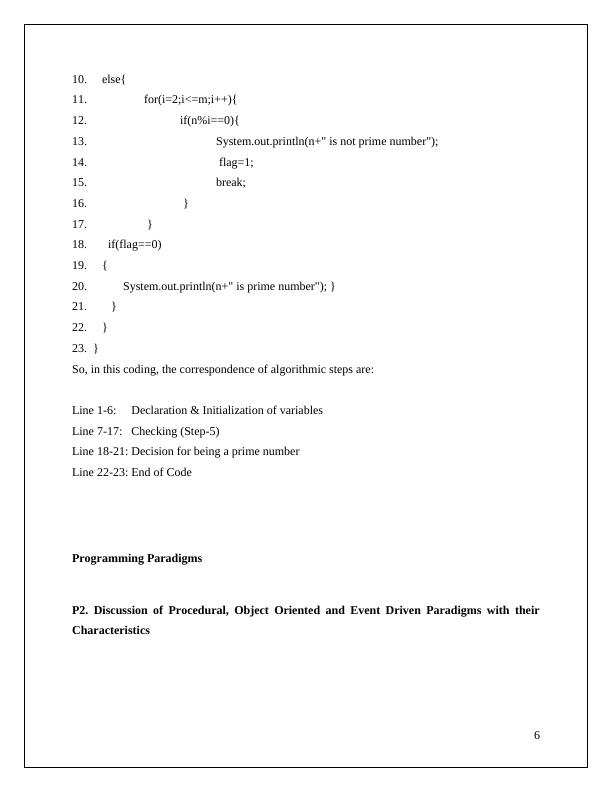
Program paradigm simply implies the way to perform a work to maintain a sequential and
concrete way to write code. This is not a language rather it is thinking and understanding how to
write a good program. Some well-known programming paradigms are:
Procedural:It deals with procedural programming with no objects.
Object-Oriented:It deals with object orientation knowledge that is based on object and classes
with the concept of interconnecting them. Object orientation can be:
Class-based: Objects are defined on basis of a class.
Prototype-based: Objects get acquires behavior from prototype object.
Event-Driven:It deals with emitters and listeners of defined or arbitrary actions.
Structured:It deals with the control structure defined by programmer.
Imperative:It deals with an explicit sequence of commands which updates the state of art.
Declarative:It deals with the declaration of functions and methods.
Functional: It deals with programming by avoiding global sets.
Flow-Driven:It deals with programs with the communication of internally or explicitly by
channels.
Logical:It deals with programming by obeying set of rules.
Procedural:
This is top-down approach or linear approach which depends upon the list of function used for it.
The attributes and flow control are as follows:
Attribute:
Variables
Function
Function calls
No concept for object creation
Modules can be used for importing some external function
Packages can be made for ease of programming to use different function
7
concrete way to write code. This is not a language rather it is thinking and understanding how to
write a good program. Some well-known programming paradigms are:
Procedural:It deals with procedural programming with no objects.
Object-Oriented:It deals with object orientation knowledge that is based on object and classes
with the concept of interconnecting them. Object orientation can be:
Class-based: Objects are defined on basis of a class.
Prototype-based: Objects get acquires behavior from prototype object.
Event-Driven:It deals with emitters and listeners of defined or arbitrary actions.
Structured:It deals with the control structure defined by programmer.
Imperative:It deals with an explicit sequence of commands which updates the state of art.
Declarative:It deals with the declaration of functions and methods.
Functional: It deals with programming by avoiding global sets.
Flow-Driven:It deals with programs with the communication of internally or explicitly by
channels.
Logical:It deals with programming by obeying set of rules.
Procedural:
This is top-down approach or linear approach which depends upon the list of function used for it.
The attributes and flow control are as follows:
Attribute:
Variables
Function
Function calls
No concept for object creation
Modules can be used for importing some external function
Packages can be made for ease of programming to use different function
7
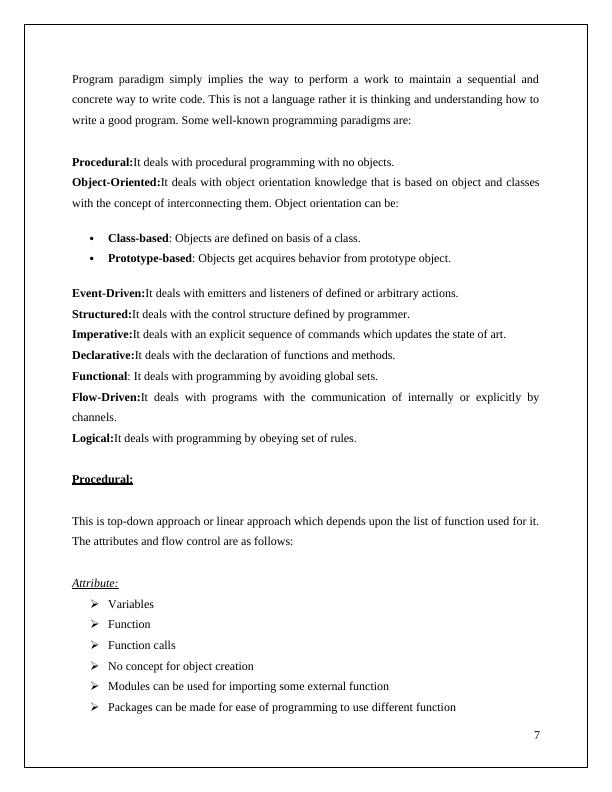
Computations are straight forward and defined in function.
Flow Control:
8
Start
Show Output & Result
Design Main Function
Route data and values for calculation
throughout the function
Declare Computation
Interconnect the Functions through Main
Build required functions
Declare variables and initialize
Import Packages and Modules
Start
Flow Control:
8
Start
Show Output & Result
Design Main Function
Route data and values for calculation
throughout the function
Declare Computation
Interconnect the Functions through Main
Build required functions
Declare variables and initialize
Import Packages and Modules
Start
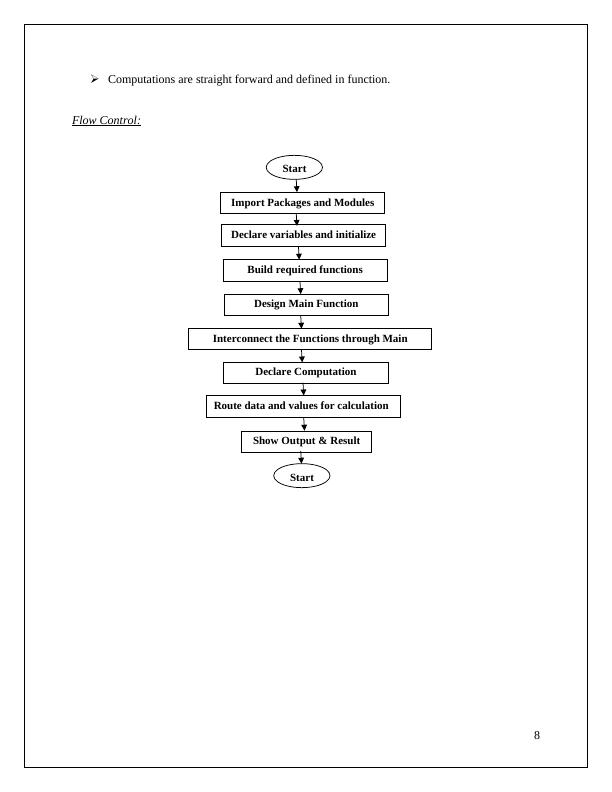
End of preview
Want to access all the pages? Upload your documents or become a member.
Related Documents
Algorithm and Flow Chart - Doclg...
|27
|5544
|268
Definition of Algorithm and Process of Building an Applicationlg...
|8
|1804
|83
Unit 1: Programming Submissionlg...
|58
|8377
|153
Assignment on Algorithms And Programminglg...
|27
|4937
|106
Case Study: Wage Commission Application Developmentlg...
|28
|5209
|493
Diploma in Computing Assignment PDFlg...
|29
|2639
|216